Text to Speech Api Swift
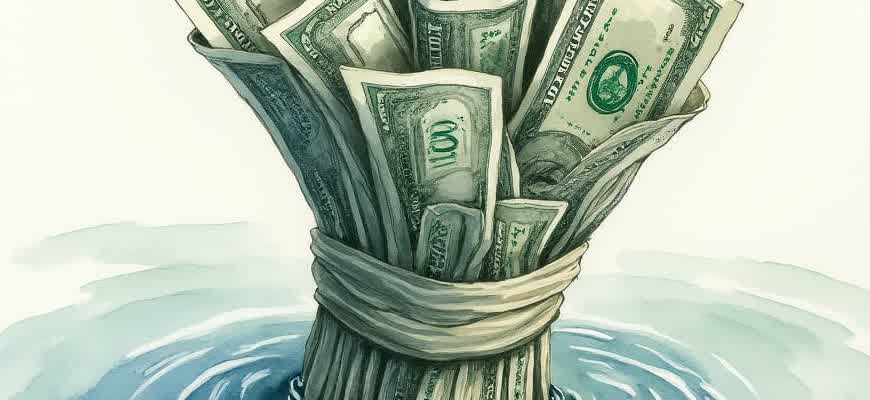
In modern application development, adding speech capabilities to apps can significantly enhance user experience. The Text-to-Speech (TTS) feature allows converting written text into spoken words, making apps more accessible and interactive. In Swift, the TTS functionality is powered by the AVFoundation framework, which provides tools to synthesize speech from text.
To begin implementing TTS, developers can utilize the AVSpeechSynthesizer class, which handles the conversion process. The key steps include initializing an instance of AVSpeechSynthesizer and configuring the voice properties, such as language and pitch. Below is a structured guide for implementing TTS in Swift:
- Import the AVFoundation framework.
- Initialize an AVSpeechSynthesizer instance.
- Create an AVSpeechUtterance with the desired text.
- Set the voice properties, including language, rate, and pitch.
- Invoke the speech synthesis process using the speak() method.
The following table illustrates the key components of TTS integration in Swift:
Component | Description |
---|---|
AVSpeechSynthesizer | Handles speech synthesis by processing text input into audible speech. |
AVSpeechUtterance | Represents the spoken text and allows customization of its properties, such as rate and pitch. |
AVSpeechSynthesisVoice | Defines the voice for speech synthesis, including language and regional accent. |
Note: It's essential to handle speech interruptions and ensure the app has the required permissions to use the device's microphone and speakers for speech synthesis.
Promoting the Benefits of Voice Synthesis API for Your Business
Integrating speech synthesis technology into your services can significantly enhance the accessibility and engagement of your business. The ability to convert written text into clear, natural speech opens up new opportunities for providing information to a broader audience. Whether it's for customer support, e-learning, or voice assistants, a robust API can streamline these processes with high-quality voice output.
By implementing a speech synthesis solution, you can provide more interactive and efficient services. Businesses can use such technologies to improve user experiences, drive better engagement, and optimize workflows. The right API can reduce costs and improve efficiency by automating many communication tasks that otherwise require manual effort.
Key Features and Advantages
- Multilingual Support: Reach a global audience with support for multiple languages and accents.
- Customizable Voice Options: Adjust voice characteristics such as pitch, speed, and tone.
- Seamless Integration: Easy integration with existing systems and platforms.
- Real-Time Processing: Instantaneous text-to-speech conversion for live interactions.
How to Promote and Maximize Its Potential
- Leverage Voice Assistants: Add speech capabilities to your customer service or product interfaces.
- Enhance Accessibility: Make your content more inclusive for users with disabilities by converting it to audio.
- Provide Personalized Experiences: Use custom voices to align with your brand’s identity and create a unique experience.
"Integrating a high-quality speech synthesis API not only enhances user experience but also boosts engagement by providing users with content in the most accessible format."
Considerations for Selecting a Solution
Feature | Importance | Consideration |
---|---|---|
Voice Quality | High | Ensure the generated speech sounds natural and clear to the listener. |
Speed & Latency | Medium | Check if the system provides real-time responses without significant delays. |
Customization Options | High | Ability to adjust pitch, tone, and language to match your brand's needs. |
Integrating Text-to-Speech API in Your Swift-based iOS App
To add text-to-speech functionality in your iOS application, leveraging a Text-to-Speech API in Swift is a powerful approach. This integration allows your app to read text aloud using natural-sounding voices. Various APIs are available to streamline the process, enabling customization for different languages, voices, and speech rates.
In this guide, we will explore the steps to integrate a popular Text-to-Speech API into your iOS project, along with practical code snippets to get you started quickly.
Steps to Set Up Text-to-Speech API
- Step 1: Install Required Dependencies
- Step 2: Set Up API Keys and Permissions
- Step 3: Implement API Calls
Begin by integrating the necessary libraries or SDKs. Many APIs offer SDKs that simplify the process of integration.
For most APIs, you will need to sign up for an account and obtain an API key. Ensure you also configure appropriate permissions in your app to access the microphone or speaker, if necessary.
Once your setup is complete, make API calls to send text and retrieve speech output. This often involves creating a speech request with the desired settings (language, voice type, etc.) and handling the resulting audio response.
Note: Always handle API responses and errors gracefully to improve user experience. Ensure the app works smoothly, even if the speech service is temporarily unavailable.
Code Example for Integration
import AVFoundation let synthesizer = AVSpeechSynthesizer() func speakText(text: String) { let utterance = AVSpeechUtterance(string: text) utterance.voice = AVSpeechSynthesisVoice(language: "en-US") synthesizer.speak(utterance) }
API Features Overview
Feature | Description |
---|---|
Language Support | Wide variety of languages and dialects available for speech synthesis. |
Voice Selection | Ability to choose different voices (male, female, etc.) and adjust pitch/tone. |
Custom Speech Rate | Control the speed at which the text is spoken to match user preferences. |
Tip: Test different voices and speech rates to find the best fit for your application's tone and audience.
Optimizing Audio Output Quality with Text to Speech API in Swift
When developing applications that utilize text-to-speech functionality, achieving high-quality audio output is a critical factor for user experience. The default voice provided by Text to Speech APIs might not always meet the desired standards, so it is essential to fine-tune the parameters and select the best settings to optimize sound quality. This process involves adjusting pitch, rate, and volume, among other aspects, to ensure the speech synthesis is clear, natural, and pleasant to the user.
Optimizing audio output quality often requires experimenting with different settings and understanding how these parameters influence the final result. By leveraging the full capabilities of the Text to Speech API in Swift, developers can create an immersive auditory experience for users, whether for accessibility features or entertainment purposes. Below are key strategies for achieving optimal audio quality.
Key Strategies for Enhancing Audio Output
- Pitch and Speed Control: Adjusting the pitch and speech rate can significantly impact the naturalness of the synthesized speech. Setting these values appropriately ensures the output matches the desired tone and tempo.
- Voice Selection: Choosing a voice that aligns with the application's context is essential. Many APIs offer a variety of voices with different accents and intonations, which can greatly affect the quality of speech.
- Audio Buffering: Efficient buffering reduces latency and enhances playback performance, especially in environments with limited resources.
- Noise Reduction: Implementing noise reduction algorithms can help reduce any artificial background noise introduced during speech synthesis.
Important Configuration Parameters
- Speech Rate: Adjusting this controls how fast the speech sounds, allowing you to either speed up or slow down the output for clarity or comfort.
- Volume Adjustment: Ensuring the audio is loud enough for clarity without distortion.
- Voice Quality: Some APIs offer "enhanced" voices that produce higher-quality audio, though these may come with additional processing costs.
Experimenting with different voice settings and paying close attention to small adjustments in speech parameters is key to achieving optimal output quality in any speech-based application.
Comparison of Voice Selection Options
Voice Type | Pros | Cons |
---|---|---|
Standard Voice | Fast loading times, wide availability | Less natural-sounding, robotic |
Enhanced Voice | More natural-sounding, smooth intonation | Higher resource consumption, slower processing |
Custom Voice | Can be tailored to specific needs | Requires advanced setup and resources |
Setting Up Language Support and Custom Voices in Swift API
When implementing text-to-speech (TTS) functionality in a Swift application, one of the first considerations is ensuring the correct language support. Apple's AVSpeechSynthesizer API allows you to specify different languages for speech output. These languages can be accessed through the AVSpeechSynthesisVoice class, which provides various voices depending on the system's locale settings.
Customizing the voice is another critical aspect of the TTS setup. By selecting specific voices, developers can tailor the experience to their application's requirements, whether it's adjusting for gender, accent, or even emotional tone. Below is a breakdown of how to configure language support and select custom voices in Swift.
1. Setting Up Language Support
- Use AVSpeechSynthesisVoice.speechVoices() to retrieve all available voices in the system.
- Languages are identified by locale codes, which can be checked and changed programmatically.
- Accessing a voice for a specific language is done via AVSpeechSynthesisVoice(language:).
- Ensure that the app supports multiple languages by implementing language fallback strategies.
2. Adding Custom Voices
- Select a voice using AVSpeechSynthesisVoice(language:) with the desired language identifier.
- To support custom voices, ensure that the app has access to additional voices downloaded from the device settings.
- To configure a voice's characteristics (e.g., pitch and rate), use the AVSpeechUtterance properties.
- Test voice clarity and performance on various devices to ensure consistency.
Note: While Apple provides a wide range of voices, custom voices must be carefully integrated to avoid performance issues or inconsistencies across different devices.
3. Voice Configuration Table
Voice Attribute | Property | Usage |
---|---|---|
Language | language (e.g., "en-US") | Selects the language of the speech output. |
Pitch | pitch | Adjusts the speech pitch. Default is 1.0. |
Rate | rate | Controls the speed of speech. Default is 0.5. |
Managing API Usage and Handling Rate Limits in Swift
When integrating a text-to-speech API in Swift, developers often encounter limitations on the number of requests that can be made in a given time period. Rate limiting is a mechanism that helps ensure fair usage and prevents overloading the API servers. Understanding how to handle these limits effectively is essential for maintaining a smooth user experience and avoiding service disruptions.
To manage API usage efficiently, developers must monitor the number of requests made and adjust the flow accordingly. This requires incorporating strategies to check for rate limits, handle responses, and implement retry mechanisms in case the limit is exceeded. Swift provides various tools that can be used to manage these tasks effectively.
Strategies for Handling Rate Limits
There are several approaches to managing rate limits in your Swift app:
- Monitor HTTP Response Headers: Many APIs return rate limit status in response headers such as X-RateLimit-Remaining or X-RateLimit-Reset. You can use these headers to track the number of requests left and when the limit will reset.
- Implement Retry Logic: When the rate limit is reached, you can implement a backoff strategy where requests are retried after a specified delay.
- Queue Requests: Instead of sending requests immediately, queue them and dispatch them based on available API slots.
Example Code Snippet
Here's an example of how you might implement a simple retry mechanism in Swift:
func makeRequestToAPI() { let url = URL(string: "https://api.example.com/speech")! var request = URLRequest(url: url) request.httpMethod = "GET" let task = URLSession.shared.dataTask(with: request) { (data, response, error) in if let httpResponse = response as? HTTPURLResponse { if httpResponse.statusCode == 429 { // Handle rate limit exceeded let resetTime = httpResponse.allHeaderFields["X-RateLimit-Reset"] as? String let delay = calculateDelayFromResetTime(resetTime) DispatchQueue.global().asyncAfter(deadline: .now() + delay) { self.makeRequestToAPI() // Retry after delay } } } } task.resume() }
Rate Limiting Information
Header | Description |
---|---|
X-RateLimit-Remaining | Indicates the number of requests remaining in the current time window. |
X-RateLimit-Reset | Indicates the time when the rate limit will reset, in Unix timestamp format. |
Status Code 429 | Indicates that the rate limit has been exceeded. |
Always check the API documentation for specific rate limits and response headers to implement an effective solution for your app.
Ensuring Accessibility: Leveraging Text to Speech API for Inclusive Design
When designing applications, accessibility should be a top priority to ensure that all users, regardless of their abilities, can fully engage with the product. One way to improve accessibility is by incorporating text-to-speech (TTS) functionality. With a Text to Speech API, developers can make their applications more inclusive, enabling users with visual impairments or reading difficulties to access content effortlessly. TTS provides an audio alternative to text, making digital experiences more accessible to a wider audience.
Integrating TTS not only aids in providing equal access but also enhances the overall user experience for diverse groups. Whether it's for people with dyslexia, low vision, or those simply preferring auditory content, TTS allows the application to be used in a more versatile manner. This flexibility ensures a more inclusive design approach that benefits all users, not just those with specific needs.
Key Benefits of Text to Speech for Accessibility
- Improved Readability: TTS converts written content into speech, allowing users to comprehend text more easily.
- Multitasking: Users can listen to content while performing other tasks, providing a hands-free experience.
- Enhanced Engagement: Auditory features keep users engaged, particularly in environments where reading may be difficult.
Implementing TTS: Steps for Accessibility
- Integrate a TTS API: Utilize a reliable API such as Apple's Speech framework or other third-party services to enable speech synthesis.
- Enable Customization: Allow users to control speech rate, pitch, and language preferences for a personalized experience.
- Focus on Clear Pronunciation: Ensure that the text is pronounced clearly, especially for complex words and names.
"Incorporating text-to-speech functionality is a powerful way to ensure that your application is usable by as many people as possible, regardless of their abilities."
Considerations for Effective Implementation
While implementing TTS functionality, it is essential to focus on user preferences. For example, providing multiple language options and adjustable speed settings can enhance the user experience. Additionally, consider testing the TTS feature across various environments to ensure the clarity of the speech output.
Factor | Recommendation |
---|---|
Speech Clarity | Test different voices to ensure the speech is clear and easy to understand. |
Language Support | Ensure that the API supports multiple languages and accents for broader accessibility. |
Customizable Settings | Offer users the ability to adjust the speed, pitch, and volume of the speech output. |
How to Test and Debug Text to Speech API in Your Swift Application
Testing and debugging the Text to Speech (TTS) API in Swift applications is essential to ensure smooth integration and correct functionality. By following systematic testing procedures, developers can identify issues early and improve the overall user experience. The TTS API is commonly used to convert text into natural speech, and improper integration can lead to unexpected results, such as poor voice quality or incorrect pronunciations.
Effective debugging techniques help identify performance bottlenecks, prevent crashes, and ensure that your TTS implementation works as expected across different devices and environments. This section outlines how to perform these steps efficiently, focusing on both testing for correctness and debugging potential issues in your Swift app.
Testing Text to Speech API
Testing the functionality of the Text to Speech API requires thorough verification of various parameters, including voice selection, pitch control, and speech rate. Here are the steps to test it properly:
- Verify Voice Selection: Ensure that the voice selected by the user works correctly across different devices and locales.
- Check Speech Quality: Test the clarity, naturalness, and tone of the speech output to ensure it matches expectations.
- Test Different Text Inputs: Use different text types (e.g., simple sentences, complex paragraphs) to check the API's ability to handle a range of content.
Debugging Common Issues
If the Text to Speech API doesn't behave as expected, debugging becomes critical. Follow these debugging steps to identify and resolve issues:
- Check API Initialization: Ensure the TTS engine is initialized properly in your application.
- Inspect Speech Configuration: Review the settings related to voice, language, and rate. Misconfigurations in these parameters often cause problems.
- Handle Errors: Implement error handling to catch and log issues related to speech synthesis failures.
Important Debugging Tips
Ensure that your app has the correct permissions to use speech synthesis on the device. Without proper permission, the TTS API may fail to work.
Sample Test Case Table
Test Case | Expected Outcome | Status |
---|---|---|
Test with standard English text | Clear and understandable speech output | Pass |
Test with special characters | Correct pronunciation of special characters | Fail |
Test speech rate adjustment | Speech speed should change according to settings | Pass |
Reducing Latency in Speech Output: Best Practices for Swift Development
When developing applications using text-to-speech (TTS) functionality, minimizing latency is crucial for enhancing the user experience. Long delays in speech output can cause frustration, especially in real-time applications such as navigation or interactive assistants. Efficient management of resources and API calls can greatly improve responsiveness.
Optimizing speech synthesis performance in Swift involves considering various factors like network speed, resource allocation, and the proper use of system libraries. By following certain practices, developers can significantly reduce delays and improve the fluidity of spoken output.
Key Techniques for Reducing Latency
- Preloading Speech Data: Load speech models and resources ahead of time to avoid delays when starting the synthesis process.
- Asynchronous Processing: Use asynchronous methods to avoid blocking the main thread, ensuring that UI responsiveness remains unaffected while generating speech.
- Batching Requests: If multiple text inputs need to be spoken, batch them together to reduce overhead from multiple API calls.
- Choosing the Right Voice Model: Some voice models are optimized for faster synthesis. Experiment with different models to find one that balances quality and speed.
Best Practices for API Integration
- Use Native iOS Frameworks: Utilize the AVSpeechSynthesizer from Apple's AVFoundation framework for optimized speech synthesis.
- Limit Background Processes: Disable unnecessary background tasks during speech output to free up system resources.
- Optimize Network Calls: If using cloud-based TTS services, ensure network requests are as fast as possible by selecting the closest servers or caching frequently used speech data locally.
Note: AVSpeechSynthesizer’s rate property controls the speed of speech output. Adjusting this can help reduce perceived latency, but be mindful of not sacrificing intelligibility for speed.
Performance Comparison: AVSpeechSynthesizer vs Cloud Services
Factor | AVSpeechSynthesizer | Cloud Services |
---|---|---|
Latency | Low (local processing) | High (network-dependent) |
Speech Quality | Good (standard voices) | Excellent (high-quality voices) |
Customization | Limited (predefined voices) | High (multiple voice options) |
Enhancing User Interaction with Speech Synthesis Technology
Understanding the way users interact with text-to-speech (TTS) functionality is crucial for developers aiming to create more engaging applications. By analyzing user engagement with TTS features, developers can identify key patterns that influence usability and refine the user experience. For instance, fine-tuning the voice quality, speed, and tone can significantly impact how users perceive the interaction, leading to either positive or negative experiences. Monitoring how frequently users access speech features can provide insights into what aspects of TTS they find most beneficial.
Improving user engagement with speech synthesis involves a variety of strategies aimed at maximizing the effectiveness of the feature. These strategies include refining voice parameters, optimizing speech clarity, and implementing context-aware adjustments based on user preferences. The ultimate goal is to create a more natural and personalized experience for users, which can be achieved by continuously iterating and testing various TTS settings.
Key Factors to Consider for Engagement Improvement
- Voice Quality: The naturalness of the speech affects how users perceive and interact with the application. A more lifelike voice tends to encourage longer and more frequent interactions.
- Customization Options: Allowing users to adjust speech speed, pitch, and tone can significantly increase satisfaction and encourage continued use.
- Context Awareness: Implementing features that adjust speech content based on user context (e.g., reading speed for longer texts) can enhance user engagement.
Personalization in TTS settings, such as adjusting voice tone or adding pauses, helps build trust and ensures that users feel more comfortable interacting with the system.
Effective Engagement Metrics
- Frequency of Use: Track how often users utilize TTS features within the app to measure engagement levels.
- User Feedback: Gather qualitative data through surveys or reviews to better understand how users perceive the speech synthesis quality.
- Completion Rates: Analyze how many users complete tasks (e.g., listening to full paragraphs) when TTS features are enabled.
Metric | Description |
---|---|
Voice Interaction Time | Measures the duration of time users spend interacting with the TTS feature. |
Repeat Usage | Tracks the frequency at which users re-enable the TTS feature during different sessions. |