How to Make Your Own Ai Voice Assistant
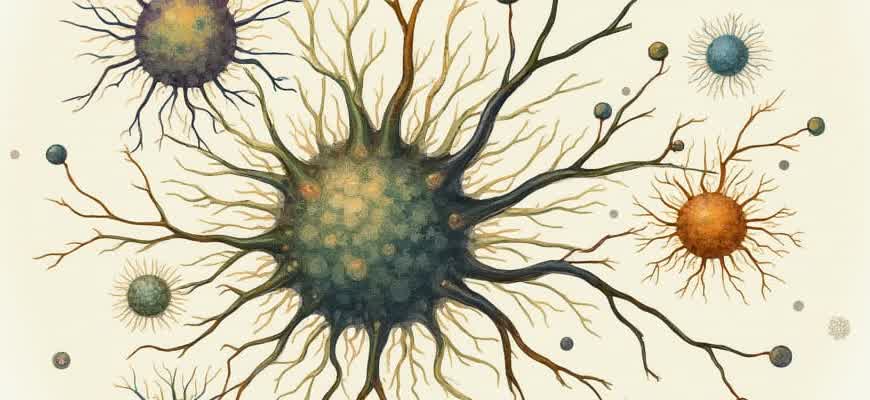
Building your own AI voice assistant involves a few key steps, from choosing the right platform to integrating natural language processing (NLP) capabilities. Below is an overview of the essential components required for this process.
- Platform Selection: Decide on the platform to host your assistant (e.g., Raspberry Pi, personal computer, cloud service).
- Voice Recognition Software: Use open-source libraries like Mozilla’s DeepSpeech or Google’s Speech-to-Text API.
- Natural Language Processing (NLP): Integrate tools like spaCy or GPT-based models to understand user commands.
- Text-to-Speech (TTS): Choose a TTS engine (e.g., Google’s Text-to-Speech, eSpeak) to convert text into voice.
Each of these steps plays a significant role in ensuring the assistant can process voice inputs and provide accurate spoken responses. Below is a quick guide to integrating these technologies:
Component | Example Tool | Function |
---|---|---|
Speech-to-Text | DeepSpeech, Google Speech API | Convert voice input to text for processing. |
Natural Language Processing | spaCy, GPT | Understand and process the meaning behind the text. |
Text-to-Speech | Google TTS, eSpeak | Convert processed text back to speech for responses. |
Tip: Ensure that your assistant has a clean dataset for NLP training to improve its accuracy and understanding of different accents and dialects.
How to Build Your Own AI Voice Assistant
Creating your own AI voice assistant involves combining several advanced technologies, such as speech recognition, natural language processing (NLP), and text-to-speech (TTS) engines. The process requires careful planning and integration of different software and hardware components to achieve a functional and responsive system. By following specific steps, you can design a custom assistant tailored to your needs.
To build an AI voice assistant, you need a solid understanding of programming, machine learning models, and system integration. Below is a guide that breaks down the process into manageable steps, as well as some key tools to help streamline your development process.
Step-by-Step Guide to Creating Your AI Voice Assistant
- Choose a Speech Recognition Tool: Select a speech recognition library like Google Speech API, Microsoft Azure Speech, or open-source options like CMU Sphinx.
- Design the NLP Model: Use libraries such as spaCy or NLTK to help the assistant understand and process user queries. Train your model with a variety of commands to make it more versatile.
- Integrate a Text-to-Speech System: Implement a TTS engine like Google Cloud Text-to-Speech or Amazon Polly to enable your assistant to respond verbally to the user.
- Create Command Processing Logic: Develop the core logic that interprets voice commands, performs relevant tasks, and gives feedback to the user.
Required Tools and Technologies
Tool | Purpose |
---|---|
Google Speech API | Speech recognition and transcription |
spaCy or NLTK | Natural language processing |
Amazon Polly | Text-to-speech conversion |
Python | Programming language for integration |
Remember to account for privacy and security when dealing with voice data. Encrypt sensitive information and ensure your assistant complies with data protection regulations.
Testing and Refining the Voice Assistant
Once your voice assistant is up and running, the next step is to test its functionality across various use cases. Use test scenarios that involve different accents, environments, and phrases to ensure the system remains responsive and accurate.
- Test the system's ability to handle noisy environments.
- Refine the NLP model by adding custom intents and actions.
- Optimize the voice output for naturalness and clarity.
Choosing the Right Platform for Your Voice Assistant
When building a voice assistant, selecting the appropriate platform is critical to ensure the functionality, scalability, and compatibility of your application. Different platforms offer unique features, integrations, and capabilities, so choosing one that aligns with your goals is essential. A well-suited platform allows you to focus on developing user-centric functionalities rather than dealing with technical limitations.
Here are some factors to consider when choosing a platform for your voice assistant:
Key Platform Considerations
- Compatibility: Ensure the platform integrates smoothly with your existing tech stack, including hardware and software.
- Ease of Development: Some platforms offer pre-built models and frameworks that speed up development, while others may require deeper customizations.
- Cost: Consider both initial development costs and long-term operational expenses. Some platforms charge based on usage, while others may have fixed pricing models.
Popular Platforms for Voice Assistants
- Google Assistant SDK: Great for integrating with Google's ecosystem and provides robust NLP tools.
- Amazon Alexa: Ideal for building Alexa skills with support for smart home integrations.
- Microsoft Azure Cognitive Services: Powerful AI tools, particularly for enterprise-level applications.
- Rasa: An open-source platform focused on customizable NLP and conversation flow.
Platform Comparison Table
Platform | Best For | Ease of Use | Pricing Model |
---|---|---|---|
Google Assistant SDK | Smartphone, IoT | Moderate | Pay-per-use |
Amazon Alexa | Smart Home, E-commerce | Easy | Free, with optional paid features |
Microsoft Azure Cognitive Services | Enterprise Applications | Advanced | Pay-per-use |
Rasa | Custom NLP Models | Advanced | Free, with paid enterprise options |
Important: It's crucial to choose a platform based on your specific use case. If you're building a simple, conversational assistant, a platform with built-in NLP features (like Alexa or Google Assistant) may suffice. For more complex or enterprise-level assistants, a platform like Azure or Rasa could be more appropriate.
Setting Up the Development Environment for AI Voice Projects
Creating an AI voice assistant requires a carefully configured development environment to ensure smooth project execution. The setup typically includes choosing the appropriate software tools, installing dependencies, and configuring platforms that provide the necessary resources for voice recognition, synthesis, and processing.
This guide will walk you through the essential steps and tools needed to create a reliable development environment for building your own voice assistant. Having a well-prepared setup allows you to focus on coding and refining the AI's functionalities without dealing with constant configuration issues.
Key Tools and Libraries
Here are the primary tools you will need:
- Python: A popular language for AI development, with libraries like SpeechRecognition, PyAudio, and TensorFlow for building voice-related features.
- Speech-to-Text (STT) Engine: Google Speech API, Mozilla DeepSpeech, or other open-source alternatives.
- Text-to-Speech (TTS) Engine: Google Text-to-Speech, eSpeak, or Amazon Polly.
- IDE: Visual Studio Code or PyCharm for seamless coding and debugging.
Step-by-Step Environment Setup
- Install Python: Download and install Python from the official website.
- Set up a Virtual Environment: Use virtualenv to create a dedicated workspace for the project to manage dependencies effectively.
- Install Libraries: Install necessary libraries like SpeechRecognition, PyAudio, and pyttsx3 for text-to-speech functionality.
- Set Up IDE: Configure your IDE with necessary extensions for Python, such as linters, debuggers, and version control integrations.
Important Considerations
Be sure to test the libraries you are using to ensure compatibility with your operating system and Python version.
Basic System Configuration
The table below summarizes the system requirements for various libraries:
Library | Supported OS | Python Version |
---|---|---|
SpeechRecognition | Windows, macOS, Linux | 3.3+ |
PyAudio | Windows, macOS, Linux | 3.3+ |
pyttsx3 | Windows, macOS, Linux | 3.4+ |
Understanding Speech Recognition and Choosing the Best API
Speech recognition technology converts human speech into a machine-readable format. This technology is crucial for building AI assistants that can understand voice commands and respond accordingly. The process involves analyzing sound waves, identifying phonetic patterns, and mapping those patterns to specific words or phrases in a given language. It is important to choose a reliable API that can accurately interpret speech and provide a seamless user experience.
When selecting a speech recognition API, various factors need to be considered, including accuracy, language support, real-time processing capabilities, and integration options. Understanding these aspects will help in choosing the right tool for your AI assistant’s needs. Below are some of the key elements to keep in mind.
Key Factors to Consider When Choosing a Speech Recognition API
- Accuracy: Ensure the API offers high accuracy in recognizing speech, even in noisy environments.
- Language Support: Look for APIs that support the languages you need for your application.
- Real-time Processing: Some applications require real-time voice processing, so choose an API that can handle this demand.
- Integration: Consider how easily the API can integrate with your existing infrastructure and other services.
- Cost: Evaluate pricing models, including free tiers and usage-based costs.
Popular Speech Recognition APIs
API | Key Features | Pricing |
---|---|---|
Google Cloud Speech-to-Text | High accuracy, supports multiple languages, real-time transcription | Pay-as-you-go |
IBM Watson Speech to Text | Custom models, speaker diarization, real-time recognition | Free tier, then pay-per-use |
Microsoft Azure Speech | Speaker identification, custom voice models, language support | Free tier, pay-per-use |
When selecting a speech recognition API, prioritize those that offer the best performance in noise-rich environments and are scalable according to your application’s needs.
Designing Natural Language Processing (NLP) Capabilities for Your Assistant
To create an effective AI voice assistant, it is crucial to design robust NLP features that allow it to understand and respond to human language naturally. The assistant should be able to process commands, questions, and even casual conversation in a way that feels intuitive to the user. This involves integrating multiple NLP tasks like speech recognition, intent detection, and context management. These processes enable the assistant to understand not only the words being spoken but also their meaning and intent.
The quality of NLP features in your assistant will directly impact user experience. Building a system that can understand various dialects, colloquialisms, and ambiguous phrases is essential for broader user adoption. To achieve this, your assistant needs sophisticated language models trained on diverse datasets. Below is an outline of the key aspects to focus on when designing NLP capabilities for your AI assistant.
Key NLP Features to Implement
- Speech-to-Text (STT): Converts spoken language into text, allowing the assistant to "hear" and process commands.
- Intent Recognition: Analyzes the input text to determine the user's underlying intent (e.g., setting an alarm, checking the weather).
- Entity Recognition: Identifies specific details in the input, such as dates, locations, or names, to provide accurate responses.
- Context Management: Keeps track of ongoing conversations to understand follow-up queries and provide relevant responses.
Steps to Build NLP Capabilities
- Data Collection: Gather diverse datasets including varied speech patterns and languages to train the assistant effectively.
- Preprocessing: Clean and preprocess the data to remove noise and irrelevant information before feeding it into the model.
- Model Selection: Choose a suitable language model (e.g., transformer models like BERT or GPT) that supports your desired functionality.
- Training and Fine-Tuning: Train the model with domain-specific data to ensure the assistant understands context-specific language.
- Evaluation and Testing: Continuously test the model with real-world inputs to identify potential improvements.
Tip: Always ensure your assistant can handle interruptions or changes in conversation flow to maintain a natural user experience.
Language Model Evaluation Table
Model | Strengths | Weaknesses |
---|---|---|
BERT | Strong contextual understanding, excels in sentence-level tasks. | Not ideal for real-time applications, lacks generative capabilities. |
GPT | Great at generating human-like responses and handling free-form conversation. | May struggle with complex tasks that require structured understanding. |
RNN | Effective in sequence prediction tasks, useful for conversational flow. | Can struggle with long-range dependencies and context retention. |
Integrating Text-to-Speech (TTS) for Realistic Voice Output
Creating a personalized AI voice assistant involves integrating various technologies, and one of the most crucial components is Text-to-Speech (TTS). TTS converts written text into speech, enabling your assistant to communicate effectively with users. Achieving a natural, human-like voice requires selecting the right TTS engine, adjusting its parameters, and optimizing its integration into your system. Several methods are available to integrate TTS, and each one has unique strengths depending on the desired outcome.
For a more authentic and engaging experience, you’ll want to focus on features that help mimic human-like conversation. Factors such as intonation, pitch, and speech pacing play an essential role in creating a realistic voice. In this context, selecting a TTS system with advanced prosody capabilities is critical. This can involve using neural networks that have been trained on vast amounts of human speech data.
Key Components for TTS Integration
- Engine Selection: Choose between cloud-based or on-premise TTS engines. Cloud engines like Google Cloud or Amazon Polly provide scalability, while on-premise solutions ensure better privacy control.
- Naturalness of Speech: Use deep learning-based models for smoother speech generation. These models can learn from context to improve pitch variation and cadence.
- Custom Voice Profiles: Many systems allow you to create unique voices based on user preferences, adding a layer of personalization.
Steps to Implement TTS in Your Assistant
- Choose a suitable TTS engine based on your requirements (cost, performance, privacy).
- Integrate the engine with your assistant's backend using the provided API or SDK.
- Configure the voice settings, such as tone, speed, and accent.
- Test and refine the speech output to ensure clarity and natural flow.
Important Considerations for Effective TTS
Factor | Description |
---|---|
Voice Quality | Ensuring the chosen voice sounds lifelike and not robotic. |
Latency | Minimizing the time between text input and voice output for a smoother experience. |
Contextual Understanding | Ensuring the TTS engine can adjust tone and emphasis based on sentence context. |
Tip: Always test your TTS integration across different devices and environments to ensure consistency in voice output.
Training Your AI Voice Assistant to Understand User Intentions
Understanding user intentions is a crucial part of developing an effective voice assistant. The goal is to ensure that your AI can interpret what users are trying to achieve, based on the words and phrases they use. This requires training the assistant to recognize patterns, context, and different forms of communication.
The process of improving user intent recognition involves collecting a wide variety of user input and training your AI model to respond accordingly. You'll need to focus on both natural language processing (NLP) and machine learning algorithms to enhance the assistant's ability to understand different commands, requests, and queries.
Key Strategies for Training Your AI Voice Assistant
- Data Collection: Gather a diverse set of phrases and dialogues from real users to train your model effectively.
- Contextual Understanding: Ensure that your assistant can interpret not only individual words but also the context of a conversation.
- Intent Mapping: Create clear mappings between different user inputs and the intended actions or responses.
- Continuous Learning: Implement feedback loops so that the assistant can improve over time with more data and interaction.
Steps to Improve User Intent Recognition
- Identify and categorize user inputs into specific commands or requests.
- Use machine learning models to analyze and predict user intent based on historical data.
- Develop fallback mechanisms for when the assistant fails to understand a request.
- Test the model frequently with new data to ensure it keeps improving.
Important: Regular updates and retraining are necessary to maintain accuracy and adapt to evolving language patterns.
Sample Intent Recognition Table
User Input | Recognized Intent | Assistant Action |
---|---|---|
"What's the weather like today?" | Weather inquiry | Provide current weather information |
"Set a reminder for my meeting at 3 PM." | Reminder creation | Set a reminder for 3 PM |
"Play some music." | Media request | Play music from the library |
Testing and Debugging Your Voice Assistant's Performance
After developing your voice assistant, it’s crucial to evaluate its efficiency and accuracy to ensure optimal performance. Testing involves assessing how well the assistant handles commands, interacts with users, and processes voice inputs. Debugging, on the other hand, identifies and fixes issues that arise during these tests. Both steps are essential in refining your system before deployment.
To achieve this, you will need a systematic approach that includes both manual and automated testing techniques. The goal is to detect problems like misinterpretations, delayed responses, or incomplete actions that might disrupt user experience.
Steps for Testing Your Voice Assistant
- Voice Input Recognition: Test the assistant's ability to understand different accents, tones, and noise environments.
- Accuracy of Responses: Ensure that the assistant delivers the correct information or performs the intended action based on voice commands.
- Response Speed: Measure how quickly the assistant reacts to commands and provides feedback.
- Error Handling: Evaluate how the system deals with unclear or incorrect input from users.
Debugging Techniques
- Log Analysis: Review the logs generated by the system to trace errors and understand where the assistant fails to process commands.
- Unit Testing: Test individual components of the assistant to identify issues at the code or algorithm level.
- Simulate Real-world Conditions: Run tests in environments with background noise to ensure the assistant performs well under varying conditions.
Common Issues and Solutions
Issue | Solution |
---|---|
Misunderstood Commands | Train the speech recognition model on a more diverse dataset, including various accents and dialects. |
Slow Response Time | Optimize the server and processing pipelines to reduce latency in command execution. |
Incorrect Actions | Refine the NLP model to ensure that it correctly understands the context and intent behind user queries. |
Tip: Regularly update the training data and models to ensure continuous improvement in accuracy and performance.
Deploying Your AI Assistant on Various Devices and Platforms
Once your AI voice assistant is ready, the next crucial step is deployment. Depending on the devices and platforms you choose, the implementation process can vary significantly. Understanding the unique requirements and limitations of each platform will help you make informed decisions on where and how to deploy your assistant. This section will cover the key steps involved in deploying your assistant across different devices and operating systems.
To effectively deploy your AI assistant, you need to target different types of devices, such as smartphones, desktops, and smart home devices. The process typically involves adapting your assistant’s software to each device’s hardware, operating system, and user interaction methods. Here, we explore deployment on some popular platforms and devices.
Deployment on Different Platforms
- Mobile Devices: For Android or iOS, you’ll need to package your AI assistant into an app, using either native SDKs or frameworks like Flutter or React Native.
- Desktops: Desktop versions of your assistant can be deployed using platform-specific packages like .exe for Windows or .dmg for macOS, or by using cross-platform tools like Electron.
- Smart Speakers: Deploying to devices like Amazon Echo or Google Home typically requires integrating your assistant with platforms like Alexa Skills or Google Actions.
Steps for Deployment
- Choose the platform or device for deployment.
- Prepare the required software and dependencies for the selected platform (e.g., Android Studio for mobile or Xcode for iOS).
- Test the assistant in a local environment, simulating the target device.
- Package your assistant into the correct format for the chosen platform.
- Deploy it and monitor performance and user feedback for improvements.
Important Considerations
Optimization: Tailoring your AI assistant’s performance to suit the processing power and memory capacity of the device is crucial. For instance, mobile devices might require lighter, more efficient models compared to desktop or server environments.
Device Compatibility Table
Device | Platform | Deployment Method |
---|---|---|
Smartphone | Android / iOS | App Store / Google Play |
Desktop | Windows / macOS | Executable File / App Package |
Smart Speaker | Amazon Alexa / Google Assistant | Skill or Action Deployment |