Speech Synthesis Programming Guide
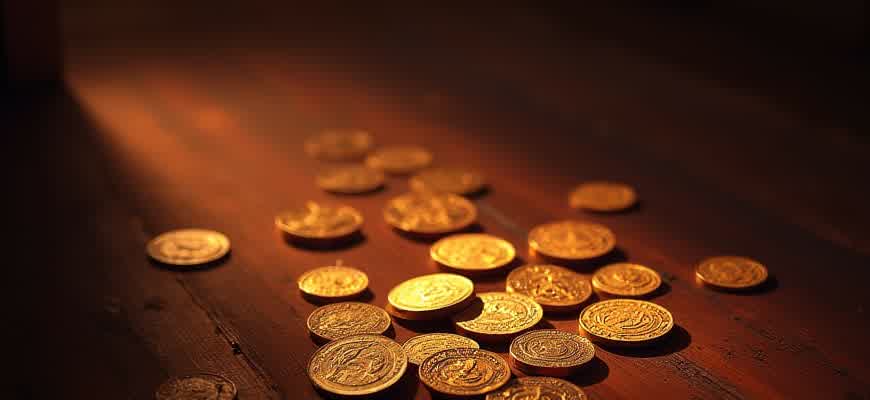
Speech synthesis is a critical aspect of modern interactive systems, allowing machines to convert text into spoken words. This guide covers the essential techniques and tools for programming speech synthesis, providing a comprehensive approach to building applications that can "speak" with human-like clarity.
Key Concepts:
- Text-to-Speech (TTS) technology
- Phonetic analysis and voice generation
- Natural language processing (NLP) integration
"Understanding the core principles of speech synthesis is vital for creating realistic and efficient TTS systems."
To begin with speech synthesis, you'll need to understand the following components:
- Input Text Processing: The process of converting raw text into a format that can be pronounced.
- Phoneme Mapping: Converting the text into phonetic symbols.
- Voice Generation: Synthesizing the phonemes into audible speech using pre-recorded voice samples or neural network-based models.
Speech Synthesis Model Comparison:
Model Type | Technology Used | Voice Quality |
---|---|---|
Rule-based | Predefined phoneme rules | Mechanical |
Concatenative | Database of recorded speech | Natural |
Neural Network-based | Deep learning algorithms | Highly natural |
Understanding Speech Synthesis Algorithms
Speech synthesis is a process that transforms text into spoken language using algorithms designed to simulate human speech. The core objective is to generate natural-sounding audio from a set of written instructions. Various approaches are used in speech synthesis, such as concatenative synthesis, formant synthesis, and parametric synthesis, each providing different levels of accuracy and naturalness in the produced speech.
Understanding how these algorithms work requires an exploration of their underlying principles, including phonetic transcription, signal processing, and prosody control. Modern systems often rely on large databases of human speech recordings, which are then manipulated to generate speech that reflects the intended message with appropriate tone, rhythm, and emphasis.
Key Concepts in Speech Synthesis Algorithms
- Phonetic Transcription: The process of converting text into a series of phonemes (distinct sound units).
- Signal Processing: Techniques used to modify and synthesize speech signals, ensuring smooth transitions and natural speech.
- Prosody Control: Adjustments to the rhythm, pitch, and intonation of speech to mimic natural human patterns.
Common Speech Synthesis Techniques
- Concatenative Synthesis: Involves stringing together pre-recorded sound segments from a speech database. While it can produce high-quality natural speech, it requires large databases and may struggle with rare words or names.
- Formant Synthesis: Generates speech by modeling the human vocal tract. This method is computationally efficient but typically sounds more robotic and less natural compared to concatenative synthesis.
- Parametric Synthesis: Uses mathematical models to generate speech based on a set of parameters. This technique can create more flexible and controllable speech output, though it might lack the richness of human-like expression.
Comparison of Speech Synthesis Methods
Technique | Quality | Flexibility | Computational Demand |
---|---|---|---|
Concatenative | High | Low | High |
Formant | Moderate | Moderate | Low |
Parametric | Moderate | High | Moderate |
Note: The choice of algorithm depends on the application. For instance, concatenative synthesis is ideal for high-quality speech in applications like audiobooks, while parametric synthesis is preferred for real-time systems with limited resources.
Setting Up a Speech Synthesis Environment in Python
To begin working with speech synthesis in Python, the first step is installing the necessary libraries and configuring your environment. Popular libraries for text-to-speech (TTS) include pyttsx3 and gTTS, both of which offer different features and functionality for generating speech from text. Each library requires specific steps to be properly set up on your system.
For this guide, we will focus on pyttsx3, as it works offline and is highly customizable. It supports various speech engines such as SAPI5 on Windows, espeak on Linux, and nsss on macOS. Follow the steps below to set up the environment and get started with speech synthesis.
Steps for Installation
- Install Python: Ensure that Python 3.x is installed on your system. You can download it from python.org.
- Install pyttsx3: To install the necessary package, use the command:
pip install pyttsx3
- Test the Installation: Create a Python script to check if the installation was successful:
import pyttsx3
engine = pyttsx3.init()
engine.say("Hello, I am ready to speak.")
engine.runAndWait()
Note: If you encounter issues with speech engines, make sure the required dependencies for the TTS engine (e.g., espeak for Linux or NSS for macOS) are installed correctly.
Basic Configuration and Usage
Once the installation is complete, you can adjust the speech synthesis settings. This includes modifying the voice rate, volume, and selecting different voices available on your system. Here’s how you can configure the speech engine:
- Set Speech Rate: Adjust how fast or slow the speech is with
engine.setProperty('rate', value)
. - Set Volume: Modify the volume of the speech using
engine.setProperty('volume', value)
. The value ranges from 0.0 to 1.0. - Set Voice: Choose between different voices available by listing them with
engine.getProperty('voices')
and selecting one.
Voice Configuration Example
Property | Command |
---|---|
Rate | engine.setProperty('rate', 150) |
Volume | engine.setProperty('volume', 0.9) |
Voice | engine.setProperty('voice', voices[1].id) |
Tip: Experiment with different voice properties (rate, volume, voice) to get the best result for your specific needs.
Choosing the Optimal Speech Synthesis API for Your Project
When selecting a speech synthesis API, it’s important to consider several factors that directly impact the performance, scalability, and user experience of your application. Different APIs offer varying degrees of control over voice characteristics, language support, and integration options. The choice depends heavily on the specific needs of your project, such as the desired voice quality, ease of integration, and budget.
To ensure you choose the best API for your application, evaluate the following aspects: feature set, pricing, platform compatibility, and customization options. Below is a guide to help navigate the decision-making process.
Key Considerations for Choosing a Speech Synthesis API
- Voice Quality: Assess the clarity and naturalness of the voices available. Some APIs use advanced deep learning models to generate lifelike voices, while others may sound more robotic.
- Language and Accent Support: Consider if the API supports all the languages and accents required for your application.
- Customization Options: Evaluate the flexibility in voice characteristics such as pitch, speed, and tone. Customizable options give you more control over the final output.
- Integration and SDK Support: Check if the API provides robust SDKs or is easy to integrate with the platform you are using (e.g., mobile apps, web, IoT devices).
- Pricing Model: Compare pricing structures–per-character, per-minute, or monthly subscriptions–and assess which fits your budget.
Comparison of Popular Speech Synthesis APIs
API | Voice Quality | Supported Languages | Customization | Pricing |
---|---|---|---|---|
Google Cloud Text-to-Speech | High (Neural Voices) | 50+ Languages | Advanced (Pitch, Speed, Volume) | Pay-as-you-go |
AWS Polly | High (Realistic) | 30+ Languages | Moderate (Voice Selection) | Pay-per-use |
Microsoft Azure Cognitive Services | Very High (Neural Voices) | 75+ Languages | Highly Customizable (SSML) | Pay-as-you-go |
Important: Make sure to thoroughly test the API before integrating it into your project to confirm its compatibility with your platform and meet the voice quality standards required for your application.
Factors to Watch Out For
- Latency: Evaluate the response time of the API, especially if real-time speech generation is essential for your application.
- Scalability: Choose an API that can handle high volumes of requests if your application requires a large number of voice outputs.
- Service Reliability: Consider the uptime and support options provided by the API vendor, as any downtime can significantly impact user experience.
Integrating Speech Synthesis into Web Applications with JavaScript
Web applications can enhance user experience by incorporating speech synthesis, allowing text to be read aloud. This feature is particularly useful for accessibility, helping users with visual impairments or reading difficulties. JavaScript provides a straightforward way to integrate text-to-speech functionality using the Web Speech API, making it accessible across modern browsers.
To begin using speech synthesis, developers need to access the SpeechSynthesis interface, which is part of the Web Speech API. This can be done by using JavaScript to control various aspects of speech output, such as voice selection, pitch, rate, and volume. The implementation process is relatively simple and can be customized to fit the specific needs of the web application.
Basic Implementation Steps
- Access the SpeechSynthesis object through speechSynthesis.
- Create a SpeechSynthesisUtterance instance, which will contain the text you want to read.
- Use speechSynthesis.speak() to start speaking the text aloud.
Here is a basic example of how to implement speech synthesis:
const utterance = new SpeechSynthesisUtterance('Hello, welcome to our website!'); speechSynthesis.speak(utterance);
Controlling Speech Properties
To make speech more dynamic, developers can adjust various properties of the SpeechSynthesisUtterance object, such as:
- rate: Controls the speed of speech (default is 1).
- pitch: Adjusts the tone of the voice (default is 1).
- volume: Sets the volume level (default is 1).
- voice: Allows selection of different voices available in the browser.
Voice Selection and Customization
Browsers often come with multiple voice options that can be accessed through the speechSynthesis.getVoices() method. Developers can use this to allow users to choose their preferred voice for speech output. The following table illustrates how to access and set voices in JavaScript:
Voice | Property |
---|---|
English (US) | speechSynthesis.getVoices()[0] |
English (UK) | speechSynthesis.getVoices()[1] |
Spanish | speechSynthesis.getVoices()[2] |
Tip: Ensure you check if voices are available in the browser before attempting to change voice properties, as availability may vary.
By incorporating speech synthesis into your web application, you can significantly improve accessibility and provide an interactive user experience. With simple API calls, developers can customize speech properties, choose different voices, and create engaging content for their users.
Optimizing Voice Quality and Naturalness in Speech Generation
Achieving high-quality and natural-sounding synthetic speech is a key challenge in the development of speech synthesis systems. It requires careful attention to multiple factors, from the selection of the voice model to the fine-tuning of speech parameters. In this process, various techniques can be applied to improve the clarity, emotional expressiveness, and overall realism of the generated voice. Ensuring that speech sounds both clear and lifelike involves addressing not only technical aspects but also linguistic and acoustic features.
Several strategies can be employed to enhance the synthesis of natural-sounding speech, with a focus on advanced modeling methods, prosody control, and optimization of signal processing. The following approaches are essential for creating a more engaging and lifelike synthetic voice.
Key Techniques for Improving Voice Quality
- Prosody Adjustment: Controlling pitch, duration, and stress patterns can significantly improve the flow and expressiveness of speech. Proper prosody makes speech sound more conversational and less robotic.
- Voice Model Enhancement: Using deep neural networks or neural vocoders to generate voice models can lead to more accurate and natural speech outputs.
- Waveform Synthesis: Leveraging advanced waveform synthesis methods like WaveNet allows for the generation of high-fidelity sound that captures the nuances of human speech.
- Speech Intelligibility: Ensuring that the speech is clear and easily understood by adjusting the speed and clarity of the voice is crucial for improving quality.
Steps to Enhance Naturalness in Speech Generation
- Training on Diverse Data: Utilizing a large and varied dataset for training ensures that the speech model can accurately replicate different voices, accents, and speech styles.
- Fine-tuning the Model: Customizing models to suit specific voice characteristics or applications helps in achieving a more natural tone and personality.
- Context-Aware Adjustments: The system should adjust intonation, pace, and emphasis based on the context of the speech to create a more dynamic output.
Important: Incorporating real-world recordings and adapting speech synthesis systems to user feedback can further refine the naturalness and quality of generated speech.
Example of Optimizing Speech Model Parameters
Parameter | Impact on Speech Quality |
---|---|
Pitch | Affects the perceived emotion and tone of the voice. |
Duration | Controls the pacing of speech, improving natural rhythm and flow. |
Volume | Ensures speech is audible while maintaining clarity. |
Expanding Speech Synthesis for Global Accessibility
To make speech synthesis universally accessible, it is essential to support multiple languages. Each language requires distinct processing of phonetic and grammatical structures, so a multilingual synthesis system must adapt to these differences. Achieving this involves integrating various language models, each tailored to the linguistic characteristics of the target audience, enabling accurate and natural speech output for speakers of different languages.
Adapting a speech synthesis system to multiple languages involves more than just translation. It requires the system to comprehend the specific sounds, rhythms, and tonal variations of each language. This section outlines the necessary steps and considerations for implementing such a system effectively.
Approaches for Effective Multilingual Synthesis
- Language-Specific Voice Models: Each language may need its own voice model, designed to reflect its unique phonetic features and speech patterns.
- Unified Framework: A synthesis system should ideally be designed to handle multiple languages simultaneously, allowing for seamless switching between them without losing quality.
- Regional Adaptation: Consider regional dialects or accents to ensure that the synthesis system sounds natural to speakers from different areas.
Steps for Developing a Multilingual System
- Collecting Linguistic Data: Gather extensive, high-quality datasets for each language, including various accents and speaking styles.
- Training Language-Specific Models: Use these datasets to train specialized models that understand the unique phonetic characteristics of each language.
- Testing and Refinement: Continuously evaluate the system's performance in each language, using feedback from native speakers to ensure the output is accurate and natural.
"Building a truly multilingual speech synthesis system involves more than just supporting multiple languages. It requires ensuring that each language is represented authentically, capturing the essence of its sound and rhythm."
Challenges in Multilingual Speech Synthesis
Challenge | Solution |
---|---|
Language-Specific Phonemes | Develop separate voice models for each language to handle unique phonemes and pronunciations. |
Accent Variations | Implement models that recognize and reproduce regional accent variations for a more localized and accurate speech output. |
Resource Constraints | Utilize data augmentation and open-source resources to enhance language models, especially for less-resourced languages. |
Identifying and Fixing Common Problems in Speech Synthesis Code
When developing speech synthesis systems, errors can occur at various stages of the code. These issues can range from incorrect voice output to performance degradation. Identifying the root causes of these problems is essential for ensuring smooth operation. Effective debugging techniques help address common problems such as poor pronunciation, delayed responses, and system crashes.
Several factors can influence the accuracy and reliability of speech synthesis. Code errors, incorrect configurations, or hardware limitations may all contribute to these issues. Below are some common problems encountered during development and how to troubleshoot them.
Common Speech Synthesis Issues
- Incorrect Pronunciation: The speech engine may not correctly interpret certain words or phrases. This can happen due to incorrect phonetic input or language setting mismatches.
- Audio Delays: Performance issues can lead to delays between text input and speech output. This is often caused by inefficient processing or improper buffer management.
- Crashes or Freezes: Incorrect handling of exceptions or memory issues can lead to program instability, resulting in crashes or freezes during synthesis.
Steps for Troubleshooting
- Check Configuration Settings: Ensure the correct language model, voice selection, and speech rate settings are applied.
- Examine Input Text: Review the input text for unhandled special characters or formatting that could cause errors in speech processing.
- Optimize Code Performance: Analyze your code for potential bottlenecks or inefficient memory usage that may lead to delays or crashes.
- Use Logging and Debugging Tools: Implement logging to track errors and identify the source of performance issues. Tools like profilers can also help diagnose bottlenecks.
Important: Regularly test the speech synthesis system with various input scenarios to catch edge cases early in the development cycle.
Helpful Debugging Tools
Tool | Description |
---|---|
Speech Synthesis Markup Language (SSML) | Used to fine-tune the prosody, pitch, and pronunciation of speech outputs. |
Profilers | Measure the performance of your speech synthesis code, highlighting areas that need optimization. |
Unit Testing | Helps ensure that the core components of the speech synthesis system work as expected under various conditions. |