Elevenlabs Text to Speech Api Key
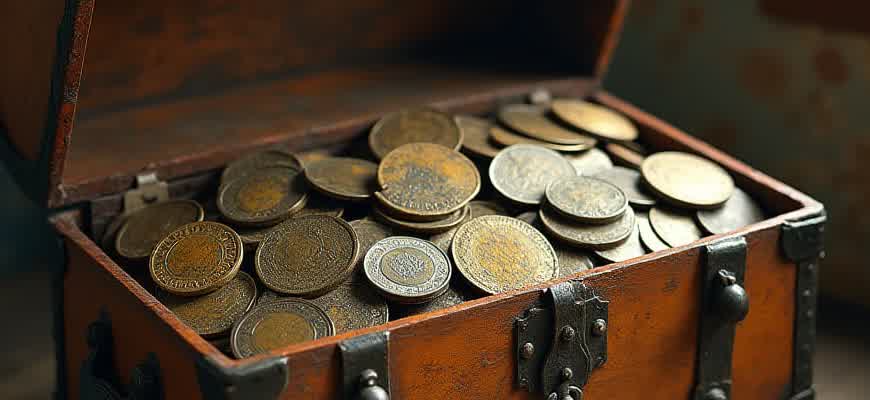
To start using the Elevenlabs Text to Speech API, you need to first acquire an API key. This key will allow you to authenticate requests and access various features provided by the platform.
The process of obtaining the key involves several straightforward steps:
- Visit the official Elevenlabs website.
- Create an account or log in to your existing account.
- Navigate to the API section of the dashboard.
- Generate a new API key, which will be provided to you immediately after.
Once you have your API key, you can start integrating the Text to Speech services into your application.
Important: Always store your API key securely and never share it publicly to prevent unauthorized access.
The key grants you access to a variety of speech synthesis features, such as language selection, voice customization, and audio output formats.
Feature | Available Options |
---|---|
Voice Selection | Multiple voices in different languages |
Audio Format | MP3, WAV, and more |
Customization | Pitch, speed, and tone adjustments |
How to Use Elevenlabs Text-to-Speech API Key in Your Project
The Elevenlabs Text-to-Speech API offers a powerful way to convert text into high-quality speech. By integrating the API into your project, you can easily add speech synthesis features to a variety of applications, such as voice assistants, e-learning platforms, or any app requiring dynamic text-to-speech conversion.
To leverage the API, you'll need to obtain an API key from Elevenlabs, which grants you access to their text-to-speech service. This key is essential for authenticating your requests and ensuring the smooth operation of your project. Here's how to get started:
Steps to Implement the Elevenlabs Text-to-Speech API
- Sign up for an account on the Elevenlabs website to obtain your API key.
- Access the API documentation on their platform to understand the parameters, endpoint, and usage guidelines.
- Integrate the API key into your application by adding the key to your headers for authentication.
- Make API requests by sending the text you want to convert, along with any additional options like voice type or language, to the relevant endpoint.
- Handle the audio response by receiving the speech output and embedding it in your app or website as needed.
Ensure to keep your API key secure and do not expose it in client-side code to prevent unauthorized usage.
API Parameters and Options
The Elevenlabs API allows you to customize your text-to-speech requests with various parameters. Below is a summary of some common options:
Parameter | Description |
---|---|
voice | Choose the desired voice model for speech synthesis. |
language | Select the language in which the speech should be generated. |
speed | Adjust the speech rate (e.g., slow, normal, fast). |
pitch | Modify the pitch of the generated speech. |
By experimenting with these parameters, you can fine-tune the voice output to match your project's requirements.
How to Get Your Elevenlabs Text to Speech API Key
To start using the Elevenlabs Text to Speech API, you first need to obtain an API key. This key allows you to make authorized requests to the platform’s service. Follow the steps below to generate and access your personal API key.
The process is straightforward but requires you to have an account with Elevenlabs. Once you’re logged in, you can easily generate the key from your dashboard. Here’s how to do it:
Steps to Obtain Your API Key
- Visit the official Elevenlabs website and log into your account.
- Navigate to the 'API' section in your user dashboard.
- Click on the "Generate API Key" button.
- Once generated, copy the key and store it securely.
Important: Keep your API key secure. Anyone with access to it can make requests on your behalf, potentially using up your credits or exposing your service to misuse.
API Key Details
Key Name | Details |
---|---|
API Key | A unique string generated by Elevenlabs to authenticate your API requests. |
Usage | Required for all interactions with the Elevenlabs Text to Speech service. |
Once you have your key, you can start integrating Elevenlabs' text-to-speech capabilities into your applications. Make sure to monitor usage through your dashboard to avoid hitting any limits associated with your plan.
Setting Up the Elevenlabs API Key in Your Application
Integrating Elevenlabs Text-to-Speech API into your application requires a few key steps, starting with obtaining your API key. This key is a unique identifier that grants your app access to Elevenlabs services. Once you have the API key, it can be used to authenticate requests and interact with the API for various functionalities like voice generation and text conversion.
Follow these instructions to properly set up and configure your Elevenlabs API key in your project. This guide assumes you have already registered for an account on Elevenlabs and received your personal API key.
Steps for Integration
- Obtain your API Key:
- Login to your Elevenlabs account.
- Navigate to the 'API Keys' section in your account settings.
- Copy the generated API key to use in your project.
- Set up the API Key in Your Application:
- Store the API key securely in your environment variables or a configuration file.
- Ensure that your application references this key when making requests to Elevenlabs API.
- Make API Calls:
- Use HTTP request libraries (like Axios or Fetch) to send requests to the API.
- Pass the API key in the authorization header of each request.
Note: Keep your API key private and never hard-code it directly in your application’s source code, especially if it's publicly accessible.
Sample API Request
Here’s an example of a simple request to convert text to speech using the Elevenlabs API:
const axios = require('axios'); const apiKey = 'your-api-key-here'; const url = 'https://api.elevenlabs.io/v1/speech/generate'; axios.post(url, { text: 'Hello, welcome to the Elevenlabs API!', voice: 'en_us_male', }, { headers: { 'Authorization': `Bearer ${apiKey}`, 'Content-Type': 'application/json' } }) .then(response => { console.log('Audio file:', response.data.audio_url); }) .catch(error => { console.error('Error:', error); });
Important Considerations
Consideration | Details |
---|---|
API Rate Limits | Be aware of the number of requests you can make within a given time frame to avoid hitting rate limits. |
Security | Always store your API key securely, preferably in environment variables or secret management systems. |
Pricing | Ensure you’re aware of the pricing structure, as excessive API calls may lead to increased costs. |
Understanding Pricing and Usage Limits of Elevenlabs API Key
When using the Elevenlabs Text-to-Speech API, it’s essential to understand the pricing structure and the associated usage limits. These factors directly influence how much you can use the service and the cost for different levels of access. Knowing these details allows for better planning and cost management when integrating text-to-speech functionality into your application.
The pricing for the Elevenlabs API is tiered, with different plans offering varying amounts of usage, features, and support. Understanding these tiers and limits is crucial to avoid unexpected charges and ensure that your usage aligns with your project needs.
Pricing Overview
- Free Tier: Limited usage with basic features, suitable for small projects or testing.
- Basic Plan: Increased usage limits and access to premium voices.
- Pro Plan: Higher limits, additional features like customization, and priority support.
- Enterprise Plan: Custom pricing for large-scale integrations and enhanced support.
Usage Limits
- Character Limit: Each plan has a specified number of characters that can be processed each month. Exceeding this limit may result in additional charges or service throttling.
- API Requests: The number of requests you can make per month is also capped, depending on your plan. If exceeded, API access may be limited or suspended.
- Voice Access: Certain plans offer access to more advanced voice models and customization options, while others may have only a selection of basic voices.
Important Considerations
Ensure that your plan aligns with your expected usage to prevent unexpected costs or service interruptions. Always review the usage limits in the API documentation before integrating it into your application.
Pricing Comparison
Plan | Monthly Price | Character Limit | API Requests |
---|---|---|---|
Free | $0 | 5,000 | 100 requests |
Basic | $19/month | 50,000 | 500 requests |
Pro | $49/month | 200,000 | 1,000 requests |
Enterprise | Custom | Custom | Custom |
Best Practices for Optimizing Audio Output with Elevenlabs TTS
When utilizing Elevenlabs' Text-to-Speech API, optimizing the audio output is crucial for ensuring natural, clear, and contextually appropriate speech. Proper tuning of various parameters can drastically improve the end-user experience, whether it’s for an AI assistant, an audiobook, or any other application where text is converted into speech.
Several key techniques can help refine the speech output, ranging from voice selection to adjusting speaking rate and volume. These settings allow developers to fine-tune the results based on the specific use case, ensuring the generated audio meets the intended tone and quality.
Key Parameters for Optimization
- Voice Selection: Choose a voice that fits the context of the content. Different voices have varying tonal qualities, which can affect the emotional impact and clarity of the speech.
- Speed and Rate: Adjust the speech rate to balance clarity and natural flow. A rate that’s too fast may lead to unclear pronunciation, while a slow rate might sound unnatural.
- Pitch Adjustment: Fine-tune the pitch to avoid monotony or excessive sharpness. A slight variation in pitch can enhance the naturalness of the voice.
- Pause Control: Implement pauses at logical points to enhance the natural pacing of speech, ensuring the listener can follow the content easily.
Advanced Customization Tips
- Emphasis and Intonation: Add emphasis to important words or phrases. This can be done through specific API features like SSML tags for stress and intonation.
- Testing Across Devices: Always test audio across multiple devices (smartphones, speakers, etc.) to ensure consistent quality and clarity.
- Volume Levels: Keep volume levels consistent to avoid distortions, especially in environments with background noise.
Key Settings Table
Setting | Description | Best Practice |
---|---|---|
Voice Type | Selection of the AI voice. | Pick a voice that aligns with content mood and audience demographics. |
Rate | Speech speed (words per minute). | Test multiple speeds and find the most natural-sounding rate for your use case. |
Pitch | Frequency of speech. | Avoid too high or low pitches; keep it within a comfortable range. |
Pauses | Strategic breaks between words/phrases. | Insert pauses where appropriate (e.g., after commas or sentence-ending punctuation). |
It’s important to remember that the goal is to make the generated speech sound as natural and human-like as possible. Fine-tuning these settings can make a significant difference in the overall user experience.
Integrating Elevenlabs Text-to-Speech API into Web and Mobile Applications
Integrating Elevenlabs' Text-to-Speech functionality into web and mobile apps offers developers a powerful way to enhance user experience with dynamic voice synthesis. This integration can be achieved using a simple API key that connects the app to Elevenlabs' advanced speech synthesis service. The process involves making API calls that convert text into realistic human-like speech, which can then be played back to the user. Such an integration is valuable for apps aimed at accessibility, e-learning, virtual assistants, and more.
To integrate the Elevenlabs Text-to-Speech API, developers need to follow specific steps. These steps usually involve obtaining an API key, setting up HTTP requests, handling responses, and managing audio playback. It’s important to consider both frontend and backend implementations to ensure smooth and seamless interactions. Below are key steps to integrate the API into your app:
Steps for Integration
- Obtain API Key: Sign up at Elevenlabs' platform and generate an API key for authentication.
- Set Up Backend Request: Configure your backend to send POST requests to the Elevenlabs API endpoint, passing the text to be converted into speech.
- Handle Audio Response: Receive the audio file (usually in MP3 or WAV format) as a response and use it within your application.
- Frontend Playback: For web apps, embed an audio player to play the synthesized speech. For mobile apps, use native audio libraries for smooth playback.
Considerations for Web and Mobile Apps
When incorporating text-to-speech features, it's crucial to handle network latency, error management, and audio quality. Below is a table outlining the differences between integrating the service in web and mobile environments:
Factor | Web Apps | Mobile Apps |
---|---|---|
API Integration | Typically involves JavaScript with Fetch or Axios for API requests. | Native SDKs or libraries (e.g., Retrofit for Android, Alamofire for iOS) to handle requests. |
Audio Playback | HTML5 Audio or JavaScript libraries (e.g., Howler.js) for audio playback. | Native audio player controls or libraries like AVPlayer (iOS) and MediaPlayer (Android). |
Authentication | API keys stored in JavaScript environment, possibly exposed in browser. | Secure key storage using mobile-specific solutions (Keychain for iOS, Keystore for Android). |
Note: Always ensure API keys are stored securely to prevent unauthorized access, especially in production environments.
Common Issues with Elevenlabs TTS API Key and How to Solve Them
When integrating the Elevenlabs Text-to-Speech API into your application, developers often encounter issues related to API keys. These problems can stem from incorrect configuration, usage limits, or security concerns. Addressing these issues quickly is crucial for smooth integration and consistent performance.
Below are some of the most common problems users face with the Elevenlabs TTS API Key and how to resolve them effectively.
1. Invalid API Key
If you encounter an error related to an invalid API key, it typically means the key you're using is incorrect, expired, or has been revoked. To resolve this, follow these steps:
- Ensure that the key is copied correctly from your Elevenlabs account.
- Check if the key has expired or been deactivated in the API dashboard.
- If necessary, generate a new API key from your Elevenlabs account settings.
Important: Always ensure your API key is stored securely and not exposed in public repositories or client-side code.
2. API Key Usage Limits
Exceeding the usage limits of your API key can lead to errors, such as rate-limiting or throttling. To prevent this, it’s essential to track your usage and adjust your implementation as needed. Consider these tips:
- Review the API documentation for rate limits associated with your plan.
- If you are hitting limits frequently, consider upgrading to a higher plan.
- Implement caching or batch processing to optimize requests.
3. Incorrect API Key Permissions
Sometimes the API key may not have the necessary permissions to access certain features. Ensure the key has the right scope for the specific resources you're trying to interact with. Here's how to resolve it:
- Go to the API key management page in your Elevenlabs account.
- Check that the key has the correct permissions for the intended tasks (e.g., text-to-speech, audio file generation).
- If necessary, regenerate the API key with the appropriate permissions enabled.
4. API Key Not Set Correctly in the Code
Incorrect configuration of the API key within your code can lead to failed requests. Ensure that the key is correctly passed in the authorization header or query parameters. Here's a checklist:
Issue | Solution |
---|---|
API key not set in the header | Make sure you add the API key in the Authorization header. |
Incorrect format | Ensure the key is prefixed with Bearer in the header (e.g., Bearer YOUR_API_KEY ). |
Tip: Double-check your code for typos or misplaced characters in the API key string.
Personalizing Voice Output with Elevenlabs API Key
The Elevenlabs API provides powerful tools to tailor voice output to your specific needs. With the API key, you can adjust various parameters such as pitch, speed, and tone, allowing for a more customized and natural voice generation. These features enable businesses and developers to create a unique auditory experience for their applications or services.
To get started with personalizing voice output, you need to understand the key elements that can be adjusted. By configuring settings through the Elevenlabs API, you can fine-tune the voice characteristics to suit your project. Below, we explore the common options available and how to apply them effectively.
Customizing Voice Parameters
There are several options you can adjust when personalizing voice output:
- Pitch: Controls the perceived height of the voice, making it higher or lower.
- Speed: Adjusts the rate at which the voice speaks, making it faster or slower.
- Volume: Allows you to control how loud or soft the voice sounds.
- Voice Type: Choose between different voice models to match the desired tone (e.g., formal, casual, friendly).
Steps to Personalize Voice Output
- Get an API key from the Elevenlabs platform.
- Choose the voice model you prefer for your project.
- Configure the voice parameters such as pitch, speed, and volume according to your requirements.
- Use the API to test and refine the output, making adjustments as necessary.
- Deploy the customized voice model in your application.
Parameter Settings Example
Parameter | Default Value | Range |
---|---|---|
Pitch | Medium | -5 to 5 |
Speed | Normal | 0.5 to 2.0 |
Volume | Medium | 0 to 100 |
Important: Always test your settings to ensure the final output meets your expectations. Small tweaks can have a significant impact on user experience.
Tracking and Analyzing API Usage for Better Management
Monitoring and evaluating API consumption is essential for optimizing performance, controlling costs, and ensuring efficient resource allocation. By carefully analyzing the usage data, businesses can identify potential issues early, prevent misuse, and ensure that the API meets its intended objectives. This allows for more effective decision-making and enhances the overall user experience.
To maintain control over API interactions, it's important to track key metrics such as call frequency, response times, and error rates. Analyzing this data provides insights into system performance, enabling improvements in both functionality and scalability. Additionally, robust tracking mechanisms help in detecting unusual usage patterns, which can prevent overloads and improve overall security.
Key Metrics to Track API Usage
- Request Volume: Number of requests made in a given time period.
- Response Time: Time taken for the API to respond to a request.
- Error Rate: Percentage of requests that result in errors.
- API Latency: Time it takes for data to travel between the client and server.
- Authentication Failures: Count of failed authentication attempts.
Steps to Improve API Usage Management
- Implement Usage Limits: Set rate limits to control the frequency of requests and prevent abuse.
- Analyze Traffic Patterns: Identify peak usage times to optimize resource allocation.
- Monitor Error Trends: Regularly review error logs to detect potential issues and resolve them.
- Use Analytics Tools: Employ tools to automatically track and generate detailed reports on API performance.
Important Considerations for Better API Tracking
Effective tracking helps businesses not only to understand current API usage but also to plan for future growth. It ensures that resources are allocated efficiently, and limits are set to avoid unnecessary downtime or outages.
Sample API Usage Analytics Table
Metric | Value |
---|---|
Requests per Day | 10,000 |
Average Response Time | 200 ms |
Error Rate | 0.5% |
Peak Traffic Time | 3:00 PM - 5:00 PM |