Text to Speech Streaming Api
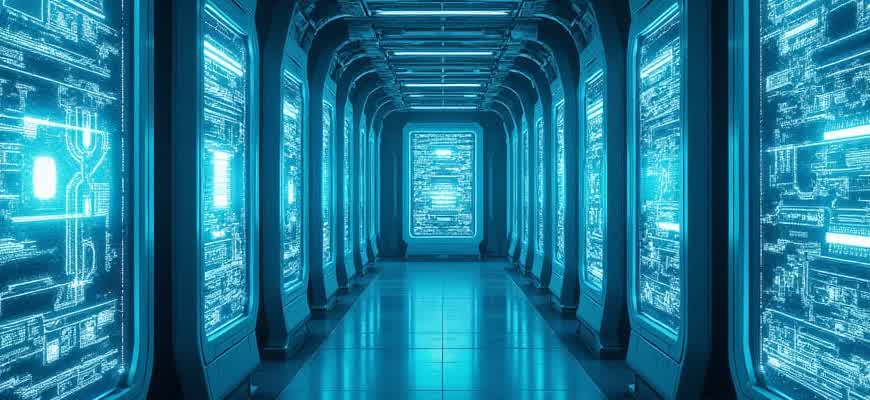
The Text-to-Speech (TTS) streaming interface enables real-time conversion of written content into audible speech. This technology is widely used across various applications, ranging from voice assistants to accessibility tools. The primary advantage of a streaming API is the ability to deliver continuous, real-time audio output without waiting for the entire text to be processed beforehand.
Key Features:
- Real-time audio generation
- Low latency processing
- Support for multiple languages and voices
- Flexible integration with different platforms and systems
Typical Use Cases:
- Voice interfaces in mobile apps
- Assistive technologies for the visually impaired
- Interactive voice response (IVR) systems
- Media and entertainment applications
The core advantage of a streaming API lies in its ability to process and deliver audio progressively, which is especially beneficial for interactive or real-time services.
Technical Details:
Feature | Description |
---|---|
Audio Format | Supports standard formats like MP3, WAV, and Ogg |
Language Support | Multiple languages, including regional accents |
Voice Types | Variety of synthetic voices, both male and female |
Text to Speech Streaming API: A Comprehensive Guide for Developers
With the growing demand for interactive applications, integrating real-time text-to-speech (TTS) functionality has become essential. A Streaming API for TTS allows developers to convert written text into spoken words, offering a smooth, continuous audio output. This guide will help you understand the core aspects of implementing TTS streaming, focusing on best practices, key considerations, and usage scenarios for developers.
Streaming APIs enable the seamless conversion of text into audio in real time, which is crucial for applications such as virtual assistants, accessibility tools, and automated customer support systems. Unlike traditional TTS, which processes entire texts before output, streaming TTS works by generating audio in chunks, providing immediate feedback with minimal latency.
Core Features of a Streaming Text to Speech API
- Low Latency: Provides almost immediate audio output, making it ideal for real-time communication systems.
- Customizable Voice Options: Many APIs offer various voice selections, including different accents, languages, and tonal variations.
- Scalability: Designed to handle high volumes of requests, suitable for large-scale applications.
- Continuous Audio Streaming: Enables a constant flow of audio, making it suitable for interactive dialogue systems.
Steps for Implementing a Streaming TTS API
- Choose a Provider: Evaluate APIs from different providers based on pricing, quality, and available features.
- Setup Authentication: Most APIs require an API key or token for secure access.
- Integrate the API: Use HTTP requests to send text to the API and receive audio data in real time.
- Handle Audio Data: Depending on the API, audio data may be provided as a stream, blob, or URL, which can be played back using media players.
"Streaming APIs offer a significant advantage over traditional TTS systems by providing a continuous flow of audio, minimizing the delay between text input and spoken output."
Key Considerations for Developers
Consideration | Details |
---|---|
Latency | Minimizing delay is crucial for real-time applications like virtual assistants and live transcription services. |
Audio Quality | Ensure that the API produces clear, natural-sounding speech without distortion or artifacts. |
Language Support | Check if the API supports the required languages and dialects for your target audience. |
Cost | Pricing models can vary based on usage, so it’s important to evaluate costs for scaling your application. |
How to Integrate a Voice Synthesis API into Your Web Application
Integrating a voice synthesis service into your web application allows you to convert text into natural-sounding speech in real time. With the growing demand for accessibility features, such integrations can help improve user experience, especially for those with visual impairments or other accessibility needs. Voice synthesis APIs typically provide simple RESTful interfaces that can be easily incorporated into your front-end or back-end code.
To integrate this functionality, you will need to choose a suitable API provider, set up the necessary authentication methods, and implement the required JavaScript to handle speech synthesis requests. Below are the key steps to follow when adding a text-to-speech feature to your web app:
Steps for Integration
- Select a Text-to-Speech API provider: Research different providers to choose the one that meets your needs in terms of features, voice options, and pricing.
- Set up an API key: Most APIs require authentication via API keys. Register with the chosen provider and obtain the necessary credentials.
- Install necessary libraries: Depending on your tech stack, you may need to install JavaScript libraries, such as axios for API calls or speechSynthesis for client-side solutions.
- Write API calls: Use JavaScript to send text to the API, and handle the response to play the generated audio on the page.
- Test for accessibility: Make sure the solution works seamlessly with assistive technologies and provides the best experience for users with different needs.
Example of API Request
const textToSpeech = async (text) => { const response = await fetch('https://api.texttospeech.com/convert', { method: 'POST', headers: { 'Content-Type': 'application/json', 'Authorization': 'Bearer your_api_key' }, body: JSON.stringify({ text: text }) }); const audioData = await response.blob(); const audioUrl = URL.createObjectURL(audioData); const audio = new Audio(audioUrl); audio.play(); };
Important Considerations
Always check the terms and conditions of the API provider. Some services may have restrictions on usage volume or limitations on voice quality based on the selected plan.
Example Integration Table
API Provider | Voice Options | Pricing | Documentation |
---|---|---|---|
Google Cloud Text-to-Speech | Multiple voices in several languages | Pay-as-you-go | Google Docs |
AWS Polly | Natural-sounding voices in various accents | Free tier available | AWS Docs |
IBM Watson | Wide range of voices and languages | Subscription-based | IBM Docs |
Optimizing Audio Quality in Real-Time with Streaming APIs
Achieving high-quality audio in real-time is essential for streaming services, especially when using text-to-speech APIs. The challenge lies in minimizing latency while maintaining clarity and natural-sounding voice output. To achieve this, several techniques must be implemented, ranging from adjusting API settings to selecting the right encoding methods.
When working with text-to-speech (TTS) systems, various factors impact the audio quality, including network bandwidth, the processing power of the client and server, and the specific configuration of the streaming API. Here are some critical considerations for optimizing audio quality during real-time streaming:
Key Strategies for Quality Enhancement
- Adaptive Bitrate Streaming: Ensures the audio stream is adjusted based on the network conditions, preventing audio dropouts.
- Low Latency Techniques: Minimizing delay between text input and speech output by choosing APIs with low-latency processing.
- Compression Algorithms: Use efficient encoding and compression schemes like Opus or AAC to balance audio quality with reduced file sizes.
Impact of API Configurations
APIs offer various settings that can be adjusted for better audio quality. Below are some factors to consider when configuring streaming APIs:
- Voice Selection: Choose a natural-sounding voice model based on the language, tone, and gender preference.
- Audio Sampling Rate: A higher sampling rate leads to better audio quality, but requires more bandwidth and processing power.
- Dynamic Adjustments: Many APIs allow you to modify speed, pitch, and volume dynamically for better control over speech output.
High-quality audio output requires continuous monitoring of network conditions and API parameters to ensure seamless delivery of speech content with minimal distortion and delay.
Table of Key Parameters for Optimizing Audio
Parameter | Impact on Audio | Recommended Setting |
---|---|---|
Bitrate | Higher bitrate = better quality, but more bandwidth needed | 160kbps or higher for optimal clarity |
Sampling Rate | Higher rates produce clearer, more natural voices | 22kHz or 44kHz for clear speech |
Compression | Balanced compression preserves audio details | Opus or AAC codec |
Best Practices for Handling Large Text-to-Speech Requests in Real-Time
When processing large text-to-speech (TTS) requests in real-time, there are several challenges that must be addressed to ensure both speed and quality. These challenges include managing large volumes of text, optimizing resource usage, and maintaining a seamless user experience. With the growing demand for interactive applications that require instant speech synthesis, it’s essential to adopt best practices that maximize efficiency and reduce latency.
Here are some proven strategies for managing large-scale TTS requests efficiently:
1. Efficient Text Segmentation
Large blocks of text should be divided into smaller segments before being processed by the TTS engine. This can significantly reduce the load on the system and improve real-time responsiveness. The following considerations are crucial:
- Segment text based on natural language processing (NLP) techniques, such as sentence or paragraph boundaries.
- Ensure that each segment can be synthesized independently, which allows parallel processing.
- Use predefined rules or heuristics to avoid breaking words or sentences in an unnatural way.
2. Optimizing Text Input for Performance
Another critical step is to optimize the way text is prepared before being sent to the TTS engine. This can help streamline the process and improve processing times:
- Preprocess the text to remove unnecessary punctuation or formatting that might not be required for speech synthesis.
- Consider language-specific optimizations, such as word contractions or phonetic adjustments, that can reduce processing complexity.
- Utilize text normalization techniques to ensure consistent input and minimize errors during synthesis.
3. Efficient Resource Management
Real-time TTS applications often need to handle multiple concurrent requests, which can strain system resources. Effective resource management is essential:
- Use load balancing strategies to distribute requests evenly across multiple servers or instances.
- Leverage caching for repeated phrases or sentences to minimize processing overhead.
- Implement dynamic scaling of resources based on demand to ensure responsiveness during peak loads.
4. Monitoring and Latency Optimization
Monitoring TTS performance in real time is key to quickly identifying and resolving bottlenecks. Regular performance checks help to optimize latency and enhance user experience:
Metric | Action |
---|---|
Latency | Optimize resource allocation and network efficiency. |
Error Rate | Monitor and refine error handling algorithms for better reliability. |
System Utilization | Ensure that hardware resources are not overtaxed by requests. |
Real-time TTS systems should be designed to scale efficiently while maintaining low latency to meet user expectations.
Customization Options for Speech Synthesis: Voices and Languages
When working with text-to-speech (TTS) streaming APIs, one of the key advantages is the ability to customize the voice and language output. These features enable developers to create a more personalized and engaging experience for users. By adjusting voice characteristics and language selection, applications can better align with their target audience's preferences or specific use cases.
Customization not only affects the linguistic aspects but also involves tuning speech parameters such as tone, pitch, and speed. This flexibility makes it possible to achieve natural-sounding speech or more robotic tones, depending on the application’s needs. Here, we will examine the customization features related to voices and languages, highlighting the importance of these tools for creating a seamless user experience.
Voice Customization Features
Voice customization options allow for changes in the tone, accent, and gender of the speaker, offering flexibility to meet different application needs. Common adjustments include:
- Gender Selection: The ability to choose between male, female, or non-binary voices.
- Accent Variations: Different accents for a language, such as American, British, or Australian English.
- Speech Rate and Pitch: Adjusting the speed and pitch of speech for better clarity or a desired tone.
- Voice Clarity and Style: Options for formal or casual speech, useful for virtual assistants or customer service bots.
Language Support and Localization
When targeting diverse regions, it's crucial to support a wide range of languages and dialects. Many TTS APIs provide a selection of languages, enabling localization for different countries. This feature is particularly important for apps aiming to serve multilingual audiences or those requiring specific regional dialects.
- Multi-Language Support: APIs typically offer a variety of languages including English, Spanish, French, Mandarin, and more.
- Dialect Variations: Some languages have specific dialects, such as British English vs. American English, which can be selected as per the target audience.
- Accent and Tone Adjustments: Accents play a vital role in language selection, as they provide an authentic regional experience.
Voice & Language Comparison Table
Feature | English (US) | Spanish (Spain) | Mandarin |
---|---|---|---|
Gender Options | Male, Female | Male, Female | Male, Female |
Accent Options | American | Castilian, Latin American | Standard, Regional |
Speech Rate Control | Yes | Yes | Yes |
Customizing the voice and language settings helps create a more natural and engaging user interaction, ensuring that the speech output matches both linguistic and regional expectations.
Enhancing Real-Time Audio Conversion in Text-to-Speech Systems
Reducing the delay in text-to-speech (TTS) applications is essential for delivering a smooth, uninterrupted user experience. Whether it’s for virtual assistants, e-learning platforms, or accessibility tools, minimizing latency ensures that users can interact with systems in a natural and efficient manner. Latency can stem from several factors, including network issues, server-side processing time, and the complexity of the speech synthesis model itself. Optimizing these components can significantly improve performance.
Key approaches to reduce latency in TTS applications involve optimizing both software and hardware processes. Efficiently handling real-time data streaming, minimizing buffer sizes, and selecting appropriate algorithms can lead to noticeable reductions in response times. Moreover, leveraging edge computing or distributed processing helps offload intensive tasks, bringing speech synthesis closer to the end user. Below are some strategies that can help achieve this goal.
Methods to Optimize Latency in TTS Systems
- Model Simplification: Using lighter models can reduce the processing time without compromising quality.
- Dynamic Buffer Management: Adjusting buffer sizes based on real-time data helps prevent delays while maintaining smooth playback.
- Edge Computing: Performing some or all of the processing locally on the user's device or nearby server helps reduce network dependency.
- Parallel Processing: Distributing tasks across multiple cores or machines can speed up the generation of audio.
Optimizing Network Efficiency
- Reducing Data Transmission: Compressing data before transmission can lower the time it takes to send audio files.
- Low-Latency Protocols: Using specialized communication protocols designed for low-latency environments improves response time.
- Network Resilience: Ensuring redundant paths and robust error correction prevents delays caused by network interruptions.
Real-Time Performance Benchmarks
Here is a comparison of common latency benchmarks for different TTS solutions:
Solution | Latency (ms) | Notes |
---|---|---|
Cloud-Based TTS | 150-500 | Depends on network and processing load. |
Edge TTS | 50-150 | Reduces network latency by processing locally. |
On-Device TTS | 20-100 | Best performance with minimal external dependencies. |
Tip: For real-time systems, aim to keep the total latency under 100ms for a seamless user experience.
Managing API Rate Limits and Scaling for High Traffic Applications
In high-traffic environments, ensuring that a text-to-speech API functions without interruptions requires close attention to rate limiting and resource management. When traffic volume increases, managing the number of API requests sent becomes critical to avoid service throttling or downtime. Failure to monitor and control API calls can lead to reaching the provider's limits and result in rejected requests or even temporary bans.
Scaling the backend to handle a large volume of simultaneous API requests without compromising on performance is essential. Leveraging smart traffic management techniques and API usage strategies can significantly improve an application’s stability and responsiveness, ensuring consistent performance even during traffic spikes.
Effective Approaches to Manage Rate Limits
- Throttling: Implement a throttling mechanism that controls the rate of API requests, ensuring it doesn't exceed the allowed number within a specified time frame.
- Exponential Backoff: If the rate limit is reached, retry the request after increasing intervals, minimizing the risk of service failure.
- Request Batching: Instead of sending numerous individual requests, group them together into batches to optimize the number of calls made.
Methods to Scale the Application for Heavy Traffic
- Load Balancing: Distribute incoming requests evenly across multiple instances to prevent overload on any single server.
- Horizontal Scaling: Increase the number of servers or containers to handle more requests in parallel, improving overall capacity.
- Caching: Cache frequent requests locally to reduce the load on the API, especially for commonly requested speech outputs.
Rate Limit Example Table
API Provider | Rate Limit | Requests per Second |
---|---|---|
Provider A | 1200 requests per hour | 20 requests/min |
Provider B | 3000 requests per hour | 50 requests/min |
Note: Monitoring and adjusting your API usage patterns are essential to avoid exceeding the rate limits and ensure smooth operation without interruptions.
Ensuring Accessibility Compliance with Text-to-Speech Streaming
When developing text-to-speech streaming services, it is essential to ensure compliance with accessibility standards to support all users, including those with disabilities. Accessibility compliance not only improves user experience but also helps avoid legal and regulatory challenges. Implementing features that allow seamless integration with assistive technologies is critical for achieving this goal. Additionally, it is important to prioritize clear and accurate voice synthesis to cater to users with various needs, such as those with hearing or visual impairments.
Text-to-speech services can provide users with audio output from written text, but developers must take extra steps to ensure their implementations meet key accessibility guidelines. Compliance with standards such as the Web Content Accessibility Guidelines (WCAG) and the Americans with Disabilities Act (ADA) is necessary. The following points outline best practices for ensuring accessibility compliance in text-to-speech streaming:
Best Practices for Accessibility
- Provide Clear and Natural Voice Output: Use high-quality, human-like voices for clarity and ease of understanding.
- Ensure Compatibility with Screen Readers: The text-to-speech API must work seamlessly with screen readers used by visually impaired users.
- Support Multilingual Capabilities: Offer speech output in multiple languages to cater to users from different linguistic backgrounds.
- Allow Customization of Speech Parameters: Provide users with control over speech rate, pitch, and volume to tailor the experience to their needs.
Compliance Standards
Standard | Description | Relevance to Text-to-Speech |
---|---|---|
WCAG 2.0 | Guidelines for making web content accessible to people with disabilities. | Ensures content is perceivable and operable by users with auditory impairments. |
ADA | U.S. law that mandates equal access to services for individuals with disabilities. | Applies to text-to-speech services to provide equal access to information. |
ARIA | Accessible Rich Internet Applications standard for enhancing accessibility of web content. | Improves accessibility of dynamic content when paired with text-to-speech APIs. |
Note: Compliance with accessibility standards is not just a regulatory requirement but also a commitment to inclusivity, ensuring that all users can benefit from the technology.