Best Text to Speech Api Python
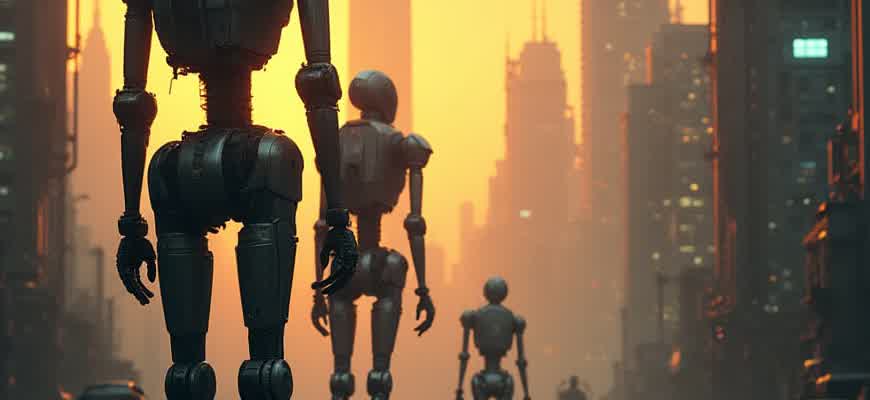
Text-to-speech (TTS) technology allows converting written text into spoken words, and there are several Python APIs that make this process seamless. These tools are essential for applications ranging from virtual assistants to accessibility features. The following are some of the best options for integrating TTS functionality into Python projects.
1. Google Text-to-Speech (gTTS)
The Google TTS API is one of the most popular and easy-to-use services for converting text into speech. It is a Python library and CLI tool to interface with Google’s Text-to-Speech API. The service offers a variety of languages and voices, making it suitable for diverse applications.
Key Features:
- Supports multiple languages and dialects.
- Simple Python integration with minimal setup.
- Free and easy to use with basic functionality.
2. Amazon Polly
Amazon Polly is another powerful TTS service, offering lifelike speech and extensive language options. It supports both standard and neural voices, allowing developers to choose between clear synthetic speech or more natural-sounding outputs.
Key Features:
- Supports SSML for advanced speech customization.
- High-quality, natural-sounding voices.
- Free tier available for limited usage.
Comparison Table
API | Languages Supported | Voice Quality | Free Tier |
---|---|---|---|
Google TTS | Multiple languages | Clear synthetic | Yes |
Amazon Polly | Multiple languages | Lifelike neural voices | Yes |
Best Text-to-Speech APIs for Python: A Practical Guide
Text-to-speech (TTS) technology enables applications to convert written text into spoken words. In Python, various libraries and APIs offer efficient solutions for integrating TTS functionality. This guide explores some of the top APIs, their features, and practical use cases, helping developers make an informed decision on which service best fits their needs.
When selecting a TTS API for Python, it's important to consider factors such as voice quality, speed, customization options, and supported languages. Below is an overview of popular options that are well-suited for both small projects and large-scale applications.
Top Text-to-Speech APIs for Python
- Google Cloud Text-to-Speech: A powerful API offering high-quality voices powered by Google's neural network models.
- AWS Polly: Known for its wide selection of voices and languages, Polly is great for scalable applications.
- Microsoft Azure Speech Service: Offers premium voices with support for SSML (Speech Synthesis Markup Language) for nuanced speech synthesis.
Comparison of Popular Text-to-Speech APIs
API | Supported Languages | Voice Quality | Customization |
---|---|---|---|
Google Cloud | Over 30 languages | High (Neural) | Supports SSML |
AWS Polly | 50+ languages | Good (Neural and standard) | Supports SSML |
Microsoft Azure | Over 75 languages | Premium | Supports SSML |
"The choice of TTS API depends on the specific use case, the required voice quality, and language support."
Conclusion
Each of the APIs mentioned has its own strengths. If you're looking for a highly customizable solution with support for neural voices, Google Cloud or AWS Polly may be the best options. On the other hand, for advanced features like real-time speech synthesis, Microsoft Azure is a strong contender.
Choosing the Right Python Library for Text-to-Speech
When working with text-to-speech (TTS) functionality in Python, selecting the right library can make a significant difference in the overall quality, flexibility, and performance of your application. A proper TTS library should be capable of converting text to clear, natural-sounding speech while offering enough customization options to suit various use cases. The decision largely depends on the specific requirements of your project, such as voice quality, language support, and speed.
Different libraries offer varying features, some of which may better serve specific needs like real-time performance, cloud integration, or local processing. It's essential to carefully evaluate the libraries based on your goals. Below are some key factors and popular Python libraries to consider when choosing the ideal TTS solution for your project.
Factors to Consider
- Voice Quality: Some libraries provide natural-sounding voices with advanced neural network models, while others may sound more robotic. Choose based on the level of quality you need.
- Language Support: Ensure the library supports the languages you plan to work with.
- Speed and Efficiency: For applications requiring real-time TTS, the library's performance is crucial.
- Integration with Other Tools: Some libraries integrate seamlessly with other services like cloud APIs or machine learning models, while others are more standalone.
Popular Libraries
- gTTS (Google Text-to-Speech): A simple, easy-to-use library for converting text to speech using Google’s TTS engine. Suitable for quick prototypes.
- pyttsx3: An offline TTS engine that works well for desktop applications, providing more control over speech properties.
- pico2wave: A lightweight library ideal for basic TTS tasks, often used in embedded systems.
Important: If you require offline speech synthesis, pyttsx3 is a suitable option as it doesn't depend on an internet connection, while gTTS requires internet access to function.
Comparison Table
Library | Voice Quality | Offline Support | Languages Supported |
---|---|---|---|
gTTS | High | No | Multiple |
pyttsx3 | Medium | Yes | Limited |
pico2wave | Low | Yes | Limited |
Integrating Text to Speech API with Your Python Project
Integrating a text-to-speech (TTS) API into your Python project allows you to add voice capabilities to your application, enhancing user experience. This process involves connecting the TTS service to your Python code, processing text input, and then converting it into audio output. Several libraries and APIs are available for this task, such as Google Text-to-Speech, Amazon Polly, and IBM Watson TTS, each offering unique features for customization.
To successfully integrate TTS into your project, you will need to understand how to interact with the chosen API, send text data, and retrieve the audio files. Most APIs offer RESTful interfaces, making them accessible via HTTP requests. The key is to choose an API that meets your specific needs, whether it's for generating high-quality voices or for handling different languages and accents.
Steps to Integrate TTS API
- Install necessary libraries: You need to install the Python package for the chosen TTS API. For example, if using Google Cloud TTS, you would install the `google-cloud-texttospeech` library.
- Authenticate API access: Most APIs require authentication via API keys. Ensure you have the correct credentials and follow the authentication steps provided by the API documentation.
- Send a text request: Use Python code to send a text request to the API. Specify the voice parameters, such as language, gender, and pitch.
- Process the audio response: Once the API processes the text, it will return an audio file (usually in MP3 or WAV format). You can play or save this file using Python's audio libraries.
- Handle errors and exceptions: Ensure your code handles errors gracefully, such as network issues or invalid text input.
Important: Always check the API's rate limits and pricing structure to avoid unexpected costs or service interruptions.
Example Code Snippet
Step | Code |
---|---|
1. Install Library | pip install google-cloud-texttospeech |
2. Authentication | from google.cloud import texttospeech |
3. Send Request | client.synthesize_speech(request) |
4. Save Audio | with open("output.mp3", "wb") as out: |
Configuring Voice Parameters: Pitch, Speed, and Volume Control
When working with text-to-speech APIs, customizing the voice output can make a significant difference in user experience. Adjusting parameters like pitch, speed, and volume allows for a more natural and engaging auditory experience. These settings can be fine-tuned to suit various applications, from virtual assistants to audio books or even accessibility features.
Among the most common adjustments are the pitch (how high or low the voice sounds), speed (how fast or slow the speech occurs), and volume (the loudness of the voice). Each of these can be controlled via the API's settings, providing flexibility for a tailored user interaction. Below are some key points for configuring these parameters:
Voice Parameters
- Pitch: Controls the tone of the voice, affecting its frequency. A higher pitch creates a more vibrant, sharp tone, while a lower pitch makes the voice deeper and more grounded.
- Speed: Adjusts the pace of speech. Faster speeds can be used for quick, energetic dialogues, while slower speeds are better for clarity and emphasis.
- Volume: Alters the loudness of the speech output. Volume control ensures that the speech is neither too faint nor too overwhelming for the listener.
Examples of Parameter Settings
- Pitch: Can be set within a certain range, for example, 0 to 2, where 0 is the default, 1 is slightly higher, and 2 is significantly higher.
- Speed: Typically measured in words per minute (WPM), for example, a setting of 150 WPM would be a standard speed, while 200 WPM might be suitable for fast-paced content.
- Volume: Usually set from 0 to 100, where 0 is silent, and 100 is the maximum loudness.
Tip: Always test your configuration settings across different devices to ensure optimal performance and readability. What sounds great on a computer speaker may not work well on mobile devices or in noisy environments.
Table of Parameter Examples
Parameter | Example Value | Effect |
---|---|---|
Pitch | 1.5 | Higher tone, more energetic voice |
Speed | 150 WPM | Standard speech rate |
Volume | 85 | Loud but not overwhelming |
Handling Different Languages and Accents with Python APIs
Text-to-speech (TTS) APIs can be powerful tools when it comes to converting text into audible speech. However, handling multiple languages and accents is crucial for ensuring that the output is accurate and contextually relevant. Python TTS APIs often come with built-in support for various languages, but each language can have unique phonetic rules, which can sometimes be tricky to process. To improve the speech synthesis quality, selecting the right TTS service is essential, and understanding its capabilities is key to dealing with linguistic diversity.
Most advanced APIs offer customization options for accents, pitch, tone, and even speed, but it’s important to know the limits of each tool. Some APIs allow users to adjust accent variations within the same language (e.g., American vs. British English), while others may provide complete language packs that accommodate regional differences. When working with multiple languages and accents, understanding how to configure these settings ensures that your application performs optimally across different regions.
Available Features for Handling Languages and Accents
- Language Support: Most TTS APIs support multiple languages, each with specific phonetic models.
- Accent Options: Regional accents can often be customized within the same language to enhance user experience.
- Gender and Voice Type: Many services allow selection between male, female, and neutral voices to suit different applications.
- Speech Adjustments: Variables such as pitch, speed, and tone can be modified for better accent handling.
Common Approaches for Improving Speech Output
- Use of Phonetic Dictionaries: Integrating phonetic dictionaries can significantly improve the accuracy of pronunciation in different languages.
- Voice Customization: Some APIs offer deep voice customization that allows users to train models with region-specific data.
- Accent-Specific Tuning: Adjusting parameters like stress patterns and rhythm is crucial for improving the natural flow of accented speech.
Note: Not all TTS services support regional accents equally. Some may offer broader coverage for major languages, while others may be specialized in certain regions or dialects.
Comparison of TTS APIs Supporting Multiple Languages and Accents
API | Languages Supported | Accent Customization |
---|---|---|
Google Cloud Text-to-Speech | 100+ Languages | Multiple accents in English and other languages |
Amazaon Polly | 60+ Languages | Different accents for English and Spanish |
IBM Watson TTS | 30+ Languages | Regional voice variations for certain languages |
Optimizing Speech Synthesis for Enhanced Accessibility
Text-to-speech (TTS) technologies are crucial for improving accessibility, allowing individuals with visual impairments, learning disabilities, and other challenges to interact with digital content. To ensure the generated speech is both clear and effective, optimizing the TTS output for accessibility is essential. This involves focusing on speech clarity, pronunciation accuracy, and control over speech parameters to make the interaction as seamless as possible.
Several aspects must be considered when adjusting TTS outputs for accessibility features. These include choosing the right voice, adjusting the pace, and fine-tuning prosody to avoid monotony or confusion. Here are some practical strategies and considerations to enhance TTS output:
Key Strategies for Optimizing TTS for Accessibility
- Voice Selection: Opt for voices that are clear, natural, and easy to understand. Different voices suit different contexts; for example, some users may prefer a calm, soothing tone, while others might need a more energetic and dynamic voice.
- Speed Control: Adjusting the speed of the speech can improve comprehension for users with cognitive disabilities or those with limited attention spans.
- Pitch Adjustment: A monotonous voice can be difficult to follow. Varying the pitch can enhance the overall clarity and help prevent user fatigue.
Important Considerations
Optimizing speech synthesis involves more than just the voice–it requires fine-tuning the parameters to meet diverse accessibility needs. Testing with different user groups is crucial to determine the ideal settings.
Essential Parameters for TTS Customization
Parameter | Purpose | Recommendation |
---|---|---|
Volume | Ensures speech is neither too soft nor too loud for users with hearing impairments. | Test with different volume levels to find the optimal range. |
Emphasis | Helps in emphasizing key information for better comprehension. | Apply emphasis sparingly to avoid overwhelming the user. |
Pauses | Appropriate pauses between phrases improve clarity. | Introduce natural pauses to enhance understanding. |
Additional Tips for Enhanced Accessibility
- Test your TTS output with real users, including those with various disabilities, to ensure it meets their needs.
- Ensure the speech synthesizer is compatible with screen readers and other assistive technologies.
- Provide users with control over speech speed and volume for a more personalized experience.
Managing API Limits and Reducing Latency in Real-Time Applications
In real-time applications, such as those involving speech synthesis, minimizing latency and staying within API rate limits are critical for smooth performance. APIs typically enforce restrictions on the number of requests you can make in a given period. Exceeding these limits could result in delays or service disruptions. Additionally, latency can degrade the user experience, especially when responsiveness is key. Therefore, managing these factors is vital for applications requiring near-instantaneous feedback, such as voice assistants and real-time transcription services.
To ensure consistent performance, developers need strategies to avoid hitting rate limits while minimizing latency. Some approaches involve optimizing request handling, adjusting the frequency of API calls, and choosing the right API services. Below are key strategies to consider when managing these two critical aspects.
Strategies for Effective API Management
- Rate Limit Management: Implementing throttling and queuing mechanisms to ensure that API requests are distributed evenly over time.
- Latency Reduction: Using edge computing or distributed servers to minimize the distance between the application and the API server.
- Request Optimization: Reducing the amount of data sent in each request to speed up the response time and lower processing overhead.
- Asynchronous Requests: Allowing requests to be processed in parallel instead of waiting for one request to complete before starting the next.
Best Practices for Minimizing Latency
- Prioritize Critical Requests: Prioritize API calls that impact the user experience directly and optimize them for speed.
- Pre-fetch Data: Anticipate future user actions by pre-fetching necessary data in advance, reducing wait times for the user.
- Use Low-Latency Services: Choose APIs known for their fast response times, especially those with dedicated infrastructure for low-latency performance.
- Monitor Latency Continuously: Regularly test and monitor latency using performance monitoring tools to identify and resolve potential bottlenecks.
Example of Rate Limits and Latency Management in API Usage
API Service | Rate Limit | Latency |
---|---|---|
Text-to-Speech API A | 100 requests per minute | 50 ms |
Text-to-Speech API B | 200 requests per minute | 30 ms |
Text-to-Speech API C | 50 requests per minute | 80 ms |
Important: Always monitor the performance of your chosen API to ensure that it aligns with your application's real-time requirements. Consistent testing helps maintain optimal user experiences.
Cost Considerations: Free vs Paid Text to Speech APIs
When choosing between free and paid text-to-speech APIs, understanding the cost implications is crucial. Free APIs often provide a limited set of features, which might be suitable for small projects or prototyping. However, as the demands of your application increase, you may face restrictions that hinder scalability, performance, or functionality. Paid APIs, on the other hand, tend to offer more robust features, higher quality voice synthesis, and scalability, but come at a cost.
For developers and businesses, it’s essential to weigh the advantages and limitations of both options to make an informed decision. Here’s a breakdown of the differences to consider when choosing a text-to-speech API based on cost:
Free Text-to-Speech APIs
- Limitations in usage: Most free APIs come with strict usage limits, including daily or monthly quotas for the number of characters or requests.
- Restricted functionality: Some features, such as premium voices, advanced customization, and extended language support, are often unavailable in free tiers.
- Lower quality: Free APIs generally offer lower-quality voices or fewer options for customization, which may impact the end-user experience.
Paid Text-to-Speech APIs
- Higher quality and more options: Paid plans often include access to high-quality voices, including natural-sounding, emotional, and multi-lingual options.
- Scalability: With paid APIs, usage limits are usually much higher, and they offer better support for large-scale applications or commercial products.
- Advanced features: Premium plans may provide advanced functionalities like speech recognition, custom voice creation, and more detailed voice control.
Cost Comparison Table
Feature | Free API | Paid API |
---|---|---|
Character Limit | Low (1000-5000 characters per month) | High (unlimited or large quotas) |
Voice Quality | Basic/robotic | Natural, high-quality voices |
Customization | Minimal | Extensive (pitch, speed, emotional tone) |
Languages Supported | Limited | Wide range of languages and accents |
Important: While free APIs can be great for quick prototypes or personal use, businesses looking for quality, flexibility, and scalability should consider investing in a paid solution to meet long-term needs.
Testing and Debugging Text-to-Speech Solutions in Python
Implementing text-to-speech (TTS) systems in Python can be challenging due to the various libraries and APIs available. Testing and debugging are essential steps to ensure the accuracy and performance of the implemented solution. It is crucial to validate the output, handle errors effectively, and test edge cases to avoid common pitfalls in TTS systems.
Testing your TTS implementation involves validating both the technical output and the user experience. For example, testing how different voices, languages, and speech speeds impact the quality of speech synthesis is necessary. Additionally, debugging issues such as incorrect phonetic output or poor voice quality is vital to optimize the solution.
Common Testing Strategies
- Unit Testing: Write unit tests to check individual functions like speech synthesis and input validation.
- Integration Testing: Ensure that the TTS API integrates well with other components of the system.
- User Experience Testing: Test the system's usability, such as responsiveness and the natural flow of speech.
- Error Handling: Ensure the implementation can handle edge cases such as unsupported characters or missing input.
Debugging Common Issues
- Voice Quality: If the synthesized speech sounds unnatural, try adjusting the parameters like speech rate and pitch.
- Phonetic Accuracy: Incorrect phonetic output can often be debugged by analyzing the text-to-phoneme conversion process.
- Performance Issues: Long processing times can be reduced by optimizing the text input handling or by using more efficient TTS engines.
Testing should always include performance benchmarks, especially if your TTS system will be used in real-time applications. This ensures that there are no lags or delays that could affect the user's experience.
Example of Testing Frameworks
Testing Type | Tools/Frameworks |
---|---|
Unit Testing | pytest, unittest |
Speech Quality Testing | Auditory Tests, Audio Analysis Libraries |
Performance Testing | Time, cProfile |