Google Text to Speech Api Node Js
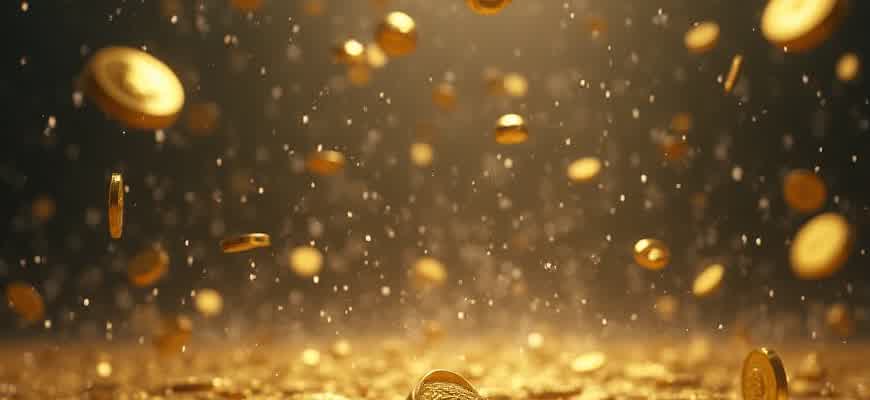
The Google Cloud Text-to-Speech API allows developers to convert text into natural-sounding speech using advanced deep learning models. When integrated with Node.js, it offers seamless voice synthesis capabilities for various applications, from virtual assistants to accessibility tools.
To get started, you'll need to follow these steps:
- Create a Google Cloud project and enable the Text-to-Speech API.
- Install the necessary Node.js client libraries.
- Authenticate using your Google Cloud credentials.
- Use the API to generate speech from text input.
Important: Make sure to secure your API key to prevent unauthorized access to your Google Cloud resources.
The Node.js client library for Google Text-to-Speech simplifies interaction with the API. The primary configuration involves defining parameters like voice selection, language, and output format. Below is a basic example of how the API can be used:
Function | Description |
---|---|
client.textToSpeech() | Generates speech from the provided text using the configured settings. |
voiceSelectionParams | Defines voice characteristics such as gender and language. |
audioConfig | Specifies the output audio format (e.g., MP3, OGG). |
Using Google Text to Speech API with Node.js: A Practical Guide
Google's Text to Speech API offers a powerful way to convert text into natural-sounding speech using machine learning models. When combined with Node.js, this API can be easily integrated into your applications to add speech capabilities. This guide will walk you through the steps needed to set up and use the Google Text to Speech API in your Node.js projects.
By leveraging Google Cloud's advanced voice synthesis technology, you can generate high-quality, human-like speech from text input. This functionality is particularly useful for building interactive applications such as voice assistants, accessibility tools, and educational apps. Below are the steps to get started and some important details to ensure smooth integration with Node.js.
Steps to Implement Google Text to Speech API in Node.js
- Set Up Google Cloud Account
Before using the API, create a Google Cloud account and enable the Text to Speech API in the Google Cloud Console. You will need to create a project and generate an API key to authenticate your requests.
- Install Node.js and Dependencies
Ensure that you have Node.js installed on your system. Then, install the Google Cloud Text to Speech client library using the following command:
npm install --save @google-cloud/text-to-speech
- Configure Authentication
Download your service account key JSON file from Google Cloud and set the environment variable to authenticate your requests:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your-service-account-file.json"
- Write the Code
Now, you can start writing the Node.js code to interact with the API. Below is an example of basic usage:
const textToSpeech = require('@google-cloud/text-to-speech'); const client = new textToSpeech.TextToSpeechClient(); async function synthesizeSpeech() { const [response] = await client.synthesizeSpeech({ input: { text: 'Hello, world!' }, voice: { languageCode: 'en-US', name: 'en-US-Wavenet-D' }, audioConfig: { audioEncoding: 'MP3' }, }); const fs = require('fs'); fs.writeFileSync('output.mp3', response.audioContent, 'binary'); } synthesizeSpeech();
Important: Make sure to handle API errors and exceptions properly in your code to ensure smooth execution and better error tracking in production environments.
Additional Considerations
When implementing the Text to Speech API, consider the following:
- Voice Selection: The API offers a wide range of voices (e.g., different languages and genders). You can fine-tune the voice parameters to suit your application's needs.
- Audio Format: Choose from various audio formats like MP3 or WAV depending on your application’s requirements.
- Quota Limits: The Google Cloud API has usage limits based on your pricing tier, so be sure to monitor your consumption.
Voice Options Table
Voice Name | Language | Gender |
---|---|---|
en-US-Wavenet-D | English (US) | Male |
en-GB-Wavenet-A | English (UK) | Female |
de-DE-Wavenet-C | German | Female |
Setting Up Google Cloud Account and Enabling Text to Speech API
Before you can use Google's Text to Speech API, you need to set up a Google Cloud account and enable the necessary API services. The process involves creating a new project in Google Cloud, enabling billing, and then activating the API. Below is a step-by-step guide to help you get started.
First, ensure you have a Google account. If not, you'll need to create one. Once your account is ready, follow the steps outlined below to set up your Google Cloud environment and enable the Text to Speech API.
1. Create a Google Cloud Project
- Go to the Google Cloud Console.
- Sign in with your Google account.
- Click on the "Select a Project" dropdown and select "New Project".
- Give your project a name and click "Create".
2. Enable Billing
To use Google Cloud services, you need to enable billing for your project. Here's how:
- In the Google Cloud Console, navigate to the "Billing" section.
- Link your billing account or create a new one if you don’t have one yet.
- Confirm that your project is linked to a billing account.
3. Enable the Text to Speech API
Once your project is set up and billing is enabled, follow these steps to activate the Text to Speech API:
- In the Google Cloud Console, go to the "API & Services" section.
- Click on "Library" and search for "Text to Speech API".
- Select the "Google Cloud Text-to-Speech API" and click "Enable".
Important: Ensure that you have set up proper billing before enabling the API. Without an active billing account, you will not be able to use the Text to Speech API.
4. Create API Key
To interact with the API, you need to create an API key:
- Go to "APIs & Services" > "Credentials".
- Click "Create Credentials" and choose "API Key".
- Copy the generated API key for later use in your Node.js application.
5. Verify API Access
After enabling the API and generating an API key, verify that everything is working correctly by checking the status of the API in the Google Cloud Console. If everything is set up properly, you're ready to start using the Text to Speech API in your project.
Summary of Setup Steps
Step | Action |
---|---|
Create Google Cloud Project | Create a new project in Google Cloud Console. |
Enable Billing | Link a billing account to your project. |
Enable Text to Speech API | Search and enable the Text to Speech API in the Google Cloud Console. |
Create API Key | Create and copy your API key to use in your Node.js application. |
Installing Node.js Client Library for Google Text to Speech
To integrate Google Text to Speech API in your Node.js application, the first step is to install the necessary client library. This library enables seamless interaction between your code and Google's services, simplifying the process of synthesizing speech from text. Below are the steps for a smooth installation process and some key considerations when setting it up.
The installation process is straightforward, but it’s crucial to ensure you have Node.js and npm set up on your machine beforehand. Once you confirm that these tools are available, you can proceed with the following steps to get started.
Step-by-Step Installation
- Install the Client Library
First, open your terminal or command prompt and run the following npm command to install the Google Cloud Text-to-Speech library:
npm install --save @google-cloud/text-to-speech
- Authentication Setup
Before using the API, ensure that you authenticate with Google Cloud. You can do this by setting up the Google Cloud Service Account and generating a private key file.
Save the generated key file and set the
GOOGLE_APPLICATION_CREDENTIALS
environment variable to its location:export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your-service-account-file.json"
- Verify Installation
Finally, ensure that the installation has been completed successfully by running a simple test script to check for any errors.
Key Considerations
- Ensure you have an active Google Cloud account with the Text-to-Speech API enabled.
- For managing multiple versions or dependencies, it's recommended to use a package manager such as npm or yarn.
- If you encounter issues with the library installation, check for any missing dependencies or permission issues related to the authentication key.
Additional Resources
Topic | Link |
---|---|
Google Cloud Documentation | Official Docs |
Node.js Client Library | GitHub Repository |
Authenticating Your Node.js Application with Google Cloud
In order to access Google Cloud services like the Text-to-Speech API from your Node.js application, proper authentication is necessary. Google uses service account credentials for secure interaction between your application and its services. Below is an outline of the authentication process, which involves generating a key file and configuring the environment to use these credentials.
Once the authentication is set up, your application will be able to securely communicate with Google Cloud, enabling you to send requests and receive responses from the Text-to-Speech API. Here’s how you can authenticate your Node.js application.
Steps for Authenticating
- Create a Google Cloud Project
If you haven't done so already, create a new project in the Google Cloud Console. Make sure to enable the Text-to-Speech API for this project.
- Set Up Service Account
To authenticate, you’ll need to create a service account and download the corresponding private key file. You can do this in the Google Cloud Console under the "IAM & Admin" section.
After creating the service account, ensure you grant the necessary roles, such as
roles/texttospeech.admin
. - Download and Set Environment Variable
Once the key file is generated, download it and set the GOOGLE_APPLICATION_CREDENTIALS environment variable to point to the file. This tells your application where to find the authentication credentials.
Use the following command to set the environment variable:
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your-service-account-file.json"
Important Authentication Considerations
- Ensure the service account has proper permissions to access the Text-to-Speech API.
- Be careful with the private key file; it should be kept secure and not exposed in public repositories.
- To manage different environments (e.g., development, production), you may need to adjust authentication settings accordingly.
Helpful Links
Resource | Link |
---|---|
Google Cloud Authentication Docs | Official Guide |
Google Cloud Console | Console Link |
Converting Text to Speech with Google API in Node.js
To enable speech synthesis in your Node.js application, integrating Google's Text-to-Speech service offers an efficient and scalable solution. This service converts input text into natural-sounding audio using Google's pre-trained models. In this guide, we will explore how to use the Google Cloud Text-to-Speech API with Node.js, providing you with step-by-step instructions to set up and implement the functionality.
Google's API supports multiple languages and voices, and it allows customization of speaking rate, pitch, and volume gain, making it a versatile tool for various use cases. Below is a breakdown of the core steps involved in setting up and using the API for text-to-speech conversion.
Setting Up the Environment
Before using the Text-to-Speech API, you need to configure a few components:
- Google Cloud Account: Create an account and set up a project in the Google Cloud Console.
- Enable the API: Enable the Text-to-Speech API for your project in the Google Cloud Console.
- Authentication: Download the service account key as a JSON file and set up your environment to authenticate using this file.
Installation and Code Setup
Once your Google Cloud project is set up, you can proceed with installing the necessary Node.js package and writing the code to convert text into speech.
- Install the Google Cloud Text-to-Speech Client:
npm install @google-cloud/text-to-speech
- Import the Package:
const textToSpeech = require('@google-cloud/text-to-speech');
- Initialize the Client:
const client = new textToSpeech.TextToSpeechClient();
- Define the Text and Request Options:
const request = { input: { text: 'Hello, how are you?' }, voice: { languageCode: 'en-US', ssmlGender: 'NEUTRAL' }, audioConfig: { audioEncoding: 'MP3' }, };
- Send the Request:
client.synthesizeSpeech(request, (err, response) => { if (err) { console.error('Error:', err); return; } // Handle the audio output here });
Important Considerations
Ensure that your API key is secured and not exposed in your code repository. Use environment variables to store sensitive credentials.
Example Response
The API will return an audio file in the specified format (e.g., MP3). Here’s an example of how to handle the audio content:
const fs = require('fs'); const writeFile = fs.createWriteStream('output.mp3'); writeFile.write(response.audioContent, 'binary'); writeFile.end();
API Configuration Options
Parameter | Description |
---|---|
languageCode | The language in which the text will be spoken (e.g., 'en-US'). |
ssmlGender | Voice gender to use, such as 'MALE', 'FEMALE', or 'NEUTRAL'. |
audioEncoding | Output audio format, such as 'MP3', 'OGG_OPUS', or 'LINEAR16'. |
Customizing Voice Parameters: Language, Gender, and Pitch
Google's Text-to-Speech API allows for a high degree of customization, enabling users to fine-tune the voice output for different applications. By adjusting parameters such as language, gender, and pitch, developers can create more natural and fitting speech experiences. These settings offer flexibility to tailor the voice to a specific audience or use case, ensuring clarity and engagement for the end users.
Understanding how to modify these settings is essential for achieving the desired output. Below, we explore the key parameters that can be adjusted to enhance the quality of the synthesized voice.
Voice Customization Parameters
- Language: This parameter controls the language in which the speech will be generated. It is essential to select the correct language to ensure proper pronunciation and meaning.
- Gender: You can choose between different voice types, typically male or female, to suit the tone and style of your application.
- Pitch: This parameter adjusts the tonal quality of the voice, making it sound higher or lower. Fine-tuning pitch can influence the expressiveness of the speech.
Key Configuration Example
Here is an example of how these parameters might be configured in a Node.js application using the Google Text-to-Speech API:
const request = { input: { text: 'Hello, how are you?' }, voice: { languageCode: 'en-US', ssmlGender: 'FEMALE', pitch: 2.0 }, audioConfig: { audioEncoding: 'MP3' } };
Important: Always ensure that the selected language code matches the available voices and regional settings for accurate synthesis.
Voice Parameter Settings in Google Text-to-Speech API
Parameter | Description | Example Value |
---|---|---|
Language | Defines the language in which the speech will be generated. | en-US, de-DE, fr-FR |
Gender | Specifies whether the voice is male or female. | MALE, FEMALE |
Pitch | Adjusts the pitch of the voice to a higher or lower tone. | 0.0 (neutral), 2.0 (higher), -2.0 (lower) |
Handling Errors and Debugging Issues with Google Text to Speech API
When working with the Google Text to Speech API in Node.js, developers may encounter several types of errors during implementation. These issues can stem from incorrect configuration, improper authentication, or network-related problems. Proper error handling and debugging practices are essential to identify and resolve these issues efficiently.
Understanding the error messages provided by the API and implementing structured error-handling routines can significantly enhance the development process. Below are common issues and how to address them when integrating the Google Text to Speech API with Node.js.
Common Errors and Their Solutions
- Authentication Errors: One of the most frequent issues is improper API key configuration. Ensure that the key provided is valid and has the correct permissions.
- Network Connectivity: API calls might fail due to network problems. Ensure that your server has access to the internet and there are no firewall restrictions blocking the API request.
- Invalid Input Data: If the request body is malformed or includes unsupported parameters, the API will return a 400 error. Always validate input data before making a request.
Steps to Debug Issues
- Check API logs to identify error codes and messages that can provide more context about the problem.
- Validate the API key and ensure it's linked to the correct Google Cloud project with the Text-to-Speech API enabled.
- Use tools like Postman to manually test API endpoints, which can help isolate issues with the request structure or authentication.
Tip: When an error occurs, the response body will often contain a detailed error message that can point to the root cause. Always inspect the message in the response for clues.
Example Error Handling in Node.js
const textToSpeech = require('@google-cloud/text-to-speech');
const client = new textToSpeech.TextToSpeechClient();
async function synthesizeSpeech() {
try {
const [response] = await client.synthesizeSpeech(request);
console.log('Audio content written to file: output.mp3');
} catch (error) {
console.error('Error during API call:', error.message);
}
}
By wrapping the API request in a try-catch block, developers can handle unexpected errors and ensure the application continues running smoothly.
Useful Debugging Tools
Tool | Description |
---|---|
Google Cloud Console | Monitor API usage, check logs for error details, and ensure the correct configuration of services. |
Postman | Test API endpoints manually and view detailed response headers and bodies for troubleshooting. |
Integrating Google Text-to-Speech into Your Web or Mobile Application
Google's Text-to-Speech API provides developers with the ability to convert text into natural-sounding speech. By integrating this API into your web or mobile applications, you can enhance user experience by offering voice-driven features such as reading content aloud, voice feedback, and interactive voice responses. In this guide, we will explore how to set up and integrate the API efficiently.
To start using Google Text-to-Speech in your application, you need to complete several steps, such as enabling the API, setting up the project, and configuring authentication. Once this is done, you can begin implementing text-to-speech functionality using simple API requests. The process will vary slightly depending on whether you are developing a web or mobile app, but the core concepts remain the same.
Setting Up the API
Before integrating Text-to-Speech into your application, you need to set up the Google Cloud Platform project and enable the API. Here’s a general setup procedure:
- Visit the Google Cloud Console and create a new project.
- Enable the Text-to-Speech API for your project.
- Create a service account to manage credentials.
- Download the credentials file (JSON) and set up authentication for your app.
Implementation Steps
Once your environment is ready, the next step is to implement the text-to-speech conversion. Here’s a basic overview of how to use it in a Node.js environment:
- Install the necessary Node.js client library:
npm install @google-cloud/text-to-speech
. - Import the client library and set up the client using your credentials.
- Send a request with the text you want to convert and specify the voice parameters (e.g., language, pitch, speed).
- Handle the response and play the audio or save it as an audio file.
To get the best results, experiment with the different voice options provided by the API, including gender, language, and speech type.
Example Code Snippet
Here is an example of how you can implement Google Text-to-Speech in a Node.js application:
const textToSpeech = require('@google-cloud/text-to-speech'); const fs = require('fs'); const client = new textToSpeech.TextToSpeechClient(); async function convertTextToSpeech() { const request = { input: { text: 'Hello, world!' }, voice: { languageCode: 'en-US', ssmlGender: 'NEUTRAL' }, audioConfig: { audioEncoding: 'MP3' }, }; const [response] = await client.synthesizeSpeech(request); fs.writeFileSync('output.mp3', response.audioContent, 'binary'); }
This code will convert the text into speech and save the audio file as 'output.mp3'.
Considerations for Web and Mobile Applications
When implementing this API for different platforms, consider the following:
Platform | Consideration |
---|---|
Web | Ensure compatibility with modern browsers and manage audio playback controls. |
Mobile | Ensure that audio files are cached locally for offline use and handle permissions for audio playback. |