How to Create Text to Speech Software
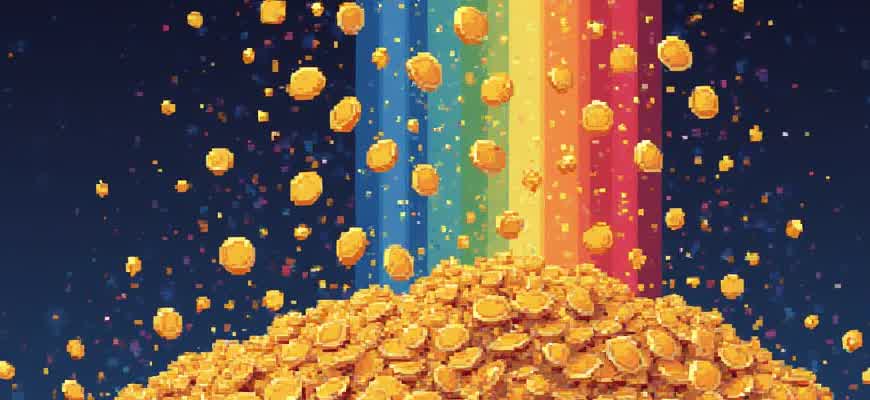
Developing text-to-speech (TTS) software requires a deep understanding of natural language processing (NLP) and speech synthesis techniques. At its core, TTS converts written text into audible speech using complex algorithms and datasets. Here's a step-by-step approach to building such a system:
- Collecting and Preparing Text Data: The first step is gathering large datasets of text and corresponding speech samples. The more diverse and representative the data, the better the system will perform.
- Preprocessing Text: Text data needs to be preprocessed to remove irrelevant elements such as punctuation, special characters, and stop words. This step ensures the text is clean and ready for analysis.
- Choosing a Speech Synthesis Model: Depending on the goals of the TTS software, a suitable speech synthesis model, such as concatenative synthesis or deep learning-based methods (like WaveNet or Tacotron), should be selected.
Note: High-quality TTS systems require a balance between natural-sounding speech and computational efficiency.
After selecting the model, training it on the prepared data is the next critical step. The system must learn to map the text input to accurate phonetic representations, ensuring clarity and expressiveness in speech.
Step | Action |
---|---|
Data Collection | Gather diverse text and audio data for training. |
Preprocessing | Clean text by removing unnecessary elements. |
Model Selection | Choose a synthesis model (e.g., WaveNet, Tacotron). |
Choosing the Ideal Speech Synthesis Engine for Your Application
When developing text-to-speech (TTS) software, one of the most crucial decisions you’ll make is selecting the right speech synthesis engine. This choice impacts the overall quality of the synthesized speech, the flexibility of your system, and its compatibility with your target platforms. Different engines offer varying levels of naturalness, speed, and customization, so it's important to carefully evaluate their features before making a decision.
Several factors should guide your decision-making process, including the intended use case, the languages supported, and the specific technical requirements of your project. Some engines are optimized for high-quality, natural-sounding voices, while others may prioritize performance or flexibility. Below are some essential criteria to consider when choosing a speech synthesis engine.
Key Considerations for Selecting a TTS Engine
- Naturalness of Voice – Assess the quality of the speech output. High-quality engines offer more human-like prosody, emotion, and natural intonation.
- Supported Languages – Ensure that the engine supports all the languages your application needs. Some engines may offer better regional dialects or accent choices.
- Customizability – Depending on your needs, you may require an engine that allows you to adjust pitch, speed, or voice tone.
- Latency and Performance – Evaluate the engine’s processing speed, particularly if your application requires real-time speech generation.
- Cost – TTS engines may have different pricing models, ranging from open-source to subscription-based services. Make sure the engine fits your budget.
Popular Speech Synthesis Engines Comparison
Engine | Voice Quality | Languages Supported | Customization | Pricing |
---|---|---|---|---|
Google Cloud Text-to-Speech | High | Multiple | Advanced | Subscription |
Amy Speech | Moderate | Few | Basic | Free/Paid |
Amazon Polly | High | Multiple | Advanced | Pay-per-use |
Tip: Open-source options like eSpeak and Festival can be a great choice for developers looking for free, customizable engines, but they may lack the advanced naturalness of commercial engines.
Setting Up Your Development Environment for Text to Speech
Before you start creating your text-to-speech software, it's essential to establish a solid development environment. This involves setting up the necessary tools, libraries, and frameworks that will allow you to implement text-to-speech features efficiently. A good environment ensures that your software development process is smooth, with minimal distractions or issues related to incompatible versions or missing dependencies.
When preparing your workspace, you should consider both the hardware and software requirements for running your speech synthesis application. It's crucial to have access to the proper text-to-speech libraries and APIs, as well as a suitable programming language. Below, we will walk through the key steps for setting up your environment and selecting the right tools.
Required Tools and Libraries
- Programming Language: Choose a language that supports speech synthesis libraries, such as Python, Java, or C#.
- Text-to-Speech Libraries: Use pre-built libraries for speech synthesis like Google Text-to-Speech (gTTS), pyttsx3, or Amazon Polly API.
- Speech Recognition Libraries: If you need both speech synthesis and recognition, consider integrating libraries like SpeechRecognition or DeepSpeech.
- IDE or Code Editor: A good code editor like VS Code or Pycharm will help with syntax highlighting and debugging.
Steps to Set Up Your Environment
- Install Programming Language: Download and install the required programming language (Python, Java, etc.) from its official website.
- Set Up Package Manager: For Python, install pip for managing libraries. For Java, set up Maven or Gradle for dependency management.
- Install Speech Synthesis Libraries: Install the necessary libraries for text-to-speech functionality using the package manager. For Python, run
pip install gTTS pyttsx3
. - Configure APIs: If you're using external APIs like Amazon Polly, sign up and get your API keys to integrate them into your application.
Example Setup Table
Tool | Description | Installation Command |
---|---|---|
Python | Programming language for scripting | sudo apt-get install python3 |
gTTS | Google Text-to-Speech library for speech synthesis | pip install gTTS |
pyttsx3 | Offline text-to-speech library | pip install pyttsx3 |
Note: When choosing a text-to-speech library, consider whether you need online or offline capabilities, as some libraries require an internet connection for API access.
How to Process Text Input for Accurate Speech Output
Effective text-to-speech (TTS) conversion relies heavily on processing text input in a way that ensures natural and accurate speech output. This involves not just converting written words into spoken language, but also handling linguistic nuances, context, and tone. In order to achieve high-quality TTS, it's essential to break down the text into smaller, meaningful units and apply linguistic rules to generate a human-like voice.
The first step in processing text for TTS is to analyze the text structure, ensuring that punctuation, sentence boundaries, and other syntactic elements are correctly identified. This allows the TTS system to properly pause, emphasize, and modulate the voice, producing a more realistic and understandable output.
Text Segmentation and Parsing
Text segmentation is crucial for determining sentence and phrase boundaries. This step involves:
- Identifying punctuation marks that indicate pauses or changes in intonation.
- Breaking down complex sentences into smaller units for easier processing.
- Tagging and categorizing words based on their part of speech (e.g., nouns, verbs, adjectives).
By properly segmenting and categorizing text, the TTS system can better understand the structure and meaning of the input, which directly impacts the quality of the speech output.
Pronunciation and Phonetic Conversion
Once the text is segmented, the next step is converting words into their phonetic representation. This process involves:
- Utilizing a dictionary or algorithm to map words to their corresponding phonemes.
- Handling exceptions, such as homophones, contractions, and proper nouns.
- Applying prosody rules to adjust stress and intonation patterns based on the context.
Accurate pronunciation relies not only on phonetic conversion but also on understanding the linguistic context in which the word appears.
Contextual Adjustments
Context is key for generating realistic speech. For example, the word "lead" can be pronounced differently depending on whether it refers to the metal or the act of leading. Contextual adjustments are made by analyzing the surrounding words, sentence structure, and intended meaning.
Word | Pronunciation (contextual) |
---|---|
lead | led (metal) |
lead | leed (to guide) |
By applying these methods, a TTS system can achieve more accurate and contextually appropriate speech output.
Integrating Natural-Sounding Voices into Your TTS Software
To create a high-quality Text-to-Speech (TTS) application, one of the most important aspects is to ensure the generated voices sound natural and engaging. The core challenge lies in simulating the nuances of human speech, such as intonation, rhythm, and emotion, without making the voice sound robotic. This requires leveraging advanced speech synthesis technologies, such as deep learning models, neural networks, and pre-recorded voice data. To achieve naturalness, it is essential to understand both the technical and linguistic aspects of speech generation.
Several key techniques are used to integrate natural-sounding voices into TTS systems, each focusing on different elements of speech. These include prosody modeling, voice cloning, and real-time adjustment of speech parameters. Below are a few strategies to improve the human-like qualities of TTS voices.
Key Approaches for Natural Speech Integration
- Prosody Modeling: This technique focuses on controlling the pitch, speed, and rhythm of speech. By varying these elements appropriately, the voice becomes more expressive and less monotonous.
- Voice Cloning: Utilizing deep learning techniques, this method enables the creation of custom voices based on specific speakers, making the TTS software capable of mimicking real human tones and accents.
- Emotional Speech Synthesis: Incorporating emotion into speech is crucial for making a voice sound more authentic. This can be achieved by adjusting speech dynamics based on emotional cues in the text.
Technical Tools and Models for Natural Sounding Voices
- WaveNet: A deep neural network model that generates raw audio waveforms directly. It produces highly realistic human speech by capturing subtle speech features.
- Tacotron: A sequence-to-sequence model that converts text to mel-spectrograms, which are then turned into audio. Tacotron significantly improves the clarity and expressiveness of synthetic speech.
- FastSpeech: A model that improves upon Tacotron by speeding up the synthesis process while maintaining high-quality speech output.
Important Considerations
High-quality TTS systems require large, diverse datasets of human speech recordings to train the models effectively. Ensuring the inclusion of various voices, accents, and emotional tones will lead to more versatile and natural-sounding outputs.
Voice Quality Comparison
Model | Realism | Processing Speed |
---|---|---|
WaveNet | Very High | Slow |
Tacotron | High | Moderate |
FastSpeech | High | Fast |
Handling Punctuation and Prosody for Realistic Speech
For a Text-to-Speech (TTS) system to produce natural and lifelike speech, it's crucial to properly manage punctuation and prosodic elements such as intonation, rhythm, and emphasis. Punctuation marks play a vital role in guiding the TTS engine on how to segment text and where to pause, while prosody helps shape the emotional and conversational tone of the output. By addressing these elements correctly, the synthesized speech will sound more human-like and less robotic.
Effective prosody involves adjusting speech patterns to mirror natural human conversations. This includes controlling pitch, rate, stress, and pauses. Proper punctuation also helps in determining where these prosodic features should change, which directly affects how a listener perceives the generated speech.
Managing Punctuation in TTS Systems
Punctuation serves as a primary tool for structuring speech and adding meaning to the text. Below is an overview of common punctuation marks and their effects on speech generation:
- Commas (,): Indicate a short pause or slight change in tone.
- Periods (.): Represent a full stop, causing a longer pause.
- Question Marks (?): Signal an upward inflection at the end of a sentence, indicating a question.
- Exclamation Marks (!): Trigger an increase in pitch and emphasis to convey excitement or surprise.
- Ellipses (...): Imply a pause for reflection or unfinished thought.
Prosody Control Techniques
In TTS systems, prosody is managed through algorithms that simulate human speech patterns. These techniques involve adjustments to several key elements:
- Pitch: Varying the pitch helps convey emotions, questions, or statements.
- Speech Rate: A slower rate can convey solemnity, while a faster pace is used for excitement or urgency.
- Stress: Emphasizing certain syllables or words to give importance or indicate meaning.
- Pauses: Inserting appropriate pauses allows for better comprehension and flow, as seen in natural speech.
Key Considerations for Accurate Prosody
Prosody Element | Impact on Speech |
---|---|
Pitch | Adjusts the overall tone and mood of the speech. |
Rate | Affects the perceived emotion and urgency of the speech. |
Stress | Helps emphasize important words and clarify meaning. |
Pauses | Improves clarity and makes speech sound more natural. |
Important: Balancing punctuation and prosody is key to achieving realistic TTS. Excessive or insufficient pauses, incorrect pitch variations, or lack of stress can all lead to unnatural-sounding speech.
Optimizing TTS Performance for Speed and Quality
To build an effective Text-to-Speech (TTS) system, it is crucial to balance both the speed of processing and the naturalness of the voice output. Achieving this balance requires tuning various components of the TTS pipeline, including speech synthesis algorithms, voice models, and resource management. By focusing on specific areas, developers can improve the overall user experience and ensure efficient performance even with large datasets or real-time applications.
Key factors in optimizing TTS systems include using efficient machine learning models, reducing processing latency, and fine-tuning parameters like sampling rates, synthesis methods, and audio output formats. These optimizations often involve trade-offs between quality and computational cost, which must be carefully managed based on the application's requirements.
Factors Affecting TTS Performance
- Speech Synthesis Models: Different models, such as concatenative or neural network-based, offer varying levels of speed and quality. Neural networks tend to offer superior quality but may require more computational power.
- Voice Database Size: A large voice dataset can improve the richness of the generated speech, but it might slow down processing if not optimized.
- Real-Time Processing: For applications like virtual assistants, ensuring low-latency processing is critical, which often involves simplifying the model or reducing the output length.
Techniques for Optimizing Performance
- Model Pruning: Reducing the size of the neural network without significantly compromising quality can help speed up inference time.
- Parallelization: Leveraging multi-core processors and distributing workload can drastically reduce processing time, especially in real-time TTS systems.
- Audio Compression: Using formats like OPUS or MP3 can decrease the file size of the output without major quality loss, improving delivery speed and storage efficiency.
Optimizing for both speed and quality requires testing and iteration. It's essential to monitor how changes to one factor, such as increasing audio quality, may impact the response time.
Comparison of Optimization Methods
Optimization Method | Impact on Speed | Impact on Quality |
---|---|---|
Model Pruning | High | Moderate |
Parallelization | High | Low |
Audio Compression | Moderate | Low |
Implementing User Customization Features in Text to Speech Software
When developing text-to-speech (TTS) software, offering customization options for users is essential to ensure accessibility and personalization. Tailoring the voice, speech rate, and pitch can significantly enhance the user experience, making the software more adaptable to different needs and preferences. These features allow users to modify the output voice to better suit their individual requirements, from educational applications to accessibility for those with disabilities.
Effective customization involves providing a variety of controls that let users fine-tune the way text is spoken. This can range from adjusting the gender and accent of the voice to more advanced settings such as speech tempo and tone. Additionally, implementing real-time adjustments can improve the usability of TTS software, ensuring a more seamless interaction.
Voice Customization Options
Providing a variety of voice options helps users select the most suitable output for their needs. This can include:
- Gender: Users can choose between male, female, or non-binary voices.
- Accent: The software can offer voices in various accents, such as American, British, or Australian English.
- Age Group: Allowing users to select between child, adult, or senior voice profiles can help cater to different contexts.
Speech Rate and Pitch Control
Control over speech rate and pitch allows users to adapt the TTS output to their personal preferences. Important features in this area include:
- Speech Rate: Users can adjust how fast or slow the text is read aloud.
- Pitch: The ability to modify the pitch of the voice can help make the speech sound more natural or fitting for specific contexts.
Advanced Customization Settings
For users requiring more specific adjustments, advanced settings can offer additional options, such as:
Customization Type | Description |
---|---|
Volume Control | Allows users to adjust the volume of the TTS output for different environments. |
Voice Emphasis | Gives users the ability to change the emphasis or intonation in certain words for improved clarity or expressiveness. |
Important: Providing an easy-to-use interface for these customization features is crucial. Overcomplicating the settings may deter users from fully utilizing the software.
Testing and Debugging Your Text-to-Speech Application
Testing and debugging are crucial steps in the development of any software, and text-to-speech (TTS) systems are no exception. Ensuring that the application provides accurate and natural-sounding speech output requires thorough validation across various use cases. During the testing phase, it's essential to evaluate both the speech synthesis and its integration with the input processing system. This process includes checking for potential errors and ensuring smooth performance across different devices and platforms.
Debugging your TTS application can be complex, as it involves not only analyzing code for syntax or logical errors but also assessing the quality of speech output. Some issues may arise from incorrect handling of text, mispronunciations, or incorrect voice modulation. Therefore, it's important to address these aspects through systematic testing and careful debugging.
Common Testing Approaches
- Unit Testing: This involves testing individual components of your TTS system, such as text parsing, phoneme generation, and audio output. Unit tests ensure that each component works correctly in isolation.
- Integration Testing: Once unit tests are passed, integration testing checks how well the components interact with each other. It also ensures that the system processes text and converts it into speech accurately across different platforms.
- Performance Testing: Evaluate the TTS system’s performance under different conditions, including varying text lengths, processing speeds, and resource usage.
- End-User Testing: Conduct usability tests with real users to identify any unexpected behavior or user interface issues that may not have been detected in the development phase.
Debugging Strategies
- Log File Analysis: Log files provide detailed information about the internal state of your application. Review them to identify potential bottlenecks or error-prone areas, especially in text parsing and synthesis.
- Real-Time Monitoring: During the debugging process, use real-time monitoring tools to observe how the system processes input and generates speech. This can help in pinpointing performance issues or discrepancies in the output.
- Feedback Loops: Continuously gather feedback from end-users and integrate it into your debugging efforts. This helps in refining both the speech output and the user experience.
Important Testing Considerations
Key Focus Areas: Test your TTS application for multiple languages, regional accents, and various speaking styles. Ensure the system handles various text formats, including punctuation, special characters, and non-standard terms.
Test Type | Objective |
---|---|
Unit Test | Validate individual components (e.g., phoneme generation, voice modulation). |
Integration Test | Ensure components work together for accurate speech synthesis. |
End-User Test | Collect real-world feedback on voice quality and user interface issues. |