Google Text to Speech Api Demo
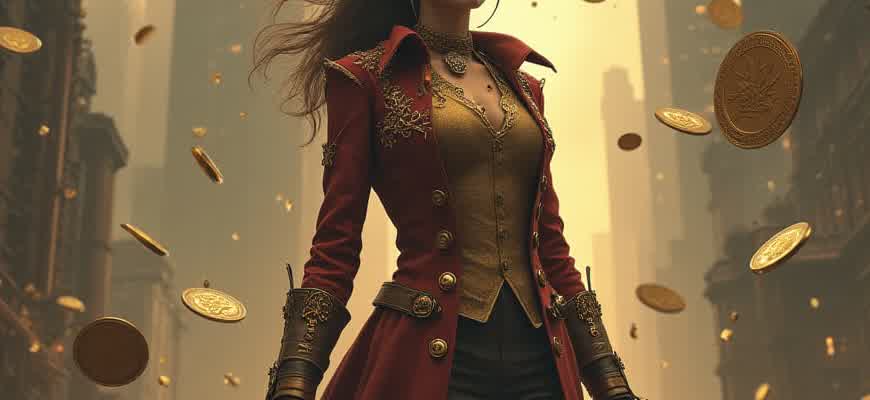
The Google Text-to-Speech API provides developers with the ability to integrate high-quality speech synthesis into their applications. This service is part of Google Cloud's AI suite, offering support for multiple languages and voices. Below is a basic overview of its features and capabilities:
- Multiple voice options in over 30 languages.
- Real-time streaming and batch synthesis for different use cases.
- Advanced control over speech rate, pitch, and volume gain.
Important: The API leverages Google's advanced neural network models, offering natural-sounding speech synthesis with various customization options.
To get started with the API, users must set up a Google Cloud project and enable the Text-to-Speech service. Here's a quick guide on how to proceed:
- Create a new project in the Google Cloud Console.
- Enable the Text-to-Speech API from the library.
- Generate API keys for authentication.
- Install the required client libraries and SDKs.
Once the setup is complete, developers can interact with the API using simple REST calls, sending text input and receiving audio output in various formats such as MP3 or WAV.
Feature | Details |
---|---|
Voice Selection | Multiple voice options (male, female, and neutral voices) |
Languages Supported | Over 30 languages including English, Spanish, French, and Japanese |
Audio Output Formats | MP3, OGG, WAV |
Google Text to Speech API Demo: A Practical Guide
The Google Text to Speech API provides developers with an easy-to-implement solution for converting written text into natural-sounding speech. This service supports multiple languages and voices, enabling integration into various applications, including virtual assistants, accessibility tools, and customer service systems. By using the API, developers can enhance user experience by offering a more interactive and accessible platform.
In this guide, we'll demonstrate how to use the Google Text to Speech API effectively by walking through key features and providing a step-by-step approach for setting up a demo. You'll be able to understand the core functionalities and start integrating the service into your own projects.
Key Features of Google Text to Speech API
- Multiple Languages and Voices: Choose from a variety of languages and accents to generate the most appropriate speech for your audience.
- SSML Support: Customize speech output with Speech Synthesis Markup Language (SSML) to control prosody, pitch, and speed.
- Real-time Streaming: The API supports real-time conversion of text into speech, ideal for live applications.
- Cloud Integration: The API is cloud-based, allowing easy scaling and integration into existing cloud infrastructure.
Steps to Set Up Google Text to Speech API
- Enable the API: Go to the Google Cloud Console, create a project, and enable the Text to Speech API.
- Authenticate: Set up the authentication using service accounts to securely access the API.
- Install SDK: Use the Google Cloud SDK to interact with the API in your preferred programming language.
- Make an API Request: Send a text input to the API, specifying language and voice parameters.
- Process the Audio: Once you receive the audio output, you can save it as an audio file or stream it directly to users.
Sample Response and Output
Request Parameter | Sample Input | Response |
---|---|---|
Text | "Hello, how are you today?" | Audio file generated |
Language | en-US | English (US) |
Voice | WaveNet-A | Male voice |
Important: Ensure that your Google Cloud billing account is enabled, as the Text to Speech API is a paid service. You may be eligible for a free tier depending on usage.
How to Begin Integrating Google Text-to-Speech API
The Google Text-to-Speech API allows developers to add high-quality text-to-speech capabilities to their applications. This service converts written text into natural-sounding speech, with support for various languages and voices. To integrate this API into your project, you need to go through a few key steps, including setting up the API, obtaining credentials, and making the API requests.
To get started, follow these steps and familiarize yourself with the necessary tools and setup. This guide provides a simple walkthrough for integrating Google Text-to-Speech into your application, ensuring smooth execution.
1. Set Up Your Google Cloud Project
- Sign in to the Google Cloud Console.
- Create a new project by clicking on "Select a Project" at the top and then choosing "New Project".
- Enable the Text-to-Speech API for your project. Navigate to "API & Services" and select "Enable APIs and Services". Search for "Cloud Text-to-Speech API" and enable it.
- Create and download API credentials in the form of a service account key. Go to the "Credentials" section, click "Create Credentials", and choose "Service Account Key".
2. Install Required Libraries
- Install the Google Cloud SDK or the necessary libraries for your programming language. For Python, you can use:
- Set up the environment variable for your credentials. This is done by exporting the path to your service account key as follows:
pip install --upgrade google-cloud-texttospeech
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/your/service-account-file.json"
3. Make Your First API Request
Once everything is set up, you can begin making requests to the Text-to-Speech API. The following Python code demonstrates a basic request:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.FEMALE ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech( request={"input": synthesis_input, "voice": voice, "audio_config": audio_config} ) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Important: Ensure your API credentials are set up correctly, as they are essential for making authenticated requests to the Google Cloud services.
4. Understanding API Options
Option | Description |
---|---|
Voice Selection | Choose from different voices (male/female) and languages (e.g., English, Spanish). |
Audio Encoding | Select the desired audio format for output (e.g., MP3, WAV). |
Speech Speed | Control the rate at which the speech is generated (e.g., normal, slow, fast). |
Once the basic integration is complete, explore additional features like customizing speech pitch, speed, or adding SSML (Speech Synthesis Markup Language) for more control over the output. With this setup, you can start integrating advanced speech capabilities into your application efficiently.
Setting Up API Credentials for Seamless Access
To begin using Google Text-to-Speech API, it is essential to configure your credentials in order to authenticate and authorize your requests. Without proper credentials, your application will not be able to access the API. Follow these steps to ensure a smooth setup process.
The first step involves creating a project in the Google Cloud Console and enabling the Text-to-Speech API. Once your project is ready, you will need to generate an API key or service account credentials to authenticate your application. Below is a step-by-step guide on how to set up the credentials.
Steps to Configure API Credentials
- Log into the Google Cloud Console and create a new project.
- Navigate to the API & Services section, and select Enable APIs and Services.
- Search for Text-to-Speech API and enable it for your project.
- Go to the Credentials section and click on Create Credentials.
- Choose between generating an API key or creating a Service Account for secure access.
Important: Service accounts are recommended for production environments as they provide enhanced security over API keys.
Managing API Credentials
Once your credentials are created, you must securely store them. API keys are often stored in environment variables, while service account keys are usually downloaded as a JSON file. Here’s an example of how you might manage them:
Credential Type | Usage | Storage Recommendation |
---|---|---|
API Key | Quick and easy for testing | Store in environment variables or a secure location |
Service Account Key | Recommended for production environments | Download as a JSON file and protect it securely |
Choosing the Right Voice Model for Your Application
When integrating speech synthesis into your application, selecting the appropriate voice model is crucial for providing the best user experience. Google Text-to-Speech API offers a variety of voice models, each with distinct features that can impact the effectiveness and accessibility of your application. Understanding these differences will help ensure that your app delivers clear, engaging, and natural-sounding speech output.
Choosing the right voice model depends on several factors such as the language, tone, and specific use case of your application. Below, we break down the available options to help you make an informed decision.
Types of Voice Models
Google Text-to-Speech API offers two main categories of voices: standard and WaveNet. Each of these models has its advantages depending on your application's needs.
- Standard Voices: These are suitable for applications where high speed and low latency are required. They offer a wide variety of languages and accents, though the quality may not be as natural as WaveNet voices.
- WaveNet Voices: Developed using deep neural networks, WaveNet voices provide more natural and human-like speech. These are ideal for applications where a more conversational tone is essential, but they may come with higher resource demands.
Key Considerations for Selecting the Right Model
- Quality: If the naturalness and clarity of the speech are a priority, WaveNet voices are the best option.
- Performance: If latency and quick response times are more important, standard voices can provide faster output without sacrificing much in terms of clarity.
- Resource Usage: WaveNet voices typically require more computational power, so if your application runs on resource-constrained devices, you may need to consider using standard voices.
- Localization: Check the available languages and accents for both voice models. While both support multiple languages, WaveNet voices may have a more limited selection compared to standard voices.
Comparing Voice Models
Feature | Standard Voices | WaveNet Voices |
---|---|---|
Naturalness | Moderate | High |
Response Time | Fast | Slower |
Resource Consumption | Low | High |
Language Support | Extensive | Limited |
Note: It is essential to balance performance with voice quality based on the specific requirements of your application. For interactive applications where user engagement is key, WaveNet might be the preferred choice. For utility-based applications, standard voices may be sufficient and more efficient.
Optimizing Speech Output Quality for Different Use Cases
When using speech synthesis technologies like the Google Text-to-Speech API, optimizing the output for specific applications is crucial. Various use cases, such as virtual assistants, navigation systems, and educational tools, each demand unique tuning of voice quality, tone, and speed to ensure clarity and engagement. Understanding how to fine-tune the speech synthesis process for different needs can improve the overall user experience.
Speech quality is influenced by multiple factors, such as the chosen voice model, speech speed, pitch adjustments, and context. A well-optimized solution will take these aspects into account to meet the distinct needs of the target application, from conversational AI to instructional content.
Key Optimization Strategies
- Voice Model Selection: Choose the appropriate voice model (e.g., WaveNet or standard models) based on the desired naturalness or clarity.
- Adjusting Speech Speed: Slow down the speech for educational tools or increase speed for fast-paced applications like navigation.
- Pitch and Intonation: Alter pitch for better emotional tone or adjust intonation for different contexts, such as formal announcements versus casual dialogue.
Application-Specific Considerations
Use Case | Key Considerations | Optimization Tips |
---|---|---|
Virtual Assistants | Natural-sounding, engaging voice | Use a conversational tone, moderate speed, and ensure responsiveness. |
Navigation Systems | Clarity and quick delivery | Increase speech speed, ensure clear articulation, and avoid unnecessary details. |
Educational Tools | Clear pronunciation, slow pace | Slow down the speech rate, focus on accurate pronunciation, and vary pitch for emphasis. |
"Optimizing speech output for specific use cases ensures that the message is communicated effectively and the user experience remains intuitive."
Handling Language and Accent Variations in Speech Synthesis
When working with speech synthesis systems, handling language and accent variations is crucial for ensuring the generated speech sounds natural and understandable to diverse audiences. Google Text to Speech API, like many others, offers a wide array of language and accent options. Correctly selecting these parameters allows the system to produce more accurate and culturally relevant speech outputs.
In the context of the Google API, managing these variations effectively involves selecting appropriate language codes and voices. It is important to choose the right combination of language and accent to match the target demographic, as this greatly influences the overall user experience.
Considerations for Language and Accent Handling
- Language Code Selection: Each supported language has a unique code that must be specified in the API request to ensure accurate pronunciation and grammar. For example, the language code "en-US" represents English spoken in the United States, while "en-GB" refers to British English.
- Voice Selection: Different voices are available for each language, offering a range of accents and styles. By default, the API uses a standard voice, but you can select from different variants based on gender, tone, and regional accent.
- Accents and Dialects: Some languages have multiple dialects or regional accents, such as "fr-FR" for French spoken in France, and "fr-CA" for French as spoken in Canada. These variations can be fine-tuned to improve the accuracy of speech synthesis.
Implementation Tips
- Test Speech Outputs: Always test various combinations of languages and voices to determine which option best suits the intended audience.
- Customize Pitch and Rate: Adjust the speech rate and pitch parameters for better clarity and more natural-sounding speech, especially in languages with complex intonations.
- Monitor for Mispronunciations: Pay attention to specific words that may be mispronounced due to accent differences and consider implementing fallback solutions if necessary.
"Choosing the right voice and accent settings can make a significant difference in how natural and effective the synthesized speech feels for end-users."
Example of Language and Accent Codes
Language Code | Language | Accent |
---|---|---|
en-US | English | American |
en-GB | English | British |
fr-FR | French | French (France) |
fr-CA | French | French (Canada) |
Implementing Real-Time Text-to-Speech Conversion in Web Applications
Real-time text-to-speech (TTS) conversion is an essential feature for enhancing user accessibility and creating more interactive web applications. By integrating a TTS system, developers can allow users to hear the text content, which is beneficial for individuals with visual impairments or reading difficulties. The implementation of TTS involves processing input text and transforming it into audible speech using an API such as Google's TTS API.
In web applications, real-time conversion involves the dynamic generation of speech from user-provided text. This is achieved through web APIs and JavaScript, which send text data to a server for processing. The server then returns an audio file, which can be played back to the user immediately. This process enhances user experience and opens up a range of interactive possibilities, including virtual assistants and automatic content narration.
Key Steps in Implementing Real-Time TTS
- Integrating the TTS API into your web application.
- Setting up a real-time connection to the server for text input.
- Processing the text and generating audio output in real-time.
- Ensuring seamless playback of the generated audio.
Implementation Workflow
- Text Input: The user provides the text to be converted.
- API Call: The input text is sent to the TTS API for processing.
- Audio Playback: The audio file returned by the API is played back in the user's browser.
- Real-Time Adjustments: Users can interact with the text or adjust settings such as speech speed and voice tone.
"Real-time TTS systems enhance web applications by providing accessibility features that improve engagement and inclusivity."
Example of TTS API Integration
Step | Action |
---|---|
1 | Set up API keys and authentication for the Google TTS service. |
2 | Send text input to the API endpoint using AJAX or Fetch API. |
3 | Handle the API response and trigger audio playback in the browser. |
Cost Management and Budgeting for Google Text to Speech API Usage
Efficient cost management is crucial when integrating the Google Text to Speech API into applications. This service charges based on usage, making it essential to carefully track and control expenses to avoid unexpected costs. Proper budgeting strategies ensure that your project remains within financial limits while benefiting from the capabilities of the API.
Google offers a flexible pricing model based on the amount of audio generated and the type of voice selected. There are different tiers for standard and premium voices, which can significantly affect the overall cost. Knowing how to manage usage efficiently will allow businesses to make the most out of their investment in the service.
Pricing Structure and Budgeting Tips
- Standard Voice: Charges are lower and more cost-effective for basic use cases.
- Premium Voice: Higher pricing for more natural-sounding speech, suitable for commercial and high-quality applications.
- Audio Duration: Pricing is calculated based on the length of the generated speech. Consider optimizing audio length to control costs.
Important: Regularly monitor usage to ensure it does not exceed planned budgets, especially during high-demand periods or when using premium voices.
Tracking and Managing Costs
- Set a monthly budget limit to avoid unexpected charges.
- Utilize the Google Cloud Console to monitor API usage in real-time.
- Take advantage of free-tier quotas to minimize expenses for smaller projects.
- Analyze usage patterns and adjust API usage accordingly to avoid overage costs.
Voice Type | Price per 1 Million Characters |
---|---|
Standard | $4.00 |
Premium | $16.00 |
Testing and Troubleshooting Common Issues in Text-to-Speech Integration
When implementing a Text-to-Speech (TTS) service, such as the Google API, various challenges can arise, which may impact the functionality and performance of the application. Testing is essential to identify and resolve these issues early on. It is important to understand potential problems related to incorrect configurations, API limits, or integration errors. Proper debugging ensures smoother user experiences and enhances the efficiency of the integration process.
In this section, we will review the most common issues encountered during the testing phase and provide practical solutions. By focusing on specific technical challenges, developers can better address integration difficulties and ensure the TTS service performs as expected.
Common Problems and Solutions
- Incorrect Language or Voice Settings: The most common issue occurs when the wrong language or voice type is set. Ensure the TTS configuration matches the desired voice model and language codes.
- Audio Quality Issues: Low-quality output may result from improper encoding or network latency. Use the right encoding formats (such as MP3 or WAV) and check network stability during testing.
- API Limits and Rate Limits: Exceeding the TTS API quota can cause service failures. Monitor usage limits and ensure efficient handling of API calls.
Debugging Tips
- Check API Response Codes: Always inspect the response codes from the TTS API to identify errors in the request.
- Log Error Details: Enable detailed logging to capture error messages and response data for better analysis.
- Test with Different Parameters: Adjust parameters like speech rate and pitch to test how they affect output and whether any issues arise.
Test Environment and Tools
Using the right testing tools can help streamline the debugging process. Here's a table listing some of the key tools to utilize when working with TTS services:
Tool | Purpose |
---|---|
Postman | Useful for testing API requests and responses without writing code. |
Charles Proxy | Helps in debugging network requests and monitoring traffic. |
Google Cloud Console | Provides insights into API usage and error logs. |
Note: Always ensure the API key and credentials are correctly set to avoid authentication errors during testing.