Twilio Text to Speech Api
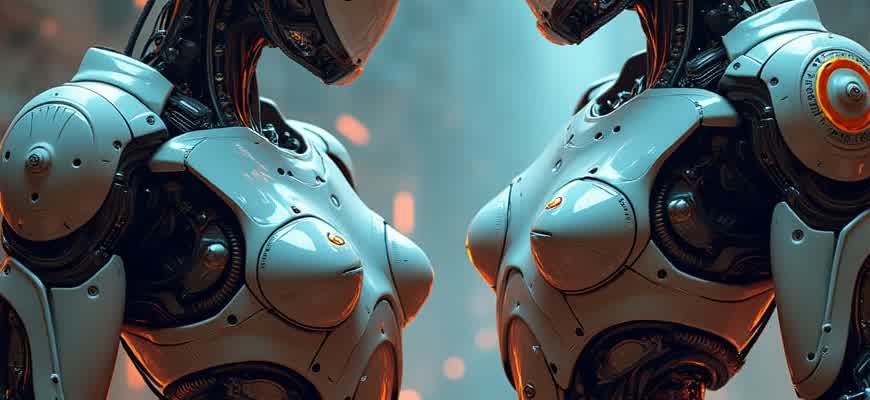
The Twilio Text to Speech API allows developers to integrate voice synthesis into their applications, enabling dynamic speech output from written text. This tool is widely used in creating interactive voice response systems (IVRs), automated customer service systems, and voice-based notifications. By leveraging the API, users can generate realistic speech from text input, which is essential for building engaging and accessible experiences.
Twilio's service supports multiple languages and voice options, providing flexibility in how spoken text is rendered. Below are some key features of the API:
- Real-time speech synthesis from text input
- Multiple language and accent options
- Customizable voice parameters (pitch, speed, volume)
- Support for SSML (Speech Synthesis Markup Language) for advanced control over speech output
Important: The API allows both male and female voices in various languages, ensuring wide applicability for global applications.
To get started, developers need to integrate the API by making HTTP requests. Here's an example of how the API works:
- Create a Twilio account and obtain your API credentials.
- Set up your environment to send HTTP requests to the Twilio API endpoint.
- Provide text input and choose your desired voice and language options.
- Receive an audio stream or file containing the synthesized speech.
For more detailed information, refer to the following table showing some common API parameters:
Parameter | Description |
---|---|
Voice | Specifies the voice to be used (e.g., male or female, specific language). |
Language | Defines the language for the speech output (e.g., English, Spanish). |
Speed | Controls the rate at which the speech is delivered (from slow to fast). |
Volume | Adjusts the loudness of the speech output. |
Twilio Text-to-Speech API: A Detailed Guide
The Twilio Text-to-Speech API provides an easy way to convert text into natural-sounding speech in your applications. It supports multiple languages and voices, offering flexibility for developers aiming to add voice capabilities to their systems. By utilizing this API, you can easily create dynamic voice interactions, such as phone calls or interactive voice responses (IVR), without the need for complex infrastructure setup.
This guide will walk you through the key features of the Twilio Text-to-Speech API, explaining how it works, how to integrate it into your project, and best practices to get the most out of its capabilities. Whether you’re building a customer service application, a notification system, or any other voice-enabled solution, this API provides a robust foundation.
Key Features of Twilio Text-to-Speech API
- Multiple Languages and Voices: Supports a variety of languages and voices, both male and female, enabling customization based on the needs of the target audience.
- Customizable Speech Rate and Pitch: Adjust the rate and pitch of the generated speech to ensure it fits the context of your application.
- SSML Support: Allows the use of SSML (Speech Synthesis Markup Language) for fine-tuning speech output, including adding pauses, emphasizing certain words, and controlling pronunciation.
How to Integrate the API
- Sign up for a Twilio account and obtain your API credentials.
- Install the Twilio SDK for your preferred programming language.
- Use the API to send a request containing the text you want to convert into speech.
- Set the desired voice, language, and other parameters (e.g., rate, pitch).
- Handle the audio response and integrate it into your application (e.g., play it over a phone call or save it as an audio file).
Parameters Overview
Parameter | Description | Example |
---|---|---|
Voice | Selects the voice to be used for speech synthesis. | alice, man, woman |
Language | Specifies the language for the speech output. | en-US, es-ES |
Rate | Controls the speed of the speech. | 0.5, 1.0 (default), 1.5 |
Pitch | Adjusts the pitch of the voice. | low, medium, high |
Important: Always ensure to test the speech output across different voices, languages, and rates to find the best configuration for your application. This helps in providing a more engaging user experience.
How to Integrate Twilio Text to Speech API into Your Application
Integrating Twilio's Text to Speech API into your app can help you deliver dynamic voice-based communication. Whether you're building an automated customer service solution or a voice assistant, Twilio offers a reliable service for converting text to human-like speech. In this guide, we'll walk you through the key steps for implementing this API into your application.
To start using Twilio’s Text to Speech functionality, you will need an active Twilio account and a few dependencies installed in your project. You can easily integrate the API into your backend code, with support for several programming languages. The process involves making a simple API request with the desired text input, and Twilio will return the corresponding audio file.
Steps to Integrate Twilio Text to Speech
- Sign Up for a Twilio Account
Create a Twilio account at Twilio's website. After signing up, you’ll receive an Account SID and Auth Token which will be required for authentication.
- Install Twilio SDK
Install the Twilio SDK for your programming language. For Python, you can use the following command:
pip install twilio
- Configure Your Application
Once the SDK is installed, configure your app to authenticate using the Account SID and Auth Token:
from twilio.rest import Client client = Client("your_account_sid", "your_auth_token")
- Generate Speech from Text
Now, make a request to the Twilio API to generate speech from a given text:
call = client.calls.create( to='+1234567890', from_='+0987654321', twiml='
' )Hello, this is a test message
Key Features and Settings
Twilio’s Text to Speech API offers several customizable settings for optimal speech quality.
Parameter | Description |
---|---|
Voice | Choose from different voice options, like 'alice' or 'man', for varied speech styles. |
Language | Select the desired language, such as English or Spanish, for localized speech. |
Speech Rate | Adjust the speed at which the text is spoken. |
Important: Remember to handle the API response carefully to ensure smooth operation within your application. You may need to handle errors such as network timeouts or incorrect authentication tokens.
By following these steps, you can seamlessly integrate Twilio's Text to Speech capabilities into your application and provide a more interactive, user-friendly experience. Whether it’s for customer engagement or accessibility, the API’s flexibility and ease of use make it a great choice for many projects.
Choosing the Right Voice and Language for Your Twilio Speech Application
When integrating Twilio's Text-to-Speech API into your application, one of the most critical decisions is selecting the optimal voice and language. The right choices can significantly improve user experience by ensuring clarity, engagement, and emotional tone. Understanding the available voice options and languages will help tailor your speech application to meet specific regional or emotional needs.
Twilio provides a wide range of voice models, each with unique characteristics, as well as numerous language options. Choosing between different voices, accents, and languages can directly affect how users interact with your application. This is particularly important in applications where user satisfaction and accessibility are key factors.
Factors to Consider When Selecting Voices and Languages
- Language Support: Ensure that the language you choose is supported by Twilio's API. Some regions may require specific dialects or accents to enhance local user comprehension.
- Voice Gender and Tone: Decide whether a male or female voice is more appropriate for your application. Additionally, the tone (neutral, friendly, authoritative) should align with your brand's personality and the context of the communication.
- Accent and Regional Variations: Different accents can influence how users perceive the voice. Choose an accent that resonates with your target audience for better understanding and relatability.
Steps for Choosing the Right Voice
- Review the list of available languages and voices in the Twilio API documentation.
- Test the voice options in different scenarios to evaluate clarity, pronunciation, and emotional tone.
- Consider feedback from your target users to understand their preferences.
- Check for localization options if your application targets multiple regions or languages.
Table of Popular Voices and Languages
Language | Voice Option | Gender | Accent |
---|---|---|---|
English (US) | Joanna | Female | American |
Spanish (Spain) | Conchita | Female | Castilian |
German | Hans | Male | German |
French | Celine | Female | French |
It is essential to evaluate the user's geographic location, language preferences, and cultural context when selecting a voice. This ensures your application sounds natural and approachable to the target audience.
Configuring Authentication and API Keys for Twilio Text to Speech
Before you can begin using the Twilio Text to Speech API, it's essential to authenticate your requests. This requires generating and securely storing API credentials that allow you to interact with Twilio's services. The key to this is Twilio’s API keys, which are used to authenticate and authorize your applications.
The process involves creating a Twilio account, retrieving your API keys, and configuring them in your application to enable secure communication with Twilio's servers. Below is a step-by-step guide to setting up authentication with the necessary credentials.
Step-by-Step Authentication Setup
- Sign up for a Twilio account at Twilio.com.
- Once logged in, navigate to the dashboard to locate your Account SID and Auth Token. These are your primary credentials for authenticating with the API.
- For additional security, consider creating API keys for more granular access control. This can be done under the "API Keys" section in your Twilio console.
- Save these credentials securely, as they are required to make API requests.
Using API Keys in Your Application
- In your code, use the Twilio SDK or make HTTP requests to the Twilio REST API using the Account SID and Auth Token.
- If using API Keys, pass the API Key SID and Secret instead of the Account SID and Auth Token.
- Ensure that your API keys are stored in a secure environment, such as environment variables or a secure secrets management tool.
Important Security Considerations
Always keep your API keys confidential. Exposing them in your source code or publicly accessible repositories can lead to unauthorized access to your Twilio account.
API Credentials Table
Credential | Description |
---|---|
Account SID | A unique identifier for your Twilio account used for general authentication. |
Auth Token | A secret token used to authenticate requests made with your Account SID. |
API Key SID | A unique identifier for API keys that grants access to specific Twilio resources. |
API Key Secret | A secret associated with the API Key SID for authentication. |
Handling Different Audio Formats in Twilio Text to Speech Responses
When integrating Twilio's Text to Speech API into your applications, choosing the right audio format for your responses is crucial. The service provides flexibility in the formats it supports, allowing you to select the most suitable option based on your use case. The available formats range from standard audio files to more advanced formats, giving developers a variety of options for optimal playback across different platforms and devices.
In order to ensure the best quality and compatibility, it’s important to understand the characteristics of each supported audio format. Twilio’s API allows you to specify the audio format for the speech response, which can be critical in scenarios where bandwidth, file size, or device compatibility is a concern. Below is an overview of the key formats available and how to handle them effectively.
Supported Audio Formats
Twilio supports several audio formats that can be used in the Text to Speech API. These formats include:
- WAV - High-quality, uncompressed audio suitable for high-fidelity applications.
- MP3 - A compressed format widely used for general-purpose audio with a good balance between quality and file size.
- OGG - Open-source compressed format, typically used in web applications for streaming and efficiency.
- PCM - Raw audio format without compression, offering high quality but larger file sizes.
Configuring Audio Format in API Requests
When making a request to the Twilio API, you can specify the desired audio format for the speech response by using the `voice` and `format` parameters. The following table summarizes how to set the format for different scenarios:
Audio Format | Parameter Value |
---|---|
WAV | audio/wav |
MP3 | audio/mp3 |
OGG | audio/ogg |
PCM | audio/pcm |
Important Considerations
Remember, each audio format comes with its own set of trade-offs in terms of quality, file size, and compatibility. It’s essential to test and select the most appropriate format based on the specific needs of your application.
Customizing Speech Rate, Pitch, and Volume with Twilio
Twilio offers robust features to enhance the speech synthesis process, allowing developers to adjust important voice parameters such as speech rate, pitch, and volume. These settings can be tailored to create a more natural or dynamic voice output, making it suitable for various applications, from customer service bots to voice alerts. By modifying these attributes, developers can significantly improve user experience and accessibility.
Understanding how to fine-tune these parameters is essential for delivering high-quality speech synthesis that aligns with the needs of your audience. Twilio provides simple controls for adjusting each of these parameters through its API, which can be accessed and modified as part of the `VoiceResponse` object in a Twilio application. The following sections describe how to configure these properties effectively using Twilio's tools.
Adjusting Speech Parameters
The key parameters that affect speech output in Twilio are rate, pitch, and volume. Each of these can be customized individually using specific attributes within the Twilio API:
- Speech Rate: Controls the speed at which the speech is delivered.
- Pitch: Determines the tone or pitch of the voice (higher pitch = more energetic tone, lower pitch = calmer tone).
- Volume: Allows control over the loudness of the speech output.
How to Implement Customizations
These attributes are adjusted within the `say` function of Twilio's API. Below is an example of how to configure these settings in a Twilio application:
Hello, this is a test message with customized parameters.
Here’s a breakdown of the parameters used in the example:
Parameter | Attribute | Possible Values |
---|---|---|
Rate | rate="slow" or rate="fast" | Normal, Slow, Fast |
Pitch | pitch="high" or pitch="low" | High, Low, Medium |
Volume | volume="x-soft", "soft", "medium", "loud" | x-soft, soft, medium, loud |
Important Notes
Remember, not all voices support every rate, pitch, or volume level. It’s essential to test different combinations to ensure compatibility and optimal clarity for your users.
Managing API Quotas and Rate Limiting in Twilio Speech API
When using Twilio’s Speech API, managing your usage is essential to avoid exceeding service limits. Twilio implements rate limits and quotas to ensure that the API can handle requests effectively and prevent system overload. These restrictions can vary based on the specific pricing plan and usage tier of your Twilio account. Understanding and tracking your limits is crucial for maintaining smooth operation and avoiding unexpected disruptions in service.
The Speech API's quotas typically apply to the number of requests you can make in a given time period, while rate limiting restricts the speed at which you can make those requests. Exceeding these limits can result in temporary blocking of requests, which can affect the performance of your application. In this context, it's essential to monitor both your usage and your rate limit status regularly.
How to Monitor API Usage and Limits
Twilio provides several ways to track your quota and usage through the API or the Twilio Console. Here are the key methods:
- Twilio Console: The console provides a dashboard where you can monitor your account’s usage in real-time.
- Usage Records: You can retrieve detailed usage reports via the API to track the number of requests made over specific periods.
- Rate Limiting Headers: Twilio sends specific headers with each API response, indicating the remaining requests you can make before hitting the rate limit.
Common Rate Limits and Quota Restrictions
The limits for the Speech API depend on your account and the specific service tier. Below are the general rate limits:
Request Type | Limit | Time Period |
---|---|---|
Speech Synthesis Requests | 1000 requests per minute | Per minute |
Text-to-Speech Requests | 500 requests per minute | Per minute |
Important: Exceeding these limits may result in a temporary suspension of service, so it’s critical to manage your request rate effectively.
Best Practices for Avoiding Rate Limits
To ensure that your application runs smoothly without exceeding quotas or rate limits, consider the following strategies:
- Implement Retries: If your request is throttled, implement automatic retries with exponential backoff to minimize disruptions.
- Batch Requests: Where possible, combine multiple requests into one to reduce the overall request rate.
- Monitor API Usage: Regularly check your API usage in the Twilio Console and adjust your request frequency as needed.
Enhancing Audio Output for Better Accessibility with Twilio Speech API
When working with speech synthesis systems, especially for accessibility purposes, it is crucial to optimize the audio output to meet the needs of diverse users. Twilio’s text-to-speech service provides developers with the ability to create customizable voice outputs that cater to individuals with various impairments, such as visual or auditory disabilities. This capability is vital for ensuring that applications are inclusive and usable by a wider audience. Key elements like speech speed, voice pitch, and clarity all play a role in making the output more accessible to those with different accessibility needs.
Optimizing the audio output involves considering multiple factors, including the choice of language, voice tone, and the pacing of speech. By adjusting these elements, developers can create audio that is clear and easy to understand for users with diverse needs. In addition, the ability to modify the volume and include pauses can further enhance the experience, making it more comfortable and less overwhelming for users.
Key Strategies for Audio Output Optimization
- Voice Clarity: Choose a voice with clear pronunciation and avoid accents or speech patterns that may be difficult for some users to understand.
- Adjustable Speech Speed: Ensure the speed of speech is adaptable, allowing users to slow down or speed up the speech as needed.
- Pauses and Pacing: Implement pauses to break up long sentences, allowing listeners to process information more easily.
- Volume Control: Allow users to control the volume of the speech output to match their hearing needs.
- Multiple Language Support: Offer various language options to cater to users who speak different languages or dialects.
Important Considerations
By fine-tuning the output characteristics, developers can ensure that speech synthesis is not only effective but also accessible to users with specific needs. Prioritize clarity and adaptability to meet diverse user requirements.
Configuring Twilio Text to Speech API for Accessibility
Setting | Details |
---|---|
Voice | Select from multiple voice options (male, female, neutral) to best suit user preferences. |
Speed | Adjust the rate of speech, with a slower pace being ideal for users who require more time to process audio. |
Volume | Control the output volume to ensure it is within a comfortable range for all users. |
Pause Duration | Include pauses between sentences or phrases to enhance comprehension. |
Implementation Example
- Choose the voice and adjust speed to suit user preferences.
- Set the speech output volume to a reasonable level based on user needs.
- Configure appropriate pauses to avoid overwhelming the listener.
- Test the audio output with users from various accessibility backgrounds to ensure effectiveness.
Debugging Common Issues in Twilio Text to Speech API
When using the Twilio Text to Speech API, users might encounter several issues that can affect the quality and functionality of the speech synthesis. It is crucial to identify and resolve these problems to ensure smooth operation. This guide highlights some of the most common challenges and how to troubleshoot them effectively. By understanding potential pitfalls, users can quickly diagnose and resolve errors in their implementation.
The main causes of errors typically stem from misconfigurations, incorrect parameters, or integration issues. This section will cover key problems, such as voice settings, audio quality, and network-related errors. Knowing how to interpret error messages and logs is essential for debugging. Below are some common problems and their solutions.
Common Issues and Solutions
- Invalid API Credentials: Ensure that your Twilio account SID and authentication token are correct. Invalid credentials can lead to authorization errors when making requests.
- Incorrect Language or Voice Settings: If the wrong language or voice is selected, the speech synthesis might fail or produce unintended results. Verify the available voice options for the chosen language.
- Audio Quality Issues: Low-quality audio or distortion can occur due to poor network connectivity or low bitrate settings. Test the connection speed and adjust the bitrate as necessary.
- Network Timeouts: The API request might time out if the server is unreachable or overloaded. Check your network settings and retry the request.
Steps to Troubleshoot
- Check the API Response: Always inspect the response from the API. Look for specific error codes and messages that indicate the problem.
- Verify Your Configuration: Ensure that all API parameters, such as language, voice, and audio format, are correctly set in the request.
- Test with Different Voices: If the issue is related to the voice, try switching to a different voice or language to see if the problem persists.
- Check Network and Server Status: Make sure there are no network issues or server outages that could be causing the problem.
Tip: Always refer to the official Twilio documentation and API logs for detailed error codes and troubleshooting steps.
Helpful Tools
Tool | Description |
---|---|
Twilio Console | Use the console to check API logs and monitor real-time requests for errors and warnings. |
Twilio API Explorer | A helpful tool for testing API requests and responses before integrating them into your application. |
Network Monitoring Tools | Tools like Wireshark can help diagnose network-related issues that may be affecting API calls. |