How to Use Openai Text to Speech Api
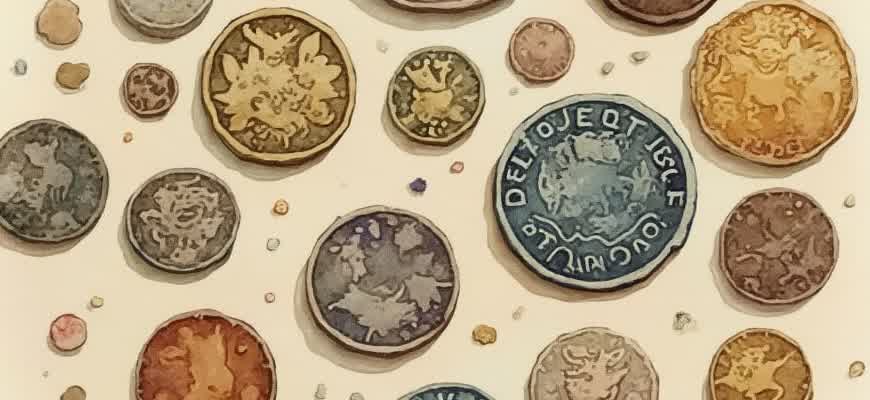
To utilize OpenAI's Text-to-Speech API, you must first set up an API key and install necessary dependencies. This guide provides a step-by-step process to help you get started with the integration. Follow these instructions to begin converting text into speech effectively.
Prerequisites:
- API key from OpenAI
- Python environment
- Familiarity with basic Python libraries
Follow the steps below to make your first text-to-speech request:
- Get your OpenAI API key from the official OpenAI platform.
- Install the required Python library using pip:
pip install openai
Ensure you have access to OpenAI's Speech API before proceeding. If you're unsure about your API limits or subscription, check your OpenAI account settings.
Now, let's look at a simple code example to convert text into speech:
Step | Action | Code Example |
---|---|---|
1 | Set up your API key | import openai openai.api_key = "your-api-key" |
2 | Make the text-to-speech request | response = openai.Audio.create( model="text-to-speech", input="Hello, this is a sample text" ) |
How to Implement OpenAI Text-to-Speech API
OpenAI's Text-to-Speech (TTS) API enables developers to convert written text into natural-sounding audio. This functionality is especially useful for creating applications that need voice output, such as virtual assistants, audiobooks, or accessibility tools. The API supports multiple languages and voices, offering flexible options for integration into various software environments.
To begin using the OpenAI Text-to-Speech API, you need to have an API key, which is provided after registering on the OpenAI platform. Once you have access, you can send a request to the API with the desired text, voice parameters, and settings. The response from the API will be an audio file in the format of your choice, typically MP3 or WAV.
Basic Steps to Use the API
- Step 1: Sign up on the OpenAI platform and obtain your API key.
- Step 2: Choose the language and voice you want to use for the speech output.
- Step 3: Send a POST request with the text and required parameters to the TTS endpoint.
- Step 4: Retrieve the audio response and integrate it into your application.
Example API Request
POST https://api.openai.com/v1/engines/text-to-speech Authorization: Bearer YOUR_API_KEY Content-Type: application/json { "text": "Hello, welcome to OpenAI's Text-to-Speech service.", "voice": "en_us_male", "audio_format": "mp3" }
Important: Always ensure that your API key is kept secure and never exposed in client-side code to prevent unauthorized access to your account.
Response Format
The response will typically be a binary audio file. Depending on the settings you choose, you can get the audio in different formats such as MP3, WAV, or OGG. Here's an example of a response:
Parameter | Description |
---|---|
audio_file | Base64 encoded audio file containing the speech output |
voice | The voice used for the speech output |
language | The language of the text-to-speech output |
Setting Up OpenAI API: A Step-by-Step Guide
Before you can start utilizing the OpenAI Text-to-Speech API, you need to set up your OpenAI API account and configure the necessary tools. The process is relatively simple but requires a few key steps to ensure everything is ready for use.
In this guide, we’ll walk through the essential steps to get you started with OpenAI's API. You'll need to create an account, generate API keys, and install any necessary libraries or dependencies. Follow this step-by-step process to ensure smooth integration.
1. Create an OpenAI Account
- Go to OpenAI's official website and sign up for an account.
- Provide the required details like your email address and password.
- After confirming your email, log into your OpenAI dashboard.
2. Generate Your API Key
- Once logged in, navigate to the API section of the dashboard.
- Click on “Create new secret key”.
- Copy your API key and store it securely, as it will be needed to authenticate your requests.
Important: Do not share your API key publicly, as it can be misused.
3. Install Dependencies
To interact with the OpenAI API, you'll need the openai Python package. Use the following command to install it:
pip install openai
4. Set Up Your Code
With the dependencies installed, it’s time to integrate the API into your project. Here's an example of how to authenticate and make a request:
import openai
openai.api_key = "your-api-key"
response = openai.Audio.create(
model="text-to-speech",
input="Hello, how can I help you today?"
)
print(response)
This code snippet will send a request to OpenAI's Text-to-Speech API and return the audio response.
5. Testing and Debugging
Test your setup to ensure that everything is working as expected. If you encounter any issues, refer to the API documentation for detailed troubleshooting steps.
Authentication and API Key Management
In order to interact with OpenAI's Text-to-Speech API, proper authentication is essential. The API relies on an API key to verify and authorize each request. This key acts as a credential, ensuring that only valid users can access the service and that usage is correctly attributed to their account. Managing these keys securely is critical to prevent unauthorized access and potential misuse of the API.
API key management involves securing, rotating, and limiting the scope of your keys to maintain best practices for security and usability. By understanding how to properly handle these keys, users can avoid common pitfalls, such as accidental exposure or overuse of resources.
Steps to Obtain Your API Key
- Create an account on the OpenAI platform.
- Navigate to the API section of your dashboard.
- Generate a new API key under the API keys tab.
- Copy the generated key to your clipboard for use in authentication.
Key Management Best Practices
- Never expose your API key in public repositories or client-side code.
- Store the key in environment variables or a secure vault on your server.
- Rotate keys periodically to limit the risks of key exposure.
- Restrict the key's permissions to specific endpoints where possible.
Important Security Tips
Always use HTTPS when sending requests to the API to ensure the key is transmitted securely.
Example of API Key Structure
Key Name | Value |
---|---|
API Key | sk-xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx |
Making Your First Text-to-Speech API Call
To get started with OpenAI's Text-to-Speech API, the first thing you need is to set up an account and obtain an API key. Once you have the API key, you're ready to integrate the service into your application. The following steps outline the process of making your first request to the Text-to-Speech API.
Once the API key is set up, you can begin by sending a request to generate speech from a text string. The process typically involves making a POST request to the API endpoint, passing the required parameters such as the text you want to convert, voice type, and language preferences.
Steps to Make the API Call
- Set up your environment: Ensure that you have a programming environment ready, such as Python with the `requests` library or Node.js with `axios`.
- Prepare the API endpoint: The endpoint for generating speech usually looks like this: `https://api.openai.com/v1/speech/generate`.
- Provide your API key: In your request headers, include the authorization token using the format `Authorization: Bearer YOUR_API_KEY`.
- Send the text data: The body of the request should include the text, along with any additional parameters such as voice selection and speech speed.
- Handle the response: Once the API processes your request, it will return an audio file, typically in a format like MP3 or WAV.
Remember: Always keep your API key secure and never share it publicly to prevent unauthorized access.
Example API Request
Below is an example of how a POST request might look in Python using the `requests` library:
import requests
url = "https://api.openai.com/v1/speech/generate"
headers = {
"Authorization": "Bearer YOUR_API_KEY",
"Content-Type": "application/json"
}
data = {
"text": "Hello, how are you today?",
"voice": "en_us_001",
"speed": 1.0
}
response = requests.post(url, json=data, headers=headers)
if response.status_code == 200:
with open("output.mp3", "wb") as f:
f.write(response.content)
In the above example, replace `YOUR_API_KEY` with your actual API key. Once the request is processed successfully, you will have a speech file named "output.mp3".
Important Considerations
- API Limits: Be aware of your usage limits to avoid hitting rate limits or additional charges.
- Voice Options: The available voices may vary depending on the region and language. Check OpenAI's documentation for a list of supported voices.
- Error Handling: Always implement error handling to catch issues such as network errors or invalid API keys.
Response Details
Parameter | Description |
---|---|
Status Code | Indicates whether the request was successful (200 OK) or failed. |
Audio File | The audio file returned by the API, which you can save and play. |
Message | Provides details about the request status or any errors encountered. |
Configuring Voice Parameters for Customization
When using the OpenAI Text-to-Speech API, it is essential to customize voice parameters to achieve the desired tone, clarity, and emotional expression. This ensures the generated speech aligns with your specific use case, whether for an application, virtual assistant, or interactive content. The API provides a variety of voice settings that can be tailored to meet your needs.
To get the most accurate and engaging voice output, developers can adjust multiple parameters like pitch, speed, volume, and voice style. These settings give you full control over how the synthesized speech sounds, creating a more personalized and interactive experience for the end user.
Voice Parameters Overview
- Pitch: Controls the highness or lowness of the voice. A higher pitch makes the voice sound more youthful, while a lower pitch provides a more serious tone.
- Speed: Defines the rate at which speech is generated. Slower speeds are ideal for clarity, while faster speeds can be useful for more dynamic interaction.
- Volume: Adjusts the loudness of the voice output. Volume levels can be fine-tuned for optimal listening experience in different environments.
- Voice Style: Some voices come with predefined styles, such as "friendly," "serious," or "emotional." These can influence how the speech sounds in terms of expressiveness and tone.
How to Set Parameters
- Select the Voice Model: Choose the appropriate voice model that supports the parameter adjustments.
- Adjust Pitch: Use the pitch parameter to either raise or lower the voice tone according to your preference.
- Control Speed: Modify the speed of the speech to suit the context. This can make a big difference in how easily the speech is understood.
- Set Volume: Adjust the volume to match the listening environment, ensuring optimal sound clarity.
- Apply Voice Style: Pick the voice style that matches your application’s tone and purpose, whether for casual interaction or formal communication.
Important: Always test the voice output after making adjustments to ensure the result aligns with your expectations. Fine-tuning these parameters can make a significant difference in the overall user experience.
Parameter Configuration Example
Parameter | Example Value | Description |
---|---|---|
Pitch | +5 | Increases the pitch for a more youthful and energetic tone. |
Speed | 1.2 | Speeds up the voice slightly for a more dynamic delivery. |
Volume | 80 | Sets the volume level to a medium-high setting for clear audibility. |
Voice Style | Friendly | Creates a warm and approachable tone in the generated speech. |
Handling API Responses and Audio Output
When working with the OpenAI Text-to-Speech API, managing the responses and ensuring proper handling of the audio output is crucial. The API provides the speech data in different formats, typically either as raw audio data or as a URL link to an audio file. Depending on the response format, your application will need to process the result accordingly, whether for direct playback, saving the audio, or other use cases.
Understanding the structure of the API's response helps ensure that the audio output is handled efficiently. The response typically includes metadata such as status codes, error messages (if any), and the audio content itself. It's important to implement error handling to gracefully deal with issues like timeouts or invalid requests, ensuring that users experience smooth audio playback or proper error notifications.
Response Format
- Status Code: Indicates whether the request was successful or if an error occurred.
- Audio Data: This can be a direct binary stream or a URL pointing to the audio file. In case of errors, this field may be empty or contain an error message.
- Metadata: Includes information such as the audio duration, voice model used, and language.
Handling Audio Output
- Check the response status code. If it's a success, proceed to extract the audio data.
- If the response contains a URL to the audio, use a library like requests (in Python) to download the file.
- For raw audio streams, use an appropriate library (e.g., pydub) to process the data and play it directly.
- Consider handling edge cases such as timeouts or incomplete responses by implementing retries or fallback options.
Note: Always validate the audio format before attempting playback. Different environments may require specific formats, such as MP3 or WAV.
Example Response Structure
Field | Description |
---|---|
status_code | HTTP status code indicating the result of the request (e.g., 200 for success). |
audio_url | URL pointing to the generated audio file, if the API provides one. |
audio_data | Raw binary audio data when the response contains audio directly. |
error_message | A string detailing the error, if the request fails. |
Error Handling and Troubleshooting Common Issues
When integrating OpenAI's Text-to-Speech API, it is essential to be prepared for potential errors that can arise during usage. This section covers how to handle common errors and offers solutions to ensure smoother operation. Understanding the root causes of issues and how to address them effectively will help minimize disruptions in your application.
By following best practices for error handling and employing troubleshooting steps, developers can quickly resolve most issues. Below, we outline common error scenarios, along with practical guidance to diagnose and fix problems that may occur while using the Text-to-Speech API.
Common Errors and Their Solutions
- Invalid API Key: Ensure the API key you’re using is correct and active. If you're receiving an authentication error, double-check the API key in your request headers.
- Quota Exceeded: If you reach the API usage limit, you’ll need to check your subscription plan. Consider upgrading or waiting until the quota resets.
- Network Issues: Ensure your internet connection is stable. In case of timeouts or connection errors, try retrying the request or checking the server status for any outages.
How to Diagnose and Fix
- Check API Response Status Codes: Always check the HTTP status code in the response to help pinpoint the error (e.g., 401 for authentication issues, 429 for rate limiting).
- Review API Documentation: Confirm you are using the correct request parameters, headers, and request structure according to the official API docs.
- Log Error Details: Implement error logging to capture detailed information for troubleshooting. This includes request payload, response, and error message from the API.
Tip: Always test API requests in a development environment before deploying them in production. This allows you to identify and resolve issues without affecting end-users.
Helpful Resources
Error Type | Possible Cause | Suggested Action |
---|---|---|
Authentication Error | Incorrect or expired API key | Verify the API key and update if necessary |
Quota Exceeded | API usage limit reached | Upgrade your plan or wait for the reset |
Request Timeout | Network connection issues | Check your network or retry the request |
Scaling API Usage for Large-Scale Applications
When integrating a text-to-speech API into a large-scale project, careful planning is essential to ensure the solution remains efficient and scalable. As the number of requests increases, both the volume of data processed and the complexity of managing these interactions grows. Thus, developers must adopt best practices to maintain performance and reliability as the application expands.
To successfully scale your use of the text-to-speech API, it’s important to consider factors such as rate limits, latency, and system architecture. Optimizing how the API interacts with your infrastructure can reduce bottlenecks and ensure smooth operation, even during peak usage times.
Key Strategies for Scaling
- Rate Limiting: Ensure that your application handles rate limits gracefully to avoid exceeding the API's maximum request thresholds. This can be achieved by implementing backoff strategies and monitoring usage patterns.
- Parallel Processing: Distribute tasks across multiple threads or servers to handle simultaneous requests more efficiently. This helps reduce latency and ensures faster processing of large volumes of requests.
- Caching Responses: For repeated requests, caching audio files or text-to-speech outputs locally or in a content delivery network (CDN) can significantly reduce API call volume and speed up response times.
Optimizing API Performance
- Use Asynchronous Calls: Implement asynchronous programming techniques to process multiple requests without blocking the main application thread, enhancing system responsiveness.
- Batch Processing: For large data sets, consider sending requests in batches to reduce overhead and better utilize available resources.
- Load Balancing: Distribute incoming traffic across multiple servers or instances to balance the load, prevent overloading a single server, and ensure availability during high traffic periods.
For highly scalable applications, employing cloud services with auto-scaling capabilities can help automatically adjust resources based on demand, ensuring consistent performance even under fluctuating loads.
Example: API Usage and Billing Model
Usage Tier | Request Limits | Pricing |
---|---|---|
Basic | 1000 requests/month | $0.02 per request |
Standard | 5000 requests/month | $0.015 per request |
Enterprise | Unlimited requests | Custom pricing |
Integrating Voice Synthesis into Web and Mobile Applications
Integrating voice synthesis functionality into web and mobile applications can enhance user experience by providing a more interactive interface. The ability to convert text into speech allows for accessibility improvements, making your application usable by individuals with visual impairments or reading difficulties. Additionally, it can help create more engaging content and improve user satisfaction by offering an alternative way to consume information.
To implement speech synthesis, developers can utilize APIs that facilitate easy integration of text-to-speech features. These APIs allow applications to convert written content into natural-sounding speech, making the process seamless and straightforward. Below are the steps to integrate this functionality into both web and mobile platforms.
Steps for Integration
- Choose a Speech API: Select an API that fits your application's needs. Popular choices include Google Cloud Text-to-Speech, Amazon Polly, and OpenAI's own API.
- API Key and Authentication: Obtain the necessary API keys for authentication. Make sure to store your keys securely.
- Integrating with Code: Implement the API within your application using JavaScript for web apps or the appropriate SDK for mobile apps (e.g., Android or iOS).
- Handling User Input: Capture text input from users or select content to be converted to speech.
- Customizing Output: Customize parameters like voice, pitch, and speed to match your app's tone and user preferences.
Example for Web Applications
For web applications, JavaScript is the primary language used to interact with text-to-speech APIs. Here's a basic example of integrating a text-to-speech feature:
const speakText = (text) => {
const utterance = new SpeechSynthesisUtterance(text);
utterance.lang = 'en-US'; // Choose the language
window.speechSynthesis.speak(utterance);
}
Considerations for Mobile Applications
For mobile applications, the integration process differs slightly based on the platform. For Android, developers can use the TextToSpeech class in the Android SDK, while iOS developers can use the AVSpeechSynthesizer class from the AVFoundation framework.
Platform | SDK/Library | API Reference |
---|---|---|
Android | TextToSpeech | TextToSpeech API |
iOS | AVSpeechSynthesizer | AVSpeechSynthesizer API |
Ensure that your text-to-speech implementation is optimized for performance, particularly in mobile apps, as this can affect battery life and overall app responsiveness.
Benefits of Voice Integration
- Improved Accessibility: Allows visually impaired users to interact with your app more effectively.
- Increased Engagement: Voice can make the user experience more immersive and interactive.
- Multitasking: Users can listen to content while performing other tasks, making your app more versatile.