How to Use Google Speech to Text Api in Javascript
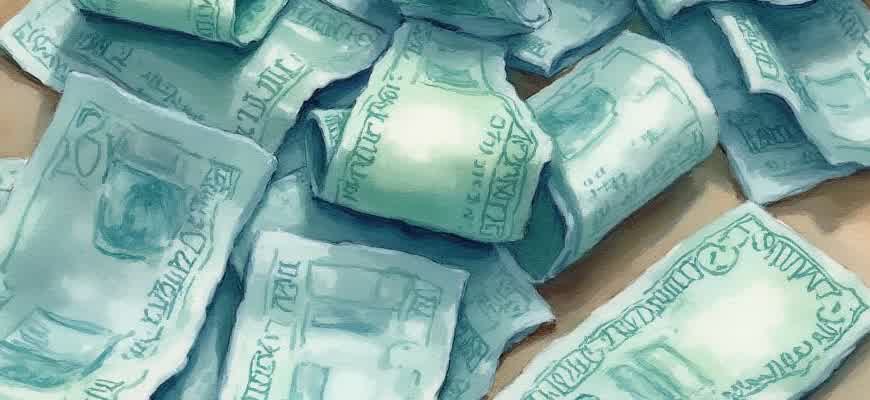
Integrating voice recognition into web applications can significantly enhance user interaction. Google Speech-to-Text API provides a powerful tool for real-time transcription and audio processing. Below is a step-by-step guide on how to get started with this service using JavaScript.
Prerequisites:
- Google Cloud account
- API key for Google Cloud Speech-to-Text
- Basic knowledge of JavaScript and web APIs
To begin, you must first enable the Google Cloud Speech-to-Text API in your Google Cloud Console. After that, you can start integrating the API into your web application.
Make sure to keep your API key secure, as unauthorized access could lead to service abuse.
Steps for integration:
- Install the necessary client libraries using npm or directly in your script.
- Set up authentication using the API key generated in your Google Cloud Console.
- Use the Speech-to-Text API to send audio data for transcription.
The process is straightforward, but be mindful of quotas and rate limits when using the API extensively in production environments.
Step | Action |
---|---|
1 | Install Google Cloud client library |
2 | Authenticate with API key |
3 | Call Speech-to-Text API with audio input |
Integrating Google Speech Recognition in JavaScript
Using the Google Speech to Text API in JavaScript allows developers to transcribe audio content into text in real-time. This powerful tool is beneficial for creating applications such as virtual assistants, voice commands, and accessibility features. To get started, you will need an API key and ensure that the Google Cloud Speech-to-Text API is enabled in your Google Cloud Console.
In this guide, we will walk through the process of using the API, setting up the necessary dependencies, and implementing a speech recognition system in JavaScript.
Steps to Use Google Speech to Text API
- Enable the Google Cloud Speech API in the Google Cloud Console and obtain your API key.
- Set up a Node.js environment with the necessary libraries such as @google-cloud/speech and express.
- Use JavaScript to send an audio file or stream directly to the Google Speech API for transcription.
Important: Be sure to authenticate properly using Google Cloud credentials. You may need to set the environment variable GOOGLE_APPLICATION_CREDENTIALS to the path of your service account key.
Basic Code Example
Here’s a basic example of how to send audio data to the API using JavaScript:
const fs = require('fs'); const speech = require('@google-cloud/speech'); const client = new speech.SpeechClient(); const filename = 'path_to_audio.wav'; const file = fs.readFileSync(filename); const audioBytes = file.toString('base64'); const audio = { content: audioBytes, }; const config = { encoding: 'LINEAR16', sampleRateHertz: 16000, languageCode: 'en-US', }; const request = { audio: audio, config: config, }; client.recognize(request) .then((responses) => { const transcription = responses[0].results .map(result => result.alternatives[0].transcript) .join('\n'); console.log(`Transcription: ${transcription}`); }) .catch((err) => { console.error('Error recognizing speech:', err); });
API Configuration Parameters
Parameter | Description |
---|---|
encoding | The audio encoding used for the input (e.g., LINEAR16, FLAC, etc.) |
sampleRateHertz | The sample rate of the audio in Hertz (e.g., 16000) |
languageCode | The language of the audio, typically 'en-US' for English |
Set Up Google Cloud Project and Enable Speech-to-Text API
To integrate Google’s Speech-to-Text functionality into your JavaScript project, the first step is to set up a Google Cloud Project. This will allow you to access the API and manage billing. The next step is to enable the Speech-to-Text API for your project and configure the necessary authentication settings.
Follow these steps to set up your project and enable the API:
- Visit the Google Cloud Console and log in with your Google account.
- Create a new project by clicking on the "Select a Project" dropdown and then "New Project". Provide a name and click "Create".
- Navigate to the "APIs & Services" dashboard, then click on the "Enable APIs and Services" button.
- Search for the "Speech-to-Text API" in the API Library and click "Enable".
- Next, go to "Credentials" to set up authentication. Create a service account key by selecting "Create Credentials" and choosing "Service Account".
- Download the JSON key file and securely store it, as it contains the credentials needed for your app to authenticate with Google’s servers.
Important: Always secure your service account key and avoid sharing it in public repositories or with unauthorized parties.
Once you’ve completed the setup, your project will be ready to use the Speech-to-Text API. The next step is to install the necessary libraries in your JavaScript environment and implement API calls to start transcribing audio.
Installing and Setting Up Google Cloud SDK for JavaScript
To start using Google Cloud services in your JavaScript application, you'll first need to set up the Google Cloud SDK. This SDK enables you to interact with various Google Cloud APIs, including the Speech-to-Text API. Below are the steps to install and configure the SDK on your local development environment.
The setup involves two main steps: installing the SDK and configuring it to interact with your Google Cloud project. These actions will allow you to access cloud resources and make API calls directly from your JavaScript application.
Steps to Install and Configure Google Cloud SDK
- Download the SDK installer from the official Google Cloud SDK installation page.
- Run the installer based on your operating system (Windows, macOS, Linux) and follow the on-screen instructions.
- Once installed, open the command-line interface and run
gcloud init
to configure the SDK.
Important: Ensure that you have a Google Cloud project with the Speech-to-Text API enabled. You can do this from the Google Cloud Console.
Configure API Access and Authentication
- Navigate to the Google Cloud Console and create a new project (or use an existing one).
- Enable the Speech-to-Text API under the "APIs & Services" section.
- Create a service account and download the JSON key file for authentication.
- Set the environment variable to point to your credentials file using the command:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/credentials-file.json"
Action | Command |
---|---|
Install SDK | curl https://sdk.cloud.google.com | bash |
Initialize SDK | gcloud init |
Set credentials file | export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/credentials-file.json" |
Tip: You can check the configuration by running
gcloud auth list
to confirm the active account.
How to Generate API Key and Authenticate Requests in JavaScript
To work with Google Speech-to-Text API, you first need to set up an API key and configure proper authentication. This process ensures that your requests are authorized to access the API. Follow these steps to generate your API key and authenticate your requests efficiently.
Google Cloud provides various ways to authenticate API requests, such as using an API key or OAuth. Below is a guide on how to generate an API key and integrate it into your JavaScript application for seamless authentication.
Step 1: Generate Your API Key
Follow these steps to create your API key in Google Cloud Console:
- Navigate to the Google Cloud Console at https://console.cloud.google.com/.
- Sign in with your Google account or create one if necessary.
- In the top-left corner, click on the Navigation menu, then select API & Services and choose Credentials.
- Click on the Create Credentials button and select API Key.
- Once the key is generated, make sure to copy it and store it securely. You will use it in your JavaScript code.
Step 2: Authenticate Requests Using the API Key
To authenticate your requests to the Speech-to-Text API, include the API key in your HTTP request headers. Here’s an example of how to do this in JavaScript:
const apiKey = 'YOUR_API_KEY'; const url = 'https://speech.googleapis.com/v1p1beta1/speech:recognize?key=' + apiKey; fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ config: { encoding: 'LINEAR16', languageCode: 'en-US' }, audio: { content: 'BASE64_ENCODED_AUDIO_CONTENT' } }) }) .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Important: Ensure your API key is never exposed in public repositories or client-side code. Use server-side code to securely handle the key if possible.
Step 3: Restrict API Key Usage
For enhanced security, it’s recommended to restrict the API key’s usage:
- In the Google Cloud Console, go to the Credentials page.
- Click on your API key to edit it.
- Under API restrictions, select Restrict key and choose the Speech-to-Text API.
- Under Application restrictions, select HTTP referrers and add the authorized referrers (URLs) that can use the key.
Example Table of Authentication Parameters
Parameter | Description |
---|---|
API Key | Your unique key used for authentication. |
Endpoint | The URL to send your requests to, e.g., https://speech.googleapis.com/v1p1beta1/speech:recognize. |
Content-Type | The header specifying the format of your request body, typically application/json. |
Real-time Voice Recognition Implementation in Your Web Application
Integrating real-time voice recognition into your web app can significantly enhance user experience by enabling hands-free interaction. By leveraging the Google Speech-to-Text API, you can transcribe spoken words instantly into text, which opens up a range of possibilities for interactive and voice-driven applications. Below is a step-by-step guide on how to set up real-time speech recognition in a JavaScript-based web application.
In this approach, we’ll focus on establishing a connection to the Google Speech-to-Text API, capturing audio from the user's microphone, and displaying transcribed text on your web page in real-time. This method relies on Web APIs such as `getUserMedia` and the SpeechRecognition API for seamless audio processing.
Step-by-step Guide
- Set up Google Cloud Project: Before starting, ensure you have an active Google Cloud account and have enabled the Speech-to-Text API. Create credentials for authentication.
- Integrate Web Speech API: Use the browser's native SpeechRecognition API for capturing speech and converting it to text.
- Capture Audio: Access the user's microphone using the `getUserMedia` method, which allows the app to record live audio.
- Stream Audio to Google API: Continuously stream audio data from the microphone to the Google Speech-to-Text service to receive real-time transcription.
Technical Details
The SpeechRecognition API sends audio input to Google's service, which returns transcribed text almost instantly. Here's an outline of how this can be set up:
- Start Recognition: Initialize the recognition process by calling `SpeechRecognition.start()`, which begins capturing audio.
- Handle Continuous Input: Use event listeners to capture the transcription results as they are returned.
- Stop Recognition: The process can be stopped either manually or automatically when the user stops speaking.
Real-time speech recognition in a web application not only improves accessibility but also enhances user interaction, especially in scenarios like virtual assistants, voice commands, and dictation tools.
Code Example
Step | Action |
---|---|
1 | Initialize SpeechRecognition object: const recognition = new SpeechRecognition(); |
2 | Start recognition: recognition.start(); |
3 | Handle results event: recognition.onresult = function(event) { ... } |
Handling Various Audio Formats and Optimizing Data Input
When working with Google Speech-to-Text API, it's crucial to handle multiple audio formats efficiently to ensure optimal performance. Google supports several common formats like WAV, MP3, FLAC, and Opus. However, each format comes with its own set of benefits and limitations, so choosing the right one depends on factors like audio quality, file size, and network constraints.
Optimizing data input is also essential for achieving accurate speech recognition results. Using properly pre-processed audio files and managing data flow can greatly impact the overall speed and accuracy of transcription. Below are some key considerations for handling different audio formats and optimizing input.
Supported Audio Formats
- WAV – Lossless audio, high quality, large file sizes.
- MP3 – Compressed audio, smaller file sizes, slightly lower quality.
- FLAC – Lossless compression, good balance between size and quality.
- Opus – Optimized for speech, lower latency, small file size.
Data Optimization Tips
- Preprocess Audio: Convert your audio to an appropriate sample rate (typically 16000 Hz) to reduce unnecessary data volume.
- Use Proper Encoding: Ensure the audio is in one of the supported formats (e.g., FLAC or WAV) to avoid conversion errors.
- Audio Chunking: For longer recordings, break the audio into smaller chunks and send them sequentially. This reduces the risk of timeout errors.
"Optimizing your audio input before sending it to the API is essential for achieving the best recognition accuracy."
Audio Format vs. File Size and Quality
Format | File Size | Quality | Latency |
---|---|---|---|
WAV | Large | High | Medium |
MP3 | Small | Medium | Low |
FLAC | Medium | High | Low |
Opus | Small | High (for speech) | Low |
Process and Display Transcribed Text from Google API
Once the speech recognition process is complete using the Google Speech-to-Text API, the transcribed text must be efficiently processed and displayed in the application. This involves handling the response from the API and integrating it into the user interface. The goal is to present the transcribed content in a clear and structured way, allowing the user to view and interact with the results seamlessly.
To achieve this, it's important to parse the response, extract relevant information, and then dynamically display it on the page. You can choose to show the transcribed text in real-time or after the entire speech has been processed, depending on the application requirements.
Processing Transcribed Data
- Retrieve the transcribed text from the API response.
- Handle potential errors or incomplete transcriptions gracefully.
- Optionally, format the text (e.g., removing unnecessary punctuation or correcting minor errors).
Displaying the Text
- Use JavaScript to update the DOM with the transcribed text.
- Display the text in a textarea or div for readability.
- Ensure the layout is responsive to accommodate varying amounts of transcribed text.
Note: It's important to process the speech recognition response asynchronously, ensuring that the UI remains responsive while the text is being transcribed and displayed.
Example Output Structure
Time Stamp | Transcribed Text |
---|---|
00:01 | Starting the speech recognition process... |
00:10 | First sentence transcribed successfully. |
Handling Errors and Debugging Common Issues in API Requests
When integrating speech recognition functionality using Google Speech-to-Text, developers often encounter various errors during API interactions. Identifying and resolving these issues is critical to ensuring smooth user experiences. Understanding common error codes and learning how to handle them effectively can save time and effort during development.
API calls may fail for several reasons, including network issues, invalid input data, or incorrect configurations. By managing these errors properly, developers can quickly pinpoint the problem and take corrective actions, improving both stability and performance.
Error Handling Techniques
Google Speech-to-Text API provides specific error codes that help identify the source of the issue. To handle errors efficiently, consider the following strategies:
- Check API response codes: The response status code often indicates whether the request was successful or encountered an issue. Common HTTP status codes include 200 (success), 400 (bad request), 404 (not found), and 500 (server error).
- Catch exceptions: Always implement try-catch blocks around your API requests to handle unexpected failures, such as network disruptions or timeouts.
- Use error messages: The API returns detailed error messages in the response body. These messages provide insight into the nature of the issue, such as invalid audio format or missing API keys.
Common Issues and How to Fix Them
When debugging API calls, it’s essential to understand some of the most frequent issues and how to address them:
- Invalid API key: Ensure that your API key is correctly included in the request headers and that it has the necessary permissions.
- Audio format issues: The API requires audio in specific formats (e.g., FLAC, WAV). Make sure your audio file meets the required specifications for processing.
- Exceeded rate limits: The API has usage limits. If you encounter a rate limit error, check the quota and consider implementing retries or using a more extensive billing plan.
Useful Debugging Tools
Using certain tools can simplify the process of debugging API calls:
Tool | Description |
---|---|
Postman | Postman allows you to test API requests and easily examine responses, helping you troubleshoot problems before integrating them into your application. |
Google Cloud Console | Check the logs and usage statistics in Google Cloud Console to track request errors and verify your configuration. |
Tip: Always log API responses and error messages for future reference. This practice can help you identify recurring issues and implement preventive measures.
Scaling Speech-to-Text Integration for Production Use
When integrating a speech-to-text solution into a production environment, it’s essential to ensure that the system can handle high traffic and a wide variety of audio inputs. This requires careful planning and optimizations to meet performance, reliability, and cost-efficiency goals. Below are key strategies for successfully scaling this technology in a production setting.
Effective scaling involves both technical and operational considerations. From optimizing API calls to managing concurrent requests, there are multiple steps to take for ensuring that your integration can handle the demands of a live environment. Implementing these practices will allow the solution to scale effectively, ensuring minimal downtime and maximum accuracy.
Key Considerations for Scaling
- Efficient Request Management: Minimize API calls by batching requests where possible and using streaming for continuous audio processing.
- Concurrency Handling: Ensure your backend can manage multiple concurrent users, especially during high-traffic periods.
- Cost Optimization: Monitor API usage to avoid over-spending, and implement rate limiting to control costs associated with high volumes of speech-to-text conversions.
Recommended Approaches
- Load Balancing: Use load balancers to distribute traffic evenly across multiple servers or instances, ensuring high availability.
- Auto-Scaling: Set up auto-scaling groups to automatically increase or decrease resources based on demand.
- Asynchronous Processing: Implement queuing mechanisms for processing speech-to-text requests asynchronously, reducing immediate processing loads.
Considerations for High Accuracy and Performance
Factor | Impact on Scaling |
---|---|
Audio Quality | Improves transcription accuracy, reduces retries, and optimizes processing time. |
Noise Reduction | Essential for handling real-world audio conditions, improving recognition accuracy in challenging environments. |
Real-Time Processing | Requires robust infrastructure to maintain low-latency and high-throughput performance. |
Note: For large-scale deployments, it is critical to continuously monitor system performance, including API response times, error rates, and throughput, to ensure smooth operation and scalability in production environments.