Ai Voice Recognition Python
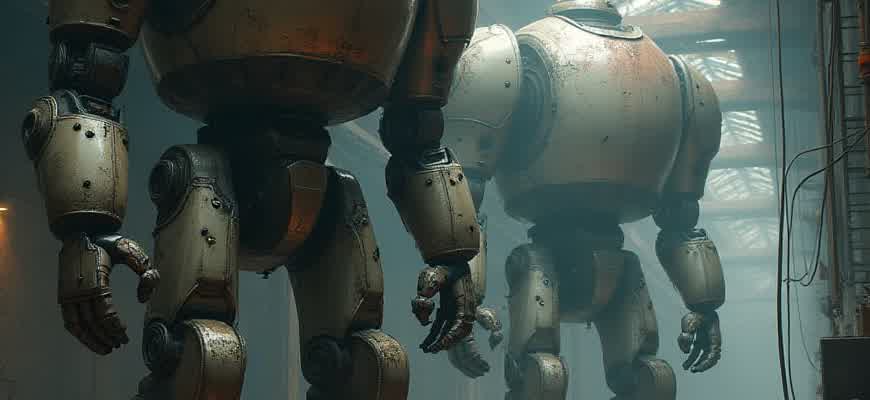
Voice recognition technology has become an integral part of many modern applications, enabling systems to understand and process human speech. By utilizing Python, developers can leverage various libraries and tools to build powerful voice recognition models. This technology allows users to interact with devices or software hands-free, making it particularly useful in areas like virtual assistants, transcription services, and accessibility tools.
Key Python Libraries for Voice Recognition:
- SpeechRecognition - A popular library for converting speech to text using multiple engines.
- PyAudio - A Python library that provides bindings for PortAudio, enabling real-time audio input and output.
- Google Speech API - An API for advanced voice recognition, offering high accuracy and cloud-based processing.
Voice recognition is transforming how humans interact with machines, and Python is a key language enabling developers to build advanced speech processing systems.
Basic Steps to Implement Voice Recognition:
- Install necessary libraries like SpeechRecognition and PyAudio.
- Record audio input from the microphone.
- Use the chosen engine to convert the speech to text.
- Process the text for further use in your application.
Example of Speech Recognition in Python:
Step | Code |
---|---|
Install Libraries | pip install SpeechRecognition PyAudio |
Import Libraries | import speech_recognition as sr |
Initialize Recognizer | recognizer = sr.Recognizer() |
Capture Audio | with sr.Microphone() as source: |
Convert Speech to Text | text = recognizer.recognize_google(audio) |
AI Voice Recognition with Python: A Practical Guide
Voice recognition technology has become an integral part of many applications, from virtual assistants to transcription services. In this guide, we will explore how to implement speech-to-text functionality in Python using various libraries and tools. Python's flexibility and extensive libraries make it an excellent choice for developing AI-powered voice recognition systems.
This guide will walk you through the necessary steps to get started, including setting up the required environment, using popular libraries like SpeechRecognition and PyAudio, and understanding the basic concepts behind voice recognition. By the end, you'll be able to integrate voice recognition into your own Python projects.
Getting Started with Python Voice Recognition
Before diving into coding, it's important to set up the necessary environment for working with voice recognition. You'll need to install a few libraries and set up your microphone for capturing audio input.
- Install Required Libraries: You need to install libraries such as SpeechRecognition and PyAudio. You can do this using pip:
pip install SpeechRecognition PyAudio
import speech_recognition as sr
recognizer = sr.Recognizer()
with sr.Microphone() as source:
print("Adjusting for ambient noise... Please wait.")
recognizer.adjust_for_ambient_noise(source)
print("Listening...")
audio = recognizer.listen(source)
print("Audio captured.")
Understanding Speech-to-Text Conversion
Speech recognition involves converting spoken words into written text. This process is broken down into several stages: capturing audio, processing it to remove noise, and then converting the speech into text using machine learning models.
- Audio Capture: The first step is capturing sound through a microphone. This audio data is then processed to remove any background noise.
- Feature Extraction: In this step, the system breaks down the captured sound into features that are easier for machine learning models to understand.
- Speech Recognition: The processed data is then passed to a speech recognition engine, which outputs the text representation of the spoken words.
Tip: If you're working with non-English languages, make sure to set the appropriate language model in your recognizer for accurate results.
Common Libraries for Speech Recognition in Python
Library | Description |
---|---|
SpeechRecognition | Easy-to-use library for speech-to-text conversion, supports multiple recognition engines. |
PyAudio | Used for audio input/output, allows capturing and playing audio from a microphone. |
pyaudio.speech_recognition | Combines PyAudio and SpeechRecognition for real-time transcription of audio. |
Setting Up a Voice Recognition System in Python
To build a voice recognition system in Python, you can leverage various libraries, with one of the most popular being SpeechRecognition. This library allows easy integration with speech recognition engines such as Google Web Speech API, Sphinx, and others. Setting up the system involves installing necessary libraries, configuring them, and writing code to capture and process audio input.
Below are the steps to set up the environment and implement basic voice recognition using Python. These steps are essential to get your system up and running smoothly, enabling real-time voice recognition from your microphone or pre-recorded audio files.
Steps for Installation and Setup
- Install Required Libraries:
- First, install the SpeechRecognition library using pip:
- Next, you may need a library for microphone support (PyAudio), install it by:
pip install SpeechRecognition
pip install pyaudio
- Setup Microphone Input: Make sure your microphone is connected and properly recognized by your operating system.
- Test with a Simple Script: Create a Python script to recognize speech from the microphone. Here’s an example:
import speech_recognition as sr recognizer = sr.Recognizer() with sr.Microphone() as source: print("Say something!") audio = recognizer.listen(source) try: print("You said: " + recognizer.recognize_google(audio)) except sr.UnknownValueError: print("Sorry, I couldn't understand that.") except sr.RequestError: print("Could not request results.")
Important Note: Ensure that you have an active internet connection when using the Google Web Speech API.
Possible Issues and Troubleshooting
Issue | Solution |
---|---|
Microphone not detected | Check your microphone settings in the OS and verify it's enabled for use. |
API request error | Make sure your internet connection is stable and that Google Web Speech API is accessible. |
Once the basic setup is complete, you can start integrating more complex features such as speech-to-text conversion, voice command recognition, or even connecting the system to other applications.
Integrating AI Voice Recognition into Your Python Application
Voice recognition technology has become an essential part of many modern applications, allowing users to interact with software through speech. Integrating AI-powered voice recognition into your Python project can significantly enhance its user interface and provide more accessible experiences. This process involves choosing the right libraries, training models if necessary, and ensuring smooth integration with the core functionality of your application.
Incorporating voice recognition in Python typically starts with selecting a suitable library or API. There are several popular frameworks available that can convert speech into text, such as Google Speech API, CMU Sphinx, or Microsoft's Azure Speech Service. Depending on your project’s requirements–like offline functionality or multi-language support–you can choose the most appropriate tool.
Steps to Integrate Voice Recognition
- Choose a Voice Recognition Library: Decide on an API or library that best fits your project’s needs, such as SpeechRecognition or PyAudio.
- Install Required Dependencies: Ensure all the necessary packages are installed, such as
speech_recognition
orpyaudio
. - Configure the Microphone Input: Set up a microphone to capture the user's speech and route it to the recognition system.
- Process the Audio Input: Convert the audio signal into text using a speech recognition library.
- Integrate with Application Logic: Use the recognized text for further processing or user interaction in your application.
Example Code Snippet
import speech_recognition as sr
recognizer = sr.Recognizer()
microphone = sr.Microphone()
with microphone as source:
print("Say something...")
audio = recognizer.listen(source)
try:
print("You said: " + recognizer.recognize_google(audio))
except sr.UnknownValueError:
print("Sorry, I couldn't understand.")
except sr.RequestError:
print("Couldn't connect to the API.")
Important Considerations
Make sure to handle errors effectively, such as poor audio quality or speech that the system cannot recognize. You may also need to account for different accents or background noise.
Voice Recognition Libraries Comparison
Library | Key Features | Pros | Cons |
---|---|---|---|
SpeechRecognition | Supports Google, Sphinx, and other services | Simple to integrate, multiple backends | Requires internet for some services |
PyAudio | Audio input/output handling | Low-level access, offline capabilities | Requires setup of additional recognition tools |
Google Speech API | Highly accurate, cloud-based | High accuracy, easy integration | Requires an API key, dependent on internet connection |
Improving Speech Recognition Accuracy with Custom Models in Python
To enhance the performance of speech-to-text systems, it is essential to fine-tune the recognition models according to specific needs and datasets. Default models like Google’s Speech-to-Text or open-source alternatives such as CMU Sphinx or DeepSpeech may not provide the highest accuracy for specialized domains, dialects, or noisy environments. Customizing these models to match particular audio characteristics and vocabulary can significantly reduce error rates and improve real-world usability.
Fine-tuning can be achieved by adapting pre-trained models with domain-specific data or adjusting the underlying algorithms. It requires collecting relevant audio samples, transcriptions, and employing machine learning techniques to optimize the model’s performance on the desired tasks. Below is an overview of key strategies and tools used for custom speech recognition models in Python.
Key Strategies for Fine-Tuning
- Data Collection and Preparation: Gathering diverse and high-quality audio data specific to the use case is crucial. This can involve recording new samples or utilizing datasets that represent the target environment.
- Noise Handling: Implementing noise reduction techniques and augmenting audio data can help improve accuracy, especially in challenging environments.
- Feature Extraction: Customizing the feature extraction process to match the characteristics of the audio data, such as adjusting Mel Frequency Cepstral Coefficients (MFCCs), can help fine-tune model performance.
- Transfer Learning: Using pre-trained models as a base and then fine-tuning them on the target data reduces training time and can yield better results than training from scratch.
Tools and Libraries for Customization
- SpeechRecognition: A popular Python library that interfaces with various speech recognition engines like Google Web Speech API, Microsoft Bing Voice Recognition, etc.
- DeepSpeech: An open-source speech-to-text engine that provides flexibility for training custom models.
- PyTorch/TensorFlow: Frameworks used for deep learning, allowing customization of neural networks for speech recognition tasks.
Table: Comparison of Popular Speech Recognition Models
Model | Strengths | Limitations |
---|---|---|
Google Speech-to-Text | High accuracy, multiple language support, easy integration. | May not work well with noisy environments or specialized vocabulary. |
CMU Sphinx | Open-source, customizable, offline recognition. | Lower accuracy, especially in non-ideal conditions. |
DeepSpeech | Open-source, good for training custom models, supports noisy environments. | Requires substantial computing resources for training. |
Important: Fine-tuning models requires a good understanding of both machine learning techniques and domain-specific requirements. Without sufficient data or computational resources, the results may not meet expectations.
Dealing with Background Noise in Python Voice Recognition Projects
When developing voice recognition systems in Python, background noise can significantly hinder the accuracy of speech-to-text conversion. This is particularly challenging in real-world scenarios where environmental factors, such as crowd noise or air conditioning hum, introduce unwanted audio signals. To improve recognition accuracy, developers must address these issues using various techniques and tools that enhance speech detection in noisy environments.
To tackle the problem of noise interference, it is essential to use preprocessing methods to clean the audio before passing it to the speech recognition model. This may include noise reduction, filtering, and enhancing the signal-to-noise ratio. Python offers several libraries to help manage these tasks and improve performance in environments with varying levels of noise.
Techniques for Noise Handling
- Noise Reduction Filters: Using algorithms like spectral gating or Wiener filtering to suppress unwanted noise while preserving the speech signal.
- Signal Enhancement: Employing techniques such as dynamic range compression to amplify the voice signal and make it more distinguishable from noise.
- Voice Activity Detection: Identifying sections of audio that contain speech and ignoring background silence or non-speech segments.
- Microphone Array: Using multiple microphones to capture sound from different angles and reduce the impact of surrounding noise.
Key Libraries and Tools for Noise Reduction
- PyAudio: Provides real-time audio input/output capabilities, allowing noise filtering before processing.
- SpeechRecognition: A Python library that integrates various speech recognition engines and can be used alongside noise reduction techniques.
- noisereduce: A simple library for noise reduction that uses deep learning models to clean up noisy audio signals.
Practical Considerations
It is important to note that, while noise reduction techniques can improve recognition accuracy, they may also introduce artifacts or degrade the clarity of speech under certain conditions. Proper testing and calibration are required to ensure the balance between noise suppression and speech intelligibility.
Example of Noise Reduction with PyAudio
Step | Description |
---|---|
1. Capture Audio | Record the audio using PyAudio from the microphone. |
2. Apply Noise Reduction | Use libraries like noisereduce to filter out background noise from the captured audio. |
3. Recognize Speech | Pass the clean audio through a speech recognition engine like Google Web Speech API or CMU Sphinx. |
Real-Time Speech Recognition and Transcription with Python and AI
Real-time speech-to-text conversion allows users to transcribe spoken language into written text as it is being spoken. In Python, this can be achieved using various AI libraries that utilize deep learning models to process audio data and output text. The ability to implement such systems effectively is crucial for applications such as voice assistants, transcription services, and real-time communication tools.
The integration of AI and machine learning models into Python-based speech recognition systems enables high accuracy, low latency, and real-time processing. Popular frameworks such as Google Speech API, SpeechRecognition, and PyAudio make it relatively simple to develop robust solutions for speech transcription, even in noisy environments.
Key Steps for Implementing Real-Time Speech-to-Text
- Audio Input: Capturing live audio using microphones or audio streams.
- Signal Processing: Converting audio signals into a digital format for analysis.
- Feature Extraction: Identifying key features such as phonemes or speech patterns from the input signal.
- Text Output: Converting recognized speech to text using AI models and algorithms.
Frameworks and Libraries Used
- SpeechRecognition: Provides support for a variety of speech engines.
- PyAudio: Used for audio capture from the microphone.
- Google Speech API: Offers cloud-based, high-accuracy speech recognition.
"Speech-to-text models can be trained to understand different accents and languages, improving the accessibility of real-time transcription."
Comparison of Common Speech Recognition Tools
Tool | Accuracy | Language Support | Latency |
---|---|---|---|
Google Speech API | High | Multiple languages | Low |
SpeechRecognition | Medium | English (default), others | Medium |
Microsoft Azure Speech | Very High | Multiple languages | Very Low |
Using Pre-trained AI Models for Voice Recognition in Python
Implementing voice recognition in Python can be simplified by leveraging pre-trained AI models. These models have already been trained on vast datasets, enabling them to recognize speech with high accuracy. By utilizing these models, developers can avoid the complex task of training their own models, saving both time and computational resources.
Pre-trained models can be accessed through various Python libraries that provide seamless integration for speech recognition. These tools allow developers to easily implement voice recognition systems for applications ranging from transcription to voice-controlled assistants.
Advantages of Pre-trained Voice Recognition Models
- Reduced Development Time: Pre-trained models are ready to use, eliminating the need for data collection and model training.
- High Accuracy: These models are trained on large, diverse datasets, ensuring a high level of speech recognition accuracy.
- Resource Efficiency: Instead of consuming significant computational resources, developers can leverage the performance of pre-trained models on their systems.
Popular Python Libraries for Voice Recognition
- SpeechRecognition: A library that offers easy integration with various speech recognition APIs, including Google Speech Recognition.
- PyAudio: Often used in conjunction with SpeechRecognition to capture microphone input.
- DeepSpeech: A deep learning-based model for speech-to-text that can be run locally without needing an internet connection.
Example: Using SpeechRecognition for Voice Input
This is a simple example of using the SpeechRecognition library to transcribe audio:
import speech_recognition as sr recognizer = sr.Recognizer() with sr.AudioFile('audio.wav') as source: audio = recognizer.record(source) text = recognizer.recognize_google(audio) print(text)
Comparison of Voice Recognition Models
Model | Accuracy | Offline Support | Languages Supported |
---|---|---|---|
Google Speech-to-Text | High | No | Multiple |
DeepSpeech | Medium | Yes | English |
Microsoft Azure Speech | High | No | Multiple |
Optimizing Voice Control Systems for Smart Devices Using Python
Voice command systems are increasingly integrated into smart devices, enabling users to interact with technology using natural language. However, achieving high performance and accuracy in these systems requires careful optimization. Python, with its rich ecosystem of libraries, offers a variety of tools to enhance the functionality of voice recognition systems for better user experience. From improving command recognition to reducing latency, Python provides solutions to meet the demands of modern smart devices.
The goal of optimization is to ensure that the voice command system can understand diverse accents, handle background noise, and process commands with minimal delay. This can be accomplished by using the right combination of machine learning models, noise reduction techniques, and efficient algorithms. By applying Python libraries like SpeechRecognition, pyttsx3, and others, developers can fine-tune these systems for seamless interaction.
Key Techniques for Optimization
- Noise Reduction: Python's pydub and librosa libraries can be used to preprocess audio inputs, removing unwanted noise and improving the quality of recorded speech.
- Model Training: Custom machine learning models can be built using TensorFlow or PyTorch to adapt to specific voice recognition tasks and improve accuracy over time.
- Latency Reduction: Optimizing the command processing pipeline by utilizing efficient data structures and algorithms can reduce response times and enhance the system's real-time performance.
Approaches for Enhancing Accuracy
- Multi-Channel Audio Processing: Using microphones arrayed in smart devices allows for better spatial audio capture, improving voice recognition in noisy environments.
- Contextual Understanding: By integrating context-aware systems with NLP (Natural Language Processing), Python-based voice command systems can better interpret commands and reduce misinterpretations.
- Continuous Learning: Implementing continuous learning in Python systems helps adapt the model to new speech patterns and languages, increasing overall reliability.
Example Table: Performance Comparison of Python Libraries
Library | Features | Performance |
---|---|---|
SpeechRecognition | Simple integration, support for multiple engines | Good for basic commands, moderate accuracy |
pydub | Audio processing and noise reduction | Effective for preprocessing, improving input clarity |
pyttsx3 | Text-to-speech synthesis | Useful for system feedback in interactive devices |
Optimizing a voice command system is not only about accuracy but also about ensuring fast and reliable response times, which is essential for seamless smart device interaction.