Ai Voice Assistant Maker
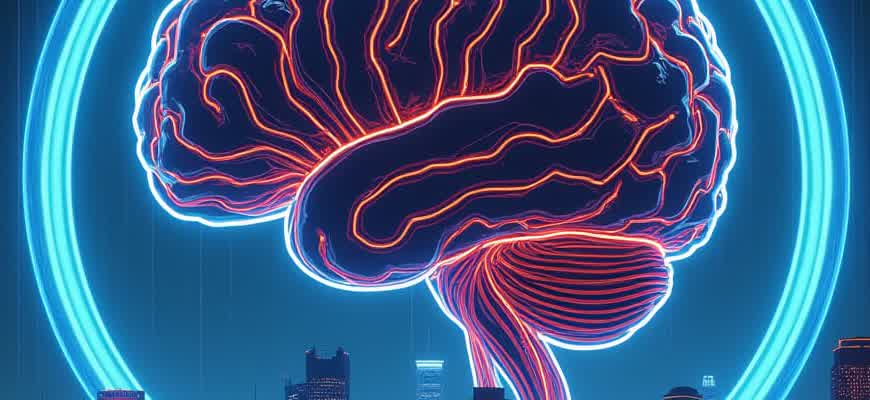
Developing an AI-based voice assistant involves several critical components that work in unison to provide a seamless user experience. The process starts with understanding the essential building blocks such as natural language processing (NLP), speech recognition, and machine learning algorithms. These technologies enable the assistant to interpret and respond to voice commands effectively.
Core Technologies:
- Natural Language Processing (NLP)
- Speech Recognition
- Machine Learning Models
- Text-to-Speech (TTS) Systems
- Dialog Management
In order to create an effective voice assistant, it's crucial to integrate a robust NLP system capable of understanding and processing human speech nuances.
Development Tools and Platforms:
- Google Dialogflow
- Amazon Lex
- Microsoft Azure Bot Service
- Rasa Open Source
- IBM Watson Assistant
The combination of these technologies and tools lays the foundation for a functional voice assistant capable of handling complex user interactions.
Key Considerations for Development:
Consideration | Importance |
---|---|
Accuracy | Critical for effective understanding of voice commands |
Speed | Important for real-time response and user satisfaction |
Security | Ensures privacy and prevents unauthorized access |
AI Voice Assistant Maker: A Practical Guide
Creating a voice assistant with AI technology requires a combination of machine learning, natural language processing (NLP), and integration with various APIs and services. The development process involves several steps, including designing the assistant's architecture, training the AI model, and implementing voice recognition. The outcome is a personalized voice assistant capable of handling tasks such as scheduling, reminders, or answering questions.
This guide provides an overview of the key steps involved in building your own AI-powered voice assistant. We will walk through the essentials of voice recognition, data processing, and how to integrate your assistant with different platforms for enhanced functionality.
Step-by-Step Approach to Building a Voice Assistant
- Define Core Features: Start by identifying the key tasks you want your assistant to handle, such as setting alarms, controlling IoT devices, or making phone calls.
- Choose the Right Tools: Select frameworks and APIs that suit your needs, such as Google's Dialogflow, Microsoft Azure, or Amazon Alexa SDK.
- Train the Model: Use machine learning algorithms and NLP models to enable your assistant to understand speech input and generate appropriate responses.
- Integrate with Platforms: Ensure compatibility with popular platforms like Android, iOS, and smart home devices.
- Test and Optimize: Continuously test the assistant’s performance and refine its responses for better accuracy.
Required Tools for Development
Tool | Functionality |
---|---|
Speech Recognition API | Converts voice input into text for processing. |
Natural Language Processing (NLP) | Analyzes the text and understands user intent. |
Text-to-Speech Engine | Generates voice responses for the assistant. |
Cloud Integration | Enables data storage and syncing across devices. |
Important: Continuously refine your assistant's conversational abilities by collecting feedback and using it to train the model further. Real-time adjustments based on user interactions are key to improving the assistant's effectiveness.
How to Choose the Right AI Voice Assistant for Your Business
Selecting the right AI-powered voice assistant is a critical decision for any business looking to enhance customer experience and streamline operations. With various options available, it can be challenging to know where to start. Understanding your business needs and the specific features of each AI assistant is key to making an informed choice. In this guide, we will outline several factors to consider when evaluating voice assistants for your company.
Before diving into technical specifications, it’s essential to define the primary objectives you want to achieve with the AI assistant. Whether you’re looking to automate customer service, improve internal workflows, or enhance user engagement, choosing a solution that aligns with your goals will ensure better performance and higher ROI.
Key Considerations When Choosing an AI Voice Assistant
- Scalability: Will the assistant be able to grow with your business? Consider its ability to handle increased traffic and expand its capabilities as your business evolves.
- Integration with Existing Systems: Check if the AI can seamlessly integrate with your CRM, helpdesk, and other business tools.
- Language and Localization: Ensure the assistant can support multiple languages if your business operates in different regions or has international clients.
- Security and Privacy: Look for features that protect sensitive customer data and comply with regulations such as GDPR.
- Customizability: Choose an AI assistant that can be tailored to your business's specific needs and branding.
Steps to Evaluate Your Options
- Identify Core Functions: Determine whether you need the AI to handle voice commands, perform tasks, or interact with customers in real-time.
- Test Voice Accuracy: Ensure the AI can accurately understand and process natural language input from a wide range of users.
- Check Support and Maintenance: Verify the availability of support and updates to ensure the assistant remains effective over time.
- Analyze Pricing Models: Evaluate the cost of the AI system, considering subscription fees, implementation costs, and ongoing maintenance.
"Choosing the right voice assistant is not just about technology; it’s about understanding how it can enhance your business processes and improve user experience."
Comparison of Popular AI Voice Assistants
Voice Assistant | Key Feature | Best For |
---|---|---|
Google Assistant | Natural language processing and integration with Google services | Businesses heavily using Google Workspace |
Amazon Alexa | Highly customizable skills and third-party integrations | Customer-facing applications and IoT solutions |
Microsoft Cortana | Integration with Microsoft 365 suite | Internal team collaboration and productivity |
How to Build Your Own Voice Assistant: A Step-by-Step Guide
Creating a personalized voice assistant can seem like a daunting task, but by breaking down the process into manageable steps, anyone can develop a fully functional system. Whether you're looking to build a simple assistant for basic tasks or a more complex one for custom functions, having a clear roadmap is essential.
The process of developing a voice assistant involves multiple stages, including gathering resources, setting up the development environment, and implementing voice recognition and natural language processing (NLP). This guide will walk you through each step, providing you with the foundation to build your own voice assistant from scratch.
Step-by-Step Process
The development process can be broken down into the following key stages:
- Gather Your Tools and Resources
- Speech recognition library (e.g., Google Speech API, CMU Sphinx)
- Natural language processing (NLP) library (e.g., spaCy, NLTK)
- Text-to-Speech engine (e.g., Google Text-to-Speech, pyttsx3)
- Programming language (usually Python, but JavaScript and others are also possible)
- Set Up the Development Environment
- Install Python 3.x
- Use pip to install speech recognition and NLP libraries
- Set up the microphone and ensure that audio input is working
- Implement Voice Recognition
- Implement Natural Language Processing (NLP)
- Text-to-Speech (TTS) Integration
- Testing and Iteration
Before diving into the technical aspects, ensure you have the necessary tools and software libraries:
Install all the necessary packages and dependencies. Here’s a quick guide to setting up:
This is the part where the assistant listens to your commands. You'll need to choose a speech recognition engine that suits your needs. Below is an example of how you can initialize the engine:
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Say something...")
audio = recognizer.listen(source)
try:
text = recognizer.recognize_google(audio)
print(f"Recognized text: {text}")
except sr.UnknownValueError:
print("Sorry, I could not understand the audio")
except sr.RequestError as e:
print(f"Could not request results; {e}")
After recognizing speech, the assistant must interpret the meaning of the words. NLP allows the assistant to break down sentences and understand commands. Use libraries like spaCy or NLTK to process the input text:
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp(text)
for token in doc:
print(token.text, token.pos_)
Once the assistant processes the command, it must respond. This is where the text-to-speech engine comes in. Below is an example using pyttsx3:
import pyttsx3
engine = pyttsx3.init()
engine.say("Hello, how can I assist you today?")
engine.runAndWait()
Test the system with different voice commands and fine-tune your algorithms to improve accuracy and functionality.
Note: Continuously improve the assistant’s NLP capabilities as more advanced techniques become available, such as deep learning models like GPT-3 or BERT, to better understand context and intent.
Resources for Further Learning
Library | Purpose | Documentation Link |
---|---|---|
SpeechRecognition | Converts speech to text | Link |
spaCy | Natural Language Processing | Link |
pyttsx3 | Text to speech conversion | Link |
Integrating Voice Interaction Features into Your Web or Mobile Platform
Adding a voice assistant to your website or mobile app can enhance user experience, providing a more interactive and accessible interface. By integrating voice recognition, you allow users to navigate, make inquiries, and execute tasks hands-free, which can be particularly useful for accessibility or multitasking scenarios. Furthermore, the voice assistant can offer personalized interactions based on previous user behavior, creating a dynamic experience that adjusts to individual needs.
To integrate voice functionality into your digital platform, a few technical elements and tools need to be considered. Whether using pre-built services or developing a custom solution, the integration process involves speech recognition, voice synthesis, and continuous feedback mechanisms to ensure smooth and efficient operation.
Key Steps for Successful Voice Assistant Integration
- Choose a Voice Recognition API: Select an API that matches the language capabilities and integration ease needed for your platform.
- Integrate Speech-to-Text and Text-to-Speech Functions: Ensure your platform can process spoken input and respond with natural-sounding speech.
- Set Up Natural Language Processing (NLP): Implement NLP to enable the assistant to understand and process user queries accurately.
- Develop User Interaction Flows: Design and test the user journey to ensure voice commands are intuitive and efficient.
Important Considerations When Adding Voice Features
Privacy and Data Security: Always make sure to implement strong encryption and compliance with data protection regulations when handling voice data.
- Ensure clear consent from users regarding data usage for voice interactions.
- Protect sensitive user information by securing voice data transmissions.
- Provide users with control over their voice data, including options to delete or manage it.
Technical Specifications
Technology | Description | Examples |
---|---|---|
Voice Recognition APIs | Platforms for converting speech into text for understanding user commands. | Google Cloud Speech-to-Text, IBM Watson Speech to Text |
Speech Synthesis | Converts written text into human-like speech for assistant responses. | Google Cloud Text-to-Speech, Amazon Polly |
Natural Language Processing (NLP) | Allows the system to comprehend and generate contextually relevant responses. | Dialogflow, Microsoft LUIS |
Enhancing Voice Recognition for Multiple Accents and Languages
When building AI-powered voice assistants, it's crucial to ensure they are capable of accurately understanding diverse accents and languages. A robust system should adapt to various speech patterns, regional pronunciations, and linguistic nuances, providing a seamless experience for users worldwide. This not only improves the assistant’s usability but also broadens its accessibility across different demographic groups.
Optimizing speech recognition for a global audience requires a multi-faceted approach that includes training the system on diverse datasets, incorporating machine learning algorithms, and fine-tuning the model based on feedback from real-world usage. Furthermore, supporting multiple languages and accents can be achieved through careful language model development and continuous adaptation to new speech data.
Key Steps for Optimizing Voice Recognition Across Languages and Accents
- Data Collection: Gather a diverse range of voice samples from different accents, dialects, and languages to train the recognition model.
- Continuous Model Training: Regularly update the language models to include new vocabulary, slang, and variations in speech patterns.
- Adaptive Algorithms: Implement algorithms that adjust to individual user voices, improving accuracy over time with machine learning.
- Localized Pronunciation Tuning: Tailor the voice assistant to understand regional pronunciations, such as tonal differences or unique phonetic sounds.
Important Considerations for Accent and Language Optimization
Inclusivity and Accessibility: Ensure that all users, regardless of accent or language, have a
Enhancing User Interaction through Personalized Voice Experiences
As voice assistants become a crucial part of user interaction, offering tailored responses plays a pivotal role in maintaining user interest. Customizable voice features allow users to engage in a more dynamic and personalized manner. This leads to a more immersive and satisfying interaction, as users feel that the assistant is responding specifically to their needs and preferences.
Adapting voice assistants to reflect individual user choices can significantly increase user satisfaction. By offering various voice styles, tones, and even accents, the experience becomes more relatable and enjoyable. This approach not only builds a deeper connection with the technology but also encourages frequent usage, ensuring that the assistant remains a valuable part of daily routines.
Key Benefits of Customizable Voice Interactions
- Personalization: Tailoring voice responses to match user preferences improves the sense of ownership.
- Increased Engagement: A more relatable voice style keeps users invested in their interaction.
- Enhanced User Comfort: Adjusting tone or speech patterns helps reduce the feeling of talking to a machine.
- Better Context Awareness: Personalized voices can reflect a deeper understanding of context, making responses more relevant.
Customizable Features to Enhance Engagement
- Voice Tone and Style: Users can choose from various tones (e.g., formal, friendly, or casual).
- Accent Options: Adding regional accents increases relatability for diverse user groups.
- Speech Speed: Allowing users to adjust speech speed helps accommodate different listening preferences.
- Emotion Simulation: Adding emotional cues to voice responses creates a more human-like experience.
"Personalized voice assistants not only meet the needs of individual users but also make the technology feel more intuitive and responsive, leading to higher user satisfaction and loyalty."
Comparison of Customizable Voice Features
Feature Impact on User Engagement Voice Tone Adjustment Increases relatability and emotional connection Accent Customization Improves accessibility and user comfort Speed Control Accommodates various listening preferences Emotion Simulation Enhances the conversational nature of the interaction Analyzing Data to Fine-Tune Your Voice Assistant's Performance
Improving the functionality of a voice assistant involves careful examination of the data it generates. This data is critical to understanding how well the assistant interacts with users and identifying areas that require optimization. Analyzing user input, feedback, and system performance helps to fine-tune the assistant’s responses, accuracy, and overall efficiency. This process can significantly enhance user satisfaction and streamline interaction with the system.
Data analysis can be conducted through various techniques, such as tracking user behavior, measuring voice recognition accuracy, and evaluating system responses. By collecting data on these parameters, developers can adjust machine learning models, refine natural language processing (NLP) capabilities, and address specific pain points that users encounter. Below are key steps for analyzing data effectively:
Key Steps in Data Analysis
- Collect User Interactions: Gather data on voice commands, queries, and feedback to analyze how users interact with the assistant.
- Measure Accuracy: Assess the precision of speech recognition, detecting misinterpretations, and errors in understanding.
- Evaluate System Responses: Review how the assistant responds to queries and the relevance of the answers provided.
- Identify Patterns: Look for recurring issues or feedback trends to target areas needing improvement.
Once the data has been analyzed, actionable insights can be extracted. This allows for targeted adjustments to enhance the assistant’s capabilities and performance. For example, improving NLP algorithms can lead to better comprehension of varied speech patterns, while optimizing speech synthesis can make responses sound more natural.
By focusing on detailed data analysis, developers can continuously refine the assistant's capabilities, ensuring it meets the evolving needs of users.
Tools for Data Analysis
Tool Purpose Speech Analytics Software Tracks and analyzes speech data for accuracy and errors. Natural Language Processing (NLP) Tools Improves the understanding and generation of human language. User Feedback Surveys Collects direct feedback to understand user satisfaction. Ensuring Privacy and Security in Voice Assistant Applications
As voice assistant technologies continue to evolve, ensuring the privacy and security of user data is critical. Many voice-enabled applications collect vast amounts of personal information, making it essential to implement robust measures to protect this data. These measures must address both the potential for unauthorized access to sensitive data and the risks of data misuse by malicious entities.
Voice assistants can gather private information such as speech patterns, location, and personal preferences, which could be exploited if not properly secured. Implementing strict protocols for data handling and storage is essential to mitigate potential vulnerabilities. Secure encryption methods and careful management of user consent are the foundations of a secure voice assistant ecosystem.
Key Privacy and Security Measures
- Data Encryption: Encrypting data during transmission and at rest ensures that unauthorized individuals cannot access sensitive information.
- User Authentication: Voice biometrics or two-factor authentication can prevent unauthorized access to voice assistants and user accounts.
- Minimal Data Collection: Only essential data should be collected, ensuring that unnecessary personal information is not stored or transmitted.
Best Practices for Developers
- Ensure Transparency: Always inform users about the type of data collected and its intended use.
- Allow User Control: Provide users with the ability to view, delete, or limit the data stored by the assistant.
- Implement Secure APIs: Secure API connections prevent third parties from gaining unauthorized access to voice assistant services.
“Incorporating security features in voice assistants is not just about protecting data but about building user trust and ensuring long-term adoption of the technology.”
Table: Common Privacy and Security Risks
Risk Impact Prevention Measures Unauthorized Access Exposure of personal data Use of strong encryption and two-factor authentication Data Breach Compromise of sensitive information Regular security audits and data masking techniques Voice Spoofing Impersonation and misuse of the system Voice recognition systems and anti-spoofing measures Cost Breakdown: Building an AI Voice Assistant
Building an AI voice assistant involves a complex set of components that require both financial investment and technical expertise. The total cost can vary significantly depending on the scope of the project, the complexity of the assistant, and the desired functionalities. Typically, the costs include development, integration, testing, and ongoing maintenance. Below is a breakdown of key expenses to consider when building an AI-powered voice assistant.
To understand the true cost, it's essential to break down the project into its core components. The initial phase involves designing the system architecture and the natural language processing (NLP) algorithms, followed by training the AI models and integrating them with speech recognition and synthesis systems. Furthermore, the ongoing costs for hosting, data processing, and model updates should not be overlooked.
Key Cost Components
- Development and Design: This includes the cost of hiring developers, AI specialists, and UX designers who will create the voice interface and its backend systems.
- Natural Language Processing (NLP) Engine: Licensing or developing a high-quality NLP engine is often a significant cost. Popular choices like Google Cloud Speech or IBM Watson come with subscription fees.
- Speech Recognition and Synthesis: This involves integrating APIs for converting speech into text and vice versa. Cloud providers charge based on the volume of audio processed.
- Cloud Infrastructure: Hosting the service on cloud platforms like AWS or Google Cloud will incur costs for server storage, bandwidth, and computing power.
Ongoing Expenses
- Maintenance and Updates: Regular updates to improve accuracy and expand the assistant's capabilities will require ongoing investment in both human and technical resources.
- Data Management: Gathering, storing, and processing data to continuously train and refine the assistant's models adds to the cost.
- Security and Compliance: Ensuring the voice assistant meets privacy and security standards (such as GDPR or HIPAA compliance) can incur additional costs.
Estimated Costs Overview
Component Estimated Cost Range Development and Design $50,000 - $200,000 NLP Engine Licensing $10,000 - $100,000 (annually) Speech Recognition & Synthesis API $0.01 - $0.10 per request Cloud Infrastructure $500 - $5,000 per month Maintenance and Updates $10,000 - $50,000 annually Important: Costs can significantly vary depending on the complexity of the project and the specific technologies chosen. Small-scale applications can be built with lower budgets, while advanced AI voice assistants with custom features will require higher investments.