R Create a Vector of Vectors
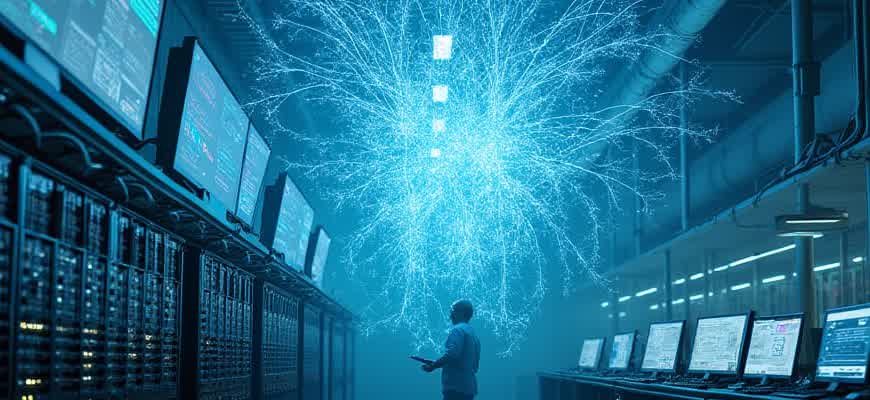
In R, vectors are essential data structures used for storing multiple elements. When dealing with more complex datasets, you may find it useful to create a vector of vectors. This approach allows you to group different vectors together, which can be particularly beneficial for organizing data with varying structures.
To create a vector of vectors, you can use the list() function, which is a versatile structure that holds vectors as its elements. Below is an example illustrating how to build and access a vector of vectors in R:
- First, create individual vectors:
- Vector 1:
v1 <- c(1, 2, 3)
- Vector 2:
v2 <- c("A", "B", "C")
- Then, combine them into a list:
nested_vectors <- list(v1, v2)
- Access elements from the list using indexing:
nested_vectors[[1]]
returnsv1
Remember, unlike a standard vector, a list in R can hold elements of different types and sizes. This makes it ideal for storing vectors that might vary in length or content.
Command | Action |
---|---|
v1 <- c(1, 2, 3) |
Creates a numeric vector |
nested_vectors <- list(v1, v2) |
Creates a list containing vectors v1 and v2 |
Mastering R: Constructing a Vector of Vectors
In R, a vector of vectors allows you to group multiple vectors into a single data structure, enabling more complex and flexible data manipulation. This method is particularly useful when dealing with heterogeneous data or when organizing data in a nested structure. The main challenge lies in correctly indexing and extracting specific elements from this composite structure, as each inner vector may have its own distinct properties and lengths.
To build a vector of vectors in R, you can leverage the list function, as it can hold multiple vectors, each of different lengths or types. Below is a simple guide for constructing and manipulating a vector of vectors.
Steps to Create and Access a Vector of Vectors
- Create a basic vector using the c() function.
- Group these vectors together using list().
- Access individual elements using [[] or [[]].
- Use loops or apply functions for more complex operations on the entire structure.
Example
Here's an example of creating a vector of vectors and accessing its elements in R:
# Create individual vectors vec1 <- c(1, 2, 3) vec2 <- c(4, 5, 6) vec3 <- c(7, 8, 9) # Create a vector of vectors vector_of_vectors <- list(vec1, vec2, vec3) # Access elements vector_of_vectors[[1]] # Outputs: 1 2 3
Table: Comparison of Different Methods
Method | Action | Purpose |
---|---|---|
c() | Combine elements into a vector | To create individual vectors |
list() | Group multiple vectors together | To form a vector of vectors |
[[ ]] | Access elements within the list | To extract a specific vector |
Mastering this technique will help you manage and manipulate complex datasets more efficiently, whether you are analyzing time series data, conducting simulations, or organizing large-scale information.
How to Create and Initialize a Nested Vector in R
In R, creating a vector of vectors is a straightforward process. A vector of vectors is essentially a list where each element is itself a vector. This structure is often useful when dealing with multi-dimensional data or when you need to represent data in a nested format. You can initialize such a structure using various methods, depending on the specific use case.
One of the most common ways to create a vector of vectors is by using the `list()` function. This function allows you to define each element of the list as a vector. Another approach involves combining vectors into a single structure using the `c()` function, but it is generally more efficient to work with lists for nested data. The flexibility of lists in R makes them ideal for handling vectors of varying lengths.
Steps to Initialize a Vector of Vectors
- Create a basic vector using the c() function.
- Group the vectors inside a list() to form the vector of vectors.
- Access and manipulate individual vectors within the list using indexing.
Important Note: A list is different from a vector in R because a list can hold elements of different types and lengths, while a vector requires all elements to be of the same type.
Example Code
# Creating individual vectors
vector1 <- c(1, 2, 3)
vector2 <- c(4, 5, 6)
vector3 <- c(7, 8, 9)
# Initializing a vector of vectors (list)
vector_of_vectors <- list(vector1, vector2, vector3)
# Accessing a specific vector
vector_of_vectors[[2]] # Returns the second vector (vector2)
Key Differences Between Vectors and Lists
Vectors | Lists |
---|---|
All elements must be of the same type. | Elements can be of different types and lengths. |
Accessed with single square brackets (e.g., vector[1]). | Accessed with double square brackets (e.g., list[[1]]). |
Typically used for homogeneous data. | Used for heterogeneous or nested data. |
Understanding Nested Data Structures: Vectors Inside Vectors
In R, the concept of nested data structures is fundamental for organizing and managing complex datasets. One common example of this is the use of vectors within other vectors, allowing for a multi-dimensional array-like structure. This technique enables the storage of various types of data in a compact, yet flexible format, which is useful when dealing with complex datasets such as time series or multidimensional measurements.
By creating vectors inside vectors, it becomes possible to group related elements together while maintaining the ability to access them individually. This type of structure can represent hierarchical relationships or organize data in a way that is easy to manipulate. Below, we explore how this nested structure can be implemented and accessed in R.
How to Create Nested Vectors
A vector inside another vector is essentially a list of vectors. Here’s a basic way to create such a structure in R:
nested_vector <- list(c(1, 2, 3), c(4, 5, 6), c(7, 8, 9))
This example creates a list containing three vectors, each with three elements. The result is a list of vectors, which can be accessed and manipulated individually.
Accessing Data in Nested Vectors
To work with elements inside nested vectors, we use indexing. The first level of indexing accesses the vector itself, and the second level accesses individual elements within that vector:
nested_vector[[1]] # Access the first vector: c(1, 2, 3) nested_vector[[1]][2] # Access the second element of the first vector: 2
In this case, the double square brackets [[ ]] are used to access the entire vector within the list, while the single square brackets [ ] are used to access specific elements within the vector.
Advantages of Using Nested Vectors
- Organized Data: Nested vectors allow for logical grouping of related data.
- Flexible Structure: Each vector can have a different length or data type, providing flexibility in data storage.
- Efficient Data Access: Indexing makes it easy to retrieve or modify specific elements in large datasets.
Example: Storing Multi-Dimensional Data
Consider the following example where nested vectors are used to store data from multiple experiments:
Experiment | Data |
---|---|
Exp 1 | c(1.2, 2.3, 3.4) |
Exp 2 | c(4.5, 5.6, 6.7) |
Exp 3 | c(7.8, 8.9, 9.0) |
Nested vectors are particularly useful for storing data that varies across different dimensions or categories, such as experimental results or time-series data.
Accessing Elements in Nested Vectors in R
In R, a vector of vectors is essentially a list where each element is itself a vector. To retrieve specific elements from these nested vectors, one must understand the proper indexing methods. R uses double square brackets for accessing sub-vectors and single square brackets for extracting individual elements within those sub-vectors. This distinction is essential for effectively manipulating nested data structures.
When dealing with a vector of vectors, it’s important to distinguish between accessing the entire sub-vector or specific values inside them. The ability to navigate through such structures is crucial for efficient data analysis in R, especially when handling more complex datasets like matrices or lists of lists.
Accessing Data from Nested Vectors
- Extracting a complete sub-vector: Use double square brackets to access a full sub-vector, for example:
vector_list[[1]]
returns the first sub-vector. - Accessing specific elements: To retrieve a value from a sub-vector, use the combination of double and single square brackets:
vector_list[[1]][2]
gets the second element of the first sub-vector. - Getting multiple sub-vectors: If you need several sub-vectors, use a range with single square brackets:
vector_list[1:3]
returns the first three sub-vectors.
Example of a Vector of Vectors
Index | Sub-Vector |
---|---|
1 | c(1, 2, 3) |
2 | c(4, 5, 6) |
3 | c(7, 8, 9) |
Important: Single square brackets return the sub-vector, while double square brackets provide access to a single element within the vector.
Code Example
Consider this vector of vectors:
vector_list <- list(c(1, 2, 3), c(4, 5, 6), c(7, 8, 9))
To access the value "6" from the second sub-vector, the following code would be used:
vector_list[[2]][3]
This command first retrieves the second sub-vector c(4, 5, 6)
and then accesses the third element, returning "6".
Common Issues When Working with Nested Vectors and How to Resolve Them
When working with nested vectors in R, it's easy to run into some common pitfalls. These can include issues related to the structure of the data, improper indexing, or unintentional changes in the type of elements within the vectors. Understanding the typical mistakes and knowing how to address them is crucial for smooth data manipulation in R.
In this section, we will look at some of the most frequent errors users encounter when working with vectors of vectors and provide simple solutions to fix them. Whether you're trying to subset a vector or manipulate the elements within, these tips will help you avoid frustration.
1. Incorrect Indexing of Nested Vectors
One of the most common mistakes when working with nested vectors is incorrect indexing. In R, when you use double square brackets ([[ ]]), you are extracting an element from a vector of vectors. However, users often mistakenly use a single bracket ([ ]) which results in a list instead of the expected vector.
- Incorrect: my_vector[1][2] (This returns a list, not a vector element.)
- Correct: my_vector[[1]][2] (This correctly extracts the second element from the first vector in the list.)
2. Uneven Vector Lengths
Another issue occurs when the sub-vectors within a nested vector have unequal lengths. This can lead to unexpected behavior when performing operations across the vectors, such as when applying a function to each sub-vector.
Solution: Ensure that all sub-vectors have the same length, or handle them individually using looping structures or `lapply()`.
- Example: If each sub-vector represents data for a different year, make sure the number of data points per year is consistent.
- Fix: Use padding or trimming to align vector lengths.
3. Changing Data Types Across Vectors
Sometimes, the vectors within the list might unintentionally change their data types. For instance, you might start with numeric vectors but end up with a mix of characters and numerics. This happens if you perform operations that return different data types or inadvertently combine different types of data into the same vector.
Vector | Data Type |
---|---|
c(1, 2, 3) | Numeric |
c("a", "b", "c") | Character |
c(1, "b", 3) | Character (mixed type) |
Solution: Check the types of data in your vectors before performing operations and convert them to a consistent type using `as.numeric()` or `as.character()` as needed.
Modifying Specific Elements in a Vector of Vectors in R
In R, a vector of vectors can be thought of as a list of vectors where each vector may contain different lengths of elements. Modifying an individual element within such a structure requires a clear approach to identify both the outer vector and the target element within it. Understanding how to access and alter elements effectively is crucial for data manipulation tasks in R.
To modify an element, first, you need to reference the specific index of the outer vector, followed by the index of the element within the inner vector. This method allows for precise control over the data within each vector.
Accessing and Modifying Elements
- Use the double bracket notation to access and modify elements in the vector of vectors.
- First specify the position of the outer vector, then the inner vector’s element you want to modify.
- Assign a new value directly to that position.
Important: Always ensure that the indices you use are within the range of the vector, otherwise R will return an error or NA.
Example
- Consider the following vector of vectors:
v <- list(c(1, 2, 3), c(4, 5, 6), c(7, 8, 9))
. - To modify the second element of the first vector, you can use:
v[[1]][2] <- 99
. - This changes the value of the second element in the first vector from 2 to 99.
Summary of Key Operations
Operation | Example |
---|---|
Accessing an Element | v[[2]][3] (Accesses the third element of the second vector) |
Modifying an Element | v[[3]][1] <- 100 (Modifies the first element of the third vector) |
Performance Considerations for Large Arrays of Arrays
Working with large collections of vectors, especially in a language like R, presents unique challenges when it comes to memory management and processing efficiency. A vector of vectors can quickly consume substantial system resources, particularly when the nested vectors are large or the total number of elements is high. This can lead to slow execution times, memory fragmentation, and even crashes if the system runs out of available memory. It’s essential to consider various strategies to mitigate these issues while handling large datasets efficiently.
Optimizing performance when dealing with arrays of arrays requires an understanding of R's internal data structures and memory allocation. Improper handling can result in performance bottlenecks, especially with operations that require frequent resizing or copying of vectors. Here, we will explore several key considerations and strategies for improving the handling of such structures.
Memory Usage and Allocation
Memory management plays a crucial role when dealing with large datasets. R allocates memory dynamically, which means that resizing vectors or lists can cause significant overhead, particularly with nested structures.
- Memory Fragmentation: Storing a large number of vectors inside a vector can cause fragmentation. When resizing vectors, R may need to allocate new memory blocks and copy the data over, which can be inefficient for large structures.
- Pre-allocating Space: It is recommended to pre-allocate memory whenever possible. This approach helps avoid the repeated reallocation of memory and enhances performance, especially with nested vectors.
- Using Lists Instead of Vectors: Lists in R can be more efficient than vectors for certain types of data. Unlike vectors, lists do not require contiguous memory blocks, which allows for better memory management when dealing with large, complex structures.
Efficient Access Patterns
Accessing elements in large arrays of vectors can be costly in terms of time complexity. Optimizing access patterns is essential for improving performance.
- Vectorization: Whenever possible, use vectorized operations instead of loops. R is optimized for vectorized computations, and this can significantly speed up your code.
- Avoiding Nested Loops: Nested loops over large vectors of vectors can be slow. Instead, explore alternative approaches, such as applying functions to vectors or using apply-family functions like lapply and sapply.
- Efficient Indexing: Accessing elements through efficient indexing methods, such as using integer indices instead of logical indices, can help speed up data retrieval.
Performance Testing
Testing the performance of your code is an essential part of optimization. Identifying where bottlenecks occur can help you make informed decisions on how to improve efficiency.
Method | Description | Use Case |
---|---|---|
Profiling | Using R’s Rprof or microbenchmark tools to measure execution time | To identify slow functions or bottlenecks in the code |
Memory Usage Analysis | Monitoring memory consumption with the pryr package | To ensure that memory is allocated efficiently and avoid memory leaks |
Important: Always keep in mind that performance optimization should be done iteratively. Start by identifying the most critical parts of your code before implementing changes.
Practical Applications of Nested Vectors in Data Analysis
In data analysis, the use of nested structures such as vectors of vectors can greatly simplify the organization and manipulation of complex datasets. These structures are especially useful when dealing with multi-dimensional data or hierarchical datasets where each element contains further sub-elements. By leveraging vectors of vectors, analysts can efficiently model relationships between different data points, facilitating tasks like clustering, group analysis, or even time-series forecasting across different groups or categories.
Vectors of vectors allow for easy categorization of data, such as when each group holds data in its sub-elements that need to be processed independently but still within a larger context. This approach provides flexibility when managing data across various levels of granularity, making it particularly effective for grouping and summarizing information based on multiple attributes.
Examples of Real-World Applications
- Time-Series Analysis: For financial data, nested vectors can store data points grouped by time periods, such as daily or monthly stock prices, where each sub-vector represents prices for different companies.
- Survey Data: When analyzing responses from multiple survey questions, each question's answers can be stored in its own vector, and all question vectors are contained within a larger vector representing the full survey dataset.
- Customer Segmentation: Groups of customers can be represented by vectors of vectors, where each sub-vector contains demographic or purchasing behavior data specific to each group.
Advantages of Using Nested Vectors
- Organized Data Structure: Grouping data into nested vectors maintains clarity and organization, especially for datasets that need to preserve sub-relationships.
- Efficient Data Manipulation: Operations like filtering, applying functions, or summarizing data become straightforward when working with nested structures.
- Enhanced Scalability: The flexibility of vectors of vectors ensures that the model scales well as the dataset expands, which is crucial for handling large datasets with multiple variables.
Note: Nested vectors are particularly useful in machine learning pipelines where features are grouped in complex ways. Their ability to store and organize diverse data structures can lead to more efficient data preparation and analysis processes.
Use Case Table
Application | Nested Vector Use | Benefit |
---|---|---|
Financial Time Series | Daily stock prices grouped by company | Facilitates analysis of trends per company over time |
Market Research | Customer feedback grouped by demographic segment | Enables focused insights based on customer profiles |
Healthcare Data | Patient visits grouped by medical condition | Improves targeting of healthcare interventions by condition |
Best Practices for Organizing Complex Data with Nested Vectors
When working with complex datasets in R, using vectors of vectors allows for better organization and flexibility. This structure enables the representation of multidimensional data, such as matrices or lists of lists, which is often encountered in real-world problems. However, effective management of such structures requires careful planning and adherence to best practices to ensure clarity and ease of use.
To structure data efficiently, it’s important to focus on organization, indexing, and performance. Following guidelines that improve code readability, prevent unnecessary complexity, and ensure that data is accessible and modifiable will significantly enhance the process of managing nested vectors.
Key Considerations for Structuring Nested Vectors
- Consistency in Data Types: Ensure that each vector within the vector of vectors has a consistent data type. Mixed types can complicate operations and decrease performance.
- Meaningful Indexing: Use descriptive names for your indices whenever possible, especially for large datasets. It makes accessing specific elements easier and reduces confusion.
- Efficient Memory Usage: Avoid redundant data storage. Consider using data frames or matrices if they provide a more efficient structure for your use case.
- Data Validation: Regularly check the integrity of your data. Nested vectors can be prone to inconsistencies if modifications are not properly tracked.
Examples of Nested Vector Use Cases
- Multi-level Lists: For a dataset that includes hierarchical information, a vector of vectors can represent each level of the hierarchy.
- Time-series Data: A vector of vectors can hold different time periods (e.g., days or years) as separate vectors within the main structure.
- Geospatial Data: Nested vectors are useful for storing coordinates, with each inner vector representing a set of geographical points.
When to Avoid Vectors of Vectors
For datasets that require complex operations such as statistical analysis or machine learning, using a data frame or matrix might be a better alternative. Nested vectors can become unwieldy when data manipulation tasks increase in complexity.
Comparison Table: Vectors of Vectors vs. Data Frames
Feature | Vectors of Vectors | Data Frames |
---|---|---|
Ease of Indexing | Moderate | High |
Memory Efficiency | Low | High |
Flexibility | High | Moderate |
Data Manipulation Support | Low | High |