Openai Text to Speech Api Github
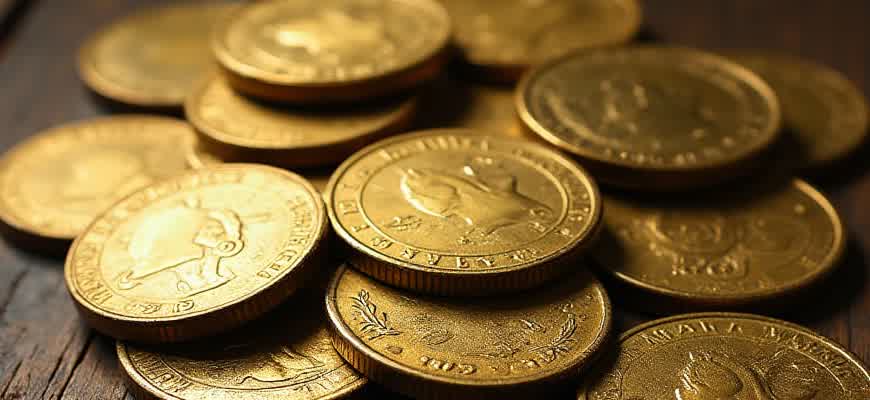
OpenAI's Text-to-Speech (TTS) API offers a powerful solution for converting written text into human-like speech, leveraging advanced machine learning models. Hosted on GitHub, this API enables developers to integrate speech synthesis into applications with ease. It provides an accessible way to interact with OpenAI's speech generation technology, offering various customization options.
Key Features of the API:
- High-quality, natural-sounding voice output.
- Support for multiple languages and accents.
- Simple integration with existing applications.
- Customizable voice tone and speech rate.
Steps to Get Started:
- Clone the repository from GitHub.
- Set up the necessary dependencies.
- Configure your API keys and authentication settings.
- Start generating speech from text input.
Note: Be sure to review the documentation on GitHub for specific setup instructions and examples to get the most out of the API.
API Usage Example:
Input | Output |
---|---|
"Hello, world!" | A spoken version of "Hello, world!" in a clear and natural voice. |
"How are you today?" | A friendly and conversational tone of speech. |
OpenAI Speech Synthesis API on GitHub: A Practical Guide
OpenAI's Text to Speech API, available on GitHub, offers developers an efficient way to integrate speech synthesis into applications. With this tool, users can convert written text into natural-sounding audio, opening a wide range of possibilities for accessibility, content creation, and more. In this guide, we’ll walk through the setup process, key features, and best practices for using the API effectively.
Whether you're building a chatbot with voice interaction or creating an audiobook application, this API provides flexibility and quality. It supports a variety of voices and languages, and can be easily customized to fit different use cases. Let's dive into the key aspects of using the OpenAI Text to Speech API and how to start integrating it into your project.
Getting Started with the API
To begin using the Text to Speech API, you need to set up a few things on GitHub. Below is a step-by-step guide on how to install and configure the API:
- Clone the repository from GitHub using the following command:
git clone https://github.com/openai/text-to-speech.git
- Navigate to the project folder:
cd text-to-speech
- Install the necessary dependencies:
pip install -r requirements.txt
- Obtain your API key by signing up on OpenAI's platform and add it to the configuration file.
- Start the API by running:
python app.py
Features and Capabilities
The API provides several useful features that can be tailored for specific needs:
- Voice Customization: Choose from a wide selection of natural-sounding voices in different languages.
- Audio Quality: Output audio in high-quality formats like MP3 or WAV.
- Speed and Pitch Control: Adjust the speed and pitch of the generated speech to match the desired tone.
- Real-Time Streaming: Stream speech as it's generated for interactive applications.
Tip: Be mindful of the rate limits set by the OpenAI platform to avoid service interruptions. You can monitor your usage in the OpenAI dashboard.
Example Use Case
Here’s an example of how the API can be integrated into a Python script to convert text to speech:
import openai openai.api_key = 'your-api-key' response = openai.Audio.create( model="text-to-speech", prompt="Hello, welcome to the OpenAI Text to Speech API!", format="mp3" ) with open("output.mp3", "wb") as audio_file: audio_file.write(response['data'])
Conclusion
With the OpenAI Text to Speech API, developers have access to a powerful tool for adding voice capabilities to their applications. By following the setup guide and utilizing the key features, you can create sophisticated applications with natural-sounding speech. Make sure to explore the repository on GitHub for further documentation and updates.
How to Configure the OpenAI Text-to-Speech API on GitHub
To integrate the OpenAI Text-to-Speech API into your project, you'll first need to set up the necessary credentials and prepare your GitHub repository. This involves creating a new repository, installing required dependencies, and configuring environment variables to connect to the OpenAI service. The steps outlined here will guide you through the process of setting up the API efficiently and correctly.
Once you have your GitHub repository ready, follow the steps below to complete the configuration of the OpenAI Text-to-Speech API. This guide assumes you have basic knowledge of GitHub, API keys, and Python programming.
Steps for Setting Up
- Create a New GitHub Repository:
- Go to GitHub and create a new repository for your project.
- Choose a descriptive name and initialize it with a README file.
- Clone the Repository: Clone the repository to your local machine using Git.
- Run the following command in your terminal:
git clone https://github.com/your-username/repository-name.git
- Run the following command in your terminal:
- Install Dependencies: Install necessary libraries and dependencies for your project.
- Ensure you have Python 3.x installed on your machine.
- Install required packages using pip:
pip install openai requests
- Set Up API Credentials:
- Go to OpenAI’s website and obtain your API key from the account settings page.
- Store this key in your environment variables for secure access:
export OPENAI_API_KEY="your-api-key"
Example Code for Text-to-Speech Integration
After setting up your environment, you can use the following Python code to call the OpenAI Text-to-Speech API and generate speech from text:
import openai import os openai.api_key = os.getenv("OPENAI_API_KEY") response = openai.Audio.create( model="whisper-1", prompt="Hello, welcome to the OpenAI Text-to-Speech API setup!", format="mp3" ) with open("output.mp3", "wb") as f: f.write(response['audio_data'])
Testing and Deployment
Once your code is ready, test the integration by running your script locally. If everything works, you can push your changes to GitHub and deploy your project to a web server or cloud platform.
Common Issues
Issue | Solution |
---|---|
API Key Not Found | Ensure your API key is set correctly in the environment variables. |
Missing Dependencies | Check if all required packages are installed and up to date. |
Integrating OpenAI Speech Synthesis API into Your Application
To integrate OpenAI's speech synthesis API into your application, you first need to set up an API key and ensure proper configuration in your development environment. The API provides robust text-to-speech capabilities, enabling developers to convert written content into high-quality, human-like audio. This integration can enhance user experiences by adding natural voice interactions to your app, making it more accessible and engaging.
Once your environment is configured, you can easily connect to the API via HTTP requests. This allows you to send text input and receive audio output in various formats. Here’s a step-by-step guide to help you integrate OpenAI’s text-to-speech functionality:
Steps to Implement the Text-to-Speech API
- Obtain an API Key: Visit the OpenAI platform to generate your API key. This key is essential for authenticating your requests.
- Install Dependencies: Ensure you have the required libraries to interact with the API, such as requests or axios for HTTP requests in your programming language of choice.
- Make an API Request: Structure a POST request with the necessary parameters, including the text you want to convert to speech and other optional settings like voice or speed.
- Handle the Response: The API will return an audio file (e.g., in WAV or MP3 format) which you can save or stream within your application.
Example API Request
POST https://api.openai.com/v1/audio/generate Content-Type: application/json Authorization: Bearer YOUR_API_KEY { "text": "Hello, welcome to our app!", "voice": "en_us_male", "output_format": "mp3" }
Important Considerations
Make sure to respect the API usage limits and rate restrictions to avoid service interruptions.
Here's an example of the response format:
Parameter | Value |
---|---|
status | success |
audio_url | https://api.openai.com/audio/response.mp3 |
By following these steps, you can integrate OpenAI’s advanced text-to-speech capabilities into your application and offer users a more dynamic, voice-enabled interface.
Configuring API Keys for Secure Access to OpenAI’s TTS Services
When integrating OpenAI’s Text-to-Speech (TTS) API into your application, securing your API keys is crucial to prevent unauthorized access. Proper management of API keys ensures that your TTS service remains protected and that the access rights are correctly configured for authorized users. This guide provides an overview of how to securely configure your API keys for OpenAI’s TTS services.
Follow these steps to properly configure and manage your API keys for OpenAI’s TTS API. By following best practices, you can ensure both the security of your service and the integrity of your application’s functionality.
Steps to Configure API Keys
- Generate an API Key: Visit the OpenAI developer platform and create a new API key under your account settings.
- Store the Key Securely: Never expose your API key directly in your source code. Use environment variables or secure vault solutions to store the key safely.
- Assign Permissions: Ensure that the key you generate has the appropriate permissions only for the actions your application requires.
- Set Expiration (Optional): Consider setting an expiration date for the API key to reduce the risks of long-term exposure.
Key Best Practices
- Use environment variables for storing the API key in your application’s runtime environment.
- Restrict API key usage to specific IP addresses or domains to prevent unauthorized access from unexpected sources.
- Rotate keys periodically to minimize the impact of a compromised key.
- Implement detailed logging to monitor any suspicious API usage or failed attempts.
Important: Never expose your API keys publicly, even in version-controlled code repositories like GitHub. Use tools such as GitHub Secrets or encrypted storage solutions to safeguard your credentials.
Example API Key Configuration
Environment Variable | Value |
---|---|
OPENAI_API_KEY | sk-XXXXXXXXXXXX |
By following these practices, you can ensure secure and reliable access to OpenAI’s TTS API, minimizing the risk of unauthorized usage and protecting your service from potential misuse.
Understanding Supported Languages and Voices in OpenAI Text to Speech API
The OpenAI Text to Speech API offers a diverse set of languages and voices, catering to a global audience. By leveraging advanced machine learning models, it allows users to generate natural-sounding speech in a variety of accents and tones. This flexibility ensures that developers can integrate realistic text-to-speech functionality into their applications, while also accommodating different linguistic and cultural nuances.
When choosing a language or voice, it’s important to understand the available options and how they impact the overall user experience. The API supports several languages, each offering distinct voices with various characteristics such as pitch, speed, and emotion. Below is a detailed overview of the supported languages and voices.
Supported Languages and Voice Options
- English (US, UK, Australia, India, etc.)
- Spanish (Spain, Mexico, etc.)
- French (France, Canadian, etc.)
- German
- Italian
- Portuguese (Brazil, Portugal)
- Chinese (Mandarin)
- Japanese
- Korean
Voice Customization Features
The API provides several options to customize the voice, such as:
- Pitch: Adjusting the tone of speech, from low to high.
- Speed: Controlling how fast or slow the text is spoken.
- Emotion: Adding nuances to the speech like happiness, sadness, or excitement.
"The OpenAI Text to Speech API allows for high levels of customization, enabling developers to create a more personalized and dynamic auditory experience."
Example of Language and Voice Table
Language | Available Voices |
---|---|
English (US) | Matthew, Joanna, Kendra |
Spanish (Mexico) | Enrique, Lupe |
French (France) | Celine, Mathieu |
Optimizing Audio Output Quality with OpenAI’s TTS Settings
When working with OpenAI's Text-to-Speech API, fine-tuning the audio output quality can significantly enhance the user experience. Several key parameters affect the clarity, naturalness, and overall performance of the speech synthesis. By adjusting specific settings, developers can ensure a smoother, more engaging auditory experience for listeners.
Among the most important adjustments are voice selection, speech rate, and pitch variation. Proper configuration of these settings can improve the intelligibility and emotional tone of the generated speech, creating a more lifelike and engaging audio output. Below are essential considerations and best practices for optimizing your TTS audio output.
Key Settings for Audio Quality Enhancement
- Voice Selection: Choosing the right voice model is crucial for achieving natural-sounding speech. OpenAI offers a variety of voices with distinct characteristics. Select the voice that best suits your content, whether it’s a neutral tone for formal settings or a more expressive one for casual dialogues.
- Speech Rate: The speed at which the TTS system generates speech affects the listener's ability to comprehend the audio. Too fast and it may sound rushed; too slow and it may become monotonous. Typical values for a natural pace range between 0.8x and 1.2x of the default rate.
- Pitch Variation: Modulating pitch helps make speech sound more dynamic and less robotic. For a more engaging experience, experiment with slight pitch variations to introduce natural-sounding inflections.
Advanced Techniques for Fine-Tuning Audio
- Adjusting the Volume: Consistent volume levels are key to preventing distortion. Set the volume to a level that matches the intended output device and environment.
- Audio Format Optimization: Depending on the target medium (e.g., mobile app, web player, or podcast), adjusting the audio format and compression can reduce file size without compromising quality.
- Context-Aware Speech Adjustments: Some APIs allow adjustments based on context. For instance, a conversational tone can be activated for interactive dialogues, while a formal tone may be better suited for instructional content.
Important Considerations
“The success of TTS output depends not only on the settings but also on the context in which the audio will be used. Always test different configurations in real-world scenarios to find the optimal balance.”
Setting | Recommended Range | Impact on Quality |
---|---|---|
Speech Rate | 0.8x - 1.2x | Enhances clarity and comprehension |
Pitch | -1 to +1 | Improves naturalness and emotional tone |
Volume | 80% - 100% | Prevents distortion while ensuring clarity |
Monitoring and Managing API Usage through GitHub Repositories
Managing API usage is crucial to ensure optimal performance and avoid overages or misuse. GitHub repositories can serve as a central hub for monitoring and controlling how APIs are being accessed, especially in the case of integration with services such as OpenAI's Text to Speech API. By utilizing various GitHub tools and strategies, developers can track API calls, manage authentication, and implement rate-limiting or other protective measures. Proper setup in a repository allows for continuous monitoring of API usage across different environments and users, making it easier to maintain system integrity.
In addition to basic tracking, GitHub repositories offer the advantage of version control, collaboration, and automated workflows to help manage API interactions efficiently. This setup ensures that teams are aware of usage patterns, can quickly identify problems, and make necessary adjustments. Below are several practical steps and tools that developers can leverage within GitHub to effectively manage API usage.
Key Techniques for API Usage Monitoring
- Logging API Requests: By storing detailed logs of every API call, including timestamps and status codes, you can track usage patterns and detect issues early.
- Rate Limiting: Use GitHub Actions or other automation tools to enforce rate limits on API calls to avoid hitting service caps.
- API Key Management: Manage your API keys securely within GitHub, using environment variables or encrypted secrets to limit access and prevent leaks.
Best Practices for GitHub Repository API Integration
- Ensure API credentials are securely stored in GitHub Secrets to prevent unauthorized access.
- Implement GitHub Actions to automate tasks like checking API usage or notifying team members when thresholds are approached.
- Utilize status badges in your repository to show the current health or status of the API integration (e.g., number of successful calls, remaining quota).
Example of API Usage Overview
Metric | Value |
---|---|
Total API Calls | 10,500 |
Successful Responses | 10,200 |
Failed Requests | 300 |
Remaining Quota | 150 |
Important: Always keep track of your remaining API usage to avoid unexpected disruptions in service. GitHub's integration with CI/CD workflows can assist in automating this process.
Debugging Common Issues When Using OpenAI’s Text to Speech API
When working with OpenAI’s text-to-speech capabilities, developers may encounter a variety of issues that prevent smooth integration. These issues can arise from API usage, configuration errors, or external dependencies. Knowing how to identify and fix these problems can save considerable time during development and help in achieving the desired speech synthesis results. Below are common challenges and how to troubleshoot them effectively.
Here are some frequent issues users experience when utilizing the Text to Speech API, along with their possible causes and solutions.
1. Invalid API Key or Authentication Errors
One of the most common problems encountered during integration is authentication failure due to an incorrect or expired API key. The API key is required to authenticate your requests to OpenAI’s servers, and any mismatch or invalidation can lead to access errors.
- Ensure the API key is correctly pasted into your request headers.
- Check if the key is expired or has been revoked by logging into your OpenAI dashboard.
- Verify that you are using the correct endpoint and API version.
Important: Always keep your API keys secure and avoid exposing them in public repositories.
2. Audio Output Quality Issues
Users may sometimes notice that the generated speech output does not meet expectations in terms of clarity or pronunciation. This issue can stem from several factors, including input text formatting or incorrect model selection.
- Ensure that the input text is properly formatted, especially when using special characters or punctuation marks.
- Check the voice model being used. Some models may have different output characteristics, so switching between models can improve quality.
- Consider adjusting the speech rate and pitch parameters to suit the desired result.
3. Rate Limiting or Quota Exhaustion
API usage limits can often lead to rate-limiting errors if the quota has been exceeded. This is particularly common in high-traffic applications or when multiple requests are being made in a short period.
Error Code | Cause | Solution |
---|---|---|
429 | Rate limit exceeded | Upgrade your plan or implement request throttling to manage API calls more effectively. |
403 | Quota exceeded | Check your current usage and consider increasing your API plan's limits. |
Note: Regularly monitor your API usage to ensure you stay within the allowed limits.
Enhancing Your App with New Capabilities from OpenAI's Text-to-Speech API
Integrating the latest updates from OpenAI's Text-to-Speech API allows you to significantly enhance your application. These new features improve voice quality, expand language options, and offer greater flexibility in user interaction. Updating your app with these additions ensures that your users experience smoother and more natural-sounding speech synthesis.
With each new version of the API, OpenAI introduces new functionalities that help developers fine-tune voice output, control tone, and even integrate more advanced customization options. By staying up-to-date with these features, you can provide your users with an engaging and dynamic experience, tailored to your application's needs.
Steps to Implement New Features
- Review the changelog for the latest API version to identify relevant updates.
- Update your API integration code by replacing deprecated functions and adding new endpoints as needed.
- Test the new voice features and fine-tune parameters such as pitch, speed, and tone to match your application's requirements.
Important: Ensure that you have the correct authentication credentials for the new API version to avoid issues when making requests.
Example: Adjusting Voice Parameters
Below is an example of how you might configure the new voice parameters in your application:
api.synthesizeSpeech({ voice: "en-US-Wavenet-D", pitch: 0.5, speed: 1.2, tone: "calm" });
This configuration allows you to fine-tune the voice output for a more natural-sounding response. By using specific parameters, you can adjust how the text is spoken in your app.
Key Changes in the API
Feature | Description |
---|---|
Multiple Language Support | New voices in additional languages, enhancing global accessibility. |
Custom Voice Profiles | Ability to create unique voices tailored to your app's requirements. |
Improved Speech Clarity | Optimized algorithms for clearer, more human-like speech. |
Make sure to test the new language models in different real-world conditions to ensure they meet your quality standards.