How to Use Google Text to Speech Api in Python
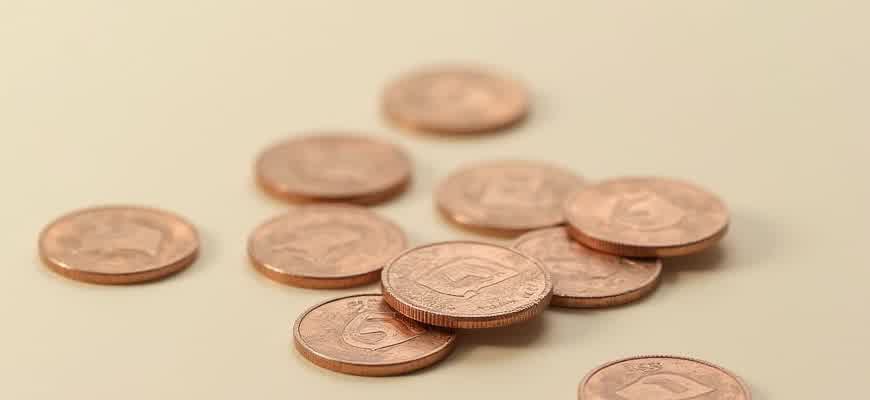
Google's Text-to-Speech API provides developers with a powerful tool for converting text into natural-sounding speech. To start using this service in Python, you'll first need to install the required library and set up an API key. Below is a step-by-step guide for integrating the API into your Python applications.
Prerequisites:
- Google Cloud account
- Enable Text-to-Speech API on Google Cloud Console
- Python 3.x installed
- Access to API key or service account credentials
Follow these steps to get started:
- Install the necessary Google Cloud client library:
pip install --upgrade google-cloud-texttospeech
Once the library is installed, you can start using the API to generate speech from text.
Example Code:
import os from google.cloud import texttospeech # Initialize client client = texttospeech.TextToSpeechClient() # Set up the text input synthesis_input = texttospeech.SynthesisInput(text="Hello, welcome to the Google Text-to-Speech API.") # Configure voice parameters voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) # Set audio configuration audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) # Request speech synthesis response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) # Save the audio file with open("output.mp3", "wb") as out: out.write(response.audio_content)
This simple example generates an MP3 file from a given text input. You can modify the parameters to adjust voice type, language, and output format.
Using Google Text to Speech API in Python
The Google Text-to-Speech (TTS) API allows you to convert written text into natural-sounding speech using deep learning models. It is widely used for accessibility applications, virtual assistants, and voice-enabled interfaces. In this guide, we will walk through the steps to integrate Google TTS in a Python project using the Google Cloud client library.
To get started with the Google TTS API, you'll first need to set up a Google Cloud account and enable the Text-to-Speech API. This process involves creating a project, configuring billing, and obtaining authentication credentials. Once you've completed these prerequisites, you can begin using the API within your Python code to generate speech from text.
Steps to Use Google TTS API
- Install the required libraries.
- Set up authentication using a service account key.
- Write a Python script to generate speech.
To install the necessary libraries, use the following command:
pip install google-cloud-texttospeech
Note: Make sure you have set up authentication correctly by exporting the path to your service account key with the environment variable:
export GOOGLE_APPLICATION_CREDENTIALS="path_to_your_service_account_file.json"
Code Example
Here's a simple example of using the API to convert text to speech in Python:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, this is a test.") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech( request={"input": synthesis_input, "voice": voice, "audio_config": audio_config} ) with open("output.mp3", "wb") as out: out.write(response.audio_content)
This code converts the text "Hello, this is a test." into an MP3 audio file. You can customize the language, gender, and speech properties according to your needs.
Key API Parameters
Parameter | Description |
---|---|
SynthesisInput | Defines the input text that will be converted into speech. |
VoiceSelectionParams | Specifies the language code and voice parameters such as gender. |
AudioConfig | Specifies the audio format and encoding of the output. |
Tip: Experiment with different parameters like speaking_rate or pitch for more natural-sounding voices.
Configuring Google Cloud Account and API Credentials for Text-to-Speech
To integrate Google's Text-to-Speech service in your Python application, you must first set up a Google Cloud account and generate the necessary API credentials. The process involves enabling the Cloud Text-to-Speech API and creating a service account with the appropriate permissions. Once set up, you can access the API through authentication using a unique key, which will allow your application to make requests to Google's cloud services securely.
Below is a step-by-step guide to get started with Google Cloud and obtain your API credentials for Text-to-Speech services. Following these instructions will ensure you have everything set up for a seamless connection between your Python code and Google's cloud platform.
Steps to Set Up Google Cloud Account and API Keys
- Go to the Google Cloud Console and sign in with your Google account.
- Create a new project by selecting "Create Project" from the dashboard.
- Once your project is created, navigate to the API & Services section and select Library.
- Search for the Text-to-Speech API and click on the "Enable" button.
- Next, go to APIs & Services → Credentials, and click Create Credentials.
- Choose the Service account option and follow the prompts to create your service account.
- After the service account is created, download the JSON file containing the API key.
Important: Keep the downloaded JSON file safe, as it contains your API key. You’ll use this file for authentication in your Python code.
Configuring the API Key in Your Python Project
To authenticate your Python application using the downloaded JSON file, set the environment variable to point to the key file. This can be done with the following command:
export GOOGLE_APPLICATION_CREDENTIALS="[PATH_TO_YOUR_JSON_FILE]"
For Windows, use this command instead:
set GOOGLE_APPLICATION_CREDENTIALS=[PATH_TO_YOUR_JSON_FILE]
This step ensures that your Python application can authenticate with Google Cloud's Text-to-Speech API using the provided credentials.
Verification and Testing
Once you’ve set up your account and API key, it's time to verify the setup by sending a test request to the API. Ensure that your environment is configured properly, and you can start utilizing the API with your Python code.
Installing the Required Python Libraries for Text-to-Speech
Before you can start converting text to speech using Google’s API in Python, it’s essential to install the necessary libraries. The primary tool for interacting with Google’s Text-to-Speech service is the `google-cloud-texttospeech` library. Additionally, you may need to install some supplementary libraries to manage authentication and handle any additional tasks in your project.
To set up your environment, you'll need to install a few packages via pip, Python's package manager. Below are the key steps to get started:
1. Install Google Cloud Text-to-Speech
- Ensure that you have pip installed on your machine.
- Install the `google-cloud-texttospeech` library by running the following command:
pip install google-cloud-texttospeech
Google Cloud's client libraries also require authentication setup. You must create a service account on the Google Cloud Console and download the corresponding JSON key file. Once you have the file, set the environment variable GOOGLE_APPLICATION_CREDENTIALS to the path of this file:
export GOOGLE_APPLICATION_CREDENTIALS="path_to_your_service_account_file.json"
Remember to keep your service account key secure and avoid sharing it publicly.
2. Optional Libraries
In some cases, you might also need to install additional libraries for advanced functionality or handling different input/output formats. Here are some optional but useful libraries:
- pydub – To work with audio files such as MP3, WAV, etc.
- google-auth – Handles authentication and token generation.
- pillow – For image manipulation if needed in the future.
3. Verifying the Installation
After installing the necessary packages, it’s a good practice to verify everything works correctly. Run a small script to check if the Google Text-to-Speech API is functional. For example:
from google.cloud import texttospeech
client = texttospeech.TextToSpeechClient()
print("Google Text-to-Speech API is ready to use!")
Library | Description |
---|---|
google-cloud-texttospeech | Main library to interact with the Text-to-Speech API |
google-auth | Handles authentication with Google Cloud services |
pydub | Optional, for working with audio file formats |
Authenticating Your Google Cloud Service with Python
Before utilizing Google Cloud services, you need to authenticate your application. Authentication allows your Python code to securely access Google Cloud APIs, including the Text to Speech API. The process involves configuring credentials and ensuring that your Python environment can interact with Google Cloud services effectively.
To begin, you will need to set up a project in Google Cloud Console and enable the required APIs. Afterward, you will create credentials in the form of a service account key that will be used in your Python code to authenticate requests.
Steps to Authenticate
- Go to the Google Cloud Console and create a new project.
- Navigate to the API & Services section, then enable the Text-to-Speech API.
- Go to the Credentials tab and create a new service account key.
- Download the generated JSON key file containing your service account credentials.
Make sure the downloaded JSON file is securely stored and not exposed in your public repositories. This file contains sensitive information required for authentication.
Setting Up Python Authentication
Once you have your service account credentials, the next step is to configure Python to use them for authentication. Follow these steps:
- Install the Google Cloud client library for Python by running the command:
pip install google-cloud
- Set the environment variable GOOGLE_APPLICATION_CREDENTIALS to the path of your JSON key file. For example, in a terminal:
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your-service-account-file.json"
Once the environment variable is set, your Python code will automatically use the provided credentials when interacting with Google Cloud APIs.
Verification
To verify that your authentication is successful, try running a simple API call in your Python script, such as requesting the available voices from the Text-to-Speech API.
If authentication fails, ensure the credentials file is correctly located and that the environment variable is set properly.
Table: Common Error Codes
Error Code | Solution |
---|---|
401 | Invalid or missing credentials. Check your service account key and environment variable. |
403 | You may not have the necessary API permissions. Ensure your service account has the correct roles. |
Choosing the Right Voice and Language Parameters for Speech Output
When using Google’s Text-to-Speech API, selecting the right voice and language settings is essential to produce natural-sounding speech. The API allows you to customize various parameters such as language, voice type, and gender, enabling you to tailor the speech output to your specific needs. Choosing the right parameters will enhance the user experience and ensure that the spoken output aligns with the context in which it is used.
Understanding the available options and their implications is key to making the right decision. Below is a breakdown of how to choose the right language and voice for your text-to-speech applications.
Language and Voice Selection
- Language: The Google API supports a wide range of languages. It’s crucial to select the language that corresponds to your text’s target audience. Different languages have unique regional variations, so ensure you choose the appropriate one to match the desired accent and dialect.
- Voice Gender: You can select either a male or female voice. The choice should reflect the tone and context of your application. For example, a professional tutorial may sound better with a neutral or authoritative male voice, while a friendly app for kids could benefit from a cheerful female voice.
- Voice Type: Google offers various voice options within each language. Some voices sound more natural and expressive, while others may have a more robotic or synthetic tone. Test different voices to find one that best fits your needs.
Parameters to Consider
- Language Code: A code that defines the language and region (e.g., “en-US” for American English).
- Voice Name: Each voice in a language has a unique name (e.g., “en-US-Wavenet-D” for a high-quality English voice).
- Pitch: Adjusting the pitch can change how high or low the voice sounds. A slight increase in pitch can make the voice sound more friendly, while lowering it can make it sound more serious.
- Speaking Rate: The rate at which the text is spoken. A higher rate can make the voice sound faster, while a lower rate will slow down the speech, which may be necessary for instructional or slow-paced content.
Important: Test different voice and language combinations before finalizing your settings to ensure the output matches the tone and clarity you desire.
Recommended Language and Voice Parameters
Language Code | Voice Type | Voice Gender |
---|---|---|
en-US | Wavenet | Male/Female |
fr-FR | Standard | Female |
de-DE | Wavenet | Male |
Converting Text to Speech: Code Walkthrough
Using Google Text-to-Speech API in Python can streamline the process of converting any text into clear, natural-sounding speech. The API supports various languages and voice options, allowing developers to integrate speech synthesis into their applications with ease. This guide will walk you through the key steps of using this API, from setup to practical implementation.
Let's start by breaking down the code. We'll first walk through the necessary installation steps and dependencies, followed by an example of how to convert a sample text into audio. By the end, you'll be able to integrate text-to-speech capabilities into your own projects effectively.
Installation and Setup
- Ensure that you have the Google Cloud SDK installed and set up on your machine.
- Create a Google Cloud project and enable the Text-to-Speech API.
- Download the service account credentials file and authenticate your environment.
- Install the required Python libraries:
google-cloud-texttospeech
.
Important: Make sure to set the GOOGLE_APPLICATION_CREDENTIALS
environment variable to the path of your service account key file.
Code Breakdown
- Import the necessary modules from the Google Cloud library.
- Set up the Text-to-Speech client.
- Define the input text and choose the voice parameters (language, gender, etc.).
- Call the
synthesize_speech()
method to convert text into audio. - Save the resulting audio to a file or play it directly using a media library.
Here’s a simple example of the code:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, welcome to our demo.") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Parameters Explained
Parameter | Description |
---|---|
synthesis_input |
The text to be converted into speech. |
voice |
Settings for language and voice characteristics (e.g., gender, pitch). |
audio_config |
Defines the audio format and other audio parameters (e.g., MP3, PCM). |
Tip: You can experiment with different voice configurations to suit your needs, such as selecting regional dialects or adjusting the pitch and rate of speech.
Handling Various Audio Formats and Storing the Results
When working with Google's Text-to-Speech API, it's essential to be familiar with different audio file formats and how to save the generated speech output. Google provides a variety of audio formats for the generated speech, such as MP3 and WAV, each with different use cases and advantages. Depending on the project requirements, the choice of format may vary, and understanding how to properly handle them is crucial for ensuring compatibility with your system or application.
In this section, we will explore how to handle the audio formats supported by the API, as well as how to save and manipulate the generated speech output in Python. This will involve setting the correct encoding parameters and saving the audio data to local files or other storage solutions. Knowing how to work with these formats effectively can significantly improve the efficiency of your project.
Supported Audio Formats
The Google Text-to-Speech API supports several audio formats that can be used based on the needs of the application. Below are the main formats and their characteristics:
- MP3: A highly compressed format that provides a good balance of quality and file size.
- LINEAR16: An uncompressed, raw audio format that provides high-quality sound at the cost of larger file sizes.
- OGG_OPUS: A compressed format that uses the Opus codec, offering high-quality audio at lower bitrates, ideal for streaming.
- FLAC: A lossless format that preserves audio quality but results in larger file sizes compared to MP3.
Saving Audio Output
Once the speech is generated, it can be saved in one of the supported audio formats. The saving process typically involves writing the audio data to a file in binary mode. Here's a step-by-step guide on how to do it:
- Set the desired audio format using the audio_config parameter when calling the API.
- Save the audio data returned by the API to a file using Python's built-in file handling methods.
- If necessary, convert or adjust the audio format to match your application's requirements.
Note: The audio format you select affects the quality and file size. Choose the format based on your needs, balancing audio fidelity with file storage considerations.
Example Code for Saving Audio Output
Here’s an example of how to save the generated speech in an MP3 format:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams(language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL) audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Audio File Format Comparison
Format | Compression | Quality | File Size |
---|---|---|---|
MP3 | Lossy | Good | Small |
LINEAR16 | None | Excellent | Large |
OGG_OPUS | Lossy | Very Good | Medium |
FLAC | Lossless | Excellent | Large |
Implementing Error Handling in Your Google Text-to-Speech Python Script
When working with the Google Text-to-Speech API in Python, handling errors is crucial for building a reliable application. If not handled correctly, errors can interrupt the functionality of the program and lead to unexpected crashes or incorrect outputs. Proper error management ensures that your application can recover gracefully from failures, provide clear feedback to the user, and maintain smooth operation.
Error handling can be achieved by using try-except blocks, logging, and catching specific exceptions that might occur during API requests or processing. By anticipating potential issues, such as network problems or invalid input, you can create a more robust script that responds effectively to different situations.
Types of Errors and How to Handle Them
The following types of errors might occur while using the Google Text-to-Speech API:
- API Connection Issues: These errors can occur if the server is down or there is a network issue.
- Invalid Input: Errors can arise if the text provided for speech generation is empty or not properly encoded.
- Authentication Errors: These happen when API keys are missing, expired, or invalid.
Handling Errors with Try-Except Blocks
Using try-except blocks allows you to catch errors and prevent them from stopping your script. For instance, handling network issues can be done by catching exceptions such as requests.exceptions.RequestException:
try: # API request code client.synthesize_speech(input_text, voice, audio_config) except requests.exceptions.RequestException as e: print(f"Network error occurred: {e}") except ValueError as e: print(f"Invalid input value: {e}") except Exception as e: print(f"An unexpected error occurred: {e}")
Logging Errors for Debugging
To make error handling even more efficient, it’s important to log errors for later debugging. By using the logging module, you can record detailed error messages and stack traces. Here's an example:
import logging logging.basicConfig(level=logging.ERROR) try: # API request code client.synthesize_speech(input_text, voice, audio_config) except Exception as e: logging.error(f"Error occurred: {e}") raise
Table: Common Error Types and Solutions
Error Type | Possible Cause | Solution |
---|---|---|
ConnectionError | Network or API server issues | Check your internet connection and try again |
ValueError | Invalid input provided | Ensure the text is not empty or malformed |
AuthenticationError | Missing or invalid API key | Verify and update your API credentials |
"By handling errors and logging exceptions, you make your script more resilient and easier to troubleshoot."