Openai Text to Speech Api Example
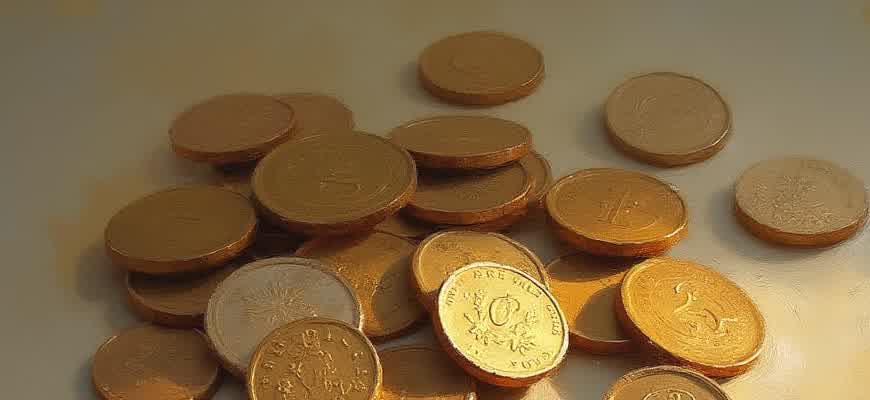
The OpenAI Text-to-Speech API allows developers to convert written text into natural-sounding speech. This functionality can be easily integrated into various applications, such as virtual assistants, accessibility tools, and automated voice systems. The API offers flexible customization options, allowing users to adjust the tone, speed, and voice characteristics.
To use the API, follow these key steps:
- Sign up for an OpenAI account and generate an API key.
- Install the necessary libraries (e.g.,
openai
Python package). - Make an API call with the desired text input and parameters for voice settings.
Here's a simple example of how to call the API in Python:
import openai
openai.Audio.create(
model="text-to-speech",
prompt="Hello, welcome to OpenAI's Text-to-Speech service.",
voice="en_us_male",
speed=1.0
)
Note: Make sure you have the correct API key and library installed before making a request.
The response from the API will return an audio file, which can be played in your application. The table below outlines some common parameters that can be adjusted:
Parameter | Description |
---|---|
model | The type of model used for speech generation (e.g., "text-to-speech"). |
voice | Specifies the voice to be used for speech synthesis (e.g., "en_us_male"). |
speed | Controls the rate at which the speech is delivered (e.g., 1.0 for normal speed). |
OpenAI Text to Speech API: Practical Guide for Integration
Integrating a Text-to-Speech API can significantly enhance user interaction in various applications, offering natural-sounding voice outputs. OpenAI's Text-to-Speech API provides robust features for converting text into speech, supporting multiple languages and customizable voices. This guide will walk through the key steps to integrate the API effectively.
Before starting the integration process, ensure you have access to OpenAI's API and the necessary credentials. The integration process involves setting up your environment, sending a text request, and receiving the corresponding audio output. Below is an overview of the process with key steps and helpful tips.
Steps for Integration
- Set up API Access: Obtain your API key by creating an account and subscribing to the OpenAI API service.
- Install Dependencies: Install the required packages such as `requests` for making API calls, and any other necessary libraries for audio playback or file handling.
- Send a Request: Prepare a text input and send a request to the API with the desired language, voice, and audio format options.
- Handle the Response: Upon receiving the audio data in response, process it accordingly–whether saving it as a file or playing it directly to the user.
Important: Ensure proper error handling to manage API limits, voice availability, or network issues. Implement fallback mechanisms where possible.
API Response Structure
Field | Description |
---|---|
status | Indicates the success or failure of the request. |
audio_url | URL to the generated speech file, if applicable. |
audio_data | Binary data for the audio response, typically in mp3 or wav format. |
By following these steps and utilizing the API response properly, developers can seamlessly integrate high-quality speech synthesis into their applications, enhancing accessibility and user experience.
Setting Up OpenAI Text-to-Speech API: A Step-by-Step Guide
To get started with OpenAI's Text-to-Speech API, you need to configure a few essential components. This includes creating an API key, installing required libraries, and setting up a basic script to interact with the API. In this guide, we will walk you through each step to help you seamlessly integrate text-to-speech functionality into your application.
By following this tutorial, you'll be able to convert written text into natural-sounding speech using OpenAI's advanced models. This API allows you to generate speech in different voices and languages, offering high-quality audio output suitable for a variety of use cases such as virtual assistants, accessibility tools, and more.
Prerequisites
- An active OpenAI account.
- API access to OpenAI's Text-to-Speech service.
- Python 3.x installed on your machine.
- Basic knowledge of programming concepts.
Step-by-Step Setup
- Obtain an API Key
Visit the OpenAI platform, log in to your account, and navigate to the API section to generate your API key. This key will be used to authenticate your requests to the service.
- Install Required Libraries
OpenAI's Text-to-Speech API can be accessed via HTTP requests, so you will need a Python HTTP client. Use the following command to install the necessary libraries:
pip install openai requests
- Create a Python Script
Write a Python script to send a text-to-speech request to the OpenAI API. Below is an example code snippet:
import openai import requests openai.api_key = 'YOUR_API_KEY' response = openai.Audio.create( model="text-to-speech", input="Hello, how are you?", voice="en_us_male" ) with open('output_audio.mp3', 'wb') as f: f.write(response['audio'])
Important Notes
Always keep your API key secure and do not expose it in public code repositories.
API Request and Response Example
Field | Description |
---|---|
Model | Specifies the Text-to-Speech model to use (e.g., "text-to-speech"). |
Input | The text that you want to convert to speech. |
Voice | Choose the desired voice (e.g., "en_us_male", "en_us_female"). |
How to Generate Natural Sounding Speech with OpenAI API
Creating natural-sounding speech using AI has become increasingly achievable thanks to OpenAI's Text-to-Speech API. To ensure the speech sounds fluid and lifelike, the process involves optimizing input text, selecting the right voice model, and fine-tuning various parameters. Here’s a guide on how to achieve high-quality, natural speech synthesis with this powerful tool.
OpenAI’s API provides several options for generating speech, such as controlling the tone, pace, and inflection of the voice. By leveraging the advanced capabilities of the API, developers can generate speech that is not only intelligible but also expressive and engaging. Below are the key steps to get started.
Key Steps to Generate Natural Sounding Speech
- Prepare the Input Text: Ensure the text is clear and concise. Ambiguities or overly complex sentences may result in unnatural speech.
- Select the Right Voice Model: Choose a voice that matches the desired tone, gender, or style.
- Adjust Speech Parameters: Fine-tune elements such as pitch, speed, and volume to better mimic natural speech.
API Configuration Example
"When configuring the API, consider the response format, voice settings, and any necessary customizations to produce realistic audio."
- Send a request to the OpenAI API with the desired text input and configuration settings.
- Choose the voice model, such as 'male' or 'female,' and further adjust nuances like speed (slow, normal, fast).
- Process the response, which will include the speech in audio file format (e.g., MP3, WAV).
Parameter Settings
Parameter | Description | Options |
---|---|---|
Voice | Specifies the voice model to use | Male, Female, Custom |
Speed | Controls the speed of the speech | Slow, Normal, Fast |
Pitch | Adjusts the tone of the voice | Low, Normal, High |
Integrating OpenAI Text-to-Speech into Your Web Application
Text-to-Speech (TTS) technology allows your web application to convert written text into spoken words. By integrating OpenAI's TTS API, you can enhance the user experience, making your application more interactive and accessible. The integration process involves a few straightforward steps, which include setting up the API, sending text input, and handling the audio output.
Once you have the necessary API credentials, you'll need to send an HTTP request to OpenAI's API endpoint with the text you want to convert. After processing, the API will return an audio file, which you can then play back to the user through your web application. Below is a step-by-step guide to help you get started.
Steps to Integrate OpenAI TTS API
- Obtain API Keys: Register on OpenAI's platform to get your unique API key for authentication.
- Set Up Your Web Application: Use JavaScript (or your preferred language) to send requests to OpenAI’s TTS API endpoint.
- Handle Audio Output: Once the API returns the audio file, you can use the audio element in HTML to play the speech in your app.
- Optimize User Experience: Consider adding features like playback controls, volume adjustments, and speech rate adjustments for a more personalized experience.
Remember to handle errors gracefully, such as when the API request fails or the audio file is unavailable. It's important to ensure a smooth experience for your users.
Example Code Snippet
Here's a basic example of how to send a request and handle the response in JavaScript:
const text = "Hello, welcome to our web application!"; const apiKey = "your-openai-api-key-here"; fetch('https://api.openai.com/v1/voice/generate', { method: 'POST', headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' }, body: JSON.stringify({ text: text }) }) .then(response => response.json()) .then(data => { const audio = new Audio(data.audio_url); audio.play(); }) .catch(error => console.error('Error:', error));
Best Practices for Effective Integration
- Use Caching: Cache the audio files to avoid repeated API calls for the same text.
- Consider Accessibility: Ensure that TTS is available for all users, including those with disabilities.
- Monitor API Usage: Keep track of your API usage to avoid exceeding any rate limits or costs associated with the service.
Common Issues to Watch Out For
Issue | Solution |
---|---|
API request failure | Ensure proper error handling and verify your API key is correct. |
Audio quality issues | Check if you are using the appropriate audio settings and adjust as necessary. |
Customizing Voice and Accent in OpenAI Text to Speech API
OpenAI's Text to Speech API allows users to adjust the voice and accent of generated speech, offering more flexibility for diverse use cases. This capability is important when you need the output to match a specific region, tone, or personality. Customizing these features enhances user experience, making the text-to-speech output feel more natural and relatable.
There are several parameters in the API that help in achieving this customization. These include selecting different voices, changing speech speed, and adjusting the accent to reflect the target audience's regional preferences. In this way, developers can fine-tune the audio output to meet the specific needs of their application.
Available Customization Options
- Voice Selection: Choose from a variety of pre-configured voices, each with its own distinct tone and style.
- Accent Adjustment: Customize accents to reflect different regions, such as American, British, Australian, or even specific dialects.
- Speech Speed: Modify the pace at which the text is read aloud to match the desired flow of conversation.
- Pitch Control: Change the pitch to make the voice sound higher or lower based on the desired effect.
Example of Using Custom Voices
- Set the language and voice parameters in your request.
- Choose from a list of supported voices and accents (e.g., US English, UK English, etc.).
- Adjust the speaking rate and pitch as needed for the application.
- Generate the speech and test to ensure it matches the required tone and accent.
Parameter Reference
Parameter | Description |
---|---|
voice | Selects the voice model for speech synthesis (e.g., "en_us_male" or "en_uk_female"). |
accent | Determines the regional accent (e.g., American English, British English, etc.). |
speed | Controls the speech speed, where a higher value results in faster speech. |
pitch | Modifies the pitch of the voice to make it higher or lower. |
Note: Some voices and accents may only be available for specific languages or regions, so be sure to check the documentation for the most up-to-date options.
Handling Errors and Troubleshooting the OpenAI Text-to-Speech API
When working with the OpenAI Text-to-Speech (TTS) API, encountering errors can disrupt the smooth operation of your application. Identifying the root cause and understanding how to handle such errors is essential for ensuring reliable functionality. Several common error types can occur, including network issues, invalid input data, and misconfigurations in API requests. Troubleshooting these issues effectively can save time and improve user experience.
Below are some key strategies for dealing with common errors and debugging problems with the TTS API. Following best practices for error handling will help you quickly diagnose and resolve issues as they arise.
Common Error Types
- Authentication Errors: These occur when the API key is missing, incorrect, or expired. Ensure the correct API key is included in the request header.
- Invalid Parameters: This happens when input data does not meet the expected format. Double-check the parameters, such as text input, voice selection, and speech speed.
- Timeout Errors: Network issues or excessive load on the API server can lead to timeouts. Ensure your network connection is stable and retry after a brief delay.
- Rate Limit Exceeded: Exceeding the allowed number of API calls in a given time period results in this error. Implement rate-limiting mechanisms to avoid hitting the maximum threshold.
Debugging Steps
- Check API Response Codes: Always inspect the status code of the API response. A 200 status code indicates success, while other codes such as 400 or 500 indicate errors that need further investigation.
- Review the Documentation: The official OpenAI TTS documentation provides valuable information on valid parameters, response formats, and error codes. Refer to it when you're unsure about your input or request structure.
- Test with Sample Data: If you're encountering errors, try simplifying the request with basic, known-to-be-valid data. This helps isolate whether the issue lies with the API or your input data.
Important: Always include detailed error messages and logs when seeking support, as this helps the support team understand the context and resolve the issue faster.
Common Troubleshooting Table
Error Code | Description | Resolution |
---|---|---|
400 | Bad request, invalid input parameters | Verify input parameters and ensure they conform to the required format |
401 | Authentication failure | Check and correct the API key or refresh it if expired |
429 | Rate limit exceeded | Reduce the frequency of requests or implement rate limiting |
500 | Internal server error | Retry after a short delay, as this is usually a temporary issue on the server side |
Scaling Your Application with OpenAI Text to Speech API
As your application grows, it’s crucial to ensure that it can handle an increasing number of requests efficiently. By leveraging OpenAI's text-to-speech technology, you can seamlessly scale your system to cater to higher user demands while maintaining performance. The API offers the flexibility needed to integrate text-to-speech features into a variety of applications, from customer service solutions to content accessibility tools.
Scaling with the OpenAI Text to Speech API involves optimizing both the infrastructure and API usage to accommodate large-scale voice synthesis requests. With a few strategic adjustments, you can ensure smooth operations without compromising quality. Here's how you can approach scaling:
Optimizing API Requests
- Batch Processing: Grouping multiple text-to-speech requests into a single batch can reduce overhead and improve efficiency.
- Rate Limiting: Implementing rate limiting ensures that your app doesn’t exceed the allowed API usage and can handle a higher number of simultaneous requests.
- Error Handling: Build robust error handling mechanisms to automatically retry or adjust requests when encountering issues like network failures.
Infrastructure Considerations
It is also essential to scale your backend infrastructure to support the increased load on your application as it grows. Consider the following approaches:
- Cloud Services: Use cloud-based hosting solutions that automatically scale with traffic demands, ensuring that you have the necessary resources at all times.
- Load Balancers: Distribute incoming requests across multiple servers to avoid overloading a single instance.
- Auto-Scaling: Set up auto-scaling for your server infrastructure, enabling dynamic scaling based on incoming traffic patterns.
Cost Management and Performance Monitoring
Effective monitoring and cost management practices are key when scaling your application. Implementing these strategies will help you manage both your system performance and API costs:
Tip: Regularly monitor API usage through logs and analytics tools to identify potential bottlenecks and optimize requests accordingly.
Strategy | Benefit |
---|---|
Usage Analytics | Identify high-traffic periods and optimize resource allocation |
Cost Prediction | Estimate potential costs based on usage trends to avoid surprise charges |
Comparing OpenAI's Speech Synthesis API with Other Speech Generation Tools
When evaluating different speech synthesis technologies, it's important to compare the capabilities and features they offer. OpenAI's text-to-speech API stands out for its ability to generate human-like voices with minimal effort. However, there are other popular alternatives that are commonly used in various applications. These include services like Google's Cloud Text-to-Speech and Amazon Polly, both of which have unique strengths and weaknesses in comparison to OpenAI’s offering.
Each of these services provides users with different levels of customization, voice variety, and integration ease. OpenAI’s API is known for its realistic and highly intelligible speech, which makes it ideal for customer service and interactive applications. However, competitors may offer additional features, such as multilingual support or a broader range of available voices. Below is a comparison of key features between OpenAI’s API and other leading tools in the market.
Feature Comparison
Feature | OpenAI | Google Cloud TTS | Amazon Polly |
---|---|---|---|
Voice Quality | Highly natural and expressive | Natural, but less expressive | High-quality, but limited emotional range |
Language Support | Limited language options | Wide variety of languages | Supports many languages and dialects |
Customization | Limited customization options | Extensive customization available | Good customization options, including voice pitch and speed |
Real-time Processing | Yes | Yes | Yes |
Pricing | Flexible pricing, based on usage | Pay-as-you-go pricing | Pay-per-use model |
Advantages of OpenAI's Speech Synthesis API
- Human-Like Voice: OpenAI's technology produces extremely lifelike, expressive speech.
- Ease of Use: The API is simple to integrate into a variety of applications.
- High-Quality Sound: It excels in providing a clear and natural sound, even in challenging contexts.
Other Considerations
“While OpenAI’s API is excellent for creating realistic voice interactions, Google Cloud TTS and Amazon Polly may be better options for applications requiring broad language support or advanced customizations in voice characteristics.”
Conclusion
In conclusion, OpenAI’s text-to-speech API excels in producing realistic and clear voice outputs, making it ideal for applications that require natural-sounding voices. However, alternatives like Google Cloud TTS and Amazon Polly offer broader language support and more customization options, which may be crucial for certain projects. Choosing the right tool depends on specific use case needs and the importance of factors like voice variety, integration ease, and language capabilities.
Pricing Considerations and Cost Optimization for OpenAI TTS API
When integrating OpenAI's Text to Speech (TTS) API into applications, understanding the cost structure is essential for effective budget management. The pricing is typically based on the number of characters or words processed, and varies depending on the chosen voice models and features. This can lead to significant cost differences depending on the usage volume and the specific needs of the project.
To minimize expenses, it is important to optimize both usage and functionality. Analyzing the application’s specific needs will help determine the most cost-effective approach, whether through controlling the frequency of requests, adjusting the voice quality, or managing the text length to avoid unnecessary processing costs.
Key Pricing Factors
- Character count: Charges are generally calculated based on the number of characters processed in a single request.
- Voice type: High-quality voices or premium models may come at a higher rate.
- Frequency of usage: Frequent calls to the API can increase costs over time.
Cost Optimization Strategies
- Text compression: Reduce the character count by condensing the text before sending it for processing.
- Batch processing: Sending multiple requests in a batch can be more efficient than sending them one by one.
- Voice selection: Opt for lower-cost voices if high quality is not essential for the use case.
Important: Ensure that usage is optimized by monitoring the volume and frequency of requests, as frequent API calls can quickly escalate costs.
Example Pricing Table
Voice Type | Cost per 1,000 Characters |
---|---|
Standard Voice | $0.02 |
Premium Voice | $0.05 |
Custom Voice | Contact for pricing |