Create Ai Voice Assistant Jarvis with Python
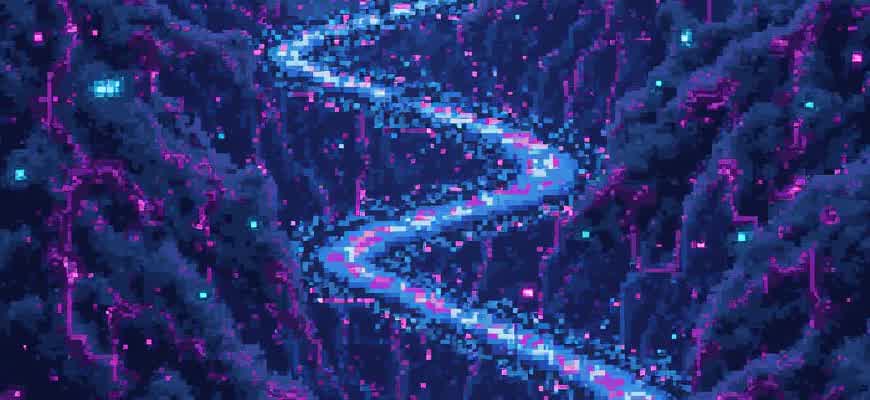
Creating a voice assistant that resembles Jarvis requires understanding of various technologies, including natural language processing (NLP), speech recognition, and text-to-speech synthesis. Python, with its rich set of libraries, provides an ideal platform to develop such a system. This guide covers the essential components and steps to build a simple voice assistant using Python.
Key Components:
- Speech Recognition
- Text-to-Speech (TTS) Engine
- Natural Language Understanding (NLU)
- System Integration (APIs and OS Control)
Steps to Build the Assistant:
- Install required libraries: SpeechRecognition, pyttsx3, pyaudio, etc.
- Set up a microphone for voice input.
- Process speech input using SpeechRecognition library.
- Convert the input into actionable commands.
- Integrate TTS engine to reply to user queries.
- Enable additional functionality like web search, system control, or task management.
Note: This project can be expanded with features like voice commands for home automation, calendar scheduling, or email reading by integrating relevant APIs.
Example Libraries and Tools:
Library | Usage |
---|---|
SpeechRecognition | Speech to text conversion |
pyttsx3 | Text to speech engine |
pyaudio | Microphone input handling |
Create Your Own AI Voice Assistant with Python: A Step-by-Step Guide
Building a voice assistant similar to Jarvis using Python can be an exciting and educational project. This guide will walk you through the process of creating a simple yet functional AI voice assistant that can handle tasks such as answering questions, sending emails, playing music, and more. The assistant can be customized to meet your specific needs and expand its capabilities as you develop your skills further.
To build the assistant, you’ll need a few core components like speech recognition, text-to-speech, and a way to interface with APIs for performing various tasks. In this guide, we will be using popular Python libraries such as SpeechRecognition, pyttsx3, and others. Follow the steps below to get started.
Essential Libraries and Tools
- SpeechRecognition: Allows the assistant to understand spoken commands.
- pyttsx3: Converts text to speech, making the assistant audible.
- pyaudio: Handles audio input from the microphone.
- Requests: Used to interact with external APIs for additional features like weather updates or news.
Steps to Create Your Assistant
- Install Required Libraries: Start by installing the necessary Python packages.
pip install SpeechRecognition pyttsx3 pyaudio requests
- Set Up Speech Recognition: Implement a function to capture audio input from the user and convert it to text.
Example:
recognizer.recognize_google(audio)
- Text-to-Speech Output: Use pyttsx3 to convert the assistant's response to speech.
Example:
engine.say("Hello, how can I assist you?")
- API Integration: For enhanced functionality, integrate APIs to get information like weather, news, or set reminders.
Example:
requests.get("http://api.weather.com")
Sample Code
Function | Code Example |
---|---|
Speech Recognition | recognizer = sr.Recognizer() |
Text-to-Speech | engine = pyttsx3.init() |
Making API Calls | response = requests.get("http://api.example.com") |
How to Configure Your Python Environment for Developing an AI Voice Assistant
Before diving into the development of an AI voice assistant, setting up your Python environment correctly is crucial. A well-prepared environment ensures smooth execution of the code, easy installation of dependencies, and minimizes potential conflicts. This guide outlines the necessary steps to configure your development environment for building an AI assistant.
The process involves installing key tools, managing dependencies, and setting up Python packages that are essential for AI voice assistant projects. Proper configuration helps streamline the development process, allowing you to focus more on writing code than troubleshooting environment issues.
Steps for Setting Up Your Python Development Environment
- Install Python: Ensure you have Python 3.7 or higher installed on your system. You can download it from the official Python website.
- Set up a Virtual Environment: Use virtual environments to isolate project dependencies. Run the following commands:
- Open a terminal/command prompt.
- Navigate to your project folder and execute:
python -m venv jarvis-env
- Activate the environment using
source jarvis-env/bin/activate
(Linux/macOS) orjarvis-env\Scripts\activate
(Windows).
- Install Required Libraries: Use pip to install essential libraries for AI voice assistant functionality:
pip install speechrecognition
– for speech recognition.pip install pyttsx3
– for text-to-speech functionality.pip install pyaudio
– to handle microphone input.pip install wikipedia
– for integrating Wikipedia searches.
Verifying the Setup
After setting up the environment and installing dependencies, it's crucial to verify that everything works correctly. You can run a simple test to check if speech recognition and text-to-speech libraries are functioning.
- In your terminal, activate the virtual environment if it’s not already activated.
- Run the following Python script to test voice input and output:
import speech_recognition as sr import pyttsx3 engine = pyttsx3.init() recognizer = sr.Recognizer() with sr.Microphone() as source: print("Say something...") audio = recognizer.listen(source) try: print("You said: " + recognizer.recognize_google(audio)) engine.say("You said: " + recognizer.recognize_google(audio)) engine.runAndWait() except Exception as e: print("Sorry, I did not get that")
Table of Recommended Tools and Libraries
Library | Purpose |
---|---|
SpeechRecognition | Converts audio speech into text. |
pyttsx3 | Enables text-to-speech functionality. |
pyaudio | Handles real-time audio input from a microphone. |
wikipedia | Facilitates searching Wikipedia articles. |
Choosing the Right Libraries for Building an AI Voice Assistant in Python
When creating a voice assistant in Python, selecting the right libraries is crucial for smooth integration of voice recognition, natural language processing, and text-to-speech features. A well-chosen library can significantly improve the efficiency and accuracy of the assistant's functionality. It’s important to focus on libraries that are reliable, well-documented, and widely used by the Python community.
Python offers a range of libraries, each tailored for specific aspects of voice assistant development. In this context, you need to evaluate libraries based on criteria like ease of use, compatibility, and community support. Below are some commonly used libraries and their functions for building a robust voice assistant.
Key Libraries for Voice Assistant Development
- SpeechRecognition: This library helps in converting speech to text and supports multiple speech recognition engines.
- PyAudio: Essential for audio handling, enabling the assistant to listen to voice commands via a microphone.
- gTTS (Google Text-to-Speech): Used for converting text into spoken words, providing natural-sounding speech.
- NLTK (Natural Language Toolkit): A comprehensive library for natural language processing, making it easier to understand and respond to user queries.
- Spacy: A powerful NLP library for more advanced processing, such as named entity recognition (NER) and dependency parsing.
Comparing Libraries for Specific Features
Library | Primary Function | Best For |
---|---|---|
SpeechRecognition | Speech to text | Simple and accurate voice input |
PyAudio | Microphone audio interface | Real-time voice input |
gTTS | Text to speech | Clear and fluent voice output |
NLTK | Natural language processing | Text analysis and processing |
Spacy | Advanced NLP | Complex language understanding and parsing |
Tip: When combining multiple libraries, always test for compatibility issues to ensure smooth integration, especially when dealing with audio and text processing in real-time.
Building Speech Recognition: Converting Audio to Text Using Python
Speech recognition is a core component of many AI-based virtual assistants. By converting spoken language into text, it enables communication between humans and machines. Implementing speech recognition with Python is straightforward, especially with the help of libraries such as SpeechRecognition and PyAudio. These libraries allow developers to build applications that can listen, process, and respond to audio input in real-time.
To build a speech recognition system, you need to capture audio from a microphone and process it into text. Python offers a variety of methods to interface with the microphone and perform recognition. The process typically involves capturing audio data, sending it to a speech recognition service, and returning the corresponding text.
Steps to Implement Speech Recognition in Python
- Install Necessary Libraries
- Install SpeechRecognition with pip:
pip install SpeechRecognition
- Install PyAudio for microphone access:
pip install pyaudio
- Install SpeechRecognition with pip:
- Capture Audio Input
- Use the microphone as an audio source through SpeechRecognition.
- Utilize PyAudio to record sound in real-time.
- Convert Audio to Text
- Send the audio data to a speech recognition API or library for transcription.
- Handle errors and ensure proper recognition.
Key Considerations for Accuracy
Proper noise filtering and microphone calibration are essential for improving speech recognition accuracy. Also, consider using pre-trained models for different languages to enhance performance.
Example Code for Basic Speech Recognition
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Say something...")
audio = recognizer.listen(source)
try:
print("You said: " + recognizer.recognize_google(audio))
except sr.UnknownValueError:
print("Sorry, I could not understand the audio.")
except sr.RequestError:
print("Sorry, there was an error with the speech recognition service.")
Popular Speech Recognition APIs
API | Features |
---|---|
Google Speech-to-Text | Free tier available, supports multiple languages |
IBM Watson Speech-to-Text | Real-time recognition, integrates with other IBM services |
Microsoft Azure Speech | Customizable models, integration with Azure services |
Implementing Natural Language Processing for Command Recognition
To build an AI voice assistant like Jarvis, it is essential to integrate Natural Language Processing (NLP) to allow the system to interpret and act upon user commands. NLP helps the assistant understand the meaning behind spoken words, transforming raw audio into actionable insights. This is particularly important for commands that are context-dependent or involve varying sentence structures.
For effective command understanding, NLP requires several key processes such as tokenization, part-of-speech tagging, and named entity recognition. By breaking down commands into components like words and phrases, the system can derive context and meaning, making it more intuitive for users.
Key Steps in NLP for Command Recognition
- Tokenization: Splitting a sentence into smaller units such as words or phrases.
- POS Tagging: Identifying the grammatical structure of words in the sentence (e.g., verbs, nouns).
- Entity Recognition: Extracting key entities like dates, places, or names from the input.
- Dependency Parsing: Understanding the relationships between words to derive sentence structure.
- Sentiment Analysis: Analyzing the mood or tone of the user's input to determine intent.
Important: Correctly handling ambiguous commands requires building a flexible NLP model capable of context switching and understanding user intent.
Practical Implementation Using Python
Python provides several libraries to integrate NLP into your voice assistant. Popular tools include:
- spaCy: A robust library that offers pre-trained models for various NLP tasks.
- NLTK: A powerful toolkit that supports tokenization, parsing, and sentiment analysis.
- Transformers (Hugging Face): For using advanced pre-trained models like GPT or BERT for understanding complex commands.
- SpeechRecognition: To convert voice input into text, which is then processed by the NLP model.
Mapping Commands to Actions
Once the command is processed and the intent is understood, the system can map it to an appropriate action. The table below outlines a simple structure for command-action mapping:
Command Example | Action |
---|---|
Play some music | Trigger music player to play a playlist |
Set the temperature to 22 degrees | Control smart thermostat to adjust temperature |
What’s the weather today? | Fetch weather information from an API |
Integrating Text-to-Speech (TTS) for Jarvis to Respond Verbally
For an AI assistant like Jarvis to engage users effectively, it must not only listen but also speak back. Text-to-Speech (TTS) technology allows Jarvis to convert written text into audible speech, making the interaction more natural and human-like. By integrating TTS, users can receive responses in real-time, creating a seamless conversational experience.
Python provides several libraries to implement TTS functionality. These libraries can synthesize speech from text using various speech engines. Common tools include pyttsx3, gTTS, and Google Cloud Text-to-Speech, each offering different features, voices, and customization options. With TTS, Jarvis can answer questions, confirm commands, or even narrate information audibly.
Steps to Integrate TTS in Jarvis
- Choose a TTS Library
- pyttsx3: Works offline, supports multiple voices.
- gTTS: Requires an internet connection, simple integration.
- Install the Library
- For pyttsx3:
pip install pyttsx3
- For gTTS:
pip install gTTS
- For pyttsx3:
- Integrate TTS into Jarvis
- Use the TTS library to convert Jarvis’s responses into speech.
- Ensure seamless interaction by synchronizing audio playback with text processing.
Example Code for Text-to-Speech with pyttsx3
import pyttsx3
engine = pyttsx3.init()
engine.setProperty('rate', 150) # Speed of speech
engine.setProperty('volume', 1) # Volume level (0.0 to 1.0)
engine.say("Hello, I am Jarvis. How can I assist you today?")
engine.runAndWait()
Key Considerations for Voice Quality
Selecting the right voice (male or female) and adjusting parameters like speech rate and volume can significantly improve the user experience. Testing different voices and speeds helps find the most natural-sounding option for your assistant.
Popular TTS Libraries for Python
Library | Features |
---|---|
pyttsx3 | Offline, supports multiple platforms (Windows, macOS, Linux) |
gTTS | Internet-based, supports multiple languages, easy to use |
Google Cloud Text-to-Speech | High-quality voices, requires API key, supports SSML |
Designing Custom Voice Commands for Your Python-based Assistant
One of the key features of a voice assistant like Jarvis is the ability to recognize and process custom voice commands tailored to your needs. In Python, you can easily create specific functions that respond to distinct phrases. By integrating libraries such as SpeechRecognition and pyttsx3, you can enhance the functionality of your assistant to perform a variety of tasks on command. Customizing these commands allows you to streamline everyday activities and build a more interactive experience for the user.
When designing voice commands, it's important to consider the structure and flow of the interactions. Creating intuitive, easy-to-remember commands ensures smooth communication between the user and the assistant. By structuring commands in a logical hierarchy, you can make the interaction more fluid and reduce the chances of errors. Below, we break down the process of designing custom voice commands in Python.
Steps to Create Custom Commands
- Define the task: Start by identifying the specific task you want your assistant to perform. This could be anything from controlling media playback to checking the weather.
- Set up voice recognition: Use the SpeechRecognition library to capture the user's voice and convert it into text for processing.
- Match the command: Create a function to match the recognized text to predefined commands. You can use
if-elif-else
statements or dictionaries for mapping. - Provide feedback: Ensure that the assistant responds with appropriate audio feedback using the pyttsx3 library or another text-to-speech tool.
Example of Command Design
Here is a simple example of how you might structure a command to check the time and another to open a web browser:
import speech_recognition as sr import pyttsx3 import webbrowser import datetime def listen_for_commands(): recognizer = sr.Recognizer() with sr.Microphone() as source: print("Listening for command...") audio = recognizer.listen(source) try: command = recognizer.recognize_google(audio).lower() return command except sr.UnknownValueError: print("Sorry, I couldn't understand that.") return "" except sr.RequestError: print("Sorry, there was an issue with the request.") return "" def execute_command(command): if "time" in command: now = datetime.datetime.now().strftime("%H:%M") engine = pyttsx3.init() engine.say(f"The current time is {now}") engine.runAndWait() elif "open browser" in command: webbrowser.open("https://www.google.com") else: print("Command not recognized.") command = listen_for_commands() execute_command(command)
Tips for Effective Command Design
- Keep it simple: Use short and clear phrases for commands. Complex or lengthy phrases can lead to misinterpretations.
- Group related commands: Bundle similar actions under a common command structure. For example, you could have all media-related commands under one group like "play music" or "pause video".
- Test and refine: Continuously test your commands to ensure accuracy. Make adjustments as needed for better performance.
Common Pitfalls
Issue | Solution |
---|---|
Misheard commands | Use more specific, unique keywords and test in different environments to improve accuracy. |
Slow response time | Optimize the speech recognition loop to reduce latency and ensure faster processing of commands. |
Note: The effectiveness of your custom voice commands relies heavily on the clarity of speech recognition and the efficiency of the code behind it. Testing different scenarios and refining your approach is key to building a responsive assistant.
Integrating Jarvis with External APIs (Weather, News, etc.)
To enhance the functionality of your AI assistant, you can integrate it with various external APIs. These APIs provide real-time data that Jarvis can use to offer services like weather updates, news briefings, and more. By making HTTP requests to these services, you can fetch relevant data and present it to the user through the assistant’s interface. This allows Jarvis to respond with dynamic, up-to-date information that goes beyond static commands.
In this section, we will explore how to integrate Jarvis with popular external APIs. Some of the commonly used APIs are for weather, news, and even virtual assistants like Google. The integration process generally involves obtaining an API key, sending requests, and handling the responses to extract useful information.
Integrating Weather API
To fetch weather updates, you can use APIs like OpenWeatherMap or WeatherStack. Here’s a brief guide on how to connect your assistant to these services:
- Sign Up and Get API Key: Register for an account and obtain an API key from the weather service provider.
- Install Required Libraries: Use Python's requests library to make HTTP requests to the weather API.
- Make API Calls: Use the API endpoint to get weather data, specifying location and other parameters.
- Parse the Response: Extract and format the weather information to be displayed to the user.
Example API request for weather data:https://api.openweathermap.org/data/2.5/weather?q={city_name}&appid={api_key}
Integrating News API
For real-time news updates, you can use news APIs like NewsAPI or New York Times API. The integration process is similar to weather data:
- Obtain an API Key: Register on a news API platform and get your API key.
- Send Request: Make an HTTP request to the news API endpoint with your key and desired parameters.
- Parse and Display News: Extract headline, source, and other relevant information and present it in a readable format.
Example API request for news data:https://newsapi.org/v2/top-headlines?country=us&apiKey={api_key}
API Integration Workflow
Here’s a basic workflow for integrating any external API into Jarvis:
Step | Description |
---|---|
1 | Get API Key |
2 | Install Required Python Libraries |
3 | Send API Request and Receive Data |
4 | Parse Data and Format it for User |
By following these steps, you can easily integrate external APIs into Jarvis, expanding its capabilities to provide a wider range of useful services to the user.