Ai Virtual Assistant Using Python Project Report
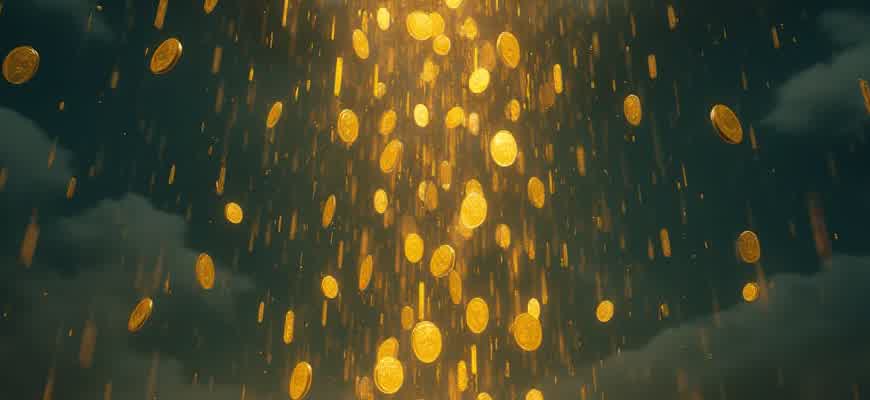
The goal of this project is to create an intelligent virtual assistant capable of performing a variety of tasks, such as voice-based interaction, information processing, and task automation. Python, with its extensive libraries and simplicity, serves as the foundation for developing a responsive and adaptive assistant. The system leverages speech recognition, natural language processing, and machine learning techniques to continuously improve its performance.
The primary goals of this project include:
- Building an interactive interface for the assistant that can recognize and respond to voice commands.
- Integrating advanced NLP algorithms for accurate interpretation of user queries.
- Employing machine learning models to refine the assistant’s capabilities based on user interactions.
Technologies used in the development process:
Technology | Description |
---|---|
SpeechRecognition | A library to convert speech to text, enabling voice command interpretation. |
pyttsx3 | Text-to-speech engine, providing verbal responses from the assistant. |
NLTK | A toolkit for processing and analyzing human language data to enable better query understanding. |
TensorFlow | A machine learning framework used to improve the assistant’s performance through data analysis. |
"By incorporating machine learning, the assistant becomes more efficient over time, adapting to user preferences."
How to Set Up a Python Environment for Building an AI Virtual Assistant
Before starting the development of an AI virtual assistant using Python, it is essential to properly set up your Python environment. This ensures compatibility with the libraries and tools you will need for machine learning, natural language processing, and speech recognition tasks. A clean and well-organized environment will streamline the entire development process, minimizing conflicts between dependencies and providing the flexibility to test different versions of packages. In this guide, we will walk through the necessary steps to set up a Python environment suited for building an AI-powered virtual assistant.
The process involves several key steps, including installing Python, setting up a virtual environment, and installing the required libraries. The virtual environment is crucial because it isolates your project dependencies from the global Python environment, reducing the risk of version conflicts and making your project easier to manage.
Step-by-Step Guide to Set Up Your Python Environment
- Install Python: Ensure that Python is installed on your system. You can download the latest version from the official Python website. It is recommended to install Python 3.8 or higher for compatibility with most AI libraries.
- Create a Virtual Environment: After installing Python, create a virtual environment using the following command:
python -m venv myenv
This will create a directory named 'myenv' where all project dependencies will be stored. - Activate the Virtual Environment: Activate the virtual environment with the following command:
source myenv/bin/activate
On Windows, use:
myenv\Scripts\activate
- Install Dependencies: With the virtual environment activated, you can now install the necessary libraries. Some commonly used libraries for AI virtual assistants include:
- SpeechRecognition - for speech-to-text capabilities
- pyttsx3 - for text-to-speech functionality
- nltk - for natural language processing
- pyaudio - for microphone input
Install them by running the following:
pip install SpeechRecognition pyttsx3 nltk pyaudio
Important Information
Remember to deactivate the virtual environment once you are done with the setup by running the command:
deactivateThis will return you to the global Python environment.
Example Setup Overview
Step | Command |
---|---|
Install Python | Download from python.org |
Create Virtual Environment | python -m venv myenv |
Activate Virtual Environment | source myenv/bin/activate |
Install Dependencies | pip install SpeechRecognition pyttsx3 nltk pyaudio |
Choosing the Right Libraries for Natural Language Processing in Python
When building a virtual assistant using Python, selecting the appropriate libraries for Natural Language Processing (NLP) is crucial for achieving efficient and accurate results. Python offers a variety of libraries designed to handle different aspects of NLP, ranging from text preprocessing to sentiment analysis and text generation. The choice of libraries directly impacts the performance and scalability of the assistant, making it essential to understand the strengths and limitations of each option.
Several factors should be considered when choosing NLP libraries, such as ease of integration, community support, and specific features offered. Libraries like NLTK and SpaCy are among the most widely used, but there are also newer options like Hugging Face's Transformers that specialize in deep learning-based NLP tasks. The right choice often depends on the complexity of the project and the specific requirements for language understanding and generation.
Key Libraries for NLP in Python
- NLTK (Natural Language Toolkit): A comprehensive library that provides tools for text processing, classification, tokenization, and stemming. NLTK is ideal for educational purposes and prototyping.
- SpaCy: Known for its speed and efficiency, SpaCy is designed for industrial-strength NLP tasks. It excels in named entity recognition (NER), part-of-speech tagging, and dependency parsing.
- Transformers by Hugging Face: A library focused on state-of-the-art deep learning models for NLP, especially useful for tasks involving contextual understanding, like question answering and text generation.
Factors to Consider
- Speed and Performance: SpaCy is faster than NLTK, especially for large datasets and real-time applications.
- Complexity of Tasks: Hugging Face’s Transformers provide more advanced capabilities like transformer-based models but require more computational resources.
- Ease of Use: NLTK is user-friendly and well-suited for beginners, while SpaCy offers a cleaner and more efficient API for experienced developers.
Comparison of Popular NLP Libraries
Library | Strengths | Weaknesses |
---|---|---|
NLTK | Comprehensive, suitable for educational purposes and prototyping. | Slower for large-scale tasks, lacks advanced deep learning models. |
SpaCy | Fast, efficient, production-ready, with excellent support for NER and dependency parsing. | Limited support for deep learning models compared to Hugging Face. |
Transformers | State-of-the-art models for complex tasks, such as language generation and contextual understanding. | Requires significant computational resources and expertise to use effectively. |
When selecting a library, consider the scale of your project, available resources, and the specific NLP tasks you need to perform. The combination of libraries can also be a powerful approach, using each for its strengths.
Integrating Voice Recognition into Your Python AI Assistant
Incorporating speech recognition into a Python-based virtual assistant significantly enhances the user experience, allowing for hands-free interaction and seamless communication. Voice commands can trigger specific functions within the assistant, such as fetching information, controlling applications, or executing tasks like setting reminders or sending messages. The key to successful integration lies in selecting a suitable speech recognition library, configuring it properly, and handling the output efficiently.
Python offers several libraries for speech recognition, with the most commonly used being the SpeechRecognition library. This library interfaces with multiple engines, including Google Web Speech API, to transcribe spoken words into text. By using a microphone as input, the assistant captures real-time voice data, processes it, and performs the requested action. Below is an overview of the steps involved in integrating speech recognition:
Steps for Integrating Speech Recognition
- Install necessary libraries like SpeechRecognition and PyAudio for microphone input.
- Set up the microphone as the audio source and capture the user’s speech.
- Convert the recorded speech into text using the speech recognition engine.
- Process the text output and execute the corresponding command in the assistant.
The integration can also include additional features, such as voice feedback, where the assistant verbally responds to the user. Here’s a basic table summarizing common speech recognition libraries and their key features:
Library | Features | Supported Engines |
---|---|---|
SpeechRecognition | Transcribes speech to text, integrates with multiple engines. | Google Web Speech API, CMU Sphinx, etc. |
PyAudio | Facilitates microphone access and audio input. | Supports any compatible speech-to-text engine. |
Tip: It’s essential to handle different accents, background noise, and language support when using voice recognition in your AI assistant to ensure accurate results.
Designing a User-Friendly Interface for Voice Commands in Python
When building a voice-controlled application in Python, creating a user-friendly interface is essential for seamless interaction. The design should prioritize ease of use, clarity, and accessibility. A good voice interface needs to understand a wide variety of commands and respond quickly. This is especially important in Python, where several libraries, such as SpeechRecognition and pyttsx3, can be utilized to handle voice inputs and outputs effectively. The goal is to ensure that the user can communicate effortlessly without any technical barriers.
The development of a voice interface in Python can be broken down into several key components: voice command recognition, feedback mechanisms, and user guidance. By integrating natural language processing (NLP) and machine learning algorithms, the interface can be improved to handle more complex tasks. The design should also consider providing real-time responses and error handling to avoid frustration during usage.
Components for Designing the Interface
- Voice Command Recognition: Using libraries like SpeechRecognition and pyaudio to convert speech to text.
- Text-to-Speech Feedback: Utilizing pyttsx3 or similar libraries for audio feedback to the user.
- Error Handling: Providing feedback when the system fails to recognize or process commands.
- Natural Language Processing (NLP): Enhancing accuracy by interpreting and categorizing user requests.
Workflow Example
- User speaks a command.
- The system converts speech to text using a speech recognition library.
- The text is analyzed using NLP techniques to determine the user's intent.
- The system executes the task and provides voice feedback.
Key Considerations for a Smooth Experience
Consideration | Details |
---|---|
Clarity of Commands | Commands should be simple and intuitive, avoiding jargon or complex phrases. |
Voice Feedback | Immediate and clear audio feedback ensures the user knows their input was understood. |
Accessibility | The system should be usable by people with varying abilities, including those with hearing or speech impairments. |
"The key to designing a successful voice interface is creating an experience where the user feels they can communicate naturally, with minimal barriers."
Implementing Task Automation Features for Your Virtual Assistant
One of the primary goals when building a virtual assistant is to enhance productivity by automating common tasks. This can range from simple tasks like setting reminders to more complex processes such as managing emails or controlling smart home devices. Python, with its rich ecosystem of libraries and frameworks, offers numerous ways to implement automation effectively. To achieve this, leveraging tools such as schedule, pyautogui, and selenium can be incredibly useful in executing these features with minimal effort.
To begin automating tasks, it's crucial to break down each task into smaller, actionable steps. Identifying the most frequent or repetitive actions users perform will allow you to design features that directly address these needs. For example, automating the sending of emails or managing calendar events involves integrating APIs such as Google Calendar API and SMTP for email services. These integrations help the assistant perform tasks without requiring continuous user input.
Key Features for Task Automation
- Automated Scheduling: Automatically set reminders or calendar events based on user commands.
- Email Management: Send and organize emails with predefined templates.
- File System Automation: Move, rename, or organize files based on specified parameters.
- Web Automation: Automatically fill forms or extract information from websites using selenium.
Implementation Example
Let's take a look at how to automate sending emails with Python using SMTP:
import smtplib from email.mime.text import MIMEText from email.mime.multipart import MIMEMultipart def send_email(): sender_email = "[email protected]" receiver_email = "[email protected]" password = "your_password" message = MIMEMultipart() message['From'] = sender_email message['To'] = receiver_email message['Subject'] = 'Automated Email' body = 'This is an automatically sent email.' message.attach(MIMEText(body, 'plain')) with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() server.login(sender_email, password) server.sendmail(sender_email, receiver_email, message.as_string())
Task Automation in Action
The effectiveness of automation becomes apparent when users can execute complex actions with a single command. This minimizes the need for manual intervention, making day-to-day operations faster and more efficient.
By automating daily tasks, the virtual assistant not only saves time but also enhances user satisfaction by reducing the chances of human error.
Task Automation Table
Task | Python Library | Use Case |
---|---|---|
Scheduling | schedule | Set reminders or appointments automatically. |
Email Management | SMTP, email | Send and manage emails with predefined templates. |
Web Automation | selenium | Automate web form submissions or data extraction. |
Managing Data Storage and User Preferences for Personalized Assistance
To provide a tailored user experience, an AI virtual assistant must effectively handle both user data and preferences. The assistant's ability to remember user-specific information, such as previous interactions, preferences, and behavioral patterns, enhances its responsiveness and accuracy in delivering assistance. Efficient data storage and management are crucial to ensure that user information is both accessible and secure, while also enabling the assistant to learn from past engagements to improve future interactions.
One key aspect of personalizing assistance is the management of user preferences. This involves storing settings that allow the assistant to adjust its responses according to user expectations. Whether it's adapting the tone, language, or specific actions, these preferences must be easily updated and retrieved. In this context, various data storage solutions can be used, such as databases, cloud storage, or local files, depending on the scale and complexity of the system.
Storing and Managing User Preferences
The management of user preferences can be divided into several key tasks:
- Data Collection: Gathering preferences through user input or interactions.
- Data Storage: Using appropriate systems like databases or cloud services to securely store user data.
- Data Retrieval: Ensuring quick and efficient access to preferences when needed to personalize responses.
- Data Updates: Allowing users to update their preferences easily through a simple interface.
Depending on the type of data, the system might use structured storage (such as relational databases) for preferences that need to be queried or unstructured formats (like JSON or text files) for more flexible user settings.
Example of User Preference Storage
User ID | Preferred Language | Response Tone | Favorite Topics |
---|---|---|---|
001 | English | Formal | Technology, Health |
002 | Spanish | Casual | Sports, Music |
Note: It's crucial to ensure that personal data is handled securely, following data privacy regulations such as GDPR. The system should incorporate encryption and access controls to protect sensitive information.
Testing Your AI Assistant for Common User Interactions and Errors
In order to ensure that an AI assistant performs as expected, it is crucial to test it for a wide range of user inputs and errors. Common user interactions should be examined, including requests for basic information, complex queries, and task execution. Simulating real-world scenarios helps identify potential flaws and improve the assistant's performance. Furthermore, testing the system for error handling is essential to guarantee that the AI can gracefully manage unexpected situations and respond appropriately when it encounters difficulties.
During the testing phase, various types of user errors must also be taken into account. This includes handling unrecognized commands, invalid inputs, and ambiguous queries. By simulating these types of interactions, developers can improve the assistant's ability to deliver meaningful responses while minimizing user frustration. Moreover, testing ensures that the AI maintains accuracy, efficiency, and user-friendliness, even in cases of input errors.
Common User Interactions
- Requesting basic information, such as time, weather, or date
- Asking for specific actions, like setting reminders or sending messages
- Engaging in conversational dialogue, with follow-up questions or clarification
Common Errors and Handling Techniques
- Unrecognized commands: Ensure the assistant asks the user for clarification or provides a list of possible commands.
- Invalid inputs: The assistant should inform the user of the error and suggest alternatives.
- Ambiguous queries: The AI should request more specific information before proceeding.
Important: Effective error handling is critical for improving user satisfaction. Users are more likely to trust an assistant that provides clear feedback and guidance when something goes wrong.
Error Handling Table
Error Type | Expected Response | Action Taken |
---|---|---|
Unrecognized Command | Provide clarification or a list of available commands | Prompt the user for further input |
Invalid Input | Inform user of the mistake and suggest alternatives | Redirect the user to the correct input format |
Ambiguous Query | Request additional details | Seek clarification before continuing |
Optimizing Performance and Memory Usage for Scalable Virtual Assistant Projects
When building a scalable virtual assistant, performance and memory optimization are key to ensuring that the system can handle increased loads and maintain responsiveness. As virtual assistants often rely on various machine learning models and real-time processing, optimizing the resources they consume can have a significant impact on their efficiency and scalability. This can be achieved through effective memory management, data processing strategies, and minimizing the computational overhead for common tasks.
Effective optimization techniques not only improve performance but also ensure that the assistant remains efficient as it scales to support larger user bases and more complex tasks. To achieve this, developers must balance between algorithm efficiency, resource allocation, and responsiveness, while considering hardware limitations and software constraints. Below are key strategies that can be employed to optimize both performance and memory usage in a virtual assistant project.
Key Optimization Strategies
- Efficient Data Structures: Using lightweight and efficient data structures, such as dictionaries, arrays, and hashmaps, can significantly reduce memory consumption. These structures should be chosen based on the type and frequency of data they are meant to handle.
- Model Compression: Reducing the size of the machine learning models used by the virtual assistant through techniques like pruning or quantization helps decrease memory usage without sacrificing much in terms of accuracy.
- Batch Processing: Instead of processing data individually, batching similar requests together reduces the overhead caused by repetitive tasks and minimizes redundant resource consumption.
Memory Management Practices
- Lazy Loading: Load only the required components or modules of the assistant when needed, instead of preloading everything into memory. This reduces memory usage at any given time.
- Garbage Collection: Proper garbage collection ensures that unused objects are cleared from memory, preventing memory leaks and unnecessary consumption of resources.
- Asynchronous Processing: Implementing asynchronous programming models allows tasks to run in parallel, ensuring that the system doesn't wait for one task to finish before starting another, ultimately improving performance and reducing idle times.
Performance Metrics
Metric | Impact | Optimization Approach |
---|---|---|
CPU Usage | High CPU usage can cause slow performance and system overload. | Optimize algorithms, reduce unnecessary computations, and use multi-threading where applicable. |
Memory Usage | Excessive memory usage leads to slowdowns and crashes in large-scale applications. | Use memory-efficient data structures, and implement lazy loading and garbage collection. |
Response Time | Delays in processing requests negatively affect user experience. | Optimize model inference times and use caching techniques for frequent queries. |
Key Takeaway: By implementing these strategies, developers can build virtual assistants that are not only scalable but also efficient, ensuring high performance and minimal memory usage even as the system grows in complexity.