Text-to-speech Api Free Offline
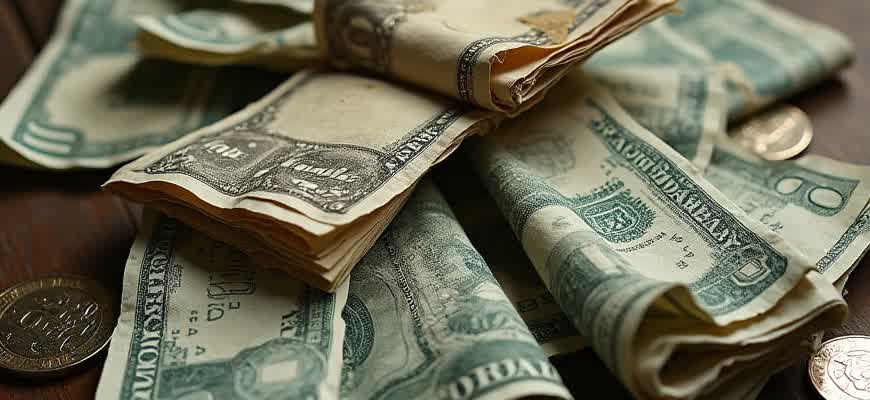
The increasing demand for text-to-speech technology has led to the development of various solutions, allowing developers to integrate voice synthesis into their applications. In particular, free offline APIs stand out for their ability to function without an internet connection, offering a more reliable and cost-effective alternative for many use cases.
Key benefits of offline text-to-speech APIs:
- Cost efficiency: Free options eliminate the need for cloud-based services, which often come with recurring charges.
- Privacy: Data does not need to be transmitted to a remote server, ensuring user privacy.
- Accessibility: Can be used in environments where internet access is unstable or unavailable.
Popular free offline text-to-speech APIs:
- eSpeak: An open-source software speech synthesizer for English and other languages.
- Festival: A general-purpose text-to-speech system with support for multiple languages and voices.
- ResponsiveVoice: Provides offline functionality in select environments, offering a variety of voices.
"Offline text-to-speech APIs provide the perfect balance between functionality and performance, enabling developers to create robust applications without relying on external servers."
For more detailed comparisons of these options, consider examining their documentation to assess compatibility and available features.
API | Supported Languages | License |
---|---|---|
eSpeak | English, French, German, and more | Open-source |
Festival | Multiple languages | Free |
ResponsiveVoice | English, Spanish, German, and others | Free for non-commercial use |
Offline Text-to-Speech API: A Practical Guide for Implementation
Offline Text-to-Speech (TTS) APIs provide the ability to convert text into speech without requiring an active internet connection. This is particularly useful for applications in remote areas or for use cases that require privacy and reduced latency. Implementing an offline TTS API is a valuable skill for developers looking to integrate speech synthesis into their applications while maintaining control over data privacy and performance.
In this guide, we will walk through the process of implementing a free offline TTS API. We will cover the essential components, key features to consider, and step-by-step instructions for getting started with popular TTS engines that can be used in offline scenarios.
Choosing the Right TTS Engine for Offline Use
There are several TTS engines available that support offline functionality. Some of them are free to use and provide high-quality speech synthesis. Here are a few options:
- eSpeak: Lightweight and supports multiple languages. Ideal for smaller projects or devices with limited resources.
- Festival: Open-source and versatile, with various voice models available. It requires some setup but offers a lot of customization.
- Voxygen: A commercial option, though it provides a free version for basic usage.
Steps to Implement Offline Text-to-Speech API
- Install the TTS engine: Choose a TTS engine that suits your project needs. For example, you can install eSpeak by running the following command on Linux:
sudo apt-get install espeak
- Configure the API: Set up the TTS API to accept text input and generate speech output. You might need to configure language and voice preferences, depending on your engine.
- Generate Speech: Once set up, pass text to the API and use the appropriate commands to synthesize speech. For eSpeak, it would look like:
espeak "Hello, this is a test message."
- Handle Output: Capture the speech output, typically as an audio file (e.g., WAV or MP3), and play it in your application.
Important Considerations
Some TTS engines may have limitations in terms of voice quality, languages, or processing power. Ensure the engine you choose aligns with your project’s needs, particularly if you are targeting mobile or embedded systems.
Comparison of Popular Offline TTS Engines
Engine | License | Languages | Voice Quality |
---|---|---|---|
eSpeak | Open-source | Multiple | Basic |
Festival | Open-source | Multiple | High |
Voxygen | Commercial (free version available) | Multiple | Excellent |
How to Integrate a Free Offline Text-to-Speech API into Your App
Integrating a text-to-speech (TTS) API into your app allows you to convert text into speech without requiring an internet connection. This can be especially useful for apps that need to function in offline mode or in areas with unreliable internet access. By using a free, offline TTS solution, you can add speech synthesis capabilities to your app without incurring additional costs. In this guide, we will walk you through the steps of integrating such an API into your application.
To integrate an offline TTS API, the first step is to choose an appropriate library or API that supports offline functionality. Some libraries come bundled with voices, while others may require you to download and store additional voice files. Once you’ve selected your API, you can follow a series of steps to get it running smoothly in your app. Below, we break down the process into manageable stages.
Steps for Integration
- Step 1: Choose an API
Start by selecting a free, offline text-to-speech API. Some popular options include eSpeak, Flite, or Google TTS (for Android). These APIs typically offer support for multiple languages and voices.
- Step 2: Set Up the API in Your Project
Download the necessary files and libraries, or install the API via a package manager (like npm for Node.js or Gradle for Android). Ensure that you follow the installation instructions specific to the API you are using.
- Step 3: Initialize the TTS Engine
Once the library is installed, initialize the TTS engine in your app’s code. Most TTS libraries provide a simple API to set up and start the engine.
- Step 4: Set Language and Voice Preferences
Configure the language, pitch, speed, and voice selection. Some APIs allow you to specify these preferences programmatically.
- Step 5: Test and Debug
Run tests to ensure that the speech output works as expected. You may need to tweak the settings depending on the performance of the TTS engine.
Important Considerations
Ensure that your app has access to the required offline resources, such as voices and language packs, as some TTS APIs may require manual downloading before use.
Example Code for Integration
Here’s an example of how you might integrate a free offline TTS API using JavaScript:
const tts = new OfflineTTS(); tts.setLanguage('en'); tts.setVoice('male'); tts.speak('Hello, how can I assist you today?');
This is a basic implementation, and your app may need more advanced features, such as handling different user inputs or integrating the TTS with other app functionalities.
Conclusion
By integrating a free offline TTS API, you can enhance your app’s accessibility and provide a more interactive experience for users. With the steps outlined above, you should be able to integrate and customize the speech functionality according to your needs.
Setting Up Text-to-Speech Functionality Without an Internet Connection
Integrating text-to-speech capabilities without relying on an internet connection is an essential feature for many applications, especially in environments where online connectivity is limited or unreliable. This approach ensures that the TTS functionality works smoothly and efficiently, even in offline scenarios. Offline solutions not only improve performance but also offer more control over voice quality and customizations.
To set up offline text-to-speech functionality, you need a software package or an API that supports offline usage. Many popular TTS engines can operate fully offline once installed, and the setup process typically involves downloading necessary voice data and configuring the software for local usage.
Steps for Setting Up Offline Text-to-Speech
- Choose an Offline TTS Engine: Select a text-to-speech engine that supports offline functionality, such as eSpeak, Festival, or the offline mode of Google Cloud TTS (via specific SDKs).
- Download Required Voice Files: Most offline engines require you to download voice data. Ensure that you download the necessary language and voice options before starting.
- Install the TTS Software: Follow the installation instructions for your chosen TTS engine. Ensure that all dependencies, such as speech synthesis libraries, are installed correctly.
- Configure the TTS Settings: Adjust settings like voice selection, speed, and pitch according to your needs. This can usually be done in the configuration files or through the engine's API.
- Test the System: Run some basic text-to-speech operations to confirm that everything works correctly in offline mode.
Popular Offline TTS Solutions
Engine | Supported Platforms | Features |
---|---|---|
eSpeak | Windows, Linux, macOS | Small footprint, supports multiple languages, customizable voice |
Festival | Windows, Linux | High-quality voices, open-source, supports different languages |
Microsoft Speech Platform | Windows | Supports high-quality voices, easy to integrate with C# |
Ensure to check if the TTS engine offers complete offline capabilities, as some solutions may still require occasional internet access for updates or additional features.
Optimizing Offline Speech Synthesis for Low-Latency Performance
For offline speech synthesis applications, achieving low-latency performance is crucial for real-time interaction. This can be especially challenging when dealing with complex models or large vocabularies. Optimizing for fast response times involves minimizing the time it takes for the system to generate audio from input text, which requires an efficient combination of software algorithms and hardware capabilities. By reducing the processing load and optimizing model parameters, the system can provide smoother and more immediate voice output without delay.
To achieve optimal performance, various techniques are employed in the design and deployment of offline speech synthesis systems. The key focus areas include optimizing the model architecture, reducing the computational load, and fine-tuning data preprocessing. Below are some of the most effective approaches that can help in minimizing latency.
Key Approaches to Reduce Latency
- Model Optimization: Streamlining the architecture of the speech synthesis model helps in reducing computational overhead. Techniques such as pruning unnecessary layers or reducing the model’s depth can significantly improve processing times without compromising voice quality.
- Hardware Acceleration: Leveraging hardware accelerators, such as GPUs or specialized DSPs, can speed up the synthesis process. Offloading computations to dedicated hardware helps in reducing CPU load, leading to lower response times.
- Efficient Data Preprocessing: Minimizing the time required to convert raw text into a usable format is another key optimization strategy. Preprocessing algorithms should be designed to handle large text inputs swiftly, with minimal need for additional steps.
Key Techniques to Enhance Latency
- Model Quantization: By reducing the precision of the model’s weights, quantization allows the synthesis system to run faster with minimal loss in audio quality.
- Speaker Adaptation: Fine-tuning the voice model to specific accents, languages, or tones can reduce the need for complex speech synthesis processes, thus improving response time.
- Caching Intermediate Results: Precomputing and storing commonly used data or frequently synthesized phrases can significantly reduce the time taken to generate the speech output for recurring inputs.
Note: Reducing model size and complexity without sacrificing output quality requires a careful balance. Excessive simplification may result in poor audio fidelity, while overly complex models can lead to performance bottlenecks.
Performance Comparison
Optimization Technique | Effect on Latency | Effect on Audio Quality |
---|---|---|
Model Pruning | Reduces latency significantly | Minimal degradation in quality |
Hardware Acceleration (GPU/DSP) | Improves speed with minimal latency | No impact on audio quality |
Data Preprocessing Optimization | Speeds up processing time | Improves overall synthesis quality |
Choosing the Right Voice Options in a Free Offline Text-to-Speech API
When using a free offline text-to-speech (TTS) API, selecting the appropriate voice settings is crucial for obtaining a natural and clear output. Many TTS systems offer a variety of voice options, each tailored for different use cases. Understanding how to customize these settings can help you achieve better results, especially when you are working with limited resources in an offline environment.
One of the key factors to consider when choosing voice options is the balance between voice quality, language support, and processing speed. Free offline TTS APIs often have limitations in terms of voice diversity and capabilities. Therefore, careful selection is necessary to ensure that the generated speech meets your needs while still being efficient.
Key Voice Settings to Consider
- Voice Type: Choose between male, female, or neutral voices based on your application's tone and context.
- Language Support: Verify that the API supports the desired languages and accents for proper pronunciation.
- Pitch and Speed: Many APIs allow adjusting the pitch and speed of speech to make it more conversational or formal.
- Audio Quality: Some TTS systems provide different audio formats and quality settings that can affect both the clarity and file size.
Things to Keep in Mind
- Compatibility: Ensure the chosen voice settings are compatible with the offline environment, as some advanced features may require online connectivity.
- Resource Constraints: Free APIs often come with resource limits, which may impact voice quality or the number of characters you can process at once.
- Customization: Look for APIs that allow fine-tuning of voice attributes, so you can achieve the most natural-sounding speech.
Comparing Voice Options
Voice Type | Language Support | Adjustable Settings |
---|---|---|
Male | English, Spanish, French | Pitch, Speed |
Female | English, German, Italian | Pitch, Speed, Volume |
Neutral | English | Speed |
Important: Some free offline TTS APIs limit the range of customizable voice attributes, so it’s essential to test different settings to determine what works best for your project.
Managing Speech Output Quality with a Free Offline API
When working with offline text-to-speech solutions, ensuring high-quality output becomes a priority, especially when utilizing free APIs. While these tools may lack some of the advanced features of premium services, there are several strategies to optimize performance and achieve clear, natural-sounding speech. Understanding the available options and their limitations is key to leveraging the full potential of an offline text-to-speech API.
Many free offline APIs focus on a basic set of functionalities, but their speech output quality can be managed through various techniques. Adjusting parameters, choosing the right voices, and optimizing text input are essential steps in improving the clarity and naturalness of the generated speech.
Key Considerations for Improving Output Quality
- Voice Selection: Choosing the right voice plays a critical role in output quality. Look for APIs that offer a variety of voice options to suit different applications, from formal to conversational tones.
- Text Preprocessing: Clean and well-formatted text leads to more accurate speech. Use punctuation, proper capitalization, and avoid excessive abbreviations to ensure better pronunciation.
- Volume and Speed Control: Adjust the speaking rate and volume to match the intended user experience. Too fast or too slow can make the speech difficult to understand.
- Audio Output Settings: Ensure the audio format and quality settings are suitable for your application, especially in environments with limited bandwidth or storage.
Important Techniques to Enhance Quality
- Phonetic Adjustments: For some APIs, manipulating the phonetic spelling of words can improve pronunciation, especially for technical or uncommon terms.
- Custom Tuning: If the API allows, fine-tune the speech synthesis parameters for optimal performance.
- Noise Filtering: Use noise reduction algorithms in conjunction with your API to eliminate background distortion in the speech output.
Optimizing text-to-speech quality in offline solutions requires careful management of input text, voice selection, and output configurations to ensure the speech sounds clear and natural, even when working with free APIs.
Comparing Offline API Speech Quality
API | Voice Options | Text Processing | Audio Quality |
---|---|---|---|
API A | 2 | Basic | Standard |
API B | 5 | Advanced | High |
API C | 3 | Moderate | Good |
Managing Multilingual Support in Offline Text-to-Speech Systems
When implementing offline text-to-speech systems that support multiple languages, several technical considerations must be addressed to ensure optimal performance. Unlike cloud-based services, offline systems rely on locally stored resources, making it critical to optimize language-specific components, such as voice models and phonetic rules, to handle diverse linguistic nuances effectively. Ensuring smooth switching between languages without compromising quality is a significant challenge, requiring tailored solutions for each language.
In addition to technical optimization, offline text-to-speech systems must deal with complexities like regional accents, varying speech patterns, and different character sets. To handle these issues, developers need to build a flexible infrastructure that can easily accommodate new languages and adapt to unique phonetic structures. Below are essential strategies for achieving multilingual support in offline environments:
Key Strategies for Multilingual Offline Systems
- Phoneme Mapping: Use a universal phonetic alphabet that can map sounds across languages to improve pronunciation consistency.
- Separate Language Models: For each supported language, create dedicated voice models to ensure high-quality speech synthesis.
- Language Detection: Implement automatic language detection to allow seamless transitions between different languages during speech generation.
- Localized Databases: Store unique linguistic data for each language to minimize system errors related to grammar, tone, and stress patterns.
Steps for Implementing Multilingual Support
- Identify Required Languages: Determine which languages are essential for the application and ensure their inclusion in the offline system.
- Create Language-Specific Voice Models: Develop and train voice models that are optimized for each language's phonetics and accent.
- Implement Language Switching Mechanism: Develop an efficient mechanism for switching languages dynamically based on input.
- Test and Optimize: Continuously test the system with real-world multilingual data to identify and resolve issues related to pronunciation and fluidity.
For example, when dealing with languages such as Chinese and English, different prosodic structures require separate models for tone-based and stress-based languages.
Language-Specific Considerations
Language | Challenges | Solution |
---|---|---|
English | Varying accents and phonetic complexity | Create models for different accents (e.g., British, American) |
Chinese | Tone-based language requiring accurate tone recognition | Implement tone-specific voice models |
Arabic | Complex script and pronunciation variations | Utilize native phonetic rules for accurate pronunciation |
Reducing Resource Consumption While Using Offline Speech Synthesis
Offline speech synthesis systems are essential for various applications, especially where internet connectivity is limited or unavailable. However, these systems often require substantial computational resources, which can affect device performance. Optimizing resource consumption in offline text-to-speech (TTS) solutions is crucial to maintain efficiency and ensure smooth operation on devices with limited processing power or battery life.
Several strategies can help minimize the resource usage of offline TTS systems without sacrificing output quality. By focusing on more efficient algorithms, leveraging hardware acceleration, and reducing unnecessary computations, developers can create more efficient TTS applications.
Key Approaches to Optimize Offline Speech Synthesis
- Algorithm Optimization: Using optimized algorithms for speech generation can significantly reduce the processing time and computational load. Techniques such as model pruning and quantization help decrease the size of speech models, making them faster to run on low-resource devices.
- Speech Model Compression: Reducing the size of speech synthesis models through compression techniques helps minimize memory and storage usage while maintaining natural-sounding output.
- Hardware Acceleration: Leveraging specialized hardware, such as GPUs or neural network accelerators, can offload complex speech synthesis tasks and boost performance without overloading the main CPU.
Effective Resource Management in TTS Applications
- Dynamic Load Balancing: Monitor and adjust resource usage based on available hardware capabilities to ensure efficient processing during high-demand tasks.
- Selective Model Activation: Load and activate only the necessary components of the speech synthesis model based on user requirements, such as voice characteristics and language.
- Power Management: Incorporating energy-saving modes can extend battery life on mobile devices, making TTS more sustainable in mobile applications.
Comparison of Resource Use in Different TTS Models
Model | Memory Usage | Processing Speed | CPU Load |
---|---|---|---|
Basic Model | Low | Fast | Low |
Advanced Model | High | Moderate | High |
Optimized Model | Medium | Fast | Medium |
Efficient management of system resources allows for smoother operation, especially when working with devices that have limited processing power, such as embedded systems or smartphones.