Text to Speech Api for Python
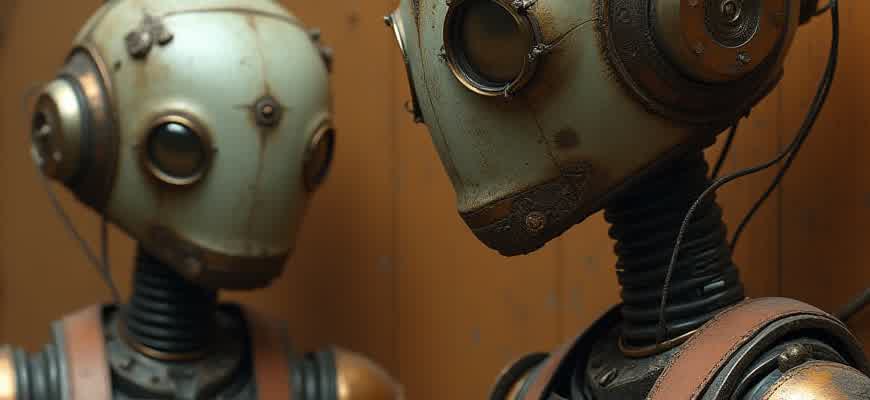
Python developers can integrate text-to-speech functionality into their applications using a variety of APIs. These APIs enable converting written text into natural-sounding audio, enhancing user interaction and accessibility. Here, we explore the process and options for implementing TTS features in Python-based projects.
To get started, developers need to choose the right API based on factors such as language support, voice quality, and ease of integration. Below are the steps to begin using a typical text-to-speech API in Python:
- Install the necessary Python libraries or SDKs.
- Obtain an API key from the service provider.
- Set up the API client in your Python environment.
- Send text data to the API and receive an audio file in return.
Important: Some APIs may require you to set specific parameters like language, voice gender, and audio format when making requests.
Here is a simple implementation using a popular Python package:
import pyttsx3 engine = pyttsx3.init() engine.say("Hello, welcome to the Text-to-Speech API tutorial.") engine.runAndWait()
Different APIs also offer various pricing models and additional features such as real-time streaming, support for multiple voices, and more. The following table compares some popular TTS providers:
Provider | Voice Options | Pricing | Additional Features |
---|---|---|---|
Google Cloud Text-to-Speech | Multiple languages, Male/Female voices | Free tier + pay-as-you-go | Real-time synthesis, SSML support |
AWS Polly | Various languages, Neural voices | Free tier + pay-as-you-go | Advanced voice modulation, Multiple formats |
Microsoft Azure TTS | Custom voices, Neural voices | Free tier + pay-as-you-go | Speech synthesis markup language (SSML) |
Text to Speech API for Python: A Practical Guide
Converting text into speech has become an essential tool in many applications, such as voice assistants, accessibility tools, and automated systems. Python, being a versatile programming language, offers various libraries and APIs that enable text-to-speech (TTS) conversion. Using a Text to Speech API, developers can integrate natural-sounding voice synthesis into their Python projects with minimal effort.
This guide will walk you through the process of using a Text to Speech API in Python. We'll explore available libraries, provide code examples, and highlight key considerations for choosing the right API for your needs. By the end, you’ll be able to implement TTS functionality effectively in your projects.
Choosing the Right Text to Speech API
When selecting a Text to Speech API, it's important to consider factors such as language support, voice quality, ease of integration, and pricing. Below are some popular TTS APIs for Python:
- Google Text-to-Speech: Offers high-quality, natural-sounding voices with support for multiple languages and accents.
- Amazon Polly: Provides lifelike voices and is highly scalable, ideal for enterprise applications.
- IBM Watson Text to Speech: Delivers high-quality audio and supports various customization options for voice tone and style.
Implementing TTS in Python
To integrate text-to-speech functionality in Python, follow these steps:
- Choose and sign up for an API service.
- Install the corresponding Python library (e.g., gTTS, boto3 for AWS Polly, or ibm-watson).
- Use the API's methods to convert text into speech.
Note: Always check the documentation of your chosen API for any specific requirements, such as API keys or setup steps.
Example Code Snippet
Here’s a basic example of how to use the gTTS (Google Text-to-Speech) library in Python:
from gtts import gTTS import os # Input text text = "Hello, this is a test of the Text to Speech API." # Language selection language = 'en' # Create TTS object tts = gTTS(text=text, lang=language, slow=False) # Save the audio file tts.save("test.mp3") # Play the audio os.system("mpg321 test.mp3")
This simple example converts text into speech and saves the audio as an MP3 file. You can experiment with different languages and voices based on the API’s capabilities.
Comparison of Popular Text-to-Speech APIs
Feature | Google TTS | Amazon Polly | IBM Watson |
---|---|---|---|
Languages Supported | Multiple | Multiple | Multiple |
Voice Quality | High | Very High | High |
Customization Options | Limited | Extensive | Moderate |
Pricing | Free Tier | Pay-as-you-go | Pay-as-you-go |
Choosing the right API depends on your specific requirements, including voice quality, customization needs, and budget. Evaluate the features that are most important for your project to ensure the best results.
Setting Up the Text-to-Speech Service in Python
To integrate Text-to-Speech (TTS) functionality into your Python project, it’s important to choose a reliable API and configure it correctly. The most common TTS services include Google Text-to-Speech, IBM Watson, and Amazon Polly. Each of these APIs provides unique features and support for multiple languages and voices. The setup process typically involves creating an account, obtaining an API key, and installing the necessary Python libraries.
In this guide, we will walk you through the basic steps of setting up a TTS API in Python using Google’s TTS API as an example. Ensure that you have Python installed on your system before beginning the installation process. You will also need to install the required libraries using Python's package manager, pip, and make some configuration adjustments to authenticate your API access.
Steps to Set Up the API
- Sign up for a Google Cloud account if you haven’t already and enable the Text-to-Speech API in the Google Cloud Console.
- Generate an API key from the Google Cloud Console and securely store it in your project folder.
- Install the required Python library using pip:
pip install google-cloud-texttospeech
- Set up authentication by setting the environment variable for your API key:
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/api-key.json"
Sample Code to Test the Setup
from google.cloud import texttospeech
# Initialize the client
client = texttospeech.TextToSpeechClient()
# Define the text input
synthesis_input = texttospeech.SynthesisInput(text="Hello, how are you today?")
# Select the voice
voice = texttospeech.VoiceSelectionParams(
language_code="en-US",
ssml_gender=texttospeech.SsmlVoiceGender.FEMALE
)
# Select the audio encoding
audio_config = texttospeech.AudioConfig(
audio_encoding=texttospeech.AudioEncoding.MP3
)
# Perform the TTS request
response = client.synthesize_speech(
input=synthesis_input,
voice=voice,
audio_config=audio_config
)
# Save the audio file
with open("output.mp3", "wb") as out:
out.write(response.audio_content)
Important: Make sure to keep your API key safe. If it is exposed, others may use your account, potentially leading to unexpected costs.
Common Errors and Troubleshooting
If you encounter issues during the setup process, check the following points:
- Ensure that your API key is correctly referenced in your environment variables.
- Verify that the required library was successfully installed.
- Check for typos or incorrect language codes when specifying the voice parameters.
Alternative Libraries
If you prefer an open-source solution, consider using the pyttsx3 library, which supports multiple TTS engines and works offline.
Library | Offline Support | API Key Required |
---|---|---|
Google TTS | No | Yes |
pyttsx3 | Yes | No |
IBM Watson | No | Yes |
How to Convert Text to Speech with Python Code
Text-to-speech (TTS) conversion is a valuable feature in many modern applications, enabling computers to "speak" out text in a human-like manner. Python provides various libraries that allow developers to easily integrate TTS functionality into their projects. One of the most commonly used libraries is pyttsx3, which works offline and supports multiple platforms such as Windows, macOS, and Linux.
In this guide, we will show you how to use pyttsx3 to convert written text into speech. The process is simple and involves installing the necessary library, configuring the speech engine, and running the Python script. Below, you will find the necessary steps to follow in order to get started with TTS in Python.
Step-by-Step Instructions
- Install the pyttsx3 library: Use the following command to install pyttsx3 via pip:
pip install pyttsx3
- Initialize the speech engine: Import the library and set up the speech engine in your Python script:
import pyttsx3
engine = pyttsx3.init()
- Set the speech properties: You can modify the rate of speech, volume, and voice properties:
engine.setProperty('rate', 150) # Speed of speech
engine.setProperty('volume', 1) # Volume level (0.0 to 1.0)
- Convert text to speech: Pass the text you want to convert into speech:
engine.say("Hello, this is an example of text to speech conversion.")
engine.runAndWait()
Code Example
import pyttsx3 engine = pyttsx3.init() engine.setProperty('rate', 150) engine.setProperty('volume', 1) engine.say("Welcome to the world of Text-to-Speech in Python!") engine.runAndWait()
Important Considerations
Ensure that you have the necessary audio drivers installed on your system for the TTS engine to function properly. Additionally, depending on your operating system, you might need to adjust certain configurations for the speech synthesis to work optimally.
Voice Customization Options
Property | Description |
---|---|
Rate | Controls the speed of the speech (words per minute). |
Volume | Adjusts the loudness of the speech (range: 0.0 to 1.0). |
Voice | Allows you to change the voice (male/female). You can choose from available system voices. |
Choosing the Right Voice for Your Application
When implementing text-to-speech functionality in your application, selecting the appropriate voice is critical for creating an engaging and seamless user experience. The choice of voice can greatly affect how users perceive the application, its usability, and its overall effectiveness. There are various factors to consider, from the tone and clarity of the voice to the gender and accent of the speaker.
One of the most important decisions is determining the type of voice that fits the context of your application. Is it for a customer support bot, a navigation system, or an educational tool? Each scenario demands different voice qualities. For example, a conversational and friendly tone might work best for a virtual assistant, while a neutral, clear voice may be more appropriate for instructions or alerts.
Factors to Consider
- Purpose: Define the function of your application. A voice for an e-learning app may need to be engaging and clear, while a voice for a product demo should be professional.
- Gender: Some applications perform better with male or female voices depending on user preferences or the nature of the content.
- Accent: The regional accent of the voice can significantly impact the user's understanding. For global applications, you may need to offer multiple accent choices.
- Tone and Pitch: A pleasant tone and moderate pitch are often more effective for maintaining user attention, while a monotonous voice may cause disengagement.
Steps to Select the Right Voice
- Identify Your Audience: Who will use the application? Consider the demographic and cultural background of your user base.
- Test Different Voices: Most APIs provide a range of voices. Test these in various contexts to see which one best suits your application's needs.
- Consider Accessibility: Ensure the voice is easy to understand and accommodates different abilities, including those with hearing impairments or cognitive difficulties.
"A good voice makes all the difference between a helpful assistant and a frustrating experience."
Voice Comparison Table
Voice Type | Pros | Cons |
---|---|---|
Male | Authoritative, Clear | Can feel formal, may not appeal to all users |
Female | Friendly, Approachable | May sound too casual or informal for some applications |
Neutral | Universal, Balanced | Can lack personality or warmth |
Customizing Voice Speed and Tone with Python Text-to-Speech API
When working with Text-to-Speech (TTS) APIs in Python, one of the most important aspects to adjust is the voice characteristics, particularly the speech rate and pitch. These parameters can greatly impact the overall quality of the generated speech, allowing developers to create more natural and user-friendly audio outputs. Fine-tuning these attributes can make the voice sound more human-like or even suited to specific use cases, such as instructional material, navigation systems, or virtual assistants.
Fortunately, most TTS APIs provide built-in options for customizing speech speed and pitch. By modifying these parameters, developers can achieve the desired auditory experience. Below are some key concepts and methods for customizing these features:
Adjusting Speech Rate
Speech rate refers to how fast or slow the speech is delivered. A higher rate can make the speech sound rushed, while a lower rate can create a more deliberate tone. The rate can be set in terms of words per minute or by defining a numeric scale where values range from slow to fast.
Important: Setting a speech rate too fast may cause the words to blend together, while a very slow rate can make the speech sound unnatural.
- Use the parameter rate to adjust the speed in TTS API calls.
- Typical values for rate range from 0 (very slow) to 1 (normal) and up to 2 or higher (fast).
- Test various rates to find the balance that works best for your project.
Controlling Pitch
Pitch controls the perceived frequency of the voice, affecting whether the speech sounds high or low. Lower pitch settings can create a deeper voice, while a higher pitch produces a more light or energetic tone. Adjusting pitch is particularly useful for simulating different characters or emotional states.
Note: Too high a pitch can make the voice sound robotic or overly enthusiastic, while too low can make it hard to understand.
- Set the pitch parameter to modify the vocal pitch.
- Common pitch settings range from -1 (low) to 1 (high), though some APIs allow more granular adjustments.
- Experiment with pitch alongside rate to find the most natural-sounding combination.
Example of Customizing Rate and Pitch
Below is an example of how to modify these parameters using the gTTS library, a popular Python TTS API:
from gtts import gTTS tts = gTTS('Hello, this is a test message with custom speed and pitch!', lang='en', slow=False) tts.rate = 1.2 # Adjust speed to be faster tts.pitch = 0.5 # Increase the pitch slightly tts.save('test_output.mp3')
Summary of Parameters
Parameter | Description | Typical Range |
---|---|---|
Rate | Controls speech speed | 0 (slow) to 2+ (fast) |
Pitch | Controls speech frequency (high/low) | -1 (low) to 1 (high) |
Managing Multilingual Support with Text to Speech API
When working with a Text to Speech API, one of the major challenges is handling multiple languages efficiently. The ability to process various languages requires an API that supports a wide range of languages and dialects, ensuring natural speech output. Each language has unique phonetic patterns, accents, and intonations, which means that the API must be capable of adapting to these differences to produce high-quality audio.
To integrate multilingual support, it is crucial to choose an API that offers flexibility in both language selection and voice customization. Many APIs provide preset voices for common languages, but the ability to adjust voice parameters–such as pitch, speed, and tone–is important for ensuring clarity and proper pronunciation across different languages.
Important Considerations for Multilingual Support
Ensure that your Text to Speech API can handle both the language and regional variations to offer the most accurate output.
- Language Availability: Not all Text to Speech services support all languages, so check the API documentation for language compatibility.
- Dialect Handling: Some languages have regional accents or variations (e.g., British vs. American English), so it's important to verify if the API offers this level of distinction.
- Pronunciation Accuracy: Languages with complex pronunciations may require APIs that have specialized training for phonetic nuances.
How to Set Up a Multilingual Text to Speech System
- Select an API with a wide range of language support.
- Choose a voice and configure language settings according to the desired output.
- Test for pronunciation accuracy in various languages, adjusting parameters if needed.
- Implement fallback logic in case the selected language or dialect is unavailable.
Sample Languages and Their Support
Language | Supported Dialects | Voice Options |
---|---|---|
English | American, British, Australian | Male, Female, Child |
Spanish | European, Latin American | Male, Female |
French | France, Canadian | Male, Female |
Mandarin | Simplified Chinese, Traditional Chinese | Male, Female |
Error Handling and Debugging in Text to Speech API
Working with a Text to Speech (TTS) API often involves addressing various issues such as connectivity problems, incorrect input formats, or unexpected responses. Proper error handling is crucial for ensuring the robustness of applications that rely on TTS systems. Developers need to be prepared to troubleshoot and manage potential problems effectively to guarantee smooth user experiences.
Incorporating well-structured debugging techniques and error-handling mechanisms will help identify root causes of issues and ensure minimal disruption. This includes validating inputs, catching exceptions, and logging errors for later analysis.
Error Handling Strategies
When integrating a TTS API into an application, it's essential to anticipate errors and handle them gracefully. Here's a breakdown of common strategies:
- Input Validation: Ensure that all text inputs conform to expected formats, such as correct character encoding or supported language codes. Invalid inputs should trigger a clear error message.
- Exception Handling: Use try-except blocks to catch errors like connection issues, timeouts, or API quota limits. This helps prevent the application from crashing unexpectedly.
- Error Logging: Keep track of errors and unexpected behaviors through logs, making it easier to track recurring issues and fine-tune the system.
Debugging Tips
When something goes wrong, efficient debugging is key. Here are several practices to streamline the process:
- Check API Response Status: Always inspect the response from the TTS API to ensure that the request was processed correctly. A non-successful status code (e.g., 400 or 500) should be handled accordingly.
- Use Mock Data: During development, use controlled inputs to simulate various scenarios. This helps isolate specific issues, such as unexpected API behavior or faulty integration points.
- Test Connectivity: Ensure that your system has stable internet connectivity, as TTS services are often hosted online. If issues persist, check the API’s server status.
Note: Always check the API's documentation for detailed error codes and their explanations. This will save you time when diagnosing issues.
Common Errors and Solutions
Error | Possible Cause | Solution |
---|---|---|
Invalid Input Format | The text input might contain unsupported characters or formatting. | Ensure the input is properly sanitized and matches the API’s expected format. |
Connection Timeout | Network issues or slow server response times. | Implement retries with exponential backoff and check network stability. |
Quota Exceeded | The API usage limits have been exceeded. | Review API limits and plan usage accordingly or switch to a higher-tier plan. |
Optimizing Speech Synthesis API Performance for Real-Time Use
For real-time speech synthesis applications, ensuring low-latency and high-quality audio output is critical. Optimizing API performance is essential in achieving seamless user experiences, especially in environments requiring quick responses, such as voice assistants, automated customer service, or interactive gaming. To achieve this, developers must address multiple factors ranging from network latency to API response time, while also considering system resources like CPU and memory usage.
In this context, effective optimization techniques can drastically improve the speed and responsiveness of the speech synthesis process. By understanding and applying best practices for API performance tuning, developers can ensure that the system consistently meets the demands of real-time applications, even under varying load conditions.
Key Strategies for Enhancing API Efficiency
- Reduce Latency: Implement low-latency protocols and optimize network communication to reduce the delay between sending a request and receiving an audio output.
- Minimize Audio File Size: Use efficient audio encoding formats (such as MP3 or OGG) to reduce the size of the generated audio files, making them faster to transmit and load.
- Preload Frequently Used Voices: Cache the most commonly used voices or phrases locally, which reduces the need for repeated API calls for the same requests.
- Asynchronous API Calls: Use asynchronous processing methods to prevent blocking operations, allowing the system to handle multiple requests concurrently without waiting for each one to complete.
Technical Considerations
- Load Balancing: Distribute API requests across multiple servers or instances to ensure consistent performance even during traffic spikes.
- Efficient Error Handling: Implement smart error detection and retry mechanisms to ensure that failed requests do not affect the overall service quality.
- Real-time Monitoring: Set up monitoring tools to track API performance and identify bottlenecks in real time, allowing for quick corrective actions.
API Performance Benchmarks
Metric | Optimal Value | Benchmark |
---|---|---|
Latency | Under 100ms | Typically 50-80ms |
Audio File Size | Under 100KB | Varies based on content |
Request Throughput | Up to 1000 req/sec | Dependent on server capacity |
Note: Optimizing the API performance requires constant evaluation and adjustments based on real-time usage data and evolving technology standards.