Azure Speech to Text Rest Api Example
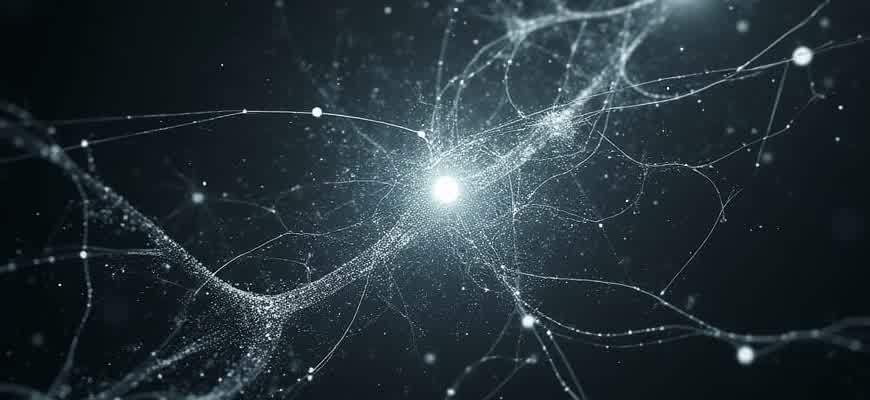
Azure Speech to Text API enables converting spoken language into text. This service can be integrated into various applications for transcription, voice recognition, and real-time processing. Below is a basic example of how to interact with the API using REST calls.
To use the Azure Speech API, follow these steps:
- Get an Azure subscription and create a Speech resource in the Azure portal.
- Obtain the subscription key and region endpoint for your Speech service.
- Make an HTTP POST request to the API with the appropriate headers and audio data.
Request Format:
Header | Value |
---|---|
Ocp-Apim-Subscription-Key | Your_Subscription_Key |
Content-Type | audio/wav |
Authorization | Bearer Access_Token |
Important: Ensure that the audio file format is supported by the API. The most commonly used format is WAV, but other formats like MP3 can also be used depending on the configuration.
In the next section, we'll break down how to send an actual audio file to the service and handle the response.
Azure Speech to Text API Example: A Practical Guide
Azure's Speech to Text API allows developers to transcribe audio files into text with high accuracy. The API supports various audio formats and languages, making it an ideal solution for transcribing voice commands, customer service calls, or converting meetings into written form. This guide provides an overview of how to interact with the API and integrate it into your applications.
In this example, we'll demonstrate how to send an audio file to the Azure Speech to Text service and receive the transcribed text. The process involves setting up the Azure account, obtaining API keys, and making REST API calls to the Azure endpoint.
1. Setting Up Azure Speech to Text API
Before using the Speech to Text API, you need to create a resource in the Azure portal. Follow these steps:
- Create an Azure account if you don't already have one.
- Navigate to the Azure portal and search for the "Speech" service.
- Click "Create" and configure the resource, selecting the region and subscription plan.
- Once the resource is created, go to the "Keys and Endpoint" section to get your API key and endpoint URL.
2. Sending Audio for Transcription
Once you have your API key, you can start making requests to the REST API. The following example demonstrates how to send an audio file for transcription.
Important: Ensure the audio file format is supported by the API (e.g., WAV, MP3).
POST https://.api.cognitive.microsoft.com/speechtotext/v3.0/transcriptions Content-Type: application/json Ocp-Apim-Subscription-Key: { "audio_url": " ", "language": "en-US" }
3. Handling Responses
The API returns a JSON response with the status of the transcription job. Here’s an example of the response format:
{ "status": "succeeded", "createdDateTime": "2025-04-10T10:00:00Z", "lastUpdatedDateTime": "2025-04-10T10:05:00Z", "results": [ { "id": "1", "text": "Hello, how can I assist you today?" } ] }
4. Example Response Table
Field | Description |
---|---|
status | The current status of the transcription process. |
createdDateTime | The timestamp when the transcription job was created. |
results | A list of transcribed text results from the audio file. |
5. Conclusion
With Azure's Speech to Text API, converting audio to text becomes straightforward. By following the steps outlined in this guide, you can quickly integrate speech recognition into your applications, making them more interactive and efficient.
Setting Up Azure Speech to Text API: Step-by-Step
To integrate the Azure Speech to Text API into your application, the first step is to set up an Azure account and create a Speech resource. Once the Speech resource is created, you can access the API by obtaining your unique API key and endpoint. Below, we will walk through the steps to configure everything correctly and start using the service for converting audio to text.
Follow these steps to set up the Azure Speech to Text API and prepare it for use in your project. This guide assumes you already have an active Azure account.
Step 1: Create a Speech Resource on Azure
- Log in to the Azure Portal (portal.azure.com).
- In the left-hand menu, click on Create a resource.
- Search for "Speech" and select Speech under the "AI + Machine Learning" category.
- Click on Create, and fill in the necessary details (subscription, resource group, region, etc.).
- Click Review + Create to create the resource.
Step 2: Retrieve API Key and Endpoint
Once your Speech resource is set up, you need to obtain the API key and endpoint to authenticate requests. Follow these instructions:
- Navigate to your newly created Speech resource in the Azure Portal.
- In the left-hand menu, select Keys and Endpoint.
- Copy the API Key and Endpoint values.
Important: The API key and endpoint are required for authentication when making requests to the Speech to Text API. Keep these credentials secure and do not expose them in public code repositories.
Step 3: Configure Your Application
Now that you have the credentials, it's time to set up your application to use the API. Below is a sample code snippet for integrating the Speech to Text API with your app using Python.
import requests # Define the API endpoint and key endpoint = "your_endpoint_url" api_key = "your_api_key" # Configure the audio file to be transcribed audio_file = open("audio_file.wav", "rb") # Set the headers headers = { 'Ocp-Apim-Subscription-Key': api_key, 'Content-Type': 'audio/wav' } # Make the API request response = requests.post(endpoint, headers=headers, data=audio_file) # Print the transcribed text print(response.json())
This setup should allow you to send audio files to the Azure Speech to Text service and receive transcriptions in return. Make sure that your audio file is in the correct format (such as .wav or .mp3).
How to Authenticate Your API Requests with Azure Cognitive Services
When working with Azure Cognitive Services, it is crucial to authenticate your API requests in order to interact with its various services. Azure uses two main methods of authentication: API keys and Azure Active Directory (AAD) tokens. Each method serves different use cases, and selecting the right one is key to securely and efficiently accessing the services.
Authentication is typically achieved by passing a key or token along with your API requests. For API key authentication, you must include the subscription key in your HTTP headers. Alternatively, for token-based authentication, you need to acquire an OAuth token via Azure Active Directory.
API Key Authentication
The most straightforward method of authentication is through an API key. Here are the key steps to authenticate your requests:
- Obtain your subscription key from the Azure portal.
- Include the key in the HTTP header of your API request.
- Use the key with the appropriate endpoint for your service (e.g., Speech API endpoint).
The structure of the authentication header will look like this:
Ocp-Apim-Subscription-Key:
Note: API keys are bound to a specific Azure subscription and service region. Make sure to use the key associated with the correct resource.
OAuth Token Authentication
For applications requiring more granular control or for accessing Azure services across different resources, OAuth authentication via Azure Active Directory is the better approach. Below are the steps to acquire and use an OAuth token:
- Register your application in Azure Active Directory.
- Obtain a client ID and client secret.
- Request a token from the Azure AD token endpoint using the client credentials.
- Include the token in the Authorization header of your API requests.
The structure of the token header will look like this:
Authorization: Bearer
Important: OAuth tokens are time-sensitive. Make sure to handle token expiration and renewal in your application.
Summary of Authentication Methods
Method | Authentication Type | Use Case |
---|---|---|
API Key | Simple Key-Based Authentication | Best for quick integration and single-tenant applications. |
OAuth Token | Token-Based Authentication | Recommended for multi-tenant or enterprise-level applications requiring advanced security. |
Sending Audio Data for Transcription via the REST API
To transcribe audio using Azure's Speech to Text service, you need to send the audio data to the API in a specific format. This can be done by making an HTTP request to the API endpoint, attaching the necessary headers, and providing the audio content. The process involves preparing the audio file, setting the correct request parameters, and understanding how to handle the response. This ensures that the audio is accurately processed and converted to text.
There are several ways to send audio data depending on the audio format and method you prefer. Below, we'll cover the key steps to effectively send your audio file via the REST API for transcription.
Key Steps to Send Audio Data
- Prepare the Audio File: Ensure your audio file is in a supported format (e.g., WAV, MP3, or Ogg). You will need to convert the audio to the required encoding if it is in a different format.
- Set the Request Headers: Include necessary headers such as 'Content-Type' and 'Authorization'. The 'Authorization' header should contain your Azure subscription key.
- Send the Audio Data: The audio file should be sent as part of the HTTP request body, either as a direct upload or through a URL pointing to the file.
- Handle the Response: The API will return a response with a URL to fetch the transcription result once the process is complete.
Request Example
POST https://.api.cognitive.microsoft.com/speech/v1.0/recognize Content-Type: audio/wav Authorization: Bearer { "audio": " " }
Important Considerations
Ensure that the audio is of good quality, as poor audio can affect transcription accuracy. It is also essential to follow the correct encoding formats supported by Azure’s Speech API to avoid errors.
Audio File Upload Methods
- Direct Upload: Send the audio file as part of the HTTP request body.
- URL Upload: Provide a URL where the audio file is hosted, which the API can access for transcription.
Response Example
Response Code | Meaning |
---|---|
202 | Request accepted. Transcription is in progress. |
200 | Transcription completed successfully. |
400 | Bad request. Invalid parameters or audio format. |
Handling Different Audio Formats for Speech Recognition
When using the Azure Speech to Text API, it is essential to ensure that the audio file is in a format supported by the service. Different audio formats may require specific handling or conversion processes to achieve optimal transcription results. Commonly supported formats include WAV, MP3, and FLAC, but not all audio formats will work out-of-the-box with the API, especially those that are proprietary or uncompressed. Understanding how to deal with these formats is crucial for maximizing the accuracy of speech recognition.
It is important to note that Azure Speech to Text provides guidelines on the appropriate audio properties, such as sample rate, bit depth, and encoding types. Audio files that don't meet these specifications may lead to transcription errors or failed recognition attempts. Additionally, converting an unsupported format to a suitable one before sending it to the API can help maintain transcription quality.
Supported Audio Formats
- WAV (Linear Pulse Code Modulation - LPCM)
- MP3 (MPEG-1 Audio Layer 3)
- FLAC (Free Lossless Audio Codec)
- OGG (Ogg Vorbis)
- PCM (Pulse Code Modulation)
Recommended Audio Settings
- Sample Rate: 16 kHz or higher
- Channels: Mono or Stereo
- Bit Depth: 16 bits or higher
- Encoding: Linear Pulse Code Modulation (LPCM) or FLAC
Important: If your audio file is in a format not supported by Azure's Speech to Text API, consider using tools like FFmpeg for conversion. This will ensure compatibility and improve transcription accuracy.
Audio File Conversion Example
Input Format | Conversion Command |
---|---|
MP3 | ffmpeg -i input.mp3 -ar 16000 -ac 1 output.wav |
OGG | ffmpeg -i input.ogg -ar 16000 -ac 1 output.wav |
Interpreting the API Response: Key Components Explained
When working with the Azure Speech to Text API, it’s crucial to understand the structure of the response you receive after sending a request. The response includes several key elements that provide detailed information about the transcription process. Each component serves a specific purpose, helping to interpret the raw data effectively and take further action if necessary.
The API response typically consists of multiple JSON objects. Each object represents different aspects of the transcription result, such as recognized speech, confidence levels, and word-level timings. To help make sense of these elements, we’ll break down the most important components in the following sections.
Key Elements of the API Response
- Recognized Phrases: The main part of the response, containing the transcribed text from the audio.
- Confidence Scores: Each recognized phrase includes a confidence score that indicates how certain the system is about the accuracy of the transcription.
- Word-Level Timing: Some responses also provide precise timestamps for each word, useful for synchronization with video or audio.
- Audio Duration: The time duration of the processed audio clip, usually provided in seconds.
Example of API Response Structure
{ "RecognitionStatus": "Success", "DisplayText": "Hello, how are you?", "Offset": 0, "Duration": 5000, "NBest": [ { "Confidence": 0.98, "DisplayText": "Hello, how are you?", "Words": [ { "Word": "Hello", "StartTime": "0.000s", "EndTime": "0.800s" }, { "Word": "how", "StartTime": "0.800s", "EndTime": "1.200s" }, { "Word": "are", "StartTime": "1.200s", "EndTime": "1.600s" }, { "Word": "you", "StartTime": "1.600s", "EndTime": "2.000s" } ] } ] }
Detailed Breakdown of the Response
- Recognition Status: Indicates whether the transcription was successful or failed.
- DisplayText: The final transcribed text based on the audio input.
- Offset: The time offset, showing where the transcription started in the audio file.
- Duration: The length of the audio clip being processed.
- NBest: A list of possible transcriptions ranked by confidence score, with the highest probability transcription listed first.
The "Confidence" score is a measure of the likelihood that the system has correctly transcribed the audio. A higher score indicates a more accurate transcription.
Word-Level Information in the Response
Word | Start Time | End Time |
---|---|---|
Hello | 0.000s | 0.800s |
how | 0.800s | 1.200s |
are | 1.200s | 1.600s |
you | 1.600s | 2.000s |
Error Handling and Troubleshooting in Azure Speech API
When working with the Azure Speech API, users might encounter various issues that can affect the transcription process. These errors can range from invalid requests to service-related problems. Understanding how to handle and debug these issues is critical for ensuring smooth interactions with the API. The following sections outline some common error types, along with troubleshooting techniques to help resolve them efficiently.
Errors are typically classified into two categories: client-side errors and server-side errors. Client-side errors may involve issues such as invalid parameters or incorrect authentication, while server-side errors could indicate problems with the API service itself. By addressing both, users can improve the robustness of their integration and minimize disruptions in functionality.
Common Errors and How to Handle Them
- Authentication Errors (401): This occurs when the API request does not have the correct authentication token. Ensure that the token is valid and has not expired.
- Bad Request (400): Often caused by malformed input data or missing required parameters. Double-check the request format and ensure all necessary fields are included.
- Quota Exceeded (429): If you exceed the allowed number of requests within a specific time frame, you will receive a rate limit error. You may need to implement retry logic or request higher limits from the Azure portal.
- Service Unavailable (503): Indicates that the API service is temporarily down. In this case, retrying the request after a brief delay often resolves the issue.
Debugging and Best Practices
- Check the Response Status Code: Always inspect the response code from the API. A code 200 indicates success, while other codes provide information on what went wrong.
- Enable Detailed Logging: Use Azure's built-in logging to capture request details and error responses. This can help pinpoint issues with request formatting or network-related problems.
- Examine the Response Body: For non-200 status codes, examine the response body for error descriptions. Azure provides error messages that often contain suggestions for resolving the issue.
- Retry on Failures: Implement retry mechanisms in your application for transient errors like 503. Azure recommends using exponential backoff strategies for retries.
Example Error Responses
Status Code | Error Type | Description |
---|---|---|
401 | Authentication Error | Invalid or expired API key. Ensure correct authentication details are provided. |
400 | Bad Request | Incorrect or missing parameters in the request. Review the API documentation for correct input. |
429 | Quota Exceeded | Too many requests in a short period. Implement rate limiting or request increased quota. |
503 | Service Unavailable | The API service is temporarily unavailable. Retry after a delay. |
Tip: Always use appropriate error handling strategies, including timeouts and retries, to ensure your application can recover gracefully from transient issues.
Integrating Speech Recognition API into Web or Mobile Applications
Integrating a speech recognition service into your application provides an effective way for users to interact with the platform through voice commands. By utilizing a Speech-to-Text API, developers can convert spoken language into written text, enabling features like transcription, voice search, and voice-controlled commands. This process can be easily implemented into both web and mobile applications using available REST APIs, making it highly versatile for various platforms.
Whether you are developing a mobile app for Android or iOS or a web-based application, integrating speech recognition is a straightforward task that allows you to enhance user experience and accessibility. The Azure Speech-to-Text API offers a flexible and scalable solution for these integrations, providing robust features like real-time transcription, multiple language support, and speaker identification.
Steps to Integrate the Speech-to-Text API
- Obtain API Key: To start, sign up for the Azure service and retrieve the API key from your Azure portal. This key will authenticate your application with the API.
- Set Up Endpoint: Choose the appropriate endpoint for your region from the Azure Speech-to-Text API documentation and configure your application to send requests to this endpoint.
- Prepare Audio Input: Ensure the audio input meets the required format for processing, typically PCM or WAV, with a sample rate of 16 kHz or higher.
- Make API Request: Use HTTP POST requests to send the audio data to the API endpoint, and specify the language model you want to use for transcription.
- Handle Response: Once the transcription process is complete, process the returned text data in your application for further use (e.g., display, analysis, etc.).
Important: Ensure the audio quality is high for better transcription accuracy. Noisy environments or poor-quality recordings can negatively affect the API's performance.
Example Integration: Web Application
Step | Action |
---|---|
1 | Set up your Azure subscription and get the API key. |
2 | Use JavaScript to capture audio from the user's microphone. |
3 | Send the audio data to the API endpoint via HTTP POST request. |
4 | Receive transcribed text and process it in your application. |
Tip: Use web technologies like WebRTC or MediaRecorder API for capturing and streaming audio from the user's device.
Integrating with Mobile Applications
- For Android: Use the Azure SDK for Android or build a custom HTTP request to interface with the Speech-to-Text API.
- For iOS: Utilize Swift or Objective-C with Alamofire to make HTTP requests to the API and process the audio input.
- Handle authentication and security by storing the API key securely within your mobile app's environment.
Optimizing API Usage for Cost-Effective Speech Transcriptions
When using speech-to-text services, optimizing API calls can significantly reduce costs without compromising the quality of transcriptions. One of the most effective ways to achieve cost savings is by managing the frequency and duration of API requests. This approach ensures that only necessary transcriptions are performed, minimizing unnecessary processing charges. Another key strategy involves batching requests to increase efficiency and reduce the number of transactions made to the API.
Understanding the pricing model of the speech-to-text API is crucial. This allows users to choose the most suitable features and configurations based on their needs. By adjusting settings such as the type of transcription (real-time vs. batch), or the inclusion of additional processing features like speaker identification, users can tailor their usage for optimal cost efficiency.
Key Strategies for Cost Efficiency
- Batch Processing: Group multiple audio files into a single request to save on API call costs.
- Real-Time vs. Batch Transcription: Choose batch transcription for longer audio or when real-time transcription is not necessary.
- Audio Quality Optimization: Ensure that the audio files uploaded are of high quality to reduce errors and the need for re-transcription.
- Use of Multiple Language Models: Select the appropriate language model to avoid unnecessary costs for languages that are not required.
Cost Optimization by Usage Patterns
- Track and analyze usage regularly to identify areas where API calls can be reduced or optimized.
- Limit the transcription of non-essential audio to avoid additional processing fees.
- Consider pre-processing audio to improve clarity, which might reduce the number of API calls needed.
Important: Always check for the latest pricing information and any available discounts or free tiers provided by the API service to maximize cost savings.
Example of API Usage Cost Breakdown
Service | Cost per Minute | Additional Features |
---|---|---|
Real-Time Transcription | $0.02 | Speaker identification, punctuation |
Batch Transcription | $0.01 | Basic transcription, no additional features |
Audio Pre-Processing | Free | Improves accuracy, reduces reprocessing |