Speech Synthesis Python
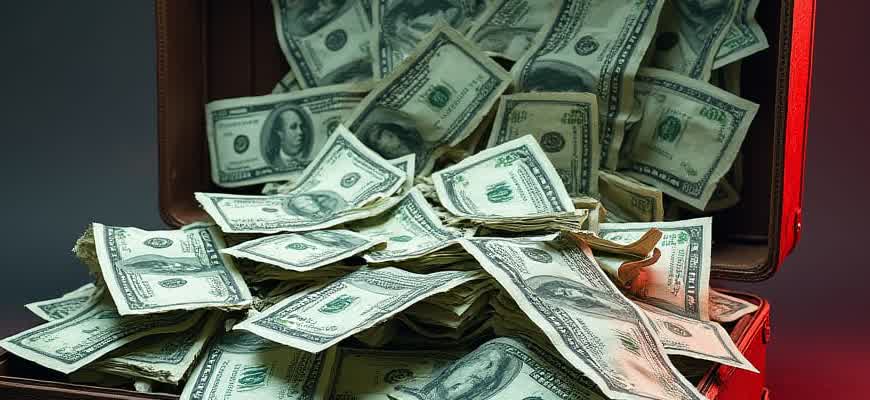
Speech synthesis in Python enables the conversion of text into human-like speech. This is a powerful tool used in various applications such as virtual assistants, accessibility features, and interactive devices. By leveraging Python libraries, developers can create dynamic, customized speech outputs tailored to specific use cases.
There are several libraries available for text-to-speech (TTS) conversion. The most popular ones include:
- pyttsx3 - A simple and offline library for TTS.
- gTTS (Google Text-to-Speech) - An online service that uses Google’s TTS engine.
- SpeechRecognition - Primarily focused on speech recognition, but can also be used for synthesis with other libraries.
Each library comes with its own features and limitations, but all of them provide easy-to-implement interfaces for generating speech.
Important Note: pyttsx3 works offline, while gTTS requires an internet connection for speech synthesis.
Below is a comparison of the key features of the most commonly used TTS libraries:
Library | Offline Capability | Voice Customization | Supported Languages |
---|---|---|---|
pyttsx3 | Yes | Limited | Multiple |
gTTS | No | High | Multiple |
SpeechRecognition | Yes (via external services) | Limited | Multiple |
Configuring Your Speech Synthesis Setup in Python
To start working with speech synthesis in Python, you'll need to install the necessary libraries and ensure your environment is ready to generate speech. Python offers various libraries for text-to-speech (TTS), with some of the most popular being gTTS (Google Text-to-Speech) and pyttsx3. The setup process is relatively straightforward, and most libraries are easy to integrate into Python projects.
Once you've decided which library suits your needs, the next step is to install it using Python's package manager. Below is a guide to set up the environment and begin using speech synthesis effectively.
Installation Steps
- gTTS Installation: This library uses Google Text-to-Speech API to convert text to speech.
- Open your terminal or command prompt.
- Run the following command to install gTTS:
pip install gTTS
- pyttsx3 Installation: This is a cross-platform TTS engine that works offline.
- Run the following command in your terminal:
pip install pyttsx3
Basic Usage
Once you've installed the necessary libraries, you can easily start generating speech from text. Here's a brief guide:
Note: Make sure your microphone and speaker setup are functioning properly before running TTS scripts to ensure the sound is output correctly.
Library | Example Code |
---|---|
gTTS | from gtts import gTTS tts = gTTS('Hello, how are you?') tts.save('output.mp3') |
pyttsx3 | import pyttsx3 engine = pyttsx3.init() engine.say('Hello, how are you?') engine.runAndWait() |
Choosing the Best Python Libraries for Text-to-Speech
When implementing text-to-speech functionality in Python, the choice of library plays a crucial role in determining the quality and performance of the generated speech. Several Python libraries provide various features, ranging from easy-to-use APIs to advanced customization options. Below, we explore some of the most popular libraries and highlight their advantages for different use cases.
Some libraries are well-suited for quick integration, while others allow for deep customization of speech synthesis parameters. When selecting the right library, factors such as naturalness of voice, language support, system compatibility, and ease of integration should all be considered.
Top Python Libraries for Speech Synthesis
- gTTS (Google Text-to-Speech) – A simple and easy-to-use library that relies on Google's Text-to-Speech engine. It supports multiple languages and offers a quick solution for generating speech.
- pyttsx3 – A popular offline library that supports both Windows and Linux. It allows for better control over speech parameters such as rate, volume, and voice.
- SpeechRecognition – While primarily known for speech-to-text conversion, it can also be used in conjunction with other libraries for text-to-speech functionalities.
- ResponsiveVoice – A robust library with a variety of voice options, great for web applications and interactive projects.
Factors to Consider When Choosing a Library
- Voice Quality: Some libraries, such as pyttsx3, offer a variety of voices, but others, like gTTS, rely on cloud services, which may affect voice quality and latency.
- Language Support: Ensure the library supports the desired languages and accents. For example, gTTS has extensive language support, while pyttsx3 might have limitations depending on the system configuration.
- Offline vs. Online: Some libraries, like pyttsx3, work offline, while others, such as gTTS, require an internet connection. Consider the use case and whether offline functionality is necessary.
Library Comparison Table
Library | Voice Options | Offline Support | Languages Supported | Ease of Use |
---|---|---|---|---|
gTTS | Limited (Cloud-based) | No | Extensive | Easy |
pyttsx3 | Varies by system | Yes | Limited | Moderate |
SpeechRecognition | Varies | No | Moderate | Easy |
ResponsiveVoice | Multiple options | No | Moderate | Easy |
Important: The best library for your project depends on whether you need offline functionality, the quality of the voice synthesis, and the specific languages you need to support. Testing several libraries before finalizing a choice is recommended.
Customizing Voices for Different Use Cases
When working with speech synthesis in Python, selecting or creating custom voices is a powerful way to tailor the audio output to the specific requirements of your project. Whether you are developing an application for accessibility, a virtual assistant, or an automated customer service bot, customizing the voice is essential to improve the user experience. There are a variety of tools and libraries available that enable voice customization for different needs.
The customization process involves adjusting several parameters, such as tone, speed, pitch, and accent. By fine-tuning these variables, you can create a voice that fits the context of the application, ensuring clarity, engagement, and appropriateness for the target audience. Some platforms even offer the option to upload and use custom voice models, providing even more flexibility in how the voice sounds.
Key Customization Parameters
- Voice Type: Choose between male, female, or gender-neutral voices depending on the target audience.
- Pitch and Speed: Control the pitch to create a more dynamic or calmer sound. Adjusting speed helps to align with the pacing of the application.
- Language and Accent: Customize the voice to reflect a specific language or regional accent, which is useful for localization.
Popular Libraries for Voice Customization
- pyttsx3: A cross-platform library that allows for easy voice adjustments, including speed, volume, and language settings.
- gTTS: This library focuses on simplicity and ease of use but offers fewer customization options compared to pyttsx3.
- Google Cloud Text-to-Speech API: Provides advanced customization features, including neural network-based voices and extensive language support.
Example of Voice Parameters
Parameter | Effect |
---|---|
Volume | Adjusts the loudness of the voice output. |
Rate | Modifies the speed of speech. A higher rate increases speech speed. |
Pitch | Alters the tone to make the voice sound higher or lower. |
Customizing voices not only improves the naturalness of speech synthesis but also enhances user interaction by aligning the voice to specific contexts, such as formal presentations, casual conversations, or technical instructions.
Integrating Speech Synthesis with Other Python Tools
Integrating speech synthesis functionality with various Python libraries allows developers to create sophisticated applications that can interact with users through both voice input and output. By combining speech synthesis with tools such as natural language processing (NLP) or machine learning frameworks, applications can gain a more human-like understanding and response system. For instance, integrating speech synthesis with a chatbot can enhance the user experience by providing audible replies, rather than just text-based ones.
Python offers a number of libraries that enable seamless integration of speech synthesis, such as pyttsx3, gTTS, and SpeechRecognition. These libraries can be linked with other Python tools to create dynamic systems that perform a variety of tasks, from basic voice-based interaction to more complex real-time translation and automated content generation. By using APIs like Google Speech API or Microsoft’s Azure Speech API, it’s possible to scale the functionality of speech synthesis in both local and cloud-based applications.
Key Libraries for Integration
- pyttsx3: A popular offline library for speech synthesis with support for multiple voices and languages.
- gTTS (Google Text-to-Speech): A cloud-based service that converts text to speech using Google's text-to-speech API.
- SpeechRecognition: Primarily used for speech-to-text conversion, which can be combined with speech synthesis to create interactive applications.
Possible Integrations
- Speech-Driven Assistants: Use NLP libraries like spaCy or NLTK along with speech synthesis tools to create voice-activated assistants that provide audible responses.
- Real-Time Translation: Integrating speech synthesis with translation APIs like Google Translate can allow applications to speak translated text back to users in different languages.
- Interactive Storytelling: Combine pyttsx3 or gTTS with Python's gaming libraries like pygame to create immersive voice-driven games or story-driven experiences.
Example Workflow: Speech and Text Interaction
Step | Action |
---|---|
1 | Capture user speech input using SpeechRecognition library. |
2 | Process the input text through NLP for sentiment analysis using spaCy. |
3 | Generate a response and convert it into speech using pyttsx3 or gTTS. |
4 | Deliver the speech output to the user, creating a dynamic, interactive experience. |
Tip: When integrating speech synthesis into your application, ensure that the response time is optimized. Delays in speech output can negatively impact the user experience, especially in real-time systems.
Improving Pronunciation Accuracy in Generated Speech
Ensuring high-quality pronunciation in speech synthesis systems is a crucial aspect of natural-sounding output. Pronunciation accuracy affects not only the clarity of speech but also its overall intelligibility. As synthetic speech technology evolves, developers are working on various techniques to enhance how generated voices handle phonetic and prosodic features, making them sound more human-like and precise in articulation.
One of the main challenges is the accurate representation of word stresses, phoneme transitions, and dialectal variations. Traditional models often fail to replicate nuanced differences in pronunciation, leading to awkward-sounding speech. To address this issue, researchers have developed several strategies and tools for refining pronunciation in speech synthesis systems.
Approaches to Improving Pronunciation
- Phonetic Transcriptions: Accurate phonetic transcriptions of input text can improve pronunciation by guiding the synthesizer on how each word should be enunciated.
- Contextual Modeling: Modeling context (such as surrounding words and sentence structure) helps adjust pronunciations for different situations (e.g., sentence intonation and emphasis).
- Voice Training: Using real-world data to train voice models on diverse accents and pronunciations helps reduce the gap between synthetic and human speech patterns.
Techniques for Better Phoneme Accuracy
- Data Augmentation: Expanding the dataset with variations of speech patterns, including non-standard pronunciations, can help refine phoneme production.
- Advanced Deep Learning: Implementing neural networks that are trained on large amounts of phonetic data can significantly improve pronunciation fidelity.
- Pronunciation Lexicons: Custom lexicons, built specifically for target languages or dialects, can resolve issues related to rare or complex word pronunciations.
Key Considerations in Pronunciation Improvement
Technique | Advantage | Challenge |
---|---|---|
Phonetic Transcriptions | Enhances word-level pronunciation accuracy | Requires precise phonetic knowledge |
Contextual Modeling | Improves sentence-level prosody | Increased complexity of model training |
Data Augmentation | Boosts pronunciation robustness | Needs large, diverse datasets |
Improving pronunciation in speech synthesis not only enhances user experience but also opens up possibilities for more accessible and diverse voice applications across languages and cultures.
Managing Speech Speed and Pitch for Better User Interaction
Adjusting speech characteristics, such as speed and pitch, plays a crucial role in enhancing user engagement with text-to-speech systems. These features influence how easily users can comprehend the spoken content and how naturally the voice sounds. By controlling the rate of speech and the tonal variations, it is possible to create a more dynamic and human-like interaction, ultimately improving user satisfaction.
Incorporating variable speech speed and pitch settings is essential for tailoring interactions to different contexts, user preferences, and environments. For instance, faster speech might be useful for conveying information quickly, while a slower pace can improve clarity for complex instructions. Similarly, adjusting the pitch can make the voice sound more friendly or authoritative depending on the need.
Controlling Speech Speed
Managing the speed of speech allows for better comprehension and user experience. A higher speed might be appropriate for users familiar with the content, whereas a slower rate is beneficial for new learners or when delivering complex information. Most text-to-speech systems provide APIs to modify this aspect.
- Use faster speech for brief, simple information.
- Opt for slower speech for instructional or complex content.
- Consider user preferences and adjust according to their needs.
Adjusting Speech Pitch
The pitch of the speech impacts its emotional tone and can make the interaction feel more personable or mechanical. Lower pitches often come across as more serious or authoritative, while higher pitches may appear friendlier or more engaging. Balancing pitch can help maintain a pleasant user experience over extended interactions.
- Low pitch: Conveys authority and seriousness.
- High pitch: More engaging and friendly, ideal for casual interactions.
- Adjust pitch to avoid monotony in longer conversations.
Key Considerations for Fine-Tuning
The following table summarizes recommended settings for speech synthesis based on specific use cases:
Use Case | Speech Speed | Pitch |
---|---|---|
News Readings | Normal | Medium |
Instructions | Slow | Low |
Casual Conversations | Normal | High |
For optimal interaction, always consider the target audience's needs when adjusting speech characteristics. Users with varying cognitive abilities or those in noisy environments may require different settings for better comprehension.
Managing Multiple Languages and Accents in Speech Synthesis
In the context of speech synthesis, handling multiple languages and accents is a complex challenge. Synthesizing natural-sounding speech in various languages requires the system to process phonetic variations, grammar structures, and specific speech characteristics unique to each language. This includes correctly pronouncing words, adapting intonation, and responding to regional variations. Accents, in particular, can significantly affect how synthesized speech is perceived by listeners, making accent recognition and modification crucial in building realistic speech synthesis systems.
To create a system capable of fluent speech synthesis in different languages, it is essential to consider several factors, such as language models, voice databases, and accent variations. Leveraging these components allows the creation of diverse speech outputs that are culturally and linguistically accurate. Below are some key techniques and strategies used in handling these challenges effectively.
Key Considerations for Handling Multiple Languages and Accents
- Language-specific phonetic models: Every language has unique phonetic rules, and speech synthesis models must account for these differences to ensure accuracy in pronunciation.
- Accurate accent representation: Accents within the same language can vary significantly, requiring the system to differentiate between regional variations.
- Customizable voice databases: Developing diverse voice datasets for each language and accent enhances the flexibility of the synthesis system.
Challenges in Multi-language Speech Synthesis
- Phoneme overlap: Certain phonemes in different languages may sound similar but have different meanings or uses, leading to confusion or incorrect synthesis.
- Intonation differences: Intonation patterns differ across languages, affecting the emotional tone and naturalness of synthesized speech.
- Computational complexity: Supporting multiple languages and accents requires extensive processing power to manage large datasets and complex models.
Note: Handling multiple languages and accents in speech synthesis often requires integrating multiple models, including those trained specifically for each language or accent. This enables a more accurate representation of linguistic diversity.
Table: Examples of Key Accent Variations
Language | Accent | Key Feature |
---|---|---|
English | American | Rhotic sounds; emphasis on certain vowels |
English | British | Non-rhotic sounds; distinct vowel shifts |
Spanish | Mexican | Clear, distinct vowels; rolling "r" |
Spanish | Castilian | Th-sound for "z" and soft "c" |
Testing and Debugging Your Speech Synthesis Implementation
Once you have implemented your speech synthesis system, it's crucial to thoroughly test and debug it to ensure the desired performance and output. The process involves verifying the quality of speech output, identifying any errors, and fine-tuning parameters to achieve optimal results. In this phase, you'll focus on detecting issues such as mispronunciations, inconsistencies in speed or pitch, and poor audio quality.
Testing a speech synthesis system typically requires a range of test cases that simulate real-world usage scenarios. Effective debugging methods will allow you to isolate and fix problems in your code or configuration, ultimately improving the overall user experience. Below are the essential steps for debugging and testing speech synthesis systems.
Key Testing and Debugging Strategies
- Test Case Variety: Ensure your system can handle various inputs, including different languages, accents, and speaking styles.
- Audio Output Quality: Listen for any unnatural pauses, distorted sounds, or robotic speech.
- Performance Testing: Evaluate the responsiveness of the system under different conditions, such as varying network speeds or resource limitations.
- Boundary Testing: Input edge cases like very short, long, or complex sentences to verify that the system performs well in all scenarios.
Common Debugging Tools
- Speech Analysis Tools: Use software that can break down speech into phonemes or visualize prosody to identify errors.
- Logs and Tracing: Add logging to capture important information about system performance and trace errors.
- Automated Tests: Develop unit tests to automatically check the functionality of the speech synthesis system during updates or improvements.
Remember to adjust parameters such as speed, pitch, and volume as needed during debugging. Small changes can often lead to significant improvements in speech quality.
Example Test Cases
Test Case | Expected Outcome | Result |
---|---|---|
Short sentence | Clear, natural speech | Pass |
Complex sentence with multiple clauses | Proper intonation and pauses | Fail (adjust pauses) |
Non-English sentence | Correct pronunciation for the language | Pass |