Speech Synthesis Tutorial
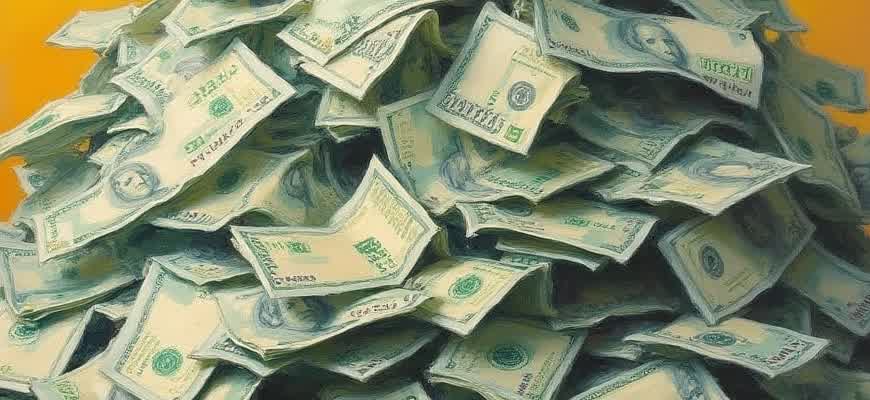
Speech synthesis is a process that converts written text into spoken words. This technology has evolved significantly, enabling machines to interact with humans in a natural and intelligible manner. In this tutorial, we will explore the core concepts behind speech synthesis and how to implement it in your projects.
Before diving into the implementation, it's important to understand the basic components that make up speech synthesis systems:
- Text Analysis: The system analyzes the text to determine the correct pronunciation, intonation, and pauses.
- Phonetic Transcription: The system converts text into phonetic representations to generate accurate sounds.
- Speech Generation: The system generates audio signals using pre-recorded sounds or a synthesized voice model.
Understanding these components is crucial for fine-tuning the output of the synthesis system.
To create a functional speech synthesis system, follow these basic steps:
- Choose a Speech Synthesis Library: Many libraries offer built-in speech synthesis features, such as Google Text-to-Speech, eSpeak, or pyttsx3.
- Prepare the Text: Ensure the input text is formatted properly and includes necessary punctuation for natural pauses.
- Configure the Voice Parameters: Customize the pitch, speed, and voice selection according to your needs.
Here's a simple comparison of popular speech synthesis libraries:
Library | Language Support | Platform | Features |
---|---|---|---|
Google Text-to-Speech | Multiple languages | Web, Android | High-quality voices, cloud-based |
eSpeak | Multiple languages | Linux, Windows | Lightweight, open-source |
pyttsx3 | English, others | Cross-platform | Offline, customizable voices |
How to Select the Best Text-to-Speech Engine for Your Application
Choosing the right text-to-speech (TTS) engine is crucial for ensuring that your application delivers a high-quality, natural-sounding speech output. The decision depends on various factors, such as the type of content being synthesized, the desired voice quality, and system requirements. Understanding your project's needs will guide you toward the most appropriate solution.
Several TTS engines are available on the market, each offering unique features, voice options, and integration capabilities. It’s important to evaluate these engines based on your specific requirements, such as voice clarity, language support, customization options, and pricing. Below are key considerations to help you make an informed decision.
Key Factors to Consider
- Voice Quality: Does the engine produce natural, clear, and engaging voices? Some engines offer more human-like voices than others, which is important for creating realistic and immersive experiences.
- Supported Languages: Ensure the engine supports the languages and dialects your project requires. If you need multilingual support, check whether the engine covers all necessary languages.
- Customization Options: Some TTS engines allow you to fine-tune the pitch, speed, and tone of the voice. Determine if these customization features are essential for your project.
- Integration Capabilities: The engine should integrate easily with your existing infrastructure. Look for APIs, SDKs, or platforms that match your development environment.
- Pricing: TTS services vary in cost, with some offering free plans for basic usage and others requiring a subscription or per-usage fees. Consider your budget and long-term usage needs.
Comparison Table
Feature | Engine A | Engine B | Engine C |
---|---|---|---|
Voice Quality | High | Medium | High |
Languages Supported | 50+ | 20+ | 30+ |
Customization Options | Advanced | Basic | Medium |
Pricing | Subscription | Free Plan Available | Pay-per-use |
Tip: Test different TTS engines before making a final decision. Many platforms offer demo versions or free trials that allow you to evaluate the voice quality and features first-hand.
Final Thoughts
When selecting a TTS engine, make sure to align its capabilities with your project's requirements. Whether you need lifelike voices for an audiobook app or simple speech for an accessibility feature, understanding the trade-offs between different engines will help you choose the best option.
Configuring Parameters to Customize Voice Tone and Speed
In speech synthesis, adjusting the voice tone and speed is crucial to creating a more natural-sounding output. By customizing these parameters, developers can fine-tune the synthetic voice to suit specific needs, whether it’s for navigation systems, virtual assistants, or audiobooks. Proper configuration of these settings ensures clarity, expressiveness, and appropriate pacing in spoken content.
The key settings that influence voice tone and speed are usually available in the speech synthesis API or software. These parameters can be adjusted individually to enhance the listening experience, ensuring that the voice matches the context and user preferences. In this section, we’ll discuss how to tweak these parameters effectively.
Adjusting Voice Speed
Speed control in speech synthesis determines how fast or slow the generated voice will speak. It’s important to balance between a pace that is too quick, making it hard for the listener to comprehend, and one that is too slow, which can sound unnatural. The speed parameter is typically set as a percentage of the default rate.
- Faster speeds: Useful for casual, energetic conversations or when there is a need to convey urgency.
- Slower speeds: Ideal for clarity, particularly in technical explanations or educational content.
Modifying Voice Tone
Voice tone refers to the emotional quality of the speech. A higher tone can make the voice sound more lively or cheerful, while a lower tone can create a serious or formal impression. Fine-tuning the tone allows for more expressive speech synthesis, enabling voices to match the intended emotional context.
- High tone: Often used for upbeat, friendly voices, like those of virtual assistants or customer service bots.
- Low tone: Best for serious applications, such as formal announcements or news reading.
Example Parameter Configuration
Parameter | Value | Effect |
---|---|---|
Speech Speed | 1.2x | Speeds up the voice, making it more energetic. |
Speech Tone | Low | Gives the voice a more serious or formal tone. |
To achieve a natural-sounding speech, always test your settings with real users to ensure the voice speed and tone are appropriate for the content and audience.
Integrating Speech Synthesis into Your Web Application
Speech synthesis is a powerful tool for enhancing user experience by converting text into speech. It can be easily integrated into web applications to improve accessibility, provide interactive content, and support voice-based interfaces. The Web Speech API, specifically the SpeechSynthesis interface, enables developers to add speech capabilities to their websites and applications.
To integrate speech synthesis, developers need to use JavaScript to interact with the SpeechSynthesis API. This API allows you to select different voices, adjust speech parameters, and control when speech starts or stops. Below is a basic guide on how to implement speech synthesis functionality in your web application.
Steps to Implement Speech Synthesis
- Access the SpeechSynthesis API: Use the
window.speechSynthesis
object to interact with speech synthesis features. - Create the SpeechSynthesisUtterance: Instantiate an utterance object that contains the text you want to be spoken.
- Configure Speech Parameters: Adjust properties like voice, pitch, and rate for a personalized experience.
- Start the Speech: Call the
speechSynthesis.speak()
method to begin speaking the text. - Handle Events: Use events like
onstart
,onend
, andonerror
to manage speech behavior.
Example Code
const utterance = new SpeechSynthesisUtterance("Hello, welcome to our site!"); utterance.voice = speechSynthesis.getVoices()[0]; // Select a voice utterance.pitch = 1; // Adjust pitch utterance.rate = 1; // Adjust rate speechSynthesis.speak(utterance);
Note: Always check browser compatibility as not all browsers support the SpeechSynthesis API equally.
Speech Synthesis Features
Feature | Description |
---|---|
Voice Selection | Choose from a variety of voices provided by the browser. |
Pitch Control | Adjust the pitch to make the voice sound higher or lower. |
Rate Control | Speed up or slow down the speech rate. |
Language Support | Set the language of the speech to match the desired audience. |
By utilizing these features, you can create a more interactive and engaging user experience. Be sure to test your implementation across various browsers to ensure consistent behavior.
Using SSML to Gain Full Control Over Speech Output
SSML (Speech Synthesis Markup Language) offers users the ability to fine-tune speech synthesis output, making it a valuable tool for developers and content creators. With SSML, it is possible to adjust various parameters, such as pitch, rate, volume, pauses, and emphasis, to generate a more natural and engaging voice experience. These adjustments help create a more tailored user interaction, whether it's for accessibility, virtual assistants, or voice-driven applications.
By utilizing SSML tags, developers can ensure that synthesized speech matches the desired tone and style. This gives them the flexibility to create voices that are more conversational, professional, or even dramatic, depending on the use case. Below are key SSML features that provide enhanced control over voice output.
Key SSML Features for Customizing Speech Output
- Pitch: Adjusting pitch allows for variation in the voice's frequency, helping to create different moods or emphasis.
- Rate: Controls the speed of speech. A slower rate may be used for clarity, while a faster rate could convey urgency.
- Volume: Adjusts how loud or soft the speech is, offering a dynamic listening experience.
- Emphasis: Used to highlight certain words or phrases, making them stand out for importance.
- Pauses: Adds natural breaks between sentences or phrases, mimicking human speech rhythms.
Practical Examples of SSML Tags
- <speak> – The root element of an SSML document that wraps the entire content.
- <voice> – Defines the specific voice used, such as male or female.
- <prosody> – Controls pitch, rate, and volume, allowing for detailed voice modulation.
- <break> – Introduces pauses of varying lengths between words or sentences.
- <emphasis> – Adds emphasis to words or phrases to alter their tone and impact.
Table of Common SSML Tags
Tag | Description | Example |
---|---|---|
<speak> | Wraps all SSML content | <speak>Hello World</speak> |
<prosody> | Modifies pitch, rate, and volume | <prosody rate="fast">This is quick!</prosody> |
<break> | Inserts a pause | <break time="500ms"> |
By applying SSML tags correctly, developers can significantly improve the quality of synthesized speech, making it sound more human-like and tailored to specific needs.
Handling Multilingual Speech and Regional Variants in Synthesis
Incorporating various languages and regional accents into speech synthesis requires a deep understanding of phonetics, prosody, and language-specific rules. Different languages have distinct sound systems, which must be accurately represented to ensure clear and natural speech output. For example, certain languages may use tones or stresses that don't exist in others, influencing both how sounds are generated and how they are perceived by the listener.
Furthermore, accents add another layer of complexity. Each accent carries unique speech patterns, including pronunciation variations, intonation, and rhythm. Properly managing these accents within a synthesis model is essential for making the generated speech sound authentic to native speakers.
Multilingual Synthesis Considerations
- Phonetic Mapping: Different languages have different phoneme inventories, so synthesis systems must adapt their models for each language's specific sounds.
- Prosody: Variations in pitch, timing, and stress patterns can differ widely between languages. It is important to ensure the generated speech mimics natural prosody for each language.
- Language Switching: Some applications require switching between languages on the fly. This needs seamless handling of context to avoid awkward pauses or mismatched intonations.
Accent Management
- Regional Variations: A single language may have multiple accents depending on the region. For instance, English spoken in the UK sounds different from that spoken in the US. Recognizing these variations is crucial for generating accurate speech.
- Contextual Adaptation: The system should be able to adjust to different accents based on user input or pre-set preferences. This ensures that speakers from different regions are represented authentically.
- Fine-tuning for Authenticity: To refine accent handling, continuous training on diverse voice samples from different regions is necessary for achieving realistic speech output.
Key Tools for Multilingual Synthesis
Tool | Language Support | Key Features |
---|---|---|
Google Cloud Text-to-Speech | Over 30 languages | Supports multiple accents, voice selection, and fine-tuning. |
AmazingTalker | English, Chinese, Spanish, and more | Focus on regional accents and tone adjustments for better cultural accuracy. |
Microsoft Azure Speech | 50+ languages and dialects | Language switching, accent recognition, and customizable voice models. |
When building multilingual speech systems, it is crucial to consider both the phonetic structure of each language and the cultural nuances that shape accents. Balancing these elements ensures that the system sounds natural and intelligible across a variety of linguistic backgrounds.
Improving Speech Synthesis Quality on Mobile and Low-Resource Devices
Optimizing speech synthesis for devices with limited resources is crucial for providing clear, natural-sounding audio while minimizing performance issues. On mobile platforms and low-resource devices, the computational power is often constrained, meaning developers need to make specific adjustments to balance speech quality and resource usage.
Speech synthesis algorithms need to be designed to work efficiently in environments where CPU and memory are limited. Achieving this involves employing a combination of hardware-aware optimization techniques, lightweight models, and carefully selected trade-offs between synthesis quality and resource consumption.
Key Techniques for Optimizing Speech Synthesis
- Model Compression: Reducing the size of neural network models or using less complex models can significantly reduce memory usage and processing power.
- Sample Rate Reduction: Lowering the audio sample rate minimizes computational load while still providing intelligible speech output.
- Resource Allocation: Prioritizing CPU and memory usage based on the specific task can optimize performance, reducing lag and stutter in synthesized speech.
Challenges Faced on Mobile Devices
Mobile devices face unique challenges in maintaining speech quality while conserving battery life and performance. This requires careful management of resources like CPU cycles, RAM, and network bandwidth.
- Battery Drain: Speech synthesis requires significant processing, which can rapidly deplete battery on mobile devices if not optimized correctly.
- Latency: Mobile devices may introduce latency in the speech output, especially when network calls are involved in fetching voice models or pre-recorded samples.
Comparison of Speech Synthesis Techniques
Technique | Pros | Cons |
---|---|---|
Waveform Generation | High quality, natural-sounding speech | High computational requirements, slow processing |
Parametric Synthesis | Lower resource usage, faster | Less natural sounding, robotic tone |
Unit Selection | Natural-sounding with minimal processing | Large model size, high memory consumption |
Testing and Debugging Speech Output for Clarity and Accuracy
When developing speech synthesis applications, it's critical to test the output for clarity and accuracy. The process involves checking the comprehensibility and correctness of the synthesized speech. It ensures that the generated speech sounds natural, delivers the intended message, and maintains consistency across different phrases and tones.
Effective debugging helps to identify issues such as mispronunciations, unnatural pauses, or inconsistencies in intonation. Testing should be done in various environments and on different devices to account for performance variations and quality differences in speech output.
Testing Process
- Check pronunciation accuracy for different words and phrases.
- Evaluate the pacing and pauses between words or sentences.
- Test the intonation and stress patterns to ensure natural speech flow.
- Verify speech output across multiple devices to ensure consistent quality.
Common Debugging Techniques
- Phonetic Analysis: Inspect the phonetic representation of the speech to identify errors in pronunciation.
- Volume and Pitch Adjustment: Adjust the volume or pitch to resolve issues related to clarity and natural sound.
- Pause Handling: Ensure proper pauses between phrases to avoid rushed or disjointed speech.
Key Points to Remember
Aspect | Importance |
---|---|
Pronunciation | Ensures the message is understood correctly without distortion. |
Intonation | Maintains natural sounding speech and avoids monotony. |
Pauses | Helps avoid rushed or awkward phrasing in speech output. |
Tip: Always test speech output with real users, if possible, to identify subtle issues that may not be apparent during automated testing.
Effective Integration of Speech Synthesis with UI Elements
When combining speech synthesis with user interface (UI) elements, it's important to ensure a seamless and intuitive experience. The goal is to enhance accessibility and usability while maintaining user engagement. This integration should be done in a way that complements the existing design and does not overwhelm or confuse the user.
To achieve this, UI elements such as buttons, forms, and interactive content should work in harmony with speech synthesis, providing clear, context-aware feedback. Effective interaction often relies on synchronizing auditory and visual elements, ensuring that they support each other without redundancy or distraction.
Best Practices for Combining Speech and UI Elements
- Contextual Feedback: Ensure that speech output is related to the UI element currently in focus, giving users meaningful and relevant information based on their actions.
- Accessibility Considerations: Provide speech synthesis for important UI components like buttons, form fields, or error messages to assist users with visual impairments.
- Paired Visual and Audio Cues: Avoid overwhelming users by combining speech with visual cues like animations or highlights, making it easier for users to process information.
Recommended Approaches
- Delay the speech output for non-critical elements: This avoids unnecessary interruptions when users interact with less important elements.
- Adjust speech speed: Allow users to modify the pace of speech synthesis to match their preference, making it more adaptable.
- Provide user controls for enabling/disabling speech: Some users may prefer a silent interface; ensure this option is easily accessible.
Key Considerations
Factor | Consideration |
---|---|
Context Awareness | Ensure that speech output is relevant to the user’s current task or location within the UI. |
Clarity | Use clear, concise language that avoids ambiguity or unnecessary jargon. |
Volume Control | Allow users to adjust the volume or mute speech output if needed. |
Tip: Avoid excessive use of speech synthesis for repetitive elements. Provide speech feedback for critical actions or important data, ensuring the experience is informative, not overwhelming.