Voice to Text Conversion Using Python
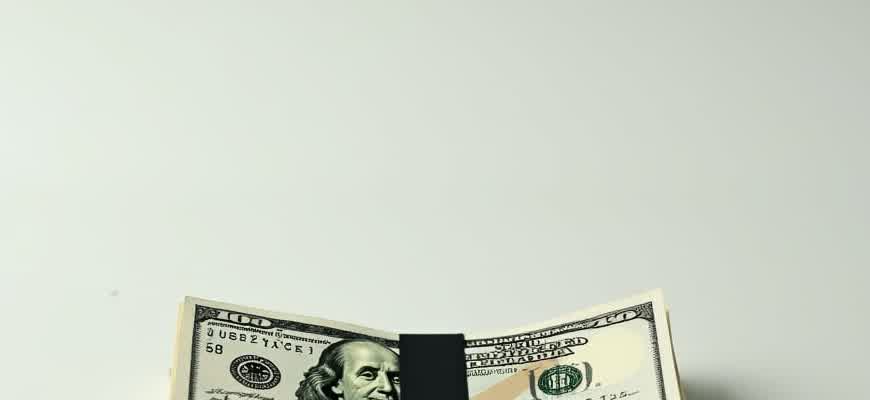
Converting spoken language into written text has become a crucial task in various fields such as accessibility, automation, and virtual assistants. Python, with its powerful libraries, offers an efficient way to perform this transformation using speech-to-text technology. This process involves capturing audio input and transcribing it into readable text format for further analysis or processing.
Key Libraries for Speech-to-Text in Python
- SpeechRecognition – A popular library that supports various speech recognition engines.
- PyAudio – Helps in capturing audio input from a microphone.
- Google Speech API – A powerful API that provides high accuracy for speech recognition tasks.
Basic Process of Converting Voice to Text
- Record audio using a microphone or other input devices.
- Process the recorded audio and send it to a speech recognition engine.
- Transcribe the audio to text, which can be further used for various purposes.
"Voice-to-text technology has significantly improved over the years, providing high accuracy and flexibility for a wide range of applications."
Example: Speech-to-Text Conversion Workflow
Step | Action |
---|---|
1 | Record the audio using a microphone. |
2 | Use the SpeechRecognition library to recognize speech from the audio. |
3 | Convert the recognized speech into text format. |
Setting Up Python for Speech-to-Text Conversion
Before starting the implementation of voice-to-text conversion, it is essential to set up Python with the necessary libraries and tools. First, you need to install libraries that allow Python to process audio input and convert it into text. One of the most common libraries for this task is SpeechRecognition, which works with various speech recognition engines.
Additionally, depending on the recognition engine you choose (e.g., Google Web Speech API, CMU Sphinx), you may need to install specific dependencies for handling audio data. Below are the necessary steps to properly configure your environment.
Steps for Installation
- Install Python packages:
- Run the command
pip install SpeechRecognition
to install the SpeechRecognition library. - For handling audio, use
pip install PyAudio
(ensure that you have the correct audio drivers installed for your system).
- Run the command
- Test the installation:
- After installation, you can test if the SpeechRecognition library is working by running a simple script.
Important: Make sure that the microphone is correctly set up and recognized by your system. If using the Google Web Speech API, ensure that an internet connection is available.
Dependencies for Different Speech Engines
Speech Engine | Dependencies |
---|---|
Google Web Speech API | No extra dependencies (internet connection required) |
CMU Sphinx | pip install pocketsphinx |
Microsoft Azure | Requires Azure Cognitive Services SDK |
Choosing the Right Libraries for Speech Recognition
When developing a voice-to-text conversion system in Python, selecting the appropriate speech recognition library is crucial to ensure both accuracy and performance. The Python ecosystem offers a wide range of libraries, each with its own strengths and limitations. Choosing the right one depends on the specific needs of the project, such as real-time transcription, language support, or integration with other tools.
To help you navigate through these options, it is important to evaluate each library based on factors like ease of use, compatibility with your hardware, and the quality of transcription. In this context, it is also helpful to consider whether a library provides offline capabilities or requires an internet connection for cloud-based processing.
Popular Python Libraries for Speech Recognition
- SpeechRecognition – A widely used library for converting speech to text. It supports various recognition engines such as Google Web Speech API, CMU Sphinx, and more.
- PyAudio – Often used in conjunction with SpeechRecognition, PyAudio is essential for capturing audio input from microphones in real time.
- DeepSpeech – An open-source speech recognition engine based on deep learning, known for its high accuracy and support for multiple languages.
- Google Cloud Speech-to-Text – A powerful API from Google that offers highly accurate transcription with minimal setup, although it requires an internet connection.
Factors to Consider When Choosing a Library
- Accuracy: Some libraries provide better accuracy in noisy environments or with specific accents.
- Offline Functionality: Libraries like CMU Sphinx offer offline recognition, while others, like Google Cloud Speech, require an internet connection.
- Language Support: Ensure that the library you choose supports the language you are working with.
- Performance: Real-time transcription may require a more optimized library, such as DeepSpeech, for faster processing.
Quick Comparison Table
Library | Offline Support | Accuracy | Supported Languages |
---|---|---|---|
SpeechRecognition | Partial (CMU Sphinx) | Good | Multiple |
PyAudio | No | Depends on recognition engine | Depends on recognition engine |
DeepSpeech | Yes | Excellent | Multiple |
Google Cloud Speech | No | Excellent | Multiple |
Keep in mind that while cloud-based services like Google Cloud Speech offer excellent accuracy, they require a reliable internet connection. On the other hand, offline solutions like CMU Sphinx may not match the same level of performance but can be essential in environments with limited connectivity.
Step-by-Step Guide to Integrating Google Speech API
Integrating Google's Speech API into your Python project allows you to convert speech into text with minimal effort. The Google Speech-to-Text service provides accurate and efficient transcription capabilities. This guide walks you through the process of setting up and utilizing the API for speech recognition tasks.
Before starting, you will need a Google Cloud account and API credentials to access the Speech API. Once these prerequisites are met, the setup process is straightforward and involves installing necessary libraries, configuring authentication, and writing Python code to send audio data for transcription.
Setting Up the Google Cloud Speech API
- Create a Google Cloud project: Go to the Google Cloud Console and create a new project.
- Enable the Speech API: Navigate to the API Library and enable the Google Cloud Speech API for your project.
- Set up authentication: Download your service account JSON key file and set the environment variable
GOOGLE_APPLICATION_CREDENTIALS
to point to it. - Install the Google Cloud Speech client library: Use the following command to install the necessary library:
pip install --upgrade google-cloud-speech
Using the Speech API in Python
Once your setup is complete, you can now write Python code to process audio files using the Speech API. Below is a simple example of how to use the API to transcribe audio into text.
Remember to replace
YOUR_API_KEY
with the path to your authentication file.
from google.cloud import speech import io def transcribe_audio(file_path): client = speech.SpeechClient() with io.open(file_path, "rb") as audio_file: content = audio_file.read() audio = speech.RecognitionAudio(content=content) config = speech.RecognitionConfig( encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code="en-US", ) response = client.recognize(config=config, audio=audio) for result in response.results: print("Transcript: {}".format(result.alternatives[0].transcript)) transcribe_audio("path_to_your_audio.wav")
Important Notes
- Audio file format: The API works best with WAV files, particularly in 16-bit encoding. Ensure your audio matches the specified format.
- Rate limits: Be aware of usage limits, as the free tier offers limited transcription time.
- Language support: The Speech API supports multiple languages. Ensure that you specify the correct language code in the configuration.
API Response Example
The API will return a structured response containing the transcribed text. Here's a basic representation of what you can expect:
Field | Description |
---|---|
transcript | The text output from the audio. |
confidence | A measure of how confident the API is in the transcription accuracy. |
alternatives | A list of alternative transcriptions that might better fit the spoken content. |
Handling Different Accents and Dialects in Speech Recognition
Speech recognition systems are designed to convert spoken words into text, but this process becomes significantly more complex when dealing with diverse accents and regional dialects. Various accents and pronunciations can greatly affect the accuracy of the transcription, as speech recognition models are often trained on standardized speech datasets. Regional accents may lead to misinterpretations if the system is not properly optimized to account for linguistic variations.
Different dialects introduce unique vocabulary, intonations, and phonetic patterns that can confuse traditional recognition algorithms. Overcoming these challenges requires adjusting models to be more adaptive and inclusive of the linguistic diversity present in real-world speech.
Challenges with Accents and Dialects
- Phonetic Variations: Accents influence the way words are pronounced, leading to discrepancies in how the system interprets speech.
- Varying Vocabulary: Different regions may use unique words or phrases that are unfamiliar to the speech recognition model.
- Speed and Intonation: Some accents may involve faster speech rates or distinct tonal variations that are not well understood by the model.
Possible Solutions for Improvement
- Data Augmentation: Expanding training datasets to include diverse accents and dialects can help the model learn variations in pronunciation.
- Accent-Specific Models: Building separate models for specific accents or regions can improve accuracy by focusing on a more defined linguistic group.
- Continuous Learning: Implementing real-time feedback mechanisms allows the system to adapt and improve as it encounters new accents and dialects.
Incorporating diverse linguistic data and adaptive models is essential for building more accurate and inclusive speech recognition systems capable of handling the nuances of accents and dialects.
Comparison of Accent Recognition Techniques
Technique | Advantage | Disadvantage |
---|---|---|
Data Augmentation | Improves model's exposure to different accents | Requires large datasets, which can be resource-intensive |
Accent-Specific Models | High accuracy for specific regions | Limited scalability for global systems |
Continuous Learning | Enables real-time adaptation | May result in slow improvements in accuracy |
Enhancing Speech Recognition Accuracy through Noise Reduction
In speech recognition systems, external noise often leads to errors in transcribing spoken words. This is particularly problematic when trying to convert speech to text in real-time, especially in environments with high levels of background noise. To improve transcription accuracy, noise reduction techniques are crucial. These techniques aim to filter out non-speech sounds, allowing the system to focus more effectively on the actual speech signal.
Effective noise reduction involves preprocessing the audio data to remove unwanted interference before passing it to the recognition model. By applying various filtering algorithms and adaptive techniques, the speech signal becomes clearer, improving the overall quality of the transcription. Several methods are available for reducing noise in voice recordings, each suited for different types of environments and applications.
Key Techniques for Noise Reduction
- Spectral Gating: Reduces background noise by filtering out frequencies that don't match typical speech patterns.
- Deep Learning-Based Models: Uses neural networks trained to distinguish between speech and noise, effectively separating them during processing.
- Echo Cancellation: Removes unwanted echoes that are common in spaces with reflective surfaces.
- Adaptive Filtering: Continuously adjusts to changing noise conditions in real-time for more effective noise suppression.
Noise Reduction Workflow
- Record audio signal.
- Apply noise estimation algorithms to detect the type of noise present.
- Use the appropriate noise reduction technique based on the identified noise.
- Pass the cleaned audio to the speech recognition model for transcription.
Comparison of Noise Reduction Techniques
Technique | Effectiveness | Use Case |
---|---|---|
Spectral Gating | High in stationary noise environments | Indoor recordings with consistent background noise |
Deep Learning-Based Models | Highly effective in dynamic noisy environments | Real-time transcription in various settings |
Echo Cancellation | Very effective in acoustically reflective spaces | Phone calls, video conferences |
"By employing noise reduction strategies, voice-to-text systems can significantly improve their accuracy, even in challenging environments with substantial background interference."
Converting Audio Files to Text: A Practical Example
In many real-world applications, converting spoken language from audio files to text has become a crucial task. Python provides various libraries to achieve this, making the process of transcription both efficient and accessible. The following example demonstrates how to use Python to convert audio files into readable text using the popular speech recognition library, `SpeechRecognition`.
Let’s break down the process of converting an audio file into text using a practical example with Python. First, the audio file needs to be loaded, and then we use a speech recognition engine to process the file and extract the speech as text. Below is a step-by-step guide for converting audio files using Python.
Step-by-Step Guide
- Install the necessary libraries: You’ll need to install the `SpeechRecognition` library and an audio handling library like `pydub` to manipulate audio files.
- Load and preprocess the audio file: Before converting speech to text, ensure the file is in a suitable format (e.g., WAV, MP3). If necessary, use `pydub` to convert MP3 files into a format that can be processed by the recognition engine.
- Transcribe the audio: Once the file is ready, pass it to the recognition engine to generate the corresponding text output.
The following code snippet demonstrates the full process:
import speech_recognition as sr from pydub import AudioSegment # Convert audio file to WAV if it's in MP3 format audio = AudioSegment.from_mp3("audio.mp3") audio.export("audio.wav", format="wav") # Initialize recognizer recognizer = sr.Recognizer() # Load the audio file with sr.AudioFile("audio.wav") as source: audio_data = recognizer.record(source) # Perform speech recognition try: text = recognizer.recognize_google(audio_data) print("Transcription: ", text) except sr.UnknownValueError: print("Audio could not be understood") except sr.RequestError: print("Could not request results from Google Speech Recognition service")
Important Considerations
- Audio Quality: The accuracy of the transcription depends on the quality of the audio. Clear recordings with minimal background noise yield the best results.
- Speech Engine: Different speech recognition engines may have varying levels of accuracy, depending on language, accent, and audio quality.
- File Formats: Ensure the audio file is in a format that can be processed by the library. Popular formats include WAV, MP3, and FLAC.
Keep in mind that while automatic speech recognition has improved significantly, it still may not capture every word perfectly, especially with noisy or unclear audio.
Output Example
Audio File | Transcription |
---|---|
example.wav | The quick brown fox jumps over the lazy dog. |
test.mp3 | Hello, this is an example transcription. |
Real-Time Speech-to-Text Conversion: Obstacles and Solutions
Real-time transcription of speech to text presents numerous technical challenges, especially when aiming for high accuracy and low latency. Processing live audio input demands both speed and precision, which makes it a complex task. Systems must be able to handle various accents, background noise, and speech variability without compromising the quality of transcription.
Additionally, real-time systems must work in dynamic environments where audio quality can fluctuate, making it even harder to maintain performance. Overcoming these challenges requires innovative solutions in speech recognition models, signal processing techniques, and hardware optimizations.
Challenges in Real-Time Voice-to-Text Conversion
- Latency: The speed of transcription is crucial in real-time systems, as delays in converting speech to text can lead to a poor user experience.
- Noise and Distortion: External noise, echoes, and distortions caused by the environment or poor microphone quality can severely affect transcription accuracy.
- Accent and Language Variability: Speech recognition models must be robust enough to accurately transcribe various accents, dialects, and languages.
- Continuous Learning: Adapting to different speakers and speech patterns on the fly requires real-time updates to the models.
Possible Solutions
- Noise Filtering: Implementing advanced noise suppression algorithms can help reduce background interference and improve the clarity of the speech signal.
- Model Optimization: Using deep learning models that can be fine-tuned to specific environments or speakers can enhance accuracy. Transfer learning techniques may also be used to adapt models quickly.
- Edge Computing: By utilizing local processing power, edge computing can reduce latency and improve response time in real-time applications.
- Adaptive Algorithms: Implementing algorithms that continuously learn and improve from the speech data being processed can make the system more efficient over time.
"Real-time speech-to-text conversion is not just about accuracy; it’s also about ensuring a seamless experience where the transcription is done in real time without noticeable delays."
Key Technologies Used
Technology | Description |
---|---|
Deep Learning Models | Used to train the system on large datasets, enabling the recognition of complex speech patterns and improving accuracy. |
Noise Suppression | Techniques like spectral subtraction and beamforming help filter out unwanted noise and enhance speech clarity. |
Edge Processing | On-device processing reduces transmission time and provides faster results by minimizing dependency on cloud services. |
Storing and Exporting Transcriptions in Various Formats
Once the transcription process is completed, the next step is storing the results for future access and exporting them in formats that best suit the user's needs. There are various file formats available for saving text data, including plain text, PDF, and JSON, each serving different purposes. The choice of format often depends on the context in which the transcription will be used and the ease with which it can be shared or processed further.
For example, when exporting transcriptions, it is important to consider the following factors: readability, compatibility with other systems, and the preservation of formatting. A well-structured system will allow for easy extraction and storage of transcriptions, facilitating sharing across different platforms and applications.
Common Formats for Exporting Transcriptions
- Text Files (.txt) - Simple and widely compatible, but lacks rich formatting options.
- PDF Files (.pdf) - Ideal for sharing professional or finalized documents with consistent formatting.
- JSON Files (.json) - Preferred for structured data, especially when integrating with APIs or other software.
Recommended Export Methods
- For plain text, use Python's built-in file handling functions to save transcriptions in a `.txt` file.
- For PDFs, leverage libraries such as ReportLab to generate formatted documents.
- For structured output, store the transcription as a JSON object for later use in applications.
When exporting to different formats, ensure proper encoding (e.g., UTF-8) to handle special characters or languages with unique scripts.
Comparison of File Formats
Format | Use Case | Advantages |
---|---|---|
Text File (.txt) | Basic, plain transcription storage | Lightweight, easy to process, universally supported |
PDF (.pdf) | Sharing finalized transcriptions in a readable, professional format | Preserves layout, widely accepted in formal contexts |
JSON (.json) | Storing transcriptions in a structured format for further processing | Flexible, easily parsed by applications, supports data structure |