Real Time Voice Changer Google Colab
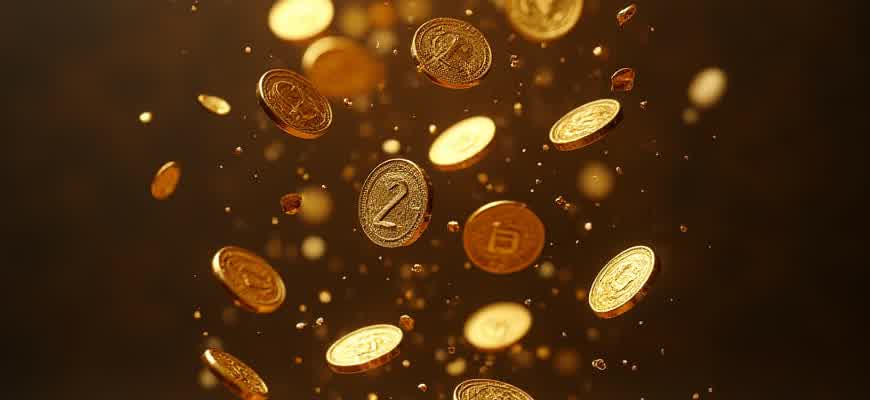
Google Colab is a powerful tool for running Python code in a cloud-based environment, which makes it ideal for real-time voice manipulation applications. By leveraging various machine learning models and libraries, it is possible to process and modify audio streams in real time. In this approach, users can experiment with altering their voice using pre-trained models directly in the cloud, without requiring any local processing power.
The following steps outline the basic setup for using real-time voice changers in a Google Colab environment:
- Set up the necessary libraries such as PyAudio and soundfile for audio input/output handling.
- Implement a real-time processing pipeline using a pre-trained neural network model for voice modulation.
- Stream audio through a microphone input and modify the voice in real time based on the selected model.
Here is a simplified breakdown of the process:
Step | Description |
---|---|
1 | Install required libraries and dependencies |
2 | Set up audio input using PyAudio |
3 | Integrate a real-time voice modulation model |
4 | Stream and modify the audio output |
Important: Ensure that the chosen model is lightweight enough to run efficiently in real-time on the cloud. Latency may vary depending on model complexity.
How to Configure Real-Time Voice Modulation on Google Colab
Setting up a real-time voice changer on Google Colab requires integrating different tools and APIs to process audio streams. Colab provides a flexible environment to experiment with machine learning models and audio processing techniques. By utilizing libraries such as PyAudio and integrating pre-trained models for voice transformation, you can create an effective real-time voice modification system directly within the browser.
The process involves installing necessary libraries, setting up the audio input/output system, and implementing a voice transformation model. Below are the steps to get everything working efficiently in Google Colab.
Steps to Set Up the Voice Modulator
- Install Required Libraries
- Start by installing essential libraries such as PyAudio, librosa, and numpy using pip.
- Ensure the necessary dependencies for audio processing are available on Colab, such as ffmpeg for handling audio encoding.
- Set Up Audio Input
- Use the IPython display module to capture microphone input in real-time.
- Ensure the proper configuration for the microphone so that audio can be recorded continuously.
- Apply Voice Transformation Model
- Choose a pre-trained model or create a custom algorithm for voice modulation.
- Process the captured audio stream through the model to alter parameters like pitch, speed, or apply effects like reverb.
- Output the Modified Audio
- Use libraries like IPython.display.Audio to play back the modified audio in real time.
- Ensure latency is minimized for seamless voice changing.
Required Libraries and Installation
Library | Installation Command |
---|---|
PyAudio | !pip install pyaudio |
librosa | !pip install librosa |
numpy | !pip install numpy |
ffmpeg | !apt-get install ffmpeg |
Real-time voice modulation depends heavily on the efficiency of the model and the system's ability to handle continuous audio input without significant delays.
Step-by-Step Guide to Integrating Your Microphone with Google Colab
Integrating your microphone with Google Colab can enhance real-time audio processing, allowing you to experiment with voice effects, speech recognition, or other audio-based projects directly within the cloud environment. This guide will walk you through the steps necessary to enable microphone access in Colab and use it in your projects.
To interact with audio data from your microphone in Google Colab, you will need to set up a few components. The process involves using the browser’s API for audio input, configuring Colab to access this data, and then processing the audio within the notebook environment. Below is a detailed guide on how to accomplish this.
Step 1: Set Up Microphone Access in Colab
- In the first cell, import the necessary libraries for audio handling, such as IPython.display and google.colab.output.
- Next, request permission to access the microphone using JavaScript's navigator.mediaDevices.getUserMedia function. This will allow the browser to ask for permission to use the microphone.
- Once permission is granted, set up an interface to capture the audio input. Use JavaScript code embedded in Colab to fetch the microphone stream.
Step 2: Process Audio Data in Real-Time
Once the microphone is active, you can process the incoming audio data within your notebook. Below are steps to stream the audio into Colab:
- Capture the audio input using the Web Audio API and send it to Colab's backend using a Python interface.
- In your Python code, use libraries like pyaudio or soundfile to process the live audio data and apply real-time changes, such as filters or effects.
- Finally, visualize or output the modified audio in the notebook using the IPython.display.Audio function.
Important Notes
Keep in mind that the microphone functionality may vary depending on browser settings, and you may need to adjust browser permissions or enable experimental features to ensure smooth operation in Colab.
Step 3: Example Code for Microphone Integration
Step | Code |
---|---|
Enable Microphone | navigator.mediaDevices.getUserMedia({ audio: true }) |
Capture Audio Stream | audioStream = await navigator.mediaDevices.getUserMedia({ audio: true }); |
Process Audio | audioContext = new (window.AudioContext || window.webkitAudioContext); |
Optimizing Audio Quality for Voice Modulation in Real-Time
Real-time voice modulation relies heavily on maintaining high audio quality throughout the entire process. The modulation itself involves altering various aspects of a voice signal, such as pitch, tone, and timbre, to create different effects. However, when dealing with real-time processing, factors like latency, computational power, and signal integrity become critical to achieving seamless and high-fidelity output. Ensuring audio quality during these processes requires a combination of optimized algorithms, effective hardware utilization, and minimizing signal degradation due to real-time manipulation.
Several key techniques can be employed to enhance the clarity and accuracy of modulated audio. By optimizing input and output processing chains, it is possible to achieve distortion-free voice modulation. In particular, the key is to balance between high-quality algorithms and system resource constraints to maintain both responsiveness and sound fidelity.
Key Optimization Strategies
- Noise Reduction: Minimizing background noise during capture and modulation ensures that only the desired audio characteristics are processed.
- Low Latency Processing: Algorithms should be optimized to ensure minimal delay in both capturing and modifying audio, which is critical in real-time applications.
- Efficient Signal Processing: Applying algorithms that effectively compress and filter audio signals without introducing artifacts helps in maintaining sound clarity.
Important Factors to Consider
- Sampling Rate: Higher sampling rates provide better resolution, but they require more computational resources. Balancing between quality and performance is essential.
- Bit Depth: A greater bit depth allows for more precise audio manipulation but also places higher demands on system resources.
- Hardware Support: Real-time voice modulation often requires specialized hardware like DSPs (Digital Signal Processors) or GPUs to handle the intensive processing load.
Optimizing audio quality for real-time voice modulation is a balancing act between computational efficiency and achieving high fidelity. Ensuring that the algorithms perform optimally on available hardware while maintaining low latency and high sound clarity is crucial to a seamless experience.
Audio Quality Metrics
Factor | Impact on Audio Quality |
---|---|
Latency | Higher latency can cause noticeable delays, making real-time applications less fluid. |
Signal-to-Noise Ratio (SNR) | A high SNR ensures that the desired voice signal remains clear and prominent over any noise. |
Dynamic Range | A broad dynamic range allows for nuanced voice modulation while preserving volume levels across different effects. |
How to Personalize Audio Effects: Easily Alter Your Voice
Adjusting and modifying voice effects can be a fun and creative way to personalize your sound. With real-time voice changers, users can explore various presets or build custom modifications to match specific needs. Whether you're enhancing your audio for gaming, streaming, or voiceovers, the ability to customize effects ensures that your output is unique. In this guide, we will walk through simple methods for adjusting and transforming your voice in real time using popular voice-changing software in Google Colab.
Customizing your voice involves a series of straightforward steps, from selecting presets to manipulating parameters like pitch, speed, and timbre. These options provide a wide range of auditory transformations, from deepening your voice to adding robotic or alien characteristics. By understanding the settings and tools available, you can create sounds that suit your project perfectly. Let's explore the common methods for tweaking and enhancing voice effects.
1. Adjusting Presets for Quick Changes
Most real-time voice changers come with built-in presets that allow for quick customization. These include options like "chipmunk," "robot," and "echo." To use them:
- Open the voice changer interface.
- Select a preset from the available options.
- Click "Apply" to hear the change in real-time.
Presets are a good starting point if you're looking for fast transformations without needing to tweak individual settings. However, you can fine-tune these effects for even more control.
2. Fine-Tuning Parameters for Detailed Control
If presets aren't enough, adjusting specific audio parameters offers detailed control over the output. Commonly adjustable parameters include:
Parameter | Description |
---|---|
Pitch | Changes the highness or lowness of your voice. |
Speed | Adjusts the rate of speech, making it faster or slower. |
Reverb | Simulates the effect of different environments, like a large hall. |
Timbre | Alters the texture or tone of your voice, making it sound richer or flatter. |
These parameters can be mixed to create more complex sounds. For example, lowering the pitch while increasing the reverb can create a "distant, deep voice" effect, perfect for certain audio projects.
For the most accurate results, make gradual adjustments to each parameter and test frequently to ensure the desired effect is achieved without distortion.
3. Combining Multiple Effects
One of the most powerful features in real-time voice changers is the ability to layer multiple effects at once. This allows for more complex transformations, such as combining an alien pitch with a robotic tone or adding a dramatic echo to a higher-pitched voice. Here's how to combine effects:
- Choose the first effect (e.g., pitch adjustment).
- Apply the second effect (e.g., reverb or distortion).
- Experiment with intensity levels to ensure that the effects complement each other.
By layering effects in various ways, you can create unique voices that are entirely tailored to your needs. Experimentation is key to finding the perfect combination!
Troubleshooting Common Issues When Using Real-Time Voice Changer
When working with real-time voice changers, various technical issues may arise that can disrupt the process. Whether you're using a Google Colab notebook or a local setup, understanding these common problems and how to fix them can ensure smooth operation. Below are some typical challenges and their corresponding solutions to help you resolve issues quickly.
From sound quality problems to connection errors, troubleshooting can often feel like a trial-and-error process. However, identifying the root cause of issues such as latency, audio distortion, or compatibility problems can significantly improve the experience. Here are the most frequent problems and how to address them.
Common Issues and Fixes
- Audio Latency: Delays in processing audio input can disrupt the flow, leading to an unnatural delay between speech and sound modification.
- Distorted Sound: Poor quality or unnatural voice effects might be caused by incorrect audio input settings.
- Input-Output Device Conflicts: If the input or output devices aren't configured properly, the software might not capture or output audio correctly.
- Permissions Issues: Lack of necessary permissions for microphone or audio device access can lead to the voice changer failing to start.
Steps to Resolve Problems
- Check Audio Settings: Ensure that both input and output devices are properly selected in your software and that the volume levels are set appropriately.
- Reconfigure Latency: In Colab, reducing the buffer size or using a different audio driver can help lower the latency for real-time performance.
- Verify Permissions: Double-check that microphone access is enabled, particularly if you're running the voice changer in a browser or cloud-based environment.
- Test with Different Devices: If the problem persists, try different microphones or speakers to rule out hardware issues.
Audio Quality Comparison
Issue | Possible Cause | Solution |
---|---|---|
Distorted Voice | Incorrect input levels or poor microphone quality | Adjust the microphone gain and ensure the microphone is in good condition |
Low Audio Volume | Low output volume setting or incorrect device selection | Increase the output volume and select the correct audio output device |
Latency Issues | High buffer size or insufficient processing power | Reduce buffer size or optimize processing settings for better performance |
Important: Always test your voice changer setup in a controlled environment before going live to avoid unexpected interruptions during use.
How to Share and Use Your Real-Time Voice Modulation Script in Google Colab
Google Colab offers an excellent environment for running Python scripts that modify audio in real time, making it an ideal tool for experimenting with voice changers. By using Colab, you can develop and test your script without the need for local setup or resource constraints. However, when you want to share your project or allow others to use it, you need to ensure that the script is easily accessible and properly configured for use across different environments.
This guide will walk you through the necessary steps to share and effectively use a real-time voice modulation script in Google Colab. Whether you are collaborating with others or just want to make your project more accessible, these instructions will ensure that your script can be shared easily and used by anyone with the proper access.
Sharing Your Script in Google Colab
To share your real-time voice changer script in Google Colab, follow these steps:
- Save your Colab notebook: Once your script is ready, save the notebook by clicking "File" and selecting "Save a copy in Drive". This will generate a link to your script that can be shared with others.
- Set permissions: Open the shared notebook and click on the "Share" button. In the sharing settings, ensure that anyone with the link can view or edit the notebook based on your preference.
- Provide dependencies: If your voice changer relies on external libraries (e.g., TensorFlow, Pyaudio), make sure to include installation commands at the beginning of the notebook for others to run, such as:
!pip install pyaudio tensorflow
How to Use the Script in Google Colab
Once the script is shared, others can easily use it by following a few simple steps. Here's a structured guide on how to get started:
- Access the shared notebook: Open the shared link and make a copy of the notebook in your own Google Drive for future edits and runs.
- Install required libraries: Ensure all dependencies are installed in the notebook environment by running the necessary installation commands.
- Enable microphone access: Google Colab does not natively support microphone input. You will need to connect external services like Google Colab Audio Recorder or use a Python script for real-time audio capture.
- Run the script: Execute each block of code in the notebook to start the real-time voice modulation process.
Important: When using Colab for real-time audio, note that Colab's limited support for audio input/output may result in latency. Consider testing the script with different configurations for optimal performance.
Table of Essential Dependencies
Library | Description | Installation Command |
---|---|---|
Pyaudio | Handles real-time audio input/output. | !pip install pyaudio |
TensorFlow | Machine learning library used for modifying voice patterns. | !pip install tensorflow |
Librosa | Library for analyzing and processing audio files. | !pip install librosa |
Advanced Settings: Fine-Tuning Your Voice for Professional Use
When working with voice changers in real-time, the ability to adjust specific audio parameters can significantly impact the quality and clarity of your output. Fine-tuning settings like pitch, modulation depth, and distortion can make your voice sound more natural or fitting for a particular context, be it a professional meeting or a content creation session. Adjusting these elements can help you avoid robotic or unnatural results while maintaining clear communication.
In professional environments, subtle changes can make a significant difference. Fine-tuning these settings will not only optimize the sound of your voice, but also help you achieve consistency across different devices and platforms. Below, we'll dive deeper into key settings that can elevate your performance.
Key Parameters for Voice Adjustment
- Pitch Control: Adjusting the pitch can help you sound either more authoritative or softer, depending on your audience. A slight shift can make the voice more engaging.
- Reverb and Echo: Adding reverb can simulate different environments, while controlling the echo ensures your voice doesn't sound distorted.
- EQ Filters: Customizing the frequency range (low, mid, high) allows you to reduce unwanted background noise and improve vocal clarity.
Advanced Effects for Professional Quality
- Compression: Applying compression helps balance your voice’s volume levels, ensuring that loud and quiet sections are evenly heard.
- Noise Gate: This tool removes unwanted background noise, such as keyboard clicks or static sounds, ensuring a clean output.
- Formant Shifting: This option adjusts the harmonic structure of your voice, useful for making dramatic voice transformations or fine-tuning your tone.
Example of Parameter Configuration
Setting | Adjustment Range | Effect |
---|---|---|
Pitch | -3 to +3 semitones | Adjusts vocal tone to sound higher or lower |
Reverb | 0 to 100% | Adds space and depth to the sound |
Compression | 1:1 to 4:1 ratio | Balances volume peaks and troughs |
Fine-tuning these settings ensures that your voice sounds professional and clear, with minimal distraction from unwanted artifacts.