Text to Speech Api Eleven Labs
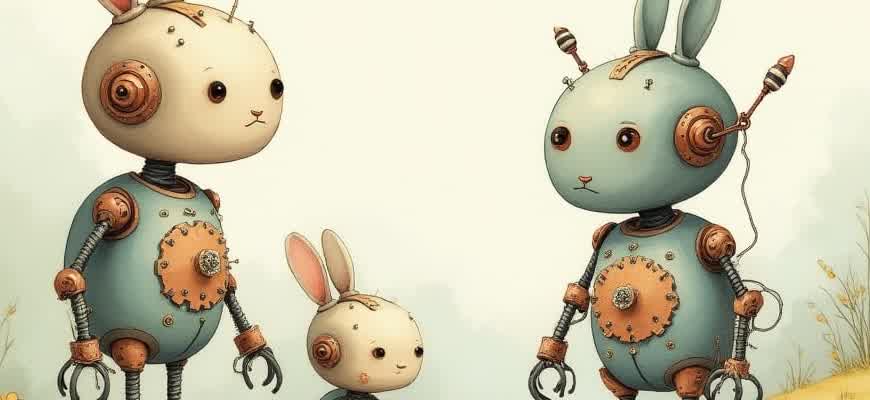
The Text-to-Speech API from Eleven Labs enables developers to integrate advanced speech synthesis capabilities into their applications. The service is designed to produce highly realistic human-like voices, utilizing deep learning models. It offers a wide variety of customization options, making it ideal for creating personalized audio experiences.
Key features of the Eleven Labs Text-to-Speech API include:
- Multiple voice options with various tones and accents
- Natural-sounding speech synthesis with expressive intonation
- Support for multiple languages and dialects
- Customizable speech speed, pitch, and volume
"With Eleven Labs' API, users can generate high-quality audio for a range of applications including virtual assistants, content narration, and interactive experiences."
Below is a comparison of some of the different voice options available:
Voice Type | Language | Accent |
---|---|---|
Male Voice 1 | English | American |
Female Voice 2 | Spanish | European |
Neutral Voice 3 | French | Neutral |
Complete Guide to Eleven Labs Text to Speech API
The Eleven Labs Text to Speech API provides a robust and easy-to-use platform for converting text into natural-sounding speech. By leveraging advanced deep learning models, this API offers high-quality voice synthesis that is highly customizable and can be integrated into various applications. Whether you're building a voice assistant, a language learning tool, or enhancing accessibility features, the API can cater to different use cases and requirements.
This guide will walk you through the key features and integration steps for using Eleven Labs Text to Speech API, providing you with a clear understanding of its capabilities and how to implement it in your projects. With options for different voices, languages, and fine-tuned control over speech parameters, Eleven Labs offers a flexible solution for creating high-quality audio outputs from text.
Key Features of Eleven Labs API
- Multiple languages and accents available
- Customizable voice settings (speed, pitch, volume)
- High-quality, natural-sounding voices
- Real-time text-to-speech conversion
- Scalable API for various project sizes
How to Use the API
- Sign up for an Eleven Labs account and obtain your API key.
- Choose a voice from the available selection of voices in the API documentation.
- Make an API request by sending a POST request with your text, voice choice, and any desired parameters.
- Handle the response by processing the audio output and integrating it into your application.
The Eleven Labs API allows for fine-tuning of voice characteristics, giving developers the ability to create personalized and highly natural audio outputs. Adjust parameters such as pitch, speed, and volume to tailor the speech to your needs.
Example API Request
Field | Value |
---|---|
text | "Hello, welcome to Eleven Labs!" |
voice | "en_us_male" |
speed | 1.0 |
pitch | 1.0 |
By using the Eleven Labs Text to Speech API, you can create dynamic, engaging audio experiences in your applications. The versatility of the service makes it an ideal choice for developers looking to enhance user interaction with speech synthesis.
Setting Up the Eleven Labs Text-to-Speech API in Your Project
Integrating Eleven Labs Text-to-Speech API into your project allows you to transform text into natural-sounding speech. This process is quite straightforward, but it requires a few essential steps to configure everything correctly. Follow the steps below to successfully integrate the API into your application.
Before diving into the setup, ensure you have an active Eleven Labs account and access to the API key. This key is crucial for authenticating requests to their API. Once you have it, you are ready to begin the integration process.
Step-by-Step Setup Guide
- Install Required Libraries
- For Python: Use
pip install requests
to get started with the required HTTP library. - For Node.js: Use
npm install axios
to install the necessary package.
- For Python: Use
- Obtain Your API Key
Log into your Eleven Labs account, navigate to the API section, and generate a unique API key. This key will authenticate all requests you make.
- Make the API Request
Once you have the necessary libraries and the API key, the next step is to configure and send the text-to-speech request. Here's an example of how to make a basic API call:
import requests api_key = 'your_api_key_here' url = 'https://api.elevenlabs.io/v1/text-to-speech' headers = { 'Authorization': f'Bearer {api_key}', 'Content-Type': 'application/json' } data = { 'text': 'Hello, welcome to Eleven Labs!', 'voice': 'en_us_male', 'speed': 1.0 } response = requests.post(url, headers=headers, json=data) audio_url = response.json().get('audio_url')
- Handle the Audio Response
The API will return a URL with the generated audio file. Use this URL to play the audio or download it as per your application needs.
Important Notes
Ensure that the API key is kept secure. Never expose it in public repositories or front-end code.
API Request Example
Parameter | Value |
---|---|
text | Text you want to convert to speech |
voice | Specify the voice model (e.g., en_us_male) |
speed | Control the speed of speech (e.g., 1.0) |
Exploring Customization Features in Eleven Labs' Voice Output API
The Eleven Labs API offers a wide range of customization options for tailoring voice output to specific needs. Users can adjust various voice attributes to create a unique auditory experience. From altering the tone and pitch to setting specific emotional inflections, the API provides flexibility to meet different use cases, including personal assistants, virtual characters, and accessibility tools. These customizations allow developers to enhance the engagement and realism of their applications significantly.
In this exploration, we will break down the most important features and settings available in the Eleven Labs API, as well as practical examples of how they can be applied. The following sections will outline voice characteristics that can be modified and provide insight into how they improve the overall output quality.
Voice Modifications Available in the API
- Pitch Adjustment: Control the pitch of the voice output, which can range from low to high frequencies, providing a natural or more robotic sound.
- Speed Control: Alter the speaking rate, making the voice faster or slower based on the context of the application.
- Emotion and Tone: Implement specific emotional tones (e.g., happy, sad, angry) that can drastically change how the speech is perceived.
- Accent and Language Selection: Choose from a variety of accents and languages to ensure the voice matches the regional and linguistic requirements of the audience.
Practical Application of Voice Settings
- Customer Support Systems: Adjust the tone and pace of speech to sound professional or empathetic depending on customer needs.
- Personal Assistants: Customize the voice's emotional range to create a more engaging interaction that reflects the user’s preferences.
- Language Learning Apps: Offer a variety of accents and speech speeds to help learners understand regional variations and improve pronunciation.
Key Features and Settings
Feature | Description |
---|---|
Voice Style | Ability to choose from a variety of voices with different characteristics (e.g., formal, casual, robotic). |
Volume Control | Set the volume level of speech output to suit different environments. |
Emotion Intensity | Modify the strength of emotions conveyed in the voice, ranging from neutral to highly expressive. |
The customization options provided by Eleven Labs empower developers to fine-tune voice interactions, ensuring that the end-user experience is as natural and contextually relevant as possible.
Integrating Eleven Labs API with Popular Programming Languages
The Eleven Labs API provides powerful text-to-speech capabilities, allowing developers to integrate voice synthesis into their applications seamlessly. With support for multiple programming languages, it offers flexibility for a wide range of use cases, from mobile apps to web services. This guide explores how to integrate Eleven Labs API into popular languages such as Python, JavaScript, and Java, highlighting the necessary steps and key considerations.
By using Eleven Labs API, developers can generate high-quality speech from text using advanced machine learning models. Below are the basic integration steps for different programming languages to help you get started quickly and efficiently.
Python Integration
Python is a great choice for integrating the Eleven Labs API due to its simplicity and extensive libraries. To get started, you need to install the requests library and authenticate with your API key. Below is an example of how to make a simple API request.
import requests api_url = "https://api.elevenlabs.io/v1/text-to-speech" headers = {"Authorization": "Bearer YOUR_API_KEY"} data = {"text": "Hello, how are you?", "voice": "en_us_male"} response = requests.post(api_url, headers=headers, json=data) if response.status_code == 200: with open("output.wav", "wb") as file: file.write(response.content)
JavaScript Integration
JavaScript is commonly used for web applications. The following example shows how to integrate Eleven Labs API using JavaScript's Fetch API for making HTTP requests.
const apiUrl = 'https://api.elevenlabs.io/v1/text-to-speech'; const apiKey = 'YOUR_API_KEY'; const data = { text: 'Hello, this is a test.', voice: 'en_us_female' }; fetch(apiUrl, { method: 'POST', headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' }, body: JSON.stringify(data) }) .then(response => response.blob()) .then(audioBlob => { const audioUrl = URL.createObjectURL(audioBlob); const audio = new Audio(audioUrl); audio.play(); }) .catch(error => console.error('Error:', error));
Java Integration
Java can be used to interact with Eleven Labs API using libraries like HttpURLConnection. Below is an example demonstrating the process of calling the API and saving the response as an audio file.
import java.io.*; import java.net.*; import javax.net.ssl.HttpsURLConnection; public class TextToSpeech { public static void main(String[] args) throws IOException { String apiUrl = "https://api.elevenlabs.io/v1/text-to-speech"; String apiKey = "YOUR_API_KEY"; String text = "Welcome to Eleven Labs API!"; String voice = "en_us_male"; URL url = new URL(apiUrl); HttpsURLConnection connection = (HttpsURLConnection) url.openConnection(); connection.setRequestMethod("POST"); connection.setRequestProperty("Authorization", "Bearer " + apiKey); connection.setRequestProperty("Content-Type", "application/json"); connection.setDoOutput(true); String jsonData = "{\"text\": \"" + text + "\", \"voice\": \"" + voice + "\"}"; try (OutputStream os = connection.getOutputStream()) { byte[] input = jsonData.getBytes("utf-8"); os.write(input, 0, input.length); } try (InputStream is = connection.getInputStream(); FileOutputStream fos = new FileOutputStream("output.wav")) { byte[] buffer = new byte[4096]; int bytesRead; while ((bytesRead = is.read(buffer)) != -1) { fos.write(buffer, 0, bytesRead); } } } }
Key Considerations
- API Key Authentication: Ensure that the correct API key is used for all requests.
- Voice Selection: Different voices are available, each with unique characteristics, so experiment to find the one that best suits your application.
- Error Handling: Implement error handling in your code to manage scenarios such as network issues or invalid input.
Response Data
The API will return an audio file containing the generated speech. The response can vary based on the input parameters such as voice choice, language, and text content.
Parameter | Description |
---|---|
text | The text to be converted into speech. |
voice | The voice model used to generate the speech. |
audio_format | The format of the returned audio (e.g., MP3, WAV). |
Important: Always handle the API responses securely and make sure to store API keys and sensitive data safely.
Understanding the Pricing Structure of Eleven Labs Text to Speech API
The pricing model of Eleven Labs' text-to-speech service is designed to provide flexibility for both small-scale developers and large enterprises. The structure is tiered to accommodate different usage levels, from casual users to those requiring extensive use. It is essential to understand these tiers to avoid unexpected costs and optimize usage based on specific needs.
Pricing is determined based on a combination of factors, such as the number of characters processed, the frequency of API calls, and any additional premium features or voices utilized. By breaking down these variables, users can better estimate their expenses and choose the right plan that fits their project requirements.
Pricing Tiers and Features
- Free Tier: Limited to 5,000 characters per month. Ideal for testing and personal projects.
- Basic Plan: $5 per month for up to 50,000 characters with access to standard voices.
- Advanced Plan: $20 per month for up to 500,000 characters with premium voice options.
- Enterprise Plan: Custom pricing based on volume and additional enterprise-specific features.
Usage and Cost Breakdown
Tier | Monthly Characters | Cost | Voice Options |
---|---|---|---|
Free | 5,000 | $0 | Standard |
Basic | 50,000 | $5 | Standard |
Advanced | 500,000 | $20 | Premium |
Enterprise | Custom | Custom | Premium + Custom |
The pricing structure offers clear tiers based on usage volume, allowing users to choose a plan that aligns with their needs. Larger projects or businesses may require customized enterprise pricing.
Fine-Tuning Voice Parameters for Natural-Sounding Speech
When using a Text-to-Speech API like Eleven Labs, fine-tuning voice parameters is essential to achieving a more lifelike and engaging output. The platform provides a set of customizable settings that allow you to adjust the tone, speed, pitch, and other factors that influence how the synthesized voice sounds. These parameters can be modified in various ways to ensure the voice output matches your desired style and clarity.
By adjusting the following key voice attributes, you can create a more natural and expressive sound. Fine-tuning involves balancing between speech rate, volume, and timbre while maintaining natural pauses and intonation.
Key Parameters for Fine-Tuning
- Pitch - Adjust the pitch to make the voice sound higher or lower, depending on the emotional tone you want to convey.
- Speed - Speed determines the rate of speech. A faster rate can convey urgency, while a slower rate can enhance clarity and attention.
- Volume - This controls the loudness of the speech. Moderate adjustments can prevent the voice from sounding too soft or too harsh.
- Emphasis - Emphasizing specific words can mimic human speech patterns and make the speech feel more expressive.
How to Adjust Parameters
- Select the Voice: Choose a voice model that suits your application (male, female, neutral, etc.).
- Set Pitch and Speed: Fine-tune the pitch to suit the context (e.g., a professional tone vs. a friendly tone). Adjust the speed to match the pacing needed for your message.
- Test and Iterate: Generate a sample output and listen for areas that need refinement. Make incremental changes to one parameter at a time to avoid overwhelming the listener.
- Optimize for Context: Consider the tone and emotions the voice should convey, adjusting parameters for different scenarios (e.g., casual conversation vs. formal presentation).
Fine-tuning voice parameters is an iterative process. Regular testing helps ensure the speech output sounds natural and consistent.
Example of Parameter Settings
Parameter | Recommended Range | Use Case |
---|---|---|
Pitch | 1.0 - 1.5 | Higher for cheerful tone, lower for serious tone |
Speed | 0.8x - 1.2x | 1.0x for normal speech, slower for clarity |
Volume | Medium (50-70%) | Ensure audibility without distortion |
Emphasis | Low to Medium | Apply emphasis to important words or phrases |
Optimizing API Requests for Faster Response Times with Eleven Labs
When working with Eleven Labs' Text to Speech API, improving response times is crucial for maintaining a smooth user experience. The faster the API can process requests, the more seamless the integration will be. By optimizing the way requests are made, developers can reduce latency, increase efficiency, and deliver faster results for end-users.
Several strategies can be employed to optimize API calls. These include minimizing data transmission, using caching techniques, and implementing request batching. Understanding the architecture of Eleven Labs' API and applying best practices for efficiency can significantly improve performance.
Key Optimization Techniques
- Request Batching: Instead of making multiple individual requests, batch them together to reduce overhead and increase efficiency.
- Data Compression: Compress request and response data to decrease the amount of data transferred, speeding up processing time.
- Effective Error Handling: Ensure that errors are quickly identified and handled to avoid unnecessary retries, which can delay the overall response.
- Optimize Audio Parameters: Minimize the length and complexity of audio output to reduce the processing load on the API.
Best Practices for API Integration
- Use asynchronous calls when possible to prevent blocking the main application flow.
- Cache frequent requests locally to avoid redundant API calls.
- Monitor and log API performance regularly to identify bottlenecks.
- Utilize throttling to manage load and ensure stable API performance.
Important Considerations
Minimizing Latency: Reducing the size of API requests and responses, as well as optimizing the structure of data sent, plays a crucial role in minimizing latency.
Technical Comparison
Optimization Method | Benefit |
---|---|
Request Batching | Reduces the number of API calls, enhancing throughput and reducing processing time. |
Data Compression | Speeds up data transfer by reducing the size of requests and responses. |
Error Handling | Prevents unnecessary retries, ensuring faster response times and fewer errors. |
Audio Parameter Optimization | Reduces processing load and improves API response time by simplifying audio output requirements. |
Best Practices for Error Handling and Troubleshooting with Eleven Labs API
When working with the Eleven Labs API, effective error handling is critical to ensuring smooth and uninterrupted integration. Developers need to be prepared for various issues, such as network failures, authentication errors, or unexpected responses from the API. Addressing these errors promptly helps maintain a robust user experience and enables quick resolution of problems during development and production phases.
In order to troubleshoot effectively, it is essential to follow a systematic approach. Identifying common error codes, understanding their causes, and implementing the correct mitigation strategies can save time and reduce frustration. Below are best practices to help you handle errors and debug issues efficiently when working with the Eleven Labs API.
Error Identification and Common Issues
- Authentication Failures: If you receive authentication errors (e.g., 401 Unauthorized), verify your API key and ensure it is active and properly configured in your requests.
- Quota Exceeded: Make sure that you have not surpassed the API usage limits. If your account has a quota, check your remaining usage and consider upgrading your plan if necessary.
- Rate Limiting: Frequent requests can trigger rate limiting. Ensure you are not exceeding the number of allowed requests per minute, as this may lead to a 429 status code.
- Malformed Requests: Double-check the structure and data format of your requests. Ensure that all required fields are included and follow the API’s specifications.
Troubleshooting Steps
- Check API Response Codes: Always review the HTTP status codes returned by the API. A 200 response means success, while other codes like 400, 401, or 500 signal specific issues.
- Review Error Messages: Pay attention to any error messages provided in the response body. These messages usually contain vital information to diagnose the issue.
- Enable Logging: Make use of logging mechanisms to capture detailed information about the API requests and responses, helping identify where the failure occurs.
- Test with Sample Data: Use known good data in test cases to isolate whether the issue is data-related or system-related.
Useful Tools for Troubleshooting
Tool | Description |
---|---|
Postman | Helps in manually testing API requests and responses, allowing you to check status codes, headers, and response bodies. |
cURL | A command-line tool for testing HTTP requests. It’s especially useful for debugging network-related issues. |
API Logs | Review detailed logs generated by the API to track every request, response, and error message for better troubleshooting. |
Note: Always ensure your API keys are kept secure and are not exposed in public repositories. Rotate keys regularly for enhanced security.