Google Speech to Text Api Python Github
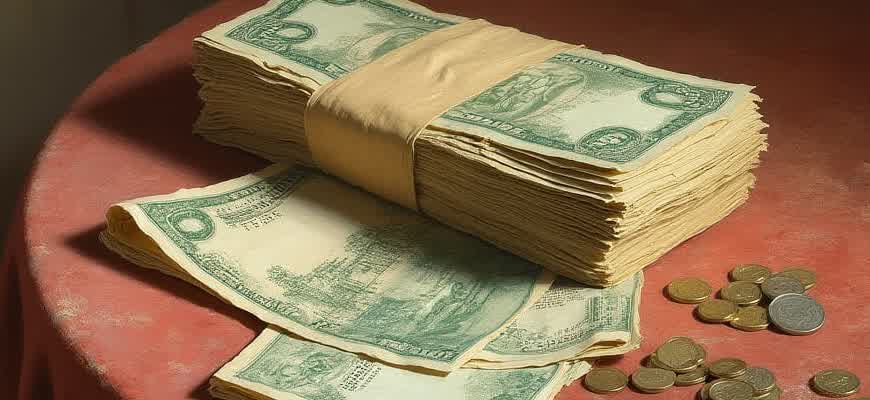
The Google Speech Recognition API provides an easy way to convert spoken language into text using Python. This tool is highly efficient and supports a wide range of languages and audio formats. It is particularly useful for applications in voice assistants, transcription services, and language processing. Below is an overview of how to set up and use this API with Python, including a simple example of implementation.
Key Features:
- Real-time speech recognition
- Support for over 125 languages
- Integration with Google Cloud services
- Easy setup and quick implementation
"This API allows developers to easily integrate speech-to-text functionality into their Python applications, enhancing user experience with voice-enabled features."
To get started, you'll need to install the necessary Python package and set up your Google Cloud account. Follow these steps:
- Create a Google Cloud project
- Enable the Speech-to-Text API
- Download and set up the credentials
- Install the Python client library:
pip install google-cloud-speech
Basic Example:
import os from google.cloud import speech # Set environment variable for authentication os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = "path_to_your_credentials.json" client = speech.SpeechClient() audio = speech.RecognitionAudio(uri="gs://your-bucket-name/audio_file.wav") config = speech.RecognitionConfig( encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code="en-US", ) response = client.recognize(config=config, audio=audio) for result in response.results: print("Transcript: {}".format(result.alternatives[0].transcript))
This code snippet demonstrates how to send an audio file to the API and receive a transcription of the spoken words. The results will display the most likely transcription based on the provided audio.
Additional Resources:
Resource | Link |
---|---|
GitHub Repository | Visit GitHub |
Google Cloud Documentation | View Docs |
Google Speech-to-Text API Python Integration Guide on GitHub
Google's Speech-to-Text API allows developers to easily convert audio recordings into text, making it a powerful tool for a wide range of applications, including transcription services and voice recognition features. The Python library provided by Google simplifies the integration of this service, allowing Python developers to quickly harness the power of Google's cloud-based speech recognition technology. In this guide, we will walk through the process of using this API with Python and explore a practical example available on GitHub.
The setup process involves several key steps such as installing necessary libraries, authenticating with Google's cloud services, and interacting with the API to send audio files for transcription. Below are the essential steps and code snippets you can follow to integrate Speech-to-Text functionality into your Python project.
Setup and Installation
To begin using Google’s Speech-to-Text API, you need to complete a few steps to get everything ready for development.
- Install the Google Cloud Speech library: Run the following command to install the Google Cloud client library for Python.
pip install --upgrade google-cloud-speech
- Create and configure a Google Cloud project: You need to set up a project in the Google Cloud Console and enable the Speech-to-Text API.
- Download service account credentials: You must authenticate your application by creating a service account key, which you can download as a JSON file.
Important: Ensure that the JSON file containing the credentials is stored in a secure location, as it allows access to the API services.
Using Speech-to-Text API in Python
Once the setup is complete, you can begin using the API to transcribe audio data. The following is a simple implementation example in Python that demonstrates how to convert an audio file into text:
from google.cloud import speech import os # Set environment variable for authentication os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = "path/to/your/service-account-file.json" client = speech.SpeechClient() # Load the audio file with open("path/to/audio-file.wav", "rb") as audio_file: content = audio_file.read() audio = speech.RecognitionAudio(content=content) config = speech.RecognitionConfig( encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code="en-US", ) # Perform speech recognition response = client.recognize(config=config, audio=audio) # Output transcriptions for result in response.results: print("Transcript: {}".format(result.alternatives[0].transcript))
Additional Resources on GitHub
For further exploration, you can access example repositories on GitHub that demonstrate different use cases of the Speech-to-Text API. Below is a brief summary of some useful GitHub repositories:
Repository | Description |
---|---|
python-speech | Official Google repository with Python client and sample code for Speech-to-Text API. |
speech-samples | Collection of example scripts for different use cases of the Speech-to-Text API. |
Tip: You can fork the repositories to experiment with the code or adapt it to your own projects.
Setting Up Google Cloud Speech-to-Text API for Python
Integrating the Google Cloud Speech-to-Text API with Python requires a few key steps to enable seamless speech recognition within your applications. This guide will help you configure the API and provide Python code examples to get you started.
Follow these instructions to set up the API and begin converting speech into text using Python. Ensure that you have a Google Cloud account and have enabled the Speech-to-Text API before proceeding.
Steps to Set Up the Google Cloud Speech-to-Text API
- Create a Google Cloud Project: Go to the Google Cloud Console and create a new project or select an existing one.
- Enable Speech-to-Text API: In the Google Cloud Console, navigate to the "API & Services" section, search for the Speech-to-Text API, and enable it for your project.
- Set Up Authentication: Create a service account key to authenticate your Python application with Google Cloud. Save the downloaded JSON key file securely.
- Install Google Cloud Client Library: Use pip to install the necessary Python libraries:
pip install --upgrade google-cloud-speech
Important: Ensure that your environment variable GOOGLE_APPLICATION_CREDENTIALS is set to the path of your service account JSON key file.
Configuring Your Python Application
- Import Libraries: Import the Google Cloud client library in your Python script.
- Authenticate with API: Set the GOOGLE_APPLICATION_CREDENTIALS environment variable in your code or system.
- Write Code for Speech Recognition: Use the following code to start recognizing speech from an audio file:
from google.cloud import speech client = speech.SpeechClient() with open("your_audio_file.wav", "rb") as audio_file: content = audio_file.read() audio = speech.RecognitionAudio(content=content) config = speech.RecognitionConfig( encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code="en-US", ) response = client.recognize(config=config, audio=audio) for result in response.results: print("Transcript: {}".format(result.alternatives[0].transcript))
This basic setup will allow you to convert speech from a .wav file into text. Adjust parameters like encoding, sample rate, and language code as needed based on your audio input.
Step-by-Step Guide to Installing Required Libraries for Python Integration
Before integrating the Google Speech-to-Text API into your Python application, it's essential to set up the necessary libraries that allow for smooth interaction between your code and the Google Cloud services. Below is a detailed process for installing these dependencies, which include the Google Cloud client library and additional packages needed for optimal functionality.
Make sure to have Python 3.6+ installed on your system. You will also need to set up a Google Cloud account and activate the Speech-to-Text API to proceed with the installation and integration steps.
1. Install Google Cloud Client Library
Start by installing the official Python library for Google Cloud. This library provides easy access to the Speech-to-Text service, simplifying the process of sending audio data to the API and receiving text responses.
- Open your terminal or command prompt.
- Run the following command to install the Google Cloud client library:
pip install --upgrade google-cloud-speech
2. Set Up Authentication
Before making requests to the Google Cloud Speech-to-Text API, you must authenticate your application. This can be done by creating a service account in your Google Cloud project and downloading the authentication key.
- Navigate to the Google Cloud Console.
- Create a new service account or use an existing one.
- Download the JSON key file for the service account.
- Set the environment variable to point to this key file:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your-service-account-file.json"
3. Optional Libraries
If you plan to work with audio files or perform additional tasks such as file conversion, consider installing the following libraries:
Library | Usage |
---|---|
pydub | For audio file conversion (e.g., from MP3 to WAV). |
google-auth | For handling Google authentication if not included in the cloud library. |
Important Notes
Ensure that the environment variable for your service account key is correctly set before running any code that communicates with Google Cloud services. This will avoid issues with authentication.
Once all dependencies are installed and authentication is configured, you're ready to begin utilizing the Google Speech-to-Text API in your Python application.
How to Authenticate and Set Up Google Speech to Text API in Python
To interact with Google Speech to Text API in Python, proper authentication and configuration are essential. This setup involves obtaining the necessary credentials and installing the required libraries. Once authenticated, you can easily make requests to the API for speech recognition tasks.
Here is a step-by-step guide to authenticate and configure the Google Speech to Text API in your Python environment.
Authentication Process
The first step is to obtain your service account key from Google Cloud Console, which will allow your application to authenticate with the API.
- Visit Google Cloud Console and sign in to your account.
- Create a new project or select an existing one.
- Navigate to the "APIs & Services" section and enable the "Speech-to-Text API".
- Go to "Credentials", then click "Create Credentials" and choose "Service Account".
- Download the generated JSON key file to your system.
Important: Store the JSON key file in a secure location. It contains sensitive information used to authenticate your app.
Setting Up the Python Environment
To interact with the API, install the Google Cloud Speech library using pip. Additionally, set the environment variable to point to your service account key.
- Install the Google Cloud Speech library with pip:
pip install --upgrade google-cloud-speech
- Set the environment variable to authenticate using your downloaded JSON key:
export GOOGLE_APPLICATION_CREDENTIALS="[PATH_TO_YOUR_SERVICE_ACCOUNT_KEY].json"
Tip: Replace "[PATH_TO_YOUR_SERVICE_ACCOUNT_KEY]" with the actual file path to your JSON key.
Configuration in Python
Once authentication is complete, you can configure your Python script to use the Speech API for transcription tasks.
Step | Action |
---|---|
1 | Import the necessary libraries in Python. |
2 | Instantiate the Speech client using the Google Cloud library. |
3 | Pass audio files for transcription to the API's recognize method. |
How to Implement Google Speech Recognition for Real-Time Audio Transcription
Google's Speech-to-Text API provides a powerful tool for converting audio into written text in real-time. By integrating this API, developers can build applications capable of processing spoken language, transcribing it instantly as it is spoken. This guide walks through how to set up and use the API for continuous transcription in Python.
To get started, the first step is to install the required libraries and authenticate with Google's API. Once set up, the system can capture audio input and send it for transcription, allowing for seamless integration into various real-time applications.
Steps to Use Google Speech-to-Text API for Real-Time Transcription
- Set up Google Cloud Platform:
- Sign up for a Google Cloud account and enable the Speech-to-Text API.
- Create a new project and generate an API key for authentication.
- Install Dependencies:
- Install the Google Cloud Speech package via pip:
pip install google-cloud-speech
- Install PyAudio for capturing audio from the microphone:
pip install pyaudio
- Install the Google Cloud Speech package via pip:
- Authenticate with the API:
- Download the service account key and set the environment variable:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/key.json"
- Download the service account key and set the environment variable:
- Implement Real-Time Audio Capture and Transcription:
- Use PyAudio to capture microphone input.
- Stream audio to the Google Speech API and get the transcribed text in real-time.
Example Code
import pyaudio from google.cloud import speech from google.cloud.speech import enums from google.cloud.speech import types client = speech.SpeechClient() # Set up audio stream stream = pyaudio.PyAudio().open(format=pyaudio.paInt16, channels=1, rate=16000, input=True, frames_per_buffer=1024) # Define the streaming configuration config = types.RecognitionConfig( encoding=enums.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code='en-US', ) streaming_config = types.StreamingRecognitionConfig(config=config) def listen_for_speech(): audio_stream = stream.read(1024) requests = (types.StreamingRecognizeRequest(audio_content=audio_stream) for audio_stream in audio_stream) responses = client.streaming_recognize(streaming_config, requests) for response in responses: for result in response.results: print("Transcript: {}".format(result.alternatives[0].transcript))
Note: Real-time transcription accuracy may vary depending on factors like microphone quality and ambient noise. For best results, use high-quality microphones and clear speech.
Considerations and Best Practices
Consideration | Recommendation |
---|---|
Audio Quality | Ensure high-quality, noise-free audio input for better accuracy. |
API Quotas | Monitor usage to avoid exceeding API limits, which could result in additional costs. |
Latency | Consider using a buffer to reduce latency in real-time applications. |
Best Practices for Handling Audio Files with Google Speech to Text API
When working with the Google Speech to Text API, it's crucial to ensure that audio files are optimized for accurate transcriptions. The API supports multiple audio formats, but some formats may work better than others depending on the quality of the input and the use case. Efficiently handling audio files before sending them for transcription can significantly improve both the performance and the quality of the results.
This guide covers the best practices for managing your audio files to get the most reliable and accurate transcriptions using the Google Speech to Text API. From selecting the correct audio formats to preparing the files for optimal processing, these tips will help you streamline the transcription process.
1. Audio Format and Quality
Choosing the right audio format is crucial for ensuring accurate results. The Google Speech to Text API supports several formats, but each has its own advantages and considerations. Here’s a breakdown:
- WAV: Ideal for lossless audio quality, but larger file sizes. Use if high accuracy is required and file size isn’t a constraint.
- FLAC: Also lossless, but offers better compression than WAV. Recommended for high-quality audio with smaller file sizes.
- MP3: A compressed format, often used for smaller files. It may introduce minor quality loss but is acceptable for general transcription tasks.
Note: While MP3 is acceptable, always prefer lossless formats (WAV or FLAC) for the best results when clarity is essential.
2. Audio Preprocessing
To get the best results, consider preprocessing your audio files before sending them to the API:
- Remove Background Noise: Use noise reduction tools to eliminate unnecessary background sounds.
- Normalize Volume: Ensure consistent volume levels throughout the file to prevent discrepancies in transcription.
- Trim Silences: Cut out long periods of silence to reduce processing time and avoid errors in transcription.
Preprocessing audio files can greatly improve transcription accuracy and speed, especially when dealing with noisy or unevenly recorded audio.
3. File Size Limitations
The Google Speech to Text API has specific limits on the size of audio files that can be processed in one request:
Audio Format | File Size Limit |
---|---|
FLAC | 10 MB |
WAV | 10 MB |
MP3 | 10 MB |
Keep in mind that larger audio files may require splitting into smaller segments to stay within the API's limits.
How to Convert Audio Streams to Text Using Google Speech to Text API
Google's Speech to Text API provides a powerful solution for transforming audio streams into text. This can be incredibly useful for applications such as transcription services, voice commands, or creating subtitles. The process typically involves capturing audio data, sending it to the API, and receiving the transcribed text in response.
The following steps outline how to effectively convert an audio stream to text using the API in Python. This includes setting up the environment, handling audio input, and interacting with the API for real-time transcription.
Steps for Converting Audio Stream to Text
- Set up your environment: Before using the Google Speech to Text API, ensure you have the necessary libraries installed and a Google Cloud project configured.
- Capture the audio stream: Use a microphone or an audio input device to capture the live audio. The input should be in a supported format, such as FLAC or WAV.
- Send the audio to the API: Once you have the audio data, send it in chunks to the API using the streaming method. This allows for real-time transcription.
- Receive the transcribed text: The API will return the transcribed text after processing the audio. This can be used immediately for display or further processing.
Important Considerations
Always ensure your audio is of high quality for better transcription accuracy. Noise reduction techniques may improve results.
Example Python Code
Here's a sample of Python code to convert an audio stream into text using the Google Speech to Text API:
import pyaudio
from google.cloud import speech_v1p1beta1 as speech
client = speech.SpeechClient()
def transcribe_streaming():
with microphone_stream() as stream:
requests = (speech.StreamingRecognizeRequest(audio_content=content) for content in stream)
responses = client.streaming_recognize(config, requests)
for response in responses:
print(response.results[0].alternatives[0].transcript)
Audio Formats Supported
Audio Format | Description |
---|---|
FLAC | Lossless compression format, ideal for high-quality transcription. |
WAV | Uncompressed format, commonly used for audio streaming. |
MP3 | Popular format for compressed audio, but may reduce quality. |
Implementing Custom Language Models in Google Speech to Text API
Google Speech-to-Text API offers a flexible platform to convert audio into text, but for specialized applications, you might need to implement custom language models. Custom models help improve accuracy when the standard language models do not suit your use case, such as recognizing industry-specific terminology, names, or slang. Google allows users to train these models through the "Speech Contexts" feature, which enables the inclusion of domain-specific terms and phrases.
To create a custom language model, you need to prepare a list of phrases or terms that are highly relevant to your use case. These terms are incorporated into the API request, allowing Google to optimize the transcription process. This approach is particularly beneficial in environments with specialized vocabulary, such as medical or legal transcription.
Steps to Implement a Custom Language Model
- Prepare a list of custom phrases: Collect a list of domain-specific terms, names, or phrases that you expect to encounter in your audio files.
- Set up a Speech Context in the API: Use the 'speechContext' parameter in your API request to include the custom list of terms.
- Send the request with custom context: When calling the API, include the prepared list in the 'speechContexts' field of the request body.
Example Request with Custom Language Model
{ "config": { "encoding": "LINEAR16", "languageCode": "en-US", "speechContexts": [ { "phrases": ["telemedicine", "artificial intelligence", "machine learning"] } ] }, "audio": { "content": "base64_audio_data" } }
Additional Considerations
- Testing: Test the custom language model with different audio files to ensure it correctly recognizes the specialized terms.
- Optimization: You can improve accuracy by continuously updating the list with new terms and phrases as they arise in your application.
- Limits: Ensure your custom list does not exceed the size limits set by Google for speech contexts.
Note: Google Speech-to-Text API does not provide full training of custom models but rather allows the adjustment of its recognition process using context terms, which helps increase accuracy for specific domains.