Text to Speech Api Chrome
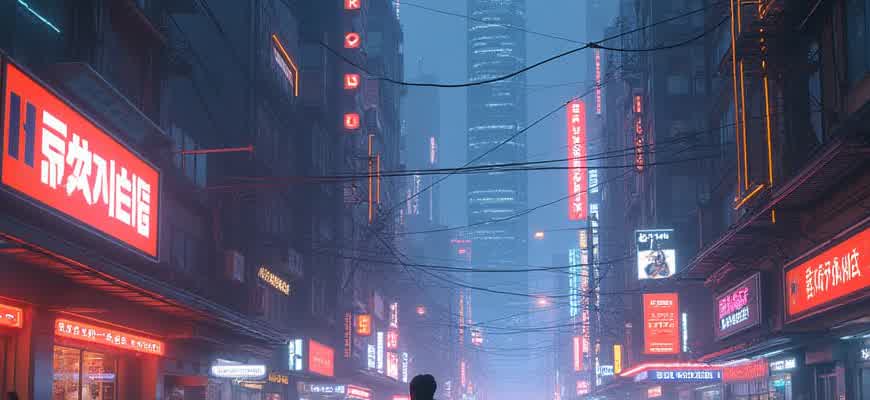
With the advancement of web technologies, integrating Text-to-Speech capabilities directly into web applications has become more seamless. Chrome offers various APIs that allow developers to convert written text into spoken words, enhancing user accessibility and interaction. The SpeechSynthesis API is one of the most commonly used solutions for this purpose.
To start using the API, follow these steps:
- Ensure that the browser supports the SpeechSynthesis API.
- Initialize the SpeechSynthesis object to access available voices.
- Call the speechSynthesis.speak() method to begin speech generation.
Note: Browser support for speech synthesis varies, so always check compatibility before deploying.
Below is a simple example of how the API can be implemented:
Step | Action |
---|---|
1 | Access the SpeechSynthesis object using window.speechSynthesis . |
2 | Select a voice from the available list using speechSynthesis.getVoices() . |
3 | Call speechSynthesis.speak() to convert text into speech. |
Text-to-Speech API in Chrome: A Comprehensive Guide
The Text-to-Speech (TTS) functionality in Chrome is a powerful tool for converting written text into audible speech. This feature is widely used in various applications, including accessibility tools, language learning apps, and content consumption. Developers can integrate TTS capabilities into their websites and apps, providing users with a richer, more interactive experience. The API works seamlessly across devices, including desktops and mobile platforms, ensuring broad compatibility and ease of use.
In this guide, we will explore how to effectively utilize the Text-to-Speech API in Chrome, covering its key features, supported languages, and integration steps. Additionally, we will provide insights into the practical aspects of implementing this API in web applications, including code samples and recommendations for smooth user experiences.
Key Features and Capabilities
- Speech Synthesis: Converts text into spoken words using a variety of voices and languages.
- Voice Selection: Offers multiple voice options, including different accents and genders.
- Rate and Pitch Control: Allows developers to adjust the speed and tone of the speech output.
- Real-time Feedback: Provides immediate vocal output for text changes or user input.
Steps for Implementing Text-to-Speech API
- Access the SpeechSynthesis API: Start by ensuring the browser supports the SpeechSynthesis interface.
- Select Voice: Choose a voice from the available options using the speechSynthesis.getVoices() method.
- Configure Settings: Set parameters such as rate and pitch using the SpeechSynthesisUtterance object.
- Trigger Speech: Use speechSynthesis.speak() to convert text into speech.
Supported Languages and Voices
Language | Voices Available |
---|---|
English | Male, Female, Different Accents |
Spanish | Male, Female |
French | Male, Female |
German | Male, Female |
Tip: Always test TTS functionality across different browsers to ensure compatibility and optimal voice performance.
How to Integrate a Speech Synthesis Service into Your Chrome Extension
Integrating a speech synthesis feature into a Chrome extension can significantly enhance user experience, providing accessibility and interactivity through voice. Using the Text to Speech API (TTS) allows developers to easily convert written text into spoken words. This guide will walk you through the steps necessary to add speech capabilities to your Chrome extension using a simple API integration.
To begin, you will need to access the SpeechSynthesis API, which is built into modern browsers like Chrome. The API allows you to convert text to voice, adjust parameters like pitch and rate, and choose from various available voices. By following the steps below, you can add this functionality to your extension and provide seamless voice support for your users.
Steps to Implement Text-to-Speech API
- Set Up Your Chrome Extension
Create a basic Chrome extension structure with a manifest file and relevant HTML/JS files. Ensure that your extension has the necessary permissions to access the SpeechSynthesis API.
- Access the API
The SpeechSynthesis API can be accessed directly via JavaScript. You will need to call the `speechSynthesis.speak()` method with a SpeechSynthesisUtterance object to initiate speech conversion.
- Configure Speech Parameters
Customize the speech properties like voice, pitch, rate, and volume according to your requirements. Here's an example:
var utterance = new SpeechSynthesisUtterance('Hello, welcome to my Chrome extension!'); utterance.pitch = 1; utterance.rate = 1; speechSynthesis.speak(utterance);
Remember, you can access the list of available voices through the `speechSynthesis.getVoices()` method. These voices may vary depending on the system and browser used.
Example Code to Trigger Speech
JavaScript Code | Description |
---|---|
var msg = new SpeechSynthesisUtterance('This is a test message.'); msg.voice = speechSynthesis.getVoices()[0]; speechSynthesis.speak(msg); |
Initializes speech synthesis with a chosen voice and speaks the message. |
msg.rate = 0.8; speechSynthesis.speak(msg); |
Adjusts the rate of speech to make it slower. |
Setting Up Voice Customization for Text to Speech on Chrome
When integrating a text-to-speech feature in Chrome, customizing the voice settings can significantly enhance the user experience. Chrome provides built-in settings for adjusting voice characteristics such as pitch, rate, and volume. Understanding these settings and how to modify them is key to tailoring the output to suit different needs, whether for accessibility or personalized interactions.
To set up voice customization in Chrome, you’ll first need to access the browser’s speech synthesis API and then configure its parameters. These customizations are available through various tools and extensions, but the core setup remains accessible within Chrome’s default settings.
Configuring Voice Parameters in Chrome
Chrome allows you to modify the following key voice attributes:
- Pitch: Adjusts the highness or lowness of the voice.
- Rate: Controls the speed of speech, which can be useful for faster or slower reading.
- Volume: Lets you control the loudness of the voice output.
These settings can be adjusted directly in the browser's settings or through JavaScript when developing web applications.
Steps for Customizing Voices
- Open Chrome and type "chrome://settings" in the address bar.
- Navigate to the "Accessibility" section.
- Click on "Manage Accessibility Features" and find the "Text-to-Speech" section.
- Choose your preferred voice from the available options.
- Adjust the pitch, rate, and volume sliders according to your preference.
Note: The default voice settings might vary based on the system's language and region. If you require a different voice, additional voice packs or extensions might be needed.
Voice Options and Their Impact
Depending on your chosen voice engine, Chrome may offer multiple voice options. These voices can range from robotic to more human-like and can impact the clarity and comfort of the speech output.
Voice Option | Characteristics |
---|---|
Standard Voice | Clear but mechanical tone suitable for general tasks. |
Natural Voice | Smoother, more human-like tone, often preferred for longer listening sessions. |
Custom Voices | Allows importing voices for a specific brand or language dialect. |
By experimenting with these settings, you can achieve a more personalized and effective text-to-speech experience, improving the accessibility and usability of your Chrome environment.
Managing Multi-Language Functionality with the Text-to-Speech API
When developing applications that utilize text-to-speech functionality, supporting multiple languages is crucial for reaching a wider audience. Many text-to-speech APIs provide functionality for switching between languages seamlessly, but handling various languages requires attention to detail to ensure the correct pronunciation and accentuation. In this guide, we’ll explore strategies for managing multi-language support with the Text-to-Speech API.
The key to effective multi-language support lies in correctly setting language parameters and choosing the appropriate voice models. Most modern APIs offer built-in language options and voices tailored to specific regions. To avoid errors, it is essential to check whether the selected language is supported by the API and ensure the proper voice is being used for each language.
Handling Language Selection
To properly switch between languages, ensure that the following steps are taken:
- Check for language availability: Some text-to-speech services may not support all languages or may offer limited voice options per language.
- Select the right voice model: Many APIs provide different voice options for each language (e.g., male, female, regional accents).
- Adjust speed and pitch settings: Different languages may require specific adjustments to speech speed or pitch to sound natural.
Best Practices for Multi-Language Support
Always test the speech output in multiple languages to ensure that the pronunciation, intonation, and pace align with native speakers' expectations.
Here are some best practices to manage multi-language support effectively:
- Ensure dynamic language switching: Implement features that can detect or allow users to choose a language dynamically.
- Cache language models: To improve performance, consider caching language models locally based on user preferences.
- Handle fallback scenarios: If a specific language is not supported, provide a clear fallback mechanism (e.g., using a neutral accent or default voice).
Supported Languages Overview
Language | Voice Options | Available Accents |
---|---|---|
English | Male, Female | American, British, Australian |
Spanish | Male, Female | Mexican, European |
French | Male, Female | French (Standard), Canadian |
German | Male, Female | Standard, Swiss |
Troubleshooting Common Issues When Using Text to Speech in Chrome
While Chrome's built-in Text to Speech functionality offers convenience, users may encounter various issues during its use. These issues can range from poor voice quality to compatibility problems with certain websites or extensions. Understanding and addressing these challenges can significantly improve the experience and effectiveness of speech synthesis in your browser.
Below are some common problems you might face and their possible solutions:
Common Issues and Fixes
- Inconsistent Voice Output: Sometimes, the text might not be read aloud correctly or may stop suddenly.
- Low-Quality Voices: The voice output may sound robotic or unnatural.
- Permissions Issues: Extensions or apps may not work due to missing permissions.
How to Fix Text to Speech Problems in Chrome
- Check Extension Permissions: Ensure that the necessary permissions are granted for the extension to use Text to Speech functionality.
- Update Chrome: Always ensure you're using the latest version of Chrome, as updates often fix bugs related to APIs.
- Clear Cache: Sometimes, clearing your browser cache can help resolve issues with extensions or apps not functioning properly.
- Adjust Voice Settings: Go to Chrome’s accessibility settings and fine-tune the voice settings such as pitch, speed, and language.
- Test with Different Voices: Some voices may be of higher quality. Test with various voices available in your settings.
Important: If none of these steps resolve the issue, you may need to reinstall your Text to Speech extension or reset Chrome’s settings to default.
Additional Tips
Issue | Solution |
---|---|
Audio not playing | Check your system’s audio settings and ensure no conflicting audio processes are running. |
Unresponsive Text to Speech | Test the feature with different websites to ensure it’s not a website-specific issue. |
Optimizing Speech Synthesis for Large Texts
When dealing with large text outputs, it becomes essential to ensure the performance and efficiency of speech synthesis systems. Slow or inefficient synthesis can result in noticeable delays, affecting user experience. Effective optimization of text-to-speech (TTS) APIs ensures that large texts are processed quickly while maintaining high-quality audio output.
Several factors can influence the performance of TTS engines, including text segmentation, pre-processing steps, and resource management. Below, we explore key strategies to enhance TTS performance for extensive text data.
Key Optimization Techniques
- Text Segmentation: Divide large blocks of text into smaller, more manageable segments. This reduces the likelihood of performance bottlenecks and allows the system to process data more efficiently.
- Pre-processing Text: Clean and format text before passing it to the TTS engine. Removing unnecessary punctuation, simplifying complex sentences, and correcting errors can significantly reduce processing time.
- Speech Caching: Cache commonly used phrases or sentences to avoid reprocessing them for every speech request, improving speed and resource usage.
Resource Allocation and Load Balancing
- Dynamic Resource Allocation: Adjust system resources based on the current workload. Allocate more resources during peak usage times and scale back during idle periods to ensure optimal processing times.
- Load Balancing: Distribute large text inputs across multiple servers or processes. This ensures that no single resource is overburdened, resulting in smoother performance.
Performance Comparison
Strategy | Impact on Performance |
---|---|
Text Segmentation | Reduces processing time by splitting the text into smaller chunks. |
Pre-processing | Improves text clarity, reducing errors and reprocessing times. |
Speech Caching | Increases efficiency by reusing cached phrases or sentences. |
Note: Optimization strategies may vary based on the specific TTS engine and API used. Always test different configurations to determine the best performance for your use case.
Enhancing User Experience with Voice Speed and Pitch Adjustments
Adjusting the voice speed and pitch can significantly improve the user experience when using text-to-speech technology. These features enable users to customize the voice output, ensuring it aligns with personal preferences or specific use cases. By controlling the speed and pitch of the voice, users can make the content more engaging, easier to follow, or more pleasant to listen to for extended periods.
Incorporating these adjustments into applications, such as Chrome extensions or web-based services, allows for a more tailored and intuitive interaction. Whether it is for accessibility, language learning, or entertainment, the ability to fine-tune voice characteristics can make a world of difference in how the text is perceived.
Key Benefits of Customizing Speed and Pitch
- Improved Accessibility: Users with different cognitive abilities or hearing impairments can benefit from slower speeds or higher pitch adjustments to aid comprehension.
- Enhanced Engagement: Adjusting pitch to match the tone of the content can make the experience more dynamic and captivating, especially in storytelling or educational apps.
- Language Learning: Slower speech speeds can help non-native speakers improve pronunciation and listening skills.
Adjusting Voice Speed and Pitch in Practice
To demonstrate the practical benefits of speed and pitch adjustments, the following table outlines common use cases and recommended settings:
Use Case | Recommended Speed | Recommended Pitch |
---|---|---|
Accessibility for cognitive impairments | Slow (0.7x) | Medium |
Language Learning | Slow (0.8x) | Medium-High |
Entertainment (storytelling) | Normal (1.0x) | High |
Tip: Experimenting with these settings can help users find the optimal combination for their specific needs, leading to a more enjoyable and effective experience.
Conclusion
Voice speed and pitch adjustments are simple yet powerful tools to enhance the interaction with text-to-speech technologies. By fine-tuning these settings, users can tailor the experience to meet their needs, whether for accessibility, learning, or enjoyment.
How to Ensure Accessibility with Text to Speech for Diverse Audiences
Ensuring that text-to-speech (TTS) technology caters to a wide range of users is essential for improving digital accessibility. From individuals with visual impairments to those with learning disabilities, TTS can significantly enhance user experience when properly implemented. To achieve this, it is crucial to understand the unique needs of various audience segments and design solutions that meet these needs effectively.
Text-to-speech services should be tailored to different languages, voices, and customization options to improve accessibility. Additionally, understanding the context in which the tool will be used helps in designing user interfaces that facilitate ease of access and interaction.
Key Features for Enhancing Accessibility
- Voice Customization: Allow users to choose from a variety of voices, accents, and speaking speeds. This flexibility ensures that the tool meets the preferences of a diverse user base.
- Language Support: Ensure the TTS tool supports multiple languages, including dialects, to accommodate users from different regions.
- Text Highlighting: Implement text highlighting as the speech progresses, allowing users to follow along more easily.
- Intuitive Controls: Provide simple, intuitive controls to pause, resume, and adjust speech settings for ease of use.
Best Practices for Implementation
- Clear and Consistent User Interface: A consistent UI makes it easier for users to navigate and use the TTS feature effectively.
- Compatibility with Screen Readers: Ensure the TTS tool is fully compatible with screen readers for users who rely on them for navigation.
- Alternative Formats: Provide alternative formats for audio content, such as downloadable files, to accommodate different user needs.
- Real-time Feedback: Offer immediate feedback to users regarding speech output to ensure clarity and improve interaction.
Key Considerations for Diverse User Groups
Audience | Needs | Recommended Features |
---|---|---|
People with Visual Impairments | Clear and consistent speech, adjustable speech speed | Voice customization, integration with screen readers |
Non-Native Language Speakers | Accurate pronunciation and language support | Multi-language support, adjustable pronunciation settings |
People with Dyslexia or Learning Disabilities | Text-to-speech with highlighted text | Text highlighting, simple and easy-to-use interface |
Important: Always test your TTS solutions with real users to ensure they meet the diverse needs of your audience. Continuous feedback and iteration are key to maintaining accessibility standards.
Best Practices for Secure and Scalable Integration of Text to Speech in Chrome
Integrating a text-to-speech solution into Chrome requires careful consideration of security and scalability. A reliable API can offer a seamless experience for users, but it’s essential to follow best practices to ensure smooth functionality without compromising security. These guidelines will help you design a robust system that accommodates future growth while safeguarding user data.
For developers integrating text-to-speech technology, maintaining a balance between performance, security, and ease of scaling is critical. It is also important to optimize the user experience while keeping the implementation flexible and maintainable. Below are some key strategies for ensuring a secure and scalable integration.
Security Considerations
- Use HTTPS: Always ensure communication with the API is encrypted using HTTPS to prevent man-in-the-middle attacks.
- Limit API Access: Restrict access to your text-to-speech API by implementing authentication mechanisms, such as OAuth tokens or API keys.
- Rate Limiting: Set up rate limiting to prevent abuse and protect from DoS (Denial of Service) attacks.
- Data Privacy: Ensure that all audio data is handled securely. Consider anonymizing any sensitive information used in the synthesis process.
Scalability and Performance
- Load Balancing: Use load balancing to distribute requests across multiple servers, ensuring a seamless experience during peak usage times.
- Cloud Services: Consider cloud-based solutions for scaling purposes, as they provide flexible and efficient resources that grow with demand.
- Caching: Cache audio responses where possible to reduce API calls and minimize latency, improving overall response time.
- Optimize Request Size: Keep requests as small as possible to reduce overhead and improve processing speed.
"Security and scalability are not just technical needs but also foundational principles that ensure user trust and optimal system performance over time."
Example API Usage
Feature | Description |
---|---|
Audio Quality | Ensure the API provides high-quality audio with clear pronunciation. |
Voice Customization | Support different voices, speeds, and languages to enhance user experience. |
Latency | Minimize latency to ensure quick text-to-speech conversion. |