How to Use Azure Speech to Text Api
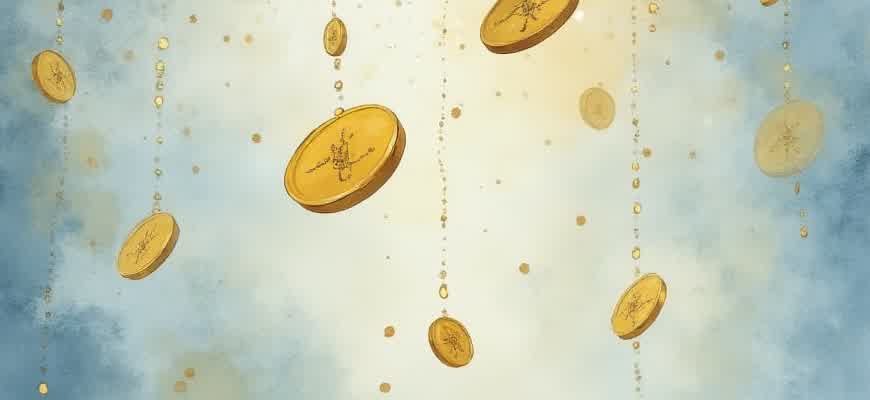
Initial Setup and Authentication
- Create an Azure account and navigate to the Azure Portal.
- Provision a new Speech resource in your preferred region.
- Note the
Key
andRegion
from the resource's "Keys and Endpoint" section – you'll need them for API requests.
Use the pricing tier that suits your project size. For prototyping, the Free F0 tier is typically sufficient.
Making Your First Request
- Install the official SDK:
pip install azure-cognitiveservices-speech
- Initialize the client with your credentials.
- Pass the path to your audio file (WAV or MP3) to transcribe.
Parameter | Description |
---|---|
speech_key |
Your subscription key from the Azure resource |
service_region |
The geographic region of your speech resource (e.g., "eastus") |
Ensure the audio sample rate matches supported formats (e.g., 16 kHz, mono) to avoid processing errors.
Converting Audio to Text with Microsoft’s Cloud Speech Services
To convert spoken words into written form using Microsoft's cloud tools, you first need to prepare an audio input and authenticate with the cognitive service endpoint. The process supports real-time transcription or analysis of pre-recorded audio files. Integration is done through REST APIs or SDKs available for various programming languages.
Once your environment is ready, send audio data in supported formats like WAV or MP3. The service processes this data and returns the textual output in JSON format, which can be parsed and used in applications like subtitles, voice commands, or transcription services.
Steps to Set Up and Run Speech Recognition
- Create a resource in the Azure Portal with speech capabilities.
- Retrieve the access key and region endpoint from your resource dashboard.
- Install the appropriate SDK (e.g., for Python:
pip install azure-cognitiveservices-speech
). - Write a script to authenticate, send the audio, and handle the response.
- Supports real-time or batch transcription.
- Returns confidence scores with transcribed text.
- Works with multiple languages and dialects.
Note: Only audio formats with PCM codec, 16-bit sample, and 16 kHz sample rate are recommended for optimal accuracy.
Feature | Description |
---|---|
Audio Input | Supports WAV, MP3, Ogg with specific encoding |
Response Format | JSON containing recognized text and metadata |
Languages | Over 90 supported languages and locales |
Setting Up Your Azure Account and Enabling Speech Services
To begin working with Microsoft's voice recognition tools, you need to establish an Azure account and activate the appropriate language service. This involves registering for the Azure portal, selecting a subscription plan, and creating a resource group to manage your configurations.
Once your account is active, the next step is to provision a new instance of the voice analysis resource in a specific region. This will provide you with the necessary endpoint and authentication credentials to interact with the API programmatically.
Step-by-Step Activation Process
- Sign in at portal.azure.com or create an account if you don't have one.
- Navigate to “Create a resource” and search for Speech.
- Choose the Speech offering and click Create.
- Select your subscription and either create or choose a resource group.
- Pick a region, assign a unique name to your service, and select the pricing tier.
- Review and confirm the creation to deploy your service instance.
You must select a region that supports the Speech resource. Not all regions provide access to all cognitive services.
Key Configuration Parameters
Parameter | Description |
---|---|
Subscription Key | Authentication token required for API access |
Service Region | Geographical location where the service is hosted |
Endpoint URL | Base URL for sending transcription requests |
- Store your subscription key securely – avoid exposing it in client-side code.
- You can manage and regenerate your keys from the resource’s “Keys and Endpoint” tab.
Creating a Speech Resource in Azure Portal Step-by-Step
To enable speech recognition in your application, the first step is to set up a dedicated speech service instance within Microsoft Azure. This involves provisioning a resource through the Azure Portal, which grants access to the necessary API keys and endpoint URL.
Once created, this resource serves as the foundation for integrating voice input features such as transcription or real-time recognition into your software or service.
Steps to Provision a Speech Resource
- Navigate to the Azure Portal and select Create a resource.
- In the search bar, type Speech and select Speech service from the results.
- Click Create to open the configuration form.
- Complete the required fields:
- Subscription – choose your Azure subscription.
- Resource group – select an existing one or create a new group.
- Region – choose the location closest to your users for lower latency.
- Name – assign a unique name for your speech service instance.
- Pricing tier – select an appropriate pricing plan based on expected usage.
- Click Review + create, verify your settings, then select Create.
After deployment, navigate to the new resource and copy the Key1 and Endpoint values. These will be required for authentication when making API calls.
Field | Description |
---|---|
Subscription | Billing source for the speech service |
Region | Determines the geographic server location |
Resource group | Container for managing related resources |
Pricing tier | Defines cost and usage limits |
Generating and Managing Subscription Keys for Authentication
To interact with Azure’s speech recognition services, a secure method of identification is required. This involves acquiring access tokens, known as subscription keys, which are tied to your Azure Speech resource. These keys enable authorized communication between your application and Azure’s Speech API endpoints.
Subscription keys are generated within the Azure portal under your Speech service instance. Each resource is assigned two keys to allow for key rotation without downtime. Effective management of these credentials ensures the continuity and security of your application’s access to Azure services.
Steps to Retrieve and Maintain Access Keys
- Sign in to the Azure Portal and navigate to your Speech resource.
- Under the “Keys and Endpoint” section, locate your two access keys and service region.
- Use either key for authentication in API calls.
- Use both keys alternately to rotate them periodically.
- Store keys securely in environment variables or key vaults.
- Revoke compromised keys immediately and replace with new ones.
Important: Never embed subscription keys directly in client-side code. Doing so exposes them to unauthorized users and compromises your Azure account.
Key | Description |
---|---|
Key1 / Key2 | Primary and secondary tokens used for authentication. |
Region | Specifies the Azure data center for API interaction. |
Installing Required SDKs and Dependencies for Your Development Environment
Before integrating Microsoft's audio transcription service into your application, it's essential to set up the necessary tools and libraries specific to your programming language. This process ensures seamless interaction with the cloud-based speech recognition engine via secure API calls. Whether you are working in Python, .NET, or JavaScript, you'll need to prepare your environment properly.
The primary requirement is the installation of the Speech SDK provided by Microsoft, along with related packages to handle HTTP requests, audio input, and authentication. Make sure your system has access to a supported version of your programming language's runtime, as well as build tools if compiling from source.
Development Setup Checklist
- Verify your platform meets the minimum runtime version (e.g., Python 3.7+, .NET 6.0+).
- Install the Speech SDK via package managers.
- Confirm audio input device permissions (microphone access).
- Configure environment variables for API credentials.
Note: For most platforms, the Speech SDK is cross-platform, but microphone support and real-time audio input may require OS-specific permissions or dependencies.
Language | Installation Command | Required Tools |
---|---|---|
Python | pip install azure-cognitiveservices-speech |
pip, Python 3.7+ |
.NET (C#) | dotnet add package Microsoft.CognitiveServices.Speech |
.NET SDK 6.0+ |
JavaScript (Node.js) | npm install microsoft-cognitiveservices-speech-sdk |
Node.js 14+ |
- Update your system and install the necessary SDK for your platform.
- Test a basic sample to verify the SDK was installed correctly.
- Store your subscription key and region in environment variables to secure authentication.
Making Your First Speech Recognition Request Using a Local Audio File
To convert spoken words from a local audio file into written text using Microsoft’s cloud-based tools, you need to interact with their voice processing endpoint via HTTP. This involves preparing an authenticated request, uploading your audio file, and receiving a JSON response with the transcription.
The process includes obtaining an access key, setting the correct request headers, specifying the audio format, and ensuring the file is in a compatible encoding like WAV with PCM codec. Below is a step-by-step overview of how to send your first audio file for transcription.
Step-by-Step Guide to Submit Audio for Transcription
- Get your Azure subscription key and endpoint from the portal.
- Prepare your audio file (recommended: WAV, 16-bit PCM, mono).
- Use a tool like curl or a script to send an HTTP POST request.
- Set headers for authentication, content type, and region.
- Send the file data in the body of the request.
Note: Only short audio files (up to 60 seconds) can be submitted using the REST API. For longer audio, use batch transcription services.
- Content-Type: audio/wav; codecs=audio/pcm
- Ocp-Apim-Subscription-Key: Your Azure key
- Region: Service region (e.g., westus)
Parameter | Description |
---|---|
Endpoint URL | https://region.stt.speech.microsoft.com/speech/recognition/conversation/cognitiveservices/v1 |
HTTP Method | POST |
Supported Audio Formats | WAV (PCM), MP3, OGG |
Streaming Audio to Azure Speech API in Real Time Using WebSockets
To interact with the Azure Speech API in real-time, one effective approach is streaming audio data via WebSockets. This method allows continuous transmission of audio from a client application to the API, enabling near-instantaneous speech recognition results. By leveraging WebSockets, developers can achieve low-latency communication, ensuring that the speech-to-text conversion occurs almost as the audio is being recorded or played back.
WebSockets provide a persistent, full-duplex connection between the client and server, making them an ideal solution for real-time audio streaming. This approach eliminates the need for continuous HTTP requests and responses, thus improving performance and responsiveness. Below is a breakdown of the process for integrating WebSocket-based audio streaming with the Azure Speech API.
Steps to Stream Audio Using WebSockets
- Establish a WebSocket Connection: First, initiate a WebSocket connection to the Azure Speech API endpoint, ensuring that the proper authorization and headers are configured.
- Stream Audio Data: Once the connection is established, begin sending audio data in small chunks. These chunks should adhere to the API's specified audio format (e.g., WAV, PCM).
- Handle Audio Response: As the audio is streamed, the API will send partial transcriptions back. Your application should listen for these messages and process the results in real-time.
- Close the Connection: Once the audio stream ends, close the WebSocket connection gracefully to avoid memory leaks or unnecessary data consumption.
Important: Ensure the audio stream is encoded correctly and that the WebSocket connection is stable for uninterrupted data flow to the Azure API.
Audio Format and Configuration
Audio Parameter | Required Format |
---|---|
Audio Encoding | PCM, WAV, or other supported formats |
Sample Rate | 16 kHz or higher |
Channels | Mono or Stereo (Mono recommended for better accuracy) |
Handling API Responses: Extracting and Structuring Transcribed Text
When interacting with the Azure Speech-to-Text API, it's important to correctly process the response data to extract usable text. The API returns a JSON structure containing detailed information, including the transcribed text, confidence scores, and potential errors. Properly parsing this information allows developers to structure it for further use, such as displaying it on a user interface or storing it in a database.
The core of the response is the transcription itself, but it's also essential to handle additional metadata, such as punctuation and timing information, to improve text accuracy. This metadata can enhance the quality of transcriptions, especially in use cases requiring high precision.
Extracting Transcribed Text
Once the API response is received, the transcribed text is typically located within the "DisplayText" or "Text" field of the JSON. Developers should focus on this key to extract the raw transcription. Here's an example of a structured API response:
Key | Value |
---|---|
status | success |
recognizedText | Today is a beautiful day |
confidence | 0.98 |
Structuring and Storing Transcription Data
Once the transcription is extracted, the next step is to format and store it according to the application's requirements. Often, additional information such as confidence scores, timing data, and speaker identification can be valuable. This metadata helps with evaluating the quality of the transcription and integrating it with other systems.
- Text – The actual transcribed content.
- Confidence – A numerical score indicating the accuracy of the transcription.
- Start and End Time – Marks the start and end time of each segment of speech in the audio.
- Speaker – Identifies the speaker if multiple speakers are detected in the audio.
Important: Always ensure proper error handling when parsing API responses. Unexpected structures or missing fields can occur if the audio input is unclear or if there are network issues.
Troubleshooting Common Errors and Interpreting API Status Codes
When working with the Azure Speech to Text API, users may encounter various issues that prevent successful transcription. Understanding how to interpret the error messages and API status codes is crucial for resolving problems efficiently. The API responds with different status codes that indicate the success or failure of a request. Identifying the root cause of the error is often the first step in troubleshooting and can save valuable time.
Errors typically arise from issues such as incorrect API keys, invalid request formats, or network connectivity problems. Recognizing these errors and understanding their status codes will help you address them promptly. The following guide outlines common errors and how to interpret the API's response codes.
Common Error Scenarios
- 401 Unauthorized: This error occurs when the API key provided is invalid or missing. Ensure that you are using the correct key from the Azure portal and that it has the necessary permissions.
- 400 Bad Request: This indicates that the request format is incorrect. Verify that the data being sent matches the expected format outlined in the API documentation.
- 429 Too Many Requests: If the API rate limit is exceeded, this error will occur. Reduce the frequency of requests or implement backoff strategies to avoid hitting the rate limits.
- 503 Service Unavailable: This error points to a temporary issue with the Azure service. Check the Azure status page for any ongoing outages or maintenance.
Understanding API Status Codes
The Azure Speech to Text API returns a variety of status codes that convey the outcome of your request. Here's a brief overview of the most common codes:
Status Code | Description |
---|---|
200 OK | The request was successful and the transcription is ready. |
400 Bad Request | The request could not be understood due to invalid syntax or missing parameters. |
401 Unauthorized | The API key is missing or invalid. Please check your credentials. |
429 Too Many Requests | The rate limit for the API has been exceeded. Try reducing the frequency of requests. |
503 Service Unavailable | The service is temporarily unavailable. Check for maintenance or outages. |
Note: Always ensure that your API keys are stored securely and never exposed in public repositories or client-side code.