Web Speech Api Text to Speech Demo
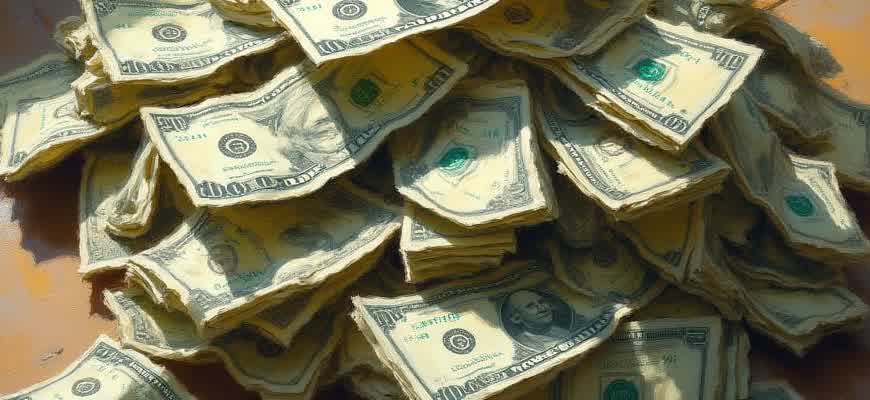
The Web Speech API provides an easy-to-implement solution for converting written text into speech. It is a powerful tool that enables web applications to "speak" text out loud. By utilizing this API, developers can create interactive applications that improve user engagement, accessibility, and functionality. In this demo, we will explore how to implement text-to-speech features using the Web Speech API.
Key Features:
- Real-time speech synthesis from text input.
- Ability to control voice attributes such as pitch, rate, and volume.
- Support for multiple languages and accents.
Usage Workflow:
- Initialize the speech synthesis engine.
- Set the properties of the speech output (e.g., voice, pitch, and rate).
- Trigger the speech by passing the text to the engine.
Web Speech API allows web developers to add speech capabilities to their sites, making them more accessible to users with visual impairments or those who prefer auditory information.
Example of Speech Synthesis:
Text Input | Voice | Pitch | Rate |
---|---|---|---|
Hello, how are you today? | Google UK English Male | 1.2 | 1.0 |
Welcome to our website! | Google UK English Female | 1.0 | 0.9 |
Web Speech API Text to Speech Demo: Practical Guide
The Web Speech API enables web developers to add speech recognition and synthesis capabilities to their applications. One of its key features is Text to Speech (TTS), which converts written text into spoken words. This demo demonstrates how you can utilize the TTS feature to create interactive web applications that respond with audio.
In this guide, we’ll walk through a basic implementation of the Web Speech API's TTS functionality. You'll learn how to configure the speech synthesis, choose voices, and control speech rate, pitch, and volume using JavaScript.
Getting Started with Web Speech API TTS
Before implementing TTS on your website, ensure that the Web Speech API is supported in the user's browser. Major browsers like Chrome, Edge, and Safari support this feature, but compatibility may vary. Here's how to get started:
- Check browser compatibility: Make sure the Web Speech API is supported by checking the browser's documentation or using feature detection.
- Access the SpeechSynthesis object: Use the
window.speechSynthesis
object to control the speech synthesis process. - Prepare text content: Convert your content into text and create a
SpeechSynthesisUtterance
instance to define what text will be spoken.
Configuring Speech Parameters
Once you've set up the basic functionality, you can configure the speech parameters to enhance the user experience. These include voice selection, rate, pitch, and volume:
- Voice: Choose from available voices on the system, which vary by language and region.
- Rate: Controls the speed at which the speech is delivered. Default rate is 1.0, with a range between 0.1 (slow) to 10 (fast).
- Pitch: Adjusts the tone of the voice. The default pitch is 1.0, with a range from 0.1 (low) to 2.0 (high).
- Volume: Sets the loudness of the speech, ranging from 0.0 (silent) to 1.0 (loudest).
Tip: Experiment with these parameters to find the best combination for your use case. Too high a rate may make the speech hard to understand, while a very low pitch might sound unnatural.
Example Implementation
Below is a basic example of using the Web Speech API to read aloud a given text:
const utterance = new SpeechSynthesisUtterance('Hello, welcome to the Web Speech API demo!');
utterance.rate = 1;
utterance.pitch = 1;
utterance.volume = 1;
window.speechSynthesis.speak(utterance);
Table: Parameters Overview
Parameter | Description | Range |
---|---|---|
Rate | Controls the speech speed | 0.1 to 10 (default: 1.0) |
Pitch | Adjusts the tone of the voice | 0.1 to 2.0 (default: 1.0) |
Volume | Sets the loudness of the speech | 0.0 to 1.0 (default: 1.0) |
How to Implement Web Speech API for Text-to-Speech on Your Website
The Web Speech API allows developers to integrate speech synthesis features directly into their websites, enabling text-to-speech functionality. This allows for more interactive and accessible user experiences, especially for users who may have visual impairments or prefer audio over text. The API provides an easy-to-use interface to convert written text into spoken words, utilizing the browser's built-in capabilities.
To implement text-to-speech functionality, you can leverage the `SpeechSynthesis` interface. This API provides several methods and properties to control speech output, including adjusting voice, rate, and pitch. The following steps guide you through integrating the Web Speech API into your website to enable text-to-speech conversion.
Steps to Integrate Text-to-Speech Functionality
- Check Browser Support: Ensure that the Web Speech API is supported in the user's browser. Most modern browsers support it, but it's always a good idea to confirm compatibility.
- Create a SpeechSynthesis Object: This object allows you to manage and control speech synthesis. You can configure voice settings, rate, and pitch.
- Convert Text to Speech: Use the `speak()` method to pass the text you want to convert into speech. This method plays the speech aloud in the user's browser.
Sample Code for Implementation
var synth = window.speechSynthesis; var textToRead = "Hello, welcome to our website!"; var utterance = new SpeechSynthesisUtterance(textToRead); utterance.voice = synth.getVoices()[0]; // Optional: Select a specific voice utterance.pitch = 1; // Optional: Set pitch utterance.rate = 1; // Optional: Set rate of speech synth.speak(utterance);
Important Considerations
It is crucial to handle errors gracefully, such as when the user’s browser does not support the Web Speech API or when speech synthesis fails.
Voice Options and Parameters
Parameter | Description |
---|---|
rate | Defines the speed at which speech is delivered. Default is 1 (normal speed). |
pitch | Defines the pitch of the voice. Default is 1 (normal pitch). |
voice | Allows you to choose from available voices in the browser. Different voices may have different accents and languages. |
Customizing Voice Parameters for Text-to-Speech in the Web Speech API
The Web Speech API allows developers to convert text into speech using built-in browser capabilities. One of the key features of this API is the ability to modify various voice parameters to create a more tailored speech experience. By adjusting specific settings, you can influence the voice's pitch, rate, and volume to fit different applications, from virtual assistants to accessibility tools.
Customizing voice parameters can significantly improve user interaction, providing a more natural and dynamic audio output. Understanding how to control these aspects allows you to fine-tune the voice output for various needs. Below are the main parameters you can adjust when working with the Text-to-Speech functionality of the Web Speech API.
Adjustable Parameters
- Pitch: Controls the perceived highness or lowness of the voice. Ranges from 0 to 2, with 1 being the default.
- Rate: Determines the speed at which the speech is delivered. The default value is 1, but values can be adjusted between 0.1 (slow) and 10 (fast).
- Volume: Sets the loudness of the speech output. It ranges from 0 (mute) to 1 (maximum volume).
Setting Parameters Programmatically
Once you've selected a voice from the available options, you can adjust the parameters using the following properties on the SpeechSynthesisUtterance object:
- Use the pitch property to set the voice's pitch. For example:
utterance.pitch = 1.5;
- To change the speaking rate, modify the rate property:
utterance.rate = 1.2;
- Adjust the volume to change the loudness:
utterance.volume = 0.8;
Available Voices
The Web Speech API provides a variety of voices, each with distinct characteristics. To list all available voices and select the desired one, use the speechSynthesis.getVoices()
method. Below is an example of voice selection:
let voices = speechSynthesis.getVoices();
let utterance = new SpeechSynthesisUtterance('Hello, world!');
utterance.voice = voices[0]; // Selects the first available voice
speechSynthesis.speak(utterance);
Note: The list of available voices may vary based on the browser and operating system used. Some voices might support additional parameters, such as gender or language.
Voice Parameter Examples
Voice | Pitch | Rate | Volume |
---|---|---|---|
Google UK English Female | 1.2 | 1.0 | 1.0 |
Google UK English Male | 0.8 | 1.2 | 0.9 |
Google US English | 1.0 | 1.0 | 1.0 |
Handling Different Languages and Accents with the Web Speech API
The Web Speech API provides a powerful way to convert text into speech, but one of its key challenges is managing multiple languages and diverse accents. When using this API, it is crucial to understand how to select and manage language-specific voices, as well as handle regional differences in pronunciation. The API allows developers to control the language and accent of the speech output, giving users a more personalized and contextually accurate experience.
By leveraging the capabilities of the Web Speech API, developers can ensure that the spoken content is not only understandable but also regionally appropriate. The API supports a wide range of languages and accents, offering a level of flexibility that is essential for applications targeting global audiences. In this section, we'll explore how different languages and accents can be handled using the available settings and options within the Web Speech API.
Configuring Language and Accent
The Web Speech API supports various languages, and each language comes with its own set of voices and accents. Developers can specify the language and accent preferences by setting the appropriate parameters within the speech synthesis API.
- Language Selection: The `SpeechSynthesisVoice.lang` property is used to define the language of the voice. For example, setting this property to "en-US" will use an American English voice, while "es-ES" will use a Spanish voice from Spain.
- Accent Variations: Some languages offer multiple accents. For example, British and American English are different accents within the same language, and the API allows you to specify these variations (e.g., "en-GB" for British English or "en-US" for American English).
Available Voices and Regional Differences
Not all languages or accents are supported equally across different browsers, and the available voices may vary depending on the platform. Below is a list of some common languages and their respective accent options:
Language | Accent Options |
---|---|
English | American, British, Australian, Indian |
Spanish | Castilian, Latin American |
French | French (France), Canadian French |
German | Standard, Austrian |
Important: The availability of specific voices and accents depends on the browser being used. Chrome, Firefox, and Safari may support different sets of voices.
Customizing Speech Output
To further customize the output, developers can adjust speech parameters such as pitch, rate, and volume. This allows for additional fine-tuning of the speech synthesis to accommodate different regional nuances. For instance, speech rate may vary between accents, and the pitch can be adjusted to better match a certain dialect's tone.
- Pitch: The `SpeechSynthesisUtterance.pitch` property determines how high or low the voice sounds.
- Rate: The `SpeechSynthesisUtterance.rate` property controls the speed of the speech, which can be useful for certain languages or regional dialects that may be spoken faster or slower.
- Volume: The `SpeechSynthesisUtterance.volume` property sets the loudness of the speech output.
Integrating Web Speech API with JavaScript for Real-Time Audio Playback
The Web Speech API provides a simple and efficient way to convert text into speech directly in a browser. By leveraging JavaScript, developers can seamlessly integrate this functionality into their web applications, allowing real-time audio playback of any text content. This API supports various voice options, speech rates, and even language selection, providing flexibility for different use cases, from accessibility features to interactive web applications.
To integrate the Web Speech API, developers can use the SpeechSynthesis interface, which enables control over the speech synthesis process. The process starts with preparing the text content and then using JavaScript functions to trigger the speech output. The Web Speech API operates directly in modern web browsers, eliminating the need for additional plugins or software, making it highly accessible for most users.
Steps to Integrate Web Speech API with JavaScript
- Check for browser support for the Web Speech API
- Set up the SpeechSynthesis instance in your JavaScript code
- Create a function to convert text to speech
- Customize parameters like voice, rate, pitch, and volume
- Trigger speech synthesis on a user action or event
Example Code
const synth = window.speechSynthesis; const textToSpeak = "Hello, welcome to the Web Speech API demo!"; const speech = new SpeechSynthesisUtterance(textToSpeak); // Optional: Set voice, pitch, and rate speech.voice = synth.getVoices()[0]; // Choose the first available voice speech.pitch = 1; // Set pitch level speech.rate = 1; // Set rate of speech // Speak the text synth.speak(speech);
Important Considerations
Always check for browser compatibility before implementing this feature, as the Web Speech API may not be supported in all browsers.
Voice Customization Options
Option | Description |
---|---|
Voice | Select the voice you want to use for speech synthesis. Different languages and accents may be available based on the system. |
Rate | Adjust the speed at which the speech is delivered. A value of 1 is normal, with higher values increasing speed and lower values decreasing it. |
Pitch | Modify the pitch of the voice. A value of 1 represents the default pitch, with values below and above altering the tone. |
Volume | Set the volume level for the speech, with 1 being the maximum and 0 being silent. |
Enhancing User Interaction with Speed and Pitch Controls in Text-to-Speech
When implementing text-to-speech functionality, providing users with control over the voice's speed and pitch is crucial for improving the overall experience. These adjustments offer personalized listening, allowing users to tailor the output to their preferences and needs. By fine-tuning the playback rate and tonal pitch, developers can create a more accessible and engaging interface for diverse audiences, from those with learning difficulties to individuals seeking a more dynamic auditory interaction.
Optimizing these settings involves careful attention to balance. For instance, altering the speed too much can compromise clarity, while modifying pitch may distort the voice. It is essential to find a sweet spot where both factors enhance the listening experience without causing discomfort. Below are key considerations for effective optimization.
Speed Control
Modifying the speech rate directly affects how fast or slow the speech is delivered. This control can be particularly beneficial for individuals with hearing impairments, non-native speakers, or those who prefer faster delivery.
- Slow Speed: Useful for clear comprehension, especially for complex information or learning purposes.
- Fast Speed: Preferred by users who are familiar with the content and require faster delivery.
- Default Speed: The standard rate at which text is read, balancing clarity and efficiency.
Pitch Control
Adjusting pitch modifies the tonal quality of the voice, which can make it sound more natural or dynamic. This setting helps to avoid monotonous readings and can also be used to make the voice more engaging.
- Lower Pitch: Typically perceived as more serious or formal, suitable for corporate or technical content.
- Higher Pitch: Often sounds friendlier or more energetic, ideal for casual conversations or children's content.
- Balanced Pitch: A neutral option that provides clarity without emphasizing tone.
Important Considerations
Factor | Impact |
---|---|
Speed | Faster speeds may reduce clarity, while slower speeds may frustrate experienced users. |
Pitch | Extremely high or low pitches can be uncomfortable, while a balanced pitch promotes natural flow. |
Finding the right balance between speed and pitch is key to creating an optimal text-to-speech experience that meets user preferences without compromising clarity or engagement.
Testing and Debugging Your Text-to-Speech Implementation in Web Browsers
When implementing text-to-speech functionality in web applications using the Web Speech API, testing and debugging are crucial to ensure that the feature works across different browsers and devices. Various factors like browser compatibility, voice availability, and performance should be considered during the testing phase. This section outlines key steps for effective debugging and testing of text-to-speech implementations.
To ensure your text-to-speech functionality performs as expected, you need to consider different aspects such as browser support, network issues, and user interactions. It is also important to verify the correct synthesis of the speech output, taking into account the selected language and voice preferences.
Common Testing Steps
- Test across multiple browsers to ensure compatibility (e.g., Chrome, Firefox, Safari, Edge).
- Check whether the correct voice is selected based on the user’s settings or default preferences.
- Ensure that the text is properly synthesized without errors in different speech rates and volumes.
- Test edge cases such as empty strings or non-English characters to verify the behavior.
Debugging Tools and Techniques
- Use the browser’s built-in developer tools to monitor the API requests and responses.
- Inspect the console for potential errors related to unsupported features or invalid parameters.
- Check the network activity to identify issues related to voice loading or missing resources.
- Test voice output on different devices (e.g., desktop, mobile) to ensure consistent behavior.
Key Considerations
Note: Keep in mind that not all browsers support the same set of voices, and some may require specific configurations or updates to function correctly.
Browser | Supported Features | Common Issues |
---|---|---|
Chrome | Full support for speech synthesis, multiple voices | Sometimes slow on mobile devices |
Firefox | Limited voice selection, basic TTS support | May require enabling experimental features |
Safari | Good support for voice selection | Speech synthesis may not work offline |
Improving Accessibility Using Web Speech API Text-to-Speech Features
The Web Speech API's text-to-speech functionality offers significant benefits for enhancing accessibility on the web. This technology allows web content to be read aloud, making it more inclusive for users with visual impairments, learning disabilities, or those who prefer auditory learning. With its straightforward implementation and compatibility across modern browsers, it helps bridge the gap for individuals who face challenges in reading text on a screen.
Text-to-speech capabilities can be particularly impactful in diverse settings, such as e-learning platforms, content-rich websites, and mobile applications. By converting written content into speech, users can interact with and comprehend material more effectively, regardless of their reading ability. Furthermore, this feature can be easily integrated with other accessibility tools, providing a seamless experience for those with additional needs.
Key Features and Benefits
- Enhanced User Interaction: Users can engage with content in a more dynamic way, improving navigation and comprehension.
- Multilingual Support: Web Speech API supports various languages, enabling global accessibility.
- Customization: Voice parameters such as pitch, rate, and volume can be adjusted for personalized experiences.
Practical Applications
- Education: E-learning platforms can use text-to-speech to help students with reading difficulties.
- Healthcare: Websites offering medical information can provide voice-based assistance for patients with visual impairments.
- Navigation: Websites and apps can use speech synthesis for better user guidance, especially in mobile environments.
Example of Text-to-Speech Integration
Feature | Benefit |
---|---|
Speech Rate Adjustment | Allows users to control the speed of speech output for easier understanding. |
Voice Selection | Offers a variety of voices, including gender and accent options, to suit individual preferences. |
Speech Volume | Users can adjust the speech volume for optimal listening experience in different environments. |
"Integrating text-to-speech capabilities into web applications not only improves accessibility but also provides a richer, more engaging user experience."
Best Practices for Deploying Web Speech API in Production Environments
When integrating the Web Speech API into a production environment, it is crucial to consider performance, accessibility, and user experience. Proper planning can prevent issues related to browser compatibility, network delays, and system resource consumption. Additionally, ensuring smooth speech synthesis and recognition performance will enhance user satisfaction, particularly for applications with real-time voice interactions.
To optimize the deployment of Web Speech API, developers must focus on various factors, including the selection of appropriate voices, handling errors gracefully, and ensuring efficient resource management. Following best practices not only leads to a better user experience but also ensures scalability and robustness in production environments.
Key Considerations for Efficient Use
- Voice Selection and Customization: Choose voices that are supported across different browsers and provide options for language and accent preferences. Customize the voice settings, such as rate and pitch, to match the application's needs.
- Error Handling: Implement error handling mechanisms to manage issues such as speech recognition failures, network interruptions, or unsupported browsers. Always provide fallback options.
- Performance Optimization: Ensure that the speech synthesis process does not cause significant delays or use excessive system resources, particularly in real-time applications.
Recommended Approaches for Scaling
- Implement asynchronous operations for speech synthesis and recognition to prevent blocking the main thread and to keep the UI responsive.
- Use caching mechanisms for pre-recorded or pre-processed speech, especially for frequently used phrases or commands, to improve performance.
- Regularly test your application on multiple devices and browsers to ensure compatibility and to identify potential issues in diverse environments.
"Smooth integration of Web Speech API can significantly enhance user engagement, but it requires careful optimization and testing to function seamlessly across various platforms and devices."
Common Pitfalls to Avoid
Issue | Recommendation |
---|---|
Limited Browser Support | Ensure fallback options for unsupported browsers, such as polyfills or alternative methods for speech synthesis. |
Performance Bottlenecks | Use throttling and debouncing techniques to optimize the performance of voice commands and reduce load times. |
Inconsistent Voice Quality | Test different voice options and ensure they are consistent across supported browsers and devices. |