Google Text to Speech Api Integration
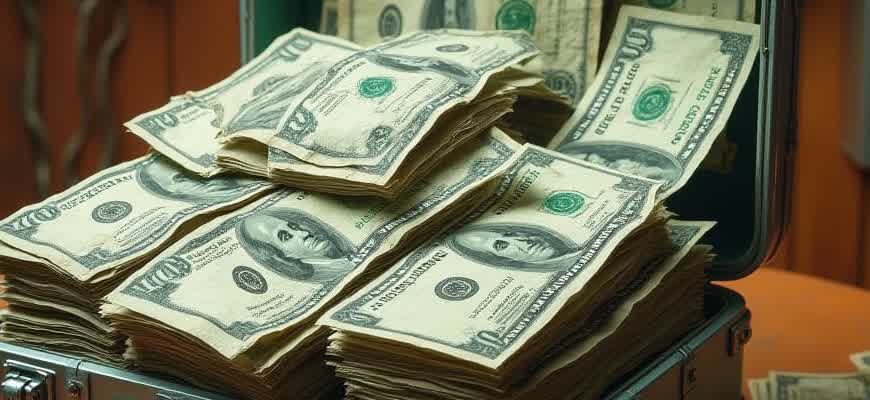
The Google Text-to-Speech API enables developers to convert text into natural-sounding speech using Google's machine learning models. This service is widely used for building applications that require voice interaction, such as virtual assistants, accessibility tools, and media applications. It supports multiple languages and offers a wide range of customization options, making it a powerful tool for developers looking to add speech synthesis to their projects.
Steps to integrate the API:
- Enable the Google Text-to-Speech API in the Google Cloud Console.
- Set up authentication by creating a service account key.
- Install the Google Cloud SDK and the necessary client libraries for your programming language.
- Use the API’s request parameters to customize the voice, language, and other settings for speech synthesis.
Key Features:
Feature | Description |
---|---|
Multiple Languages | Supports a wide range of languages and dialects for global use. |
Voice Selection | Allows choosing different voices and genders to personalize speech. |
Customizable Speech Parameters | Enables control over speech rate, pitch, and volume. |
Note: Always ensure you have a valid API key and that your usage complies with Google Cloud’s billing and usage guidelines.
Google Text to Speech API Integration: A Comprehensive Guide
The integration of Google Text to Speech (TTS) API into applications allows developers to convert written text into natural-sounding speech. This capability is useful for creating accessible applications, virtual assistants, and other services where voice output is required. In this guide, we'll walk through the necessary steps for integrating the TTS API, along with key features, best practices, and some important configuration options.
Google TTS API offers high-quality voices in various languages, enabling personalized user experiences across different platforms. With simple implementation, developers can easily add speech synthesis to their applications using RESTful API calls. This guide covers everything from setting up your environment to making API requests for text-to-speech functionality.
Key Features and Setup
To start using Google Text to Speech API, you need to follow a few key steps. Below is an outline of the setup process and the core features:
- Creating a Google Cloud Project: Begin by creating a project in the Google Cloud Console to access the Text to Speech API.
- Enabling the API: Enable the TTS API for your project from the Google Cloud Console.
- Authenticating Requests: Set up authentication by downloading the credentials (API key or service account key) from the Cloud Console.
- Installing Google Cloud SDK: Ensure the necessary libraries are installed in your environment, such as Google Cloud client libraries for Python, Node.js, etc.
Making Text-to-Speech Requests
Once the environment is set up, you can start making API requests. The TTS API provides several customizable options for voice selection, pitch, speaking rate, and more. Here's how to structure a basic request:
- Text Input: Specify the text you want to convert to speech.
- Voice Parameters: Choose language and voice settings (gender, accent, etc.).
- Audio Output: Define the format for the audio response (e.g., MP3, OGG).
Important: Ensure you handle API quotas and rate limits to prevent service interruptions.
Sample API Request
Here's an example of how you can make a simple API call using the Google Cloud SDK for Python:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, welcome to the Google TTS API!") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.FEMALE ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Customizing Voice and Language Settings
The Google TTS API offers a variety of voices and languages. Below is a table with some available voices and their language codes:
Language | Voice Name | Gender |
---|---|---|
English (US) | en-US-Wavenet-D | Male |
English (UK) | en-GB-Wavenet-B | Female |
Spanish (ES) | es-ES-Wavenet-A | Male |
French | fr-FR-Wavenet-C | Female |
Note: Explore the full list of available voices and languages in the official documentation.
How to Set Up Google Cloud Account for API Access
To get started with Google Text to Speech API, you'll need to create and configure a Google Cloud account. This process involves enabling the API, generating API keys, and managing billing. Below are the necessary steps to set up your Google Cloud account and gain access to the Text to Speech API.
Follow the steps outlined below to configure your account and ensure you're ready to use the API effectively. This will involve setting up a Google Cloud project, activating the Text to Speech API, and managing your API credentials.
1. Create a Google Cloud Project
- Visit the Google Cloud Console at https://console.cloud.google.com/.
- If you do not already have a Google Cloud account, sign up or log in with your existing Google account.
- Click on the Select a project dropdown in the top navigation bar and select New Project.
- Enter a project name and choose a billing account if needed. Click on Create.
2. Enable the Text to Speech API
- Navigate to the API Library within the Google Cloud Console.
- Search for "Text to Speech" in the API library search bar.
- Click on the Google Cloud Text to Speech API and then click on Enable.
- This will activate the API for your Google Cloud project.
3. Generate API Credentials
- In the Google Cloud Console, go to APIs & Services > Credentials.
- Click on Create Credentials and choose API Key.
- Your new API key will be displayed. Copy and save it securely for later use in your application.
Important: Keep your API key private. Do not expose it in public repositories or on the web.
4. Set Up Billing
In order to use the Text to Speech API, you must have billing enabled for your Google Cloud project. Google provides a free tier, but usage beyond that will incur charges. To link billing:
- Go to the Billing section in the Google Cloud Console.
- Link a billing account to your project, if not already linked.
- If necessary, set up a payment method in the billing console.
5. Verify Your Setup
Once the above steps are complete, you can test the API by calling it via your preferred programming language or through Google's provided SDKs. Verify your credentials and ensure that you have successfully linked your billing account to avoid any disruptions in service.
Step-by-Step Process for Integrating Google Text-to-Speech API with Your Application
Integrating Google Text-to-Speech (TTS) API into your application allows you to convert written text into natural-sounding speech. The process is straightforward, but requires proper setup and configuration. This guide will take you through the necessary steps to implement TTS functionality in your application using Google's API.
Before starting, make sure you have a Google Cloud project with billing enabled and that the Google Cloud SDK is installed on your machine. The integration involves setting up the API, obtaining credentials, and using the client libraries to send requests and receive audio data.
Prerequisites
- Google Cloud account with billing enabled
- API key or Service account credentials
- Installed Google Cloud SDK or client library for your programming language
- Text-to-Speech API enabled in the Google Cloud Console
Step-by-Step Guide
- Enable the API: Go to the Google Cloud Console and navigate to the "APIs & Services" section. Search for the "Text-to-Speech API" and enable it for your project.
- Get Credentials: In the Google Cloud Console, generate a service account key or API key. If using a service account, download the JSON file containing your credentials.
- Install the Client Library: Use the Google Cloud client library for your programming language. For example, in Python, you can install it using the following command:
pip install google-cloud-texttospeech
- Write Code to Send Request: In your application, initialize the TTS client using the credentials and send a request with the text you want to convert to speech. Below is an example in Python:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams(language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.FEMALE) audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
- Test the Integration: After executing the code, check the output audio file to ensure the text is converted correctly to speech.
Important Notes
Remember that the Text-to-Speech API is not free, and pricing is based on the number of characters processed. Check Google’s pricing page for detailed information.
Sample Configuration Table
Configuration | Value |
---|---|
Language Code | en-US |
Voice Gender | Female |
Audio Encoding | MP3 |
Choosing the Right Voice and Language for Your Project
When integrating speech synthesis into your application using Google Text-to-Speech API, selecting the appropriate voice and language is crucial to delivering a high-quality user experience. The choice of voice impacts how natural the speech sounds, and the language determines its compatibility with your target audience. These factors will directly influence user engagement, comprehension, and the overall effectiveness of the project.
Google Text-to-Speech API offers a wide range of options, but understanding the nuances of voice selection and language support can be challenging. It's important to make informed decisions based on your project goals and audience needs. Below are some key aspects to consider when choosing the voice and language for your speech application.
Voice Selection: Gender, Tone, and Quality
Google Text-to-Speech provides both male and female voices, which can be further customized for tone, speed, and pitch. To select the best voice, you should consider the following criteria:
- Project Purpose: Is your project intended for formal or casual use? For instance, a formal voice might suit customer support applications, while a more casual tone is better for entertainment or games.
- Audience Preference: If your users are diverse, offering multiple voice options can help cater to different preferences.
- Language Compatibility: Some languages may only have a limited selection of voices, so consider the languages you need and check the availability of corresponding voices.
Language Selection: Regional Variations and Accuracy
Each language has its own set of characteristics and regional variations. For a project aimed at a specific region, choosing the appropriate language variant is essential for ensuring accuracy and understanding. For example, the English language has several variants, including American, British, and Australian English, each with distinct pronunciations and idioms.
- Regional Variants: Always check for regional versions of a language to ensure clarity and relevance to your target audience.
- Language Support: Ensure the language you choose is supported by Google Text-to-Speech API, as some languages may be in beta or not supported at all.
- Pronunciation Precision: For specialized content like medical or technical terminology, consider how accurately the language model can handle these words.
Quick Reference: Voice and Language Availability
Language | Available Voices | Regional Variants |
---|---|---|
English | Male, Female | US, UK, Australia, India |
Spanish | Male, Female | Spain, Latin America |
German | Male, Female | Standard, Austria |
Choosing the right voice and language for your project not only affects user satisfaction but also improves the accessibility and inclusivity of your content. Always test different combinations to find the most effective setup for your needs.
Managing API Limits and Quotas: Essential Information
When integrating the Google Text to Speech API into your application, it’s important to understand the limitations and quotas imposed by the service. These constraints can significantly impact your usage, especially in production environments where high traffic is expected. Being aware of these limits helps prevent unexpected interruptions or slowdowns, and ensures that your service remains stable under load.
Each API call consumes a portion of your allocated quota, and if you exceed these limits, your application may experience delays or errors. Properly managing these quotas can be achieved by monitoring usage patterns and optimizing your calls. Below is an overview of key aspects to consider when dealing with API limits.
Types of Limits to Be Aware Of
- Daily Quotas: The number of requests you can make per day is often capped. Be mindful of the daily limits set by Google, as exceeding these may require you to wait until the next reset period.
- Request Frequency: Google imposes a rate limit on how frequently you can make API calls. These limits can prevent misuse and ensure fair access to resources.
- Resource Consumption: Each call consumes resources, and certain features may cost more in terms of API usage. For instance, higher-quality voices or longer audio outputs could result in higher charges.
How to Monitor and Optimize Usage
- Use the Google Cloud Console: Regularly check the quota usage in the Google Cloud Console to stay informed about your API consumption.
- Optimize API Calls: Implement batching or caching techniques to reduce the number of requests. For example, avoid sending duplicate or unnecessary requests for the same content.
- Set Up Alerts: Configure email notifications for when you approach your limits, ensuring you can take action before you hit the quota ceiling.
Important: Google may apply different pricing tiers depending on the quota you use. Always review your billing information and adjust usage accordingly to avoid unexpected costs.
Understanding API Limits in Detail
API Feature | Limit | Action Required |
---|---|---|
Standard API Calls | 500,000 characters per day | Monitor usage and optimize calls to avoid exceeding the limit. |
Advanced API Calls | Up to 50,000 characters per day | Consider upgrading your API plan if higher usage is expected. |
Premium Voices | 5,000 characters per day | Limit use of premium voices or switch to standard voices to reduce costs. |
Tip: Review Google’s official documentation for the most up-to-date information on usage limits and pricing adjustments.
How to Enhance Audio Quality in Text-to-Speech Implementation
Integrating a text-to-speech system can significantly improve accessibility and user experience. However, achieving high-quality audio output requires careful attention to several factors, from voice selection to language processing. The right configuration ensures clear, natural-sounding speech that enhances user engagement and reduces listener fatigue.
Optimizing audio quality involves fine-tuning various parameters of the text-to-speech system, such as voice tone, speed, and pitch. Additionally, providing the appropriate language models and ensuring compatibility with the target platform play a crucial role in delivering a polished, professional product.
Key Steps to Optimize Speech Output
- Choose the Right Voice: Selecting the most natural and clear voice is critical. Most text-to-speech APIs, including Google’s, offer multiple voices in different languages. Test different voices to determine which suits your application's tone and user preferences.
- Adjust Speech Speed: Altering the speed can significantly impact the clarity of the speech. Too fast can make the speech unclear, while too slow can become monotonous. Find a balance that suits your audience.
- Fine-tune Pitch and Intonation: Modifying pitch can help in delivering more expressive and varied speech, making it feel less robotic and more human-like.
Factors to Consider for Better Audio Clarity
- Background Noise Reduction: Ensure that your system filters out unnecessary background noise that could distort the audio.
- Phonetic Accuracy: A high-quality text-to-speech engine should interpret and pronounce words correctly, especially for uncommon terms or industry jargon.
- Sampling Rate: Use a high sampling rate for better audio fidelity, ensuring smooth transitions and reducing distortions.
To ensure optimal results, continually test and adjust the voice characteristics based on user feedback and specific use cases. A well-tuned voice can make a significant difference in user experience.
Technical Parameters for Better Quality
Parameter | Recommended Range |
---|---|
Sampling Rate | 16 kHz to 22 kHz |
Speech Speed | 150 - 180 words per minute |
Pitch | Default, adjust within 0.8 to 1.2 |
Real-Time Voice Synthesis: Optimizing for Low Latency
Integrating a text-to-speech engine in real-time applications requires configuring the system to ensure minimal delay between text input and audio output. When aiming for low latency, several factors need to be considered, from network performance to API settings. By carefully adjusting these configurations, developers can achieve a smoother experience that feels natural to the user.
Optimizing for latency involves selecting the right synthesis models, managing system resources, and fine-tuning the parameters of the speech synthesis API. Additionally, proper data handling and network optimization can drastically reduce delays, leading to faster and more accurate audio output in real-time scenarios.
Key Configurations for Low Latency
- API Selection: Use the appropriate model for your needs. Google Cloud offers various TTS models that differ in complexity and processing time.
- Audio Format: Choose the most efficient audio format (e.g., MP3 or OGG) that balances quality and processing speed.
- Network Optimization: Minimize network latency by choosing a region for the API that is closest to your server or user location.
Important Considerations
Reducing the complexity of the synthesis model can lead to faster response times but might sacrifice audio quality. Striking a balance between the two is essential for real-time applications.
Configuration Steps
- Select a low-latency synthesis model (e.g., WaveNet or standard models).
- Optimize the input text by simplifying or pre-processing it to reduce the time taken by the API to generate speech.
- Use caching techniques for repetitive phrases to avoid re-synthesizing the same text.
- Set the audio encoding format to a more efficient type (e.g., low-bitrate MP3) to reduce data size and processing time.
Latency Factors Table
Factor | Impact on Latency |
---|---|
Model Complexity | Higher complexity increases processing time. |
Network Speed | Slower connections add delay to request and response times. |
Audio Format | Uncompressed formats increase data size and processing load. |
Monitoring API Usage and Managing Costs with Google Cloud Billing
When integrating Google Text to Speech API, it's crucial to track your API usage to avoid unexpected charges. Google Cloud provides several tools and features to help users monitor their resource consumption effectively. By understanding the usage patterns, you can optimize your integration, ensuring both efficiency and cost-effectiveness.
Google Cloud Billing offers a robust system to manage costs. You can set up budgets, receive notifications, and track usage in real-time. This allows you to have full control over your spending, ensuring that the integration remains within your budget while maintaining the required service level.
Tracking API Usage
- Cloud Console Dashboard: View your API usage in real-time and identify any spikes or trends that could lead to unexpected costs.
- Usage Reports: Detailed reports can provide insights into API consumption over various time periods.
- Cost Analysis: Use the Google Cloud Cost Management tools to break down your expenses by service or project.
Setting Budget Alerts
- Create a budget in the Google Cloud Console.
- Set up email notifications to alert you when your spending approaches or exceeds your budgeted amount.
- Adjust your budget based on your project's growth and usage patterns to stay within financial limits.
Important: Regularly review your billing reports and usage data to prevent unforeseen charges. Use Google Cloud’s billing alerts to ensure costs do not spiral out of control.
Cost Control Tips
Tip | Action |
---|---|
Optimize API Calls | Group requests to reduce the number of calls and minimize costs. |
Use Quotas | Set quotas for API usage to prevent overuse and unexpected charges. |
Monitor Billing Reports | Review detailed billing reports to spot any inefficient API usage. |