How to Use Text to Speech on Websites
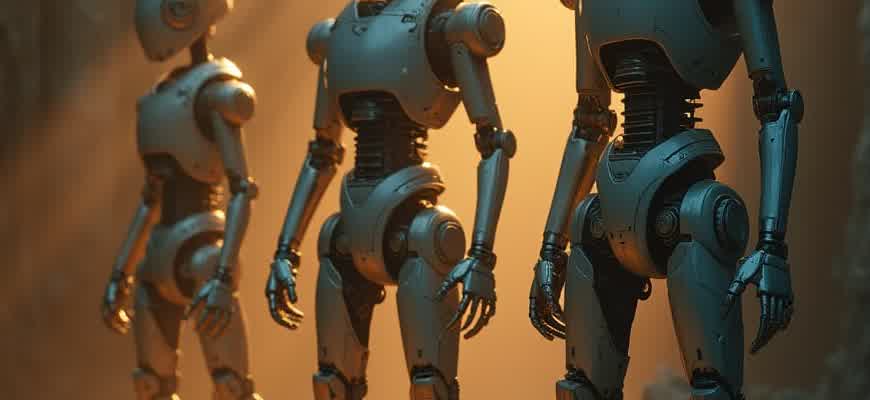
Text-to-speech (TTS) functionality allows users to listen to the content of a webpage rather than reading it. This feature is especially helpful for individuals with visual impairments or those who prefer auditory learning. Here’s a guide on how to activate TTS on various websites:
- Install a TTS extension on your browser
- Use built-in browser tools
- Explore website-specific TTS settings
Most modern browsers, such as Chrome and Firefox, offer extensions that enable text-to-speech. Follow the steps below to set it up:
- Visit the browser’s extension marketplace.
- Search for TTS tools like "Read Aloud" or "Speechify".
- Click "Add to Browser" and follow the installation instructions.
- Once installed, select the text you want read aloud and click the TTS extension icon.
Note: Some websites have built-in TTS options that can be activated from their accessibility menu.
Additionally, you can enable TTS features directly from operating system settings, making it easier to use across different websites without needing third-party tools.
Choosing the Right Text-to-Speech Tool for Your Website
When selecting a text-to-speech tool for your website, it is crucial to consider factors like voice quality, language support, and customization options. A good TTS solution will enhance the user experience, making it more accessible and engaging for visitors. There are various platforms and services available, each offering unique features tailored to different needs.
To make an informed decision, evaluate the following factors: performance, ease of integration, and cost-effectiveness. Below is a breakdown of key considerations when choosing a text-to-speech service for your site.
Key Factors to Consider
- Voice Quality: Ensure the TTS tool offers clear, natural-sounding voices that match the tone of your website.
- Language Support: Check if the tool supports the languages your audience speaks.
- Customizability: Look for options to adjust speed, pitch, and volume for a personalized experience.
- Integration: Make sure the tool integrates seamlessly with your website's platform (e.g., WordPress, custom HTML).
- Cost: Compare pricing plans to ensure they fit within your budget while meeting your needs.
Comparison Table of Popular Text-to-Speech Tools
Tool | Voice Quality | Languages Supported | Customization | Pricing |
---|---|---|---|---|
Tool A | Natural | English, Spanish, French | Speed, Pitch | Free tier, Paid from $20/month |
Tool B | Clear, Robotic | English, German | Volume | $10/month |
Tool C | Natural | Multiple languages | Speed, Volume, Pitch | Free, Paid from $15/month |
Tip: Always test the TTS tool on your site before finalizing your decision to ensure compatibility and user satisfaction.
Integrating Text-to-Speech in Website Code
Adding text-to-speech functionality to a website allows users to interact with content in an innovative way. This can enhance accessibility and user experience, particularly for those with visual impairments or reading difficulties. By leveraging modern web technologies, you can easily integrate text-to-speech services into your website with just a few lines of code.
In this section, we will explore how to embed text-to-speech features directly into your website's code using JavaScript. This approach provides flexibility and control over how text is read aloud and allows for customization of the voice, pitch, and speed.
Steps to Implement Text-to-Speech
- Ensure your website uses JavaScript to handle dynamic actions.
- Leverage the Web Speech API's SpeechSynthesis interface.
- Use HTML elements to trigger speech events, such as buttons or text areas.
- Customize speech settings, such as voice, pitch, and rate.
The most important part of integrating text-to-speech is ensuring compatibility across different browsers. Most modern browsers support the SpeechSynthesis API, but it's essential to test functionality on all platforms you expect your users to use.
Note: Some browsers may require users to enable certain settings to make the speech feature work, so it’s crucial to provide instructions or fallback solutions for compatibility issues.
Example Code
Here’s a simple example of how to implement text-to-speech in your code:
const speakButton = document.getElementById('speakBtn');
speakButton.addEventListener('click', function() {
const text = document.getElementById('textInput').value;
const utterance = new SpeechSynthesisUtterance(text);
speechSynthesis.speak(utterance);
});
This code allows users to type text into a field, and when they click a button, the text will be read aloud.
Customizing Speech Settings
Setting | Description |
---|---|
Voice | Choose a specific voice from the available voices in the browser. |
Rate | Adjusts the speed of the speech (normal is 1.0). |
Pitch | Controls the pitch of the voice (normal is 1.0). |
To customize these settings, you can modify the SpeechSynthesisUtterance
object properties as shown below:
const utterance = new SpeechSynthesisUtterance(text);
utterance.voice = speechSynthesis.getVoices()[0];
utterance.rate = 1.2;
utterance.pitch = 1.1;
Customizing Voice and Language Settings for Accessibility
Personalizing the voice and language settings of text-to-speech tools is crucial for enhancing the web accessibility experience. By tailoring these settings, users can ensure that the content is delivered in a way that suits their preferences and needs, whether they have specific language requirements or prefer a certain voice tone. These adjustments help create a more inclusive digital environment for individuals with various accessibility challenges, such as those with visual impairments or learning disabilities.
To improve user experience, most websites with text-to-speech functionality offer a variety of options to modify voice characteristics, speed, pitch, and language preferences. This ensures that users can find a setup that is comfortable and effective for them, making the web more accessible to a broader audience.
Voice Customization Options
- Voice Selection: Choose from different male or female voices, or select regional accents that better suit user preferences.
- Speed Adjustment: Modify the reading speed to make the text more understandable and suitable for the listener's pace.
- Pitch Control: Adjust the pitch of the voice to make it higher or lower based on individual comfort and clarity.
- Volume Settings: Increase or decrease the volume to meet the user's hearing needs.
Language Preferences
- Select Primary Language: Choose the main language for content reading, including options for regional dialects and variations.
- Secondary Language Support: Enable multilingual support for websites offering content in multiple languages.
- Translate Text: In some cases, websites offer automatic translation to different languages, improving accessibility for non-native speakers.
Table: Example Language and Voice Settings
Language | Voice Options | Speed Adjustment |
---|---|---|
English | Male, Female, Regional Accents | Slow, Normal, Fast |
Spanish | Male, Female, Regional Accents | Slow, Normal, Fast |
French | Male, Female | Slow, Normal, Fast |
Customizing voice settings helps improve comprehension and listening comfort for users with diverse accessibility needs. The ability to modify pitch, speed, and language can transform how users interact with online content, making it more inclusive and user-friendly.
Optimizing Performance for Seamless Audio Playback
To ensure smooth and efficient text-to-speech (TTS) functionality on websites, optimizing the performance is crucial. High-quality audio output with minimal delays enhances user experience. However, the speed and stability of the TTS feature largely depend on several technical factors such as server-side processing, resource management, and compatibility with different browsers and devices.
By focusing on these optimization techniques, developers can improve the responsiveness of the TTS system, reduce loading times, and ensure that the audio plays without interruptions. Below are some key strategies to enhance performance.
Key Optimization Techniques
- Audio File Compression: Minimize the size of audio files to reduce loading time and prevent buffering. Use modern audio formats like MP3 or Ogg for better efficiency.
- Lazy Loading: Implement lazy loading of audio elements, so that audio data is only loaded when necessary, preventing unnecessary delays.
- Preload Audio: Preloading frequently used text-to-speech data allows the audio to be ready for playback as soon as the user interacts with the content.
Recommended Server-Side Strategies
- Load Balancing: Distribute the TTS workload evenly across multiple servers to avoid overloading any single server and ensure fast response times.
- Use of Caching: Cache commonly requested text-to-speech responses to minimize repeated processing and improve load times.
- API Optimization: Optimize TTS APIs to reduce the number of requests needed and minimize network latency.
Important: Regularly test the TTS implementation across different devices and browsers to ensure that performance remains optimal for all users.
Performance Benchmarking
Strategy | Expected Result |
---|---|
Audio Compression | Reduced load times and improved user experience. |
Lazy Loading | Improved initial page load speed and smoother interaction. |
Preloading Audio | Quick audio playback with minimal delay. |
Ensuring Compatibility Across Different Browsers and Devices
Integrating text-to-speech functionality on websites requires careful attention to browser compatibility, as not all browsers support the SpeechSynthesis API in the same way. For example, while modern browsers like Chrome and Safari offer full support, others like Internet Explorer or older versions of Firefox may present limitations, such as fewer voice options or slower processing. To ensure that text-to-speech features work as expected for all users, developers must test across various browsers and implement fallback strategies for unsupported environments.
In addition to browser differences, device capabilities must also be considered. Desktop systems typically handle speech synthesis without issues, but mobile devices, especially older models, may experience delays or reduced quality in speech output. For smooth user experience across devices, it's essential to optimize text-to-speech settings based on the device’s processing power and network capabilities, ensuring that the feature works well even on less powerful smartphones or tablets.
Best Practices for Cross-Browser and Device Compatibility
- Perform thorough testing on major browsers such as Chrome, Firefox, Safari, and Edge to ensure consistent performance.
- Use feature detection to confirm whether the SpeechSynthesis API is supported by the user's browser before triggering speech functionality.
- Incorporate fallback mechanisms, such as JavaScript polyfills or third-party libraries, to ensure compatibility with browsers that have limited support.
- Optimize text-to-speech for mobile devices by adjusting speech speed and quality based on the device’s performance.
- Ensure that voice selection, language settings, and speech pacing are consistent across various platforms and devices.
Important: Test text-to-speech features across a variety of devices and browsers to catch any issues early and improve overall user experience.
Platform Support Overview
Platform | API Support | Common Issues |
---|---|---|
Chrome | Full support | Minor variations in voice selection based on region |
Firefox | Partial support | Limited number of voices and languages |
Safari | Good support | Limited selection of voices |
Mobile Devices (iOS/Android) | Varies by device | Performance issues on lower-end devices, delayed audio |
Adding Text to Speech Functionality to Content Management Systems
Integrating text-to-speech features into content management systems (CMS) is an effective way to enhance accessibility and user experience. This functionality allows website content to be read aloud to users, making it easier for individuals with visual impairments or reading difficulties to access information. Moreover, it can improve engagement by offering an alternative way to consume content, especially for auditory learners or those on the go.
To incorporate text-to-speech capabilities into a CMS, developers must ensure compatibility with existing content formats and frameworks. It involves selecting the right tools, configuring APIs, and implementing a user-friendly interface. Below are key steps to follow when adding this functionality:
Steps for Implementing Text to Speech
- Choose a TTS service: Select a reliable text-to-speech API such as Google Cloud Text-to-Speech, IBM Watson, or Amazon Polly.
- Integrate the API: Connect the chosen API with the CMS backend to enable text-to-speech functionality across the site.
- Customize voice options: Configure voice preferences such as language, pitch, speed, and volume for a personalized experience.
- Create user controls: Add controls like play, pause, stop, and skip to allow users to manage the audio output.
Important Considerations
When adding text-to-speech to a CMS, it's essential to consider performance and usability. Below are some important factors to keep in mind:
Factor | Consideration |
---|---|
Compatibility | Ensure the TTS system supports the CMS's content format and provides seamless integration. |
Quality of Speech | Select high-quality voices that sound natural and clear for an optimal user experience. |
Customization | Provide options for users to adjust speech settings according to their preferences. |
Note: Always test the text-to-speech functionality across different devices and browsers to ensure compatibility and reliability for all users.
Testing Text to Speech Features for Accuracy and User Experience
When integrating text-to-speech (TTS) functionality into a website, it’s crucial to test both the accuracy of speech synthesis and the overall user experience. Accuracy refers to how well the software interprets and reads the text, while user experience involves the ease and satisfaction of interacting with the TTS feature. Effective testing ensures that the TTS feature works correctly across different devices and provides a smooth experience for all users.
To test TTS functionality, developers must evaluate both technical performance and user interaction. Below are key aspects to assess when testing text-to-speech integration:
Key Testing Areas
- Pronunciation Accuracy: Ensure that the TTS system correctly pronounces words, especially complex terms, names, or abbreviations.
- Naturalness of Speech: Evaluate whether the voice sounds natural and fluid, rather than robotic or monotonous.
- Responsiveness: Check how quickly the system responds to user input and whether there are any noticeable delays.
- Volume and Clarity: Ensure that the speech output is clear, and the volume is adjustable for different user needs.
Steps for Effective Testing
- Test Across Devices: Verify the TTS feature works on multiple devices and screen sizes, including smartphones, tablets, and desktops.
- Test for Different Languages: Check that the TTS system handles multiple languages or dialects correctly.
- Conduct User Feedback Sessions: Ask real users to interact with the TTS feature and provide feedback on their experience.
Reminder: It’s important to test the TTS functionality in various environmental conditions, such as different network speeds or in noisy settings, to ensure consistent performance.
Performance Metrics
Metric | Evaluation Criteria |
---|---|
Speed | Measure the time it takes for text to be converted to speech and played back. |
Accuracy | Assess how accurately the system pronounces all elements of the text. |
Ease of Use | Evaluate the simplicity of the interface for interacting with the TTS controls. |
Promoting Speech Synthesis Features to Your Website Audience
Integrating speech synthesis into your website can offer users an enhanced and accessible browsing experience. By promoting this feature, you ensure that visitors can take full advantage of the content, whether they are visually impaired or simply prefer listening over reading. Clear communication about the availability of text-to-speech (TTS) can significantly increase its usage and improve user satisfaction.
To encourage website visitors to use the TTS functionality, it's crucial to highlight the feature in a user-friendly manner. You can do this through intuitive design, placement, and direct communication of the benefits. Here's how you can effectively promote this feature:
Effective Ways to Highlight TTS Features
- Visibility: Place TTS buttons prominently on your site, especially on content-heavy pages like blogs, articles, and product descriptions.
- Interactive Prompts: Use tooltips or small pop-ups to inform users about the availability of the speech feature as they navigate the site.
- Instructions: Provide clear and concise instructions on how to activate the TTS feature. This can be a small tutorial or an FAQ section.
Benefits of Using TTS Features:
- Enhanced Accessibility: Allows visually impaired users to consume content.
- Convenience: Visitors can multitask while listening to the content, making it easier to engage with your site.
- Engagement: Audio content can increase time spent on the website and boost interaction with the content.
By promoting text-to-speech options, you're not only improving accessibility but also providing a modern and convenient way for users to interact with your content.
Tracking User Engagement
Metric | Impact |
---|---|
Click-through Rate | Higher click-through rate on content when TTS is prominently displayed |
User Retention | Increased user retention due to enhanced usability and convenience |