Apple Text to Speech Api
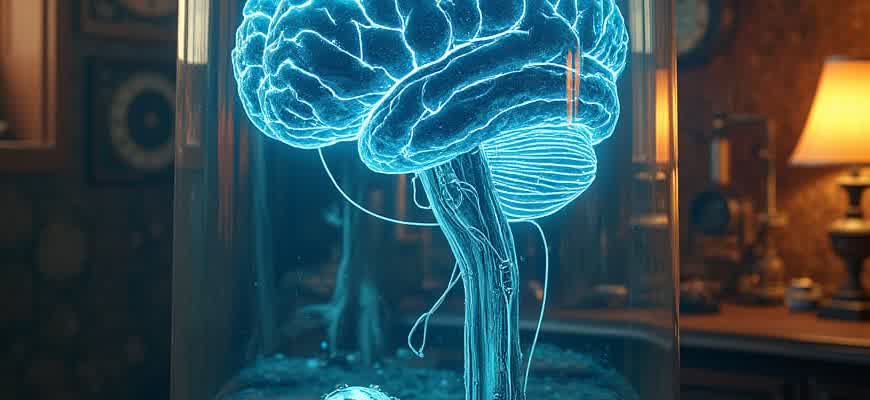
The Apple Text to Speech API offers a powerful tool for converting written text into natural-sounding speech. It integrates seamlessly into macOS and iOS applications, allowing developers to add speech synthesis capabilities with ease. This API is highly customizable, offering a variety of voices, languages, and accents. The core technology behind the API is Apple's advanced machine learning models, ensuring high-quality and lifelike speech output.
Key features of the Apple Text to Speech API:
- Multiple Voices: Choose from different voices and accents for enhanced user experience.
- Language Support: The API supports a wide range of languages and dialects.
- Real-time Processing: Converts text to speech on the fly for interactive applications.
Important information about using the Apple Text to Speech API:
This API is available as part of Apple's Speech framework, and it requires developers to have an active Apple Developer account to access the full range of features.
Example of available voices and their characteristics:
Voice | Language | Gender | Sample Rate |
---|---|---|---|
Alex | English | Male | 44.1 kHz |
Siri | English | Female | 44.1 kHz |
Daniel | English (UK) | Male | 44.1 kHz |
Integrating Apple’s Text-to-Speech API: A Practical Guide
Apple's Text-to-Speech (TTS) functionality provides developers with a powerful tool to convert written text into spoken words. This can be applied across various platforms such as iOS, macOS, and watchOS. The API is simple to use, highly customizable, and integrates smoothly with Apple’s ecosystem, making it ideal for a range of applications including accessibility features, interactive applications, and voice assistants.
In this guide, we will focus on how to effectively implement the TTS API, highlighting key steps, available options, and best practices for its use. Understanding the core components of this API and how to customize it to meet specific needs is essential for seamless integration into your app.
Step-by-Step Integration
To begin using the TTS API, follow these steps:
- Set Up Your Project: Ensure that your development environment is ready. You will need Xcode and an Apple Developer account.
- Import AVFoundation Framework: The TTS capabilities are part of the AVFoundation framework, so you must import this into your project.
- Initialize AVSpeechSynthesizer: This object is used to manage the text-to-speech conversion. You’ll create an instance of this class to start speaking text.
- Provide Text Input: Input text can be any string that you want to be read aloud. You pass it to the AVSpeechUtterance class.
- Configure Voice and Language: The API allows customization of voice types, rates, and languages. You can choose between different accents and genders.
Available Options for Customization
The Apple TTS API provides multiple settings to fine-tune the speech output:
- Voice Selection: You can choose from a variety of built-in voices available for different languages and accents.
- Speech Rate: Control the speed at which the text is read, from slow to fast.
- Pitch: Adjust the pitch of the voice for a more natural or specific sound.
- Volume: Set the volume for the speech output.
Note: Customizing speech parameters can help make the experience more natural and suitable for your app's user experience needs.
Practical Example
Here’s a simple code snippet to demonstrate basic usage of the TTS API:
import AVFoundation let utterance = AVSpeechUtterance(string: "Hello, welcome to the Apple TTS API!") utterance.voice = AVSpeechSynthesisVoice(language: "en-US") utterance.rate = AVSpeechUtteranceDefaultSpeechRate let synthesizer = AVSpeechSynthesizer() synthesizer.speak(utterance)
Table: Key Parameters for AVSpeechUtterance
Parameter | Description | Default |
---|---|---|
Voice | Selects the language and accent of the voice. | Default system voice |
Rate | Controls the speed of speech (range 0.0 to 1.0). | AVSpeechUtteranceDefaultSpeechRate |
Pitch | Adjusts the pitch of the voice (range 0.5 to 2.0). | 1.0 (neutral pitch) |
Volume | Sets the volume of the speech output. | 1.0 (maximum volume) |
By following these guidelines, you can easily integrate and customize Apple’s Text-to-Speech API in your application. With the flexibility of the API and its various configuration options, you can tailor the voice output to suit different use cases and improve user experience.
Getting Started with Apple Text to Speech API
Apple provides a powerful API for converting text to speech, which is available through the Speech framework. This tool allows developers to integrate speech synthesis capabilities into their applications seamlessly. By leveraging the built-in voices and customization features, users can have text read aloud in a natural-sounding voice, making it ideal for accessibility features or interactive applications.
To begin using the API, developers need to set up their environment and integrate the necessary libraries into their projects. This involves enabling the speech recognition capability in Xcode, managing permissions, and learning how to interact with the API methods. Below is a simple guide on how to get started with Apple’s Text-to-Speech API.
Steps to Integrate Apple Text to Speech API
- Setup Xcode Project - Create a new Xcode project or open an existing one where you want to implement the text-to-speech feature.
- Import Speech Framework - In your project, import the AVFoundation framework which contains the necessary methods for speech synthesis.
- Request Permission - Make sure to ask for microphone and speech recognition permissions from the user. This is essential for functionality.
- Configure Speech Synthesis - Create a speech synthesizer object and configure it with voice parameters like rate, pitch, and volume.
- Start Synthesis - Call the speechSynthesizer.speak() method to begin reading the text aloud.
Important Information
Make sure to handle errors appropriately when requesting permissions. Failure to ask for user consent will lead to the application being unable to use the text-to-speech features.
Example Code
import AVFoundation let synthesizer = AVSpeechSynthesizer() func speakText(_ text: String) { let utterance = AVSpeechUtterance(string: text) utterance.voice = AVSpeechSynthesisVoice(language: "en-US") synthesizer.speak(utterance) }
Voice Options
Voice | Language | Gender |
---|---|---|
Alex | English (US) | Male |
Samantha | English (US) | Female |
Daniel | English (UK) | Male |
Guide to Generating an Apple Text-to-Speech API Key
To start using Apple's Text-to-Speech API, you first need to obtain an API key from Apple's developer platform. This key will grant you access to various text-to-speech features, such as converting text to natural speech using Apple's advanced machine learning models. Setting up your API key is a straightforward process, but it does require careful attention to ensure everything is correctly configured.
Below is a step-by-step guide to help you generate and configure your Apple Text-to-Speech API key. Follow the instructions closely to ensure smooth integration with your projects.
Steps to Obtain Your Apple Text-to-Speech API Key
- Sign in to the Apple Developer Portal: Go to developer.apple.com and log in using your Apple ID. If you don't have an account, create one.
- Access the "Certificates, Identifiers & Profiles" section: Once logged in, navigate to this section from the main dashboard.
- Create a new API key: Under the "Keys" tab, click on the "+" button to generate a new key.
- Configure the key: Choose the appropriate options for your Text-to-Speech service and assign a name to the key for easier identification.
- Download the key: After configuration, you will be able to download the API key file. Store it in a secure location as you won't be able to download it again.
Important: Ensure your Apple Developer account is enrolled in the appropriate program (i.e., the Apple Developer Program) to access these services.
Table of Key Configuration Details
Step | Description |
---|---|
1 | Sign in to your Apple Developer account |
2 | Navigate to "Certificates, Identifiers & Profiles" |
3 | Create a new API key under "Keys" |
4 | Assign a name and configure the key settings |
5 | Download and store the API key file securely |
Remember: The API key file is only downloadable once, so ensure it is securely stored for future use.
Customizing Speech Output for Different Languages and Accents
When using Apple's speech synthesis technology, fine-tuning the voice output to match various languages and regional accents is crucial for a natural-sounding experience. The system provides an array of options to modify the voice parameters, ensuring it can deliver speech accurately for different linguistic contexts. Whether it's for an English dialect, a regional variation of Spanish, or an entirely different language, Apple’s API offers flexibility to meet the needs of diverse users.
Customizing speech output involves adjusting not only the voice selection but also language-specific nuances, including pitch, rate, and even phonetic details. By configuring these settings, developers can create a more personalized and culturally appropriate interaction for their users, enhancing both functionality and user satisfaction.
Language and Accent Options
- Multiple languages support: English, Spanish, French, Chinese, and many more.
- Variety of regional accents available: British English, American English, Australian English, etc.
- Choice of male and female voices for each language.
Voice Parameters Customization
- Rate of Speech: Adjusts the speed at which the text is spoken. Faster or slower rates can be selected based on user preference.
- Pitch Control: Modifies the frequency of the voice. A higher pitch may be used for a lighter, more youthful sound, while a lower pitch can provide a deeper tone.
- Volume: Sets the loudness of the voice output for clearer audibility across different environments.
Important: Always ensure that the language settings are properly aligned with the user's preferences for optimal pronunciation and accent accuracy.
Example of Available Voices in Different Languages
Language | Available Voices | Accent |
---|---|---|
English | Daniel, Siri, Samantha | American, British, Australian |
Spanish | Jorge, Monica | Latin American, European |
French | Thomas, Julie | French (France), Canadian |
Best Practices for Seamlessly Integrating Text-to-Speech in Your Application
Integrating a text-to-speech engine into your application can significantly enhance the user experience, especially for accessibility purposes. Whether your app is designed for visually impaired users or simply for those who prefer auditory over visual content, using the right approach is crucial. Apple’s Text-to-Speech API offers a powerful toolset for converting written text into natural-sounding speech, but understanding the best practices for implementation is essential for creating a smooth user experience.
To ensure a seamless and efficient integration, developers should focus on optimizing performance, user control, and accessibility. Below are key strategies to consider when incorporating text-to-speech features into your app.
1. Optimize User Experience
When implementing text-to-speech functionality, consider the user's context and preferences. The voice settings, language support, and speed of speech should be easily customizable.
- Voice Selection: Allow users to choose from a range of voices, including different accents, genders, and languages. This helps users feel more comfortable with the app.
- Speed Control: Providing speed adjustment ensures that users can tailor the experience to their needs. Some users may prefer faster speech, while others might need it slower for better comprehension.
- Volume and Pitch: Let users adjust pitch and volume for a more personalized auditory experience.
2. Ensure Accessibility
Make sure that the text-to-speech feature is not just an add-on but an integral part of the app’s accessibility features. Ensuring your app works well for users with disabilities is a legal and ethical responsibility.
- Clear Announcements: Ensure that text-to-speech notifications are clear and concise, so that visually impaired users can understand them quickly.
- Real-Time Speech Conversion: Provide real-time text-to-speech conversion for dynamic content, such as chat or live feeds, so users can always follow along.
- Incorporate Support for Multiple Languages: If your app supports multiple languages, ensure that the text-to-speech feature can handle those languages accurately.
3. Monitor Performance and Reliability
Text-to-speech functionality can impact your app’s performance if not managed properly. It's essential to regularly monitor and optimize the integration to ensure smooth operation.
Best Practice | Description |
---|---|
Memory Management | Ensure that audio buffers are released when no longer needed to prevent memory leaks. |
Real-Time Processing | Use background threads to prevent UI freezes when speech synthesis is active. |
Error Handling | Implement robust error handling for situations like network failures or unsupported languages. |
Important: Always test the text-to-speech feature across different devices and network conditions to ensure optimal performance and responsiveness.
How to Effectively Manage API Rate Limits and Maximize Efficiency
When working with Apple’s Text to Speech API, managing rate limits is essential to ensure smooth functionality and avoid disruptions. Rate limits dictate how many requests can be made to the API within a certain timeframe. Exceeding these limits can lead to temporary blocks, resulting in delays and potentially increasing operational costs. Proper management requires understanding both the limit constraints and best practices for optimizing request flow.
Optimizing API usage is not only about respecting rate limits, but also about minimizing unnecessary requests and making each one as efficient as possible. By doing so, you can improve performance, reduce costs, and ensure that resources are utilized effectively. Here are some strategies to achieve this:
Key Strategies to Optimize API Usage
- Batch Requests: Send multiple speech synthesis tasks in one request if the API allows batch processing, thus reducing the number of calls.
- Use Caching: Store frequently requested texts or speech outputs in a cache to avoid repeating requests for the same data.
- Rate Limit Monitoring: Implement monitoring tools to track usage in real-time, adjusting requests dynamically to avoid hitting the limit.
- Exponential Backoff: In case of hitting the rate limit, use exponential backoff strategies to retry requests after increasing delays.
Best Practices for API Rate Limit Management
- Know Your Rate Limits: Understand the specific limits imposed by the API provider, including both daily and per-minute restrictions.
- Leverage Retry Mechanisms: Include automated retry mechanisms with appropriate delays when rate limits are reached to handle errors gracefully.
- Prioritize Requests: Implement priority queues to ensure critical speech synthesis tasks are processed first, minimizing wait times for high-priority users.
Important: Always monitor your API usage against the rate limits to avoid any unexpected interruptions and ensure you are staying within the allowed thresholds.
Rate Limit and Usage Table
Limit Type | Max Requests | Time Period |
---|---|---|
Standard API | 1000 | Per Hour |
Premium API | 5000 | Per Hour |
Additional Quotas | Negotiable | Per Day |
Troubleshooting Common Issues with Apple Text to Speech API
The Apple Text to Speech API offers a robust solution for converting written text into spoken audio. However, developers may encounter certain issues during implementation or usage. Common problems can stem from improper API configuration, incorrect input formats, or system-related restrictions. Addressing these issues promptly can improve the performance of your application and ensure seamless integration of the Text to Speech functionality.
By identifying the root causes and applying targeted solutions, developers can troubleshoot effectively. Below are some of the most frequently encountered issues and their resolutions when using the Apple Text to Speech API.
1. Speech Output Quality Issues
One of the most common concerns is the quality of the synthesized speech. If the speech sounds unnatural or has distortions, it could be related to various factors such as incorrect voice selection or improper API parameters.
- Voice Selection: Ensure the correct voice is being selected for the language and dialect you want to use. The API provides multiple voices; using an unsupported voice may cause issues.
- Speech Rate: Setting a speech rate that is too fast or too slow can affect the clarity of speech. Try adjusting the rate to find a balance that works for your content.
- Audio Format: Incorrect audio format settings may result in audio files that cannot be played properly. Double-check that the audio output is in a compatible format like WAV or MP3.
2. API Authentication Errors
Authentication errors can prevent your application from accessing the Text to Speech services. This often occurs when API keys are missing, expired, or incorrectly configured.
- Ensure that the API key is correctly integrated into your application’s configuration.
- Check if your API key has expired or if there are usage restrictions placed on your account.
- Make sure your application is properly authenticated with the Apple Developer account and that the required permissions are enabled.
It is crucial to renew API keys periodically and verify the access permissions within the Apple Developer account to avoid service disruptions.
3. Handling System Limitations
System limitations, such as insufficient memory or processing power, can also hinder the proper functioning of the Text to Speech API.
- Memory Usage: Ensure your system has adequate memory to handle multiple concurrent speech synthesis requests.
- Network Issues: Slow or intermittent internet connections can lead to timeouts. Consider implementing error handling to retry requests in case of failures.
- Device Compatibility: Some older devices may not fully support all the features of the API. Ensure your application is optimized for the hardware specifications of your target devices.
4. API Rate Limiting
Apple Text to Speech API imposes rate limits to prevent abuse of the service. If your application exceeds these limits, requests may be rejected, resulting in delays or failure to generate speech.
Rate Limit | Issue | Solution |
---|---|---|
Exceeded Requests | Your application has made too many requests within a short time. | Implement request queuing and delay subsequent requests to stay within the rate limit. |
Too Frequent Access | Requests are being made too frequently, causing the server to throttle the connection. | Use backoff strategies, such as exponential backoff, to handle request retries. |
Utilizing Speech Synthesis to Enhance App Accessibility
Incorporating speech synthesis into mobile applications can significantly enhance their accessibility features, making them more inclusive for users with visual or cognitive impairments. By integrating text-to-speech functionality, developers can provide a seamless and interactive experience for individuals who may find it difficult to interact with text-heavy content. This approach helps users engage with applications without relying on traditional visual cues, opening up new possibilities for accessibility and inclusion.
Apple’s Text-to-Speech API offers a powerful toolset for developers to integrate speech synthesis into their apps. By leveraging this technology, developers can allow their users to listen to text-based content, navigate interfaces, and even receive spoken feedback in real-time. This functionality not only improves the usability of apps but also makes them compliant with various accessibility standards, ensuring a broader audience can benefit from the technology.
Key Features of Speech Synthesis for Accessibility
- Voice Customization: Users can select different voices, accents, and speaking rates, which helps tailor the experience to individual needs.
- Real-Time Feedback: Text-to-speech technology can read aloud notifications, alerts, and on-screen text, offering immediate auditory feedback.
- Support for Multiple Languages: The API supports a wide range of languages, ensuring global accessibility for non-native speakers.
Practical Use Cases
- Reading Content: Users can have articles, books, or messages read aloud, eliminating the need for manual reading.
- Guiding Navigation: Visually impaired users can receive spoken directions when navigating through app interfaces.
- Interactive Assistance: Voice responses can be used in customer support features to guide users through troubleshooting steps.
Speech Synthesis Settings Overview
Setting | Description |
---|---|
Voice Selection | Choose from a variety of voices, including gender and accent preferences. |
Speech Rate | Adjust the speed at which the text is read aloud. |
Pitch Control | Modify the pitch of the voice for a more comfortable listening experience. |
“Enabling text-to-speech technology in apps not only provides a better user experience but also promotes inclusivity for individuals with disabilities.”