Google Speech to Text Api React Native
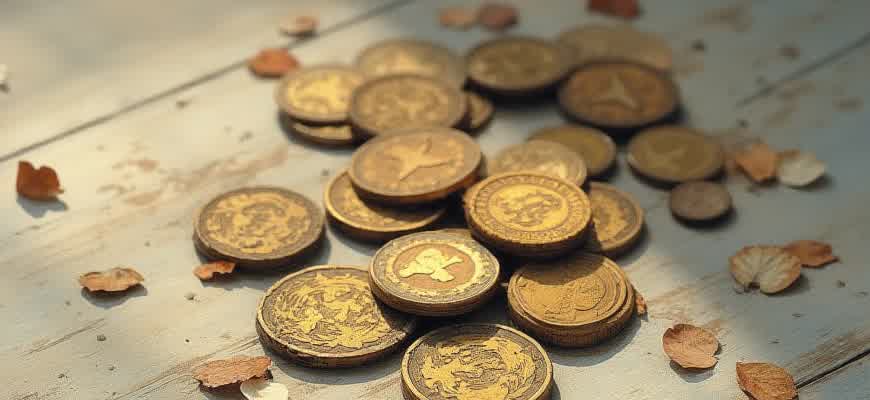
Google's Speech-to-Text API offers powerful functionality for converting spoken language into text. By utilizing this API, developers can easily add speech recognition capabilities to their React Native applications. This can enhance user experience in various scenarios, including voice commands, transcription, and accessibility features.
In this guide, we will explore how to integrate this service into a React Native app using available libraries and APIs. Here's a brief overview of the steps involved:
- Set up a Google Cloud account and enable the Speech-to-Text API.
- Install necessary libraries and dependencies in your React Native project.
- Configure the API client to handle audio input and manage the response.
- Handle the speech recognition results and implement real-time transcription.
Important: Make sure you have a valid Google Cloud API key and enable billing for the Speech-to-Text service before you proceed.
To begin, you will need to install the required package for integrating Google Speech Recognition. Here’s a basic example of the setup:
npm install react-native-google-speech-to-text
Next, ensure you have properly set up the permissions for accessing the device’s microphone, as this is essential for speech input.
Step | Action |
---|---|
1 | Enable the Speech-to-Text API in Google Cloud Console. |
2 | Install the React Native library for Google Speech API. |
3 | Set up authentication and configure API keys in your app. |
Google Speech-to-Text Integration in React Native: A Complete Guide
Integrating speech recognition into your React Native app allows users to interact with the app through voice commands. Google provides an efficient Speech-to-Text API, enabling high-quality speech recognition capabilities. With the right tools and setup, React Native developers can easily add this feature, improving user experience and accessibility.
In this guide, we will walk through the steps required to integrate Google's Speech-to-Text API into a React Native project. You'll learn how to set up the environment, configure the API, and implement key features for real-time transcription.
Setting Up the Google Speech-to-Text API
To use Google’s Speech-to-Text API in React Native, follow these steps:
- Set up a Google Cloud account and create a new project in the Google Cloud Console.
- Enable the Speech-to-Text API for the project.
- Download the credentials (API key or JSON file) and store them securely in your project.
- Install required dependencies in your React Native project, such as the react-native-google-apis package.
- Ensure that necessary permissions (microphone access) are added in your app's configuration files.
Implementing Speech Recognition in Your App
Once the API is set up, implement speech recognition functionality within your app. The key steps are:
- Initialize the Speech-to-Text API client.
- Start the microphone for audio input.
- Configure the transcription options (language, encoding, etc.).
- Process the audio input and receive the transcribed text in real-time.
- Handle errors and edge cases (e.g., no speech detected, API limits exceeded).
Important: Make sure to handle permissions carefully, especially for Android and iOS, to avoid crashes or unresponsive behavior.
Sample Code
Here’s a simplified example of implementing speech recognition in React Native:
import SpeechRecognition from 'react-native-google-speech-to-text'; const startListening = async () => { try { const result = await SpeechRecognition.startListening({ language: 'en-US', encoding: 'LINEAR16', sampleRateHertz: 16000 }); console.log('Transcribed Text: ', result); } catch (error) { console.error('Error during speech recognition: ', error); } };
Best Practices
- Test your app thoroughly with different speech patterns and accents to ensure optimal accuracy.
- Keep track of API usage to avoid hitting limits, especially if your app processes large amounts of audio data.
- Use background noise filters to improve transcription accuracy in real-world environments.
Conclusion
Integrating Google’s Speech-to-Text API into a React Native app opens up numerous possibilities for voice-driven user interfaces. By following the steps outlined in this guide and keeping best practices in mind, developers can create seamless voice interaction experiences that are both accurate and efficient.
Integrating Google Speech to Text API with React Native Projects
When working with React Native applications, integrating voice recognition features can enhance user experience significantly. Google’s Speech-to-Text API offers robust speech recognition capabilities that can be seamlessly added to mobile applications. With the rise of voice-driven interactions, it’s important to understand how to implement this API effectively within a React Native project.
To begin with, you will need to set up the Google Cloud Speech-to-Text API and configure your React Native project to work with it. This involves setting up API keys, installing dependencies, and ensuring that your application has the necessary permissions for microphone access. Let’s explore the basic steps involved in this integration process.
Steps for Integration
- Set up a Google Cloud account and enable the Speech-to-Text API.
- Create and configure API credentials (API key or service account).
- Install necessary packages in your React Native project, such as react-native-google-speech-to-text.
- Link the API to your project, ensuring that both iOS and Android platforms are supported.
- Configure permissions to allow microphone access on both platforms.
- Test the integration to ensure speech recognition works as expected.
Key Considerations
Ensure you handle both network errors and permission issues gracefully within your app.
There are a few important things to keep in mind while integrating speech recognition:
- Microphone Permissions: Always ask for microphone permissions before attempting to start the speech recognition.
- API Quotas: Google Cloud services may have usage limits, so it's essential to monitor your API consumption to avoid unexpected costs.
- Real-time Transcription: If your app requires real-time transcription, be mindful of network latency and adjust for optimal performance.
Common Issues and Solutions
Issue | Solution |
---|---|
Microphone access denied | Check app permissions in settings and request microphone access programmatically. |
Incorrect API key | Ensure your API key is valid and properly linked to your Google Cloud project. |
Speech recognition not working | Verify that the internet connection is stable and that the correct language model is selected. |
Setting Up API Credentials and Configuring Permissions in React Native
Before integrating Google Speech to Text into your React Native project, it's necessary to properly set up API credentials in the Google Cloud Console. Additionally, configuring the correct permissions ensures the app has access to required resources like the microphone and internet connection.
In the following steps, you will generate API credentials and configure permissions for a seamless integration with Google Speech-to-Text service in your mobile application.
Generating API Credentials
To interact with Google Cloud Speech-to-Text API, you must first enable the API and generate the credentials required for authentication.
- Navigate to the Google Cloud Console.
- Create a new project or select an existing one.
- Enable the Speech-to-Text API under the API Library section.
- Go to the Credentials tab and create a new API key or service account key.
- Download the credentials file in JSON format and keep it in a secure location.
Configuring Permissions
After generating the API credentials, you will need to configure the permissions for your React Native application to ensure proper functionality.
Important: Ensure that your app requests permissions to access the microphone for recording audio, which is essential for the speech-to-text functionality.
- For Android, modify the
AndroidManifest.xml
file to include microphone permissions. - For iOS, add the
NSMicrophoneUsageDescription
to theInfo.plist
to request permission to use the microphone.
API Integration in React Native
Once your credentials and permissions are configured, you can proceed with integrating the Speech-to-Text functionality into your React Native project using the generated API key or service account credentials.
Platform | Permission Required |
---|---|
Android | android.permission.RECORD_AUDIO |
iOS | NSMicrophoneUsageDescription |
Implementing Real-time Speech Recognition in React Native Apps
Real-time speech recognition offers a powerful feature for mobile applications, allowing users to interact through voice input. With the Google Speech-to-Text API, React Native developers can leverage its real-time capabilities to build apps that understand spoken language instantly. Integrating this technology requires setting up the correct environment and using the appropriate libraries to access the Google API in a seamless manner.
In order to enable real-time speech recognition, you must first ensure that the necessary packages and permissions are in place. The key steps involve configuring your React Native app to communicate with the Google Cloud services and handle audio streams effectively. This setup includes enabling the Speech-to-Text API on Google Cloud, installing required dependencies, and implementing listeners that process voice data as it’s captured.
Steps for Integration
- Enable Google Cloud Speech-to-Text API in your Google Cloud Console.
- Install the required packages in React Native, such as
react-native-speech-to-text
or similar libraries. - Request microphone permissions from the user to access voice input.
- Set up a listener for real-time audio capture.
- Integrate the Google API for voice recognition to convert audio into text on the fly.
Handling Real-time Data
Real-time speech-to-text requires handling streaming audio data. This can be done by constantly feeding audio input to the API for continuous processing. Below is a general approach:
- Start the microphone capture process.
- Stream audio data to the Google API for real-time recognition.
- Display the recognized text as the user speaks.
- Implement error handling to manage connectivity or recognition issues.
Tip: Be aware of network latency and microphone access delays that may impact real-time recognition accuracy.
Best Practices
Optimizing speech recognition involves several key practices, including:
- Using noise-cancellation techniques to improve accuracy in noisy environments.
- Ensuring a stable internet connection to minimize delays during speech processing.
- Implementing fallback mechanisms for offline scenarios, if applicable.
Example Code for Integration
Step | Action |
---|---|
1 | Enable Google Speech-to-Text API |
2 | Install React Native dependencies |
3 | Request permissions for microphone |
4 | Set up speech recognition listener |
5 | Process audio stream and display text |
Handling Speech to Text Errors and Troubleshooting Common Issues
Integrating Google Speech-to-Text API into a React Native application can sometimes result in errors or issues that may disrupt functionality. These problems can stem from various factors such as network issues, incorrect API key usage, or even incorrect configuration of the speech recognition service. Understanding how to diagnose and resolve these problems is crucial for ensuring smooth operation.
Common issues that developers face include inaccurate transcriptions, recognition failures, or even complete silence from the API. Fortunately, most of these errors can be traced to specific causes, such as misconfigured settings, issues with permissions, or even incompatible device hardware. Below are the key areas to focus on while troubleshooting these problems.
Common Issues and Solutions
- Incorrect API Key: Ensure that the API key used is correctly set up in the Google Cloud console and matches the one used in your React Native app.
- Network Problems: The Speech-to-Text service requires an active internet connection. Check for network issues that might be causing failed requests.
- Permission Issues: Make sure that the necessary permissions for microphone access are granted within your app.
- Device Compatibility: Older devices or those with less processing power may struggle with real-time transcription.
Steps for Troubleshooting
- Verify API Key: Double-check that the API key is valid and linked to the correct project in Google Cloud.
- Test Network Connectivity: Run a network speed test to ensure the app has sufficient bandwidth for real-time data transmission.
- Check Permissions: Review the app's permissions to ensure that microphone access is properly granted in both the app and device settings.
- Check Logs: Use the debugging tools available in React Native to check for specific errors related to the API or device.
Important: Always ensure that your API usage adheres to Google's rate limits and quotas. Exceeding these can result in failed requests or degraded performance.
Additional Troubleshooting Tips
Sometimes, even after fixing the obvious issues, the Speech-to-Text API might still perform suboptimally. In these cases, try the following:
- Test with Different Audio Samples: Low-quality or noisy audio may affect recognition. Test with high-quality, clear recordings.
- Optimize Recording Settings: Ensure your audio input settings are optimized for clear voice capture.
- Check for Rate Limit Exceedance: If you have high traffic, make sure you're not exceeding the API's rate limit or quota. If needed, request an increase in quota from Google Cloud.
Issue | Possible Cause | Solution |
---|---|---|
API Key Error | Invalid or expired API key | Regenerate and configure the API key in the Google Cloud Console |
Failed Transcription | Low-quality audio input | Use a noise-reducing microphone or high-quality recording setup |
Permission Denied | Missing microphone access permission | Ensure microphone permissions are granted both in-app and on the device |
Improving Accuracy of Speech Recognition in React Native
Achieving high accuracy in speech recognition within React Native apps requires addressing several factors that impact the quality of transcriptions. One key element is the proper configuration and tuning of speech-to-text services, like Google’s API. Various settings, such as language preference, speech model, and noise sensitivity, can directly influence recognition accuracy.
Besides choosing the right API and adjusting its settings, developers must also consider the interaction between speech recognition and the app’s UI/UX design. Ensuring that the microphone captures clear and consistent audio, optimizing background noise filters, and providing users with visual feedback can significantly reduce errors in transcription.
Key Strategies for Better Speech Recognition
- Choose the correct language model: Select the most appropriate speech model based on the user’s region and language. Google’s API supports various dialects, and using the correct model can improve transcription accuracy.
- Handle noise effectively: Utilize noise suppression algorithms to filter out unwanted sounds that could interfere with speech clarity.
- Implement real-time feedback: Provide immediate feedback to users while they speak, such as visual cues or prompts to rephrase unclear speech.
- Fine-tune with custom vocabulary: If the app involves industry-specific terms or unique phrases, include a custom vocabulary to help the speech recognition engine understand niche language.
Common Configuration Adjustments
- Enable automatic punctuation: This ensures that transcriptions are not only accurate but also readable by adding punctuation marks where necessary.
- Set appropriate audio sample rates: Choose an optimal audio quality setting that ensures clarity without overburdening the processing capabilities of the device.
- Adjust timeouts and session durations: Fine-tune the recognition session duration to handle longer speech inputs without cutting off prematurely.
Tip: Using higher sample rates and cleaner audio will result in better recognition, especially in noisy environments. Ensure that the device microphone is working optimally.
Table of Configuration Adjustments
Setting | Effect on Accuracy |
---|---|
Language Model | Choosing the correct dialect improves contextual recognition. |
Noise Reduction | Minimizes environmental noise interference, leading to cleaner input. |
Custom Vocabulary | Improves understanding of specific jargon, reducing recognition errors. |
Testing Google Speech-to-Text Integration in React Native Applications
When integrating Google's Speech-to-Text API into a React Native app, thorough testing is crucial to ensure seamless functionality. Since speech recognition involves multiple factors, such as microphone access, language models, and connectivity, it’s essential to validate each part of the integration independently. This can help prevent issues such as inaccurate transcriptions, delays in response, or crashes during usage. Testing should be structured, focusing on both technical performance and user experience.
To achieve efficient testing of the API in your React Native app, several methods can be employed. These include manual testing, unit tests, and performance monitoring. It's vital to ensure that all edge cases are covered, such as different accents, background noise, and intermittent network issues. In this article, we will cover the testing methods and provide an outline for testing the integration of Google's speech recognition service in a React Native environment.
Methods for Testing
- Unit Testing: Ensure that components interacting with the API are functioning as expected.
- Manual Testing: Simulate real-life use cases to validate transcription accuracy, including diverse speech patterns.
- End-to-End Testing: Verify that the app performs correctly when connected to the internet and interacts with the Google API in a production environment.
Test Scenarios
- Ensure microphone permissions are handled correctly.
- Test speech-to-text conversion accuracy under various environmental conditions.
- Check API error handling, such as what happens when the network is lost.
- Verify response times, ensuring minimal delay in converting speech to text.
Key Test Considerations
Always test with multiple accents, speech rates, and background noise to ensure robust performance. Additionally, test various device types, as performance may vary between iOS and Android devices.
Testing Tools
Tool | Purpose |
---|---|
Jest | Unit testing for React Native components |
Detox | End-to-end testing for React Native applications |
Mock Service Worker (MSW) | Mock API requests for offline testing |
Managing Audio Data and Ensuring Smooth Integration with React Native
When working with audio data in React Native, managing the recording, processing, and transmission of audio becomes crucial for seamless functionality. The complexity increases when integrating with third-party services like speech recognition, which requires handling real-time audio streams efficiently. The main goal is to ensure that the application can process audio input without lag or performance drops, providing a smooth user experience.
Integrating such functionalities typically requires interaction with native modules to handle low-level tasks. React Native’s bridging mechanism allows for communication between JavaScript and native code, which is necessary to work with Android or iOS-specific audio APIs for optimal performance. This setup is particularly important when implementing services such as speech-to-text that rely on precise audio capture.
Audio Management Workflow
- Recording: Start by capturing audio using native recording libraries or modules like react-native-audio or react-native-sound. These tools offer interfaces for managing microphone input.
- Processing: Once the audio is recorded, it's often necessary to pre-process it for noise reduction, quality enhancement, or conversion to formats suitable for recognition engines.
- Sending to API: After processing, the audio is sent to the API for transcription. The key here is optimizing data transmission to avoid delays during recognition.
Ensuring Smooth Integration
- Use a real-time audio stream rather than processing audio in chunks to avoid latency.
- Implement error handling for cases where audio cannot be captured or the API fails to process the input correctly.
- Optimize performance by offloading heavy tasks to native code where necessary, especially for audio encoding and transmission.
When working with audio in React Native, it's essential to ensure that the integration remains efficient across both platforms (iOS and Android). This includes managing dependencies and choosing the right libraries for native audio capture and handling streaming data.
Performance Considerations
Task | Consideration |
---|---|
Audio Recording | Ensure low-latency audio capture using native modules. |
Real-time Processing | Use efficient audio buffers and minimize the use of JavaScript for heavy audio processing tasks. |
Data Transmission | Use compressed formats to reduce bandwidth usage and improve transmission speed. |