Google Text to Speech Api Test
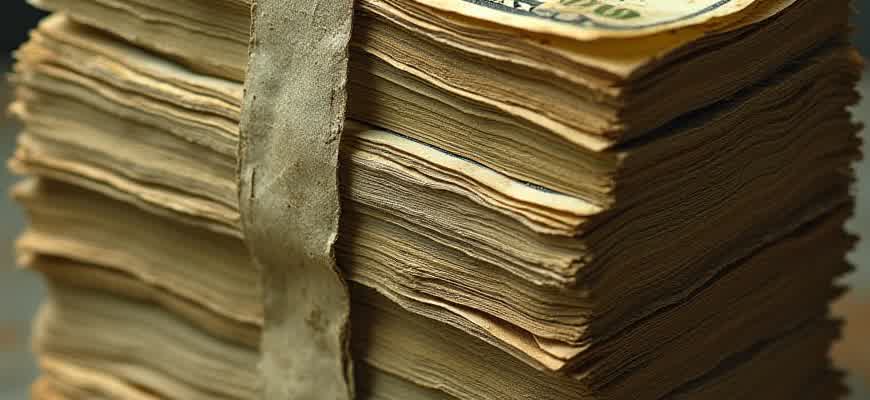
The Google Text-to-Speech API provides an easy and efficient way to convert written text into natural-sounding speech. This API supports multiple languages and voices, allowing developers to integrate speech synthesis capabilities into their applications. In this test, we explore the core features and performance of the service.
Test Parameters
- Voice types: Male, Female, Neural
- Supported languages: English, Spanish, French, and more
- API response time
- Audio quality and clarity
Steps to Test
- Set up Google Cloud account and enable Text-to-Speech API.
- Choose the desired voice and language.
- Input a text sample and initiate the synthesis.
- Evaluate the generated audio file for clarity, pitch, and tone.
"The API can convert text into speech with a variety of customizable parameters, such as voice speed and pitch."
Test Results Summary
Test Case | Result |
---|---|
Male Voice, English | Clear and natural sounding |
Neural Voice, Spanish | High clarity, minimal distortion |
Female Voice, French | Smooth tone, excellent pronunciation |
Google Text to Speech API Test: A Practical Guide for Users
The Google Text to Speech API allows developers to convert text into natural-sounding speech using Google's advanced machine learning models. This tool is highly useful for applications involving accessibility, voice assistants, and automated customer support. In this guide, we will walk through the basic process of testing and utilizing this API to integrate speech functionality into your applications.
Before getting started, it is important to set up your Google Cloud account, enable the Text to Speech API, and install the necessary client libraries. This guide assumes that you have basic knowledge of APIs and some experience with programming. Below, we outline the steps for testing the API and some of its key features.
Setting Up Google Text to Speech API
- Sign in to your Google Cloud Console.
- Create a new project or select an existing one.
- Enable the Text to Speech API from the API library.
- Generate an API key or set up OAuth 2.0 credentials.
- Install the appropriate client library (e.g., Python, Node.js, Java).
Testing the API
Once your environment is set up, you can start testing the API. The simplest test involves sending a text input to the API and receiving an audio output. Here’s an example of how you can make a basic request:
client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, world!") voice = texttospeech.VoiceSelectionParams(language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.FEMALE) audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Key Features of the API
- Multiple Voice Options: You can choose from a variety of voices and languages.
- Speech Speed and Pitch Control: Adjust speech speed and pitch for more customized output.
- Audio Formats: Supports multiple audio formats including MP3, OGG, and WAV.
Note: Be aware of quota limits when testing the API, as there are usage restrictions based on your account's billing setup.
Sample Output and Troubleshooting
After making a request, the API will return an audio file that can be played. If you encounter any issues, check the error messages for helpful details. Common issues include incorrect API credentials, exceeding quota limits, or invalid text input.
Error | Solution |
---|---|
401 Unauthorized | Check your API key or OAuth credentials. |
403 Forbidden | Ensure that the API is enabled and your billing is set up. |
How to Configure Google Text-to-Speech API for Your Application
Integrating the Google Text-to-Speech API into your application can provide powerful voice synthesis capabilities. By following a few simple steps, you can enable your app to convert text into high-quality speech using Google's advanced machine learning models. Below is a guide to setting up the API correctly and efficiently for use in your project.
Before starting, ensure you have a Google Cloud account and have enabled billing. You'll also need to create a new project in the Google Cloud Console. Once your project is ready, you can begin configuring the API and connecting it to your application.
Steps to Set Up Google Text-to-Speech API
- Go to the Google Cloud Console and log in to your account.
- Create a new project or select an existing one.
- Enable the Text-to-Speech API in the APIs & Services section.
- Generate an API key or set up OAuth credentials to authenticate your app.
- Install the appropriate client library for your programming language (e.g., Python, Java, Node.js).
Important Configuration Notes
Ensure that the API key or OAuth credentials are securely stored and never hardcoded directly into your application’s source code.
Once the API is enabled, you can begin sending text input to the API and receive audio responses. The following table shows a sample configuration for Python:
Step | Action |
---|---|
1 | Install the Google Cloud library: pip install google-cloud-texttospeech |
2 | Import the library and authenticate using your credentials file. |
3 | Use the API to synthesize text by passing a text string and desired parameters. |
Once your application is configured, you can experiment with different voices, languages, and audio formats to fine-tune the speech output for your specific needs.
Step-by-Step Instructions for Testing Google Text to Speech Output
Google Text to Speech API allows developers to convert text into spoken words in a variety of languages. Testing its functionality is crucial to ensure the API performs accurately and efficiently. The following guide will help you set up and test the output of the Google Text to Speech API by providing clear instructions and examples.
Before diving into the testing process, make sure you have an active Google Cloud account and the necessary credentials (API keys) to access the service. You will also need to install the required libraries to interact with the API, such as the Google Cloud SDK or relevant client libraries for your programming language of choice.
Instructions for Testing
- Set up Google Cloud SDK:
- Install the Google Cloud SDK on your machine.
- Authenticate using your Google account with the command:
gcloud auth login
. - Set your project with:
gcloud config set project [PROJECT_ID]
.
- Enable the Text to Speech API:
- Navigate to the Google Cloud Console.
- Search for "Text to Speech API" and enable it for your project.
- Install the Client Library:
- Install the library using pip (Python example):
pip install google-cloud-texttospeech
.
- Install the library using pip (Python example):
- Write the Test Script:
- Import necessary libraries and initialize the API client.
- Define the text input that you want to convert into speech.
- Specify the desired voice parameters (language, gender, etc.) and audio output format.
- Execute the Script:
- Run your script to generate the speech output and save the audio file (e.g., MP3 or OGG format).
Test Example
from google.cloud import texttospeech client = texttospeech.TextToSpeechClient() synthesis_input = texttospeech.SynthesisInput(text="Hello, welcome to Google Text to Speech API test!") voice = texttospeech.VoiceSelectionParams( language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL ) audio_config = texttospeech.AudioConfig( audio_encoding=texttospeech.AudioEncoding.MP3 ) response = client.synthesize_speech(input=synthesis_input, voice=voice, audio_config=audio_config) with open("output.mp3", "wb") as out: out.write(response.audio_content)
Note: Ensure you have set up the API credentials before running the script. Use the GOOGLE_APPLICATION_CREDENTIALS environment variable to authenticate.
Output Test Results
Test Aspect | Expected Outcome |
---|---|
Text-to-Speech Conversion | Audio should be clear and accurate to the text provided. |
Voice Quality | Speech should match the selected parameters (e.g., gender, accent). |
Audio File Format | MP3 or OGG format, playable on standard audio players. |
Adjusting Voice Parameters: Language, Voice, and Speed
The Google Text-to-Speech API offers several parameters that allow fine-tuning of the generated speech. These adjustments include the selection of language, voice, and the rate at which speech is produced. Customizing these aspects can enhance the user experience, whether for accessibility tools or conversational interfaces. By understanding how to manipulate these settings, developers can create more natural and contextually appropriate voice outputs.
For an effective implementation, it is important to configure each of these parameters carefully. Below is a breakdown of the options available within the API that can be adjusted for better control over speech synthesis.
Voice Configuration
Choosing the correct voice can significantly impact the tone and clarity of the speech output. The Google API provides a variety of voices, ranging from male and female options to different accents. These can be chosen based on the user’s preference or regional needs.
- Language: The voice will match the selected language code (e.g., "en-US" for English, "de-DE" for German).
- Gender: Options include male or female voices in various languages.
- Accent: Depending on the language, different regional accents may be available (e.g., American English vs. British English).
Speech Rate
The speech rate determines how fast or slow the speech is delivered. This can be important for readability or accessibility in various use cases.
- Standard Rate: Default rate suitable for most applications.
- Faster Rate: Used for applications requiring quicker delivery of information, such as news summaries.
- Slower Rate: Helps ensure clarity, especially for users with hearing difficulties or for complex information.
Language Settings
Language settings must be carefully adjusted to ensure proper pronunciation and accentuation. The language parameter must match the intended output language, as some voices are optimized for specific languages.
Important: Always verify the availability of language and voice pairs in the Google API documentation, as some languages may only have specific voices associated with them.
Example of Configuration
Parameter | Option |
---|---|
Language | en-US |
Voice | male, en-US-Wavenet-D |
Speech Rate | 1.0 (normal speed) |
Integrating Google Text-to-Speech API into Web and Mobile Apps
Integrating Google Text-to-Speech API into web and mobile applications allows developers to add speech synthesis functionality, enabling apps to convert written text into natural-sounding speech. This integration can be particularly useful for accessibility features, educational tools, and apps aimed at enhancing user engagement through auditory interaction. The API supports multiple languages and voices, providing flexibility to cater to different user needs.
For developers, implementing the Google Text-to-Speech API involves a few steps, including setting up the API, making requests to the service, and handling the generated audio. The process differs slightly depending on the platform–whether it’s a web or mobile application–but the core concept remains the same. Below is a breakdown of the integration process for both environments.
Integration Steps
- API Setup: First, enable the Text-to-Speech API in the Google Cloud Console and generate API credentials.
- Choose Language and Voice: Specify the language and voice options required for the app.
- Send Text for Conversion: Make API calls to convert text into speech by sending a POST request with the required text input and settings.
- Play the Audio: Once the speech is generated, the audio data can be played back on the user's device.
Platform-Specific Considerations
- Web Applications:
- Use JavaScript to handle API calls and audio playback.
- Leverage browser features like the
Audio
object for easy integration.
- Mobile Applications:
- For Android, use Google’s official SDK to integrate the API within the app.
- For iOS, implement the API using RESTful requests and handle audio output using native libraries.
Important Considerations
Make sure to handle network latency and errors properly, as API calls rely on external servers for speech synthesis. It's also important to manage the audio playback quality and ensure compatibility with different devices.
API Limitations and Pricing
Feature | Description |
---|---|
Supported Languages | Multiple languages and dialects, including regional accents. |
Audio Quality | High-quality natural speech synthesis with a range of voices. |
Pricing | Based on the number of characters processed. Free tier available with usage limits. |
Common Issues During Google Text to Speech Testing and How to Fix Them
When working with the Google Text to Speech API, developers often encounter various issues that can hinder the testing process. These issues can range from configuration problems to limitations in speech synthesis capabilities. Identifying and addressing these problems early ensures a smoother integration and usage of the API.
In this section, we will discuss some of the most common problems faced during testing and provide actionable solutions for resolving them. Whether you are experiencing unexpected voice output or connectivity issues, these tips will help guide you through the troubleshooting process.
1. Incorrect Audio Output
One common issue developers face is incorrect or unexpected audio output when using the Text to Speech API. This can occur for a variety of reasons, including improper configuration or incompatible parameters.
- Solution 1: Verify API Request Parameters - Ensure that the parameters for voice selection (language, gender, etc.) are set correctly in the API request. Incorrect values can lead to inaccurate or undesired speech output.
- Solution 2: Check Audio Encoding - The output format must match the intended use case. For example, if the default encoding is set to "LINEAR16" but you're expecting "MP3," make sure to adjust the encoding in your request.
Always validate the chosen encoding format and voice model to prevent inconsistencies in speech synthesis.
2. API Quota and Rate Limits
Another frequent issue is hitting the API's rate limits, especially during heavy testing or repeated calls within a short timeframe.
- Solution 1: Monitor Usage - Use the Google Cloud Console to track your API usage and ensure you don't exceed your allotted quota. Set up alerts to notify you when you're approaching the limit.
- Solution 2: Optimize API Calls - Reduce the frequency of requests by batching them or implementing a delay between calls. This approach can help stay within the API limits without impacting performance.
3. Voice Selection Issues
Sometimes, the expected voice doesn't sound right or isn't available. This can happen if the requested voice is not supported for the specified language or region.
Language | Available Voices |
---|---|
English (US) | en-US-Wavenet-A, en-US-Wavenet-B |
French | fr-FR-Wavenet-A, fr-FR-Wavenet-B |
German | de-DE-Wavenet-A, de-DE-Wavenet-B |
To resolve voice-related issues:
- Solution 1: Confirm Voice Availability - Refer to the official documentation to ensure that the selected voice is supported for your chosen language.
- Solution 2: Use Default Voices - If custom voices are causing issues, try switching to default voices provided by the API, which are generally more stable.
Managing Multiple Languages in Google Text-to-Speech API
Google's Text-to-Speech API provides the ability to convert text into speech in various languages, which is crucial for applications that require multilingual support. Handling multiple languages effectively within this API can be challenging but is essential for delivering accurate and natural-sounding speech. The API supports a wide range of languages, each with different voices and accents, making it adaptable for global applications.
To use the API efficiently with multiple languages, developers need to understand how to specify language codes, select appropriate voices, and manage translations. The key is to integrate language-specific settings, ensuring that the speech output aligns with the user's linguistic preferences.
Steps for Handling Multiple Languages
- Language Identification: Start by detecting the language of the input text. This can be done using Google Cloud's language detection API or another tool.
- Voice Selection: Once the language is identified, choose the appropriate voice from the available options for that language. Google Text-to-Speech supports male and female voices with various accents.
- API Request Configuration: Specify the desired language and voice in the API request. This can be done by setting the "languageCode" and "voice" parameters correctly.
Supported Languages
Language | Voice Options |
---|---|
English (US) | Male, Female |
Spanish (Spain) | Male, Female |
French (France) | Male, Female |
Tip: It is essential to experiment with different voices to determine which one best suits the context of your application, especially for different languages.
Monitoring API Usage and Understanding Cost Structures
When using a text-to-speech service like Google’s API, tracking usage is critical for ensuring that resources are used efficiently and that the service stays within budget. Understanding how API usage translates to costs is essential for managing the overall expenditure and optimizing the service for specific needs. Google Cloud provides tools to track usage in real-time and set limits to prevent overages.
Accurate monitoring can prevent unexpected charges by highlighting usage trends, alerting users to abnormal spikes, and providing insights into the most resource-intensive operations. Understanding how these usage patterns impact costs is important for making informed decisions about optimizing API calls and minimizing unnecessary expenses.
API Usage Tracking
Google Cloud Console provides built-in tools for monitoring the API usage, including metrics on request volume, data processed, and response time. By setting up usage reports, users can track their consumption over different periods and assess the cost against their allocated budget.
- Real-time consumption monitoring
- Customizable billing reports
- Usage limits to prevent overages
Cost Structure Breakdown
Google’s text-to-speech API uses a pay-as-you-go pricing model based on the number of characters processed in requests. This cost structure can vary based on the selected voice quality and the region of operation. For example, premium voices may incur additional charges compared to standard voices. Users should carefully choose their voice settings based on budget constraints.
Important: The price of text-to-speech requests can change based on factors like language, voice type, and regional data handling policies. Always verify current pricing before deploying applications at scale.
Sample Cost Breakdown
Voice Type | Price per 1 million characters |
---|---|
Standard Voice | $4.00 |
WaveNet Voice | $16.00 |
To keep costs under control, consider using the Google Cloud pricing calculator to estimate potential costs based on expected usage and project requirements.
Advanced Features: SSML Integration and Custom Voice Models
Google Text-to-Speech API offers a range of advanced capabilities that enhance speech synthesis. One of the most notable features is the support for SSML (Speech Synthesis Markup Language), which allows developers to fine-tune the generated speech. With SSML, developers can control various aspects such as pitch, rate, volume, and emphasis, providing a more natural and dynamic audio output. This level of control is essential for applications that require specific vocal characteristics or a customized listening experience.
In addition to SSML support, the API also enables the creation of custom voice models. This feature is crucial for companies seeking to create a unique auditory identity or require a specific voice for their application. By training the model on custom datasets, businesses can generate voices that align with their brand, tone, and target audience preferences. These custom voices go beyond the default offerings, giving companies a competitive edge in user experience.
SSML Features Overview
- Prosody Control: Adjust pitch, speaking rate, and volume to match specific requirements.
- Phonetic Markup: Provide phonetic hints for better pronunciation of complex words.
- Break Tags: Add pauses for natural pacing in speech.
- Emphasis: Emphasize certain words or phrases to convey meaning more effectively.
Custom Voice Model Capabilities
- Voice Customization: Tailor the voice to reflect the desired tone, accent, and vocal traits.
- Branding Opportunities: Create a signature voice to enhance brand recognition.
- Scalability: Train and deploy models for large-scale applications.
- Data Privacy: Control how the voice model is trained with your own proprietary data.
Important: The ability to create and deploy custom voice models allows for a completely personalized user experience. This feature is particularly valuable in sectors like healthcare, e-commerce, and customer service, where brand voice consistency is critical.
Comparison of SSML and Custom Voices
Feature | SSML | Custom Voice Models |
---|---|---|
Voice Customization | Limited to predefined voices | Full control over tone, accent, and characteristics |
Use Case | For fine-tuning speech | For creating a unique brand voice |
Complexity | Easy to implement | Requires more resources and training |