Azure Text to Speech Api Python
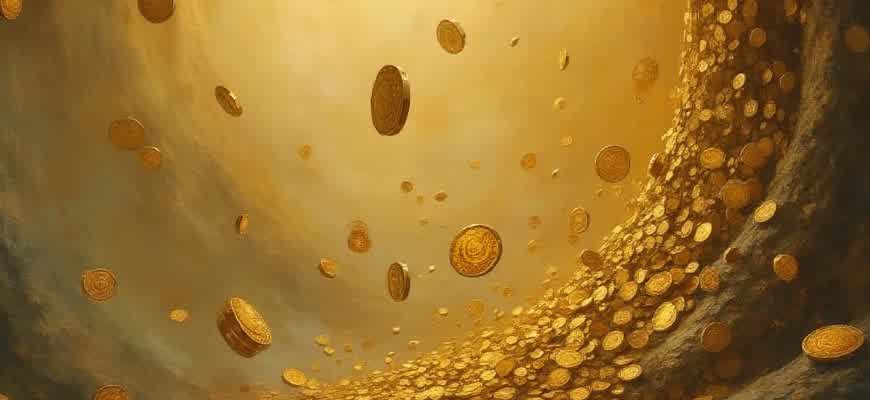
Azure's Text-to-Speech API offers a powerful way to convert written text into lifelike speech. This API can be easily integrated into Python applications to enable voice synthesis capabilities. To use the service, you'll need to set up an Azure account and obtain the necessary API keys.
Here's a brief overview of the steps to start using the API:
- Sign up for an Azure account and create a Speech resource in the Azure portal.
- Obtain the API key and endpoint URL from the Azure portal.
- Install the Azure Speech SDK in your Python environment using pip.
- Write Python code to make requests to the API and convert text to speech.
Below is an example of a simple Python script to convert text into speech:
import azure.cognitiveservices.speech as speechsdk # Set up the subscription info and region subscription_key = "" region = " " # Initialize the speech configuration speech_config = speechsdk.SpeechConfig(subscription=subscription_key, region=region) speech_synthesizer = speechsdk.SpeechSynthesizer(speech_config=speech_config) # Synthesize text to speech text = "Hello, welcome to Azure Text to Speech!" speech_synthesizer.speak_text_async(text)
Important: Always keep your API key secure and do not expose it in public repositories or client-side code.
The Azure Text-to-Speech API provides various voices and languages. You can customize the voice by choosing from a variety of male and female options. The following table lists some supported languages and their respective voices:
Language | Male Voice | Female Voice |
---|---|---|
English (US) | en-US-GuyNeural | en-US-JessaNeural |
Spanish (Spain) | es-ES-AlvaroNeural | es-ES-LauraNeural |
German | de-DE-KarstenNeural | de-DE-HeddaNeural |
Azure Text-to-Speech API in Python: A Practical Guide
Azure’s Text-to-Speech API provides a powerful tool for converting written text into spoken words. By leveraging cloud technology, developers can integrate high-quality voice synthesis into their Python applications, creating a wide range of possibilities for voice-driven solutions, from virtual assistants to interactive audio systems.
This guide will walk you through the steps to use the Azure Text-to-Speech service effectively with Python. You’ll learn how to authenticate, make API calls, and handle different voices and languages to suit your project needs. Azure offers a variety of voices, including neural voices, which bring a more natural and fluid tone to the generated speech.
Setting Up the Environment
Before you start, you need to set up a few things to use the Azure Text-to-Speech API in Python. Follow these essential steps:
- Create an Azure account and get your API key from the Azure portal.
- Install the required Python packages using pip:
pip install azure-cognitiveservices-speech
Make sure you have a valid subscription to the Azure Cognitive Services to access the Text-to-Speech API.
Making the First API Call
Once the environment is set up, you can begin using the API to convert text into speech. Here’s a basic implementation:
import azure.cognitiveservices.speech as speechsdk
speech_key = "YourAzureAPIKey"
region = "YourAzureRegion"
speech_config = speechsdk.SpeechConfig(subscription=speech_key, region=region)
audio_config = speechsdk.audio.AudioOutputConfig(use_default_speaker=True)
speech_synthesizer = speechsdk.SpeechSynthesizer(speech_config=speech_config, audio_config=audio_config)
text = "Hello, welcome to the world of Azure Text to Speech!"
speech_synthesizer.speak_text_async(text)
This code initializes the synthesizer and plays the generated speech using your system's default speaker. You can customize it by selecting different voices and languages.
Available Voices and Languages
Azure provides several voices and language options. Below is a table summarizing some of the available voices:
Voice Name | Language | Voice Type |
---|---|---|
en-US-AriaNeural | English (US) | Neural |
de-DE-KatjaNeural | German (Germany) | Neural |
fr-FR-DeniseNeural | French (France) | Neural |
Choosing a neural voice provides a more natural, expressive sound compared to the standard voices.
Setting Up Azure Text to Speech API for Python Projects
To integrate the Azure Text to Speech service into your Python project, there are a few essential steps you need to follow. Azure provides a REST API to convert text into natural-sounding speech, and you can easily access it using Python. To get started, you must create an Azure account, set up a Speech resource, and configure the environment for your Python code.
Once the setup is complete, you can make use of the Azure SDK for Python to interact with the API and generate speech from text. Below is a step-by-step guide for setting everything up, including prerequisites, installation, and code implementation.
Prerequisites
- An Azure account and subscription.
- A Speech resource created in the Azure portal.
- Python 3.x installed on your machine.
- The Azure SDK for Python installed via pip.
Steps to Set Up
- Create an Azure Account: If you don’t have an account, sign up for one on the Azure website.
- Create a Speech Resource: In the Azure portal, create a new Speech service resource. This will give you the API keys and region information you need to access the service.
- Install Azure SDK: Run the following command to install the required Python library:
pip install azure-cognitiveservices-speech
- Set up Authentication: Ensure you have your Speech API key and region details from the Azure portal. These will be used in your Python code to authenticate requests.
Code Example
Here’s a simple Python script to demonstrate how to use the Azure Text to Speech API:
import azure.cognitiveservices.speech as speechsdk speech_key = "" region = " " speech_config = speechsdk.SpeechConfig(subscription=speech_key, region=region) speech_synthesizer = speechsdk.SpeechSynthesizer(speech_config=speech_config) text = "Hello, welcome to the Azure Text to Speech service!" speech_synthesizer.speak_text_async(text)
Important Notes
Ensure that you replace <YOUR_API_KEY> and <YOUR_REGION> with your actual API key and region from your Azure account.
Additional Configurations
For more advanced use cases, you may want to adjust parameters like voice type, language, or audio output format. The Azure SDK provides flexibility to control various aspects of speech synthesis. Here’s a table outlining some common configuration options:
Option | Description |
---|---|
Voice Name | Specifies the voice used for speech synthesis (e.g., "en-US-JessaNeural"). |
Audio Format | Defines the audio format of the output (e.g., "mp3", "wav"). |
Language | The language to use for speech (e.g., "en-US", "ru-RU"). |
Authenticating and Connecting to the Azure API Service
To interact with the Azure Text to Speech API using Python, proper authentication is essential. Azure uses a subscription-based model, where you need a subscription key and an endpoint to authenticate and make requests. These keys allow you to access different services within the Azure ecosystem securely.
Before diving into Python code, you should first create an Azure Cognitive Services resource in the Azure portal. Once created, you will be provided with the necessary credentials–an API key and an endpoint URL–that you will use to authenticate and send requests from your Python script.
Authentication Process
To connect to the Azure Text to Speech API, follow these steps:
- Sign in to the Azure portal and navigate to the Cognitive Services section.
- Create a new instance of the Text to Speech API.
- Locate the API key and endpoint from the resource you created.
- Install the Azure SDK in Python if you haven't already:
pip install azure-cognitiveservices-speech
Once these steps are complete, you can authenticate and connect to the service in your Python code.
Connecting to the API
Now that you have your credentials, you can use them to initialize the connection in your Python script. Here's a sample code snippet:
import azure.cognitiveservices.speech as speechsdk
# Replace with your actual API key and region endpoint
subscription_key = "YourSubscriptionKey"
region = "YourRegion"
# Create a speech configuration instance
speech_config = speechsdk.SpeechConfig(subscription=subscription_key, region=region)
# Create a speech synthesizer instance
speech_synthesizer = speechsdk.SpeechSynthesizer(speech_config=speech_config)
# Start synthesizing speech
speech_synthesizer.speak_text_async("Hello, this is a test.")
Important: Always store your API key and endpoint securely. Do not expose them in your code or public repositories to prevent unauthorized access.
Configuration Table
Field | Description |
---|---|
subscription_key | Your unique key to authenticate requests to the API. |
region | The geographical region for your Azure Cognitive Services resource (e.g., "eastus"). |
With these configurations set, you can now make authenticated requests to the Azure Text to Speech API and perform various speech synthesis operations.
Integrating Speech Synthesis in Python Applications
To incorporate speech synthesis into your Python projects, leveraging the Azure Text-to-Speech API can significantly enhance your application's functionality. This integration allows your program to convert text into natural-sounding speech, making it accessible to a broader audience, including users with visual impairments or those in environments where reading text isn't practical.
The Azure Text-to-Speech API is highly versatile, offering numerous features such as different voice models, accents, and languages. It provides developers with the tools to customize the speech output to fit the needs of their application. Below is a breakdown of the essential steps and considerations for integrating this API into your Python code.
Steps to Integrate Azure Speech Synthesis API in Python
- First, create an account on Azure and set up a Speech service.
- Obtain the API key and endpoint URL for your Speech service.
- Install the Azure SDK for Python by running pip install azure-cognitiveservices-speech.
- Initialize the Speech SDK in your Python script using your API key and endpoint.
- Use the API to send text data and receive synthesized speech in response.
Remember to handle errors and exceptions in your code, especially when dealing with network connectivity or service limits.
Configuration Example
Step | Action |
---|---|
Step 1 | Set up your Speech API on the Azure Portal and get the API key. |
Step 2 | Install the Azure SDK in Python using pip install azure-cognitiveservices-speech. |
Step 3 | Write Python code to initialize the SDK with your API key and endpoint. |
Common Parameters for Speech Synthesis
- Voice Name - Choose from a variety of voices available in the service, such as male or female voices, and different languages.
- Speech Rate - Control how fast or slow the speech is generated.
- Speech Volume - Adjust the loudness of the generated speech.
Customizing Voice Parameters: Speed, Pitch, and Language
When working with Azure's Text-to-Speech API, one of the key aspects that can significantly enhance the user experience is the customization of voice parameters. These include adjusting the speed of speech, altering the pitch, and selecting the appropriate language. These features allow developers to fine-tune the generated voice to better suit the needs of their application or target audience.
Customizing these parameters provides more flexibility in creating natural and engaging speech. By controlling factors like speech speed and pitch, developers can produce voices that sound more human-like and fitting for various use cases. This can be particularly important in scenarios like virtual assistants, educational tools, or customer service applications.
Speed Adjustment
Speech speed affects how quickly the synthesized voice reads out text. It can be modified using the rate property in the Azure API. Adjusting the speed of the voice helps ensure that the speech is easily understandable for the user.
- Normal Speed – Default speech rate for natural delivery.
- Fast Speed – Increased rate, useful for brief statements or quick information.
- Slow Speed – Reduced rate, ideal for instructions or clarifications.
Pitch Control
The pitch parameter modifies the tone of the voice. By fine-tuning pitch, developers can make the voice sound higher or lower. This can be useful in making the voice more friendly, professional, or serious, depending on the intended audience.
- High Pitch – Results in a more youthful and energetic tone.
- Medium Pitch – Balanced tone, suitable for most applications.
- Low Pitch – Produces a deeper, more formal sound.
Language Selection
The language setting allows you to choose the voice language, which is crucial for reaching a global audience. Azure supports multiple languages and regional accents to accommodate different user bases.
Language | Voice Type | Accent |
---|---|---|
English (US) | Male/Female | American |
French (France) | Male/Female | French |
German | Male/Female | German |
Adjusting voice parameters like speed, pitch, and language can drastically improve user interaction, ensuring the speech synthesis meets the tone and pace required for any application.
Handling API Errors and Debugging in Python
When interacting with the Azure Text to Speech API in Python, it's important to handle potential errors that may arise during the request and response cycle. These errors can include network issues, incorrect parameters, or API-related limits. Proper error handling ensures that your application doesn't crash unexpectedly and provides useful feedback for debugging. In this guide, we'll explore how to handle these issues efficiently in Python.
Azure API provides error messages and status codes that can help pinpoint the source of the issue. By implementing robust error handling strategies, you can ensure your application is both reliable and user-friendly. Below are some essential practices for handling errors and debugging issues effectively when working with Azure's Text to Speech API.
Error Handling Best Practices
- Handle HTTP Status Codes: Check the response status code to determine if the request was successful or if an error occurred.
- Use Try-Except Blocks: Wrap your API calls in try-except blocks to catch and handle exceptions like connection errors, timeouts, or HTTP errors.
- Log Error Responses: Ensure that the error responses from the API are logged for later review and troubleshooting.
Common Error Scenarios and Solutions
- 400 Bad Request: This typically happens when parameters are missing or incorrect. Check your request format and verify that the body of the request matches the expected structure.
- 401 Unauthorized: This error indicates authentication issues. Make sure your subscription key is valid and properly included in the request headers.
- 403 Forbidden: The subscription may not have access to the service. Verify your subscription and resource permissions.
Tip: Use the Azure SDK’s logging functionality to get detailed error information for debugging. This can often reveal hidden issues such as malformed requests or missing authentication keys.
Debugging Techniques
To debug and troubleshoot API requests, you can use several techniques:
- Enable Logging: Log all API responses, including both successful and failed requests, to capture detailed error messages.
- Inspect Response Objects: The response object contains status codes and error messages that can help pinpoint the issue.
Helpful API Debugging Tools
Tool | Description |
---|---|
Postman | Use Postman to test API requests before implementing them in Python. It helps visualize the request-response cycle and debug errors more easily. |
Azure SDK Logs | The Azure SDK provides built-in logging that can track requests, responses, and error messages for deeper insights. |
Optimizing Audio Output for Different Devices and Formats
When using the Azure Text-to-Speech API in Python, it's essential to ensure the generated audio output is optimized for various devices and formats. Different platforms, such as smartphones, desktops, and voice assistants, have specific requirements for audio quality, format compatibility, and playback performance. By tailoring the output to these requirements, you can provide the best user experience across a range of devices.
One of the key factors to consider is the choice of audio format. Different devices may support distinct audio encodings, so it's crucial to use formats that are widely supported and efficient for the given use case. Additionally, the bitrate and sample rate can significantly affect both the file size and audio clarity. Optimizing these parameters for each target device or platform ensures that the audio plays smoothly without compromising performance.
Audio Format Selection
When choosing an audio format, it's important to evaluate the device's capabilities. For example:
- MP3: Widely supported on most devices, ideal for general purposes with good balance between quality and file size.
- WAV: Best for high-quality sound but results in larger file sizes, typically used on desktop systems or for professional-grade applications.
- OGG: A good option for web-based applications, offering excellent compression without sacrificing too much quality.
- PCM: Often used in telephony systems where clarity is paramount, but can result in large file sizes.
Bitrate and Sample Rate Considerations
Both bitrate and sample rate impact the final audio quality and file size. When optimizing for devices, consider the following:
- For smartphones and low-bandwidth environments, using a lower bitrate (e.g., 64kbps) can reduce file sizes and improve streaming performance.
- For high-quality audio output, such as for desktop speakers or professional audio systems, higher bitrates (e.g., 192kbps or 320kbps) and sample rates (e.g., 48kHz) should be considered.
Important: Be sure to adjust the audio settings based on both the device's capabilities and the intended usage, whether it's for background voice assistance or high-fidelity listening.
Optimizing for Specific Devices
Device | Recommended Format | Suggested Bitrate |
---|---|---|
Smartphone | MP3, OGG | 64kbps - 128kbps |
Desktop | WAV, MP3 | 128kbps - 320kbps |
Voice Assistants | MP3, OGG | 64kbps |
Best Practices for Managing API Costs in Long-Term Projects
When working with Azure Text to Speech API over an extended period, managing costs efficiently is crucial. With the increasing scale of operations and usage, ensuring that API costs remain within budget can be challenging. It is important to implement strategies that help monitor, optimize, and control expenses while maintaining high-quality performance in your project.
By understanding usage patterns, implementing proper monitoring tools, and optimizing API calls, developers can significantly reduce unnecessary expenditures. Below are several key practices to follow when managing API costs in long-term projects.
Key Strategies for Cost Management
- Set Usage Limits: Establish clear usage limits and track consumption regularly. This will help prevent unexpected spikes in usage and associated costs.
- Use Caching Mechanisms: Cache the results of API calls whenever possible to reduce the number of requests made to the API, thus minimizing expenses.
- Choose the Right Pricing Tier: Analyze the pricing tiers of the API and select the one that aligns best with your expected usage, balancing cost with feature requirements.
Optimizing API Call Frequency
- Consolidate requests where possible. Instead of making multiple calls for similar data, batch them into a single request.
- Use less expensive voices or features for non-essential tasks or during low-usage times.
- Consider reducing the frequency of API calls by implementing asynchronous processing or scheduling tasks during off-peak hours.
Monitoring and Analytics Tools
Utilizing the built-in analytics and monitoring tools provided by Azure can be extremely valuable in tracking the performance and costs of your API usage. Set up alerts to monitor your budget limits and receive notifications when costs exceed predefined thresholds. This can help you adjust strategies before running over budget.
"Regular monitoring of usage trends and costs ensures that you're able to make informed decisions on optimizing your API strategy, rather than reacting after costs have spiraled."
Table: Cost Optimization Strategies
Strategy | Description |
---|---|
Usage Caps | Limit the number of API calls or set monthly quotas to avoid unexpected costs. |
Cache Responses | Store common responses locally to avoid repetitive calls to the API. |
Optimize Request Frequency | Reduce unnecessary API calls by batching requests or handling them asynchronously. |
Scaling Azure Text to Speech API for Production Applications
When integrating Azure Text to Speech API into production environments, it is essential to ensure that the system can scale efficiently to handle increasing loads while maintaining performance and responsiveness. As the demand for speech synthesis grows, optimizing the deployment process becomes crucial for smooth operation across various workloads.
To achieve a scalable solution, you need to consider several factors, such as API rate limits, concurrency, and resource allocation. Proper management of these aspects helps prevent bottlenecks and ensures that the service remains reliable and cost-effective during high-demand periods.
Key Considerations for Scaling
- API Rate Limiting: Understand the maximum number of requests the API can handle per minute to avoid exceeding usage limits.
- Concurrency Control: Manage the number of concurrent requests to prevent overloading the system and ensure that all requests are processed in a timely manner.
- Optimal Resource Allocation: Adjust the amount of computing resources based on traffic patterns to maintain high availability and low latency.
Scaling the Text to Speech API efficiently requires balancing between performance, cost, and available resources to meet the application's requirements.
Performance Optimization Techniques
- Use batch processing for multiple requests, reducing the total number of individual calls to the API.
- Leverage regional deployments to minimize latency by selecting the closest data center to the end users.
- Implement caching mechanisms to store frequently requested speech data, reducing redundant calls to the API.
Resource Allocation and Pricing
Azure provides different pricing tiers for the Text to Speech API, offering flexibility depending on your application’s needs. The following table outlines the key pricing options:
Pricing Tier | Monthly Quota | Price per Million Characters |
---|---|---|
Standard | 5 million characters | $4.00 |
Neural | Up to 10 million characters | $16.00 |