C Create Array with Values
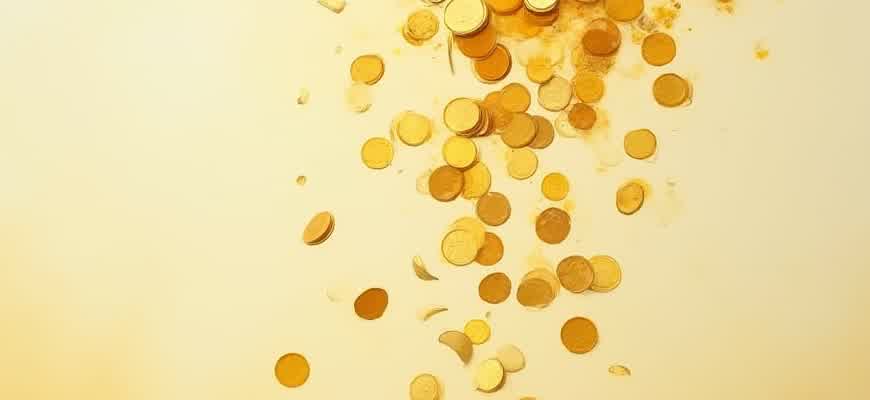
In programming, arrays are used to store multiple values in a single variable. To initialize an array with predefined elements, developers can assign values during its creation. The syntax for creating an array varies across programming languages, but the fundamental approach remains the same.
Important: Arrays can be initialized with values directly at the time of creation, allowing for immediate use in computations or data storage.
Here are the steps to create an array in a few common languages:
- In JavaScript: You can create an array by listing the values inside square brackets.
- In Python: Arrays are typically created using lists, defined by square brackets containing values.
- In Java: Arrays are initialized by specifying the type and size, followed by assignment of values.
Let’s see an example of array creation:
// JavaScript example
let numbers = [1, 2, 3, 4, 5];
Arrays can hold different data types, such as integers, strings, or even other arrays.
Language | Array Creation Syntax | Example |
---|---|---|
JavaScript | let arr = [value1, value2, ...]; | let numbers = [1, 2, 3]; |
Python | arr = [value1, value2, ...] | numbers = [1, 2, 3] |
Java | int[] arr = {value1, value2, ...}; | int[] numbers = {1, 2, 3}; |
How to Define an Array with Predefined Values in Programming
Arrays are a fundamental data structure used in various programming languages to store multiple values in a single variable. In many cases, you may need to initialize an array with specific values, either when declaring the array or after its creation. This process is essential for organizing data that needs to be accessed sequentially or in bulk.
There are several ways to create arrays with predefined values. The most common approach involves specifying the values directly within the array declaration or using methods provided by the language to populate the array with data.
Creating Arrays with Values in Different Languages
Different programming languages offer specific syntax to initialize arrays with values. Below are examples of how to define an array with values in some popular languages:
- In C: Arrays are defined by specifying the size and elements in curly braces.
- In Python: Lists (similar to arrays) are initialized directly by using square brackets.
- In JavaScript: Arrays can be created using square brackets and separating values with commas.
Example: Array Initialization Syntax
Language | Syntax |
---|---|
C | int arr[] = {1, 2, 3, 4}; |
Python | arr = [1, 2, 3, 4] |
JavaScript | let arr = [1, 2, 3, 4]; |
Note: While arrays in some languages (like Python) can grow dynamically, in languages like C, you must define the size at the time of declaration if you don't use dynamic memory allocation.
Best Practices for Initializing Arrays
- Always specify values in the correct order: Ensure the values correspond to the intended use case.
- Use meaningful variable names: This helps improve code readability and maintainability.
- Consider performance: In certain cases, initializing arrays with large amounts of data can affect performance, especially in low-level languages like C.
Step-by-Step Guide to Initializing Arrays in Your Code
Initializing an array is one of the first steps when working with data structures in programming. In C, you must declare the array size and type before using it. The initialization process allows you to define values for each element in the array, which is crucial for efficient data handling and manipulation.
There are several ways to initialize an array in C, and the method you choose depends on the specific use case. Below, you will find a step-by-step guide to initializing arrays correctly and efficiently in your programs.
Ways to Initialize Arrays
- Static Initialization – Assigning values at the time of array declaration.
- Dynamic Initialization – Assigning values to the array elements after the array has been declared.
- Partial Initialization – Providing values for some elements, with others initialized to default values.
Detailed Steps for Array Initialization
- Define the array type and size: Specify the data type (e.g., int, float) and the number of elements in the array.
- Assign values to array elements: Use either curly braces or individual assignments to fill the array.
- Check array boundaries: Always ensure you do not exceed the array’s defined size to avoid accessing invalid memory.
Important: Arrays in C are zero-indexed, meaning the first element of the array is accessed using index 0.
Example: Static Array Initialization
Declaration | Initialization |
---|---|
int arr[5]; | int arr[5] = {1, 2, 3, 4, 5}; |
Understanding Different Data Types for Array Elements
In C programming, arrays are used to store multiple values of the same data type. However, it's important to understand that arrays can hold various types of data, which influences how the data is stored and accessed. The data type chosen for an array dictates the kind of values it can store, how much memory is allocated, and how operations on those values are handled.
Choosing the correct data type for array elements is crucial for optimizing both memory usage and program performance. In C, common data types include integers, floating-point numbers, and characters, each with different characteristics. Let's explore these types in more detail.
Common Data Types for Arrays
- Integer Arrays: Store whole numbers (e.g., int). These arrays are used when you need to store numerical values without decimals.
- Float Arrays: Used for numbers that require decimals (e.g., float or double). These arrays allow precise storage of values like measurements or scientific data.
- Character Arrays: Store individual characters (e.g., char). These arrays are useful for storing text or strings in programs.
Memory Considerations
Data Type | Size (in bytes) | Range of Values |
---|---|---|
int | 4 bytes | -2,147,483,648 to 2,147,483,647 |
float | 4 bytes | 3.4e-38 to 3.4e+38 |
char | 1 byte | -128 to 127 (or 0 to 255 for unsigned char) |
Choosing the right data type for your array elements ensures efficient memory usage and faster execution. A mismatch can lead to incorrect results or wasted memory space.
How to Dynamically Populate Arrays Based on User Input
In programming, it’s common to create arrays that need to be populated dynamically based on user input. This approach allows the program to adapt to different scenarios and inputs without requiring the coder to hardcode values. User input can vary, so the array's size and content can change on the fly depending on what the user provides. This is especially useful when working with data from forms or interactive applications.
One way to achieve this in most programming languages is by using loops and conditions to prompt the user for input and then add values to the array accordingly. Below are methods and considerations for working with user-provided data.
Steps to Populate Arrays Dynamically
- Prompt the user for input: Use functions or methods to gather the required data. For example, you might ask the user to enter the number of items they want to input.
- Store the input: Create an empty array to store the user's entries. Each time the user inputs data, the array can be expanded to include the new value.
- Check input validity: Validate user input (e.g., ensure that the data entered is of the correct type) to prevent errors.
- Process the data: Once all user input is collected, you can use the array for further processing or display it.
It's crucial to handle input validation carefully. If a user enters incorrect data, your array might become inconsistent, which could lead to unexpected behavior.
Example Table for Storing User Input
Index | User Input |
---|---|
0 | Apple |
1 | Banana |
2 | Orange |
This table represents an array populated with user input. Each entry corresponds to a user-provided value stored at a specific index in the array.
Remember, the array size can grow or shrink based on the user’s input. Be prepared to handle different data types and formats depending on your application needs.
Handling Arrays with Predefined Values: A Quick Method
In many programming scenarios, you need to initialize an array with specific values. This can be done efficiently by directly assigning values to the array at the time of its creation. In C, you can use this approach to quickly populate an array without the need for loops or manual insertion. Below are some examples of how this can be achieved with simple syntax and clear steps.
When working with predefined values, there are different techniques to handle array initialization. This allows you to simplify your code and avoid unnecessary complexity. By using these methods, you can ensure that your arrays are properly set up and ready for use without extra overhead.
Simple Initialization
One straightforward way to create an array with specific values is by listing the values within curly braces. The compiler automatically determines the array's size based on the number of elements provided.
int numbers[] = {1, 2, 3, 4, 5};
This method is useful when you know the exact values you want to store. If the size of the array is not explicitly specified, it will be inferred from the number of elements listed inside the braces.
Using Loops for Initialization
If you have a set of values that follow a predictable pattern, you can use loops to initialize an array. For example, if you want to fill an array with numbers from 1 to 10, a simple loop can handle the task efficiently.
int numbers[10]; for(int i = 0; i < 10; i++) { numbers[i] = i + 1; }
Alternative Methods for Predefined Arrays
In cases where you need to initialize an array based on a condition or external input, you can use functions or macros to populate arrays with predefined values.
Note: Direct initialization with curly braces is the most efficient method for static values. Using loops or functions is suitable for more dynamic scenarios.
Example of Using a Table to Represent Predefined Values
Index | Value |
---|---|
0 | 10 |
1 | 20 |
2 | 30 |
3 | 40 |
Summary of Methods
- Curly braces: Direct initialization with predefined values.
- Loops: Ideal for dynamic or sequential value assignment.
- Functions/Macros: Suitable for more complex initialization patterns.
Working with Multidimensional Arrays: Best Practices
Multidimensional arrays are powerful tools for organizing complex data structures, particularly when dealing with grids, matrices, or tables. However, managing these arrays can quickly become complicated without a structured approach. By following best practices, you can ensure that your code is both efficient and easy to maintain.
When working with arrays of more than one dimension, it's essential to carefully consider their organization, initialization, and access methods. Below are some key points to keep in mind when dealing with multidimensional arrays in C.
Best Practices for Managing Multidimensional Arrays
- Consistent Indexing: Always ensure that the array indices are accessed in a predictable and logical manner. This reduces errors and makes the code easier to read.
- Initialization: Proper initialization is crucial to avoid uninitialized memory errors. It’s common to initialize arrays with specific values, but don’t forget to include edge cases.
- Memory Management: Be cautious when allocating memory for large multidimensional arrays, especially dynamically allocated ones. Make sure to free memory after use to prevent memory leaks.
- Access Patterns: Consider how you’ll access the array. Accessing elements row by row is often more efficient in languages like C, where memory is typically stored in a row-major order.
Tips for Working with Dynamic Multidimensional Arrays
- Use
malloc
orcalloc
to allocate memory for dynamic arrays. - Ensure that each row is separately allocated, especially when dealing with arrays of varying sizes.
- Always check for successful memory allocation to avoid dereferencing null pointers.
Important: For large data sets, consider using a more sophisticated memory management strategy, such as allocating memory in blocks, to avoid fragmentation and improve performance.
Example of a 2D Array Initialization
Row | Values |
---|---|
0 | {1, 2, 3} |
1 | {4, 5, 6} |
2 | {7, 8, 9} |
In this example, a 2D array is created with three rows and three columns. Such an array can be initialized with values in a simple format, and accessed efficiently through looping structures.
Modifying Array Values After Initialization
Once an array is initialized in C, its values can be modified using different methods. These methods allow developers to change specific elements or manipulate the entire array at once. In C, arrays are zero-indexed, meaning that the first element is accessed with an index of 0. To modify an array element, simply reference the index and assign a new value to it.
One common way to modify array values is through direct indexing, where each element is accessed by its position. Another method is using loops to iterate through the array and apply changes to multiple elements. Both techniques are essential for handling dynamic data effectively within arrays.
Modifying Individual Array Elements
To change the value of a specific element in an array, you need to specify the index and assign the new value. For example:
int arr[5] = {1, 2, 3, 4, 5}; arr[2] = 10; // Changes the third element from 3 to 10
In this case, the element at index 2 (the third element) is modified from its initial value of 3 to 10.
Modifying Arrays Using Loops
Another way to change multiple values in an array is by using loops, such as a for loop. This method allows you to iterate over the array and modify each element individually or apply some condition to decide how to change them.
for (int i = 0; i < 5; i++) { arr[i] = arr[i] * 2; // Doubles each element }
This example multiplies every element of the array by 2.
Important Considerations
Be cautious when modifying array values. Always ensure that the index you are modifying is within the bounds of the array to prevent undefined behavior or memory access errors.
Alternative Methods
In certain cases, you may need to use additional techniques like pointer arithmetic or built-in functions to modify arrays more efficiently, especially for large datasets.
Index | Old Value | New Value |
---|---|---|
0 | 1 | 2 |
1 | 2 | 4 |
2 | 3 | 6 |
3 | 4 | 8 |
4 | 5 | 10 |
Summary of Array Modification Techniques
- Direct indexing is the simplest way to change an element.
- Using loops allows for modifying multiple elements efficiently.
- Be mindful of array boundaries to avoid runtime errors.
Always validate the array size before accessing or modifying its values to avoid out-of-bounds errors.
Debugging Common Errors When Creating Arrays
When programming in C, creating arrays can sometimes lead to unexpected errors. These issues are often caused by mistakes such as incorrect indexing, memory allocation errors, or improper initialization. Understanding these common pitfalls and knowing how to debug them is crucial for any developer.
Some errors are easy to spot, while others require a deeper understanding of memory management and the array’s structure. Identifying these problems early on can save time and help prevent larger issues in the program's logic.
1. Out of Bounds Indexing
One of the most frequent issues when dealing with arrays is accessing an index outside the valid range. This occurs when the index is either negative or greater than the maximum allowed index (size-1). In C, array indices start at 0, and accessing an out-of-bounds index can result in unpredictable behavior.
Important: Always ensure that array indices stay within the bounds of the array size.
- Index less than 0
- Index greater than or equal to the array size
Example of incorrect indexing:
int arr[5]; arr[5] = 10; // Error: index 5 is out of bounds for an array of size 5
2. Uninitialized Arrays
Another common mistake is using uninitialized arrays. In C, arrays are not automatically initialized, and if you attempt to use uninitialized values, they could contain garbage data. This can lead to undefined behavior and incorrect results in your program.
Tip: Always initialize your arrays before using them to ensure that the values are valid.
- Initialize arrays when declaring them:
- Or assign values in a loop:
int arr[3] = {0};
for (int i = 0; i < 3; i++) arr[i] = 0;
3. Memory Allocation Errors
Dynamic arrays, created using malloc() or calloc(), can also cause issues if not properly allocated. If memory allocation fails, the array will not be created, and any attempt to use it will result in a segmentation fault or other runtime errors.
Error | Solution |
---|---|
Memory allocation fails | Check the return value of malloc() or calloc() for NULL. |
Memory leak | Free the memory after use with free(). |
Optimizing Memory Consumption When Initializing Large Arrays
Efficient memory usage becomes crucial when working with large arrays in C, especially when dealing with limited system resources. A common issue developers face is the high memory consumption that occurs when initializing arrays of large sizes. In such cases, understanding different approaches for managing memory can significantly enhance the performance of an application. For example, instead of allocating a huge array at once, using dynamic memory allocation can help save space when necessary.
Another strategy for optimizing memory usage is choosing the right data type. Often, using a larger data type than required can lead to wasted memory. By selecting the smallest possible type that fits the need, the memory footprint can be reduced substantially. Additionally, implementing more advanced techniques like memory pooling can help avoid fragmentation and improve overall memory management.
Ways to Reduce Memory Usage
- Use dynamic memory allocation (e.g., malloc, realloc) to allocate memory only when needed.
- Choose appropriate data types for array elements to minimize unnecessary space usage.
- Use memory pools to avoid frequent allocations and deallocations, reducing fragmentation.
- Consider using bitfields or compact data structures when storing multiple smaller values in a single element.
Techniques for Memory Allocation
- Static Allocation: Pre-allocating memory at compile time is efficient but can lead to wasted memory if not properly sized.
- Dynamic Allocation: Using malloc or calloc allows arrays to grow and shrink as needed, saving memory during runtime.
- Memory Pooling: Pooling involves pre-allocating a block of memory for reuse, which reduces overhead from frequent allocations.
Tip: Always check the return value of memory allocation functions like malloc to ensure that memory is successfully allocated before using the pointer.
Example of Efficient Memory Allocation
Method | Pros | Cons |
---|---|---|
Static Allocation | Fast access time, no overhead. | May waste memory if array size is too large. |
Dynamic Allocation | Memory is allocated as needed, reducing waste. | Overhead from allocation and deallocation. |
Memory Pooling | Reduces fragmentation, increases allocation speed. | Complex to implement, memory may be wasted if pool size is too large. |