Microsoft Text to Speech Api Example
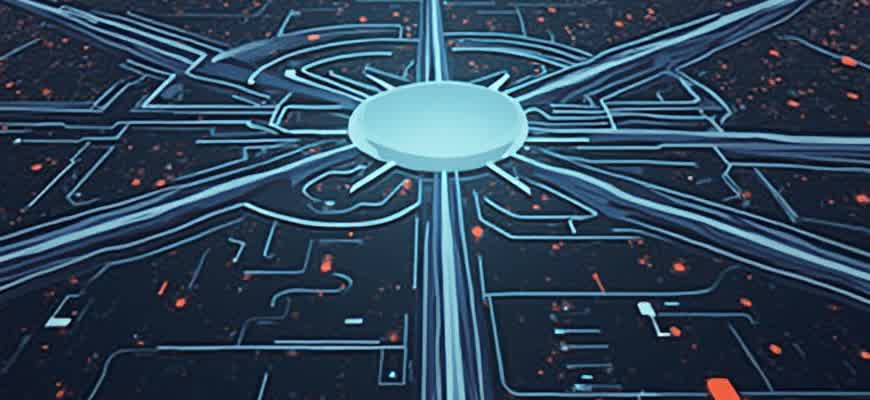
The Microsoft Text-to-Speech API offers a powerful tool for converting written text into natural-sounding speech. It leverages advanced machine learning models to generate high-quality audio from text input. Below is a step-by-step guide on how to use the API for your own applications.
Steps to Use Microsoft Text-to-Speech API:
- Sign up for an Azure account and create a Speech resource.
- Get your API key and endpoint URL from the Azure portal.
- Choose a programming language or framework to interact with the API (e.g., Python, Node.js, C#).
- Install the required SDK or library for the chosen programming language.
- Set up your request by including the necessary headers and body parameters.
- Send a POST request to the Text-to-Speech endpoint with your text input.
Important Information:
Remember that you must authenticate your requests using the API key and include the appropriate headers, such as 'Content-Type' and 'Authorization'.
The API response includes an audio stream in a variety of formats, such as MP3 or WAV. You can process this audio data or save it to a file for further use.
Example Request Parameters:
Parameter | Description |
---|---|
Text | The input text you wish to convert to speech. |
VoiceName | The voice model to be used for generating speech. |
AudioFormat | The desired audio format for the output, such as MP3 or WAV. |
Microsoft Text-to-Speech API Example
Microsoft's Text-to-Speech API allows developers to convert written text into spoken words using neural voice models. This service provides a way to integrate speech synthesis into applications, websites, or services, enabling a more interactive and accessible user experience. The API supports multiple languages and offers customizable voices, allowing businesses to tailor the speech output to their specific needs.
To use the Text-to-Speech API, developers need to follow a simple process that includes setting up an Azure account, acquiring an API key, and making REST API calls. The service can be integrated with various platforms, from web applications to mobile apps, and can be used for tasks such as voice assistants, accessibility features, and automated customer service systems.
Getting Started
- Sign up for an Azure account and create a Speech service resource.
- Obtain your API key and endpoint URL from the Azure portal.
- Make an HTTP POST request to the API with the desired text and parameters for voice selection.
Basic API Request Example
Below is an example of how to structure a request to the Microsoft Text-to-Speech API:
The API request includes the following parameters:
- Text: The string of text to be converted into speech.
- Voice Name: The name of the voice to use for speech synthesis.
- Language: The language of the input text and the desired voice.
Example Request and Response
Request | Response |
---|---|
POST https:// |
HTTP/1.1 200 OK Content-Type: audio/wav |
Setting Up Microsoft Text to Speech API on Your Server
Integrating Microsoft’s Text to Speech API into your server environment involves several important steps. First, you need to set up an Azure account and subscribe to the appropriate services. Once your account is active, you’ll acquire the API keys necessary for authenticating and making API requests. This process can be straightforward, but it’s crucial to follow each step carefully to ensure a smooth integration.
After obtaining your API keys, you can proceed to configure your server environment. The setup process varies slightly depending on whether you are using Windows or Linux, but the core steps remain the same. Below, we’ll walk through a generic method for setting up the API and making your first speech synthesis request.
Steps to Set Up the API
- Sign in to your Azure portal and navigate to the Speech service.
- Create a new Speech resource and select the region where your service will be hosted.
- Obtain the API keys and the region endpoint from the Azure portal.
- Install the SDKs or libraries relevant to your server’s programming language (e.g., Python, C#, Node.js).
- Configure your server with the API keys and region endpoint to authenticate requests.
- Test your connection by sending a basic speech synthesis request.
Server Configuration Details
To start using the Text to Speech API, you’ll need to install the appropriate SDKs. Below is an example of how to install the Python SDK:
pip install azure-cognitiveservices-speech
Once installed, you can proceed with the following code snippet to authenticate and synthesize speech:
import azure.cognitiveservices.speech as speechsdk
speech_config = speechsdk.SpeechConfig(subscription="Your_API_Key", region="Your_Region")
speech_synthesizer = speechsdk.SpeechSynthesizer(speech_config=speech_config)
speech_synthesizer.speak_text_async("Hello, world!")
Important Notes
Make sure to keep your API keys secure and never expose them in public code repositories.
API Response Example
The API will respond with a status code indicating success or failure. Below is an example of a successful response:
Field | Description |
---|---|
status | Success |
audio | Audio file containing the synthesized speech |
Once you’ve completed these steps, your server will be ready to use the Microsoft Text to Speech API for voice synthesis in your applications.
Step-by-Step Guide to Authenticating Microsoft Text to Speech API
Before you can start utilizing the Microsoft Text to Speech API, you need to authenticate your application. Authentication ensures that only authorized users can access the service and helps track usage. In this guide, you will learn how to authenticate your API requests using Microsoft Azure's authentication mechanisms.
Microsoft provides a seamless authentication process via Azure, where you create an Azure account, register your application, and obtain necessary credentials. Follow the steps below to set up authentication for your Text to Speech API requests.
1. Create and Set Up Azure Account
The first step is to create an Azure account if you don't already have one. After creating the account, you will need to access the Azure portal to register your app and generate authentication credentials.
- Go to Azure Portal and log in with your Microsoft account.
- Navigate to Azure Active Directory and click on App Registrations.
- Click on New Registration, provide your application details, and click Register.
- Once registered, you will be given an Application (Client) ID and a Directory (Tenant) ID.
2. Generate API Key for Authentication
To securely authenticate your requests, you need to generate an API key. This key will be used to identify your application when making requests to the Text to Speech API.
- In the Azure portal, navigate to Azure Cognitive Services and click on Text to Speech resource.
- Under the Keys and Endpoint section, you will find two keys. You can use either key for API authentication.
- Copy one of the keys and securely store it. This will be used as the subscription key when making API requests.
3. Set Up Authentication in Your Application
Now that you have the necessary credentials, you need to include them in your API request headers to authenticate. Follow these steps to include the authentication details in your API calls:
Important: Ensure you never expose your API key in public repositories or on client-side applications to avoid unauthorized usage.
// Example in C#: HttpClient client = new HttpClient(); client.DefaultRequestHeaders.Add("Ocp-Apim-Subscription-Key", "your_subscription_key"); client.DefaultRequestHeaders.Add("Content-Type", "application/json");
Once this setup is complete, your application will be ready to interact with the Text to Speech API securely.
Choosing the Ideal Voice and Language for Your Speech Application
When integrating text-to-speech (TTS) capabilities into an application, selecting the correct voice and language is essential to ensure a natural user experience. Microsoft’s Text to Speech API provides a variety of voices and languages, making it crucial to understand the needs of your target audience and application context. The right choice of voice can enhance the accessibility and effectiveness of your application, whether it’s for customer support, educational tools, or any other type of interactive interface.
The first step in selecting the appropriate voice is identifying the language requirements of your application. This should be based on your users’ primary language or regional dialect. Next, you should consider whether a male or female voice would be more appropriate for your use case. Additionally, certain voices may have different tones, accents, and levels of clarity that can impact the overall experience.
Key Factors to Consider
- Language Support: Choose a language that corresponds to your user base’s region. Microsoft’s API supports a wide range of global languages.
- Voice Gender: Decide whether a male or female voice best suits the context of your app.
- Accent and Tone: Opt for a voice with the accent or tone that matches your target audience’s expectations and preferences.
- Speech Style: Some voices have specific styles, such as formal, conversational, or emotional tones.
Practical Tips
- Test various voices and languages to ensure they sound natural and clear in your specific application context.
- Consider the regional variations of the language to ensure correct pronunciation and accent.
- Review any available documentation or voice samples provided by Microsoft to better understand the characteristics of each voice.
Important: Ensure that the voice selected fits well with the user experience you want to create. A poorly chosen voice can affect user engagement and lead to a less effective application.
Voice Comparison Table
Voice | Language | Gender | Accent |
---|---|---|---|
Aria | English | Female | Neutral American |
Benjamin | English | Male | Neutral American |
Elena | Spanish | Female | Castilian Spanish |
Adjusting Speech Parameters for Better User Experience
When using text-to-speech (TTS) technology, the ability to customize voice settings can significantly enhance the user experience. By modifying various speech parameters, developers can create more natural, engaging, and clear speech outputs tailored to specific use cases. The Microsoft Text to Speech API provides a range of options to adjust voice pitch, speed, and volume, ensuring optimal user satisfaction. These adjustments are particularly valuable when integrating TTS into applications that require nuanced vocal outputs, such as virtual assistants, accessibility tools, and interactive systems.
Effective manipulation of speech parameters allows developers to strike a balance between clarity and expressiveness. For instance, adjusting the pitch and rate can make the speech sound more dynamic and less robotic. This is crucial when building applications aimed at engaging users for extended periods. Understanding the best practices for modifying these settings ensures that the TTS voice fits the context and meets the specific needs of the audience.
Key Parameters for Adjustment
- Pitch: The perceived highness or lowness of the voice. Adjusting the pitch can make the voice sound more engaging or calming depending on the context.
- Rate: The speed at which the speech is delivered. A faster rate can make the speech feel more energetic, while a slower rate may improve comprehension.
- Volume: The loudness of the voice. This can be adjusted to ensure the speech is neither too loud nor too soft for the user's environment.
- Voice Selection: The choice of voice can drastically impact user experience. Different voices have varying tones and personalities, so selecting the appropriate voice is key for creating the desired effect.
Best Practices for Fine-Tuning TTS
- Maintain Clarity: Ensure that speech rate and pitch are set to levels that allow users to easily understand the content.
- Context Awareness: Adapt speech settings based on the context of the application. For example, a navigation app might benefit from a slightly faster rate, while an audiobook app could use a slower pace.
- User Customization: Allow users to modify speech parameters based on personal preferences, enhancing accessibility and user control.
Fine-tuning speech parameters not only improves accessibility but also creates a more engaging and personalized experience for users, making them feel more connected to the application.
Example: Voice Adjustment Parameters in Microsoft API
Parameter | Value Range | Impact on User Experience |
---|---|---|
Pitch | -100 to 100 | Higher pitch can make speech more lively, while lower pitch adds a more serious tone. |
Rate | 0 to 1.0 (Normal speed) | Faster rates can make speech sound urgent, while slower rates improve comprehension. |
Volume | 0 to 100 | Adjusting volume ensures optimal audibility in different environments. |
Voice | Multiple options (e.g., en-US-GuyNeural) | Different voices help set the tone and personality of the application. |
Integrating Microsoft Text to Speech API with Popular Frameworks
The Microsoft Text to Speech API provides developers with a powerful tool to convert written text into lifelike speech, making it an essential feature for a variety of applications. With its support for multiple languages and customizable voices, it can be integrated with a wide range of frameworks to enhance user interaction. Below, we explore how developers can integrate this API into popular frameworks, such as Node.js, Python, and .NET, to create seamless speech synthesis functionality in their projects.
Integration with these frameworks generally follows the same basic steps: obtaining API credentials, installing the necessary SDKs or libraries, and configuring the API to process text input. Each framework offers specific tools and modules to streamline the process. Below is a brief guide to integrating the Microsoft Text to Speech API with some of the most widely-used development environments.
Integration with Node.js
To integrate the Microsoft Text to Speech API with a Node.js application, follow these key steps:
- Install required dependencies: Install the
axios
orrequest
package to handle API requests. - Set up authentication: Use your Azure subscription key to authenticate requests to the API.
- Make API calls: Send HTTP requests to the Text to Speech endpoint with the desired text and voice parameters.
- Handle the audio response: Store or stream the audio data returned from the API.
Important: Make sure to securely store your API key and avoid exposing it in public repositories or client-side code.
Integration with Python
Python developers can utilize the requests
library to communicate with the API. The general process involves:
- Install the required libraries: Install the
requests
andpydub
libraries to handle HTTP requests and audio processing, respectively. - Authenticate: Provide the API key in the headers of the request.
- Make a request: Use POST requests to send text and configure voice parameters such as pitch and speed.
- Handle the response: Store the audio data or play it directly using Python libraries like
pygame
.
Integration with .NET
For .NET developers, integrating the Microsoft Text to Speech API is straightforward, thanks to the Azure SDK. Follow these steps:
- Install the Azure Cognitive Services SDK: Use NuGet to install the
Microsoft.CognitiveServices.Speech
SDK. - Set up credentials: Initialize the Speech Config object with your API key and region.
- Generate speech: Use the
SpeechSynthesizer
class to generate speech from text and configure additional parameters such as language and voice type.
Comparison Table
Framework | Required Libraries | Authentication Method |
---|---|---|
Node.js | axios or request | API Key in request headers |
Python | requests, pydub | API Key in request headers |
.NET | Microsoft.CognitiveServices.Speech SDK | API Key in SpeechConfig |
By integrating the Microsoft Text to Speech API with these popular frameworks, developers can enhance their applications with voice synthesis capabilities that improve accessibility and user experience.
Real-time Audio Streaming: Leveraging Microsoft Text-to-Speech API
Microsoft's Text-to-Speech API enables real-time voice generation from text, a key feature for applications requiring audio feedback on the fly. This capability allows developers to integrate speech synthesis directly into their platforms, ensuring seamless communication with users in dynamic environments. By utilizing this API, applications can provide instantaneous audio output from text, supporting a wide range of use cases such as virtual assistants, accessibility tools, and educational software.
Real-time audio streaming involves transforming input text into audio almost instantly, providing a fluid user experience. With the Microsoft Text-to-Speech API, developers can stream the generated audio data without delay, enhancing the interactivity and responsiveness of applications. This section outlines the critical steps to effectively utilize the API for real-time audio streaming.
Key Steps to Stream Audio in Real-Time
- Authentication: Obtain an API key from the Azure portal to authenticate your application with the Text-to-Speech service.
- Audio Streaming Setup: Initialize the API client and configure the stream to handle audio data efficiently.
- Stream Configuration: Set parameters such as voice selection, language, and speaking rate to match your application's needs.
- Audio Output Handling: Process the audio data as it streams to play it in real-time on the client device.
Important Considerations for Real-Time Audio Streaming
Latency: Minimize any delays in speech synthesis to ensure smooth audio playback during interactions.
Bandwidth: High-quality audio streaming may require sufficient network bandwidth to prevent interruptions or degraded sound quality.
Audio Format Support
The API supports various audio formats for streaming. Below is a table showcasing the available formats and their respective properties:
Format | Sample Rate | Bitrate |
---|---|---|
MP3 | 22 kHz | 64 kbps |
WAV | 44 kHz | 1411 kbps |
OGG | 22 kHz | 96 kbps |
Benefits of Real-Time Streaming
- Enhanced user experience with immediate, context-aware responses.
- Supports dynamic content updates, allowing for real-time feedback and adjustments.
- Improves accessibility by enabling audio interaction for users with visual impairments.
Debugging Common Issues with Microsoft Text to Speech API
When integrating Microsoft's Text to Speech API into your application, several challenges may arise that hinder performance or prevent it from functioning correctly. Understanding common issues and how to resolve them will streamline the development process and ensure that your application works as expected. Below are some frequent problems developers encounter and solutions to address them effectively.
From authentication errors to voice configuration problems, there are many potential pitfalls. By paying attention to the API documentation and ensuring proper setup, you can avoid or quickly resolve these challenges. Let's dive into the most common issues and how to troubleshoot them.
Authentication Failures
One of the most common issues when using the Text to Speech API is authentication failure, usually due to incorrect API keys or expired credentials. Make sure your keys are valid and properly configured.
- Double-check the subscription key in your application code.
- Ensure that the API key is associated with the correct Azure subscription.
- Verify that the resource has been deployed in the correct region.
Note: If the subscription key is invalid or has expired, the API will return an "Unauthorized" error. Renew your credentials via the Azure portal.
Voice Configuration Issues
Another issue developers may face is related to voice configuration, such as incorrect language or voice selection. The API provides a range of voices in different languages, but an invalid configuration can lead to errors in the generated speech.
- Ensure the selected voice is supported in your region.
- Check that the language setting matches the voice capabilities.
- Verify the gender and voice type are valid options in the API request.
Language | Supported Voices |
---|---|
en-US | Aria, Guy, Jessa, etc. |
en-GB | Hazel, George, etc. |
Tip: Always refer to the official documentation for an up-to-date list of available voices for each region.
Optimizing API Requests for Speed and Cost-Effectiveness
When integrating a Text to Speech API, it’s crucial to optimize the requests to ensure they are both fast and cost-efficient. Poor optimization can lead to unnecessary delays and increased expenses, especially when working with high volumes of data. By applying certain strategies, developers can reduce the load on servers, minimize costs, and improve the overall user experience.
One effective way to optimize requests is by adjusting the frequency and size of the text being processed. Smaller, more concise requests are often more efficient, leading to quicker responses and lower costs. Additionally, leveraging features like caching or batching requests can reduce the overall number of API calls.
Best Practices for Optimizing API Usage
- Use batch processing: Group multiple requests into one to minimize the overhead of individual API calls.
- Adjust text length: Process smaller chunks of text instead of sending long passages, as shorter texts are faster to process and cheaper to process.
- Cache frequently used data: Store results of common queries locally to avoid redundant requests.
- Choose the right voice and language model: Select the most cost-effective voice that still meets your quality requirements.
Cost-Efficiency Considerations
Reducing the number of API calls and optimizing the data being sent can significantly reduce the overall cost of using the service.
- Use the right pricing tier based on expected usage to avoid unnecessary charges.
- Monitor usage patterns and adjust the frequency of API calls during off-peak hours to minimize costs.
- Take advantage of free quotas and lower-cost options if available for testing or lower-volume use.
Example of API Request Optimization
Action | Impact on Speed | Impact on Cost |
---|---|---|
Batch multiple requests | Increases speed by reducing individual request overhead | Reduces cost by processing more data in fewer requests |
Reduce text length | Increases processing speed for shorter texts | Lower cost due to less resource consumption per request |
Cache responses | Reduces response time for repeated queries | Reduces cost by avoiding redundant API calls |