How to Make a Speech Synthesizer
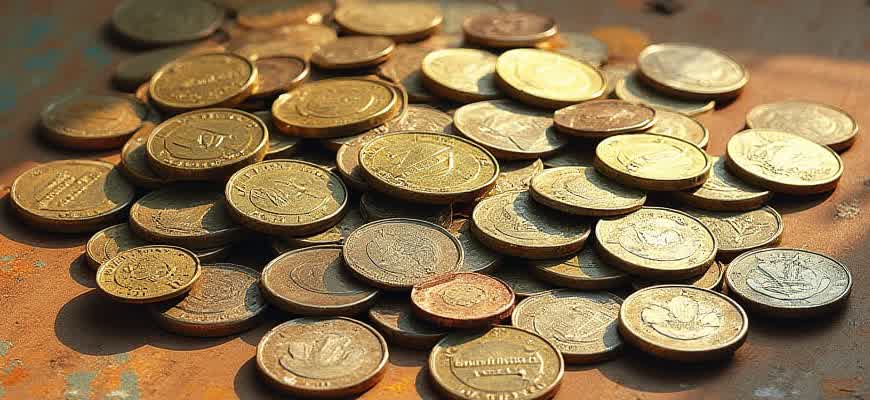
Developing a speech synthesizer involves multiple stages, including the selection of algorithms, processing of text input, and converting it into an audio output. This process requires knowledge in signal processing, machine learning, and natural language processing (NLP).
The main steps to create a functional speech synthesizer are as follows:
- Text analysis and preprocessing
- Phoneme conversion and prosody generation
- Waveform synthesis
- Audio output generation
Key Technologies Used:
Technology | Description |
---|---|
Deep Learning | For natural-sounding voice generation and prosody prediction |
Text-to-Phoneme Conversion | Translates written text into phonemes, the smallest units of sound |
"Creating a realistic speech synthesizer requires a balance of high-quality training data and sophisticated algorithmic models."
Choosing the Right Programming Language for Your Speech Synthesizer
When developing a speech synthesizer, the choice of programming language is crucial. It directly impacts the performance, scalability, and integration with other systems. Several factors should be taken into consideration before making the final decision, such as the language's support for audio processing, real-time performance, and compatibility with existing libraries or frameworks.
There are multiple programming languages available, each with its own strengths and weaknesses for building a speech synthesizer. In this article, we will explore some of the most commonly used languages, as well as their suitability for speech synthesis tasks.
Factors to Consider
- Audio Processing Support: The language should have robust libraries for handling audio input, processing, and output.
- Real-time Performance: Speech synthesis often requires real-time processing, so the language must be capable of handling time-sensitive tasks.
- Ease of Integration: The programming language should be compatible with speech synthesis APIs or frameworks that can facilitate the development process.
- Community and Documentation: A strong developer community and extensive documentation are essential for troubleshooting and learning.
Popular Programming Languages for Speech Synthesis
- Python: Python is widely used in speech synthesis due to its extensive libraries like Pyttsx3 and gTTS. Its simplicity and strong community support make it an excellent choice for rapid prototyping.
- C++: Known for its high performance and low-level control over hardware, C++ is ideal for building real-time, high-performance speech synthesizers.
- Java: Java's cross-platform capabilities and mature text-to-speech libraries like FreeTTS make it a good choice for building scalable and portable speech synthesis applications.
Comparison of Key Languages
Language | Performance | Libraries | Community Support |
---|---|---|---|
Python | Moderate | High | Strong |
C++ | High | Moderate | Strong |
Java | Moderate | High | Moderate |
Note: The best choice of programming language depends on your project's specific requirements, such as real-time performance and the need for cross-platform compatibility.
Setting Up the Speech Synthesis Environment on Your Machine
Before diving into creating a speech synthesizer, it’s essential to configure your system properly. A solid environment will ensure smooth execution of speech synthesis tasks and enable the use of libraries and frameworks that support text-to-speech (TTS) functionalities. Depending on your platform, there are specific steps you need to follow to prepare your development environment.
This section covers the essential tools, libraries, and dependencies you'll need to install, along with steps to get started on both Windows and Unix-based systems. We will also discuss the prerequisites for using popular TTS libraries such as Google Text-to-Speech and Festival.
Installing Dependencies
To begin, you need to ensure that the appropriate libraries and dependencies are installed on your machine. Below are the key packages required for speech synthesis:
- Python (recommended version: 3.x)
- SpeechRecognition (for integrating recognition with synthesis)
- pyttsx3 (offline text-to-speech engine)
- gTTS (Google Text-to-Speech API wrapper)
- pyaudio (for microphone input, if using speech recognition)
System Setup for Different Platforms
Follow the specific steps below for installing the necessary tools depending on your operating system:
- Windows:
- Install Python from the official website.
- Open Command Prompt and run:
pip install pyttsx3 SpeechRecognition gTTS pyaudio
- Linux:
- Use package managers like
apt-get
oryum
to install dependencies:
sudo apt-get install python3-pip python3-dev
- Use package managers like
- Install required libraries using pip:
pip3 install pyttsx3 SpeechRecognition gTTS pyaudio
Important Considerations
Ensure that your microphone and speakers are working properly if you intend to implement speech recognition or audio playback.
Example Setup Overview
Library | Purpose | Installation Command |
---|---|---|
pyttsx3 | Offline text-to-speech engine | pip install pyttsx3 |
gTTS | Google Text-to-Speech API | pip install gTTS |
SpeechRecognition | Speech recognition integration | pip install SpeechRecognition |
pyaudio | Microphone input handling | pip install pyaudio |
Understanding Phonetic Algorithms for Accurate Speech Generation
Phonetic algorithms are integral to the conversion of written text into natural speech. These algorithms break down the text into phonetic components, which represent sounds that correspond to specific letters or combinations of letters in a language. The primary goal is to ensure that the synthesized speech sounds smooth, accurate, and true to the linguistic rules of the language being processed. A well-designed algorithm accounts for phonemes, which are distinct sound units, as well as syllables, stress patterns, and contextual variations in pronunciation.
Different approaches to phonetic algorithms exist, each with its own strengths in producing precise speech. Rule-based systems rely on predefined linguistic rules, statistical models use data-driven methods to predict phonetic outcomes, and modern deep learning techniques can generate highly nuanced speech by learning from large speech datasets. Understanding the trade-offs between these methods is crucial when building a speech synthesizer that can handle complex language features.
Types of Phonetic Algorithms
- Rule-based systems: These systems follow a strict set of phonetic rules that map written characters to specific sounds, ensuring high accuracy in languages with well-defined pronunciation patterns.
- Statistical models: These algorithms analyze vast amounts of language data to predict phonetic structures, making them adaptable to various accents and dialects.
- Neural network approaches: Leveraging deep learning, these methods generate highly realistic speech by modeling complex relationships between textual input and auditory output.
Challenges in Phonetic Algorithm Development
The challenge for phonetic algorithms lies in their ability to handle variations such as homophones, word stress, and the impact of context on pronunciation.
- Homophones: Words that sound the same but have different meanings or spellings, like "sew" and "so," can confuse the system if not properly distinguished.
- Contextual pronunciation: The same word can be pronounced differently depending on surrounding words or sentence structure.
- Accents and dialects: Pronunciation can vary widely across regions, requiring the algorithm to account for these differences for accurate synthesis.
Comparison of Phonetic Algorithm Approaches
Algorithm Type | Advantages | Limitations |
---|---|---|
Rule-based | High accuracy for languages with consistent pronunciation rules | Limited flexibility in dealing with exceptions and irregular words |
Statistical | Adaptable to different accents and linguistic contexts | Requires large datasets and can struggle with rare words |
Neural networks | Produces natural-sounding speech, even for complex language structures | Computationally intensive and requires significant training data |
Building a Text-to-Speech Engine Using Open Source Libraries
Creating a text-to-speech (TTS) system from scratch may seem like a daunting task, but leveraging open-source libraries makes the process more manageable and efficient. There are several frameworks and tools available that can help developers implement a fully functional TTS engine. By combining speech synthesis algorithms with readily available data models, developers can create natural-sounding voices with minimal effort.
In this guide, we will explore how to build a TTS engine using popular open-source libraries, highlighting key components, features, and steps involved in the process. We will focus on some of the most widely used libraries in the TTS community, such as eSpeak, Festival, and Mozilla's TTS. By understanding the setup, configuration, and customization of these tools, you'll be able to develop your own voice synthesis application.
Key Open Source Libraries for TTS Development
Here are a few notable open-source libraries you can use to create a text-to-speech engine:
- eSpeak - A compact open-source software speech synthesizer for English and other languages.
- Festival - A general-purpose, multi-lingual speech synthesis system that supports both text-to-speech and voice training.
- Mozilla TTS - A deep learning-based TTS system that provides high-quality voice synthesis with pre-trained models.
Steps to Build a TTS Engine
- Install the chosen TTS library: Download and configure the library of your choice (e.g., eSpeak, Festival, or Mozilla TTS).
- Set up the required dependencies: Ensure that all necessary dependencies and prerequisites, such as Python or additional libraries, are installed.
- Prepare text input: Convert your input text into the correct format required by the TTS system.
- Customize the voice model: Many open-source TTS engines allow you to modify or train custom voice models. This is crucial if you need a specific accent or tone.
- Integrate the TTS engine: Finally, integrate the TTS engine into your application, ensuring it can process dynamic text and generate speech in real-time.
Tip: For better results with Mozilla TTS, consider using high-quality pre-trained models. These models are capable of producing more natural-sounding voices and require less computational effort.
Table: Comparison of Open Source TTS Libraries
Library | Supported Languages | Voice Quality | Installation Complexity |
---|---|---|---|
eSpeak | Multiple languages | Basic, robotic | Easy |
Festival | Multiple languages | Moderate, synthetic | Moderate |
Mozilla TTS | English, others | High-quality, natural | Moderate to Complex |
Creating Custom Voice Profiles and Adjusting Pitch and Speed
Customizing voice profiles is essential for tailoring the speech synthesis system to match a desired tone, accent, or personality. By adjusting various parameters, the synthesized voice can become more suitable for specific use cases, whether it's for virtual assistants, audiobook narration, or accessibility tools. One of the most important aspects is ensuring that the voice sounds natural and fits the intended purpose, making the listener feel more engaged with the content.
Key features such as pitch and speed have a significant impact on the overall quality of the speech output. These parameters are adjustable to create a personalized and dynamic auditory experience. By modifying pitch, you can control the height of the voice, and speed adjustments allow you to change how fast or slow the speech is delivered. Both aspects can be altered to create a unique voice profile, enhancing the user experience.
Adjusting Pitch
- Low Pitch: Produces a deeper voice, which may be more suitable for authoritative or calming effects.
- High Pitch: Creates a lighter, more energetic voice, often used for friendly or enthusiastic tones.
- Dynamic Pitch: A combination of high and low pitch variations throughout the speech, adding natural fluctuation and expression.
Speed Control
- Faster Speed: Suitable for scenarios where information needs to be delivered quickly, such as in news readers or virtual assistants.
- Slower Speed: Ideal for scenarios requiring clarity and focus, such as tutorials or accessibility tools for visually impaired users.
- Variable Speed: Changing the rate dynamically during speech to emphasize certain parts of a sentence or to reflect emotional tone.
Table of Common Voice Settings
Parameter | Setting Example | Use Case |
---|---|---|
Pitch | Medium-Low | Professional tone for corporate settings |
Speed | Normal | General purpose, easy to understand |
Emotion | Warm | Customer service, supportive communication |
"A well-adjusted voice profile enhances user engagement and satisfaction, particularly in applications where the tone and pace are integral to the interaction."
Integrating Speech Synthesis with Natural Language Processing
When combining speech synthesis with natural language processing (NLP), the goal is to create systems that can both understand and generate human-like speech. The integration enhances the communication between machines and users, allowing for more natural interactions in various applications, such as virtual assistants, accessibility tools, and voice-based interfaces. NLP focuses on interpreting and processing human language, while speech synthesis converts text into spoken words. Together, these technologies enable systems to respond to spoken commands and communicate back in a way that feels more natural to users.
To successfully integrate these technologies, it is crucial to focus on several key factors. Firstly, the NLP component must accurately parse and understand the meaning of the input text. Then, the speech synthesis component must convert this text into natural-sounding speech that considers prosody, tone, and cadence. Both technologies rely heavily on machine learning algorithms and deep learning models to improve their performance and provide more accurate, context-aware responses.
Key Challenges in Integration
- Contextual Understanding: Speech synthesis systems need to incorporate the contextual meaning of the text to produce speech that sounds coherent and appropriate.
- Real-time Processing: Ensuring that the system can generate speech instantly without lag is crucial for maintaining natural communication.
- Voice Naturalness: The quality and expressiveness of the generated voice play a significant role in making the speech output more engaging and human-like.
Successful integration of speech synthesis and NLP relies on continuous improvements in machine learning models, allowing the system to better mimic human speech patterns and adapt to various contexts.
Approaches for Effective Integration
- Deep Neural Networks: These models are particularly effective in both speech synthesis and natural language processing tasks, enabling the system to learn complex patterns in text and speech.
- Transfer Learning: This technique allows models trained on large datasets in one domain (e.g., speech) to be applied to another (e.g., NLP), improving overall system performance.
- End-to-End Systems: Rather than using separate components for text analysis and speech generation, end-to-end models process input and output within a unified framework, enhancing efficiency.
System Components and Workflow
Component | Function |
---|---|
Text Preprocessing | Transforms raw text into a structured format for analysis (e.g., tokenization, sentence segmentation). |
NLP Model | Analyzes the meaning and context of the input text (e.g., named entity recognition, sentiment analysis). |
Speech Synthesis Engine | Generates spoken output from processed text, incorporating prosody, pitch, and speed. |
Optimizing Your Speech Synthesizer for Different Platforms
Creating a speech synthesizer involves more than just converting text into speech. To ensure it performs effectively across various platforms, you must tailor the synthesizer to meet the specific requirements and constraints of each platform. This includes adapting the code for different hardware capabilities, optimizing performance, and ensuring compatibility with various operating systems and devices.
Different platforms may have unique resource constraints, such as processing power, memory, and network bandwidth. It's crucial to optimize the speech synthesis engine to provide a seamless user experience across mobile devices, desktops, and embedded systems. Below are key optimization techniques and considerations for improving the efficiency and performance of your speech synthesizer.
Key Optimization Techniques
- Platform-Specific APIs: Utilize platform-specific speech APIs, such as Android's TextToSpeech or Apple's Speech Framework, for better integration and performance.
- Memory Management: Optimize memory usage by reducing the size of speech synthesis models and using techniques like dynamic loading and unloading of resources based on platform constraints.
- Network Efficiency: For cloud-based synthesizers, minimize the amount of data transferred by compressing audio files and using low-latency protocols.
- Device-Specific Tuning: Adjust speech rate, pitch, and volume based on the capabilities of the device's speaker system.
Platform-Specific Considerations
Platform | Optimization Focus |
---|---|
Mobile | Battery usage, processing power, and network data consumption. |
Desktop | Processing speed, multi-threading capabilities, and high-quality audio output. |
Embedded Systems | Memory limitations, real-time performance, and power efficiency. |
Tip: Always conduct extensive testing across multiple platforms to ensure your speech synthesizer adapts smoothly to different environments and devices.
Testing and Troubleshooting Speech Synthesis Problems
Once the speech synthesis system is implemented, it's crucial to test its functionality to ensure it delivers accurate and high-quality speech output. Identifying common issues early helps in refining the system for better performance. Thorough testing can address problems such as mispronunciations, unnatural pauses, or incorrect intonation.
During the troubleshooting phase, developers should systematically check different aspects of the speech synthesis process, from phoneme generation to output clarity. Addressing issues promptly will ensure a more reliable user experience. Below are some common problems encountered in speech synthesis systems and their potential solutions.
Common Issues in Speech Synthesis and Solutions
- Mispronunciations: This occurs when the synthesizer misinterprets a word or string of characters. It can happen if the phoneme-to-sound mapping is incorrect.
- Speech Rate Issues: The voice may sound too fast or too slow. This can be adjusted by fine-tuning the system's speech rate parameters.
- Unnatural Pauses: Excessive or insufficient pauses between words can disrupt speech flow. Checking the punctuation handling and pause logic in the system can resolve this.
Steps to Identify and Fix Problems
- Test the speech output with a variety of phrases and sentences to spot specific errors.
- Check the configuration settings, such as voice model, rate, pitch, and volume.
- Ensure the system properly handles special characters and different languages if applicable.
- Analyze the logs to identify any warnings or errors that could point to a source of the issue.
Key Considerations
Testing should always cover edge cases, such as words with complex pronunciation, homophones, and abbreviations. These often cause unexpected errors in speech synthesis.
Example: Phoneme Mapping Table
Word | Expected Phoneme Output | Actual Phoneme Output |
---|---|---|
Example | /ɪɡˈzæmpəl/ | /ɪɡˈzæmpl/ |
Through | /θruː/ | /θrʊ/ |