Android Text to Speech Api Example
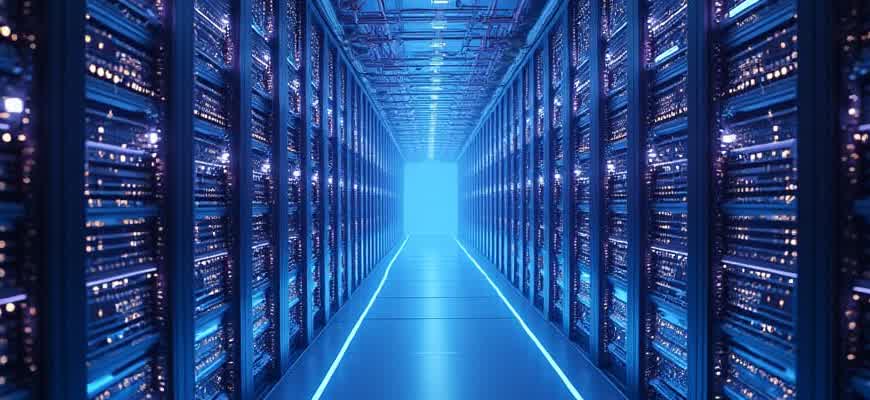
Android provides a built-in Text-to-Speech (TTS) API that allows developers to convert written text into spoken words. The process of integrating TTS into an Android application involves initializing the TTS engine, setting language preferences, and using the appropriate methods to trigger speech synthesis. Below is an example demonstrating how to integrate and use this API.
Steps for implementation:
- Initialize the TextToSpeech object.
- Set the desired language for speech synthesis.
- Trigger speech output by passing text to the TextToSpeech engine.
Important: The Text-to-Speech service requires network access for language downloading on some devices.
Code Example:
TextToSpeech tts = new TextToSpeech(this, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { int langResult = tts.setLanguage(Locale.US); if (langResult == TextToSpeech.LANG_MISSING_DATA || langResult == TextToSpeech.LANG_NOT_SUPPORTED) { Log.e("TTS", "Language not supported or missing data."); } else { tts.speak("Hello, this is a sample text-to-speech.", TextToSpeech.QUEUE_FLUSH, null, null); } } else { Log.e("TTS", "Initialization failed."); } } });
Parameters in the example:
Parameter | Description |
---|---|
TextToSpeech.SUCCESS | Indicates that the TTS engine has been successfully initialized. |
Locale.US | Specifies the language used for speech output (in this case, English). |
TextToSpeech.QUEUE_FLUSH | Indicates that the speech queue should be cleared before playing new speech. |
Android Text to Speech API: Practical Implementation Guide
Text-to-Speech (TTS) functionality is a vital feature for many Android applications, allowing for the conversion of text into audible speech. The Android Text to Speech API provides developers with a simple and effective way to integrate TTS into their apps, making them more interactive and accessible. This guide walks through the setup and use of the Android Text to Speech API with practical steps.
In this example, we will cover the essential aspects of initializing the TTS engine, converting text to speech, handling lifecycle events, and managing settings. By the end of this guide, you will have a functional TTS implementation in your Android application.
Setting Up the Text to Speech Engine
Before using the TTS functionality, ensure that your Android app is set up to use the Text to Speech API. First, you'll need to initialize the TTS engine in your activity or fragment. Here's an overview of the essential steps:
- Import necessary classes.
- Initialize the TextToSpeech object.
- Set the TTS language.
- Handle the lifecycle events like onInit() to check if the TTS engine is ready.
Important: Always check for the TextToSpeech.SUCCESS status in onInit() to ensure the engine is properly initialized.
Code Example: Basic Setup
Here's a simple example of initializing and using TTS in an Android app:
TextToSpeech tts = new TextToSpeech(this, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { int langResult = tts.setLanguage(Locale.US); if (langResult == TextToSpeech.LANG_MISSING_DATA || langResult == TextToSpeech.LANG_NOT_SUPPORTED) { // Handle error } else { tts.speak("Hello, this is an example of text to speech!", TextToSpeech.QUEUE_FLUSH, null, null); } } } });
Important Considerations
- Resource Management: Always release the TTS object in the onDestroy() method to free up system resources.
- Language Support: Check if the desired language is supported on the device.
- Speech Rate and Pitch: Adjust the speech rate and pitch for better user experience.
Note: Be mindful of device limitations. Some devices might not support certain languages or voice types.
Advanced Settings: Speech Rate and Pitch
To provide a more customized experience, you can adjust the speech rate and pitch. These settings influence how the speech sounds, providing either a faster or slower pace, or a higher or lower tone.
Setting | Range |
---|---|
Speech Rate | 0.5 (slow) to 2.0 (fast) |
Pitch | 0.5 (low) to 2.0 (high) |
Setting Up Android Text to Speech API in Your Project
To integrate the Text to Speech (TTS) functionality into your Android app, the first step is to ensure that your project is properly configured to use the Android Text to Speech API. The process involves adding the necessary dependencies, initializing the TTS engine, and handling any required permissions for smooth operation.
Follow these steps to successfully implement TTS in your Android application:
1. Add Required Dependencies
To begin with, you need to include the TTS library in your project. This can be done by adding the appropriate dependencies to your project’s `build.gradle` file. There is no need for an additional external library as Android provides built-in support for TTS.
- Ensure that your project is targeting API level 21 or higher.
- Open the `build.gradle` file of your app and check for compatibility.
- Make sure the minSdkVersion is set to at least 21 for full support.
2. Initialize the TTS Engine
After ensuring that the dependencies are set up correctly, the next step is to initialize the TTS engine in your activity. The engine can be initialized in the `onCreate` method of your activity.
- Create an instance of TextToSpeech.
- Override the onInit() method to check if the TTS engine has initialized successfully.
- Pass the appropriate Locale parameter to set the desired language.
Important: Always handle the initialization asynchronously, as it may take time for the TTS engine to load and be ready for use.
3. Check Permissions
Make sure that your app has the necessary permissions to use the Text to Speech API. In some cases, you may need to request permissions dynamically at runtime.
Permission | Required |
---|---|
INTERNET | No, unless you’re using a network-based voice service. |
ACCESS_NETWORK_STATE | No, for offline TTS use only. |
Integrating Text to Speech API with Custom Speech Settings
The Text-to-Speech (TTS) API in Android allows developers to convert text into spoken words. However, to create a more personalized and engaging user experience, it is essential to customize the speech settings according to the application’s requirements. Android provides several ways to modify voice parameters such as pitch, speed, and language preferences, which can significantly enhance the interaction for the users. This section explores how to integrate custom speech settings into the TTS API to achieve better results.
By customizing the speech settings, developers can control the voice quality, rate, and pitch of the generated speech. These features allow for better accessibility and the ability to create voices that are suitable for specific applications, such as navigation or accessibility apps. The following steps highlight how to implement and adjust these settings effectively within an Android application.
Customizing Speech Rate and Pitch
To customize the speech rate and pitch of the TTS, developers use the setSpeechRate() and setPitch() methods. These functions allow developers to define how fast or slow the speech should be and how high or low the voice tone should sound. Below is a simple example of how to integrate these settings:
- Set speech rate: This controls how quickly the speech is delivered.
- Set pitch: This controls the tone of the voice used for speech.
The example code snippet for customizing speech rate and pitch is as follows:
TextToSpeech textToSpeech = new TextToSpeech(context, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { textToSpeech.setLanguage(Locale.US); textToSpeech.setSpeechRate(1.0f); // Default speech rate textToSpeech.setPitch(1.0f); // Default pitch } } });
Choosing the Voice and Language
Android allows developers to choose from multiple available voices and languages, enhancing user experience by matching the application's context with the appropriate voice. To select a custom voice or language, developers can use the setLanguage() method to specify the desired locale, and setVoice() to select a specific voice variant.
- Select language: Use setLanguage() to define the language.
- Set voice: Use setVoice() to select a specific voice from the available options.
Example code to set language and voice:
textToSpeech.setLanguage(Locale.FRENCH); textToSpeech.setVoice(Voice.Name("fr-CA-latest")); // Set specific voice
Table of Common Speech Settings
Setting | Default Value | Range |
---|---|---|
Speech Rate | 1.0f | 0.1f to 2.0f |
Pitch | 1.0f | 0.5f to 2.0f |
Language | English (US) | Locale-based (e.g., en-US, fr-FR) |
Customizing TTS settings can improve accessibility for diverse user groups, especially when tailoring the voice to the context of the application. This ensures that the generated speech meets the expectations of the audience while providing a seamless and interactive experience.
Managing Multiple Languages with Android Text to Speech API
Android's Text to Speech (TTS) API provides a robust way to integrate speech synthesis into mobile applications, supporting various languages. This flexibility allows developers to cater to a global audience, offering localized experiences. However, handling multiple languages efficiently can pose challenges, such as selecting the appropriate voice or managing speech rate and pitch based on the language being spoken.
To handle multiple languages, developers must ensure that the system's language support is properly configured and that the TTS engine can switch between different languages seamlessly. Android allows setting the language before speaking a text, ensuring that the synthesized speech matches the intended language's phonetic structure and accent.
Setting Up the Correct Language
Before using TTS to speak in different languages, it is crucial to initialize the correct language for each text. The language can be set through the TTS engine with the `setLanguage()` method. Each language has a specific language code (e.g., "en_US" for English, "fr_FR" for French), which needs to be passed when switching languages.
- Initialize the TTS engine with a context.
- Use the setLanguage() method to define the language.
- Handle errors if the language is not supported on the device.
Note: Not all devices support every language. Always check if the language is available before attempting to use it with TextToSpeech.LANG_AVAILABLE.
Switching Between Languages Dynamically
Switching between languages dynamically can be done based on the user input or application context. Here’s a simple approach to toggle between different languages:
- Detect the user's preferred language or set it manually based on context.
- Before speaking, call setLanguage() with the appropriate language code.
- Handle language switching efficiently to avoid delay or errors during speech synthesis.
Supported Languages
Here is a table showing some common languages and their corresponding language codes:
Language | Language Code |
---|---|
English (US) | en_US |
Spanish | es_ES |
French | fr_FR |
German | de_DE |
Russian | ru_RU |
Managing Voice Speed and Pitch for Optimal Output
When working with the Android Text-to-Speech (TTS) API, adjusting the voice's speed and pitch is crucial to creating a more natural and engaging user experience. These settings allow for fine-tuning the speech output to meet specific requirements, whether for accessibility or aesthetic purposes. Proper management of these parameters can significantly improve clarity and make the spoken content easier to comprehend.
The TTS engine provides a way to modify both the rate of speech and its tonal quality. By changing the speed, users can control how fast or slow the speech is delivered, while altering the pitch adjusts the height of the voice. Finding the right balance between these factors can enhance user interaction, ensuring that the speech output sounds both clear and pleasant.
Adjusting Speed and Pitch
The TTS API allows developers to set both the speed and pitch of the voice programmatically. Below are the primary methods for adjusting these parameters:
- Speed: Controlled by the setSpeechRate(float rate) method, where values range from 0.1 to 2.0, with 1.0 as the default speed.
- Pitch: Adjusted using the setPitch(float pitch) method, where values range from 0.5 to 2.0, with 1.0 being the default pitch.
Fine-tuning these values is necessary for achieving an optimal output. A voice that is too fast or too high-pitched may become difficult to understand, while a slow or deep voice could sound monotonous or unnatural.
Recommended Ranges
Parameter | Recommended Range | Notes |
---|---|---|
Speech Rate | 0.8 to 1.2 | Adjust for clarity and listener comfort. |
Pitch | 0.9 to 1.1 | Maintain natural-sounding voices; avoid extremes. |
It is important to test different combinations of speed and pitch to ensure that the output is not only understandable but also comfortable for the user.
Detecting and Handling Errors in Text to Speech API
When integrating text-to-speech functionality into an Android app, handling potential errors is crucial to ensure a seamless user experience. The Android Text-to-Speech (TTS) API provides various mechanisms to detect issues and respond appropriately. This helps prevent crashes and ensures that the application remains responsive, even when errors occur.
Error detection is typically achieved through the use of listeners and callbacks, which allow developers to catch specific exceptions or failures during the TTS initialization or speech synthesis process. By implementing error handling, you can gracefully notify users about issues and provide alternatives when the speech synthesis cannot proceed as expected.
Common Errors and Their Handling
- Initialization Failures: When the TTS engine fails to initialize, it may be due to missing language data or system resource issues. It is important to check the availability of the required language using the
TextToSpeech.OnInitListener
callback. - Unsupported Language: If the specified language is not supported by the device, it is important to handle this error by either selecting a fallback language or notifying the user to install the required language pack.
- Speech Synthesis Errors: Errors during speech synthesis, such as the inability to process the input text, can occur. This can be handled by checking the synthesis status and responding accordingly.
Error Handling Strategies
- Check initialization status in the
onInit()
method. - Use
setOnUtteranceProgressListener()
to monitor the progress and catch errors during speech synthesis. - Provide fallback options, such as retrying the operation or offering text-based responses when speech fails.
Tip: Always ensure that your app provides feedback to users when an error occurs, to maintain a smooth user experience.
Error Handling Table
Error Type | Possible Cause | Recommended Action |
---|---|---|
Initialization Failure | Missing language data or system failure | Check language availability, prompt user to install missing data |
Unsupported Language | Language not installed on the device | Fallback to default language or prompt user to install required language |
Speech Synthesis Error | Text cannot be processed or spoken | Retry synthesis or notify the user with an alternative message |
Using Android Text to Speech API with Android Services
Integrating the Android Text to Speech (TTS) API with Android Services allows for creating applications that can handle speech synthesis in the background, providing a seamless user experience. This setup is particularly useful for applications like navigation, news reading, or accessibility tools, where the app needs to speak text while continuing to run other tasks without interruption. By using a Service, you ensure that speech synthesis can continue running even when the user navigates away from the app's UI.
Android Services provide an ideal environment for performing tasks that should run in the background. To implement TTS within a Service, you first need to initialize the TextToSpeech object and ensure that it is properly set up before speaking any text. This approach helps in preventing the UI thread from being blocked, ensuring a responsive application. Below is a basic example of how to implement TTS within a Service.
Steps to Implement Text to Speech in a Service
- Define a Service that extends Service.
- Initialize TextToSpeech inside the Service's onCreate() method.
- Override onStartCommand() to handle the speech input and output.
- Ensure proper lifecycle management by handling TTS initialization and shutdown in onDestroy().
Code Example
public class TTSService extends Service { private TextToSpeech textToSpeech; @Override public void onCreate() { super.onCreate(); textToSpeech = new TextToSpeech(this, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { textToSpeech.setLanguage(Locale.US); } } }); } @Override public int onStartCommand(Intent intent, int flags, int startId) { String textToRead = intent.getStringExtra("text"); if (textToRead != null && !textToRead.isEmpty()) { textToSpeech.speak(textToRead, TextToSpeech.QUEUE_FLUSH, null, null); } return START_NOT_STICKY; } @Override public void onDestroy() { if (textToSpeech != null) { textToSpeech.stop(); textToSpeech.shutdown(); } super.onDestroy(); } }
Important Considerations
Always ensure that the TextToSpeech instance is properly initialized and released when the service is destroyed. Failure to do so may result in memory leaks or unexpected behavior.
Key Points to Remember
- Use a Service to run TTS in the background without blocking the main thread.
- Properly manage the lifecycle of the TextToSpeech object to avoid memory issues.
- Ensure the app has necessary permissions, such as INTERNET and ACCESS_NETWORK_STATE, if the TTS engine requires network access.
Managing Text-to-Speech with Multiple Languages
Language | Locale | Status |
---|---|---|
English | Locale.US | SUCCESS |
Spanish | Locale("es", "ES") | SUCCESS |
French | Locale("fr", "FR") | SUCCESS |
Testing and Debugging Android Speech Output
When working with Android Text-to-Speech functionality, it's crucial to ensure that the speech output behaves as expected across different devices and scenarios. The testing process involves checking various aspects, such as voice clarity, speech speed, and appropriate language selection. Proper debugging techniques can help developers identify issues related to pronunciation, speech delays, or device-specific quirks. Understanding how to test and debug effectively will lead to a more polished user experience.
Debugging Text-to-Speech functionality can be tricky due to the wide range of voices, languages, and speech engines available. It's important to consider error handling, especially for situations where the system fails to initialize the speech engine or when certain voices are unavailable on the device. Below are some useful techniques for testing and troubleshooting.
Testing Output
- Test various languages and voices to ensure compatibility with the device's available options.
- Check the speech speed and pitch settings to make sure they are adjustable and functional.
- Verify that the system handles edge cases, such as empty strings or very short input, without crashing.
- Ensure that the speech output is synchronized with the intended application actions (e.g., buttons, UI elements).
Common Debugging Techniques
- Log errors related to the Text-to-Speech engine initialization to identify issues early.
- Use the Android logcat tool to view detailed logs when speech output does not work as expected.
- Ensure proper resource cleanup by calling shutdown() after the TTS service is no longer needed.
- Test on multiple devices and Android versions to catch device-specific issues.
Example of TTS Testing Table
Test Case | Expected Outcome | Result |
---|---|---|
Test with English voice | Clear, understandable speech output | Passed |
Test with French voice | Correct pronunciation in French | Failed |
Test with slow speech rate | Speech is clearly understandable at slower pace | Passed |
Tip: Always check for exceptions such as SpeechError to handle failures gracefully, especially when the engine is not available or there's an issue with network connectivity.
Optimizing Performance and Memory Usage in Speech Synthesis Applications
When developing speech synthesis applications, especially for Android devices, it is crucial to optimize both performance and memory usage to ensure smooth operation and minimal resource consumption. Proper optimization helps reduce processing time and prevents memory leaks, ensuring that the app functions efficiently across various devices with different capabilities.
In this context, developers must focus on several aspects such as reducing the overhead of TTS (Text-to-Speech) engine initialization, minimizing unnecessary resource allocations, and properly managing background tasks related to speech synthesis. Here are some best practices to help with performance and memory optimization:
Best Practices for Optimizing TTS Applications
- Lazy Initialization of TTS Engine: Avoid initializing the TTS engine at the start of the app. Instead, initialize it only when needed, and release resources as soon as they are no longer required.
- Speech Resource Management: Load only the required language packs or voices. Unnecessary voices can be unloaded to save memory.
- Speech Rate and Pitch Adjustments: Keep the speech rate and pitch within a reasonable range. Extreme values can put unnecessary load on the system, negatively impacting performance.
- Thread Management: TTS operations should run on background threads to avoid blocking the main UI thread. This ensures that the app remains responsive while performing speech synthesis tasks.
Techniques for Memory Management
- Releasing Resources After Use: After a TTS task is complete, ensure the TTS engine is properly shut down to release any system resources.
- Using Efficient Data Structures: Use memory-efficient data structures for managing text input. Avoid holding large strings or objects in memory for prolonged periods.
- Profiling and Testing: Regularly profile the application for memory leaks and performance bottlenecks using tools like Android Profiler.
"Managing memory efficiently in speech synthesis applications is key to ensuring that the app runs smoothly even on lower-end devices. Always release resources and adjust parameters to balance performance and memory usage."
Performance and Memory Optimization Table
Technique | Description |
---|---|
Lazy Initialization | Initialize TTS engine only when required, not at the app start. |
Speech Rate Control | Adjust speech rate to avoid overloading system resources. |
Memory Profiling | Regularly profile app memory usage to detect leaks and optimize resource usage. |