Text to Speech Program in Android
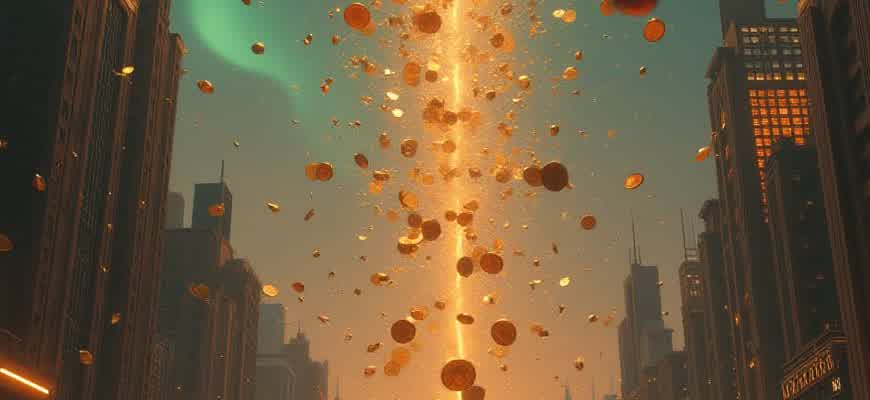
Speech synthesis technology, commonly referred to as text-to-speech (TTS), allows mobile devices to convert written text into spoken words. In Android, this functionality is powered by the TextToSpeech class, which is part of the Android SDK. This feature is particularly useful for enhancing accessibility and providing hands-free interactions in apps.
To implement TTS in an Android application, follow these key steps:
- Initialize the TextToSpeech engine
- Set the language and locale for speech output
- Convert text to speech using the appropriate method
The following table highlights some of the essential methods used in the TextToSpeech class:
Method | Description |
---|---|
setLanguage() | Sets the language for speech synthesis |
speak() | Converts the provided text into speech |
isLanguageAvailable() | Checks if a particular language is supported by the device |
Important: Ensure to handle the lifecycle of the TextToSpeech engine properly by releasing it when no longer needed to avoid memory leaks.
Text to Speech Application for Android: A Step-by-Step Guide
Creating a text-to-speech app on Android can enhance accessibility and provide an engaging experience for users. Whether you are developing for visually impaired users or looking to add voice feedback to your app, the Text to Speech (TTS) API in Android offers an easy and effective solution. This guide will walk you through the core concepts and practical steps to integrate TTS functionality into your Android app.
The Text to Speech service allows Android apps to convert text into spoken words, making it versatile for various use cases like voice assistants, reading apps, and real-time notifications. This guide focuses on the implementation process, essential configurations, and the coding steps necessary to get your app speaking to users.
Key Concepts and Setup
Before starting the development process, ensure you have set up your environment correctly. Here are the main steps:
- Ensure your app has the necessary permissions to use the TTS engine.
- Configure the Android Manifest to include the appropriate settings.
- Understand the TTS API's main components such as the TextToSpeech class and its methods.
It's essential to verify the availability of TTS engines on user devices before using them in your app. This prevents runtime errors and ensures a smoother experience.
Integrating TTS in Your App
To integrate the TTS functionality, follow the steps below:
- Initialize the TextToSpeech object in your activity.
- Set the language and check for TTS engine availability.
- Use the TextToSpeech.speak() method to convert text to speech.
- Handle lifecycle events, such as destroying the TTS object when it's no longer needed.
Example Code
Here's an example of how to implement the basic TTS functionality:
TextToSpeech tts = new TextToSpeech(context, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { int langResult = tts.setLanguage(Locale.US); if (langResult == TextToSpeech.LANG_MISSING_DATA || langResult == TextToSpeech.LANG_NOT_SUPPORTED) { Log.e("TTS", "Language not supported or missing data"); } else { tts.speak("Hello, welcome to the TTS demo!", TextToSpeech.QUEUE_FLUSH, null, null); } } } });
Additional Considerations
While implementing TTS in Android, consider the following:
Aspect | Consideration |
---|---|
Voice Quality | Choose the best available voice for your users based on their preferences. |
Device Compatibility | Ensure your app handles devices without TTS engines or with different language settings. |
Performance | Be mindful of the TTS engine's impact on performance, especially when using it frequently. |
How to Add Speech Synthesis to Your Android Application
Integrating speech synthesis into your Android application is a powerful way to enhance user experience. By converting written text into audible speech, you can cater to a wide variety of users, including those with visual impairments or those who prefer audio content. Android provides a built-in Text-to-Speech (TTS) API that makes it relatively easy to implement this functionality.
In this guide, we will walk through the essential steps to implement text-to-speech in your app. The process involves setting up the TTS engine, handling speech synthesis features, and managing user settings such as language and pitch.
Steps to Implement Text-to-Speech in Android
- Set Up the Text-to-Speech Engine
To get started, create an instance of the TextToSpeech class. You need to initialize it in your app's activity and ensure it is ready to use.
- Configure Language and Settings
Before using the TTS engine, you must configure it by setting the desired language, pitch, and speech rate.
- Synthesize Text to Speech
Call the TextToSpeech.speak() method with the text you want to convert to speech. Make sure to handle any exceptions or errors that may arise during the process.
Tip: Always handle the initialization and shutdown of the TTS engine properly to avoid memory leaks or crashes. This can be done in the onInit() method for setup and in the onDestroy() method for cleanup.
Basic Code Example
TextToSpeech tts = new TextToSpeech(context, new TextToSpeech.OnInitListener() { @Override public void onInit(int status) { if (status == TextToSpeech.SUCCESS) { int langResult = tts.setLanguage(Locale.US); if (langResult == TextToSpeech.LANG_MISSING_DATA || langResult == TextToSpeech.LANG_NOT_SUPPORTED) { // Handle language issues here } else { tts.speak("Hello, welcome to the Android app!", TextToSpeech.QUEUE_FLUSH, null, null); } } } });
Considerations for Optimizing User Experience
- Support Multiple Languages
Ensure that your app can handle various languages and accents. You can check available languages with TextToSpeech.getLanguages() and select the best option.
- Allow User Customization
Let users adjust the speed, pitch, and voice of the speech output to suit their preferences.
- Handle Errors Gracefully
Always check for potential issues such as missing language data or unsupported voices. Provide feedback to the user in case of errors.
Table: Common Methods in Text-to-Speech API
Method | Description |
---|---|
init() | Initializes the Text-to-Speech engine and sets up the callback for initialization. |
speak() | Converts the provided text into speech and plays it through the device's speaker. |
setLanguage() | Sets the language for the speech engine. |
shutdown() | Releases resources and shuts down the Text-to-Speech engine. |
Top Android Text to Speech APIs: Features and Comparisons
Android offers a variety of Text to Speech (TTS) APIs, each designed to provide high-quality voice synthesis for different app needs. These APIs vary in functionality, customization options, and integration ease. Developers must consider these differences when selecting the best solution for their applications. Below is a comparison of the top TTS APIs for Android, highlighting their unique features and capabilities.
When choosing a TTS API, important factors include voice quality, language support, customization features, and ease of integration. Some APIs offer natural-sounding voices with multiple languages, while others focus on performance or additional functionalities like pitch or speed adjustments. The table below summarizes the key features of the most popular APIs.
Comparison of Android TTS APIs
API | Supported Languages | Voice Customization | Integration Complexity |
---|---|---|---|
Google Text-to-Speech | Multiple languages | Speed, pitch | Easy |
Amazon Polly | 25+ languages | Voice selection, SSML | Moderate |
Microsoft Azure TTS | 50+ languages | Voice styles, SSML | Moderate |
IBM Watson TTS | Multiple languages | Voice customization | Hard |
Key Features of Popular APIs
- Google Text-to-Speech: Known for its simplicity and ease of use. Ideal for basic applications where quick setup is important.
- Amazon Polly: Provides high-quality voices with SSML support, allowing more control over speech output, making it suitable for more advanced projects.
- Microsoft Azure TTS: Offers a wide selection of voices and languages, with advanced features like emotional tone modulation and style control.
- IBM Watson TTS: Known for its high-level customization options but requires more effort to integrate and use effectively.
“Choosing the right Text to Speech API depends on the specific needs of your application, such as voice quality, language diversity, and integration ease.”
Optimizing Speech Output Quality in Android Applications
When developing Android applications that use text-to-speech functionality, ensuring high-quality audio output is crucial for creating a seamless user experience. Optimizing speech synthesis involves a combination of system-level settings and fine-tuning of specific speech parameters. The Android Text-to-Speech (TTS) engine provides various configuration options to control the quality of synthesized speech, including pitch, rate, and language-specific adjustments.
Additionally, developers must consider external factors such as network connectivity, device capabilities, and user preferences when optimizing TTS output. For instance, using high-quality voices may require a stable internet connection for downloading specific voice data or may consume more system resources. Below are key factors to focus on when optimizing speech output in Android applications.
Key Factors for Optimizing Speech Quality
- Voice Selection: Choose voices that match the language and accent preferences of your target audience. Most Android devices offer both male and female voices, as well as different regional variants.
- Speech Rate Adjustment: The default speech rate may not be ideal for all users. Allow users to customize the rate of speech to make the content easier to understand.
- Pitch Control: Fine-tune the pitch to avoid robotic or unnatural speech sounds. Adjusting pitch can make speech more pleasant and engaging.
- Audio Stream Selection: Ensure that the TTS output uses an appropriate audio stream (e.g., STREAM_MUSIC or STREAM_NOTIFICATION) to avoid interference with other sounds on the device.
Important Considerations
Always test speech output on different devices and under various network conditions to ensure consistent quality. Some lower-end devices may have limitations in processing power, which can affect TTS performance.
Configuring Text-to-Speech Parameters
Parameter | Description |
---|---|
Speech Rate | Controls the speed at which the text is spoken. Values range from 0.1 (slow) to 2.0 (fast). |
Pitch | Adjusts the tone of the voice. Higher values make the voice sound higher-pitched, while lower values make it deeper. |
Volume | Controls the loudness of the speech output. Volume can be adjusted according to the system's audio settings. |
Advanced Optimization Techniques
- Preload voices: Load voices in advance to minimize delays during speech synthesis.
- Use of External TTS Engines: Consider integrating third-party TTS engines like Google Cloud Text-to-Speech for better voice quality and additional language support.
- Handle Audio Focus: Manage the audio focus properly to ensure TTS output does not conflict with other audio sources, such as music or calls.
Customizing Voice Settings in Android Text to Speech
Android Text to Speech (TTS) provides developers with the ability to customize the voice output for a more personalized user experience. By adjusting the voice characteristics such as pitch, rate, and language, users can achieve a more natural and engaging speech synthesis. The Android TTS engine offers a set of tools that allow both basic and advanced configuration options to tailor speech synthesis to specific needs.
Customizing the voice settings in Android involves selecting the desired voice from a list of available options and modifying its attributes. These modifications can greatly enhance the clarity and expressiveness of the synthesized speech. Additionally, developers can integrate settings that let users choose voices based on gender, accent, and language preferences.
Voice Characteristics Customization
- Pitch: Controls the frequency of the voice, affecting how high or low the speech sounds.
- Speech Rate: Adjusts the speed at which the voice speaks. This can be increased or decreased based on the user's preference.
- Language and Locale: Allows selection of different languages and regional variations to match user needs.
Steps to Customize Voice Settings
- Access the TTS settings from the device's system settings menu.
- Select the preferred voice from the available options, including male, female, and diverse accents.
- Adjust the pitch and speech rate sliders to fit the desired tone and pace.
- Test the changes using the "Listen to an example" button to ensure satisfaction.
Note: Customizations applied through the system settings will affect all applications using the TTS engine, providing a unified experience across the device.
Voice Settings Table
Setting | Description |
---|---|
Pitch | Adjusts the height of the voice's tone (low or high). |
Speech Rate | Controls the speed at which the voice speaks. |
Language | Allows the selection of different languages and dialects. |
Common Problems in Android Text-to-Speech and How to Address Them
Android's Text-to-Speech (TTS) functionality provides a great way to create accessible apps, but developers often encounter specific challenges when implementing it. From voice quality issues to incorrect language settings, these problems can impact user experience. Understanding these issues and their solutions is essential for improving app performance and user satisfaction.
In this section, we explore some common issues developers face with Android TTS and offer practical solutions for addressing them. Whether it's dealing with unresponsive voices or incorrect pronunciation, the right approach can make a significant difference in app usability.
1. Poor Voice Quality
Low-quality voices are a common issue in Android's TTS system, often causing unnatural or robotic speech. This can occur due to outdated or missing voice data, or incompatible voice engines.
Solution: Ensure that your app uses the latest available voice data. Users can manually update the TTS engine in the settings, and developers should include instructions for users to download high-quality voices.
- Check if the device has the latest TTS engine version installed.
- Encourage users to download premium or high-quality voices from the Play Store.
- Consider offering users the option to select their preferred TTS voice in the app settings.
2. Incorrect Language Settings
Text-to-speech functionality may fail to pronounce words correctly if the language settings are not properly configured, leading to mismatched or jumbled output.
Solution: Ensure the app detects and uses the correct language and locale. Android offers an API to set the language dynamically, so developers should integrate language checks and fallback options.
- Use the TextToSpeech.setLanguage() method to ensure the correct language is set before speaking.
- Include error handling to manage situations where the desired language is not supported.
- Provide language options to the user in case automatic detection fails.
3. TTS Engine Not Responding
At times, the TTS engine may fail to respond, rendering the feature useless. This issue can arise from faulty initialization, incorrect engine settings, or system limitations.
Solution: Check the TTS engine status at startup and implement fallback mechanisms in case the engine fails to initialize correctly.
Error | Solution |
---|---|
Engine not initialized | Reinitialize the TTS engine on failure, and check for proper permissions. |
Audio not playing | Ensure the device volume is not muted and the correct audio output is selected. |
How to Add Multiple Language Support in Your Android Text to Speech App
To create a versatile Text to Speech (TTS) application for Android, it's essential to support multiple languages. This approach enhances the app's usability and accessibility for users around the world. The Android platform offers a powerful Text-to-Speech API that can be leveraged to add this functionality. By configuring language options, you can make sure your app speaks in different languages depending on the user's preference.
One of the key components of enabling multi-language support is properly managing language locales. Android provides a default system language, but developers can switch the TTS engine's language settings to another locale by calling the appropriate methods. This allows users to hear text in their desired language with just a few lines of code.
Steps to Implement Multiple Language Support
- Step 1: Initialize TextToSpeech with the desired language.
- Step 2: Check for available languages on the device using TextToSpeech.isLanguageAvailable().
- Step 3: If the language is supported, set it using TextToSpeech.setLanguage().
- Step 4: Provide a mechanism for users to select their preferred language in the app.
Language Support Check
Language | Status |
---|---|
English | Available |
French | Available |
Spanish | Available |
Chinese | Not Available |
Remember to handle cases where the selected language might not be available on the user's device. Always provide fallback options or notify users about the absence of certain languages.
Additional Considerations
- Voice selection: Some languages may have different voices (male, female, etc.), so it’s beneficial to let users choose the voice type.
- Performance: Using multiple languages can increase the app's size, so ensure that only necessary languages are bundled.
- Testing: Test your app with various languages and regions to ensure correct pronunciation and functionality.
Ensuring Accessibility in Android Apps Using Text to Speech
One of the key aspects of creating inclusive Android applications is making them accessible to a wider audience, including those with visual impairments. Text-to-Speech (TTS) technology plays a pivotal role in achieving this goal. By integrating TTS functionality, developers can ensure that their apps are usable by people who cannot read text on a screen. This approach not only enhances user experience but also adheres to accessibility standards that are increasingly demanded by users and regulators alike.
Implementing TTS effectively requires careful planning and attention to detail. It is not enough to simply convert text into speech; developers must ensure that the spoken output is clear, accurate, and contextually appropriate. Furthermore, the app must be responsive to different accessibility settings, offering users control over speech speed, pitch, and language preferences.
Key Strategies for Integration
- Use of Android's Built-In TTS Engine: Android offers a powerful TTS engine that allows developers to easily incorporate speech functionality into their apps. Developers should ensure that the TTS engine is initialized properly and supports the desired languages and voices.
- Adjusting for Speech Clarity: TTS should be configured to speak at a comfortable speed and pitch. Allowing users to adjust these settings ensures a better experience for individuals with different hearing or cognitive abilities.
- Accessibility Testing: It is crucial to test the TTS functionality under different conditions, ensuring that all text is read out properly, including dynamic content that might be added during app use.
Best Practices for Ensuring Accessibility
- Clear and Concise Language: Use simple, understandable language for text that will be spoken aloud. This ensures that the information is accessible to a broader range of users, including those with cognitive impairments.
- Consistent Interface: Provide consistent prompts and navigation cues to help users understand how to interact with the app through speech.
- Customizable Features: Allow users to customize speech parameters such as voice pitch, rate, and volume to suit their personal preferences.
"Making Android apps accessible using text-to-speech is not just about fulfilling legal requirements. It is about offering users an equal opportunity to interact with technology in a meaningful and effective way."
Important Considerations
Consideration | Description |
---|---|
Language Support | Ensure the app supports multiple languages and dialects to cater to a global user base. |
Voice Quality | Choose high-quality voices for a natural and pleasant listening experience. |
Real-Time Updates | Update the TTS output dynamically as the content of the app changes to keep users informed. |
Monetizing Your Android Text to Speech Application
Creating an Android Text to Speech (TTS) app can be a rewarding project, but once it’s developed, it’s crucial to implement a monetization strategy. There are several ways to generate income from your TTS application while maintaining a good user experience. Below are the most effective methods for turning your app into a profitable venture.
By offering additional features, providing a premium version, or using ad-based models, you can effectively monetize your TTS app. Below are some of the most commonly used methods to earn revenue from your app.
Popular Monetization Methods
- In-App Advertising: Integrating ads is one of the easiest ways to generate income from your app. Platforms like Google AdMob can help you incorporate ads into your app seamlessly.
- Subscription Model: Offering a subscription plan for premium features, such as advanced voices or offline usage, can generate recurring revenue.
- Freemium Model: Allow users to access basic features for free, while charging for premium features such as high-quality voices or additional languages.
- One-Time Purchase: Offering your app for a one-time fee can work well if the app provides significant value, such as offline TTS or support for multiple languages.
Key Considerations for Monetization
Ensure User Experience is Prioritized: While implementing monetization strategies, always make sure that the user experience is not compromised. Annoying ads or excessive paywalls may lead to user dissatisfaction.
Comparing Monetization Models
Monetization Model | Pros | Cons |
---|---|---|
In-App Advertising | Easy to implement, no upfront cost for users | Can disrupt user experience, lower earnings in the long run |
Subscription Model | Provides recurring revenue, high potential for growth | Users may hesitate to commit to subscriptions |
Freemium Model | Attracts a wide user base, high conversion rate for premium users | Free version may lack essential features, limiting appeal |
Ultimately, the best monetization strategy will depend on your target audience, the app’s features, and the type of value it provides to users. Testing different models and analyzing user feedback can help you refine your approach and maximize revenue.