Speech to Text Api Docs
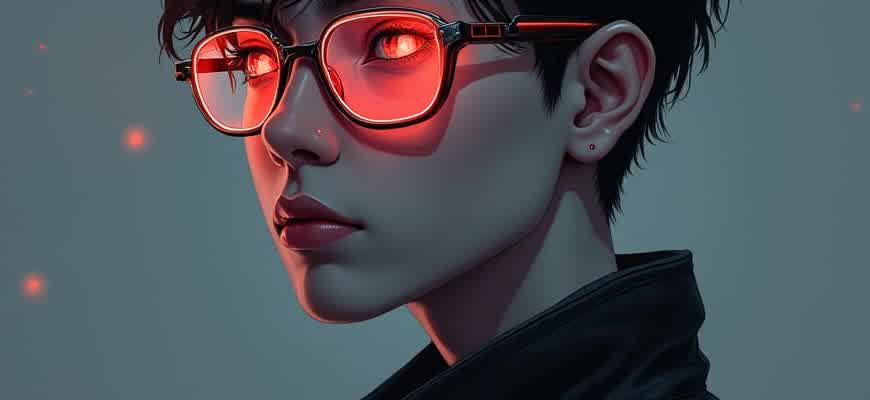
The Speech-to-Text API allows developers to integrate speech recognition into applications, enabling the conversion of audio into written text. This documentation outlines the key features, setup instructions, and usage guidelines for integrating the API into your projects.
To get started with the Speech-to-Text API, follow these steps:
- Sign up for an account and generate API keys.
- Set up the required dependencies in your development environment.
- Authenticate by passing your API key in the request headers.
Important: Ensure you have sufficient access permissions for the required audio data. Some endpoints may have usage limitations based on your account tier.
API responses include detailed information on transcription accuracy, language model selection, and audio processing time. Below is an overview of the most common response fields:
Field | Description |
---|---|
transcript | Text generated from the audio input |
language | The detected language of the spoken content |
confidence | Confidence score of the transcription accuracy |
Speech to Text API Documentation
The Speech to Text API enables developers to convert audio files into written text. By utilizing advanced speech recognition models, this service processes spoken language in various formats and outputs the transcribed text with high accuracy. It is commonly used in applications such as transcription services, voice commands, and automated subtitling.
To use this service, developers need to integrate the API into their systems, send audio data to the server, and retrieve the transcription results in a structured format. Below are key aspects to understand when working with the Speech to Text API.
Key Features
- Real-time transcription for live audio streams.
- Support for multiple languages and accents.
- Customizable models for specific industry terminologies.
- Secure data handling with encryption for sensitive information.
Authentication and Setup
To begin using the Speech to Text API, you must first authenticate via an API key. This key is used to authorize requests and track usage for billing purposes. The setup process typically involves the following steps:
- Register for an API key through the developer portal.
- Install the required SDK or make direct HTTP requests.
- Configure your application with the provided API key.
Note: Ensure that your API key is stored securely and never exposed publicly to prevent unauthorized access.
Request and Response Format
The API accepts audio in multiple formats (WAV, MP3, OGG) and provides responses in JSON format. The request contains parameters such as the language, audio file, and optional metadata. Below is an example of a typical request and response:
Field | Type | Description |
---|---|---|
audio_file | File | Path to the audio file to be transcribed. |
language | String | Language code (e.g., "en-US" for English). |
transcription | String | The transcribed text result. |
How to Integrate Speech Recognition API in Your Application
Integrating a speech recognition API into your application allows you to convert spoken language into text in real-time. This functionality is valuable for voice-activated commands, transcription services, and accessibility features. The process of setting up a speech-to-text API involves a few key steps, including choosing the right API, configuring your environment, and handling API responses effectively.
Before you start, ensure your application has access to the necessary permissions to record audio and interact with the chosen API. Commonly used speech recognition APIs include Google Cloud Speech-to-Text, Microsoft Azure Speech Service, and IBM Watson Speech-to-Text. Each API may have different methods of integration, so following the documentation carefully is crucial.
Steps to Integrate Speech Recognition API
- Choose an API: Select the API that best fits your requirements in terms of language support, accuracy, and cost.
- Set up authentication: Most APIs require API keys or other authentication mechanisms to ensure secure access.
- Install necessary libraries: Depending on your programming environment, you may need to install libraries or SDKs provided by the API provider.
- Capture audio: Integrate an audio capturing method in your application to collect voice data for processing.
- Send audio data to the API: Send the recorded audio in the correct format (usually as an audio file or audio stream) to the API for transcription.
- Handle API responses: Process the transcription result returned by the API, and display or store the converted text.
Here’s a simple overview of the process in a table format:
Step | Action | Details |
---|---|---|
1 | Choose API | Select based on features, pricing, and language support. |
2 | Authentication | Get API key or configure OAuth for secure access. |
3 | Install SDK | Follow installation instructions for the appropriate SDK or library. |
4 | Capture Audio | Use a microphone input or audio file. |
5 | Send Audio | Send audio to the API endpoint for transcription. |
6 | Process Response | Handle the text result and implement error handling. |
Important: Make sure to handle errors like network failures or audio issues to ensure a smooth user experience.
Configuring API Keys for Secure Access
To interact with a speech-to-text API securely, you must first obtain and configure API keys. These keys serve as unique identifiers that authenticate and authorize access to the service, ensuring that only authorized users can send requests. Setting up the keys properly is essential to prevent unauthorized usage and to maintain the integrity of your data.
Each API provider has a different process for generating and managing API keys. Typically, the key is generated through the provider’s dashboard after you register for an account. Once obtained, these keys must be securely stored and included in your API requests for validation.
Steps to Generate and Implement API Keys
- Register for an account with the speech-to-text service provider.
- Navigate to the API section in the provider’s dashboard.
- Click on "Generate New API Key" or a similar option.
- Store the API key securely. Do not expose it in public code repositories.
- Include the key in your API requests as a header or query parameter, depending on the service documentation.
Important: Never expose your API key in client-side code, as it can be easily extracted. Use server-side solutions to keep the key hidden from unauthorized access.
Best Practices for API Key Management
- Regularly rotate your API keys to mitigate the risk of compromise.
- Use environment variables to store keys securely in your server's configuration.
- Set permissions for API keys to limit access to only necessary resources.
- Monitor API usage regularly to identify any unusual activity or potential security breaches.
API Key Security Table
Action | Recommendation |
---|---|
Storing API Keys | Use environment variables or secure vaults, such as AWS Secrets Manager. |
Including API Keys in Requests | Use HTTPS for secure transmission of keys in API requests. |
Key Expiry | Set expiration dates for API keys to limit their lifespan. |
Handling Real-time Audio Streams with Speech Recognition API
Real-time audio processing is a critical feature for many applications, such as voice assistants, transcription services, and customer support systems. With speech-to-text APIs, developers can convert audio streams into text as they are being captured. This allows for interactive and responsive user experiences. However, handling audio streams in real-time introduces certain challenges, such as latency, data synchronization, and error correction.
To efficiently work with continuous audio data, the API must be able to process incoming sound without delays and maintain high accuracy. Real-time streaming requires careful management of audio buffers and error handling to ensure that the transcription is both accurate and timely. The following strategies and tools can be used to manage these challenges:
Key Considerations for Real-time Audio Stream Handling
- Buffer Management: Ensure that audio data is processed in manageable chunks to avoid memory overflow and maintain smooth transcription.
- Latency Reduction: Minimize processing time between capturing audio and displaying the transcribed text to users.
- Data Synchronization: Ensure the alignment of the incoming audio with the transcribed text, especially in noisy or complex environments.
- Error Handling: Implement strategies to deal with interruptions or unclear speech, providing real-time feedback to users.
Implementation Workflow
- Start Streaming: Capture and send audio data continuously to the speech-to-text API.
- Real-time Transcription: The API processes the audio stream and outputs transcribed text.
- Handle Errors and Corrections: Use techniques like confidence scoring or fallback models to improve transcription quality.
- Display Transcribed Text: Continuously show the real-time output on the user interface with minimal delay.
Note: Implementing real-time speech recognition requires significant optimization for latency and network reliability. Any interruptions or delays in the audio stream can affect the quality of transcription.
Example of Audio Stream Settings
Setting | Description |
---|---|
Audio Format | Choose between formats like PCM, MP3, or WAV for optimal streaming quality. |
Buffer Size | Adjust the buffer size to balance between real-time processing and memory usage. |
Sampling Rate | Higher sampling rates offer better audio quality but may increase latency. |
Optimizing Audio Quality for Accurate Transcription
Ensuring high-quality audio input is crucial for achieving precise transcription results when using speech-to-text APIs. Poor audio conditions, such as background noise, distorted sound, or low volume, can significantly impact the accuracy of the transcribed text. By improving audio quality before sending it for processing, users can enhance transcription performance and minimize the need for manual corrections.
Key factors that influence transcription accuracy include microphone quality, environmental conditions, and audio file specifications. Below are some tips to optimize audio quality for better transcription outcomes.
Best Practices for Improving Audio Quality
- Use a high-quality microphone: Invest in a good microphone that can capture clear sound and reduce background noise.
- Minimize background noise: Record in a quiet environment to avoid interference from sounds like traffic or people talking.
- Adjust microphone position: Ensure the microphone is placed at an appropriate distance from the speaker to avoid distortion.
- Ensure consistent volume levels: Speak at a steady volume to help the API detect speech patterns more accurately.
Audio File Settings and Format
- Sample Rate: Use a sample rate of at least 16kHz for clear voice capture.
- Audio Codec: WAV or FLAC are recommended for higher quality; MP3 may lose some clarity.
- Bitrate: Aim for a bitrate of 128kbps or higher for optimal sound fidelity.
Common Audio Issues to Avoid
Issue | Impact | Solution |
---|---|---|
Background noise | Can lead to errors in transcription and misidentification of words. | Use noise-canceling equipment and record in a quiet space. |
Low volume | Speech may not be detected accurately, leading to missed words. | Adjust input gain and test microphone sensitivity. |
Distorted audio | Distortion can cause parts of the speech to be unrecognizable. | Ensure the microphone is properly connected and use proper recording levels. |
Note: For the best results, always test your audio quality before submitting it to the speech-to-text API, making necessary adjustments as needed.
Managing Different Language Models with Speech to Text API
When working with a speech-to-text API, the ability to handle various language models is crucial for achieving accurate transcription results. Different languages have distinct phonetic and grammatical rules, and a model optimized for one language might not work well for another. Therefore, it's essential to configure the API to use the correct language model for each use case to ensure high transcription quality.
Many speech-to-text services offer multiple language models, each tailored to specific languages or regions. Depending on your application's needs, you may need to switch between these models or even fine-tune them for specific domains, like medical or legal transcription. This flexibility allows developers to create more precise and efficient transcription systems.
Types of Language Models
- General Language Models: These are pre-trained models optimized for general transcription across a wide range of languages.
- Domain-Specific Models: These models are fine-tuned for specific fields like healthcare, finance, or legal industries, improving accuracy in specialized terminology.
- Accent or Regional Variants: Some models are trained to recognize specific regional accents, improving transcription quality in non-native speech patterns.
Managing Language Models
Managing different language models requires the following steps:
- Selection: Choose the appropriate language model based on the target language and domain.
- Configuration: Set up the API to recognize the model parameters for language and dialect preferences.
- Optimization: Fine-tune models for specific use cases to enhance accuracy.
- Switching: Implement functionality to dynamically switch between models based on real-time needs.
For example, when transcribing a medical interview, it's crucial to switch to a domain-specific model trained on medical terminology to ensure accurate results.
Table of Supported Language Models
Language | Model Type | Use Case |
---|---|---|
English | General | Standard transcription tasks |
Spanish | General | Common transcription tasks for Spanish speakers |
French | Regional | Transcription for French-Canadian dialects |
German | Domain-Specific | Legal transcription |
Common Issues and Solutions for Speech-to-Text API Requests
When working with Speech-to-Text APIs, users often encounter specific issues that can interfere with the success of transcribing audio. Troubleshooting these errors efficiently is key to ensuring accurate and smooth conversion. This section will help you identify and resolve the most common problems that arise when using Speech-to-Text services.
To avoid frequent errors and reduce downtime, it's essential to understand the potential issues and follow best practices. Below are common problems and the steps to resolve them.
1. Invalid Audio Format
One of the most frequent issues is uploading audio in an unsupported format. This can cause the API to fail to process the request. Check if the audio file adheres to the API's supported formats, such as WAV, MP3, or FLAC.
Ensure the correct codec is used when recording or converting audio files.
2. Network or Connectivity Issues
Speech-to-Text services rely on a stable internet connection. If the network is unstable or interrupted, the request might time out, leading to failed transcriptions.
- Check the internet connection for stability.
- Verify if the API server is reachable.
- Consider retrying the request with exponential backoff to mitigate temporary failures.
3. API Authentication Failure
Incorrect API keys or expired credentials can prevent the Speech-to-Text service from authenticating requests. Always verify that the credentials provided are valid and up to date.
Issue | Solution |
---|---|
Invalid API Key | Check if the key is correctly entered and has the required permissions. |
Expired Credentials | Generate new API credentials and update your configuration. |
4. Audio Quality Problems
Low-quality audio can lead to poor transcription results. Ensure that the audio is clear, with minimal background noise and distortion.
Consider using noise reduction tools or high-quality microphones when recording audio.
5. Incorrect Language or Locale Settings
Ensure the correct language code is specified for accurate transcription. Mismatched language settings can result in poor transcription accuracy.
- Verify the language code in the API request matches the audio language.
- Use the proper locale setting if dealing with dialects or regional variations.
Understanding API Rate Limits and Usage Quotas
API rate limits and usage quotas are essential concepts that determine how much you can interact with a service within a specified time period. They are imposed to ensure the stability and efficiency of the system by preventing overuse and ensuring fair access for all users. These restrictions are critical to understanding when and how to manage your API calls to avoid service interruptions or unexpected costs.
To avoid hitting limits, developers need to plan their API usage carefully. Many services have predefined limits based on factors like subscription tiers, traffic demands, or the nature of the requests made. Below, we’ll dive into the types of rate limiting and quotas often encountered in API documentation.
Types of Rate Limits
- Requests Per Minute (RPM): This limit restricts the number of requests you can make within a minute.
- Requests Per Hour (RPH): This limit imposes a cap on the number of requests per hour.
- Daily Limits: Some APIs may set a daily limit, where a user can only make a certain number of requests per day.
- Monthly Limits: A more extended limit for long-term planning, restricting API calls within a monthly period.
How Quotas and Limits Are Calculated
Important: Quotas may reset depending on your subscription level or the API provider’s policy. Ensure you check the reset period to optimize your usage.
API providers often use a combination of these types of limits to ensure that the service is not overwhelmed. Some common methods of calculation include:
- Fixed Limit: A set number of requests allowed, regardless of traffic or usage patterns.
- Sliding Window: A dynamic limit that adjusts based on usage over time.
- Leaky Bucket: A gradual reduction of requests per time window based on usage trends.
Tracking Your Usage
It’s essential to monitor your usage to prevent exceeding the allowed quotas. Many APIs offer built-in tools to help track your usage and notify you when you are nearing the limits.
Usage Metric | Limit | Reset Time |
---|---|---|
Requests Per Minute | 1000 | Every minute |
Requests Per Hour | 5000 | Every hour |
Requests Per Day | 10000 | Every 24 hours |
Best Practices for Storing and Using Transcribed Text Data
When dealing with transcribed speech data, proper management is essential to ensure both efficiency and security. Storing transcriptions requires attention to data structure, accessibility, and privacy concerns. To maximize the utility of transcriptions, it's important to establish a clear system for organizing and retrieving the data as needed. Below are key practices for handling transcribed speech data effectively.
Utilizing optimized formats and security protocols can significantly improve the management of transcribed data. By following well-established practices, users can avoid issues related to data loss, inefficiency, or unauthorized access. Here are the main recommendations for best practices when storing and using transcriptions.
Organizing Transcribed Data
- Use Structured Formats – Store transcribed data in structured formats such as JSON or CSV to maintain readability and easy retrieval.
- Implement Metadata – Include timestamps, speaker identification, and contextual tags to enhance searchability and relevance.
- Optimize Database Use – Choose a database system tailored for text storage, such as NoSQL for scalability or SQL for relational data.
Data Privacy and Security
It is critical to follow data protection regulations like GDPR and HIPAA to safeguard sensitive transcribed information from unauthorized access or misuse.
- Encryption – Encrypt both stored and transmitted data to ensure it remains secure at all times.
- Access Control – Implement role-based access control (RBAC) to restrict who can view or modify transcriptions.
- Data Retention – Keep transcriptions only as long as necessary for your specific use case and delete them when no longer needed.
Efficient Use of Transcribed Text
- Search and Indexing – Use full-text search tools and indexing techniques to quickly find relevant transcriptions based on keywords or phrases.
- Integration with Analytics – Integrate transcribed data into analytics platforms to gain insights from the text, such as sentiment analysis or keyword frequency.
- API Usage – Develop APIs that allow for seamless integration of transcription data into other applications and workflows.
Key Considerations
Consideration | Best Practice |
---|---|
Data Format | Use structured formats like JSON or CSV for ease of access and processing. |
Data Privacy | Ensure compliance with data protection laws and encrypt sensitive information. |
Access Control | Limit access to transcriptions based on user roles to prevent unauthorized usage. |