R Deep Learning Packages
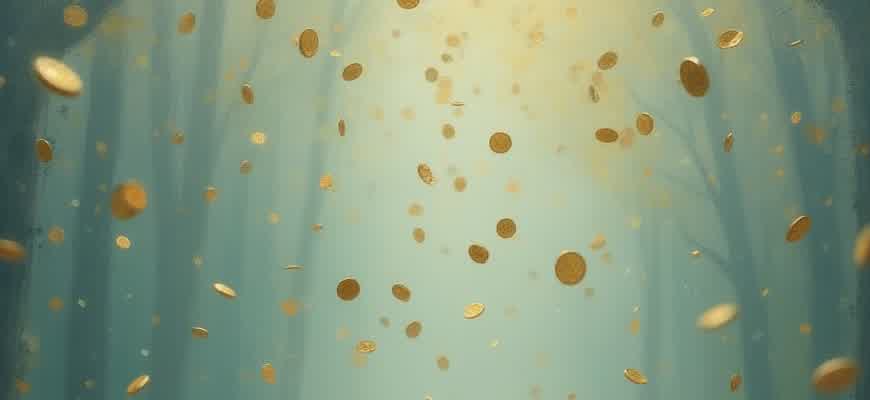
R offers a variety of libraries that support deep learning workflows, providing tools for building, training, and deploying complex neural networks. These packages simplify the process of implementing models like convolutional neural networks (CNNs) and recurrent neural networks (RNNs), making deep learning more accessible for R users.
Among the most commonly used packages for deep learning in R are:
- keras - A high-level interface for building neural networks, wrapping TensorFlow and Theano backends.
- tensorflow - Provides an interface to TensorFlow, allowing users to perform deep learning tasks directly in R.
- mxnet - A deep learning framework that focuses on efficiency and scalability, suitable for both research and production.
Important: Keras is particularly favored for its simplicity and ease of use, while TensorFlow offers more flexibility and control over the model-building process.
In addition to these libraries, R also provides several packages for data preprocessing and visualization, such as:
- dplyr for data manipulation.
- ggplot2 for visualizing training results and model performance.
Package | Primary Use | Backend |
---|---|---|
keras | Neural Network Interface | TensorFlow, Theano |
tensorflow | Deep Learning Framework | TensorFlow |
mxnet | Deep Learning Framework | MXNet |
Key Features of R Deep Learning Libraries: A Comparison
R provides a diverse set of libraries designed to facilitate deep learning model development. Each library comes with its own set of strengths and use cases, catering to various needs within the machine learning and data science community. Comparing these libraries helps in identifying the most suitable tool based on project requirements, such as speed, scalability, or ease of use.
Among the popular deep learning libraries in R, we find frameworks like keras, tensorflow, and mxnet. These packages provide unique features, which make them attractive for different types of deep learning tasks, from image recognition to natural language processing.
Comparison of Key Features
- Keras: Focuses on simplicity and rapid prototyping, with a user-friendly API that allows quick model development. It is built on top of TensorFlow and provides higher-level abstractions.
- TensorFlow: A more robust framework, TensorFlow is suitable for large-scale machine learning applications. It offers greater flexibility and scalability, often used in production environments.
- MXNet: Known for its performance and scalability, MXNet supports multiple languages and is designed for both academic research and industrial use.
Key Features Comparison Table
Library | Ease of Use | Performance | Scalability | Community Support |
---|---|---|---|---|
Keras | High | Moderate | Moderate | Strong |
TensorFlow | Moderate | High | Very High | Very Strong |
MXNet | Moderate | High | Very High | Moderate |
TensorFlow is often the go-to choice for large-scale applications, while Keras is ideal for those looking for ease of use and quick prototyping.
When deciding which library to use, consider the specific needs of your project. Keras might be more suitable for beginners or those in need of a fast prototyping tool, while TensorFlow excels in handling complex and scalable models. MXNet’s strong performance also makes it a compelling choice for production environments.
Setting Up Neural Networks with R: A Step-by-Step Tutorial
Neural networks have become an essential tool in the machine learning landscape, and R provides a wide range of packages to implement them efficiently. In this tutorial, we will guide you through the process of setting up a neural network in R, from installing necessary packages to training your model and evaluating its performance. By the end, you'll have a clear understanding of how to apply neural networks to real-world problems using R.
We'll focus on the keras and tensorflow R packages, which are both powerful and popular for deep learning tasks. These libraries provide a user-friendly interface to build, train, and evaluate complex neural network models. Let’s break the process down into manageable steps.
Step 1: Install Required Packages
Before we begin, make sure that the necessary libraries are installed. You can do this by running the following commands in your R console:
install.packages("keras")
install.packages("tensorflow")
After installation, load the libraries:
library(keras)
library(tensorflow)
Step 2: Prepare Data for Training
Data preparation is crucial for training a neural network. You can use any dataset suitable for your task. In this example, we'll use the built-in iris dataset to predict flower species.
Tip: Always scale your data before feeding it into a neural network to improve model convergence.
Next, separate the features and labels:
data(iris)
x <- as.matrix(iris[, 1:4])
y <- as.factor(iris$Species)
y <- to_categorical(as.integer(y) - 1, 3) # Convert labels to categorical format
Step 3: Build the Neural Network Model
Now we’ll define the structure of the neural network. We’ll use a simple model with one hidden layer:
model <- keras_model_sequential() %>%
layer_dense(units = 10, activation = 'relu', input_shape = c(4)) %>%
layer_dense(units = 3, activation = 'softmax')
Step 4: Compile the Model
Before training the model, compile it by specifying the optimizer, loss function, and evaluation metrics:
model %>% compile(
loss = 'categorical_crossentropy',
optimizer = optimizer_adam(),
metrics = c('accuracy')
)
Step 5: Train the Model
Train the neural network by passing the features and labels along with the number of epochs and batch size:
model %>% fit(x, y, epochs = 50, batch_size = 10, validation_split = 0.2)
Step 6: Evaluate the Model
After training, you can evaluate the performance of your model using the test data. Here’s how to do it:
score <- model %>% evaluate(x, y)
cat("Test loss:", score$loss, "Test accuracy:", score$accuracy)
Step 7: Make Predictions
Once your model is trained, you can make predictions. For example, predicting the species of a new flower:
predictions <- model %>% predict(x)
predicted_class <- apply(predictions, 1, which.max) - 1
Summary of the Workflow
Step | Action |
---|---|
Step 1 | Install and load necessary libraries |
Step 2 | Prepare your data for training |
Step 3 | Build the neural network model |
Step 4 | Compile the model |
Step 5 | Train the model |
Step 6 | Evaluate the model |
Step 7 | Make predictions |
Important: Always monitor the model's performance on a validation set during training to avoid overfitting.
Fine-Tuning Hyperparameters for Deep Learning Models in R
When building deep learning models in R, fine-tuning hyperparameters plays a crucial role in optimizing model performance. Hyperparameters such as learning rate, batch size, and number of epochs can significantly affect the convergence rate and accuracy of the model. In R, several packages offer the flexibility to adjust these hyperparameters, including popular libraries like keras, tensorflow, and caret. Properly fine-tuning these parameters can help achieve the best possible results for a given task.
Several techniques and strategies can be employed to tune hyperparameters effectively. These include grid search, random search, and Bayesian optimization, all of which can be integrated into the training process using R. Below, we outline some of the key hyperparameters to consider and methods for tuning them.
Important Hyperparameters and Their Adjustment
- Learning Rate: Controls how quickly the model adjusts its weights. A rate that is too high can cause the model to converge too quickly to a suboptimal solution, while a rate too low can slow down the training process.
- Batch Size: Refers to the number of training examples used in one iteration. Smaller batch sizes may offer better generalization, while larger sizes improve computational efficiency.
- Number of Epochs: Determines how many times the entire dataset is passed through the network. More epochs generally improve accuracy, but excessive epochs may lead to overfitting.
Methods for Hyperparameter Tuning
- Grid Search: Exhaustively searches through a manually specified subset of the hyperparameter space. It’s simple but computationally expensive.
- Random Search: Randomly samples the hyperparameter space. This can often outperform grid search with fewer evaluations.
- Bayesian Optimization: Uses a probabilistic model to predict the most promising hyperparameters. It is more efficient than random or grid search, especially for high-dimensional spaces.
Example of Hyperparameter Tuning Using R
Here is an example of using the caret package for tuning a deep learning model:
library(caret) trainControl <- trainControl(method = "cv", number = 10) tuneGrid <- expand.grid(.layer1 = c(5, 10), .layer2 = c(5, 10), .epoch = c(10, 20)) model <- train(target ~ ., data = dataset, method = "mlp", trControl = trainControl, tuneGrid = tuneGrid)
Table of Hyperparameters and Their Influence
Hyperparameter | Effect on Model |
---|---|
Learning Rate | Affects convergence speed and stability. |
Batch Size | Impacts memory usage and model generalization. |
Number of Epochs | Controls training duration and potential overfitting. |
Training Convolutional Neural Networks (CNNs) in R: Best Practices
Training Convolutional Neural Networks (CNNs) in R has gained significant traction, especially with the rise of deep learning frameworks like Keras and TensorFlow, which have R bindings. These frameworks simplify the process of building and training CNNs, allowing data scientists to leverage R's ecosystem for advanced machine learning tasks. However, optimal CNN training requires careful consideration of several key factors, from data preprocessing to model tuning.
To ensure effective model training, it is important to follow best practices that address issues such as overfitting, computational efficiency, and model generalization. Below are some recommended guidelines and considerations for training CNNs in R, along with techniques for improving performance.
Key Practices for Efficient CNN Training
- Data Augmentation: Use techniques like image rotation, zoom, and flipping to artificially increase the size of your dataset. This helps prevent overfitting and improves model generalization.
- Batch Normalization: This technique normalizes the inputs of each layer, leading to faster convergence and better overall model performance.
- Early Stopping: Monitor the validation loss during training and stop when the model begins to overfit, thus avoiding excessive training and preserving computational resources.
Model Optimization Techniques
- Learning Rate Scheduling: Adjust the learning rate during training to speed up convergence and avoid overshooting the optimal point.
- Transfer Learning: Start with a pre-trained model and fine-tune it for your specific task. This approach reduces training time and improves performance, especially when the dataset is small.
- Regularization: Techniques such as L2 regularization can help prevent overfitting by penalizing large weights.
"Transfer learning can significantly improve CNN performance on small datasets by leveraging the knowledge gained from large-scale datasets."
Training Data and Model Comparison
Data Augmentation Technique | Effect on Model Performance |
---|---|
Rotation | Improves robustness to orientation variations |
Zoom | Helps with scale variations in images |
Flipping | Increases dataset diversity and enhances generalization |
Final Considerations
Optimizing CNN training in R involves a combination of using the right tools and techniques to balance model complexity, computation time, and generalization ability. By leveraging R's deep learning frameworks and following the best practices outlined above, you can train models that are both efficient and accurate.
Using R for Natural Language Processing with Deep Learning
R offers a powerful set of tools for performing Natural Language Processing (NLP) with deep learning techniques. By combining packages like keras and tensorflow, R allows users to build and train complex neural networks tailored to text analysis tasks. These packages simplify the integration of pre-trained models and custom architectures for various NLP applications such as sentiment analysis, named entity recognition, and text generation.
Several R libraries facilitate the preprocessing, tokenization, and transformation of raw text data into a format suitable for deep learning models. For instance, text and tm are commonly used for text preprocessing, while deep learning models can be built using packages such as keras and torch. These tools make it possible to implement state-of-the-art NLP techniques like word embeddings (Word2Vec, GloVe) and transformer-based architectures (BERT, GPT).
Steps for Building NLP Models in R
- Text Preprocessing: Clean and prepare raw text data, including tokenization, stop-word removal, and stemming.
- Vectorization: Convert text into numerical representations, using methods such as bag-of-words, TF-IDF, or word embeddings.
- Model Building: Design a deep learning architecture, typically a Recurrent Neural Network (RNN), Long Short-Term Memory (LSTM), or Transformer-based model.
- Training and Evaluation: Train the model using training datasets and evaluate its performance on test datasets.
Common Deep Learning Libraries for NLP in R
Package | Functionality |
---|---|
keras | Provides an interface for building and training deep learning models, including NLP models. |
tensorflow | Offers tools for constructing neural networks and integrating them with R for large-scale deep learning tasks. |
text | A package for advanced text processing, including tokenization and embeddings. |
"R’s deep learning ecosystem provides an intuitive framework for working with large text datasets, enabling efficient model creation for diverse NLP applications."
Handling Large Datasets for Deep Learning in R
Working with large datasets in R for deep learning can be challenging due to memory constraints and computational limitations. However, several strategies can help optimize the workflow and allow for effective model training. These strategies focus on data management, preprocessing, and leveraging R's powerful packages designed to work with large datasets.
Efficiently handling large datasets involves breaking down the data into manageable chunks, using memory-efficient data structures, and utilizing parallel processing. Here are some key techniques and tools you can use to handle large datasets for deep learning models in R.
Key Techniques for Efficient Data Handling
- Data Streaming: Use streaming methods to load data in chunks, instead of loading the entire dataset at once. This reduces memory usage and speeds up preprocessing.
- Data Augmentation: For deep learning tasks, augmenting your data can help create more training examples without requiring additional storage space. Techniques like image rotation, flipping, or text modification can expand your dataset while keeping memory usage low.
- Parallel Processing: Leveraging multiple cores for data processing and model training can significantly improve performance. R packages like parallel or future can be used to distribute tasks across multiple CPUs.
- Efficient Data Storage: Use formats like HDF5 or Parquet for storing large datasets, as these formats are optimized for fast access and reduced memory footprint.
Useful R Packages for Deep Learning with Large Datasets
- data.table: A high-performance package for data manipulation that allows you to work efficiently with large datasets.
- keras: The R interface for Keras can be used to build deep learning models, and it efficiently handles large datasets with TensorFlow backend.
- disk.frame: An R package that supports disk-based operations, enabling you to work with datasets that don’t fit in memory by using lazy evaluation.
- h2o: A scalable machine learning platform that provides distributed deep learning support and is optimized for large datasets.
Data Preprocessing Best Practices
Preprocessing large datasets is a crucial step before training deep learning models. Below are some best practices that can help streamline this process:
Step | Action |
---|---|
1. Data Cleaning | Handle missing values, remove duplicates, and filter irrelevant features. |
2. Normalization | Scale the data using techniques like min-max or Z-score normalization. |
3. Data Splitting | Split the data into training, validation, and test sets efficiently, ensuring that the model can generalize well. |
4. Feature Engineering | Create new features that might help improve model performance based on domain knowledge. |
Note: When working with large datasets, consider using cloud-based platforms or distributed computing frameworks such as Spark or Hadoop for parallel processing and faster computation.