Openai Text to Speech Api Reference
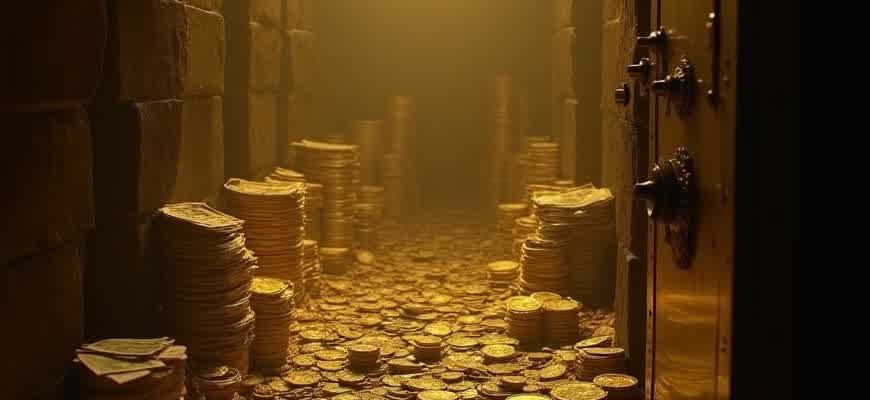
The OpenAI Text-to-Speech API allows developers to integrate advanced voice synthesis capabilities into their applications. By leveraging deep learning models, this API converts text input into natural-sounding speech with customizable parameters.
Key features of the API include:
- Support for multiple languages and voices
- Adjustable pitch, speed, and tone of speech
- High-quality, realistic voice generation
- Scalable for various use cases, from simple apps to large-scale deployments
To get started, you need to authenticate with your API key and make a POST request to the endpoint with the required parameters. Here's a simple flow:
- Obtain your API key from the OpenAI dashboard
- Set up the HTTP request with necessary headers
- Submit the text to be converted into speech
- Handle the audio output returned by the API
Note: Ensure that you properly configure your request with all required parameters to avoid errors in the response.
Below is an example of the request structure:
Parameter | Type | Description |
---|---|---|
text | String | The text to be converted into speech |
voice | String | Specifies which voice to use for the speech synthesis |
speed | Float | Adjusts the rate at which the speech is delivered |
pitch | Float | Modifies the pitch of the generated speech |
OpenAI Text to Speech API: A Comprehensive Guide
The OpenAI Text to Speech API enables developers to convert text into natural-sounding audio with ease. This API offers various configurations to adjust the speech's tone, pace, and pitch, providing a more human-like audio experience. It supports multiple languages and can be integrated into various applications, from virtual assistants to accessibility tools.
This guide will explore the key features of the API, the available options, and how to implement it effectively for different use cases. It aims to provide an in-depth understanding of how to leverage the API for enhanced user interactions and personalized content delivery.
Key Features and Configuration Options
- Voice Selection: Choose from a range of voices tailored to different languages and accents.
- Speech Parameters: Fine-tune the rate, pitch, and volume of the output voice.
- Multiple Languages: Support for various languages, making it adaptable to global audiences.
How to Use the API
- Authenticate with your OpenAI account and get an API key.
- Choose the desired voice and configure speech parameters.
- Send a POST request with the text to be converted to audio.
- Receive the audio file in the chosen format (e.g., MP3, WAV).
Important: Be sure to monitor your API usage to avoid exceeding rate limits. OpenAI provides detailed usage statistics in your account dashboard.
Example API Request
Parameter | Description |
---|---|
text | The input text to be converted to speech. |
voice | The selected voice for the audio output. |
rate | Speed of speech (0.5 - 2.0). |
pitch | Pitch of the speech (-1.0 to 1.0). |
format | The desired audio file format (e.g., MP3, WAV). |
How to Integrate OpenAI's Speech Synthesis API into Your Application
Integrating OpenAI’s Text-to-Speech API into your project is a straightforward process, but it requires careful configuration and understanding of the available features. To begin using the API, you’ll first need to create an API key, set up the correct environment, and then implement the API calls in your code. Below, we'll walk you through the steps required to set it up successfully in your project.
Before starting the integration process, make sure that you have the necessary software, libraries, and dependencies installed. The OpenAI API requires a stable internet connection and access to your project’s backend, where API requests can be securely made. Once you have these prerequisites in place, proceed with the following setup steps.
Steps to Set Up OpenAI Text-to-Speech API
- Step 1: Obtain API Key
To use the OpenAI Text-to-Speech API, you first need to register on the OpenAI platform and generate an API key. This key will be used to authenticate your requests. Keep it secure, as it grants access to your usage limits and billing information.
- Step 2: Install Required Libraries
Depending on the programming language you’re using, install the corresponding libraries that will allow your project to communicate with the OpenAI API. For example, in Python, you can install the OpenAI package with the following command:
pip install openai
- Step 3: Set Up the API Request
Make a POST request to the OpenAI API using your API key. You will need to specify the text you wish to convert into speech and any additional parameters like voice or language.
Code Example
import openai openai.api_key = 'your-api-key' response = openai.Audio.create( model="text-to-speech", input="Hello, how are you today?", voice="en_us_male", ) audio_url = response['audio_url']
Important: Ensure that your API key is not exposed in public repositories or shared in an unsecured environment. Always use environment variables or a secure method to store sensitive information.
Common Configuration Options
Option | Description |
---|---|
voice | Choose the voice for speech synthesis. Options include male, female, and other accents and languages. |
language | Specify the language for text-to-speech. Make sure it matches the voice you choose. |
audio_format | Set the desired audio format (e.g., mp3, wav). |
Understanding the Key Parameters for Optimizing Speech Output
When working with a text-to-speech system, there are several key parameters that directly affect the quality and clarity of the generated audio. These parameters allow users to fine-tune the voice output according to the desired characteristics, such as tone, pace, and clarity. By mastering these settings, developers can significantly improve user experience in applications like virtual assistants, audiobooks, and accessibility tools.
In this section, we will explore some of the most crucial parameters available in a typical Text to Speech API, highlighting their role and impact on the final audio output. Understanding how to adjust these settings can help you create a more natural, engaging, and tailored listening experience for your audience.
Key Parameters for Speech Output Optimization
- Voice Selection: The choice of voice is essential in determining the gender, age, and tone of the generated speech. Selecting the appropriate voice helps create a more relatable and engaging experience for the user.
- Speech Rate: This parameter controls the speed of the speech. A faster rate can be useful for dynamic content, while a slower rate can enhance clarity, particularly for complex topics or when accessibility is a concern.
- Pitch: Adjusting pitch allows you to make the voice sound more high-pitched or low-pitched. Modifying pitch can make speech sound more expressive, affecting the emotional tone of the output.
- Volume Gain: Volume control is crucial for ensuring that the audio output is neither too soft nor too loud. Proper volume gain ensures that speech is clear and intelligible in various environments.
Important Considerations for Fine-Tuning
Note: It is essential to test the speech output in the context of your application. A setting that works well in one scenario may not be as effective in another.
- Start with default settings and adjust incrementally, evaluating the results after each change.
- Consider your audience's preferences and needs (e.g., age, language proficiency, or hearing impairments) when choosing the voice and adjusting settings.
- Ensure the pace and clarity of the speech are balanced. Too fast speech can overwhelm the listener, while too slow speech might sound unnatural.
Sample Speech Settings Comparison
Parameter | Low Setting | High Setting |
---|---|---|
Speech Rate | Slow and clear | Fast and energetic |
Pitch | Low and steady | High and expressive |
Volume Gain | Soft and subtle | Loud and impactful |
How to Personalize Speech Styles and Tones with OpenAI TTS
OpenAI's Text-to-Speech (TTS) API provides a range of options to adjust voice parameters, allowing developers to create customized audio outputs that fit specific use cases. By modifying the tone, style, and speech patterns, you can enhance the user experience, whether for conversational agents, audio books, or virtual assistants. Customizing voice parameters is essential for making the output sound more natural and aligned with the desired context.
Several key parameters can be manipulated to achieve the desired vocal characteristics. The TTS API allows you to adjust pitch, rate, and emphasis, along with other settings. Additionally, pre-built voice models provide various options for gender, accent, and even emotion. Understanding how to use these features will enable you to tailor the voice to meet specific needs.
Adjusting Pitch and Rate
Pitch and speech rate are fundamental factors in modifying the sound of speech. The API provides fine control over these parameters, allowing you to make the voice sound higher or lower, faster or slower. These adjustments can significantly change the perception of a voice, making it more engaging or suitable for particular content.
- Pitch: Changes the frequency of the voice's sound, creating a higher or lower tone.
- Rate: Controls the speed of speech, influencing how quickly words are spoken.
Incorporating Emotional Styles
To further personalize the output, developers can apply different emotional tones to the generated speech. OpenAI's TTS API supports a variety of emotional voices, such as happy, sad, or angry. This allows for a more nuanced communication style, ideal for interactive systems or content that requires emotional engagement.
- Happy: Adds a cheerful and positive tone to the speech.
- Sad: Introduces a more somber or melancholic tone.
- Angry: Creates a voice with intensity and frustration.
Configuring Accents and Genders
OpenAI's API also allows you to adjust the accent and gender of the voice. This can be particularly useful for making the output more region-specific or ensuring the voice matches the target audience. You can select from a variety of options, including American, British, and Australian accents.
Accent | Voice Option |
---|---|
American | Available in both male and female voices |
British | Options for male and female voices |
Australian | Distinct male and female voice selections |
Note: Always ensure that the selected voice style and tone align with the purpose of the content, as mismatches can lead to misinterpretations or a less engaging user experience.
Integrating OpenAI's Text-to-Speech Capabilities with Web and Mobile Applications
Integrating OpenAI’s Text-to-Speech (TTS) API into your web and mobile applications offers a seamless way to convert written content into natural, high-quality speech. By doing so, you can enhance user experience, particularly for accessibility features, voice assistants, and content consumption. This integration allows users to interact with your app in a more dynamic and engaging way, improving usability and providing a broader range of interaction methods.
To integrate OpenAI’s TTS technology into your application, you must follow several essential steps, including setting up the API, handling user inputs, and properly managing audio output. In both web and mobile environments, the integration process can vary slightly depending on the platform. However, the core principles remain consistent: connecting to the API, passing the necessary text, and handling the generated speech effectively.
Steps for Integration
- Set Up API Access: To start using the TTS service, you need to register and obtain an API key from OpenAI’s platform.
- Make API Requests: Send requests to the TTS API, providing text data, preferred language, and voice settings.
- Handle Audio Output: Once the speech data is returned, manage the playback on the client-side, either through an audio player in web apps or using the native audio capabilities on mobile apps.
- Optimize for Performance: Ensure minimal latency and smooth audio playback by optimizing the way audio files are cached and played.
Key Features to Consider
- Voice Customization: Choose from a variety of voices, accents, and speech styles to match your application's tone and target audience.
- Multi-Language Support: OpenAI's TTS system can generate speech in multiple languages, expanding your app’s accessibility and reach.
- Real-time Generation: The API generates speech quickly, allowing for responsive interactions within the app.
“Text-to-Speech API integration not only improves accessibility but also enhances user engagement by allowing real-time, interactive voice-based experiences.”
Implementation in Web vs. Mobile
Aspect | Web | Mobile |
---|---|---|
API Integration | HTTP requests, JavaScript libraries for handling audio | SDKs for iOS and Android, native audio handling |
Audio Playback | HTML5 audio or JavaScript-based players | Native audio players or system-level support |
Customization | Wide range of browser-based customizations | Platform-specific optimizations for mobile devices |
Handling Multiple Languages and Accents in OpenAI TTS
One of the key features of OpenAI's Text-to-Speech (TTS) API is its ability to produce speech in different languages and accents. This flexibility allows developers to create applications that cater to diverse linguistic audiences, ensuring a more personalized and accessible user experience. To effectively handle multiple languages and accents, it's important to understand how OpenAI's TTS system processes linguistic data and how to adjust settings accordingly.
When integrating TTS into a multilingual application, proper configuration is essential. The system provides various parameters for selecting languages and accents, allowing developers to tailor the speech output to their specific requirements. Understanding the API's language models, as well as accent variants, can significantly enhance the accuracy and quality of the generated speech.
Language and Accent Selection
OpenAI TTS supports a broad range of languages and regional accents. To ensure correct pronunciation and natural-sounding speech, the language and accent should be explicitly specified when making API calls. Below are key considerations for managing different languages and accents:
- Language Codes: Use standardized language codes (e.g., "en" for English, "es" for Spanish) to specify the desired language.
- Accent Variants: Within a single language, multiple accents may be available, such as "en-US" for American English and "en-GB" for British English.
- Contextual Adjustments: Some languages or accents might require additional tuning for more accurate pronunciation based on regional variations or formal vs. informal contexts.
Key Parameters for TTS API
To properly handle different languages and accents, you should be familiar with the following API parameters:
Parameter | Description |
---|---|
language | Specifies the primary language for the speech output (e.g., "en" for English, "fr" for French). |
accent | Defines the accent variant within the specified language (e.g., "en-GB" for British English). |
voice | Allows selection of specific voice models optimized for different languages and accents. |
Important: Always check the supported languages and accents for the specific API version you are using, as available options may vary.
Challenges and Best Practices
When dealing with multiple languages and accents, certain challenges may arise, such as pronunciation issues or poor quality in less common accents. To mitigate these challenges, consider the following:
- Test thoroughly: Always test the speech output in various languages and accents to identify any inconsistencies or issues.
- Use localized data: For regions with strong dialectal differences, ensure that regional speech data is used for better accuracy.
- Provide fallback options: In cases where a specific accent is not available, ensure that a neutral or default accent is used to avoid poor user experience.
Effective Strategies for Cost Management with OpenAI Text-to-Speech API
When using OpenAI's Text-to-Speech API, it’s crucial to monitor and optimize your usage to avoid unexpected costs. A strategic approach can help you maximize the efficiency of the API while staying within your budget. By understanding the key pricing factors and implementing best practices, you can ensure cost-effective utilization of the service.
Optimizing API usage requires a thorough understanding of the available features and their respective costs. For instance, processing a high volume of text or utilizing premium voices can significantly affect your expenditure. To maintain cost efficiency, follow proven practices that limit unnecessary usage and scale your operations thoughtfully.
Best Practices for Optimizing API Costs
- Monitor Usage Regularly: Regularly check the API usage through your OpenAI account to ensure that you are not exceeding the limits you set for yourself. Frequent monitoring helps in identifying patterns that may lead to higher costs.
- Choose the Appropriate Voice: OpenAI offers different voice models, with varying costs. Selecting a lower-cost model may be suitable for standard use, while premium voices should only be used when necessary.
- Batch Processing: Instead of making multiple API calls for small chunks of text, batch larger sections of content together to reduce the number of calls and, consequently, the cost.
Cost Control Tools and Techniques
- Set Usage Limits: Utilize the API's usage limit settings to cap your daily or monthly usage. This ensures that you do not unintentionally exceed your budget.
- Optimize Text Input: Review and trim the text that is being processed. Eliminating unnecessary content from the input will reduce the processing time and the associated costs.
- Leverage Caching: Cache the results of frequently requested text-to-speech outputs to avoid repeated calls for the same content.
Always stay updated with the latest changes in API pricing. OpenAI may update pricing models or offer new cost-saving options, and staying informed will help you adapt your usage accordingly.
Cost Breakdown
Service | Pricing Model |
---|---|
Standard Voices | Per minute of audio generated |
Premium Voices | Per minute of audio generated (higher rate) |
Text Input Length | Each character counts toward usage limits |
Managing Errors and Troubleshooting OpenAI TTS API Requests
When interacting with the OpenAI Text-to-Speech (TTS) API, it's essential to understand how to manage and troubleshoot potential issues that may arise during API calls. Errors can occur for a variety of reasons, from network problems to incorrect parameters in the request. Addressing these errors promptly ensures smooth integration and consistent performance.
By following a few best practices and understanding common error responses, developers can easily debug and resolve issues with the TTS API. The most common errors are related to improper API keys, incorrect data formats, and exceeding rate limits. This section provides an overview of these issues and how to handle them effectively.
Common API Error Codes
Below are some of the most frequent error responses returned by the OpenAI TTS API:
Error Code | Explanation | Solution |
---|---|---|
400 | Bad Request – This indicates an invalid input or malformed request. | Check the request structure, including the parameters. Ensure that the audio format and language are correctly specified. |
401 | Unauthorized – The API key is either missing or invalid. | Verify your API key. Ensure that it's correctly passed in the header of the request. |
429 | Rate Limit Exceeded – Too many requests in a short period. | Review the rate limits for your API key and adjust the frequency of your requests. Consider implementing retries with exponential backoff. |
Debugging Tips
Here are some steps to help you troubleshoot common issues with the OpenAI TTS API:
- Check API Response Body: Always review the error message in the response body. It often contains additional details about the issue.
- Ensure Correct Audio Format: Ensure that the requested audio format matches one of the supported formats, such as WAV or MP3.
- Verify Input Parameters: Double-check the parameters sent in the request, including the text to be converted and any specific voice settings.
- Monitor Rate Limits: Keep track of the rate limits associated with your API key to avoid hitting the maximum request threshold.
Key Considerations
Important: Always ensure that your API key is kept secure and not exposed in public repositories or shared with unauthorized users.
How to Track and Assess Voice Synthesis Quality and User Interaction
When using text-to-speech (TTS) technology, ensuring that the generated voice quality is high and the user experience (UX) is seamless is crucial for both developers and end users. Monitoring speech performance involves both technical and user-centric aspects, focusing on how accurately the speech is rendered and how well it engages the user. By collecting data on various parameters such as speech clarity, naturalness, and emotional tone, developers can fine-tune the system for optimal performance.
To monitor and analyze these elements effectively, it’s essential to employ both objective measurements (such as signal quality) and subjective evaluations (such as user satisfaction surveys). Implementing real-time monitoring tools, user feedback mechanisms, and conducting regular quality audits can help ensure the system remains robust and responsive to changes.
Key Metrics for Speech Quality Evaluation
- Speech Clarity: Assess the sharpness and intelligibility of the output speech. A system with high clarity ensures words are easily distinguishable.
- Naturalness: Measure how human-like the voice sounds. High-quality TTS systems should aim for a natural cadence and tone.
- Emotional Expression: Evaluate if the system can convey different emotions based on context or user intent.
- Speed and Latency: Track how quickly the system processes input and outputs the speech, ensuring minimal delay for user interaction.
Approaches for Gathering Feedback
- User Surveys: Direct feedback from users helps in understanding their satisfaction with the voice output. Ask about clarity, tone, and overall experience.
- A/B Testing: Run experiments with different voices or speech parameters and compare user preferences to select the best configuration.
- Automated Speech Analytics: Use tools to analyze speech quality in real-time, looking for issues like unnatural pauses, monotony, or mispronunciations.
Continuous monitoring and feedback are critical to maintaining high-quality speech synthesis. Use both quantitative and qualitative data to refine the TTS system over time.
Sample Metrics for Analysis
Metric | Target Range | Measurement Method |
---|---|---|
Speech Clarity | 90% - 100% | User Feedback, Automated Testing |
Naturalness | High | Perceptual Evaluation, Expert Review |
Emotional Range | Varies by Context | Subjective User Ratings, Speech Synthesis Analysis |
Latency | Less than 1 second | Real-time Monitoring |