C++ Virtual Assignment Operator
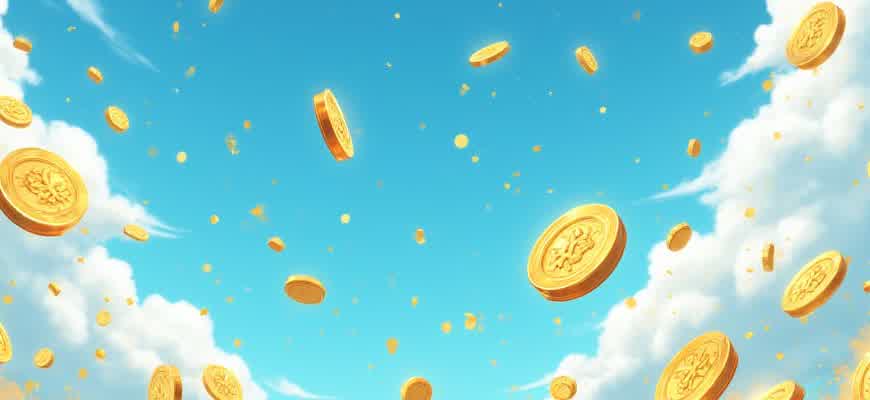
The assignment operator in C++ plays a crucial role in managing object copying, particularly in cases where objects need to be assigned to each other. However, the assignment operator must be carefully implemented when dealing with polymorphic objects and base-derived class relationships. In such cases, a virtual assignment operator ensures that the correct version of the operator is called at runtime, preventing potential issues related to slicing or incomplete copying of base-class data.
In C++, if a class contains dynamic memory or manages resources, the assignment operator must handle proper copying to avoid resource leaks or shallow copying issues. This becomes more complex in a class hierarchy. A virtual assignment operator can solve these challenges by ensuring that derived class objects correctly handle their own resources when assigned to base class objects.
Important: Always declare the assignment operator as virtual in a base class when dealing with polymorphism to avoid undefined behavior.
- Base class: Contains common functionalities and a virtual assignment operator.
- Derived class: Implements specific behaviors and may override the virtual assignment operator.
- Override the virtual assignment operator in the derived class.
- Use the base class assignment operator to ensure proper assignment of common members.
- Ensure the derived class handles its specific resources, such as dynamic memory.
Class Type | Assignment Operator Behavior |
---|---|
Base Class | Virtual operator that handles generic assignment. |
Derived Class | Override virtual operator for specific behavior, calling the base class operator. |
Understanding the Need for a Virtual Assignment Operator in C++
In C++, assignment operators are crucial for managing the correct assignment of object values. However, the default assignment operator does not always meet the needs of complex class hierarchies, especially when dealing with polymorphic objects. To ensure proper handling of resources in derived classes, a virtual assignment operator may be necessary. This concept becomes more critical when objects of derived classes are assigned through base class pointers or references.
Without a virtual assignment operator, polymorphic behavior cannot be achieved, which may lead to resource mismanagement or incomplete object copying. The operator needs to be overridden in derived classes to ensure that specific class logic is applied during the assignment process. Below are key points explaining why such an operator is required.
Reasons for Virtual Assignment Operator
- Proper Resource Management: A virtual assignment operator ensures that derived class resources are correctly handled when an assignment is made through a base class pointer or reference.
- Polymorphism Support: Without a virtual operator, assignment behavior may not behave polymorphically, leading to potential runtime errors and memory issues.
- Correct Copy Semantics: Each class in the hierarchy can provide its own logic for assignment, preventing shallow copying of resources and ensuring deep copies when needed.
Example: Why the Default Assignment Is Insufficient
The default assignment operator only performs a shallow copy of the class data members. This could lead to improper behavior if the class deals with dynamically allocated memory or other resources that require deep copying.
Important: When the base class has a non-trivial destructor or manages resources, the virtual assignment operator ensures that derived class destructors are properly invoked during assignment, avoiding resource leaks.
When to Implement a Virtual Assignment Operator
- When your class manages resources that cannot be simply copied with a shallow copy (e.g., dynamic memory, file handles, etc.).
- When you are using polymorphic objects and need to ensure that the right assignment behavior is invoked at runtime.
- If your base class is part of a class hierarchy and you want to allow proper copying of derived class objects.
Assignment Operator Overriding: An Example
In the following table, the left column shows a class hierarchy and the right column demonstrates where a virtual assignment operator should be defined to handle the assignment correctly:
Class | Needs Virtual Assignment Operator? |
---|---|
BaseClass | No (if no dynamic memory is used) |
DerivedClass | Yes (if dynamic memory or resources are managed) |
How to Implement a Virtual Assignment Operator in Your Classes
In C++, the assignment operator allows objects to be assigned to each other, and it can be overridden for custom behavior. Typically, when implementing a virtual assignment operator in your class, it's crucial to ensure that the operator correctly handles memory management and object state, especially when your class is involved in polymorphism. A virtual assignment operator is particularly useful when working with base classes in a polymorphic hierarchy, allowing derived classes to properly copy or assign objects via pointers to base class types.
While the assignment operator is not declared as virtual by default in C++, making it virtual ensures that derived classes implement their own version of the operator. When you define the assignment operator in a base class as virtual, it allows derived classes to override this behavior, which helps maintain proper object semantics in complex inheritance hierarchies.
Steps to Implement a Virtual Assignment Operator
- Ensure that the base class defines a virtual assignment operator.
- Check for self-assignment to prevent redundant memory operations.
- Handle memory management and copy any dynamically allocated resources from the source object.
- Return *this to enable chaining of assignment operations.
Here’s a typical example of implementing a virtual assignment operator:
class Base {
public:
virtual Base& operator=(const Base& other) {
if (this != &other) {
// Perform the necessary deep copy operations here
}
return *this;
}
};
Remember: If a class manages resources (like dynamically allocated memory), always implement the copy constructor and assignment operator to avoid memory leaks or undefined behavior.
Important Considerations
- Self-assignment check: Always check if the object is being assigned to itself, as this can cause undefined behavior or unnecessary operations.
- Copying resources: If your class allocates memory, ensure proper copying of the resources to prevent shallow copies.
- Return type: The operator should return a reference to *this to allow assignment chaining.
Factor | Action |
---|---|
Self-assignment | Check and skip operations if the object is being assigned to itself. |
Memory Management | Allocate new memory for the copied data to avoid shallow copies. |
Return | Ensure the operator returns a reference to the object itself (*this). |
Handling Deep Copying with a Virtual Assignment Operator
When dealing with custom resource management in C++, handling deep copying in classes is crucial. A virtual assignment operator is a powerful tool when managing dynamic resources like memory or file handles. This operator allows you to control how objects are copied, ensuring that each object maintains its own copy of dynamically allocated data, instead of sharing pointers between instances.
For classes that need to implement deep copying, a virtual assignment operator should be carefully designed to avoid shallow copying pitfalls. This often involves creating a copy of the resource and ensuring that each assignment results in a proper duplication rather than just copying the pointer values.
Steps to Implement Deep Copying with Virtual Assignment Operator
- Allocate memory for the new object: Ensure that each new object gets its own separate memory allocation.
- Copy the resource: Copy the content of the resource (e.g., arrays, buffers) into the newly allocated space.
- Release old resources: Before copying new resources, ensure that previously allocated resources are properly freed to avoid memory leaks.
Important Note: Be cautious with self-assignment. If an object is assigned to itself, no action should be performed, as it could lead to unexpected behavior or memory corruption.
Example of Virtual Assignment Operator
Method | Description |
---|---|
copy constructor | Used for creating a deep copy of an object by allocating new memory for each field. |
assignment operator | Ensures that one object can be assigned to another while handling deep copying of resources. |
In cases where objects manage dynamically allocated memory, it’s essential to properly handle resource management in both the copy constructor and assignment operator to prevent memory leaks and undefined behavior.
Key Considerations
- Self-assignment check: Ensure the assignment operator handles self-assignment correctly.
- Exception safety: The assignment operator should ensure that no resources are leaked in case of an exception during the copying process.
- Return *this: Returning a reference to the current object allows chaining assignments.
Challenges of Using Virtual Assignment Operators in Inheritance Hierarchies
In object-oriented programming, the use of a virtual assignment operator in inheritance hierarchies introduces several complications that can lead to subtle bugs and unexpected behavior. A virtual assignment operator is intended to allow derived classes to properly assign values to objects through polymorphism, but this goal can be difficult to achieve due to issues with object slicing, resource management, and performance concerns. Furthermore, the semantics of assignment in the presence of inheritance can become unclear when the assignment operator is declared virtual, leading to potential confusion for developers.
One of the main difficulties arises from the need to ensure that derived class assignments correctly manage resources inherited from base classes. Without careful handling, an assignment operator may fail to properly copy or move the underlying resources, resulting in memory leaks, dangling pointers, or other resource management issues. Additionally, because the operator is virtual, there is a risk of inadvertently invoking the wrong function in certain contexts, especially when working with base class pointers or references to derived class objects.
Key Issues in Using Virtual Assignment Operators
- Object Slicing: When assigning one object to another through base class pointers, the derived class parts of the object might be sliced off, leading to loss of data.
- Ambiguity of Assignment Semantics: In complex inheritance hierarchies, it can become unclear whether the assignment should apply only to the base class portion or to the entire derived object.
- Resource Management Problems: Properly handling dynamic memory, file handles, or other resources in the virtual assignment operator is challenging, as the derived class may need to perform additional cleanup or allocation.
- Performance Overhead: Virtual function calls are generally slower than direct function calls, which may introduce unwanted performance penalties when assignment operations are frequent.
Strategies to Mitigate Issues
- Explicit Copy Constructors: Implement explicit copy constructors in the base and derived classes to ensure proper resource management before assignment occurs.
- Override Base Class Assignment Operators: Derived classes should override the base class assignment operator to handle the specific needs of their resources and state.
- Use of Smart Pointers: For managing resources, smart pointers like std::unique_ptr or std::shared_ptr can help reduce the risk of memory leaks or dangling pointers.
- Avoid Virtual Assignment for Simple Inheritance: In cases where polymorphism is not necessary for the assignment operation, it may be better to avoid making the operator virtual, thus simplifying the design.
Impact on Code Maintenance
Challenge | Impact |
---|---|
Memory Management | Increased complexity in ensuring correct handling of dynamically allocated memory. |
Polymorphism Overhead | Performance degradation due to the use of virtual calls for assignment. |
Code Clarity | Ambiguity in assignment behavior can make code harder to understand and maintain. |
It is essential to carefully consider whether a virtual assignment operator is necessary for a given class hierarchy, as its usage may introduce more complexity than it resolves.
Best Practices for Overloading the Virtual Assignment Operator
Overloading the assignment operator in C++ is a critical part of managing resource ownership and ensuring that objects are copied or assigned correctly. When implementing a virtual assignment operator, you need to follow best practices to avoid issues like memory leaks, shallow copies, and undefined behavior. It is especially important when working with polymorphic classes to ensure proper object management during assignment operations.
In order to ensure consistency, efficiency, and correctness in the overloaded operator, developers should adhere to several key guidelines. Below are some best practices that can help in implementing the virtual assignment operator in a reliable and efficient manner.
1. Implement Copy-and-Swap Idiom
One of the most effective ways to implement the assignment operator is to use the copy-and-swap idiom. This technique helps avoid code duplication and ensures exception safety. Here’s how you can implement it:
- Create a copy constructor that takes a constant reference to the object being copied.
- Define the assignment operator as a non-throwing member function that returns a reference to the current object.
- Swap the internal state of the current object with the new copy.
- Ensure the object is properly managed, with the old state being destroyed automatically by the destructor.
2. Proper Handling of Virtual Functions
When overloading the assignment operator in polymorphic classes, ensure that you consider the virtual functions of the base and derived classes. This is necessary to avoid slicing and memory management issues when working with inherited objects.
Note: The virtual assignment operator should be implemented in the base class, and any derived classes should override it, ensuring that the base class's assignment mechanism is invoked first, followed by any derived-specific logic.
3. Ensure Self-Assignment Safety
A common pitfall is self-assignment, where an object is assigned to itself. This can lead to unnecessary resource deallocation and reallocation. To avoid this, check whether the object being assigned is the same as the current object, and return *this immediately if they are identical:
if (this == &other) return *this;
4. Prevent Resource Leaks and Undefined Behavior
It's crucial to handle resource management carefully. If your class allocates dynamic memory or other resources, make sure you properly release those resources in the destructor and avoid shallow copies. The use of RAII (Resource Acquisition Is Initialization) helps mitigate memory leaks.
5. Example Table: Assignment Operator Implementation
Step | Action |
---|---|
1 | Check for self-assignment and handle it early. |
2 | Create a copy of the object being assigned. |
3 | Swap the current state with the copied object. |
4 | Ensure exception safety by using a non-throwing swap function. |
5 | Return *this to allow chained assignments. |
Debugging Common Issues with Virtual Assignment Operators in C++
The virtual assignment operator is a critical component in C++ when working with polymorphic objects. Its primary responsibility is to handle correct object copying when derived class objects are assigned to base class objects, ensuring proper memory management. However, debugging issues related to the virtual assignment operator can be challenging. Developers often face difficulties related to object slicing, improper memory handling, or confusion around the use of base class assignment operators in polymorphic situations.
Common issues with virtual assignment operators arise when they are not correctly implemented, causing undefined behavior or memory leaks. These problems typically occur when the operator does not properly handle resource management or fails to perform the assignment in a virtual context. Below are some key pitfalls and strategies for debugging them.
1. Object Slicing and Incorrect Assignment Behavior
Object slicing occurs when a derived class object is assigned to a base class object, causing the derived class's data to be "sliced off." This leads to loss of data and often unexpected behavior during runtime. This is particularly troublesome when working with polymorphic types and assignment operators.
- Ensure that the assignment operator is implemented correctly in the base class.
- Use dynamic_cast to verify the actual type of the object being assigned.
- Consider using reference or pointer types to avoid object slicing.
2. Resource Management Issues in Assignment Operators
Memory leaks and double deletions can occur when assignment operators fail to properly manage dynamic memory. If the assignment operator does not handle deep copies or free previously allocated memory, it can lead to leaks.
Always implement proper deep copy logic in the assignment operator for polymorphic types. Failure to do so may result in memory corruption or leaks.
- Check for the correct implementation of the copy-and-swap idiom.
- Ensure that the operator first deletes the old resources before copying new data.
- Use smart pointers to handle resource management where applicable.
3. Virtual Assignment Operator Overloading
In certain cases, developers forget to mark the assignment operator as virtual in the base class, leading to incomplete or incorrect polymorphic behavior.
Problem | Solution |
---|---|
Base class assignment operator is not virtual | Make sure to declare the operator as virtual in the base class. |
Base class operator ignores derived class behavior | Ensure that the derived class operator calls the base class operator correctly and handles the derived class state. |
When to Use a Virtual Assignment Operator and When to Avoid It
In C++, the assignment operator plays a critical role in ensuring that objects can be properly copied or assigned. The virtual assignment operator is a specialized variant used when polymorphism and dynamic memory management are needed. However, its usage requires careful consideration, as improper use can lead to unnecessary performance overheads or undefined behavior. Understanding when to implement a virtual assignment operator, and when to refrain from doing so, is key to writing robust and efficient C++ code.
When working with inheritance hierarchies, polymorphic types, or objects involving dynamic memory allocation, there are specific scenarios in which overriding the assignment operator as virtual can be beneficial. However, the decision to do so should not be taken lightly, as it comes with certain trade-offs and complexities, especially with resource management and object ownership. Below are important points to consider when deciding whether to use a virtual assignment operator.
When to Use It
- Polymorphic Objects: When assigning between objects of derived classes, and if polymorphism is involved, a virtual assignment operator ensures the correct behavior.
- Resource Management: If an object is managing resources that require custom handling (e.g., memory or file handles), implementing a virtual assignment operator can allow you to handle such resources more safely during assignments.
- Deep Copying: If the base class needs to ensure that the derived class performs a deep copy when assigned, the virtual operator can be leveraged to avoid shallow copying.
When to Avoid It
- Unnecessary Overhead: Virtual functions introduce performance overhead due to the mechanism of dynamic dispatch. If the assignment operator is not essential for polymorphism or resource management, it is better to avoid virtual functions.
- Incorrect Memory Management: If not properly implemented, a virtual assignment operator may lead to memory leaks, double deletions, or undefined behavior when managing dynamically allocated resources.
- Base Class Assignment: If the base class assignment does not require polymorphic behavior, it’s often best to avoid making the operator virtual to simplify the design and prevent misuse.
Summary
Use Case | Reason |
---|---|
Polymorphic behavior with derived objects | To ensure proper dynamic dispatch during assignments |
Resource management or deep copying | To manage dynamic resources or perform deep copies in derived classes |
Performance critical applications | Avoid virtual to minimize overhead |
Carefully evaluate whether virtual assignment truly adds value to your design. Often, default assignment behavior suffices, and virtual overrides introduce unnecessary complexity and cost.