Text to Speech Api Free Python
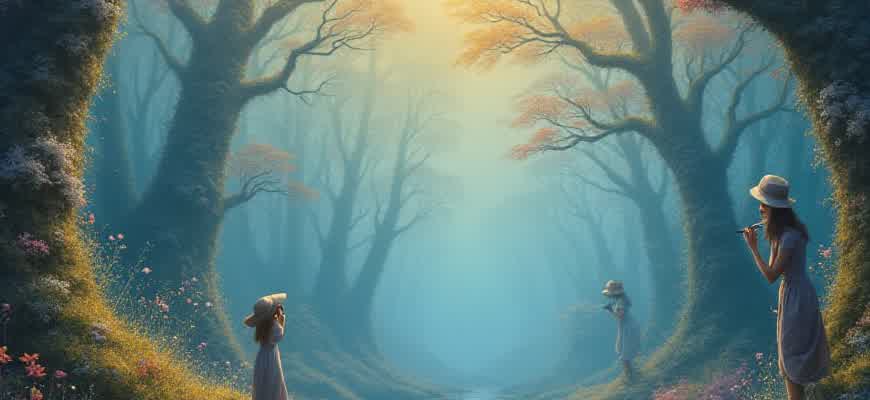
Text-to-speech (TTS) technology allows applications to convert written content into spoken words. For developers seeking to integrate this functionality into their Python projects, several free APIs can be utilized. These APIs offer various features, such as different voices, languages, and customization options for speech synthesis. Below are some popular free solutions.
- Google Text-to-Speech API - Offers high-quality speech synthesis with multiple languages and voice options.
- ResponsiveVoice API - Provides an easy-to-use API with support for over 50 languages and various accents.
- pyttsx3 - A Python library that works offline and supports both text-to-speech and speech-to-text functions.
Each of these APIs has unique features, and choosing the right one depends on the specific requirements of your project.
Note: While many TTS APIs offer free tiers, be aware of usage limits and restrictions on requests per day or month.
To give you a clearer overview, here's a comparison of these free APIs:
API | Languages Supported | Voice Options | Free Tier Limitations |
---|---|---|---|
Google TTS | Multiple languages | Multiple voices and accents | 500,000 characters per month |
ResponsiveVoice | Over 50 languages | Multiple voices | Limited free usage |
pyttsx3 | Limited to installed voices | System-specific voices | Unlimited usage (offline) |
Comprehensive Guide to Using Free Python Text to Speech API
Python offers several libraries for converting text to speech (TTS), and many of them come with free usage tiers that can be leveraged for personal projects or small-scale applications. These APIs provide easy integration into Python scripts and require minimal setup to start converting text into spoken audio.
In this guide, we will explore some of the popular free TTS APIs in Python, their features, and how to set them up in a simple script. This will help you choose the most suitable one based on your requirements.
1. Selecting a Free Text-to-Speech API
There are multiple free options for text-to-speech functionality, each with different features and limitations. Below is a list of some popular ones:
- gTTS (Google Text-to-Speech): A lightweight wrapper around Google’s TTS engine, offering support for multiple languages.
- pyttsx3: A local TTS library that works offline and supports multiple speech engines.
- ResponsiveVoice: A free API that supports various languages and voices with a limited number of requests.
2. Setting Up the gTTS API
gTTS is one of the most widely used Python libraries for converting text into speech. It leverages Google's Text-to-Speech engine and supports multiple languages. The following steps demonstrate how to integrate gTTS into your Python project:
- Install gTTS: To get started, you first need to install the library using pip:
pip install gTTS
- Write your Python Script: Import the library, create a TTS object, and save the audio as an MP3 file:
from gtts import gTTS text = "Hello, welcome to Python text to speech!" speech = gTTS(text=text, lang='en') speech.save("output.mp3")
- Play the Speech: You can use a library like playsound to play the generated MP3:
from playsound import playsound playsound("output.mp3")
3. Comparing Different Free Text-to-Speech APIs
The following table compares some of the features of popular TTS APIs for Python:
API | Offline Support | Languages Supported | Usage Limit |
---|---|---|---|
gTTS | No | Multiple | Free for small projects |
pyttsx3 | Yes | English, Spanish, etc. | No usage limits |
ResponsiveVoice | No | Multiple | Limited free tier |
Important Considerations
Note: While gTTS provides high-quality speech, it requires an internet connection, as it relies on Google's cloud services. If you need an offline solution, consider using pyttsx3.
How to Set Up a Free Text to Speech API for Python Projects
Integrating Text-to-Speech (TTS) functionality into your Python applications can significantly enhance user interaction. There are various free APIs available to help you add speech synthesis capabilities. In this guide, we'll walk through the process of setting up one of the most popular TTS APIs for use in Python projects. These APIs offer a simple way to convert text into audio without the need for complex setups or licensing costs.
One of the easiest and most accessible APIs is Google Cloud Text-to-Speech. It offers high-quality voices and supports multiple languages. However, you will need to set up an API key to use it. Below, we'll explain how to integrate it into your project using Python.
Steps to Set Up Google Cloud Text-to-Speech API
- Sign up for a Google Cloud account.
- Create a new project in the Google Cloud Console.
- Enable the Text-to-Speech API for your project.
- Set up authentication by creating an API key or service account credentials.
- Install the required libraries in Python.
Note: Google Cloud provides $300 in free credits for new users, so you can test the service without incurring charges initially.
Once you've completed the setup, you can start implementing TTS functionality in your Python scripts. Below is a quick guide on how to install and use the necessary libraries:
- Install the Google Cloud TTS library using pip:
pip install google-cloud-texttospeech
- Set up authentication by exporting your service account key as an environment variable:
- Write a simple script to convert text into speech.
export GOOGLE_APPLICATION_CREDENTIALS="path/to/your/service-account-file.json" |
Example Code:
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, how are you?") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech( request={"input": synthesis_input, "voice": voice, "audio_config": audio_config} ) with open("output.mp3", "wb") as out: out.write(response.audio_content) print("Audio content written to file 'output.mp3'")
By following these steps, you can easily set up a free Text-to-Speech API for your Python projects, offering a simple and efficient way to add voice functionality.
Steps to Integrate Text to Speech API in Your Python Application
Integrating a Text to Speech (TTS) API into your Python application can add value by providing voice output capabilities. It’s particularly useful for accessibility purposes, like reading aloud text for visually impaired users or creating interactive voice-based applications. Below is a step-by-step guide to help you set up a TTS API for your project using Python.
Before starting, you need to have a basic understanding of Python programming, as well as an active API key from a TTS provider like Google, IBM Watson, or any other service you prefer. Once you have your API credentials, you can proceed with the integration process.
Step 1: Install the Required Libraries
To begin, you need to install the necessary Python libraries for working with the TTS API. Commonly used libraries include `requests`, `gTTS`, or `pyttsx3` depending on your selected API. You can install these using pip:
pip install gTTS requests pyttsx3
Step 2: Set Up Your API Client
Next, you need to set up your Python script to make requests to the TTS API. This involves creating an instance of the API client and providing your authentication details. Here’s a general process:
- Obtain an API key from the TTS provider.
- Configure your API client with the API key.
- Ensure you have an internet connection to make API requests.
Step 3: Convert Text to Speech
Now that you have your API client set up, you can start converting text to speech. Typically, this involves sending a text string to the API and receiving an audio file in return. The following is an example using the `gTTS` library:
from gtts import gTTS
text = "Hello, this is a text to speech example"
tts = gTTS(text)
tts.save("output.mp3")
Important Note:
Be aware of the rate limits and usage terms of the TTS API you choose. Some APIs offer a free tier with limited features or a restricted number of characters for conversion.
Step 4: Play or Save the Audio
After the text has been converted to audio, you can either play the generated speech directly or save it to a file. Here’s an example to play the audio using Python:
import os
os.system("start output.mp3") # Windows
Step 5: Handle Errors and Edge Cases
It’s important to handle potential errors such as invalid text, connectivity issues, or API limits. Use Python’s try-except blocks to manage these cases and ensure a smoother experience for your users:
try:
tts = gTTS("Test text")
tts.save("test.mp3")
except Exception as e:
print(f"Error: {e}")
Summary Table: Key Steps
Step | Action |
---|---|
Step 1 | Install Python libraries for TTS |
Step 2 | Set up API client with authentication |
Step 3 | Convert text to speech |
Step 4 | Play or save audio |
Step 5 | Handle errors and edge cases |
Choosing the Right Free Text to Speech API for Your Python Needs
When integrating speech synthesis into Python applications, selecting an appropriate free Text-to-Speech (TTS) API is critical. With a multitude of options available, understanding the unique features and limitations of each API can significantly impact the quality and performance of your project. Free APIs often come with constraints, so it’s important to evaluate their capabilities against your specific requirements.
There are several factors to consider when choosing the right TTS solution, such as language support, voice quality, ease of integration, and available features. Below, we explore some of the key aspects to consider, as well as a comparison of popular free options.
Key Factors to Consider
- Voice Quality: Check whether the API offers natural-sounding voices or if they are robotic and hard to understand.
- Language Support: Make sure the API supports the languages and accents that are essential for your application.
- Rate Limiting: Free APIs typically have limits on usage, so understanding these boundaries is essential to avoid disruptions.
- Ease of Integration: Evaluate whether the API has Python libraries or SDKs that streamline the integration process.
Popular Free Text-to-Speech APIs
API | Voice Quality | Languages | Limitations |
---|---|---|---|
Google Text-to-Speech | High | Multiple | Limited daily requests |
ResponsiveVoice | Moderate | Multiple | Free tier available for non-commercial use |
IBM Watson | High | Multiple | Limited free tier with small usage |
Note: Always review the API’s documentation to understand the rate limits and the specific use cases supported by the free tier before committing to any solution.
Conclusion
Choosing the right free TTS API requires balancing the trade-offs between voice quality, language support, and the limitations of the free tier. By thoroughly evaluating the options and considering your project’s needs, you can find the optimal solution that allows you to create a seamless text-to-speech experience in Python.
How to Convert Text to Speech in Multiple Languages Using Python
Python provides a variety of libraries for converting text into speech, and many of them support multiple languages. These libraries typically use cloud-based APIs or offline engines to generate speech from text input. One of the most popular libraries for this task is gTTS (Google Text-to-Speech), which supports several languages and provides a straightforward interface for text-to-speech conversion.
Another widely used library is pyttsx3, which offers offline functionality and also supports multiple languages. Both of these libraries are effective for integrating text-to-speech capabilities into Python applications, whether you're building an accessibility feature, a virtual assistant, or simply automating voice-based outputs.
Using gTTS to Convert Text to Speech in Different Languages
The gTTS library allows easy conversion of text into speech and supports a range of languages such as English, Spanish, French, German, and more. Here’s how to use it:
- Install the gTTS library:
pip install gTTS
- Import the library in your Python script:
from gtts import gTTS
- Create a text object and specify the language using the
lang
parameter: - Save the speech as an audio file:
- Play the audio using an audio player or library (optional).
tts = gTTS(text='Hello, how are you?', lang='en')
tts.save("output.mp3")
Supported Languages in gTTS
Language | Language Code |
---|---|
English | en |
Spanish | es |
French | fr |
German | de |
Italian | it |
Hindi | hi |
Offline Text-to-Speech with pyttsx3
For those who need an offline solution, pyttsx3 is a great choice. Unlike gTTS, which requires an internet connection, pyttsx3 operates entirely offline and also supports multiple languages.
Note: Pyttsx3 may not support as many languages as gTTS, but it is a valuable option for projects requiring offline capabilities.
To use pyttsx3, follow these steps:
- Install pyttsx3:
pip install pyttsx3
- Import the library:
import pyttsx3
- Initialize the engine:
- Set the properties for voice and rate:
- Convert text to speech:
engine = pyttsx3.init()
engine.setProperty('rate', 150) engine.setProperty('voice', 'english')
engine.say("This is an offline text to speech example.") engine.runAndWait()
Common Issues When Using Free Python Text-to-Speech APIs and How to Resolve Them
When working with free Python Text-to-Speech (TTS) APIs, developers often encounter a range of errors that can disrupt the process of generating speech from text. These issues may arise due to limitations of free-tier services, improper API usage, or simple configuration mistakes. Identifying the source of the problem and knowing how to resolve it can save valuable time and effort.
This guide will highlight some of the most common errors when using free TTS APIs in Python and provide practical solutions to fix them. Whether you are dealing with authentication failures, incorrect library setups, or API call issues, the following troubleshooting steps will help streamline your TTS integration.
1. Authentication Errors
One of the first issues that developers face when using free TTS APIs is the authentication error. Free services often require an API key, and failure to correctly set this key in your code can prevent the application from functioning.
Solution: Double-check your API key and ensure it is placed correctly in your script. Some APIs also require you to enable certain permissions or features in the dashboard. Make sure that these settings are configured correctly on the service’s website.
2. Rate Limiting and Quotas
Free-tier TTS APIs typically impose usage restrictions, such as a limited number of requests per day or a maximum number of characters that can be converted to speech within a given time frame. Hitting these limits can result in errors like "Quota Exceeded" or "Rate Limit Exceeded."
Solution: Monitor your usage carefully. If you’re approaching the limit, consider optimizing your code to reduce unnecessary requests or split longer texts into smaller parts. Alternatively, you might explore upgrading to a paid plan if you require more extensive use.
3. Incorrect Library Installation
Another common issue is the improper installation or incompatibility of the required libraries for the TTS service. Libraries such as gTTS, pyttsx3, or others may not work correctly if dependencies are not installed properly or if you are using an outdated version of Python.
Solution: Ensure you have the correct version of Python and that all necessary libraries are installed. Use the following commands to install libraries:pip install gttspip install pyttsx3
4. Audio Output Problems
Audio-related errors, such as no sound or distorted speech, can occur if there is an issue with how the audio is generated or played back. This could be due to incorrect audio format specifications or issues with your sound driver.
Solution: Verify that the correct audio format is specified when saving the output (e.g., .mp3 or .wav). Additionally, check that your system’s audio drivers are up-to-date and that no other program is using the sound device.
5. Error Handling Best Practices
While debugging the errors mentioned above, it’s essential to implement good error handling in your Python script to catch potential issues early on.
try: # Your TTS code here except Exception as e: print(f"Error: {e}")
Table: Comparison of Common Python TTS Libraries
Library | Free Usage Limit | Supported Formats | Offline Support |
---|---|---|---|
gTTS | Up to 100 requests per day | MP3 | No |
pyttsx3 | Unlimited | WAV, MP3 | Yes |
Coqui TTS | Unlimited (with setup) | WAV, MP3 | Yes |
How to Adjust Voice and Speech Rate in Your Python Project
When integrating text-to-speech (TTS) functionality into your Python applications, customizing voice characteristics such as tone and speed can greatly enhance the user experience. Python offers various libraries like pyttsx3 and gTTS, which allow developers to easily modify speech properties. This guide will show you how to adjust the voice pitch, speed, and even language in your TTS project to make it more personalized.
To get started, it's important to understand the core settings you can modify. Most TTS libraries provide options to control aspects such as voice type (male, female, etc.), speech rate (how fast or slow the voice speaks), and volume. With just a few lines of code, you can tailor the speech output to suit your needs.
Customizing Voice Properties
Customizing the voice involves selecting different voice types and adjusting their pitch. Here's how you can do it:
- Voice Selection: You can choose between male or female voices and different regional accents (e.g., British or American English).
- Pitch Adjustment: By modifying the pitch, you can make the voice sound higher or lower, depending on your preference.
- Volume Control: Increase or decrease the loudness of the speech output.
Adjusting Speech Speed
The speech rate determines how quickly the text is spoken. To modify the speed, you'll typically adjust a numeric value that dictates the words per minute (WPM). A lower number will make the speech slower, while a higher number will speed it up.
Tip: Test different speech speeds to find the most natural-sounding rate for your application.
- Set Speech Rate: You can change the speed in most libraries by setting a rate value. For example, pyttsx3 allows setting the rate with a simple command like
engine.setProperty('rate', 150)
. - Test Speech Speed: Experiment with different values to achieve the ideal speed. Typical values range from 100 to 200 for natural speech.
- Optimize for Clarity: Avoid setting a speed too high as it might affect the clarity of the speech output.
Code Example: Using pyttsx3
Here's a basic example of how to change voice and speech speed in Python using the pyttsx3 library:
import pyttsx3 # Initialize the TTS engine engine = pyttsx3.init() # Set the voice to female voices = engine.getProperty('voices') engine.setProperty('voice', voices[1].id) # Female voice # Set the speech rate engine.setProperty('rate', 150) # Set speed to 150 words per minute # Speak the text engine.say("Hello, welcome to the world of Text-to-Speech.") engine.runAndWait()
In this example, the code first selects a female voice and sets the speech rate to 150 WPM. You can replace the voice index with any available voice type, and adjust the rate as necessary.
Summary of Key Parameters
Property | Description | Example Value |
---|---|---|
Voice | Selects the voice type (male, female, etc.) | voices[1].id |
Rate | Controls the speed of the speech | 150 |
Volume | Adjusts the loudness of the speech | 1.0 (Max) |
Best Practices for Optimizing Text-to-Speech API Performance in Python
When working with Text-to-Speech (TTS) APIs in Python, it’s crucial to understand how to maximize performance to ensure a smooth user experience and efficient resource utilization. TTS systems can be resource-intensive, so applying optimization strategies helps in reducing latency and improving output quality. Below are several best practices for achieving optimal TTS performance in Python.
By following these practices, developers can ensure faster and more accurate speech synthesis. The following sections will cover strategies for efficient integration, API usage, and performance improvements.
Optimizing Text Input and Request Handling
Efficient handling of text input and API requests is essential for reducing processing time. Here are a few recommendations:
- Minimize text length: Keep the text within a reasonable length to avoid delays in processing. Long texts should be split into smaller chunks.
- Preprocess text: Clean and preprocess input text by removing unnecessary characters, special symbols, or unwanted formatting that might slow down the API.
- Cache results: If the same text is being processed repeatedly, consider caching the results to prevent unnecessary calls to the API.
Optimizing API Usage and Configuration
Optimizing how you interact with the TTS API is crucial for enhancing performance and reducing costs. Consider the following suggestions:
- Choose the correct voice model: Select a lightweight and suitable voice model. Some models may produce high-quality output but are more resource-heavy.
- Limit concurrency: Managing the number of simultaneous requests ensures you don’t overwhelm the system. Throttling concurrent calls improves performance.
- Adjust rate and pitch: Tuning speech rate and pitch can balance between performance and quality, making the system more responsive without sacrificing clarity.
Efficient Data Handling and Error Management
Proper data handling and error management are essential for maintaining smooth operation. Follow these practices:
- Use asynchronous processing: Asynchronous calls allow the program to continue processing other tasks while waiting for the TTS response.
- Handle API failures gracefully: Implement retry mechanisms or fallbacks in case of API failures to ensure the system remains responsive.
Performance Monitoring and Benchmarking
Regular monitoring and testing are key to identifying potential bottlenecks and ensuring that your system performs efficiently under various conditions. Below is a table outlining different metrics to monitor:
Metric | Description | Why It Matters |
---|---|---|
Latency | Time taken from sending a request to receiving the audio output. | Reducing latency improves user experience by providing quicker responses. |
Resource Usage | Amount of CPU and memory consumed during TTS operations. | Efficient resource usage ensures system scalability and prevents bottlenecks. |
Success Rate | Percentage of successful API calls. | A high success rate minimizes the need for retries and ensures smooth operations. |
Important: Always monitor the API’s usage limits to avoid exceeding rate limits and resulting in throttling or blocking.