Speech to Text Api Qwik Start Github
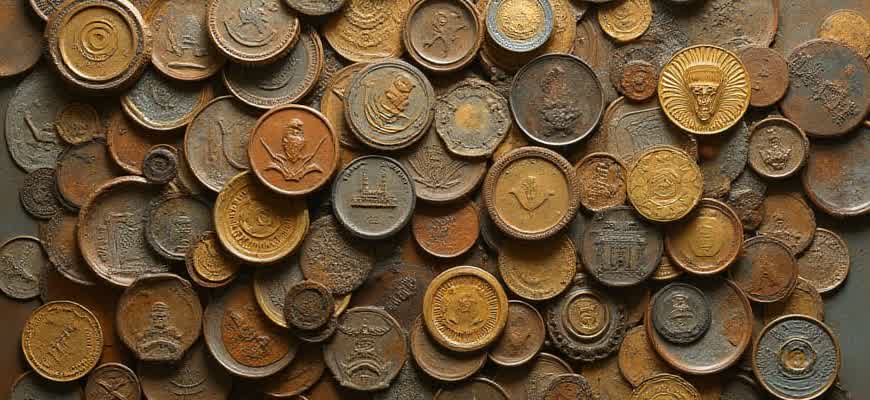
Introduction: Speech recognition is a powerful tool for converting spoken language into text. This guide will walk you through integrating a speech-to-text API using the necessary tools and libraries hosted on GitHub. Whether you're building a voice assistant, transcription service, or improving accessibility, this API can be a valuable asset for your project.
Prerequisites: Before diving into the code, ensure you have the following setup:
- A GitHub account for accessing and cloning repositories.
- Basic knowledge of programming in Python or JavaScript.
- Access to the API key of the speech-to-text service you plan to use.
Steps to Get Started:
- Clone the GitHub repository containing the speech-to-text integration code.
- Install required libraries or dependencies based on your programming language.
- Configure your API key within the code to authenticate requests.
- Run the example script to verify if the speech-to-text service is working correctly.
Important: Ensure that the speech-to-text service you are using supports the language and audio quality you require for accurate transcription.
Sample Code: Here is a basic table summarizing key functions available in the GitHub repository:
Function | Description |
---|---|
initSpeechRecognition() | Initializes the speech recognition engine. |
transcribeAudio() | Converts audio input into text output. |
handleErrors() | Manages errors and exceptions during speech-to-text conversion. |
Speech to Text API Quick Start GitHub Guide
If you're looking to integrate speech recognition functionality into your application, the Speech to Text API offers an easy and efficient way to get started. The GitHub repository provides all the necessary resources, including code samples and setup instructions, to help developers quickly implement speech-to-text capabilities. This guide will walk you through the essential steps to get up and running with the Speech to Text API using the resources available on GitHub.
This guide focuses on providing a practical overview of the setup process, including API key configuration, installation of required dependencies, and code examples. By following the steps outlined in the GitHub repository, you'll be able to efficiently integrate voice recognition into your app or service.
Steps to Get Started
- Clone the Repository: Start by cloning the GitHub repository to your local machine.
- Set Up API Key: To access the Speech to Text API, you'll need to configure an API key. Follow the instructions in the repository to obtain and securely store your API key.
- Install Dependencies: Install the necessary libraries and dependencies listed in the repository's requirements file. This will ensure all tools are ready for use.
- Run Sample Code: Once the setup is complete, run the provided sample code to test the API. The sample code demonstrates how to send audio data and receive transcriptions.
Make sure to test your integration thoroughly to ensure proper functionality and handle potential errors effectively.
Example Code Overview
The GitHub repository includes example code that highlights the integration process with the Speech to Text API. Below is an outline of how the code interacts with the API:
Step | Action | Code Example |
---|---|---|
1 | Import Required Libraries | import speech_recognition as sr |
2 | Initialize Recognizer | recognizer = sr.Recognizer() |
3 | Capture Audio from Microphone | audio = recognizer.listen(source) |
4 | Send Audio for Transcription | text = recognizer.recognize_google(audio) |
How to Integrate a Speech-to-Text API into Your Project
Integrating a Speech-to-Text API into your application allows you to convert spoken words into text, which can be useful for a variety of use cases such as transcription services, voice commands, or accessibility features. The process generally involves setting up authentication, making API calls, and processing the returned data. Here's how you can integrate the API seamlessly into your codebase.
To get started, you need to follow a few important steps, including installing the necessary libraries, setting up authentication keys, and calling the API endpoints for speech recognition. Make sure that the audio input format and quality meet the API’s requirements to ensure optimal performance.
Step-by-Step Setup Guide
- Install the API Client
- Depending on the language or framework you're using, install the corresponding API client. For Python, you can use
pip install google-cloud-speech
, or if you're working with Node.js, usenpm install @google-cloud/speech
.
- Depending on the language or framework you're using, install the corresponding API client. For Python, you can use
- Configure Authentication
- Obtain the API key or credentials from your service provider (e.g., Google Cloud). Store these securely in your project.
- Set up environment variables for the API credentials (e.g.,
GOOGLE_APPLICATION_CREDENTIALS
for Google services).
- Set Up the Audio Input
- Ensure that your audio input is clear and in a format accepted by the API (commonly FLAC, WAV, or MP3).
- Use a library or built-in functionality to capture microphone input if required.
- Make an API Request
- Send the audio data to the API endpoint. The request usually involves sending the audio file along with parameters such as language, model, and encoding type.
Note: Always check the specific documentation for your chosen API provider for details on the required request format and any limits on request size or frequency.
Handling Responses and Errors
Once the API processes your audio input, it will return a response containing the transcribed text. This response can include additional metadata such as timestamps or confidence scores. Make sure to handle potential errors such as network issues or invalid audio format errors by implementing proper error handling logic in your code.
Error Type | Possible Cause | Solution |
---|---|---|
Invalid Audio Format | Audio file format not supported by the API. | Convert the audio file to a supported format (e.g., FLAC or WAV). |
Authentication Error | Invalid API credentials. | Double-check your authentication keys and ensure the correct environment variable is set. |
Integrating API Keys and Authentication for Speech to Text API
When implementing a Speech to Text service, securely handling API keys and user authentication is crucial for ensuring smooth communication with the service. API keys serve as unique identifiers that authenticate requests made to the service, preventing unauthorized access. Integration of these keys should be done in a way that ensures they are protected from exposure while maintaining access control.
To set up the API keys and authentication mechanism, follow a few straightforward steps. Most services require the generation of an API key from the platform's dashboard, which you can then embed into your application's configuration. Additionally, you may need to configure environment variables to securely store these keys and use them in your backend system.
Steps to Set Up API Keys
- Register for an account on the Speech to Text service provider’s platform.
- Navigate to the API section of the platform’s dashboard and generate a new API key.
- Securely store the API key in your project’s environment variables or a secure secrets management system.
- Configure your application to use the API key in the request headers for authentication.
Key Authentication Methods
Different services provide distinct authentication methods. The most common approach involves sending the API key in the request headers. Below is an example of how to structure an authentication request:
Example request header for API key authentication:
Authorization: Bearer YOUR_API_KEY
Key Management and Security
It’s important to follow best practices for key management to prevent unauthorized access and misuse. Here's a list of key considerations:
- Do not hardcode API keys directly into your application's source code.
- Use environment variables to store keys securely, especially in production environments.
- Regularly rotate API keys and revoke unused or compromised keys.
- Implement role-based access controls to restrict who can access or manage the API keys.
Example API Authentication Process
Step | Action |
---|---|
1 | Generate API Key from the provider's dashboard. |
2 | Store the API key securely in your app’s configuration. |
3 | Use the API key in HTTP request headers to authenticate requests. |
Configuring Language and Recognition Settings for Accurate Transcription
Setting the correct language and recognition parameters is crucial for obtaining precise and reliable transcription results from speech recognition APIs. By specifying the appropriate language model, the transcription system can better identify words and phrases, adapting to the nuances of different dialects or regional accents. Additionally, fine-tuning recognition settings can help improve accuracy, especially in noisy environments or with specialized vocabulary.
When configuring these settings, it’s important to focus on two main aspects: language selection and recognition mode. Language models are typically tailored to specific languages, while recognition modes can vary in terms of real-time processing, background noise filtering, and the handling of multiple speakers.
Key Configuration Parameters
- Language Model: Select the appropriate language or dialect to match the expected speech input. This ensures the system uses the right phonetic and grammatical rules.
- Recognition Mode: Choose between modes like continuous or event-based transcription depending on the application’s needs.
- Noise Suppression: Enable background noise filtering to improve accuracy in noisy environments.
Steps to Configure Language and Recognition Settings
- Identify the target language or dialect for transcription.
- Set the recognition mode based on your specific use case (e.g., real-time vs. batch processing).
- Activate noise suppression if needed, especially for noisy or crowded environments.
- Test the configuration with sample audio data to fine-tune accuracy.
Important: Ensure that the language model you select supports the specific dialect or variant you intend to use. Some services may offer region-specific models for enhanced accuracy.
Example of Language and Recognition Settings Configuration
Setting | Value |
---|---|
Language Model | English (US) |
Recognition Mode | Real-time |
Noise Suppression | Enabled |
Handling Audio Input: Supported Formats and Quality Requirements
When integrating speech-to-text technology, selecting the appropriate audio format and ensuring optimal quality are crucial for accurate transcriptions. Different APIs and services support various audio formats, and understanding these formats can significantly affect transcription accuracy and performance. This section outlines the most common audio formats supported and the quality standards to ensure seamless speech-to-text conversion.
High-quality audio input is a prerequisite for achieving precise transcription results. The quality of the audio recording, including sample rate, bit depth, and noise levels, can impact the effectiveness of the speech-to-text system. Below is a detailed look at the essential format and quality requirements.
Supported Audio Formats
- WAV: A widely supported uncompressed format that provides high-quality audio.
- MP3: A compressed format that balances file size and audio quality, commonly used in various applications.
- FLAC: A lossless compression format that offers high fidelity audio and is preferred for detailed speech analysis.
- OGG: A free, open-source audio format that is efficient in terms of compression and quality.
- M4A: Common in mobile devices and compatible with many modern systems.
Audio Quality Requirements
For optimal transcription accuracy, audio recordings should adhere to specific quality standards. Below are key factors to consider:
- Sample Rate: A sample rate of at least 16 kHz is recommended for clear recognition of speech.
- Bit Depth: A bit depth of 16-bit or higher ensures detailed sound capture.
- Audio Length: Shorter recordings with clearer speech patterns are easier to transcribe.
- Noise Levels: Minimizing background noise or using noise-canceling microphones improves accuracy.
Recommended Settings
Parameter | Recommended Value |
---|---|
Sample Rate | 16 kHz or higher |
Bit Depth | 16-bit or higher |
Audio Format | WAV, MP3, FLAC |
For best results, ensure that the audio file is recorded in a quiet environment and with clear, articulate speech. Audio with excessive background noise or distortion may result in inaccuracies during transcription.
Real-Time Speech Processing with WebSockets and Streaming
When building real-time speech recognition applications, the ability to process audio streams as they are being captured is crucial. Using WebSockets for communication between the client and server allows for continuous, low-latency data transmission, enabling real-time speech processing. Streaming audio data over WebSockets allows for immediate transcription, which is key for applications like live captioning, voice-controlled systems, or interactive assistants.
In this approach, the audio stream is captured and sent to the server in small chunks. The server processes these chunks as they arrive, ensuring that the transcription is as close to real-time as possible. This technique significantly reduces the delay between speech input and text output compared to traditional request-response methods.
How WebSockets Enable Real-Time Speech Processing
- Low Latency: WebSockets provide a persistent connection between client and server, allowing for instantaneous data transfer without the overhead of opening new connections for each message.
- Continuous Data Flow: The real-time audio is sent in continuous packets, which is ideal for streaming applications that require uninterrupted processing.
- Scalability: WebSockets can handle multiple concurrent connections efficiently, making them suitable for systems that require large-scale real-time speech processing.
Processing Audio Data in Chunks
- Audio Capture: The client continuously captures audio data from the microphone, typically in small packets (e.g., every 10ms).
- Data Transmission: These audio packets are sent to the server over the WebSocket connection.
- Real-Time Processing: The server processes each audio packet as it is received, running the speech-to-text algorithm to generate transcription.
- Text Output: The transcribed text is sent back to the client in real time, displayed to the user immediately.
Real-time audio streaming with WebSockets enables continuous and low-latency processing, essential for interactive voice applications.
Example Data Flow
Step | Action |
---|---|
1 | Capture audio from the user in real time |
2 | Send audio chunks to the server over WebSocket |
3 | Process the audio stream using speech-to-text models |
4 | Receive transcribed text and update the UI in real time |
Handling Error Responses and Managing API Limits
When interacting with a speech-to-text API, it’s important to implement robust error handling to ensure smooth operation. APIs often return errors due to various reasons such as invalid input, connectivity issues, or exceeding service limits. Properly handling these errors allows your application to recover gracefully, provide useful feedback to users, and avoid unnecessary interruptions in the workflow.
In addition to error handling, understanding and managing API rate limits is crucial for maintaining the reliability of your application. Exceeding API limits can result in temporary bans or throttling, causing delays in speech-to-text conversions. By respecting these limits, users can ensure consistent performance while avoiding disruptions in service.
Error Handling Best Practices
When the API responds with an error, it’s essential to process the response appropriately. The typical error codes include 4xx and 5xx categories, indicating client-side and server-side issues respectively. Below are some common error scenarios and their suggested handling strategies:
- 400 Bad Request: This error typically occurs when the input format is incorrect. Ensure that the audio file or parameters are properly formatted before sending the request.
- 401 Unauthorized: This means that your authentication credentials are invalid. Verify your API key and ensure that it’s being sent correctly in the request header.
- 429 Too Many Requests: This indicates that you’ve exceeded your API usage limit. Implement a retry mechanism with exponential backoff to prevent further issues.
Always log error responses for debugging and optimization purposes. Analyzing these logs can help identify recurring issues and optimize the API integration process.
Managing API Usage Limits
To avoid disruptions caused by hitting rate limits, consider the following strategies for managing your API calls:
- Monitor usage: Implement monitoring tools to track the number of API requests made over time. This will help identify any patterns that might lead to exceeding the limit.
- Rate-limiting strategies: Introduce time-based request limits, such as batching requests or reducing the frequency of calls during peak hours, to ensure you stay within the allowed usage.
- Retry mechanism: When you receive a 429 error, retry the request after a delay. Implementing an exponential backoff algorithm helps to space out retries and avoid further throttling.
API Rate Limits Table
Limit Type | Description | Example Action |
---|---|---|
Requests per minute | Limits the number of API calls within a specific minute | Reduce the frequency of requests or batch them together |
Requests per day | Limits the number of API calls over a 24-hour period | Monitor daily usage and implement a queue system for non-urgent requests |
By proactively managing error responses and usage limits, your application can ensure smoother operation and reduce the chances of hitting service disruptions.
Best Practices for Optimizing Transcription Speed and Accuracy
When implementing a speech-to-text solution, achieving high accuracy and speed in transcription is paramount. Whether processing live audio streams or pre-recorded content, several factors can directly impact the performance of speech recognition APIs. By following best practices, you can optimize both transcription speed and accuracy, ensuring high-quality results while reducing delays in processing.
Key techniques involve proper preprocessing of audio data, using language models suited to the context of the speech, and leveraging API-specific configurations. Below are several guidelines to help improve transcription performance effectively.
Audio Quality and Preprocessing
Before sending audio to the transcription API, it's essential to ensure high-quality input. The cleaner the audio, the more accurately the system will transcribe it.
- Use clear, high-quality recordings: Ensure audio is recorded at a high bitrate with minimal background noise.
- Normalize volume levels: Consistent audio volume reduces the chance of misinterpretations.
- Remove background noise: Use noise reduction tools to eliminate non-speech sounds that may interfere with transcription.
- Segment audio effectively: Break down long recordings into smaller, manageable chunks to ensure better handling by the API.
Optimizing Transcription Settings
Choosing the right configurations for the transcription service is crucial for accuracy and speed. Many APIs allow fine-tuning based on the content being transcribed.
- Choose the appropriate model: Select a model that is specialized for your type of content (e.g., medical, legal, conversational). This will increase accuracy.
- Specify language and accent: Specify the speaker’s language or accent to enhance recognition quality.
- Utilize speaker diarization: For multi-speaker scenarios, enable speaker identification to avoid confusion in transcriptions.
- Leverage real-time transcription: For live scenarios, prioritize APIs that support real-time processing with low latency.
Table of Common Settings and their Impact
Setting | Impact on Accuracy | Impact on Speed |
---|---|---|
Audio Bitrate | Higher bitrate improves clarity and reduces misinterpretation. | High bitrate can increase processing time if the file is large. |
Language Model | Specialized models enhance recognition accuracy for specific fields. | Models tailored to your content type can speed up processing by reducing the need for corrections. |
Speaker Diarization | Improves clarity in multi-speaker recordings by distinguishing voices. | May slow down processing, but improves accuracy in complex situations. |
Tip: Always test the configuration settings with sample data to find the optimal balance between speed and accuracy for your use case.