Text to Speech Program in Python
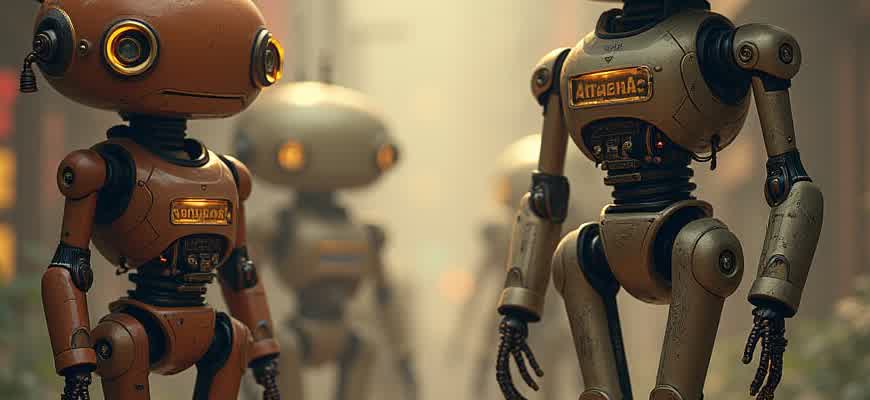
Text-to-speech (TTS) is a technology that enables computers to read aloud the written text. Python, with its vast library ecosystem, offers various tools to implement this functionality. By leveraging Python, developers can easily create applications that convert text into natural-sounding speech. Below, we outline the primary steps and libraries required to build a TTS program in Python.
Important note: TTS systems are widely used in accessibility tools, virtual assistants, and other applications that require human-like voice generation.
- Choose the right library: Python offers several libraries for speech synthesis, such as gTTS (Google Text-to-Speech) and pyttsx3.
- Install necessary packages: Use pip to install the required libraries.
- Set up the speech engine: Initialize the TTS engine to convert the text into speech.
Below is a simple example using the gTTS library:
import gTTS import os text = "Hello, welcome to the world of text to speech!" language = 'en' speech = gTTS(text=text, lang=language, slow=False) speech.save("output.mp3") os.system("start output.mp3")
This script takes the text input and saves the output as an MP3 file that can be played on your system. You can customize the voice, speed, and language as per your needs.
Choosing the Best Text to Speech Library for Your Python Project
When selecting a text-to-speech (TTS) library for your Python application, it's crucial to understand the specific requirements of your project. Various libraries offer different features, voice options, and ease of integration. The choice depends on factors such as speech quality, customization options, and compatibility with your system. Below are some important considerations to help you choose the right tool for your needs.
Understanding the different options available can simplify the decision-making process. Some libraries focus on delivering high-quality, natural-sounding speech, while others prioritize lightweight implementations with faster setup times. Let’s break down the key options and their capabilities to make your decision easier.
Key Factors to Consider
- Speech Quality: Evaluate how natural and clear the generated speech sounds. Some libraries offer more lifelike voices, while others might sound robotic.
- Supported Languages and Accents: Ensure that the library supports the languages and accents you need.
- Ease of Use: Look for libraries with simple APIs and good documentation.
- Platform Compatibility: Make sure the library works across your target platforms, whether that's Windows, macOS, or Linux.
- Customization: If your project requires unique speech patterns, check if the library allows fine-tuning the speech output.
Popular Text to Speech Libraries in Python
- gTTS (Google Text-to-Speech) - A simple and fast option that uses Google's text-to-speech API. It's easy to set up and supports many languages, but it requires an internet connection.
- pyttsx3 - A great choice for offline use. pyttsx3 supports multiple platforms and is highly customizable, though the speech quality can vary depending on the system.
- SpeechRecognition + SAPI5 - Best for integrating speech recognition with TTS, using Microsoft's SAPI5 engine on Windows. It’s useful for more complex applications that involve both speech input and output.
Tip: Consider the needs of your specific project, such as offline capabilities or multi-language support, to ensure you select the right library for your use case.
Comparison of Libraries
Library | Speech Quality | Offline Support | Customization | Supported Languages |
---|---|---|---|---|
gTTS | Good | No | Low | Multiple |
pyttsx3 | Moderate | Yes | High | Multiple |
SpeechRecognition + SAPI5 | Good | Yes | Moderate | English (default) |
Setting Up Your Python Environment for Text to Speech
Before you can begin developing a Text to Speech (TTS) application in Python, it’s important to ensure that your environment is properly configured. Python has several libraries that can assist with speech synthesis, and the right setup is crucial to ensuring smooth integration and functionality. This guide will walk you through the necessary steps to prepare your system for TTS development, focusing on the essential libraries and their installation.
The setup process involves installing Python itself, followed by specific dependencies such as TTS engines and supporting packages. Below, we’ll walk through the steps to install a popular text-to-speech library called pyttsx3 along with other tools you might need. Follow the instructions carefully to avoid common setup issues.
1. Install Python and Required Libraries
- First, make sure Python is installed on your system. You can check by running
python --version
in the terminal. If it’s not installed, download the latest version from python.org. - Next, install the pyttsx3 library. This can be done through pip by running the following command:
pip install pyttsx3
This will install the core TTS engine, which can convert text into speech across various platforms.
2. Verify the Installation
After installation, it’s important to verify that everything is working as expected. You can do so by testing the installation with a simple script. Here’s an example:
import pyttsx3 engine = pyttsx3.init() engine.say("Hello, welcome to text to speech!") engine.runAndWait()
If you hear the speech output, the installation was successful.
3. Optional: Install Additional Libraries
- If you want more advanced features, consider installing SpeechRecognition for voice input, or gTTS for Google-powered text-to-speech:
pip install SpeechRecognition
pip install gTTS
These libraries can be used in combination to create more dynamic voice-interactive applications.
4. Troubleshooting Common Issues
Problem | Solution |
---|---|
No sound output | Check that your system audio drivers are properly installed and that the volume is up. |
Installation errors | Ensure that pip and Python are properly installed, and check for any missing dependencies. |
Invalid voices | Verify that your system supports the voices you’re trying to use. You may need to install additional TTS packages for custom voices. |
Converting Text to Speech with gTTS: A Simple Approach
Python offers multiple libraries for text-to-speech (TTS) conversion, and one of the simplest methods is using the gTTS (Google Text-to-Speech) library. gTTS leverages Google's Text-to-Speech API, which is fast, easy to use, and supports various languages. This method allows developers to quickly convert text content into speech with minimal effort.
To begin, gTTS requires only a few lines of code. By importing the library and calling its functions, users can convert text input into an audio file (MP3 format). This makes it an ideal choice for creating applications with speech output, such as voice assistants or interactive applications.
Steps for Converting Text to Speech Using gTTS
- Install the gTTS library using pip:
pip install gTTS
- Import the gTTS module into your script:
from gtts import gTTS
- Pass the text string and desired language code to the gTTS function:
tts = gTTS('Hello, how are you?', lang='en')
- Save the resulting speech to an audio file:
tts.save('output.mp3')
- Play the audio file with any standard audio player or Python's
playsound
module
Example Code
from gtts import gTTS import os text = "Hello, welcome to the Python tutorial on gTTS." tts = gTTS(text, lang='en') tts.save("output.mp3") os.system("start output.mp3")
Key Benefits of Using gTTS
gTTS is a simple and effective solution for converting text to speech, supporting a wide range of languages. It is an excellent tool for projects where quick integration of speech synthesis is required.
Advanced Options
- Support for different languages and accents (e.g.,
lang='en', lang='fr', lang='de'
) - Customizable speech speed using the
slow
parameter (e.g.,tts = gTTS(text, slow=True)
) - Ability to change audio file formats, although MP3 is the default
Comparison of gTTS and Other TTS Libraries
Feature | gTTS | pyttsx3 |
---|---|---|
Language Support | Multiple languages | Multiple languages |
Offline Functionality | Requires internet | Offline support |
Custom Voice | No | Yes |
Installation | Easy, via pip | Requires more setup |
Advanced Control with Pyttsx3: Customizing Speech Speed and Volume
When working with text-to-speech systems, such as Pyttsx3 in Python, it's crucial to have full control over the generated speech's characteristics. This includes modifying the speed at which the speech is delivered and adjusting its volume for better clarity or effect. Fine-tuning these parameters can significantly improve the user experience, making it more natural and tailored to specific needs.
Pyttsx3 provides a straightforward way to manipulate these speech properties. By interacting with the engine's properties, you can easily adjust both the rate and volume of the speech synthesis, allowing you to create a customized auditory experience. This can be particularly useful for applications in accessibility, entertainment, or automation systems that require dynamic speech output.
Modifying Speech Rate
The rate of speech refers to the speed at which the words are spoken. By default, Pyttsx3 uses a moderate pace, but you can adjust this to suit your needs. The rate can be set as a value between 100 and 500 words per minute, where a higher value results in faster speech.
To adjust the rate, use the following code:
engine = pyttsx3.init() rate = engine.getProperty('rate') engine.setProperty('rate', rate + 50) # Increase rate by 50
Adjusting Volume
Volume control is another critical aspect, especially when you need the speech output to be louder or softer. The default volume is set to 1.0 (100%), but it can be modified to a range between 0.0 (mute) and 1.0 (maximum volume).
To change the volume, you can use the following command:
engine = pyttsx3.init() volume = engine.getProperty('volume') engine.setProperty('volume', 0.8) # Set volume to 80%
Summary of Control Parameters
Property | Default Value | Range | Description |
---|---|---|---|
Rate | 200 | 100 - 500 | Speech speed in words per minute |
Volume | 1.0 | 0.0 - 1.0 | Speech loudness |
Practical Tips
- Test different rate settings to find a speed that suits your application or audience.
- Consider adjusting the volume based on the environment where the speech will be played.
- Ensure that the rate and volume settings work well together to avoid distortion or unclear speech.
Conclusion
By understanding and utilizing the rate and volume controls in Pyttsx3, you can significantly enhance the usability of your text-to-speech applications. These simple yet effective customizations help you cater to specific needs, ensuring that your program sounds just the way you want it.
Handling Multiple Languages in Text to Speech Programs
Integrating multiple languages in text-to-speech systems requires careful consideration of language-specific features. A TTS system must be able to correctly interpret and generate speech in different languages, accounting for distinct phonetics, syntax, and intonation patterns. This ensures that the speech output is both natural-sounding and intelligible in the target language.
One of the main challenges when supporting multiple languages is ensuring that the text is parsed and pronounced accurately. Different languages may require distinct linguistic models, voice databases, and pronunciation rules, which makes handling a wide range of languages more complex. Additionally, some languages may not have as robust TTS support, leading to limitations in available voices or accuracy in speech synthesis.
Key Considerations for Multilingual TTS Systems
- Language Detection: Automatic identification of the language of input text is crucial for switching between appropriate voice models.
- Voice Selection: Each language may require a separate voice model. Users should be able to select voices that match their linguistic needs.
- Phonetic Representation: Different languages have different phonetic structures that require specialized algorithms to accurately convert text to speech.
- Regional Variations: Even within a single language, regional accents and dialects may require distinct speech synthesis models.
Approaches for Supporting Multiple Languages
- Predefined Language Models: Create separate models for each supported language, ensuring the highest quality output for each.
- Dynamic Language Switching: Implement real-time language detection, which automatically switches between models based on the language of the input text.
- Customizable User Input: Allow users to manually select the language and voice they wish to use, ensuring flexibility.
Important: When designing a multilingual TTS system, it's essential to ensure that the text-to-speech conversion is contextually accurate for each language, as direct translations might result in mispronunciations or unnatural speech.
Comparison of TTS Support for Various Languages
Language | Voice Availability | Phonetic Complexity | Accent Support |
---|---|---|---|
English | High | Moderate | Multiple |
Mandarin Chinese | Moderate | High | Limited |
Spanish | High | Moderate | Multiple |
Arabic | Low | High | Limited |
Integrating Voice Synthesis with Real-Time User Input
Real-time applications often require immediate processing of user input, and when combined with text-to-speech (TTS) functionality, it allows for a more interactive user experience. One of the challenges in integrating TTS with real-time input is ensuring that the voice synthesis system can respond quickly and accurately to user commands, which can range from simple instructions to more complex queries. By utilizing Python libraries, developers can create systems that convert text into speech as soon as it's entered by the user.
To implement this, Python provides several libraries like pyttsx3 or gTTS for converting text into speech. The integration of these tools with real-time user input can be done through event-driven programming, ensuring that the system responds immediately. The flow typically involves capturing user input, processing it, and then feeding the resulting text to the TTS engine for instant audio output.
Steps for Integration
- Capture user input via a graphical user interface (GUI) or command-line interface (CLI).
- Process the input text for any commands or information.
- Feed the processed text into the TTS engine.
- Output the synthesized speech in real-time.
Example: Real-Time Text to Speech
- User types a message or command into the interface.
- The program processes the input (e.g., checking for errors or specific commands).
- The processed text is sent to a TTS engine like pyttsx3.
- The engine immediately converts the text to speech and plays it back to the user.
Note: For a more fluid experience, ensure that the system can handle multiple inputs without lag. This may involve optimizing the TTS engine's speed and responsiveness.
Table: TTS Library Features
Library | Key Features | Pros | Cons |
---|---|---|---|
pyttsx3 | Offline functionality, supports multiple platforms | Fast, no internet required | Voice quality is not as natural as some online engines |
gTTS | Online-based, Google-powered voices | High-quality, natural-sounding voices | Requires internet connection |
Saving and Exporting Audio Files in Python
When working with text-to-speech applications in Python, one common task is to save the generated audio to files for later use or distribution. By utilizing popular libraries like `gTTS` (Google Text-to-Speech) or `pyttsx3`, you can easily convert text into audio and save it in various formats such as MP3 or WAV. This process not only allows users to create voice outputs but also enables them to export and share these files across different platforms.
In Python, saving speech output can be done by specifying the desired file format and destination path. The following steps outline the basic process of converting text to audio and saving it as an audio file:
- Choose the right library: Popular libraries like `gTTS` or `pyttsx3` provide simple APIs to convert text to speech.
- Set file format: Most libraries allow you to choose between different file formats, such as `.mp3` for gTTS or `.wav` for pyttsx3.
- Save to file: The generated speech can be saved to a local file by specifying the path and name.
Below is an example demonstrating how to save audio using the `gTTS` library:
from gtts import gTTS text = "Hello, this is a speech output." tts = gTTS(text, lang='en') tts.save("output.mp3")
Note: Always ensure the correct path is provided for saving the file, and be cautious about file permissions when writing to directories.
Additional Export Options
For more advanced features, consider using `pyttsx3` for local speech synthesis, as it can be integrated with other audio processing libraries for deeper control over speech quality and effects. This library supports saving audio in `.wav` format, which is highly versatile for offline usage. Below is a comparison of the features:
Feature | gTTS | pyttsx3 |
---|---|---|
Offline Support | No | Yes |
Supported Formats | MP3 | WAV |
Speed Control | No | Yes |
Important: For offline capabilities, use pyttsx3 as gTTS requires an internet connection to work.
Troubleshooting Common Issues in Python Text to Speech Projects
When working on text-to-speech applications in Python, developers often encounter specific challenges that can disrupt the functionality of their programs. These issues can range from incorrect audio output to compatibility problems with speech synthesis libraries. Understanding the typical pitfalls and knowing how to address them can greatly improve the development experience.
To effectively troubleshoot these issues, it is important to have a systematic approach. Here are some common problems you might face, along with solutions and suggestions to resolve them efficiently.
Common Problems and Solutions
- Missing or Incorrect Dependencies: Many text-to-speech libraries require specific dependencies that may not be installed by default.
- Audio Output Not Playing: Sometimes, the program may run without errors but produce no audio.
- Voice Quality Issues: Text-to-speech synthesis can produce robotic or unnatural voices if the correct settings are not configured.
- Ensure all dependencies are installed using
pip install pyttsx3
or the relevant library. - Check that the audio output device is correctly set and that no conflicts exist between libraries.
- Test different speech engines or adjust voice parameters (rate, volume) to enhance audio quality.
Technical Details and Common Errors
Error Type | Solution |
---|---|
ModuleNotFoundError | Verify if the library is properly installed. Run pip install pyttsx3 . |
AudioDeviceError | Ensure that the sound output is configured correctly in your system settings. |
VoiceNotFoundError | Make sure the chosen voice is supported by the text-to-speech engine and correctly installed. |
Tip: If using pyttsx3, always check the available voices using
pyttsx3.init().getProperty('voices')
to ensure the right voice is being selected.